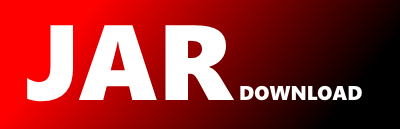
com.microsoft.azure.keyvault.implementation.KeyVaultClientBaseImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-keyvault Show documentation
Show all versions of azure-keyvault Show documentation
This package contains Microsoft Azure Key Vault SDK.
/**
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for
* license information.
*
* Code generated by Microsoft (R) AutoRest Code Generator.
*/
package com.microsoft.azure.keyvault.implementation;
import com.google.common.base.Joiner;
import com.google.common.reflect.TypeToken;
import com.microsoft.azure.AzureClient;
import com.microsoft.azure.AzureServiceClient;
import com.microsoft.azure.AzureServiceFuture;
import com.microsoft.azure.keyvault.KeyVaultClientBase;
import com.microsoft.azure.keyvault.models.BackupKeyResult;
import com.microsoft.azure.keyvault.models.BackupSecretResult;
import com.microsoft.azure.keyvault.models.BackupStorageResult;
import com.microsoft.azure.keyvault.models.CertificateAttributes;
import com.microsoft.azure.keyvault.models.CertificateBundle;
import com.microsoft.azure.keyvault.models.CertificateCreateParameters;
import com.microsoft.azure.keyvault.models.CertificateImportParameters;
import com.microsoft.azure.keyvault.models.CertificateIssuerItem;
import com.microsoft.azure.keyvault.models.CertificateIssuerSetParameters;
import com.microsoft.azure.keyvault.models.CertificateIssuerUpdateParameters;
import com.microsoft.azure.keyvault.models.CertificateItem;
import com.microsoft.azure.keyvault.models.CertificateMergeParameters;
import com.microsoft.azure.keyvault.models.CertificateOperation;
import com.microsoft.azure.keyvault.models.CertificateOperationUpdateParameter;
import com.microsoft.azure.keyvault.models.CertificatePolicy;
import com.microsoft.azure.keyvault.models.CertificateUpdateParameters;
import com.microsoft.azure.keyvault.models.Contacts;
import com.microsoft.azure.keyvault.models.DeletedCertificateBundle;
import com.microsoft.azure.keyvault.models.DeletedCertificateItem;
import com.microsoft.azure.keyvault.models.DeletedKeyBundle;
import com.microsoft.azure.keyvault.models.DeletedKeyItem;
import com.microsoft.azure.keyvault.models.DeletedSasDefinitionBundle;
import com.microsoft.azure.keyvault.models.DeletedSasDefinitionItem;
import com.microsoft.azure.keyvault.models.DeletedSecretBundle;
import com.microsoft.azure.keyvault.models.DeletedSecretItem;
import com.microsoft.azure.keyvault.models.DeletedStorageAccountItem;
import com.microsoft.azure.keyvault.models.DeletedStorageBundle;
import com.microsoft.azure.keyvault.models.IssuerAttributes;
import com.microsoft.azure.keyvault.models.IssuerBundle;
import com.microsoft.azure.keyvault.models.IssuerCredentials;
import com.microsoft.azure.keyvault.models.JsonWebKeyCurveName;
import com.microsoft.azure.keyvault.models.KeyAttributes;
import com.microsoft.azure.keyvault.models.KeyBundle;
import com.microsoft.azure.keyvault.models.KeyCreateParameters;
import com.microsoft.azure.keyvault.models.KeyImportParameters;
import com.microsoft.azure.keyvault.models.KeyItem;
import com.microsoft.azure.keyvault.models.KeyOperationResult;
import com.microsoft.azure.keyvault.models.KeyOperationsParameters;
import com.microsoft.azure.keyvault.models.KeyRestoreParameters;
import com.microsoft.azure.keyvault.models.KeySignParameters;
import com.microsoft.azure.keyvault.models.KeyUpdateParameters;
import com.microsoft.azure.keyvault.models.KeyVaultErrorException;
import com.microsoft.azure.keyvault.models.KeyVerifyParameters;
import com.microsoft.azure.keyvault.models.KeyVerifyResult;
import com.microsoft.azure.keyvault.models.OrganizationDetails;
import com.microsoft.azure.keyvault.models.PageImpl;
import com.microsoft.azure.keyvault.models.SasDefinitionAttributes;
import com.microsoft.azure.keyvault.models.SasDefinitionBundle;
import com.microsoft.azure.keyvault.models.SasDefinitionCreateParameters;
import com.microsoft.azure.keyvault.models.SasDefinitionItem;
import com.microsoft.azure.keyvault.models.SasDefinitionUpdateParameters;
import com.microsoft.azure.keyvault.models.SasTokenType;
import com.microsoft.azure.keyvault.models.SecretAttributes;
import com.microsoft.azure.keyvault.models.SecretBundle;
import com.microsoft.azure.keyvault.models.SecretItem;
import com.microsoft.azure.keyvault.models.SecretRestoreParameters;
import com.microsoft.azure.keyvault.models.SecretSetParameters;
import com.microsoft.azure.keyvault.models.SecretUpdateParameters;
import com.microsoft.azure.keyvault.models.StorageAccountAttributes;
import com.microsoft.azure.keyvault.models.StorageAccountCreateParameters;
import com.microsoft.azure.keyvault.models.StorageAccountItem;
import com.microsoft.azure.keyvault.models.StorageAccountRegenerteKeyParameters;
import com.microsoft.azure.keyvault.models.StorageAccountUpdateParameters;
import com.microsoft.azure.keyvault.models.StorageBundle;
import com.microsoft.azure.keyvault.models.StorageRestoreParameters;
import com.microsoft.azure.ListOperationCallback;
import com.microsoft.azure.Page;
import com.microsoft.azure.PagedList;
import com.microsoft.rest.credentials.ServiceClientCredentials;
import com.microsoft.rest.RestClient;
import com.microsoft.rest.ServiceCallback;
import com.microsoft.rest.ServiceFuture;
import com.microsoft.rest.ServiceResponse;
import com.microsoft.rest.Validator;
import java.io.IOException;
import java.util.List;
import java.util.Map;
import okhttp3.ResponseBody;
import retrofit2.http.Body;
import retrofit2.http.GET;
import retrofit2.http.Header;
import retrofit2.http.Headers;
import retrofit2.http.HTTP;
import retrofit2.http.PATCH;
import retrofit2.http.Path;
import retrofit2.http.POST;
import retrofit2.http.PUT;
import retrofit2.http.Query;
import retrofit2.http.Url;
import retrofit2.Response;
import rx.functions.Func1;
import rx.Observable;
import com.microsoft.azure.keyvault.webkey.JsonWebKey;
import com.microsoft.azure.keyvault.webkey.JsonWebKeyEncryptionAlgorithm;
import com.microsoft.azure.keyvault.webkey.JsonWebKeyOperation;
import com.microsoft.azure.keyvault.webkey.JsonWebKeySignatureAlgorithm;
import com.microsoft.azure.keyvault.webkey.JsonWebKeyType;
/**
* Initializes a new instance of the KeyVaultClientBaseImpl class.
*/
public class KeyVaultClientBaseImpl extends AzureServiceClient implements KeyVaultClientBase {
/** The Retrofit service to perform REST calls. */
private KeyVaultClientBaseService service;
/** the {@link AzureClient} used for long running operations. */
private AzureClient azureClient;
/**
* Gets the {@link AzureClient} used for long running operations.
* @return the azure client;
*/
public AzureClient getAzureClient() {
return this.azureClient;
}
/** Client API version. */
private String apiVersion;
/**
* Gets Client API version.
*
* @return the apiVersion value.
*/
public String apiVersion() {
return this.apiVersion;
}
/** Gets or sets the preferred language for the response. */
private String acceptLanguage;
/**
* Gets Gets or sets the preferred language for the response.
*
* @return the acceptLanguage value.
*/
public String acceptLanguage() {
return this.acceptLanguage;
}
/**
* Sets Gets or sets the preferred language for the response.
*
* @param acceptLanguage the acceptLanguage value.
* @return the service client itself
*/
public KeyVaultClientBaseImpl withAcceptLanguage(String acceptLanguage) {
this.acceptLanguage = acceptLanguage;
return this;
}
/** Gets or sets the retry timeout in seconds for Long Running Operations. Default value is 30. */
private int longRunningOperationRetryTimeout;
/**
* Gets Gets or sets the retry timeout in seconds for Long Running Operations. Default value is 30.
*
* @return the longRunningOperationRetryTimeout value.
*/
public int longRunningOperationRetryTimeout() {
return this.longRunningOperationRetryTimeout;
}
/**
* Sets Gets or sets the retry timeout in seconds for Long Running Operations. Default value is 30.
*
* @param longRunningOperationRetryTimeout the longRunningOperationRetryTimeout value.
* @return the service client itself
*/
public KeyVaultClientBaseImpl withLongRunningOperationRetryTimeout(int longRunningOperationRetryTimeout) {
this.longRunningOperationRetryTimeout = longRunningOperationRetryTimeout;
return this;
}
/** When set to true a unique x-ms-client-request-id value is generated and included in each request. Default is true. */
private boolean generateClientRequestId;
/**
* Gets When set to true a unique x-ms-client-request-id value is generated and included in each request. Default is true.
*
* @return the generateClientRequestId value.
*/
public boolean generateClientRequestId() {
return this.generateClientRequestId;
}
/**
* Sets When set to true a unique x-ms-client-request-id value is generated and included in each request. Default is true.
*
* @param generateClientRequestId the generateClientRequestId value.
* @return the service client itself
*/
public KeyVaultClientBaseImpl withGenerateClientRequestId(boolean generateClientRequestId) {
this.generateClientRequestId = generateClientRequestId;
return this;
}
/**
* Initializes an instance of KeyVaultClientBase client.
*
* @param credentials the management credentials for Azure
*/
public KeyVaultClientBaseImpl(ServiceClientCredentials credentials) {
this("https://{vaultBaseUrl}", credentials);
}
/**
* Initializes an instance of KeyVaultClientBase client.
*
* @param baseUrl the base URL of the host
* @param credentials the management credentials for Azure
*/
private KeyVaultClientBaseImpl(String baseUrl, ServiceClientCredentials credentials) {
super(baseUrl, credentials);
initialize();
}
/**
* Initializes an instance of KeyVaultClientBase client.
*
* @param restClient the REST client to connect to Azure.
*/
public KeyVaultClientBaseImpl(RestClient restClient) {
super(restClient);
initialize();
}
protected void initialize() {
this.apiVersion = "7.0-preview";
this.acceptLanguage = "en-US";
this.longRunningOperationRetryTimeout = 30;
this.generateClientRequestId = true;
this.azureClient = new AzureClient(this);
initializeService();
}
/**
* Gets the User-Agent header for the client.
*
* @return the user agent string.
*/
@Override
public String userAgent() {
return String.format("%s (%s, %s)", super.userAgent(), "KeyVaultClientBase", "7.0-preview");
}
private void initializeService() {
service = restClient().retrofit().create(KeyVaultClientBaseService.class);
}
/**
* The interface defining all the services for KeyVaultClientBase to be
* used by Retrofit to perform actually REST calls.
*/
interface KeyVaultClientBaseService {
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase createKey" })
@POST("keys/{key-name}/create")
Observable> createKey(@Path("key-name") String keyName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Body KeyCreateParameters parameters, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase importKey" })
@PUT("keys/{key-name}")
Observable> importKey(@Path("key-name") String keyName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Body KeyImportParameters parameters, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase deleteKey" })
@HTTP(path = "keys/{key-name}", method = "DELETE", hasBody = true)
Observable> deleteKey(@Path("key-name") String keyName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase updateKey" })
@PATCH("keys/{key-name}/{key-version}")
Observable> updateKey(@Path("key-name") String keyName, @Path("key-version") String keyVersion, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Body KeyUpdateParameters parameters, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase getKey" })
@GET("keys/{key-name}/{key-version}")
Observable> getKey(@Path("key-name") String keyName, @Path("key-version") String keyVersion, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase getKeyVersions" })
@GET("keys/{key-name}/versions")
Observable> getKeyVersions(@Path("key-name") String keyName, @Query("maxresults") Integer maxresults, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase getKeys" })
@GET("keys")
Observable> getKeys(@Query("maxresults") Integer maxresults, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase backupKey" })
@POST("keys/{key-name}/backup")
Observable> backupKey(@Path("key-name") String keyName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase restoreKey" })
@POST("keys/restore")
Observable> restoreKey(@Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Body KeyRestoreParameters parameters, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase encrypt" })
@POST("keys/{key-name}/{key-version}/encrypt")
Observable> encrypt(@Path("key-name") String keyName, @Path("key-version") String keyVersion, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Body KeyOperationsParameters parameters, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase decrypt" })
@POST("keys/{key-name}/{key-version}/decrypt")
Observable> decrypt(@Path("key-name") String keyName, @Path("key-version") String keyVersion, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Body KeyOperationsParameters parameters, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase sign" })
@POST("keys/{key-name}/{key-version}/sign")
Observable> sign(@Path("key-name") String keyName, @Path("key-version") String keyVersion, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Body KeySignParameters parameters, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase verify" })
@POST("keys/{key-name}/{key-version}/verify")
Observable> verify(@Path("key-name") String keyName, @Path("key-version") String keyVersion, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Body KeyVerifyParameters parameters, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase wrapKey" })
@POST("keys/{key-name}/{key-version}/wrapkey")
Observable> wrapKey(@Path("key-name") String keyName, @Path("key-version") String keyVersion, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Body KeyOperationsParameters parameters, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase unwrapKey" })
@POST("keys/{key-name}/{key-version}/unwrapkey")
Observable> unwrapKey(@Path("key-name") String keyName, @Path("key-version") String keyVersion, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Body KeyOperationsParameters parameters, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase getDeletedKeys" })
@GET("deletedkeys")
Observable> getDeletedKeys(@Query("maxresults") Integer maxresults, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase getDeletedKey" })
@GET("deletedkeys/{key-name}")
Observable> getDeletedKey(@Path("key-name") String keyName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase purgeDeletedKey" })
@HTTP(path = "deletedkeys/{key-name}", method = "DELETE", hasBody = true)
Observable> purgeDeletedKey(@Path("key-name") String keyName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase recoverDeletedKey" })
@POST("deletedkeys/{key-name}/recover")
Observable> recoverDeletedKey(@Path("key-name") String keyName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase setSecret" })
@PUT("secrets/{secret-name}")
Observable> setSecret(@Path("secret-name") String secretName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Body SecretSetParameters parameters, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase deleteSecret" })
@HTTP(path = "secrets/{secret-name}", method = "DELETE", hasBody = true)
Observable> deleteSecret(@Path("secret-name") String secretName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase updateSecret" })
@PATCH("secrets/{secret-name}/{secret-version}")
Observable> updateSecret(@Path("secret-name") String secretName, @Path("secret-version") String secretVersion, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Body SecretUpdateParameters parameters, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase getSecret" })
@GET("secrets/{secret-name}/{secret-version}")
Observable> getSecret(@Path("secret-name") String secretName, @Path("secret-version") String secretVersion, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase getSecrets" })
@GET("secrets")
Observable> getSecrets(@Query("maxresults") Integer maxresults, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase getSecretVersions" })
@GET("secrets/{secret-name}/versions")
Observable> getSecretVersions(@Path("secret-name") String secretName, @Query("maxresults") Integer maxresults, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase getDeletedSecrets" })
@GET("deletedsecrets")
Observable> getDeletedSecrets(@Query("maxresults") Integer maxresults, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase getDeletedSecret" })
@GET("deletedsecrets/{secret-name}")
Observable> getDeletedSecret(@Path("secret-name") String secretName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase purgeDeletedSecret" })
@HTTP(path = "deletedsecrets/{secret-name}", method = "DELETE", hasBody = true)
Observable> purgeDeletedSecret(@Path("secret-name") String secretName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase recoverDeletedSecret" })
@POST("deletedsecrets/{secret-name}/recover")
Observable> recoverDeletedSecret(@Path("secret-name") String secretName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase backupSecret" })
@POST("secrets/{secret-name}/backup")
Observable> backupSecret(@Path("secret-name") String secretName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase restoreSecret" })
@POST("secrets/restore")
Observable> restoreSecret(@Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Body SecretRestoreParameters parameters, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase getCertificates" })
@GET("certificates")
Observable> getCertificates(@Query("maxresults") Integer maxresults, @Query("includePending") Boolean includePending, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase deleteCertificate" })
@HTTP(path = "certificates/{certificate-name}", method = "DELETE", hasBody = true)
Observable> deleteCertificate(@Path("certificate-name") String certificateName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase setCertificateContacts" })
@PUT("certificates/contacts")
Observable> setCertificateContacts(@Body Contacts contacts, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase getCertificateContacts" })
@GET("certificates/contacts")
Observable> getCertificateContacts(@Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase deleteCertificateContacts" })
@HTTP(path = "certificates/contacts", method = "DELETE", hasBody = true)
Observable> deleteCertificateContacts(@Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase getCertificateIssuers" })
@GET("certificates/issuers")
Observable> getCertificateIssuers(@Query("maxresults") Integer maxresults, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase setCertificateIssuer" })
@PUT("certificates/issuers/{issuer-name}")
Observable> setCertificateIssuer(@Path("issuer-name") String issuerName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Body CertificateIssuerSetParameters parameter, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase updateCertificateIssuer" })
@PATCH("certificates/issuers/{issuer-name}")
Observable> updateCertificateIssuer(@Path("issuer-name") String issuerName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Body CertificateIssuerUpdateParameters parameter, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase getCertificateIssuer" })
@GET("certificates/issuers/{issuer-name}")
Observable> getCertificateIssuer(@Path("issuer-name") String issuerName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase deleteCertificateIssuer" })
@HTTP(path = "certificates/issuers/{issuer-name}", method = "DELETE", hasBody = true)
Observable> deleteCertificateIssuer(@Path("issuer-name") String issuerName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase createCertificate" })
@POST("certificates/{certificate-name}/create")
Observable> createCertificate(@Path("certificate-name") String certificateName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Body CertificateCreateParameters parameters, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase importCertificate" })
@POST("certificates/{certificate-name}/import")
Observable> importCertificate(@Path("certificate-name") String certificateName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Body CertificateImportParameters parameters, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase getCertificateVersions" })
@GET("certificates/{certificate-name}/versions")
Observable> getCertificateVersions(@Path("certificate-name") String certificateName, @Query("maxresults") Integer maxresults, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase getCertificatePolicy" })
@GET("certificates/{certificate-name}/policy")
Observable> getCertificatePolicy(@Path("certificate-name") String certificateName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase updateCertificatePolicy" })
@PATCH("certificates/{certificate-name}/policy")
Observable> updateCertificatePolicy(@Path("certificate-name") String certificateName, @Body CertificatePolicy certificatePolicy, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase updateCertificate" })
@PATCH("certificates/{certificate-name}/{certificate-version}")
Observable> updateCertificate(@Path("certificate-name") String certificateName, @Path("certificate-version") String certificateVersion, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Body CertificateUpdateParameters parameters, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase getCertificate" })
@GET("certificates/{certificate-name}/{certificate-version}")
Observable> getCertificate(@Path("certificate-name") String certificateName, @Path("certificate-version") String certificateVersion, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase updateCertificateOperation" })
@PATCH("certificates/{certificate-name}/pending")
Observable> updateCertificateOperation(@Path("certificate-name") String certificateName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Body CertificateOperationUpdateParameter certificateOperation, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase getCertificateOperation" })
@GET("certificates/{certificate-name}/pending")
Observable> getCertificateOperation(@Path("certificate-name") String certificateName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase deleteCertificateOperation" })
@HTTP(path = "certificates/{certificate-name}/pending", method = "DELETE", hasBody = true)
Observable> deleteCertificateOperation(@Path("certificate-name") String certificateName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase mergeCertificate" })
@POST("certificates/{certificate-name}/pending/merge")
Observable> mergeCertificate(@Path("certificate-name") String certificateName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Body CertificateMergeParameters parameters, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase getDeletedCertificates" })
@GET("deletedcertificates")
Observable> getDeletedCertificates(@Query("maxresults") Integer maxresults, @Query("includePending") Boolean includePending, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase getDeletedCertificate" })
@GET("deletedcertificates/{certificate-name}")
Observable> getDeletedCertificate(@Path("certificate-name") String certificateName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase purgeDeletedCertificate" })
@HTTP(path = "deletedcertificates/{certificate-name}", method = "DELETE", hasBody = true)
Observable> purgeDeletedCertificate(@Path("certificate-name") String certificateName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase recoverDeletedCertificate" })
@POST("deletedcertificates/{certificate-name}/recover")
Observable> recoverDeletedCertificate(@Path("certificate-name") String certificateName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase getStorageAccounts" })
@GET("storage")
Observable> getStorageAccounts(@Query("maxresults") Integer maxresults, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase getDeletedStorageAccounts" })
@GET("deletedstorage")
Observable> getDeletedStorageAccounts(@Query("maxresults") Integer maxresults, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase getDeletedStorageAccount" })
@GET("deletedstorage/{storage-account-name}")
Observable> getDeletedStorageAccount(@Path("storage-account-name") String storageAccountName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase purgeDeletedStorgeAccount" })
@HTTP(path = "deletedstorage/{storage-account-name}", method = "DELETE", hasBody = true)
Observable> purgeDeletedStorgeAccount(@Path("storage-account-name") String storageAccountName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase recoverDeletedStorageAccount" })
@POST("deletedstorage/{storage-account-name}/recover")
Observable> recoverDeletedStorageAccount(@Path("storage-account-name") String storageAccountName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase backupStorageAccount" })
@POST("storage/{storage-account-name}/backup")
Observable> backupStorageAccount(@Path("storage-account-name") String storageAccountName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase restoreStorageAccount" })
@POST("storage/restore")
Observable> restoreStorageAccount(@Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Body StorageRestoreParameters parameters, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase deleteStorageAccount" })
@HTTP(path = "storage/{storage-account-name}", method = "DELETE", hasBody = true)
Observable> deleteStorageAccount(@Path("storage-account-name") String storageAccountName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase getStorageAccount" })
@GET("storage/{storage-account-name}")
Observable> getStorageAccount(@Path("storage-account-name") String storageAccountName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase setStorageAccount" })
@PUT("storage/{storage-account-name}")
Observable> setStorageAccount(@Path("storage-account-name") String storageAccountName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Body StorageAccountCreateParameters parameters, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase updateStorageAccount" })
@PATCH("storage/{storage-account-name}")
Observable> updateStorageAccount(@Path("storage-account-name") String storageAccountName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Body StorageAccountUpdateParameters parameters, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase regenerateStorageAccountKey" })
@POST("storage/{storage-account-name}/regeneratekey")
Observable> regenerateStorageAccountKey(@Path("storage-account-name") String storageAccountName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Body StorageAccountRegenerteKeyParameters parameters, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase getSasDefinitions" })
@GET("storage/{storage-account-name}/sas")
Observable> getSasDefinitions(@Path("storage-account-name") String storageAccountName, @Query("maxresults") Integer maxresults, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase getDeletedSasDefinitions" })
@GET("deletedstorage/{storage-account-name}/sas")
Observable> getDeletedSasDefinitions(@Path("storage-account-name") String storageAccountName, @Query("maxresults") Integer maxresults, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase getDeletedSasDefinition" })
@GET("deletedstorage/{storage-account-name}/sas/{sas-definition-name}")
Observable> getDeletedSasDefinition(@Path("storage-account-name") String storageAccountName, @Path("sas-definition-name") String sasDefinitionName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase recoverDeletedSasDefinition" })
@POST("deletedstorage/{storage-account-name}/sas/{sas-definition-name}/recover")
Observable> recoverDeletedSasDefinition(@Path("storage-account-name") String storageAccountName, @Path("sas-definition-name") String sasDefinitionName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase deleteSasDefinition" })
@HTTP(path = "storage/{storage-account-name}/sas/{sas-definition-name}", method = "DELETE", hasBody = true)
Observable> deleteSasDefinition(@Path("storage-account-name") String storageAccountName, @Path("sas-definition-name") String sasDefinitionName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase getSasDefinition" })
@GET("storage/{storage-account-name}/sas/{sas-definition-name}")
Observable> getSasDefinition(@Path("storage-account-name") String storageAccountName, @Path("sas-definition-name") String sasDefinitionName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase setSasDefinition" })
@PUT("storage/{storage-account-name}/sas/{sas-definition-name}")
Observable> setSasDefinition(@Path("storage-account-name") String storageAccountName, @Path("sas-definition-name") String sasDefinitionName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Body SasDefinitionCreateParameters parameters, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase updateSasDefinition" })
@PATCH("storage/{storage-account-name}/sas/{sas-definition-name}")
Observable> updateSasDefinition(@Path("storage-account-name") String storageAccountName, @Path("sas-definition-name") String sasDefinitionName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Body SasDefinitionUpdateParameters parameters, @Header("x-ms-parameterized-host") String parameterizedHost, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase getKeyVersionsNext" })
@GET
Observable> getKeyVersionsNext(@Url String nextUrl, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase getKeysNext" })
@GET
Observable> getKeysNext(@Url String nextUrl, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase getDeletedKeysNext" })
@GET
Observable> getDeletedKeysNext(@Url String nextUrl, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase getSecretsNext" })
@GET
Observable> getSecretsNext(@Url String nextUrl, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase getSecretVersionsNext" })
@GET
Observable> getSecretVersionsNext(@Url String nextUrl, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase getDeletedSecretsNext" })
@GET
Observable> getDeletedSecretsNext(@Url String nextUrl, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase getCertificatesNext" })
@GET
Observable> getCertificatesNext(@Url String nextUrl, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase getCertificateIssuersNext" })
@GET
Observable> getCertificateIssuersNext(@Url String nextUrl, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase getCertificateVersionsNext" })
@GET
Observable> getCertificateVersionsNext(@Url String nextUrl, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase getDeletedCertificatesNext" })
@GET
Observable> getDeletedCertificatesNext(@Url String nextUrl, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase getStorageAccountsNext" })
@GET
Observable> getStorageAccountsNext(@Url String nextUrl, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase getDeletedStorageAccountsNext" })
@GET
Observable> getDeletedStorageAccountsNext(@Url String nextUrl, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase getSasDefinitionsNext" })
@GET
Observable> getSasDefinitionsNext(@Url String nextUrl, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.keyvault.KeyVaultClientBase getDeletedSasDefinitionsNext" })
@GET
Observable> getDeletedSasDefinitionsNext(@Url String nextUrl, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
}
/**
* Creates a new key, stores it, then returns key parameters and attributes to the client.
* The create key operation can be used to create any key type in Azure Key Vault. If the named key already exists, Azure Key Vault creates a new version of the key. It requires the keys/create permission.
*
* @param vaultBaseUrl The vault name, for example https://myvault.vault.azure.net.
* @param keyName The name for the new key. The system will generate the version name for the new key.
* @param kty The type of key to create. For valid values, see JsonWebKeyType. Possible values include: 'EC', 'EC-HSM', 'RSA', 'RSA-HSM', 'oct'
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws KeyVaultErrorException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the KeyBundle object if successful.
*/
public KeyBundle createKey(String vaultBaseUrl, String keyName, JsonWebKeyType kty) {
return createKeyWithServiceResponseAsync(vaultBaseUrl, keyName, kty).toBlocking().single().body();
}
/**
* Creates a new key, stores it, then returns key parameters and attributes to the client.
* The create key operation can be used to create any key type in Azure Key Vault. If the named key already exists, Azure Key Vault creates a new version of the key. It requires the keys/create permission.
*
* @param vaultBaseUrl The vault name, for example https://myvault.vault.azure.net.
* @param keyName The name for the new key. The system will generate the version name for the new key.
* @param kty The type of key to create. For valid values, see JsonWebKeyType. Possible values include: 'EC', 'EC-HSM', 'RSA', 'RSA-HSM', 'oct'
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture createKeyAsync(String vaultBaseUrl, String keyName, JsonWebKeyType kty, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(createKeyWithServiceResponseAsync(vaultBaseUrl, keyName, kty), serviceCallback);
}
/**
* Creates a new key, stores it, then returns key parameters and attributes to the client.
* The create key operation can be used to create any key type in Azure Key Vault. If the named key already exists, Azure Key Vault creates a new version of the key. It requires the keys/create permission.
*
* @param vaultBaseUrl The vault name, for example https://myvault.vault.azure.net.
* @param keyName The name for the new key. The system will generate the version name for the new key.
* @param kty The type of key to create. For valid values, see JsonWebKeyType. Possible values include: 'EC', 'EC-HSM', 'RSA', 'RSA-HSM', 'oct'
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the KeyBundle object
*/
public Observable createKeyAsync(String vaultBaseUrl, String keyName, JsonWebKeyType kty) {
return createKeyWithServiceResponseAsync(vaultBaseUrl, keyName, kty).map(new Func1, KeyBundle>() {
@Override
public KeyBundle call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Creates a new key, stores it, then returns key parameters and attributes to the client.
* The create key operation can be used to create any key type in Azure Key Vault. If the named key already exists, Azure Key Vault creates a new version of the key. It requires the keys/create permission.
*
* @param vaultBaseUrl The vault name, for example https://myvault.vault.azure.net.
* @param keyName The name for the new key. The system will generate the version name for the new key.
* @param kty The type of key to create. For valid values, see JsonWebKeyType. Possible values include: 'EC', 'EC-HSM', 'RSA', 'RSA-HSM', 'oct'
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the KeyBundle object
*/
public Observable> createKeyWithServiceResponseAsync(String vaultBaseUrl, String keyName, JsonWebKeyType kty) {
if (vaultBaseUrl == null) {
throw new IllegalArgumentException("Parameter vaultBaseUrl is required and cannot be null.");
}
if (keyName == null) {
throw new IllegalArgumentException("Parameter keyName is required and cannot be null.");
}
if (this.apiVersion() == null) {
throw new IllegalArgumentException("Parameter this.apiVersion() is required and cannot be null.");
}
if (kty == null) {
throw new IllegalArgumentException("Parameter kty is required and cannot be null.");
}
final Integer keySize = null;
final List keyOps = null;
final KeyAttributes keyAttributes = null;
final Map tags = null;
final JsonWebKeyCurveName curve = null;
KeyCreateParameters parameters = new KeyCreateParameters();
parameters.withKty(kty);
parameters.withKeySize(null);
parameters.withKeyOps(null);
parameters.withKeyAttributes(null);
parameters.withTags(null);
parameters.withCurve(null);
String parameterizedHost = Joiner.on(", ").join("{vaultBaseUrl}", vaultBaseUrl);
return service.createKey(keyName, this.apiVersion(), this.acceptLanguage(), parameters, parameterizedHost, this.userAgent())
.flatMap(new Func1, Observable>>() {
@Override
public Observable> call(Response response) {
try {
ServiceResponse clientResponse = createKeyDelegate(response);
return Observable.just(clientResponse);
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
/**
* Creates a new key, stores it, then returns key parameters and attributes to the client.
* The create key operation can be used to create any key type in Azure Key Vault. If the named key already exists, Azure Key Vault creates a new version of the key. It requires the keys/create permission.
*
* @param vaultBaseUrl The vault name, for example https://myvault.vault.azure.net.
* @param keyName The name for the new key. The system will generate the version name for the new key.
* @param kty The type of key to create. For valid values, see JsonWebKeyType. Possible values include: 'EC', 'EC-HSM', 'RSA', 'RSA-HSM', 'oct'
* @param keySize The key size in bytes. For example, 1024 or 2048.
* @param keyOps the List<JsonWebKeyOperation> value
* @param keyAttributes the KeyAttributes value
* @param tags Application specific metadata in the form of key-value pairs.
* @param curve Elliptic curve name. For valid values, see JsonWebKeyCurveName. Possible values include: 'P-256', 'P-384', 'P-521', 'SECP256K1'
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws KeyVaultErrorException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the KeyBundle object if successful.
*/
public KeyBundle createKey(String vaultBaseUrl, String keyName, JsonWebKeyType kty, Integer keySize, List keyOps, KeyAttributes keyAttributes, Map tags, JsonWebKeyCurveName curve) {
return createKeyWithServiceResponseAsync(vaultBaseUrl, keyName, kty, keySize, keyOps, keyAttributes, tags, curve).toBlocking().single().body();
}
/**
* Creates a new key, stores it, then returns key parameters and attributes to the client.
* The create key operation can be used to create any key type in Azure Key Vault. If the named key already exists, Azure Key Vault creates a new version of the key. It requires the keys/create permission.
*
* @param vaultBaseUrl The vault name, for example https://myvault.vault.azure.net.
* @param keyName The name for the new key. The system will generate the version name for the new key.
* @param kty The type of key to create. For valid values, see JsonWebKeyType. Possible values include: 'EC', 'EC-HSM', 'RSA', 'RSA-HSM', 'oct'
* @param keySize The key size in bytes. For example, 1024 or 2048.
* @param keyOps the List<JsonWebKeyOperation> value
* @param keyAttributes the KeyAttributes value
* @param tags Application specific metadata in the form of key-value pairs.
* @param curve Elliptic curve name. For valid values, see JsonWebKeyCurveName. Possible values include: 'P-256', 'P-384', 'P-521', 'SECP256K1'
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture createKeyAsync(String vaultBaseUrl, String keyName, JsonWebKeyType kty, Integer keySize, List keyOps, KeyAttributes keyAttributes, Map tags, JsonWebKeyCurveName curve, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(createKeyWithServiceResponseAsync(vaultBaseUrl, keyName, kty, keySize, keyOps, keyAttributes, tags, curve), serviceCallback);
}
/**
* Creates a new key, stores it, then returns key parameters and attributes to the client.
* The create key operation can be used to create any key type in Azure Key Vault. If the named key already exists, Azure Key Vault creates a new version of the key. It requires the keys/create permission.
*
* @param vaultBaseUrl The vault name, for example https://myvault.vault.azure.net.
* @param keyName The name for the new key. The system will generate the version name for the new key.
* @param kty The type of key to create. For valid values, see JsonWebKeyType. Possible values include: 'EC', 'EC-HSM', 'RSA', 'RSA-HSM', 'oct'
* @param keySize The key size in bytes. For example, 1024 or 2048.
* @param keyOps the List<JsonWebKeyOperation> value
* @param keyAttributes the KeyAttributes value
* @param tags Application specific metadata in the form of key-value pairs.
* @param curve Elliptic curve name. For valid values, see JsonWebKeyCurveName. Possible values include: 'P-256', 'P-384', 'P-521', 'SECP256K1'
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the KeyBundle object
*/
public Observable createKeyAsync(String vaultBaseUrl, String keyName, JsonWebKeyType kty, Integer keySize, List keyOps, KeyAttributes keyAttributes, Map tags, JsonWebKeyCurveName curve) {
return createKeyWithServiceResponseAsync(vaultBaseUrl, keyName, kty, keySize, keyOps, keyAttributes, tags, curve).map(new Func1, KeyBundle>() {
@Override
public KeyBundle call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Creates a new key, stores it, then returns key parameters and attributes to the client.
* The create key operation can be used to create any key type in Azure Key Vault. If the named key already exists, Azure Key Vault creates a new version of the key. It requires the keys/create permission.
*
* @param vaultBaseUrl The vault name, for example https://myvault.vault.azure.net.
* @param keyName The name for the new key. The system will generate the version name for the new key.
* @param kty The type of key to create. For valid values, see JsonWebKeyType. Possible values include: 'EC', 'EC-HSM', 'RSA', 'RSA-HSM', 'oct'
* @param keySize The key size in bytes. For example, 1024 or 2048.
* @param keyOps the List<JsonWebKeyOperation> value
* @param keyAttributes the KeyAttributes value
* @param tags Application specific metadata in the form of key-value pairs.
* @param curve Elliptic curve name. For valid values, see JsonWebKeyCurveName. Possible values include: 'P-256', 'P-384', 'P-521', 'SECP256K1'
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the KeyBundle object
*/
public Observable> createKeyWithServiceResponseAsync(String vaultBaseUrl, String keyName, JsonWebKeyType kty, Integer keySize, List keyOps, KeyAttributes keyAttributes, Map tags, JsonWebKeyCurveName curve) {
if (vaultBaseUrl == null) {
throw new IllegalArgumentException("Parameter vaultBaseUrl is required and cannot be null.");
}
if (keyName == null) {
throw new IllegalArgumentException("Parameter keyName is required and cannot be null.");
}
if (this.apiVersion() == null) {
throw new IllegalArgumentException("Parameter this.apiVersion() is required and cannot be null.");
}
if (kty == null) {
throw new IllegalArgumentException("Parameter kty is required and cannot be null.");
}
Validator.validate(keyOps);
Validator.validate(keyAttributes);
Validator.validate(tags);
KeyCreateParameters parameters = new KeyCreateParameters();
parameters.withKty(kty);
parameters.withKeySize(keySize);
parameters.withKeyOps(keyOps);
parameters.withKeyAttributes(keyAttributes);
parameters.withTags(tags);
parameters.withCurve(curve);
String parameterizedHost = Joiner.on(", ").join("{vaultBaseUrl}", vaultBaseUrl);
return service.createKey(keyName, this.apiVersion(), this.acceptLanguage(), parameters, parameterizedHost, this.userAgent())
.flatMap(new Func1, Observable>>() {
@Override
public Observable> call(Response response) {
try {
ServiceResponse clientResponse = createKeyDelegate(response);
return Observable.just(clientResponse);
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse createKeyDelegate(Response response) throws KeyVaultErrorException, IOException, IllegalArgumentException {
return this.restClient().responseBuilderFactory().newInstance(this.serializerAdapter())
.register(200, new TypeToken() { }.getType())
.registerError(KeyVaultErrorException.class)
.build(response);
}
/**
* Imports an externally created key, stores it, and returns key parameters and attributes to the client.
* The import key operation may be used to import any key type into an Azure Key Vault. If the named key already exists, Azure Key Vault creates a new version of the key. This operation requires the keys/import permission.
*
* @param vaultBaseUrl The vault name, for example https://myvault.vault.azure.net.
* @param keyName Name for the imported key.
* @param key The Json web key
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws KeyVaultErrorException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the KeyBundle object if successful.
*/
public KeyBundle importKey(String vaultBaseUrl, String keyName, JsonWebKey key) {
return importKeyWithServiceResponseAsync(vaultBaseUrl, keyName, key).toBlocking().single().body();
}
/**
* Imports an externally created key, stores it, and returns key parameters and attributes to the client.
* The import key operation may be used to import any key type into an Azure Key Vault. If the named key already exists, Azure Key Vault creates a new version of the key. This operation requires the keys/import permission.
*
* @param vaultBaseUrl The vault name, for example https://myvault.vault.azure.net.
* @param keyName Name for the imported key.
* @param key The Json web key
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture importKeyAsync(String vaultBaseUrl, String keyName, JsonWebKey key, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(importKeyWithServiceResponseAsync(vaultBaseUrl, keyName, key), serviceCallback);
}
/**
* Imports an externally created key, stores it, and returns key parameters and attributes to the client.
* The import key operation may be used to import any key type into an Azure Key Vault. If the named key already exists, Azure Key Vault creates a new version of the key. This operation requires the keys/import permission.
*
* @param vaultBaseUrl The vault name, for example https://myvault.vault.azure.net.
* @param keyName Name for the imported key.
* @param key The Json web key
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the KeyBundle object
*/
public Observable importKeyAsync(String vaultBaseUrl, String keyName, JsonWebKey key) {
return importKeyWithServiceResponseAsync(vaultBaseUrl, keyName, key).map(new Func1, KeyBundle>() {
@Override
public KeyBundle call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Imports an externally created key, stores it, and returns key parameters and attributes to the client.
* The import key operation may be used to import any key type into an Azure Key Vault. If the named key already exists, Azure Key Vault creates a new version of the key. This operation requires the keys/import permission.
*
* @param vaultBaseUrl The vault name, for example https://myvault.vault.azure.net.
* @param keyName Name for the imported key.
* @param key The Json web key
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the KeyBundle object
*/
public Observable> importKeyWithServiceResponseAsync(String vaultBaseUrl, String keyName, JsonWebKey key) {
if (vaultBaseUrl == null) {
throw new IllegalArgumentException("Parameter vaultBaseUrl is required and cannot be null.");
}
if (keyName == null) {
throw new IllegalArgumentException("Parameter keyName is required and cannot be null.");
}
if (this.apiVersion() == null) {
throw new IllegalArgumentException("Parameter this.apiVersion() is required and cannot be null.");
}
if (key == null) {
throw new IllegalArgumentException("Parameter key is required and cannot be null.");
}
Validator.validate(key);
final Boolean hsm = null;
final KeyAttributes keyAttributes = null;
final Map tags = null;
KeyImportParameters parameters = new KeyImportParameters();
parameters.withHsm(null);
parameters.withKey(key);
parameters.withKeyAttributes(null);
parameters.withTags(null);
String parameterizedHost = Joiner.on(", ").join("{vaultBaseUrl}", vaultBaseUrl);
return service.importKey(keyName, this.apiVersion(), this.acceptLanguage(), parameters, parameterizedHost, this.userAgent())
.flatMap(new Func1, Observable>>() {
@Override
public Observable> call(Response response) {
try {
ServiceResponse clientResponse = importKeyDelegate(response);
return Observable.just(clientResponse);
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
/**
* Imports an externally created key, stores it, and returns key parameters and attributes to the client.
* The import key operation may be used to import any key type into an Azure Key Vault. If the named key already exists, Azure Key Vault creates a new version of the key. This operation requires the keys/import permission.
*
* @param vaultBaseUrl The vault name, for example https://myvault.vault.azure.net.
* @param keyName Name for the imported key.
* @param key The Json web key
* @param hsm Whether to import as a hardware key (HSM) or software key.
* @param keyAttributes The key management attributes.
* @param tags Application specific metadata in the form of key-value pairs.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws KeyVaultErrorException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the KeyBundle object if successful.
*/
public KeyBundle importKey(String vaultBaseUrl, String keyName, JsonWebKey key, Boolean hsm, KeyAttributes keyAttributes, Map tags) {
return importKeyWithServiceResponseAsync(vaultBaseUrl, keyName, key, hsm, keyAttributes, tags).toBlocking().single().body();
}
/**
* Imports an externally created key, stores it, and returns key parameters and attributes to the client.
* The import key operation may be used to import any key type into an Azure Key Vault. If the named key already exists, Azure Key Vault creates a new version of the key. This operation requires the keys/import permission.
*
* @param vaultBaseUrl The vault name, for example https://myvault.vault.azure.net.
* @param keyName Name for the imported key.
* @param key The Json web key
* @param hsm Whether to import as a hardware key (HSM) or software key.
* @param keyAttributes The key management attributes.
* @param tags Application specific metadata in the form of key-value pairs.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture importKeyAsync(String vaultBaseUrl, String keyName, JsonWebKey key, Boolean hsm, KeyAttributes keyAttributes, Map tags, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(importKeyWithServiceResponseAsync(vaultBaseUrl, keyName, key, hsm, keyAttributes, tags), serviceCallback);
}
/**
* Imports an externally created key, stores it, and returns key parameters and attributes to the client.
* The import key operation may be used to import any key type into an Azure Key Vault. If the named key already exists, Azure Key Vault creates a new version of the key. This operation requires the keys/import permission.
*
* @param vaultBaseUrl The vault name, for example https://myvault.vault.azure.net.
* @param keyName Name for the imported key.
* @param key The Json web key
* @param hsm Whether to import as a hardware key (HSM) or software key.
* @param keyAttributes The key management attributes.
* @param tags Application specific metadata in the form of key-value pairs.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the KeyBundle object
*/
public Observable importKeyAsync(String vaultBaseUrl, String keyName, JsonWebKey key, Boolean hsm, KeyAttributes keyAttributes, Map tags) {
return importKeyWithServiceResponseAsync(vaultBaseUrl, keyName, key, hsm, keyAttributes, tags).map(new Func1, KeyBundle>() {
@Override
public KeyBundle call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Imports an externally created key, stores it, and returns key parameters and attributes to the client.
* The import key operation may be used to import any key type into an Azure Key Vault. If the named key already exists, Azure Key Vault creates a new version of the key. This operation requires the keys/import permission.
*
* @param vaultBaseUrl The vault name, for example https://myvault.vault.azure.net.
* @param keyName Name for the imported key.
* @param key The Json web key
* @param hsm Whether to import as a hardware key (HSM) or software key.
* @param keyAttributes The key management attributes.
* @param tags Application specific metadata in the form of key-value pairs.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the KeyBundle object
*/
public Observable> importKeyWithServiceResponseAsync(String vaultBaseUrl, String keyName, JsonWebKey key, Boolean hsm, KeyAttributes keyAttributes, Map tags) {
if (vaultBaseUrl == null) {
throw new IllegalArgumentException("Parameter vaultBaseUrl is required and cannot be null.");
}
if (keyName == null) {
throw new IllegalArgumentException("Parameter keyName is required and cannot be null.");
}
if (this.apiVersion() == null) {
throw new IllegalArgumentException("Parameter this.apiVersion() is required and cannot be null.");
}
if (key == null) {
throw new IllegalArgumentException("Parameter key is required and cannot be null.");
}
Validator.validate(key);
Validator.validate(keyAttributes);
Validator.validate(tags);
KeyImportParameters parameters = new KeyImportParameters();
parameters.withHsm(hsm);
parameters.withKey(key);
parameters.withKeyAttributes(keyAttributes);
parameters.withTags(tags);
String parameterizedHost = Joiner.on(", ").join("{vaultBaseUrl}", vaultBaseUrl);
return service.importKey(keyName, this.apiVersion(), this.acceptLanguage(), parameters, parameterizedHost, this.userAgent())
.flatMap(new Func1, Observable>>() {
@Override
public Observable> call(Response response) {
try {
ServiceResponse clientResponse = importKeyDelegate(response);
return Observable.just(clientResponse);
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse importKeyDelegate(Response response) throws KeyVaultErrorException, IOException, IllegalArgumentException {
return this.restClient().responseBuilderFactory().newInstance(this.serializerAdapter())
.register(200, new TypeToken() { }.getType())
.registerError(KeyVaultErrorException.class)
.build(response);
}
/**
* Deletes a key of any type from storage in Azure Key Vault.
* The delete key operation cannot be used to remove individual versions of a key. This operation removes the cryptographic material associated with the key, which means the key is not usable for Sign/Verify, Wrap/Unwrap or Encrypt/Decrypt operations. This operation requires the keys/delete permission.
*
* @param vaultBaseUrl The vault name, for example https://myvault.vault.azure.net.
* @param keyName The name of the key to delete.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws KeyVaultErrorException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the DeletedKeyBundle object if successful.
*/
public DeletedKeyBundle deleteKey(String vaultBaseUrl, String keyName) {
return deleteKeyWithServiceResponseAsync(vaultBaseUrl, keyName).toBlocking().single().body();
}
/**
* Deletes a key of any type from storage in Azure Key Vault.
* The delete key operation cannot be used to remove individual versions of a key. This operation removes the cryptographic material associated with the key, which means the key is not usable for Sign/Verify, Wrap/Unwrap or Encrypt/Decrypt operations. This operation requires the keys/delete permission.
*
* @param vaultBaseUrl The vault name, for example https://myvault.vault.azure.net.
* @param keyName The name of the key to delete.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture deleteKeyAsync(String vaultBaseUrl, String keyName, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(deleteKeyWithServiceResponseAsync(vaultBaseUrl, keyName), serviceCallback);
}
/**
* Deletes a key of any type from storage in Azure Key Vault.
* The delete key operation cannot be used to remove individual versions of a key. This operation removes the cryptographic material associated with the key, which means the key is not usable for Sign/Verify, Wrap/Unwrap or Encrypt/Decrypt operations. This operation requires the keys/delete permission.
*
* @param vaultBaseUrl The vault name, for example https://myvault.vault.azure.net.
* @param keyName The name of the key to delete.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the DeletedKeyBundle object
*/
public Observable deleteKeyAsync(String vaultBaseUrl, String keyName) {
return deleteKeyWithServiceResponseAsync(vaultBaseUrl, keyName).map(new Func1, DeletedKeyBundle>() {
@Override
public DeletedKeyBundle call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Deletes a key of any type from storage in Azure Key Vault.
* The delete key operation cannot be used to remove individual versions of a key. This operation removes the cryptographic material associated with the key, which means the key is not usable for Sign/Verify, Wrap/Unwrap or Encrypt/Decrypt operations. This operation requires the keys/delete permission.
*
* @param vaultBaseUrl The vault name, for example https://myvault.vault.azure.net.
* @param keyName The name of the key to delete.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the DeletedKeyBundle object
*/
public Observable> deleteKeyWithServiceResponseAsync(String vaultBaseUrl, String keyName) {
if (vaultBaseUrl == null) {
throw new IllegalArgumentException("Parameter vaultBaseUrl is required and cannot be null.");
}
if (keyName == null) {
throw new IllegalArgumentException("Parameter keyName is required and cannot be null.");
}
if (this.apiVersion() == null) {
throw new IllegalArgumentException("Parameter this.apiVersion() is required and cannot be null.");
}
String parameterizedHost = Joiner.on(", ").join("{vaultBaseUrl}", vaultBaseUrl);
return service.deleteKey(keyName, this.apiVersion(), this.acceptLanguage(), parameterizedHost, this.userAgent())
.flatMap(new Func1, Observable>>() {
@Override
public Observable> call(Response response) {
try {
ServiceResponse clientResponse = deleteKeyDelegate(response);
return Observable.just(clientResponse);
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse deleteKeyDelegate(Response response) throws KeyVaultErrorException, IOException, IllegalArgumentException {
return this.restClient().responseBuilderFactory().newInstance(this.serializerAdapter())
.register(200, new TypeToken() { }.getType())
.registerError(KeyVaultErrorException.class)
.build(response);
}
/**
* The update key operation changes specified attributes of a stored key and can be applied to any key type and key version stored in Azure Key Vault.
* In order to perform this operation, the key must already exist in the Key Vault. Note: The cryptographic material of a key itself cannot be changed. This operation requires the keys/update permission.
*
* @param vaultBaseUrl The vault name, for example https://myvault.vault.azure.net.
* @param keyName The name of key to update.
* @param keyVersion The version of the key to update.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws KeyVaultErrorException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the KeyBundle object if successful.
*/
public KeyBundle updateKey(String vaultBaseUrl, String keyName, String keyVersion) {
return updateKeyWithServiceResponseAsync(vaultBaseUrl, keyName, keyVersion).toBlocking().single().body();
}
/**
* The update key operation changes specified attributes of a stored key and can be applied to any key type and key version stored in Azure Key Vault.
* In order to perform this operation, the key must already exist in the Key Vault. Note: The cryptographic material of a key itself cannot be changed. This operation requires the keys/update permission.
*
* @param vaultBaseUrl The vault name, for example https://myvault.vault.azure.net.
* @param keyName The name of key to update.
* @param keyVersion The version of the key to update.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture updateKeyAsync(String vaultBaseUrl, String keyName, String keyVersion, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(updateKeyWithServiceResponseAsync(vaultBaseUrl, keyName, keyVersion), serviceCallback);
}
/**
* The update key operation changes specified attributes of a stored key and can be applied to any key type and key version stored in Azure Key Vault.
* In order to perform this operation, the key must already exist in the Key Vault. Note: The cryptographic material of a key itself cannot be changed. This operation requires the keys/update permission.
*
* @param vaultBaseUrl The vault name, for example https://myvault.vault.azure.net.
* @param keyName The name of key to update.
* @param keyVersion The version of the key to update.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the KeyBundle object
*/
public Observable updateKeyAsync(String vaultBaseUrl, String keyName, String keyVersion) {
return updateKeyWithServiceResponseAsync(vaultBaseUrl, keyName, keyVersion).map(new Func1, KeyBundle>() {
@Override
public KeyBundle call(ServiceResponse response) {
return response.body();
}
});
}
/**
* The update key operation changes specified attributes of a stored key and can be applied to any key type and key version stored in Azure Key Vault.
* In order to perform this operation, the key must already exist in the Key Vault. Note: The cryptographic material of a key itself cannot be changed. This operation requires the keys/update permission.
*
* @param vaultBaseUrl The vault name, for example https://myvault.vault.azure.net.
* @param keyName The name of key to update.
* @param keyVersion The version of the key to update.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the KeyBundle object
*/
public Observable> updateKeyWithServiceResponseAsync(String vaultBaseUrl, String keyName, String keyVersion) {
if (vaultBaseUrl == null) {
throw new IllegalArgumentException("Parameter vaultBaseUrl is required and cannot be null.");
}
if (keyName == null) {
throw new IllegalArgumentException("Parameter keyName is required and cannot be null.");
}
if (keyVersion == null) {
throw new IllegalArgumentException("Parameter keyVersion is required and cannot be null.");
}
if (this.apiVersion() == null) {
throw new IllegalArgumentException("Parameter this.apiVersion() is required and cannot be null.");
}
final List keyOps = null;
final KeyAttributes keyAttributes = null;
final Map tags = null;
KeyUpdateParameters parameters = new KeyUpdateParameters();
parameters.withKeyOps(null);
parameters.withKeyAttributes(null);
parameters.withTags(null);
String parameterizedHost = Joiner.on(", ").join("{vaultBaseUrl}", vaultBaseUrl);
return service.updateKey(keyName, keyVersion, this.apiVersion(), this.acceptLanguage(), parameters, parameterizedHost, this.userAgent())
.flatMap(new Func1, Observable>>() {
@Override
public Observable> call(Response response) {
try {
ServiceResponse clientResponse = updateKeyDelegate(response);
return Observable.just(clientResponse);
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
/**
* The update key operation changes specified attributes of a stored key and can be applied to any key type and key version stored in Azure Key Vault.
* In order to perform this operation, the key must already exist in the Key Vault. Note: The cryptographic material of a key itself cannot be changed. This operation requires the keys/update permission.
*
* @param vaultBaseUrl The vault name, for example https://myvault.vault.azure.net.
* @param keyName The name of key to update.
* @param keyVersion The version of the key to update.
* @param keyOps Json web key operations. For more information on possible key operations, see JsonWebKeyOperation.
* @param keyAttributes the KeyAttributes value
* @param tags Application specific metadata in the form of key-value pairs.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws KeyVaultErrorException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the KeyBundle object if successful.
*/
public KeyBundle updateKey(String vaultBaseUrl, String keyName, String keyVersion, List keyOps, KeyAttributes keyAttributes, Map tags) {
return updateKeyWithServiceResponseAsync(vaultBaseUrl, keyName, keyVersion, keyOps, keyAttributes, tags).toBlocking().single().body();
}
/**
* The update key operation changes specified attributes of a stored key and can be applied to any key type and key version stored in Azure Key Vault.
* In order to perform this operation, the key must already exist in the Key Vault. Note: The cryptographic material of a key itself cannot be changed. This operation requires the keys/update permission.
*
* @param vaultBaseUrl The vault name, for example https://myvault.vault.azure.net.
* @param keyName The name of key to update.
* @param keyVersion The version of the key to update.
* @param keyOps Json web key operations. For more information on possible key operations, see JsonWebKeyOperation.
* @param keyAttributes the KeyAttributes value
* @param tags Application specific metadata in the form of key-value pairs.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture updateKeyAsync(String vaultBaseUrl, String keyName, String keyVersion, List keyOps, KeyAttributes keyAttributes, Map tags, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(updateKeyWithServiceResponseAsync(vaultBaseUrl, keyName, keyVersion, keyOps, keyAttributes, tags), serviceCallback);
}
/**
* The update key operation changes specified attributes of a stored key and can be applied to any key type and key version stored in Azure Key Vault.
* In order to perform this operation, the key must already exist in the Key Vault. Note: The cryptographic material of a key itself cannot be changed. This operation requires the keys/update permission.
*
* @param vaultBaseUrl The vault name, for example https://myvault.vault.azure.net.
* @param keyName The name of key to update.
* @param keyVersion The version of the key to update.
* @param keyOps Json web key operations. For more information on possible key operations, see JsonWebKeyOperation.
* @param keyAttributes the KeyAttributes value
* @param tags Application specific metadata in the form of key-value pairs.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the KeyBundle object
*/
public Observable updateKeyAsync(String vaultBaseUrl, String keyName, String keyVersion, List keyOps, KeyAttributes keyAttributes, Map tags) {
return updateKeyWithServiceResponseAsync(vaultBaseUrl, keyName, keyVersion, keyOps, keyAttributes, tags).map(new Func1, KeyBundle>() {
@Override
public KeyBundle call(ServiceResponse response) {
return response.body();
}
});
}
/**
* The update key operation changes specified attributes of a stored key and can be applied to any key type and key version stored in Azure Key Vault.
* In order to perform this operation, the key must already exist in the Key Vault. Note: The cryptographic material of a key itself cannot be changed. This operation requires the keys/update permission.
*
* @param vaultBaseUrl The vault name, for example https://myvault.vault.azure.net.
* @param keyName The name of key to update.
* @param keyVersion The version of the key to update.
* @param keyOps Json web key operations. For more information on possible key operations, see JsonWebKeyOperation.
* @param keyAttributes the KeyAttributes value
* @param tags Application specific metadata in the form of key-value pairs.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the KeyBundle object
*/
public Observable> updateKeyWithServiceResponseAsync(String vaultBaseUrl, String keyName, String keyVersion, List keyOps, KeyAttributes keyAttributes, Map tags) {
if (vaultBaseUrl == null) {
throw new IllegalArgumentException("Parameter vaultBaseUrl is required and cannot be null.");
}
if (keyName == null) {
throw new IllegalArgumentException("Parameter keyName is required and cannot be null.");
}
if (keyVersion == null) {
throw new IllegalArgumentException("Parameter keyVersion is required and cannot be null.");
}
if (this.apiVersion() == null) {
throw new IllegalArgumentException("Parameter this.apiVersion() is required and cannot be null.");
}
Validator.validate(keyOps);
Validator.validate(keyAttributes);
Validator.validate(tags);
KeyUpdateParameters parameters = new KeyUpdateParameters();
parameters.withKeyOps(keyOps);
parameters.withKeyAttributes(keyAttributes);
parameters.withTags(tags);
String parameterizedHost = Joiner.on(", ").join("{vaultBaseUrl}", vaultBaseUrl);
return service.updateKey(keyName, keyVersion, this.apiVersion(), this.acceptLanguage(), parameters, parameterizedHost, this.userAgent())
.flatMap(new Func1, Observable>>() {
@Override
public Observable> call(Response response) {
try {
ServiceResponse clientResponse = updateKeyDelegate(response);
return Observable.just(clientResponse);
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse updateKeyDelegate(Response response) throws KeyVaultErrorException, IOException, IllegalArgumentException {
return this.restClient().responseBuilderFactory().newInstance(this.serializerAdapter())
.register(200, new TypeToken() { }.getType())
.registerError(KeyVaultErrorException.class)
.build(response);
}
/**
* Gets the public part of a stored key.
* The get key operation is applicable to all key types. If the requested key is symmetric, then no key material is released in the response. This operation requires the keys/get permission.
*
* @param vaultBaseUrl The vault name, for example https://myvault.vault.azure.net.
* @param keyName The name of the key to get.
* @param keyVersion Adding the version parameter retrieves a specific version of a key.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws KeyVaultErrorException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the KeyBundle object if successful.
*/
public KeyBundle getKey(String vaultBaseUrl, String keyName, String keyVersion) {
return getKeyWithServiceResponseAsync(vaultBaseUrl, keyName, keyVersion).toBlocking().single().body();
}
/**
* Gets the public part of a stored key.
* The get key operation is applicable to all key types. If the requested key is symmetric, then no key material is released in the response. This operation requires the keys/get permission.
*
* @param vaultBaseUrl The vault name, for example https://myvault.vault.azure.net.
* @param keyName The name of the key to get.
* @param keyVersion Adding the version parameter retrieves a specific version of a key.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture getKeyAsync(String vaultBaseUrl, String keyName, String keyVersion, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(getKeyWithServiceResponseAsync(vaultBaseUrl, keyName, keyVersion), serviceCallback);
}
/**
* Gets the public part of a stored key.
* The get key operation is applicable to all key types. If the requested key is symmetric, then no key material is released in the response. This operation requires the keys/get permission.
*
* @param vaultBaseUrl The vault name, for example https://myvault.vault.azure.net.
* @param keyName The name of the key to get.
* @param keyVersion Adding the version parameter retrieves a specific version of a key.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the KeyBundle object
*/
public Observable getKeyAsync(String vaultBaseUrl, String keyName, String keyVersion) {
return getKeyWithServiceResponseAsync(vaultBaseUrl, keyName, keyVersion).map(new Func1, KeyBundle>() {
@Override
public KeyBundle call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Gets the public part of a stored key.
* The get key operation is applicable to all key types. If the requested key is symmetric, then no key material is released in the response. This operation requires the keys/get permission.
*
* @param vaultBaseUrl The vault name, for example https://myvault.vault.azure.net.
* @param keyName The name of the key to get.
* @param keyVersion Adding the version parameter retrieves a specific version of a key.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the KeyBundle object
*/
public Observable> getKeyWithServiceResponseAsync(String vaultBaseUrl, String keyName, String keyVersion) {
if (vaultBaseUrl == null) {
throw new IllegalArgumentException("Parameter vaultBaseUrl is required and cannot be null.");
}
if (keyName == null) {
throw new IllegalArgumentException("Parameter keyName is required and cannot be null.");
}
if (keyVersion == null) {
throw new IllegalArgumentException("Parameter keyVersion is required and cannot be null.");
}
if (this.apiVersion() == null) {
throw new IllegalArgumentException("Parameter this.apiVersion() is required and cannot be null.");
}
String parameterizedHost = Joiner.on(", ").join("{vaultBaseUrl}", vaultBaseUrl);
return service.getKey(keyName, keyVersion, this.apiVersion(), this.acceptLanguage(), parameterizedHost, this.userAgent())
.flatMap(new Func1, Observable>>() {
@Override
public Observable> call(Response response) {
try {
ServiceResponse clientResponse = getKeyDelegate(response);
return Observable.just(clientResponse);
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse getKeyDelegate(Response response) throws KeyVaultErrorException, IOException, IllegalArgumentException {
return this.restClient().responseBuilderFactory().newInstance(this.serializerAdapter())
.register(200, new TypeToken() { }.getType())
.registerError(KeyVaultErrorException.class)
.build(response);
}
/**
* Retrieves a list of individual key versions with the same key name.
* The full key identifier, attributes, and tags are provided in the response. This operation requires the keys/list permission.
*
* @param vaultBaseUrl The vault name, for example https://myvault.vault.azure.net.
* @param keyName The name of the key.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws KeyVaultErrorException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the PagedList<KeyItem> object if successful.
*/
public PagedList getKeyVersions(final String vaultBaseUrl, final String keyName) {
ServiceResponse> response = getKeyVersionsSinglePageAsync(vaultBaseUrl, keyName).toBlocking().single();
return new PagedList(response.body()) {
@Override
public Page nextPage(String nextPageLink) {
return getKeyVersionsNextSinglePageAsync(nextPageLink).toBlocking().single().body();
}
};
}
/**
* Retrieves a list of individual key versions with the same key name.
* The full key identifier, attributes, and tags are provided in the response. This operation requires the keys/list permission.
*
* @param vaultBaseUrl The vault name, for example https://myvault.vault.azure.net.
* @param keyName The name of the key.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture> getKeyVersionsAsync(final String vaultBaseUrl, final String keyName, final ListOperationCallback serviceCallback) {
return AzureServiceFuture.fromPageResponse(
getKeyVersionsSinglePageAsync(vaultBaseUrl, keyName),
new Func1>>>() {
@Override
public Observable>> call(String nextPageLink) {
return getKeyVersionsNextSinglePageAsync(nextPageLink);
}
},
serviceCallback);
}
/**
* Retrieves a list of individual key versions with the same key name.
* The full key identifier, attributes, and tags are provided in the response. This operation requires the keys/list permission.
*
* @param vaultBaseUrl The vault name, for example https://myvault.vault.azure.net.
* @param keyName The name of the key.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the PagedList<KeyItem> object
*/
public Observable> getKeyVersionsAsync(final String vaultBaseUrl, final String keyName) {
return getKeyVersionsWithServiceResponseAsync(vaultBaseUrl, keyName)
.map(new Func1>, Page>() {
@Override
public Page call(ServiceResponse> response) {
return response.body();
}
});
}
/**
* Retrieves a list of individual key versions with the same key name.
* The full key identifier, attributes, and tags are provided in the response. This operation requires the keys/list permission.
*
* @param vaultBaseUrl The vault name, for example https://myvault.vault.azure.net.
* @param keyName The name of the key.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the PagedList<KeyItem> object
*/
public Observable>> getKeyVersionsWithServiceResponseAsync(final String vaultBaseUrl, final String keyName) {
return getKeyVersionsSinglePageAsync(vaultBaseUrl, keyName)
.concatMap(new Func1>, Observable>>>() {
@Override
public Observable>> call(ServiceResponse> page) {
String nextPageLink = page.body().nextPageLink();
if (nextPageLink == null) {
return Observable.just(page);
}
return Observable.just(page).concatWith(getKeyVersionsNextWithServiceResponseAsync(nextPageLink));
}
});
}
/**
* Retrieves a list of individual key versions with the same key name.
* The full key identifier, attributes, and tags are provided in the response. This operation requires the keys/list permission.
*
* @param vaultBaseUrl The vault name, for example https://myvault.vault.azure.net.
* @param keyName The name of the key.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the PagedList<KeyItem> object wrapped in {@link ServiceResponse} if successful.
*/
public Observable>> getKeyVersionsSinglePageAsync(final String vaultBaseUrl, final String keyName) {
if (vaultBaseUrl == null) {
throw new IllegalArgumentException("Parameter vaultBaseUrl is required and cannot be null.");
}
if (keyName == null) {
throw new IllegalArgumentException("Parameter keyName is required and cannot be null.");
}
if (this.apiVersion() == null) {
throw new IllegalArgumentException("Parameter this.apiVersion() is required and cannot be null.");
}
final Integer maxresults = null;
String parameterizedHost = Joiner.on(", ").join("{vaultBaseUrl}", vaultBaseUrl);
return service.getKeyVersions(keyName, maxresults, this.apiVersion(), this.acceptLanguage(), parameterizedHost, this.userAgent())
.flatMap(new Func1, Observable>>>() {
@Override
public Observable>> call(Response response) {
try {
ServiceResponse> result = getKeyVersionsDelegate(response);
return Observable.just(new ServiceResponse>(result.body(), result.response()));
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
/**
* Retrieves a list of individual key versions with the same key name.
* The full key identifier, attributes, and tags are provided in the response. This operation requires the keys/list permission.
*
* @param vaultBaseUrl The vault name, for example https://myvault.vault.azure.net.
* @param keyName The name of the key.
* @param maxresults Maximum number of results to return in a page. If not specified the service will return up to 25 results.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws KeyVaultErrorException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the PagedList<KeyItem> object if successful.
*/
public PagedList getKeyVersions(final String vaultBaseUrl, final String keyName, final Integer maxresults) {
ServiceResponse> response = getKeyVersionsSinglePageAsync(vaultBaseUrl, keyName, maxresults).toBlocking().single();
return new PagedList