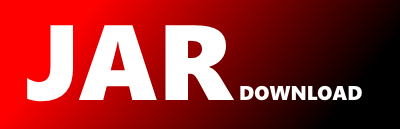
com.microsoft.azure.management.cognitiveservices.CheckSkuAvailabilityResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-mgmt-cognitiveservices Show documentation
Show all versions of azure-mgmt-cognitiveservices Show documentation
This package contains Microsoft CognitiveServices Management SDK.
/**
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for
* license information.
*
* Code generated by Microsoft (R) AutoRest Code Generator.
*/
package com.microsoft.azure.management.cognitiveservices;
import com.fasterxml.jackson.annotation.JsonProperty;
/**
* Check SKU availability result.
*/
public class CheckSkuAvailabilityResult {
/**
* The Kind of the resource. Possible values include: 'Academic',
* 'Bing.Autosuggest', 'Bing.Search', 'Bing.Speech', 'Bing.SpellCheck',
* 'ComputerVision', 'ContentModerator', 'CustomSpeech', 'Emotion', 'Face',
* 'LUIS', 'Recommendations', 'SpeakerRecognition', 'Speech',
* 'SpeechTranslation', 'TextAnalytics', 'TextTranslation', 'WebLM'.
*/
@JsonProperty(value = "kind")
private Kind kind;
/**
* The Type of the resource.
*/
@JsonProperty(value = "type")
private String type;
/**
* The SKU of Cognitive Services account. Possible values include: 'F0',
* 'P0', 'P1', 'P2', 'S0', 'S1', 'S2', 'S3', 'S4', 'S5', 'S6'.
*/
@JsonProperty(value = "skuName")
private SkuName skuName;
/**
* Indicates the given SKU is available or not.
*/
@JsonProperty(value = "skuAvailable")
private Boolean skuAvailable;
/**
* Reason why the SKU is not available.
*/
@JsonProperty(value = "reason")
private String reason;
/**
* Additional error message.
*/
@JsonProperty(value = "message")
private String message;
/**
* Get the kind value.
*
* @return the kind value
*/
public Kind kind() {
return this.kind;
}
/**
* Set the kind value.
*
* @param kind the kind value to set
* @return the CheckSkuAvailabilityResult object itself.
*/
public CheckSkuAvailabilityResult withKind(Kind kind) {
this.kind = kind;
return this;
}
/**
* Get the type value.
*
* @return the type value
*/
public String type() {
return this.type;
}
/**
* Set the type value.
*
* @param type the type value to set
* @return the CheckSkuAvailabilityResult object itself.
*/
public CheckSkuAvailabilityResult withType(String type) {
this.type = type;
return this;
}
/**
* Get the skuName value.
*
* @return the skuName value
*/
public SkuName skuName() {
return this.skuName;
}
/**
* Set the skuName value.
*
* @param skuName the skuName value to set
* @return the CheckSkuAvailabilityResult object itself.
*/
public CheckSkuAvailabilityResult withSkuName(SkuName skuName) {
this.skuName = skuName;
return this;
}
/**
* Get the skuAvailable value.
*
* @return the skuAvailable value
*/
public Boolean skuAvailable() {
return this.skuAvailable;
}
/**
* Set the skuAvailable value.
*
* @param skuAvailable the skuAvailable value to set
* @return the CheckSkuAvailabilityResult object itself.
*/
public CheckSkuAvailabilityResult withSkuAvailable(Boolean skuAvailable) {
this.skuAvailable = skuAvailable;
return this;
}
/**
* Get the reason value.
*
* @return the reason value
*/
public String reason() {
return this.reason;
}
/**
* Set the reason value.
*
* @param reason the reason value to set
* @return the CheckSkuAvailabilityResult object itself.
*/
public CheckSkuAvailabilityResult withReason(String reason) {
this.reason = reason;
return this;
}
/**
* Get the message value.
*
* @return the message value
*/
public String message() {
return this.message;
}
/**
* Set the message value.
*
* @param message the message value to set
* @return the CheckSkuAvailabilityResult object itself.
*/
public CheckSkuAvailabilityResult withMessage(String message) {
this.message = message;
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy