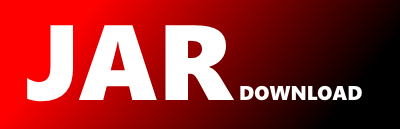
com.microsoft.azure.management.batch.implementation.BatchAccountsInner Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-mgmt-batch Show documentation
Show all versions of azure-mgmt-batch Show documentation
This package contains Microsoft Azure Batch Account Management SDK. This package has been deprecated. A replacement package com.azure.resourcemanager:azure-resourcemanager-batch is available as of 31-March-2022. We strongly encourage you to upgrade to continue receiving updates. See Migration Guide https://aka.ms/java-track2-migration-guide for guidance on upgrading. Refer to our deprecation policy https://azure.github.io/azure-sdk/policies_support.html for more details.
/**
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for
* license information.
*
* Code generated by Microsoft (R) AutoRest Code Generator.
*/
package com.microsoft.azure.management.batch.implementation;
import retrofit2.Retrofit;
import com.google.common.reflect.TypeToken;
import com.microsoft.azure.AzureServiceCall;
import com.microsoft.azure.AzureServiceResponseBuilder;
import com.microsoft.azure.CloudException;
import com.microsoft.azure.ListOperationCallback;
import com.microsoft.azure.management.batch.AccountKeyType;
import com.microsoft.azure.management.batch.BatchAccountRegenerateKeyParameters;
import com.microsoft.azure.Page;
import com.microsoft.azure.PagedList;
import com.microsoft.rest.ServiceCall;
import com.microsoft.rest.ServiceCallback;
import com.microsoft.rest.ServiceResponse;
import com.microsoft.rest.Validator;
import java.io.IOException;
import java.util.List;
import okhttp3.ResponseBody;
import retrofit2.http.Body;
import retrofit2.http.GET;
import retrofit2.http.Header;
import retrofit2.http.Headers;
import retrofit2.http.HTTP;
import retrofit2.http.PATCH;
import retrofit2.http.Path;
import retrofit2.http.POST;
import retrofit2.http.PUT;
import retrofit2.http.Query;
import retrofit2.Response;
import rx.functions.Func1;
import rx.Observable;
/**
* An instance of this class provides access to all the operations defined
* in BatchAccounts.
*/
public final class BatchAccountsInner {
/** The Retrofit service to perform REST calls. */
private BatchAccountsService service;
/** The service client containing this operation class. */
private BatchManagementClientImpl client;
/**
* Initializes an instance of BatchAccountsInner.
*
* @param retrofit the Retrofit instance built from a Retrofit Builder.
* @param client the instance of the service client containing this operation class.
*/
public BatchAccountsInner(Retrofit retrofit, BatchManagementClientImpl client) {
this.service = retrofit.create(BatchAccountsService.class);
this.client = client;
}
/**
* The interface defining all the services for BatchAccounts to be
* used by Retrofit to perform actually REST calls.
*/
interface BatchAccountsService {
@Headers("Content-Type: application/json; charset=utf-8")
@PUT("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Batch/batchAccounts/{accountName}")
Observable> create(@Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Path("subscriptionId") String subscriptionId, @Body BatchAccountCreateParametersInner parameters, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers("Content-Type: application/json; charset=utf-8")
@PUT("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Batch/batchAccounts/{accountName}")
Observable> beginCreate(@Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Path("subscriptionId") String subscriptionId, @Body BatchAccountCreateParametersInner parameters, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers("Content-Type: application/json; charset=utf-8")
@PATCH("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Batch/batchAccounts/{accountName}")
Observable> update(@Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Path("subscriptionId") String subscriptionId, @Body BatchAccountUpdateParametersInner parameters, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers("Content-Type: application/json; charset=utf-8")
@HTTP(path = "subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Batch/batchAccounts/{accountName}", method = "DELETE", hasBody = true)
Observable> delete(@Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Path("subscriptionId") String subscriptionId, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers("Content-Type: application/json; charset=utf-8")
@HTTP(path = "subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Batch/batchAccounts/{accountName}", method = "DELETE", hasBody = true)
Observable> beginDelete(@Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Path("subscriptionId") String subscriptionId, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers("Content-Type: application/json; charset=utf-8")
@GET("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Batch/batchAccounts/{accountName}")
Observable> get(@Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Path("subscriptionId") String subscriptionId, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers("Content-Type: application/json; charset=utf-8")
@GET("subscriptions/{subscriptionId}/providers/Microsoft.Batch/batchAccounts")
Observable> list(@Path("subscriptionId") String subscriptionId, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers("Content-Type: application/json; charset=utf-8")
@GET("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Batch/batchAccounts")
Observable> listByResourceGroup(@Path("resourceGroupName") String resourceGroupName, @Path("subscriptionId") String subscriptionId, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers("Content-Type: application/json; charset=utf-8")
@POST("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Batch/batchAccounts/{accountName}/syncAutoStorageKeys")
Observable> synchronizeAutoStorageKeys(@Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Path("subscriptionId") String subscriptionId, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers("Content-Type: application/json; charset=utf-8")
@POST("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Batch/batchAccounts/{accountName}/regenerateKeys")
Observable> regenerateKey(@Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Path("subscriptionId") String subscriptionId, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Body BatchAccountRegenerateKeyParameters parameters, @Header("User-Agent") String userAgent);
@Headers("Content-Type: application/json; charset=utf-8")
@POST("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Batch/batchAccounts/{accountName}/listKeys")
Observable> getKeys(@Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Path("subscriptionId") String subscriptionId, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers("Content-Type: application/json; charset=utf-8")
@GET("{nextLink}")
Observable> listNext(@Path(value = "nextLink", encoded = true) String nextPageLink, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers("Content-Type: application/json; charset=utf-8")
@GET("{nextLink}")
Observable> listByResourceGroupNext(@Path(value = "nextLink", encoded = true) String nextPageLink, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
}
/**
* Creates a new Batch account with the specified parameters. Existing accounts cannot be updated with this API and should instead be updated with the Update Batch Account API.
*
* @param resourceGroupName The name of the resource group that contains the new Batch account.
* @param accountName A name for the Batch account which must be unique within the region. Batch account names must be between 3 and 24 characters in length and must use only numbers and lowercase letters. This name is used as part of the DNS name that is used to access the Batch service in the region in which the account is created. For example: http://accountname.region.batch.azure.com/.
* @param parameters Additional parameters for account creation.
* @return the BatchAccountInner object if successful.
*/
public BatchAccountInner create(String resourceGroupName, String accountName, BatchAccountCreateParametersInner parameters) {
return createWithServiceResponseAsync(resourceGroupName, accountName, parameters).toBlocking().last().getBody();
}
/**
* Creates a new Batch account with the specified parameters. Existing accounts cannot be updated with this API and should instead be updated with the Update Batch Account API.
*
* @param resourceGroupName The name of the resource group that contains the new Batch account.
* @param accountName A name for the Batch account which must be unique within the region. Batch account names must be between 3 and 24 characters in length and must use only numbers and lowercase letters. This name is used as part of the DNS name that is used to access the Batch service in the region in which the account is created. For example: http://accountname.region.batch.azure.com/.
* @param parameters Additional parameters for account creation.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @return the {@link ServiceCall} object
*/
public ServiceCall createAsync(String resourceGroupName, String accountName, BatchAccountCreateParametersInner parameters, final ServiceCallback serviceCallback) {
return ServiceCall.create(createWithServiceResponseAsync(resourceGroupName, accountName, parameters), serviceCallback);
}
/**
* Creates a new Batch account with the specified parameters. Existing accounts cannot be updated with this API and should instead be updated with the Update Batch Account API.
*
* @param resourceGroupName The name of the resource group that contains the new Batch account.
* @param accountName A name for the Batch account which must be unique within the region. Batch account names must be between 3 and 24 characters in length and must use only numbers and lowercase letters. This name is used as part of the DNS name that is used to access the Batch service in the region in which the account is created. For example: http://accountname.region.batch.azure.com/.
* @param parameters Additional parameters for account creation.
* @return the observable for the request
*/
public Observable createAsync(String resourceGroupName, String accountName, BatchAccountCreateParametersInner parameters) {
return createWithServiceResponseAsync(resourceGroupName, accountName, parameters).map(new Func1, BatchAccountInner>() {
@Override
public BatchAccountInner call(ServiceResponse response) {
return response.getBody();
}
});
}
/**
* Creates a new Batch account with the specified parameters. Existing accounts cannot be updated with this API and should instead be updated with the Update Batch Account API.
*
* @param resourceGroupName The name of the resource group that contains the new Batch account.
* @param accountName A name for the Batch account which must be unique within the region. Batch account names must be between 3 and 24 characters in length and must use only numbers and lowercase letters. This name is used as part of the DNS name that is used to access the Batch service in the region in which the account is created. For example: http://accountname.region.batch.azure.com/.
* @param parameters Additional parameters for account creation.
* @return the observable for the request
*/
public Observable> createWithServiceResponseAsync(String resourceGroupName, String accountName, BatchAccountCreateParametersInner parameters) {
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (accountName == null) {
throw new IllegalArgumentException("Parameter accountName is required and cannot be null.");
}
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (parameters == null) {
throw new IllegalArgumentException("Parameter parameters is required and cannot be null.");
}
if (this.client.apiVersion() == null) {
throw new IllegalArgumentException("Parameter this.client.apiVersion() is required and cannot be null.");
}
Validator.validate(parameters);
Observable> observable = service.create(resourceGroupName, accountName, this.client.subscriptionId(), parameters, this.client.apiVersion(), this.client.acceptLanguage(), this.client.userAgent());
return client.getAzureClient().getPutOrPatchResultAsync(observable, new TypeToken() { }.getType());
}
/**
* Creates a new Batch account with the specified parameters. Existing accounts cannot be updated with this API and should instead be updated with the Update Batch Account API.
*
* @param resourceGroupName The name of the resource group that contains the new Batch account.
* @param accountName A name for the Batch account which must be unique within the region. Batch account names must be between 3 and 24 characters in length and must use only numbers and lowercase letters. This name is used as part of the DNS name that is used to access the Batch service in the region in which the account is created. For example: http://accountname.region.batch.azure.com/.
* @param parameters Additional parameters for account creation.
* @return the BatchAccountInner object if successful.
*/
public BatchAccountInner beginCreate(String resourceGroupName, String accountName, BatchAccountCreateParametersInner parameters) {
return beginCreateWithServiceResponseAsync(resourceGroupName, accountName, parameters).toBlocking().single().getBody();
}
/**
* Creates a new Batch account with the specified parameters. Existing accounts cannot be updated with this API and should instead be updated with the Update Batch Account API.
*
* @param resourceGroupName The name of the resource group that contains the new Batch account.
* @param accountName A name for the Batch account which must be unique within the region. Batch account names must be between 3 and 24 characters in length and must use only numbers and lowercase letters. This name is used as part of the DNS name that is used to access the Batch service in the region in which the account is created. For example: http://accountname.region.batch.azure.com/.
* @param parameters Additional parameters for account creation.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @return the {@link ServiceCall} object
*/
public ServiceCall beginCreateAsync(String resourceGroupName, String accountName, BatchAccountCreateParametersInner parameters, final ServiceCallback serviceCallback) {
return ServiceCall.create(beginCreateWithServiceResponseAsync(resourceGroupName, accountName, parameters), serviceCallback);
}
/**
* Creates a new Batch account with the specified parameters. Existing accounts cannot be updated with this API and should instead be updated with the Update Batch Account API.
*
* @param resourceGroupName The name of the resource group that contains the new Batch account.
* @param accountName A name for the Batch account which must be unique within the region. Batch account names must be between 3 and 24 characters in length and must use only numbers and lowercase letters. This name is used as part of the DNS name that is used to access the Batch service in the region in which the account is created. For example: http://accountname.region.batch.azure.com/.
* @param parameters Additional parameters for account creation.
* @return the observable to the BatchAccountInner object
*/
public Observable beginCreateAsync(String resourceGroupName, String accountName, BatchAccountCreateParametersInner parameters) {
return beginCreateWithServiceResponseAsync(resourceGroupName, accountName, parameters).map(new Func1, BatchAccountInner>() {
@Override
public BatchAccountInner call(ServiceResponse response) {
return response.getBody();
}
});
}
/**
* Creates a new Batch account with the specified parameters. Existing accounts cannot be updated with this API and should instead be updated with the Update Batch Account API.
*
* @param resourceGroupName The name of the resource group that contains the new Batch account.
* @param accountName A name for the Batch account which must be unique within the region. Batch account names must be between 3 and 24 characters in length and must use only numbers and lowercase letters. This name is used as part of the DNS name that is used to access the Batch service in the region in which the account is created. For example: http://accountname.region.batch.azure.com/.
* @param parameters Additional parameters for account creation.
* @return the observable to the BatchAccountInner object
*/
public Observable> beginCreateWithServiceResponseAsync(String resourceGroupName, String accountName, BatchAccountCreateParametersInner parameters) {
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (accountName == null) {
throw new IllegalArgumentException("Parameter accountName is required and cannot be null.");
}
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (parameters == null) {
throw new IllegalArgumentException("Parameter parameters is required and cannot be null.");
}
if (this.client.apiVersion() == null) {
throw new IllegalArgumentException("Parameter this.client.apiVersion() is required and cannot be null.");
}
Validator.validate(parameters);
return service.beginCreate(resourceGroupName, accountName, this.client.subscriptionId(), parameters, this.client.apiVersion(), this.client.acceptLanguage(), this.client.userAgent())
.flatMap(new Func1, Observable>>() {
@Override
public Observable> call(Response response) {
try {
ServiceResponse clientResponse = beginCreateDelegate(response);
return Observable.just(clientResponse);
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse beginCreateDelegate(Response response) throws CloudException, IOException, IllegalArgumentException {
return new AzureServiceResponseBuilder(this.client.mapperAdapter())
.register(202, new TypeToken() { }.getType())
.register(200, new TypeToken() { }.getType())
.registerError(CloudException.class)
.build(response);
}
/**
* Updates the properties of an existing Batch account.
*
* @param resourceGroupName The name of the resource group that contains the Batch account.
* @param accountName The name of the account.
* @param parameters Additional parameters for account update.
* @return the BatchAccountInner object if successful.
*/
public BatchAccountInner update(String resourceGroupName, String accountName, BatchAccountUpdateParametersInner parameters) {
return updateWithServiceResponseAsync(resourceGroupName, accountName, parameters).toBlocking().single().getBody();
}
/**
* Updates the properties of an existing Batch account.
*
* @param resourceGroupName The name of the resource group that contains the Batch account.
* @param accountName The name of the account.
* @param parameters Additional parameters for account update.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @return the {@link ServiceCall} object
*/
public ServiceCall updateAsync(String resourceGroupName, String accountName, BatchAccountUpdateParametersInner parameters, final ServiceCallback serviceCallback) {
return ServiceCall.create(updateWithServiceResponseAsync(resourceGroupName, accountName, parameters), serviceCallback);
}
/**
* Updates the properties of an existing Batch account.
*
* @param resourceGroupName The name of the resource group that contains the Batch account.
* @param accountName The name of the account.
* @param parameters Additional parameters for account update.
* @return the observable to the BatchAccountInner object
*/
public Observable updateAsync(String resourceGroupName, String accountName, BatchAccountUpdateParametersInner parameters) {
return updateWithServiceResponseAsync(resourceGroupName, accountName, parameters).map(new Func1, BatchAccountInner>() {
@Override
public BatchAccountInner call(ServiceResponse response) {
return response.getBody();
}
});
}
/**
* Updates the properties of an existing Batch account.
*
* @param resourceGroupName The name of the resource group that contains the Batch account.
* @param accountName The name of the account.
* @param parameters Additional parameters for account update.
* @return the observable to the BatchAccountInner object
*/
public Observable> updateWithServiceResponseAsync(String resourceGroupName, String accountName, BatchAccountUpdateParametersInner parameters) {
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (accountName == null) {
throw new IllegalArgumentException("Parameter accountName is required and cannot be null.");
}
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (parameters == null) {
throw new IllegalArgumentException("Parameter parameters is required and cannot be null.");
}
if (this.client.apiVersion() == null) {
throw new IllegalArgumentException("Parameter this.client.apiVersion() is required and cannot be null.");
}
Validator.validate(parameters);
return service.update(resourceGroupName, accountName, this.client.subscriptionId(), parameters, this.client.apiVersion(), this.client.acceptLanguage(), this.client.userAgent())
.flatMap(new Func1, Observable>>() {
@Override
public Observable> call(Response response) {
try {
ServiceResponse clientResponse = updateDelegate(response);
return Observable.just(clientResponse);
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse updateDelegate(Response response) throws CloudException, IOException, IllegalArgumentException {
return new AzureServiceResponseBuilder(this.client.mapperAdapter())
.register(200, new TypeToken() { }.getType())
.registerError(CloudException.class)
.build(response);
}
/**
* Deletes the specified Batch account.
*
* @param resourceGroupName The name of the resource group that contains the Batch account to be deleted.
* @param accountName The name of the account to be deleted.
*/
public void delete(String resourceGroupName, String accountName) {
deleteWithServiceResponseAsync(resourceGroupName, accountName).toBlocking().last().getBody();
}
/**
* Deletes the specified Batch account.
*
* @param resourceGroupName The name of the resource group that contains the Batch account to be deleted.
* @param accountName The name of the account to be deleted.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @return the {@link ServiceCall} object
*/
public ServiceCall deleteAsync(String resourceGroupName, String accountName, final ServiceCallback serviceCallback) {
return ServiceCall.create(deleteWithServiceResponseAsync(resourceGroupName, accountName), serviceCallback);
}
/**
* Deletes the specified Batch account.
*
* @param resourceGroupName The name of the resource group that contains the Batch account to be deleted.
* @param accountName The name of the account to be deleted.
* @return the observable for the request
*/
public Observable deleteAsync(String resourceGroupName, String accountName) {
return deleteWithServiceResponseAsync(resourceGroupName, accountName).map(new Func1, Void>() {
@Override
public Void call(ServiceResponse response) {
return response.getBody();
}
});
}
/**
* Deletes the specified Batch account.
*
* @param resourceGroupName The name of the resource group that contains the Batch account to be deleted.
* @param accountName The name of the account to be deleted.
* @return the observable for the request
*/
public Observable> deleteWithServiceResponseAsync(String resourceGroupName, String accountName) {
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (accountName == null) {
throw new IllegalArgumentException("Parameter accountName is required and cannot be null.");
}
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (this.client.apiVersion() == null) {
throw new IllegalArgumentException("Parameter this.client.apiVersion() is required and cannot be null.");
}
Observable> observable = service.delete(resourceGroupName, accountName, this.client.subscriptionId(), this.client.apiVersion(), this.client.acceptLanguage(), this.client.userAgent());
return client.getAzureClient().getPostOrDeleteResultAsync(observable, new TypeToken() { }.getType());
}
/**
* Deletes the specified Batch account.
*
* @param resourceGroupName The name of the resource group that contains the Batch account to be deleted.
* @param accountName The name of the account to be deleted.
*/
public void beginDelete(String resourceGroupName, String accountName) {
beginDeleteWithServiceResponseAsync(resourceGroupName, accountName).toBlocking().single().getBody();
}
/**
* Deletes the specified Batch account.
*
* @param resourceGroupName The name of the resource group that contains the Batch account to be deleted.
* @param accountName The name of the account to be deleted.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @return the {@link ServiceCall} object
*/
public ServiceCall beginDeleteAsync(String resourceGroupName, String accountName, final ServiceCallback serviceCallback) {
return ServiceCall.create(beginDeleteWithServiceResponseAsync(resourceGroupName, accountName), serviceCallback);
}
/**
* Deletes the specified Batch account.
*
* @param resourceGroupName The name of the resource group that contains the Batch account to be deleted.
* @param accountName The name of the account to be deleted.
* @return the {@link ServiceResponse} object if successful.
*/
public Observable beginDeleteAsync(String resourceGroupName, String accountName) {
return beginDeleteWithServiceResponseAsync(resourceGroupName, accountName).map(new Func1, Void>() {
@Override
public Void call(ServiceResponse response) {
return response.getBody();
}
});
}
/**
* Deletes the specified Batch account.
*
* @param resourceGroupName The name of the resource group that contains the Batch account to be deleted.
* @param accountName The name of the account to be deleted.
* @return the {@link ServiceResponse} object if successful.
*/
public Observable> beginDeleteWithServiceResponseAsync(String resourceGroupName, String accountName) {
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (accountName == null) {
throw new IllegalArgumentException("Parameter accountName is required and cannot be null.");
}
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (this.client.apiVersion() == null) {
throw new IllegalArgumentException("Parameter this.client.apiVersion() is required and cannot be null.");
}
return service.beginDelete(resourceGroupName, accountName, this.client.subscriptionId(), this.client.apiVersion(), this.client.acceptLanguage(), this.client.userAgent())
.flatMap(new Func1, Observable>>() {
@Override
public Observable> call(Response response) {
try {
ServiceResponse clientResponse = beginDeleteDelegate(response);
return Observable.just(clientResponse);
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse beginDeleteDelegate(Response response) throws CloudException, IOException, IllegalArgumentException {
return new AzureServiceResponseBuilder(this.client.mapperAdapter())
.register(202, new TypeToken() { }.getType())
.register(204, new TypeToken() { }.getType())
.register(200, new TypeToken() { }.getType())
.build(response);
}
/**
* Gets information about the specified Batch account.
*
* @param resourceGroupName The name of the resource group that contains the Batch account.
* @param accountName The name of the account.
* @return the BatchAccountInner object if successful.
*/
public BatchAccountInner get(String resourceGroupName, String accountName) {
return getWithServiceResponseAsync(resourceGroupName, accountName).toBlocking().single().getBody();
}
/**
* Gets information about the specified Batch account.
*
* @param resourceGroupName The name of the resource group that contains the Batch account.
* @param accountName The name of the account.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @return the {@link ServiceCall} object
*/
public ServiceCall getAsync(String resourceGroupName, String accountName, final ServiceCallback serviceCallback) {
return ServiceCall.create(getWithServiceResponseAsync(resourceGroupName, accountName), serviceCallback);
}
/**
* Gets information about the specified Batch account.
*
* @param resourceGroupName The name of the resource group that contains the Batch account.
* @param accountName The name of the account.
* @return the observable to the BatchAccountInner object
*/
public Observable getAsync(String resourceGroupName, String accountName) {
return getWithServiceResponseAsync(resourceGroupName, accountName).map(new Func1, BatchAccountInner>() {
@Override
public BatchAccountInner call(ServiceResponse response) {
return response.getBody();
}
});
}
/**
* Gets information about the specified Batch account.
*
* @param resourceGroupName The name of the resource group that contains the Batch account.
* @param accountName The name of the account.
* @return the observable to the BatchAccountInner object
*/
public Observable> getWithServiceResponseAsync(String resourceGroupName, String accountName) {
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (accountName == null) {
throw new IllegalArgumentException("Parameter accountName is required and cannot be null.");
}
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (this.client.apiVersion() == null) {
throw new IllegalArgumentException("Parameter this.client.apiVersion() is required and cannot be null.");
}
return service.get(resourceGroupName, accountName, this.client.subscriptionId(), this.client.apiVersion(), this.client.acceptLanguage(), this.client.userAgent())
.flatMap(new Func1, Observable>>() {
@Override
public Observable> call(Response response) {
try {
ServiceResponse clientResponse = getDelegate(response);
return Observable.just(clientResponse);
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse getDelegate(Response response) throws CloudException, IOException, IllegalArgumentException {
return new AzureServiceResponseBuilder(this.client.mapperAdapter())
.register(200, new TypeToken() { }.getType())
.registerError(CloudException.class)
.build(response);
}
/**
* Gets information about the Batch accounts associated with the subscription.
*
* @return the PagedList<BatchAccountInner> object if successful.
*/
public PagedList list() {
ServiceResponse> response = listSinglePageAsync().toBlocking().single();
return new PagedList(response.getBody()) {
@Override
public Page nextPage(String nextPageLink) {
return listNextSinglePageAsync(nextPageLink).toBlocking().single().getBody();
}
};
}
/**
* Gets information about the Batch accounts associated with the subscription.
*
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @return the {@link ServiceCall} object
*/
public ServiceCall> listAsync(final ListOperationCallback serviceCallback) {
return AzureServiceCall.create(
listSinglePageAsync(),
new Func1>>>() {
@Override
public Observable>> call(String nextPageLink) {
return listNextSinglePageAsync(nextPageLink);
}
},
serviceCallback);
}
/**
* Gets information about the Batch accounts associated with the subscription.
*
* @return the observable to the PagedList<BatchAccountInner> object
*/
public Observable> listAsync() {
return listWithServiceResponseAsync()
.map(new Func1>, Page>() {
@Override
public Page call(ServiceResponse> response) {
return response.getBody();
}
});
}
/**
* Gets information about the Batch accounts associated with the subscription.
*
* @return the observable to the PagedList<BatchAccountInner> object
*/
public Observable>> listWithServiceResponseAsync() {
return listSinglePageAsync()
.concatMap(new Func1>, Observable>>>() {
@Override
public Observable>> call(ServiceResponse> page) {
String nextPageLink = page.getBody().getNextPageLink();
if (nextPageLink == null) {
return Observable.just(page);
}
return Observable.just(page).concatWith(listNextWithServiceResponseAsync(nextPageLink));
}
});
}
/**
* Gets information about the Batch accounts associated with the subscription.
*
* @return the PagedList<BatchAccountInner> object wrapped in {@link ServiceResponse} if successful.
*/
public Observable>> listSinglePageAsync() {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (this.client.apiVersion() == null) {
throw new IllegalArgumentException("Parameter this.client.apiVersion() is required and cannot be null.");
}
return service.list(this.client.subscriptionId(), this.client.apiVersion(), this.client.acceptLanguage(), this.client.userAgent())
.flatMap(new Func1, Observable>>>() {
@Override
public Observable>> call(Response response) {
try {
ServiceResponse> result = listDelegate(response);
return Observable.just(new ServiceResponse>(result.getBody(), result.getResponse()));
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse> listDelegate(Response response) throws CloudException, IOException, IllegalArgumentException {
return new AzureServiceResponseBuilder, CloudException>(this.client.mapperAdapter())
.register(200, new TypeToken>() { }.getType())
.registerError(CloudException.class)
.build(response);
}
/**
* Gets information about the Batch accounts associated within the specified resource group.
*
* @param resourceGroupName The name of the resource group whose Batch accounts to list.
* @return the PagedList<BatchAccountInner> object if successful.
*/
public PagedList listByResourceGroup(final String resourceGroupName) {
ServiceResponse> response = listByResourceGroupSinglePageAsync(resourceGroupName).toBlocking().single();
return new PagedList(response.getBody()) {
@Override
public Page nextPage(String nextPageLink) {
return listByResourceGroupNextSinglePageAsync(nextPageLink).toBlocking().single().getBody();
}
};
}
/**
* Gets information about the Batch accounts associated within the specified resource group.
*
* @param resourceGroupName The name of the resource group whose Batch accounts to list.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @return the {@link ServiceCall} object
*/
public ServiceCall> listByResourceGroupAsync(final String resourceGroupName, final ListOperationCallback serviceCallback) {
return AzureServiceCall.create(
listByResourceGroupSinglePageAsync(resourceGroupName),
new Func1>>>() {
@Override
public Observable>> call(String nextPageLink) {
return listByResourceGroupNextSinglePageAsync(nextPageLink);
}
},
serviceCallback);
}
/**
* Gets information about the Batch accounts associated within the specified resource group.
*
* @param resourceGroupName The name of the resource group whose Batch accounts to list.
* @return the observable to the PagedList<BatchAccountInner> object
*/
public Observable> listByResourceGroupAsync(final String resourceGroupName) {
return listByResourceGroupWithServiceResponseAsync(resourceGroupName)
.map(new Func1>, Page>() {
@Override
public Page call(ServiceResponse> response) {
return response.getBody();
}
});
}
/**
* Gets information about the Batch accounts associated within the specified resource group.
*
* @param resourceGroupName The name of the resource group whose Batch accounts to list.
* @return the observable to the PagedList<BatchAccountInner> object
*/
public Observable>> listByResourceGroupWithServiceResponseAsync(final String resourceGroupName) {
return listByResourceGroupSinglePageAsync(resourceGroupName)
.concatMap(new Func1>, Observable>>>() {
@Override
public Observable>> call(ServiceResponse> page) {
String nextPageLink = page.getBody().getNextPageLink();
if (nextPageLink == null) {
return Observable.just(page);
}
return Observable.just(page).concatWith(listByResourceGroupNextWithServiceResponseAsync(nextPageLink));
}
});
}
/**
* Gets information about the Batch accounts associated within the specified resource group.
*
ServiceResponse> * @param resourceGroupName The name of the resource group whose Batch accounts to list.
* @return the PagedList<BatchAccountInner> object wrapped in {@link ServiceResponse} if successful.
*/
public Observable>> listByResourceGroupSinglePageAsync(final String resourceGroupName) {
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (this.client.apiVersion() == null) {
throw new IllegalArgumentException("Parameter this.client.apiVersion() is required and cannot be null.");
}
return service.listByResourceGroup(resourceGroupName, this.client.subscriptionId(), this.client.apiVersion(), this.client.acceptLanguage(), this.client.userAgent())
.flatMap(new Func1, Observable>>>() {
@Override
public Observable>> call(Response response) {
try {
ServiceResponse> result = listByResourceGroupDelegate(response);
return Observable.just(new ServiceResponse>(result.getBody(), result.getResponse()));
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse> listByResourceGroupDelegate(Response response) throws CloudException, IOException, IllegalArgumentException {
return new AzureServiceResponseBuilder, CloudException>(this.client.mapperAdapter())
.register(200, new TypeToken>() { }.getType())
.registerError(CloudException.class)
.build(response);
}
/**
* Synchronizes access keys for the auto storage account configured for the specified Batch account.
*
* @param resourceGroupName The name of the resource group that contains the Batch account.
* @param accountName The name of the Batch account.
*/
public void synchronizeAutoStorageKeys(String resourceGroupName, String accountName) {
synchronizeAutoStorageKeysWithServiceResponseAsync(resourceGroupName, accountName).toBlocking().single().getBody();
}
/**
* Synchronizes access keys for the auto storage account configured for the specified Batch account.
*
* @param resourceGroupName The name of the resource group that contains the Batch account.
* @param accountName The name of the Batch account.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @return the {@link ServiceCall} object
*/
public ServiceCall synchronizeAutoStorageKeysAsync(String resourceGroupName, String accountName, final ServiceCallback serviceCallback) {
return ServiceCall.create(synchronizeAutoStorageKeysWithServiceResponseAsync(resourceGroupName, accountName), serviceCallback);
}
/**
* Synchronizes access keys for the auto storage account configured for the specified Batch account.
*
* @param resourceGroupName The name of the resource group that contains the Batch account.
* @param accountName The name of the Batch account.
* @return the {@link ServiceResponse} object if successful.
*/
public Observable synchronizeAutoStorageKeysAsync(String resourceGroupName, String accountName) {
return synchronizeAutoStorageKeysWithServiceResponseAsync(resourceGroupName, accountName).map(new Func1, Void>() {
@Override
public Void call(ServiceResponse response) {
return response.getBody();
}
});
}
/**
* Synchronizes access keys for the auto storage account configured for the specified Batch account.
*
* @param resourceGroupName The name of the resource group that contains the Batch account.
* @param accountName The name of the Batch account.
* @return the {@link ServiceResponse} object if successful.
*/
public Observable> synchronizeAutoStorageKeysWithServiceResponseAsync(String resourceGroupName, String accountName) {
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (accountName == null) {
throw new IllegalArgumentException("Parameter accountName is required and cannot be null.");
}
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (this.client.apiVersion() == null) {
throw new IllegalArgumentException("Parameter this.client.apiVersion() is required and cannot be null.");
}
return service.synchronizeAutoStorageKeys(resourceGroupName, accountName, this.client.subscriptionId(), this.client.apiVersion(), this.client.acceptLanguage(), this.client.userAgent())
.flatMap(new Func1, Observable>>() {
@Override
public Observable> call(Response response) {
try {
ServiceResponse clientResponse = synchronizeAutoStorageKeysDelegate(response);
return Observable.just(clientResponse);
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse synchronizeAutoStorageKeysDelegate(Response response) throws CloudException, IOException, IllegalArgumentException {
return new AzureServiceResponseBuilder(this.client.mapperAdapter())
.register(204, new TypeToken() { }.getType())
.build(response);
}
/**
* Regenerates the specified account key for the specified Batch account.
*
* @param resourceGroupName The name of the resource group that contains the Batch account.
* @param accountName The name of the account.
* @param keyName The type of account key to regenerate. Possible values include: 'Primary', 'Secondary'
* @return the BatchAccountKeysInner object if successful.
*/
public BatchAccountKeysInner regenerateKey(String resourceGroupName, String accountName, AccountKeyType keyName) {
return regenerateKeyWithServiceResponseAsync(resourceGroupName, accountName, keyName).toBlocking().single().getBody();
}
/**
* Regenerates the specified account key for the specified Batch account.
*
* @param resourceGroupName The name of the resource group that contains the Batch account.
* @param accountName The name of the account.
* @param keyName The type of account key to regenerate. Possible values include: 'Primary', 'Secondary'
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @return the {@link ServiceCall} object
*/
public ServiceCall regenerateKeyAsync(String resourceGroupName, String accountName, AccountKeyType keyName, final ServiceCallback serviceCallback) {
return ServiceCall.create(regenerateKeyWithServiceResponseAsync(resourceGroupName, accountName, keyName), serviceCallback);
}
/**
* Regenerates the specified account key for the specified Batch account.
*
* @param resourceGroupName The name of the resource group that contains the Batch account.
* @param accountName The name of the account.
* @param keyName The type of account key to regenerate. Possible values include: 'Primary', 'Secondary'
* @return the observable to the BatchAccountKeysInner object
*/
public Observable regenerateKeyAsync(String resourceGroupName, String accountName, AccountKeyType keyName) {
return regenerateKeyWithServiceResponseAsync(resourceGroupName, accountName, keyName).map(new Func1, BatchAccountKeysInner>() {
@Override
public BatchAccountKeysInner call(ServiceResponse response) {
return response.getBody();
}
});
}
/**
* Regenerates the specified account key for the specified Batch account.
*
* @param resourceGroupName The name of the resource group that contains the Batch account.
* @param accountName The name of the account.
* @param keyName The type of account key to regenerate. Possible values include: 'Primary', 'Secondary'
* @return the observable to the BatchAccountKeysInner object
*/
public Observable> regenerateKeyWithServiceResponseAsync(String resourceGroupName, String accountName, AccountKeyType keyName) {
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (accountName == null) {
throw new IllegalArgumentException("Parameter accountName is required and cannot be null.");
}
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (this.client.apiVersion() == null) {
throw new IllegalArgumentException("Parameter this.client.apiVersion() is required and cannot be null.");
}
if (keyName == null) {
throw new IllegalArgumentException("Parameter keyName is required and cannot be null.");
}
BatchAccountRegenerateKeyParameters parameters = new BatchAccountRegenerateKeyParameters();
parameters.withKeyName(keyName);
return service.regenerateKey(resourceGroupName, accountName, this.client.subscriptionId(), this.client.apiVersion(), this.client.acceptLanguage(), parameters, this.client.userAgent())
.flatMap(new Func1, Observable>>() {
@Override
public Observable> call(Response response) {
try {
ServiceResponse clientResponse = regenerateKeyDelegate(response);
return Observable.just(clientResponse);
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse regenerateKeyDelegate(Response response) throws CloudException, IOException, IllegalArgumentException {
return new AzureServiceResponseBuilder(this.client.mapperAdapter())
.register(200, new TypeToken() { }.getType())
.registerError(CloudException.class)
.build(response);
}
/**
* Gets the account keys for the specified Batch account.
*
* @param resourceGroupName The name of the resource group that contains the Batch account.
* @param accountName The name of the account.
* @return the BatchAccountKeysInner object if successful.
*/
public BatchAccountKeysInner getKeys(String resourceGroupName, String accountName) {
return getKeysWithServiceResponseAsync(resourceGroupName, accountName).toBlocking().single().getBody();
}
/**
* Gets the account keys for the specified Batch account.
*
* @param resourceGroupName The name of the resource group that contains the Batch account.
* @param accountName The name of the account.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @return the {@link ServiceCall} object
*/
public ServiceCall getKeysAsync(String resourceGroupName, String accountName, final ServiceCallback serviceCallback) {
return ServiceCall.create(getKeysWithServiceResponseAsync(resourceGroupName, accountName), serviceCallback);
}
/**
* Gets the account keys for the specified Batch account.
*
* @param resourceGroupName The name of the resource group that contains the Batch account.
* @param accountName The name of the account.
* @return the observable to the BatchAccountKeysInner object
*/
public Observable getKeysAsync(String resourceGroupName, String accountName) {
return getKeysWithServiceResponseAsync(resourceGroupName, accountName).map(new Func1, BatchAccountKeysInner>() {
@Override
public BatchAccountKeysInner call(ServiceResponse response) {
return response.getBody();
}
});
}
/**
* Gets the account keys for the specified Batch account.
*
* @param resourceGroupName The name of the resource group that contains the Batch account.
* @param accountName The name of the account.
* @return the observable to the BatchAccountKeysInner object
*/
public Observable> getKeysWithServiceResponseAsync(String resourceGroupName, String accountName) {
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (accountName == null) {
throw new IllegalArgumentException("Parameter accountName is required and cannot be null.");
}
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (this.client.apiVersion() == null) {
throw new IllegalArgumentException("Parameter this.client.apiVersion() is required and cannot be null.");
}
return service.getKeys(resourceGroupName, accountName, this.client.subscriptionId(), this.client.apiVersion(), this.client.acceptLanguage(), this.client.userAgent())
.flatMap(new Func1, Observable>>() {
@Override
public Observable> call(Response response) {
try {
ServiceResponse clientResponse = getKeysDelegate(response);
return Observable.just(clientResponse);
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse getKeysDelegate(Response response) throws CloudException, IOException, IllegalArgumentException {
return new AzureServiceResponseBuilder(this.client.mapperAdapter())
.register(200, new TypeToken() { }.getType())
.registerError(CloudException.class)
.build(response);
}
/**
* Gets information about the Batch accounts associated with the subscription.
*
* @param nextPageLink The NextLink from the previous successful call to List operation.
* @return the PagedList<BatchAccountInner> object if successful.
*/
public PagedList listNext(final String nextPageLink) {
ServiceResponse> response = listNextSinglePageAsync(nextPageLink).toBlocking().single();
return new PagedList(response.getBody()) {
@Override
public Page nextPage(String nextPageLink) {
return listNextSinglePageAsync(nextPageLink).toBlocking().single().getBody();
}
};
}
/**
* Gets information about the Batch accounts associated with the subscription.
*
* @param nextPageLink The NextLink from the previous successful call to List operation.
* @param serviceCall the ServiceCall object tracking the Retrofit calls
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @return the {@link ServiceCall} object
*/
public ServiceCall> listNextAsync(final String nextPageLink, final ServiceCall> serviceCall, final ListOperationCallback serviceCallback) {
return AzureServiceCall.create(
listNextSinglePageAsync(nextPageLink),
new Func1>>>() {
@Override
public Observable>> call(String nextPageLink) {
return listNextSinglePageAsync(nextPageLink);
}
},
serviceCallback);
}
/**
* Gets information about the Batch accounts associated with the subscription.
*
* @param nextPageLink The NextLink from the previous successful call to List operation.
* @return the observable to the PagedList<BatchAccountInner> object
*/
public Observable> listNextAsync(final String nextPageLink) {
return listNextWithServiceResponseAsync(nextPageLink)
.map(new Func1>, Page>() {
@Override
public Page call(ServiceResponse> response) {
return response.getBody();
}
});
}
/**
* Gets information about the Batch accounts associated with the subscription.
*
* @param nextPageLink The NextLink from the previous successful call to List operation.
* @return the observable to the PagedList<BatchAccountInner> object
*/
public Observable>> listNextWithServiceResponseAsync(final String nextPageLink) {
return listNextSinglePageAsync(nextPageLink)
.concatMap(new Func1>, Observable>>>() {
@Override
public Observable>> call(ServiceResponse> page) {
String nextPageLink = page.getBody().getNextPageLink();
if (nextPageLink == null) {
return Observable.just(page);
}
return Observable.just(page).concatWith(listNextWithServiceResponseAsync(nextPageLink));
}
});
}
/**
* Gets information about the Batch accounts associated with the subscription.
*
ServiceResponse> * @param nextPageLink The NextLink from the previous successful call to List operation.
* @return the PagedList<BatchAccountInner> object wrapped in {@link ServiceResponse} if successful.
*/
public Observable>> listNextSinglePageAsync(final String nextPageLink) {
if (nextPageLink == null) {
throw new IllegalArgumentException("Parameter nextPageLink is required and cannot be null.");
}
return service.listNext(nextPageLink, this.client.acceptLanguage(), this.client.userAgent())
.flatMap(new Func1, Observable>>>() {
@Override
public Observable>> call(Response response) {
try {
ServiceResponse> result = listNextDelegate(response);
return Observable.just(new ServiceResponse>(result.getBody(), result.getResponse()));
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse> listNextDelegate(Response response) throws CloudException, IOException, IllegalArgumentException {
return new AzureServiceResponseBuilder, CloudException>(this.client.mapperAdapter())
.register(200, new TypeToken>() { }.getType())
.registerError(CloudException.class)
.build(response);
}
/**
* Gets information about the Batch accounts associated within the specified resource group.
*
* @param nextPageLink The NextLink from the previous successful call to List operation.
* @return the PagedList<BatchAccountInner> object if successful.
*/
public PagedList listByResourceGroupNext(final String nextPageLink) {
ServiceResponse> response = listByResourceGroupNextSinglePageAsync(nextPageLink).toBlocking().single();
return new PagedList(response.getBody()) {
@Override
public Page nextPage(String nextPageLink) {
return listByResourceGroupNextSinglePageAsync(nextPageLink).toBlocking().single().getBody();
}
};
}
/**
* Gets information about the Batch accounts associated within the specified resource group.
*
* @param nextPageLink The NextLink from the previous successful call to List operation.
* @param serviceCall the ServiceCall object tracking the Retrofit calls
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @return the {@link ServiceCall} object
*/
public ServiceCall> listByResourceGroupNextAsync(final String nextPageLink, final ServiceCall> serviceCall, final ListOperationCallback serviceCallback) {
return AzureServiceCall.create(
listByResourceGroupNextSinglePageAsync(nextPageLink),
new Func1>>>() {
@Override
public Observable>> call(String nextPageLink) {
return listByResourceGroupNextSinglePageAsync(nextPageLink);
}
},
serviceCallback);
}
/**
* Gets information about the Batch accounts associated within the specified resource group.
*
* @param nextPageLink The NextLink from the previous successful call to List operation.
* @return the observable to the PagedList<BatchAccountInner> object
*/
public Observable> listByResourceGroupNextAsync(final String nextPageLink) {
return listByResourceGroupNextWithServiceResponseAsync(nextPageLink)
.map(new Func1>, Page>() {
@Override
public Page call(ServiceResponse> response) {
return response.getBody();
}
});
}
/**
* Gets information about the Batch accounts associated within the specified resource group.
*
* @param nextPageLink The NextLink from the previous successful call to List operation.
* @return the observable to the PagedList<BatchAccountInner> object
*/
public Observable>> listByResourceGroupNextWithServiceResponseAsync(final String nextPageLink) {
return listByResourceGroupNextSinglePageAsync(nextPageLink)
.concatMap(new Func1>, Observable>>>() {
@Override
public Observable>> call(ServiceResponse> page) {
String nextPageLink = page.getBody().getNextPageLink();
if (nextPageLink == null) {
return Observable.just(page);
}
return Observable.just(page).concatWith(listByResourceGroupNextWithServiceResponseAsync(nextPageLink));
}
});
}
/**
* Gets information about the Batch accounts associated within the specified resource group.
*
ServiceResponse> * @param nextPageLink The NextLink from the previous successful call to List operation.
* @return the PagedList<BatchAccountInner> object wrapped in {@link ServiceResponse} if successful.
*/
public Observable>> listByResourceGroupNextSinglePageAsync(final String nextPageLink) {
if (nextPageLink == null) {
throw new IllegalArgumentException("Parameter nextPageLink is required and cannot be null.");
}
return service.listByResourceGroupNext(nextPageLink, this.client.acceptLanguage(), this.client.userAgent())
.flatMap(new Func1, Observable>>>() {
@Override
public Observable