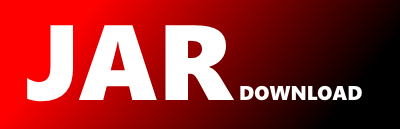
com.microsoft.azure.eventhubs.EventDataUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-eventhubs Show documentation
Show all versions of azure-eventhubs Show documentation
Client library for talking to Microsoft Azure Event Hubs.
/*
* Copyright (c) Microsoft. All rights reserved.
* Licensed under the MIT license. See LICENSE file in the project root for full license information.
*/
package com.microsoft.azure.eventhubs;
import java.util.*;
import java.util.function.*;
import org.apache.qpid.proton.message.*;
/*
* Internal utility class for EventData
*/
final class EventDataUtil
{
private EventDataUtil(){}
static LinkedList toEventDataCollection(final Collection messages)
{
if (messages == null)
{
return null;
}
// TODO: no-copy solution
LinkedList events = new LinkedList();
for(Message message : messages)
{
events.add(new EventData(message));
}
return events;
}
static Iterable toAmqpMessages(final Iterable eventDatas, final String partitionKey)
{
final LinkedList messages = new LinkedList();
eventDatas.forEach(new Consumer()
{
@Override
public void accept(EventData eventData)
{
Message amqpMessage = partitionKey == null ? eventData.toAmqpMessage() : eventData.toAmqpMessage(partitionKey);
messages.add(amqpMessage);
}
});
return messages;
}
static Iterable toAmqpMessages(final Iterable eventDatas)
{
return EventDataUtil.toAmqpMessages(eventDatas, null);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy