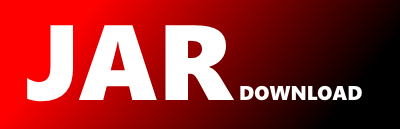
com.microsoft.azure.servicebus.IteratorUtil Maven / Gradle / Ivy
/*
* Copyright (c) Microsoft. All rights reserved.
* Licensed under the MIT license. See LICENSE file in the project root for full license information.
*/
package com.microsoft.azure.servicebus;
import java.util.Iterator;
public final class IteratorUtil
{
private IteratorUtil()
{
}
public static boolean sizeEquals(Iterable iterable, int expectedSize)
{
Iterator iterator = iterable.iterator();
int currentSize = 0;
while(iterator.hasNext())
{
if (expectedSize > currentSize)
{
currentSize++;
iterator.next();
continue;
}
else
{
return false;
}
}
return true;
}
public static T getLast(Iterator iterator)
{
T last = null;
while(iterator.hasNext())
{
last = iterator.next();
}
return last;
}
public static T getFirst(final Iterable iterable)
{
if (iterable == null)
{
return null;
}
final Iterator iterator = iterable.iterator();
if (iterator == null)
{
return null;
}
return iterator.hasNext() ? iterator.next() : null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy