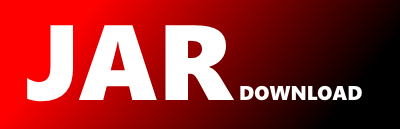
com.microsoft.azure.management.cosmosdb.DatabaseAccountUpdateParameters Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-mgmt-cosmosdb Show documentation
Show all versions of azure-mgmt-cosmosdb Show documentation
This package contains Microsoft Azure CosmosDB SDK. A new set of management libraries are now Generally Available. For documentation on how to use the new libraries, please see https://aka.ms/azsdk/java/mgmt
/**
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for
* license information.
*
* Code generated by Microsoft (R) AutoRest Code Generator.
*/
package com.microsoft.azure.management.cosmosdb;
import java.util.Map;
import java.util.List;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.microsoft.rest.serializer.JsonFlatten;
/**
* Parameters for patching Azure Cosmos DB database account properties.
*/
@JsonFlatten
public class DatabaseAccountUpdateParameters {
/**
* The tags property.
*/
@JsonProperty(value = "tags")
private Map tags;
/**
* The location of the resource group to which the resource belongs.
*/
@JsonProperty(value = "location")
private String location;
/**
* The consistency policy for the Cosmos DB account.
*/
@JsonProperty(value = "properties.consistencyPolicy")
private ConsistencyPolicy consistencyPolicy;
/**
* An array that contains the georeplication locations enabled for the
* Cosmos DB account.
*/
@JsonProperty(value = "properties.locations")
private List locations;
/**
* Cosmos DB Firewall Support: This value specifies the set of IP addresses
* or IP address ranges in CIDR form to be included as the allowed list of
* client IPs for a given database account. IP addresses/ranges must be
* comma separated and must not contain any spaces.
*/
@JsonProperty(value = "properties.ipRangeFilter")
private String ipRangeFilter;
/**
* Flag to indicate whether to enable/disable Virtual Network ACL rules.
*/
@JsonProperty(value = "properties.isVirtualNetworkFilterEnabled")
private Boolean isVirtualNetworkFilterEnabled;
/**
* Enables automatic failover of the write region in the rare event that
* the region is unavailable due to an outage. Automatic failover will
* result in a new write region for the account and is chosen based on the
* failover priorities configured for the account.
*/
@JsonProperty(value = "properties.enableAutomaticFailover")
private Boolean enableAutomaticFailover;
/**
* List of Cosmos DB capabilities for the account.
*/
@JsonProperty(value = "properties.capabilities")
private List capabilities;
/**
* List of Virtual Network ACL rules configured for the Cosmos DB account.
*/
@JsonProperty(value = "properties.virtualNetworkRules")
private List virtualNetworkRules;
/**
* Enables the account to write in multiple locations.
*/
@JsonProperty(value = "properties.enableMultipleWriteLocations")
private Boolean enableMultipleWriteLocations;
/**
* Enables the cassandra connector on the Cosmos DB C* account.
*/
@JsonProperty(value = "properties.enableCassandraConnector")
private Boolean enableCassandraConnector;
/**
* The cassandra connector offer type for the Cosmos DB database C*
* account. Possible values include: 'Small'.
*/
@JsonProperty(value = "properties.connectorOffer")
private ConnectorOffer connectorOffer;
/**
* Disable write operations on metadata resources (databases, containers,
* throughput) via account keys.
*/
@JsonProperty(value = "properties.disableKeyBasedMetadataWriteAccess")
private Boolean disableKeyBasedMetadataWriteAccess;
/**
* Get the tags value.
*
* @return the tags value
*/
public Map tags() {
return this.tags;
}
/**
* Set the tags value.
*
* @param tags the tags value to set
* @return the DatabaseAccountUpdateParameters object itself.
*/
public DatabaseAccountUpdateParameters withTags(Map tags) {
this.tags = tags;
return this;
}
/**
* Get the location of the resource group to which the resource belongs.
*
* @return the location value
*/
public String location() {
return this.location;
}
/**
* Set the location of the resource group to which the resource belongs.
*
* @param location the location value to set
* @return the DatabaseAccountUpdateParameters object itself.
*/
public DatabaseAccountUpdateParameters withLocation(String location) {
this.location = location;
return this;
}
/**
* Get the consistency policy for the Cosmos DB account.
*
* @return the consistencyPolicy value
*/
public ConsistencyPolicy consistencyPolicy() {
return this.consistencyPolicy;
}
/**
* Set the consistency policy for the Cosmos DB account.
*
* @param consistencyPolicy the consistencyPolicy value to set
* @return the DatabaseAccountUpdateParameters object itself.
*/
public DatabaseAccountUpdateParameters withConsistencyPolicy(ConsistencyPolicy consistencyPolicy) {
this.consistencyPolicy = consistencyPolicy;
return this;
}
/**
* Get an array that contains the georeplication locations enabled for the Cosmos DB account.
*
* @return the locations value
*/
public List locations() {
return this.locations;
}
/**
* Set an array that contains the georeplication locations enabled for the Cosmos DB account.
*
* @param locations the locations value to set
* @return the DatabaseAccountUpdateParameters object itself.
*/
public DatabaseAccountUpdateParameters withLocations(List locations) {
this.locations = locations;
return this;
}
/**
* Get cosmos DB Firewall Support: This value specifies the set of IP addresses or IP address ranges in CIDR form to be included as the allowed list of client IPs for a given database account. IP addresses/ranges must be comma separated and must not contain any spaces.
*
* @return the ipRangeFilter value
*/
public String ipRangeFilter() {
return this.ipRangeFilter;
}
/**
* Set cosmos DB Firewall Support: This value specifies the set of IP addresses or IP address ranges in CIDR form to be included as the allowed list of client IPs for a given database account. IP addresses/ranges must be comma separated and must not contain any spaces.
*
* @param ipRangeFilter the ipRangeFilter value to set
* @return the DatabaseAccountUpdateParameters object itself.
*/
public DatabaseAccountUpdateParameters withIpRangeFilter(String ipRangeFilter) {
this.ipRangeFilter = ipRangeFilter;
return this;
}
/**
* Get flag to indicate whether to enable/disable Virtual Network ACL rules.
*
* @return the isVirtualNetworkFilterEnabled value
*/
public Boolean isVirtualNetworkFilterEnabled() {
return this.isVirtualNetworkFilterEnabled;
}
/**
* Set flag to indicate whether to enable/disable Virtual Network ACL rules.
*
* @param isVirtualNetworkFilterEnabled the isVirtualNetworkFilterEnabled value to set
* @return the DatabaseAccountUpdateParameters object itself.
*/
public DatabaseAccountUpdateParameters withIsVirtualNetworkFilterEnabled(Boolean isVirtualNetworkFilterEnabled) {
this.isVirtualNetworkFilterEnabled = isVirtualNetworkFilterEnabled;
return this;
}
/**
* Get enables automatic failover of the write region in the rare event that the region is unavailable due to an outage. Automatic failover will result in a new write region for the account and is chosen based on the failover priorities configured for the account.
*
* @return the enableAutomaticFailover value
*/
public Boolean enableAutomaticFailover() {
return this.enableAutomaticFailover;
}
/**
* Set enables automatic failover of the write region in the rare event that the region is unavailable due to an outage. Automatic failover will result in a new write region for the account and is chosen based on the failover priorities configured for the account.
*
* @param enableAutomaticFailover the enableAutomaticFailover value to set
* @return the DatabaseAccountUpdateParameters object itself.
*/
public DatabaseAccountUpdateParameters withEnableAutomaticFailover(Boolean enableAutomaticFailover) {
this.enableAutomaticFailover = enableAutomaticFailover;
return this;
}
/**
* Get list of Cosmos DB capabilities for the account.
*
* @return the capabilities value
*/
public List capabilities() {
return this.capabilities;
}
/**
* Set list of Cosmos DB capabilities for the account.
*
* @param capabilities the capabilities value to set
* @return the DatabaseAccountUpdateParameters object itself.
*/
public DatabaseAccountUpdateParameters withCapabilities(List capabilities) {
this.capabilities = capabilities;
return this;
}
/**
* Get list of Virtual Network ACL rules configured for the Cosmos DB account.
*
* @return the virtualNetworkRules value
*/
public List virtualNetworkRules() {
return this.virtualNetworkRules;
}
/**
* Set list of Virtual Network ACL rules configured for the Cosmos DB account.
*
* @param virtualNetworkRules the virtualNetworkRules value to set
* @return the DatabaseAccountUpdateParameters object itself.
*/
public DatabaseAccountUpdateParameters withVirtualNetworkRules(List virtualNetworkRules) {
this.virtualNetworkRules = virtualNetworkRules;
return this;
}
/**
* Get enables the account to write in multiple locations.
*
* @return the enableMultipleWriteLocations value
*/
public Boolean enableMultipleWriteLocations() {
return this.enableMultipleWriteLocations;
}
/**
* Set enables the account to write in multiple locations.
*
* @param enableMultipleWriteLocations the enableMultipleWriteLocations value to set
* @return the DatabaseAccountUpdateParameters object itself.
*/
public DatabaseAccountUpdateParameters withEnableMultipleWriteLocations(Boolean enableMultipleWriteLocations) {
this.enableMultipleWriteLocations = enableMultipleWriteLocations;
return this;
}
/**
* Get enables the cassandra connector on the Cosmos DB C* account.
*
* @return the enableCassandraConnector value
*/
public Boolean enableCassandraConnector() {
return this.enableCassandraConnector;
}
/**
* Set enables the cassandra connector on the Cosmos DB C* account.
*
* @param enableCassandraConnector the enableCassandraConnector value to set
* @return the DatabaseAccountUpdateParameters object itself.
*/
public DatabaseAccountUpdateParameters withEnableCassandraConnector(Boolean enableCassandraConnector) {
this.enableCassandraConnector = enableCassandraConnector;
return this;
}
/**
* Get the cassandra connector offer type for the Cosmos DB database C* account. Possible values include: 'Small'.
*
* @return the connectorOffer value
*/
public ConnectorOffer connectorOffer() {
return this.connectorOffer;
}
/**
* Set the cassandra connector offer type for the Cosmos DB database C* account. Possible values include: 'Small'.
*
* @param connectorOffer the connectorOffer value to set
* @return the DatabaseAccountUpdateParameters object itself.
*/
public DatabaseAccountUpdateParameters withConnectorOffer(ConnectorOffer connectorOffer) {
this.connectorOffer = connectorOffer;
return this;
}
/**
* Get disable write operations on metadata resources (databases, containers, throughput) via account keys.
*
* @return the disableKeyBasedMetadataWriteAccess value
*/
public Boolean disableKeyBasedMetadataWriteAccess() {
return this.disableKeyBasedMetadataWriteAccess;
}
/**
* Set disable write operations on metadata resources (databases, containers, throughput) via account keys.
*
* @param disableKeyBasedMetadataWriteAccess the disableKeyBasedMetadataWriteAccess value to set
* @return the DatabaseAccountUpdateParameters object itself.
*/
public DatabaseAccountUpdateParameters withDisableKeyBasedMetadataWriteAccess(Boolean disableKeyBasedMetadataWriteAccess) {
this.disableKeyBasedMetadataWriteAccess = disableKeyBasedMetadataWriteAccess;
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy