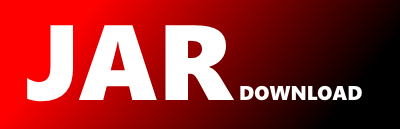
com.microsoft.azure.management.batch.Application Maven / Gradle / Ivy
Show all versions of azure-mgmt-batch Show documentation
/**
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for
* license information.
*/
package com.microsoft.azure.management.batch;
import com.microsoft.azure.management.apigeneration.Fluent;
import com.microsoft.azure.management.batch.implementation.ApplicationInner;
import com.microsoft.azure.management.resources.fluentcore.arm.models.ExternalChildResource;
import com.microsoft.azure.management.resources.fluentcore.model.Attachable;
import com.microsoft.azure.management.resources.fluentcore.model.Settable;
import com.microsoft.azure.management.resources.fluentcore.model.Wrapper;
import java.util.Map;
/**
* An immutable client-side representation of an Azure batch account application.
*/
@Fluent
public interface Application extends
ExternalChildResource,
Wrapper {
/**
* @return the display name for application
*/
String displayName();
/**
* @return the list of application packages
*/
Map applicationPackages();
/**
* @return true if automatic updates are allowed, otherwise false
*/
boolean updatesAllowed();
/**
* @return the default version for application.
*/
String defaultVersion();
/**************************************************************
* Fluent interfaces to provision an Application
**************************************************************/
/**
* The entirety of a application definition as a part of parent definition.
*
* @param the return type of the final {@link Attachable#attach()}
*/
interface Definition extends
DefinitionStages.Blank,
DefinitionStages.WithAttach {
}
/**
* Grouping of all the storage account definition stages.
*/
interface DefinitionStages {
/**
* The first stage of a batch account application definition.
*
* @param the return type of the final {@link WithAttach#attach()}
*/
interface Blank extends WithAttach {
}
/**
* A application definition to allow creation of application package.
*
* @param the return type of the final {@link WithAttach#attach()}
*/
interface WithApplicationPackage {
/**
* First stage to create new application package in Batch account application.
*
* @param applicationPackageName the version of the application
* @return next stage to create the application.
*/
DefinitionStages.WithAttach defineNewApplicationPackage(String applicationPackageName);
}
/**
* The final stage of the application definition.
*
* At this stage, any remaining optional settings can be specified, or the application definition
* can be attached to the parent batch account definition using {@link Application.DefinitionStages.WithAttach#attach()}.
* @param the return type of {@link Application.DefinitionStages.WithAttach#attach()}
*/
interface WithAttach extends
Attachable.InDefinition,
WithApplicationPackage {
/**
* Allow automatic application updates.
*
* @param allowUpdates true to allow the automatic updates of application, otherwise false
* @return parent batch account definition.
*/
DefinitionStages.WithAttach withAllowUpdates(boolean allowUpdates);
/**
* Specifies the display name for the application.
*
* @param displayName the displayName value to set
* @return parent batch account definition.
*/
DefinitionStages.WithAttach withDisplayName(String displayName);
}
}
/**
* The entirety of a application definition as a part of parent update.
* @param the return type of the final {@link Attachable#attach()}
*/
interface UpdateDefinition extends
UpdateDefinitionStages.Blank,
UpdateDefinitionStages.WithAttach {
}
/**
* Grouping of application definition stages as part of parent batch account update.
*/
interface UpdateDefinitionStages {
/**
* The first stage of a application definition.
*
* @param the return type of the final {@link WithAttach#attach()}
*/
interface Blank
extends WithAttach {
}
/**
* A application definition to allow creation of application package.
*
* @param the return type of the final {@link DefinitionStages.WithAttach#attach()}
*/
interface WithApplicationPackage {
/**
* First stage to create new application package in Batch account application.
*
* @param version the version of the application
* @return next stage to create the application.
*/
UpdateDefinitionStages.WithAttach defineNewApplicationPackage(String version);
}
/** The final stage of the application definition.
*
* At this stage, any remaining optional settings can be specified, or the application definition
* can be attached to the parent batch account definition using {@link Application.DefinitionStages.WithAttach#attach()}.
* @param the return type of {@link Application.DefinitionStages.WithAttach#attach()}
*/
interface WithAttach extends
Attachable.InUpdate,
WithApplicationPackage {
/**
* Allow automatic application updates.
*
* @param allowUpdates true to allow the automatic updates of application, otherwise false
* @return parent batch account update definition.
*/
UpdateDefinitionStages.WithAttach withAllowUpdates(boolean allowUpdates);
/**
* Specifies the display name for the application.
*
* @param displayName display name for the application.
* @return parent batch account update definition.
*/
UpdateDefinitionStages.WithAttach withDisplayName(String displayName);
}
}
/**
* Grouping of application update stages.
*/
interface UpdateStages {
/**
* A application definition to allow creation of application package.
*/
interface WithApplicationPackage {
/**
* First stage to create new application package in Batch account application.
*
* @param version the version of the application
* @return next stage to create the application.
*/
Update defineNewApplicationPackage(String version);
/**
* Deletes specified application package from the application.
*
* @param version the reference version of the application to be removed
* @return the stage representing updatable batch account definition.
*/
Update withoutApplicationPackage(String version);
}
/**
* The stage of the application update allowing to enable or disable auto upgrade of the
* application.
*/
interface WithOptionalProperties {
/**
* Allow automatic application updates.
*
* @param allowUpdates true to allow the automatic updates of application, otherwise false
* @return the next stage of the update
*/
Update withAllowUpdates(boolean allowUpdates);
/**
* Specifies the display name for the application.
*
* @param displayName the displayName value to set
* @return the next stage of the update
*/
Update withDisplayName(String displayName);
}
}
/**
* The entirety of application update as a part of parent batch account update.
*/
interface Update extends
Settable,
UpdateStages.WithOptionalProperties,
UpdateStages.WithApplicationPackage {
}
}