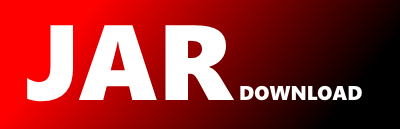
com.microsoft.azure.management.cosmosdb.PrivateEndpointConnection Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-mgmt-cosmosdb Show documentation
Show all versions of azure-mgmt-cosmosdb Show documentation
This package contains Microsoft Azure CosmosDB SDK. A new set of management libraries are now Generally Available. For documentation on how to use the new libraries, please see https://aka.ms/azsdk/java/mgmt
/**
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for
* license information.
*/
package com.microsoft.azure.management.cosmosdb;
import com.microsoft.azure.management.apigeneration.Beta;
import com.microsoft.azure.management.apigeneration.Fluent;
import com.microsoft.azure.management.cosmosdb.implementation.PrivateEndpointConnectionInner;
import com.microsoft.azure.management.resources.fluentcore.arm.models.ExternalChildResource;
import com.microsoft.azure.management.resources.fluentcore.model.Attachable;
import com.microsoft.azure.management.resources.fluentcore.model.HasInner;
import com.microsoft.azure.management.resources.fluentcore.model.Settable;
/**
* A private endpoint connection.
*/
@Fluent
@Beta(Beta.SinceVersion.V1_28_0)
public interface PrivateEndpointConnection extends
HasInner,
ExternalChildResource {
/**
* Get private endpoint which the connection belongs to.
*
* @return the privateEndpoint value
*/
PrivateEndpointProperty privateEndpoint();
/**
* Get connection State of the Private Endpoint Connection.
*
* @return the privateLinkServiceConnectionState value
*/
PrivateLinkServiceConnectionStateProperty privateLinkServiceConnectionState();
/**
* Grouping of private endpoint connection definition stages as a port of cosmos db account definition.
*/
interface DefinitionStages {
/**
* The first stage of a private endpoint connection definition.
* @param the stage of the parent definition to return to after attaching this definition
*/
interface Blank extends
WithState {
}
/**
* The final stage of the private endpoint connection definition.
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithAttach extends
Attachable.InDefinition,
WithState {
}
/**
* The stage of the private endpoint connection definition allowing to set state.
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithState {
/**
* Specifies state property.
* @param property a private link service connection state property
* @return the next stage of definition
*/
WithAttach withStateProperty(PrivateLinkServiceConnectionStateProperty property);
/**
* Specifies status of state property.
* @param status the status of state property
* @return the next stage of definition
*/
WithAttach withStatus(String status);
/**
* Specifies description of state property.
* @param description the description of state property
* @return the next stage of definition
*/
WithAttach withDescription(String description);
}
}
/**
* The entirety of a private endpoint connection definition as a part of parent definition.
* @param the stage of the parent definition to return to after attaching this definition
*/
interface Definition extends
DefinitionStages.Blank,
DefinitionStages.WithState,
DefinitionStages.WithAttach {
}
/**
* Grouping of private endpoint connection definition stages as part of parent cosmos db account update.
*/
interface UpdateDefinitionStages {
/**
* The first stage of a private endpoint connection definition.
* @param the stage of the parent update to return to after attaching this definition
*/
interface Blank extends
WithState {
}
/**
* The final stage of the private endpoint connection definition.
* @param the stage of the parent update to return to after attaching this definition
*/
interface WithAttach extends
Attachable.InUpdate,
WithState {
}
/**
* The stage of the private endpoint connection definition allowing to set state.
* @param the stage of the parent update to return to after attaching this definition
*/
interface WithState {
/**
* Specifies state property.
* @param property a private link service connection state property
* @return the next stage of definition
*/
WithAttach withStateProperty(PrivateLinkServiceConnectionStateProperty property);
/**
* Specifies status of state property.
* @param status the status of state property
* @return the next stage of definition
*/
WithAttach withStatus(String status);
/**
* Specifies description of state property.
* @param description the description of state property
* @return the next stage of definition
*/
WithAttach withDescription(String description);
}
}
/**
* The entirety of a private endpoint connection definition definition as a part of parent update.
* @param the stage of the parent update to return to after attaching this definition
*/
interface UpdateDefinition extends
UpdateDefinitionStages.Blank,
UpdateDefinitionStages.WithState,
UpdateDefinitionStages.WithAttach {
}
/**
* Grouping of private endpoint connection update stages.
*/
interface UpdateStages {
/**
* The stage of the private endpoint connection update allowing to specify state.
*/
interface WithState {
/**
* Specifies state property.
* @param property a private link service connection state property
* @return the next stage of update
*/
Update withStateProperty(PrivateLinkServiceConnectionStateProperty property);
/**
* Specifies status of state property.
* @param status the status of state property
* @return the next stage of update
*/
Update withStatus(String status);
/**
* Specifies description of state property.
* @param description the description of state property
* @return the next stage of update
*/
Update withDescription(String description);
}
}
/**
* The entirety of private endpoint connection update as a part of parent virtual machine update.
*/
interface Update extends
Settable,
UpdateStages.WithState {
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy