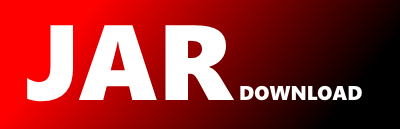
com.microsoft.azure.management.batch.implementation.BatchManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-mgmt-batch Show documentation
Show all versions of azure-mgmt-batch Show documentation
This package contains Microsoft Azure Batch Account Management SDK. This package has been deprecated. A replacement package com.azure.resourcemanager:azure-resourcemanager-batch is available as of 31-March-2022. We strongly encourage you to upgrade to continue receiving updates. See Migration Guide https://aka.ms/java-track2-migration-guide for guidance on upgrading. Refer to our deprecation policy https://azure.github.io/azure-sdk/policies_support.html for more details.
package com.microsoft.azure.management.batch.implementation;
import com.microsoft.azure.RestClient;
import com.microsoft.azure.credentials.AzureTokenCredentials;
import com.microsoft.azure.management.batch.BatchAccounts;
import com.microsoft.azure.management.resources.fluentcore.arm.AzureConfigurable;
import com.microsoft.azure.management.resources.fluentcore.arm.implementation.AzureConfigurableImpl;
import com.microsoft.azure.management.resources.fluentcore.arm.implementation.Manager;
import com.microsoft.azure.management.storage.implementation.StorageManager;
/**
* Entry point to Azure Batch Account resource management.
*/
public class BatchManager extends Manager {
private BatchAccounts batchAccounts;
private StorageManager storageManager;
protected BatchManager(RestClient restClient, String subscriptionId) {
super(
restClient,
subscriptionId,
new BatchManagementClientImpl(restClient).withSubscriptionId(subscriptionId));
storageManager = StorageManager.authenticate(restClient, subscriptionId);
}
/**
* Get a Configurable instance that can be used to create BatchManager with optional configuration.
*
* @return Configurable
*/
public static Configurable configure() {
return new BatchManager.ConfigurableImpl();
}
/**
* Creates an instance of BatchManager that exposes Compute resource management API entry points.
*
* @param credentials the credentials to use
* @param subscriptionId the subscription
* @return the BatchManager
*/
public static BatchManager authenticate(AzureTokenCredentials credentials, String subscriptionId) {
return new BatchManager(credentials.getEnvironment().newRestClientBuilder()
.withCredentials(credentials)
.build(), subscriptionId);
}
/**
* Creates an instance of BatchManager that exposes Compute resource management API entry points.
*
* @param restClient the RestClient to be used for API calls.
* @param subscriptionId the subscription
* @return the BatchManager
*/
public static BatchManager authenticate(RestClient restClient, String subscriptionId) {
return new BatchManager(restClient, subscriptionId);
}
/**
* The interface allowing configurations to be set.
*/
public interface Configurable extends AzureConfigurable {
/**
* Creates an instance of BatchManager that exposes Compute resource management API entry points.
*
* @param credentials the credentials to use
* @param subscriptionId the subscription
* @return the BatchManager
*/
BatchManager authenticate(AzureTokenCredentials credentials, String subscriptionId);
}
/**
* The implementation for Configurable interface.
*/
private static final class ConfigurableImpl extends AzureConfigurableImpl implements Configurable {
@Override
public BatchManager authenticate(AzureTokenCredentials credentials, String subscriptionId) {
return BatchManager.authenticate(buildRestClient(credentials), subscriptionId);
}
}
/**
* @return the batch account management API entry point
*/
public BatchAccounts batchAccounts() {
if (batchAccounts == null) {
batchAccounts = new BatchAccountsImpl(
super.innerManagementClient.batchAccounts(),
this,
super.innerManagementClient.applications(),
super.innerManagementClient.applicationPackages(),
super.innerManagementClient.locations(),
this.storageManager);
}
return batchAccounts;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy