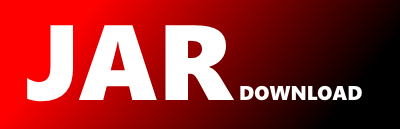
com.microsoft.azure.management.cosmosdb.implementation.DatabaseAccountsInner Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-mgmt-cosmosdb Show documentation
Show all versions of azure-mgmt-cosmosdb Show documentation
This package contains Microsoft Azure CosmosDB SDK. A new set of management libraries are now Generally Available. For documentation on how to use the new libraries, please see https://aka.ms/azsdk/java/mgmt
/**
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for
* license information.
*
* Code generated by Microsoft (R) AutoRest Code Generator.
*/
package com.microsoft.azure.management.cosmosdb.implementation;
import com.microsoft.azure.management.resources.fluentcore.collection.InnerSupportsGet;
import com.microsoft.azure.management.resources.fluentcore.collection.InnerSupportsDelete;
import com.microsoft.azure.management.resources.fluentcore.collection.InnerSupportsListing;
import retrofit2.Retrofit;
import com.google.common.reflect.TypeToken;
import com.microsoft.azure.CloudException;
import com.microsoft.azure.management.cosmosdb.DatabaseAccountCreateUpdateParameters;
import com.microsoft.azure.management.cosmosdb.DatabaseAccountRegenerateKeyParameters;
import com.microsoft.azure.management.cosmosdb.DatabaseAccountUpdateParameters;
import com.microsoft.azure.management.cosmosdb.ErrorResponseException;
import com.microsoft.azure.management.cosmosdb.FailoverPolicies;
import com.microsoft.azure.management.cosmosdb.FailoverPolicy;
import com.microsoft.azure.management.cosmosdb.KeyKind;
import com.microsoft.azure.management.cosmosdb.RegionForOnlineOffline;
import com.microsoft.azure.Page;
import com.microsoft.azure.PagedList;
import com.microsoft.rest.ServiceCallback;
import com.microsoft.rest.ServiceFuture;
import com.microsoft.rest.ServiceResponse;
import com.microsoft.rest.Validator;
import java.io.IOException;
import java.util.List;
import okhttp3.ResponseBody;
import retrofit2.http.Body;
import retrofit2.http.GET;
import retrofit2.http.HEAD;
import retrofit2.http.Header;
import retrofit2.http.Headers;
import retrofit2.http.HTTP;
import retrofit2.http.PATCH;
import retrofit2.http.Path;
import retrofit2.http.POST;
import retrofit2.http.PUT;
import retrofit2.http.Query;
import retrofit2.Response;
import rx.functions.Func1;
import rx.Observable;
/**
* An instance of this class provides access to all the operations defined
* in DatabaseAccounts.
*/
public class DatabaseAccountsInner implements InnerSupportsGet, InnerSupportsDelete, InnerSupportsListing {
/** The Retrofit service to perform REST calls. */
private DatabaseAccountsService service;
/** The service client containing this operation class. */
private CosmosDBImpl client;
/**
* Initializes an instance of DatabaseAccountsInner.
*
* @param retrofit the Retrofit instance built from a Retrofit Builder.
* @param client the instance of the service client containing this operation class.
*/
public DatabaseAccountsInner(Retrofit retrofit, CosmosDBImpl client) {
this.service = retrofit.create(DatabaseAccountsService.class);
this.client = client;
}
/**
* The interface defining all the services for DatabaseAccounts to be
* used by Retrofit to perform actually REST calls.
*/
interface DatabaseAccountsService {
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.DatabaseAccounts getByResourceGroup" })
@GET("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}")
Observable> getByResourceGroup(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.DatabaseAccounts update" })
@PATCH("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}")
Observable> update(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Query("api-version") String apiVersion, @Body DatabaseAccountUpdateParameters updateParameters, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.DatabaseAccounts beginUpdate" })
@PATCH("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}")
Observable> beginUpdate(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Query("api-version") String apiVersion, @Body DatabaseAccountUpdateParameters updateParameters, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.DatabaseAccounts createOrUpdate" })
@PUT("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}")
Observable> createOrUpdate(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Query("api-version") String apiVersion, @Body DatabaseAccountCreateUpdateParameters createUpdateParameters, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.DatabaseAccounts beginCreateOrUpdate" })
@PUT("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}")
Observable> beginCreateOrUpdate(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Query("api-version") String apiVersion, @Body DatabaseAccountCreateUpdateParameters createUpdateParameters, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.DatabaseAccounts delete" })
@HTTP(path = "subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}", method = "DELETE", hasBody = true)
Observable> delete(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.DatabaseAccounts beginDelete" })
@HTTP(path = "subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}", method = "DELETE", hasBody = true)
Observable> beginDelete(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.DatabaseAccounts failoverPriorityChange" })
@POST("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/failoverPriorityChange")
Observable> failoverPriorityChange(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Body FailoverPolicies failoverParameters, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.DatabaseAccounts beginFailoverPriorityChange" })
@POST("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/failoverPriorityChange")
Observable> beginFailoverPriorityChange(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Body FailoverPolicies failoverParameters, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.DatabaseAccounts list" })
@GET("subscriptions/{subscriptionId}/providers/Microsoft.DocumentDB/databaseAccounts")
Observable> list(@Path("subscriptionId") String subscriptionId, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.DatabaseAccounts listByResourceGroup" })
@GET("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts")
Observable> listByResourceGroup(@Path("resourceGroupName") String resourceGroupName, @Path("subscriptionId") String subscriptionId, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.DatabaseAccounts listKeys" })
@POST("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/listKeys")
Observable> listKeys(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.DatabaseAccounts listConnectionStrings" })
@POST("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/listConnectionStrings")
Observable> listConnectionStrings(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.DatabaseAccounts offlineRegion" })
@POST("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/offlineRegion")
Observable> offlineRegion(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Body RegionForOnlineOffline regionParameterForOffline, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.DatabaseAccounts beginOfflineRegion" })
@POST("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/offlineRegion")
Observable> beginOfflineRegion(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Body RegionForOnlineOffline regionParameterForOffline, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.DatabaseAccounts onlineRegion" })
@POST("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/onlineRegion")
Observable> onlineRegion(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Body RegionForOnlineOffline regionParameterForOnline, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.DatabaseAccounts beginOnlineRegion" })
@POST("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/onlineRegion")
Observable> beginOnlineRegion(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Body RegionForOnlineOffline regionParameterForOnline, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.DatabaseAccounts getReadOnlyKeys" })
@GET("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/readonlykeys")
Observable> getReadOnlyKeys(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.DatabaseAccounts listReadOnlyKeys" })
@POST("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/readonlykeys")
Observable> listReadOnlyKeys(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.DatabaseAccounts regenerateKey" })
@POST("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/regenerateKey")
Observable> regenerateKey(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Body DatabaseAccountRegenerateKeyParameters keyToRegenerate, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.DatabaseAccounts beginRegenerateKey" })
@POST("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/regenerateKey")
Observable> beginRegenerateKey(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Body DatabaseAccountRegenerateKeyParameters keyToRegenerate, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.DatabaseAccounts checkNameExists" })
@HEAD("providers/Microsoft.DocumentDB/databaseAccountNames/{accountName}")
Observable> checkNameExists(@Path("accountName") String accountName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.DatabaseAccounts listMetrics" })
@GET("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/metrics")
Observable> listMetrics(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Query("api-version") String apiVersion, @Query("$filter") String filter, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.DatabaseAccounts listUsages" })
@GET("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/usages")
Observable> listUsages(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Query("api-version") String apiVersion, @Query("$filter") String filter, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.DatabaseAccounts listMetricDefinitions" })
@GET("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/metricDefinitions")
Observable> listMetricDefinitions(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
}
/**
* Retrieves the properties of an existing Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the DatabaseAccountGetResultsInner object if successful.
*/
public DatabaseAccountGetResultsInner getByResourceGroup(String resourceGroupName, String accountName) {
return getByResourceGroupWithServiceResponseAsync(resourceGroupName, accountName).toBlocking().single().body();
}
/**
* Retrieves the properties of an existing Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture getByResourceGroupAsync(String resourceGroupName, String accountName, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(getByResourceGroupWithServiceResponseAsync(resourceGroupName, accountName), serviceCallback);
}
/**
* Retrieves the properties of an existing Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the DatabaseAccountGetResultsInner object
*/
public Observable getByResourceGroupAsync(String resourceGroupName, String accountName) {
return getByResourceGroupWithServiceResponseAsync(resourceGroupName, accountName).map(new Func1, DatabaseAccountGetResultsInner>() {
@Override
public DatabaseAccountGetResultsInner call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Retrieves the properties of an existing Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the DatabaseAccountGetResultsInner object
*/
public Observable> getByResourceGroupWithServiceResponseAsync(String resourceGroupName, String accountName) {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (accountName == null) {
throw new IllegalArgumentException("Parameter accountName is required and cannot be null.");
}
final String apiVersion = "2019-08-01";
return service.getByResourceGroup(this.client.subscriptionId(), resourceGroupName, accountName, apiVersion, this.client.acceptLanguage(), this.client.userAgent())
.flatMap(new Func1, Observable>>() {
@Override
public Observable> call(Response response) {
try {
ServiceResponse clientResponse = getByResourceGroupDelegate(response);
return Observable.just(clientResponse);
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse getByResourceGroupDelegate(Response response) throws CloudException, IOException, IllegalArgumentException {
return this.client.restClient().responseBuilderFactory().newInstance(this.client.serializerAdapter())
.register(200, new TypeToken() { }.getType())
.registerError(CloudException.class)
.build(response);
}
/**
* Updates the properties of an existing Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param updateParameters The parameters to provide for the current database account.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the DatabaseAccountGetResultsInner object if successful.
*/
public DatabaseAccountGetResultsInner update(String resourceGroupName, String accountName, DatabaseAccountUpdateParameters updateParameters) {
return updateWithServiceResponseAsync(resourceGroupName, accountName, updateParameters).toBlocking().last().body();
}
/**
* Updates the properties of an existing Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param updateParameters The parameters to provide for the current database account.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture updateAsync(String resourceGroupName, String accountName, DatabaseAccountUpdateParameters updateParameters, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(updateWithServiceResponseAsync(resourceGroupName, accountName, updateParameters), serviceCallback);
}
/**
* Updates the properties of an existing Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param updateParameters The parameters to provide for the current database account.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable for the request
*/
public Observable updateAsync(String resourceGroupName, String accountName, DatabaseAccountUpdateParameters updateParameters) {
return updateWithServiceResponseAsync(resourceGroupName, accountName, updateParameters).map(new Func1, DatabaseAccountGetResultsInner>() {
@Override
public DatabaseAccountGetResultsInner call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Updates the properties of an existing Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param updateParameters The parameters to provide for the current database account.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable for the request
*/
public Observable> updateWithServiceResponseAsync(String resourceGroupName, String accountName, DatabaseAccountUpdateParameters updateParameters) {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (accountName == null) {
throw new IllegalArgumentException("Parameter accountName is required and cannot be null.");
}
if (updateParameters == null) {
throw new IllegalArgumentException("Parameter updateParameters is required and cannot be null.");
}
Validator.validate(updateParameters);
final String apiVersion = "2019-08-01";
Observable> observable = service.update(this.client.subscriptionId(), resourceGroupName, accountName, apiVersion, updateParameters, this.client.acceptLanguage(), this.client.userAgent());
return client.getAzureClient().getPutOrPatchResultAsync(observable, new TypeToken() { }.getType());
}
/**
* Updates the properties of an existing Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param updateParameters The parameters to provide for the current database account.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the DatabaseAccountGetResultsInner object if successful.
*/
public DatabaseAccountGetResultsInner beginUpdate(String resourceGroupName, String accountName, DatabaseAccountUpdateParameters updateParameters) {
return beginUpdateWithServiceResponseAsync(resourceGroupName, accountName, updateParameters).toBlocking().single().body();
}
/**
* Updates the properties of an existing Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param updateParameters The parameters to provide for the current database account.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture beginUpdateAsync(String resourceGroupName, String accountName, DatabaseAccountUpdateParameters updateParameters, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(beginUpdateWithServiceResponseAsync(resourceGroupName, accountName, updateParameters), serviceCallback);
}
/**
* Updates the properties of an existing Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param updateParameters The parameters to provide for the current database account.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the DatabaseAccountGetResultsInner object
*/
public Observable beginUpdateAsync(String resourceGroupName, String accountName, DatabaseAccountUpdateParameters updateParameters) {
return beginUpdateWithServiceResponseAsync(resourceGroupName, accountName, updateParameters).map(new Func1, DatabaseAccountGetResultsInner>() {
@Override
public DatabaseAccountGetResultsInner call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Updates the properties of an existing Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param updateParameters The parameters to provide for the current database account.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the DatabaseAccountGetResultsInner object
*/
public Observable> beginUpdateWithServiceResponseAsync(String resourceGroupName, String accountName, DatabaseAccountUpdateParameters updateParameters) {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (accountName == null) {
throw new IllegalArgumentException("Parameter accountName is required and cannot be null.");
}
if (updateParameters == null) {
throw new IllegalArgumentException("Parameter updateParameters is required and cannot be null.");
}
Validator.validate(updateParameters);
final String apiVersion = "2019-08-01";
return service.beginUpdate(this.client.subscriptionId(), resourceGroupName, accountName, apiVersion, updateParameters, this.client.acceptLanguage(), this.client.userAgent())
.flatMap(new Func1, Observable>>() {
@Override
public Observable> call(Response response) {
try {
ServiceResponse clientResponse = beginUpdateDelegate(response);
return Observable.just(clientResponse);
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse beginUpdateDelegate(Response response) throws CloudException, IOException, IllegalArgumentException {
return this.client.restClient().responseBuilderFactory().newInstance(this.client.serializerAdapter())
.register(200, new TypeToken() { }.getType())
.registerError(CloudException.class)
.build(response);
}
/**
* Creates or updates an Azure Cosmos DB database account. The "Update" method is preferred when performing updates on an account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param createUpdateParameters The parameters to provide for the current database account.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the DatabaseAccountGetResultsInner object if successful.
*/
public DatabaseAccountGetResultsInner createOrUpdate(String resourceGroupName, String accountName, DatabaseAccountCreateUpdateParameters createUpdateParameters) {
return createOrUpdateWithServiceResponseAsync(resourceGroupName, accountName, createUpdateParameters).toBlocking().last().body();
}
/**
* Creates or updates an Azure Cosmos DB database account. The "Update" method is preferred when performing updates on an account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param createUpdateParameters The parameters to provide for the current database account.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture createOrUpdateAsync(String resourceGroupName, String accountName, DatabaseAccountCreateUpdateParameters createUpdateParameters, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(createOrUpdateWithServiceResponseAsync(resourceGroupName, accountName, createUpdateParameters), serviceCallback);
}
/**
* Creates or updates an Azure Cosmos DB database account. The "Update" method is preferred when performing updates on an account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param createUpdateParameters The parameters to provide for the current database account.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable for the request
*/
public Observable createOrUpdateAsync(String resourceGroupName, String accountName, DatabaseAccountCreateUpdateParameters createUpdateParameters) {
return createOrUpdateWithServiceResponseAsync(resourceGroupName, accountName, createUpdateParameters).map(new Func1, DatabaseAccountGetResultsInner>() {
@Override
public DatabaseAccountGetResultsInner call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Creates or updates an Azure Cosmos DB database account. The "Update" method is preferred when performing updates on an account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param createUpdateParameters The parameters to provide for the current database account.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable for the request
*/
public Observable> createOrUpdateWithServiceResponseAsync(String resourceGroupName, String accountName, DatabaseAccountCreateUpdateParameters createUpdateParameters) {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (accountName == null) {
throw new IllegalArgumentException("Parameter accountName is required and cannot be null.");
}
if (createUpdateParameters == null) {
throw new IllegalArgumentException("Parameter createUpdateParameters is required and cannot be null.");
}
Validator.validate(createUpdateParameters);
final String apiVersion = "2019-08-01";
Observable> observable = service.createOrUpdate(this.client.subscriptionId(), resourceGroupName, accountName, apiVersion, createUpdateParameters, this.client.acceptLanguage(), this.client.userAgent());
return client.getAzureClient().getPutOrPatchResultAsync(observable, new TypeToken() { }.getType());
}
/**
* Creates or updates an Azure Cosmos DB database account. The "Update" method is preferred when performing updates on an account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param createUpdateParameters The parameters to provide for the current database account.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the DatabaseAccountGetResultsInner object if successful.
*/
public DatabaseAccountGetResultsInner beginCreateOrUpdate(String resourceGroupName, String accountName, DatabaseAccountCreateUpdateParameters createUpdateParameters) {
return beginCreateOrUpdateWithServiceResponseAsync(resourceGroupName, accountName, createUpdateParameters).toBlocking().single().body();
}
/**
* Creates or updates an Azure Cosmos DB database account. The "Update" method is preferred when performing updates on an account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param createUpdateParameters The parameters to provide for the current database account.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture beginCreateOrUpdateAsync(String resourceGroupName, String accountName, DatabaseAccountCreateUpdateParameters createUpdateParameters, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(beginCreateOrUpdateWithServiceResponseAsync(resourceGroupName, accountName, createUpdateParameters), serviceCallback);
}
/**
* Creates or updates an Azure Cosmos DB database account. The "Update" method is preferred when performing updates on an account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param createUpdateParameters The parameters to provide for the current database account.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the DatabaseAccountGetResultsInner object
*/
public Observable beginCreateOrUpdateAsync(String resourceGroupName, String accountName, DatabaseAccountCreateUpdateParameters createUpdateParameters) {
return beginCreateOrUpdateWithServiceResponseAsync(resourceGroupName, accountName, createUpdateParameters).map(new Func1, DatabaseAccountGetResultsInner>() {
@Override
public DatabaseAccountGetResultsInner call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Creates or updates an Azure Cosmos DB database account. The "Update" method is preferred when performing updates on an account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param createUpdateParameters The parameters to provide for the current database account.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the DatabaseAccountGetResultsInner object
*/
public Observable> beginCreateOrUpdateWithServiceResponseAsync(String resourceGroupName, String accountName, DatabaseAccountCreateUpdateParameters createUpdateParameters) {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (accountName == null) {
throw new IllegalArgumentException("Parameter accountName is required and cannot be null.");
}
if (createUpdateParameters == null) {
throw new IllegalArgumentException("Parameter createUpdateParameters is required and cannot be null.");
}
Validator.validate(createUpdateParameters);
final String apiVersion = "2019-08-01";
return service.beginCreateOrUpdate(this.client.subscriptionId(), resourceGroupName, accountName, apiVersion, createUpdateParameters, this.client.acceptLanguage(), this.client.userAgent())
.flatMap(new Func1, Observable>>() {
@Override
public Observable> call(Response response) {
try {
ServiceResponse clientResponse = beginCreateOrUpdateDelegate(response);
return Observable.just(clientResponse);
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse beginCreateOrUpdateDelegate(Response response) throws CloudException, IOException, IllegalArgumentException {
return this.client.restClient().responseBuilderFactory().newInstance(this.client.serializerAdapter())
.register(200, new TypeToken() { }.getType())
.registerError(CloudException.class)
.build(response);
}
/**
* Deletes an existing Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
*/
public void delete(String resourceGroupName, String accountName) {
deleteWithServiceResponseAsync(resourceGroupName, accountName).toBlocking().last().body();
}
/**
* Deletes an existing Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture deleteAsync(String resourceGroupName, String accountName, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(deleteWithServiceResponseAsync(resourceGroupName, accountName), serviceCallback);
}
/**
* Deletes an existing Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable for the request
*/
public Observable deleteAsync(String resourceGroupName, String accountName) {
return deleteWithServiceResponseAsync(resourceGroupName, accountName).map(new Func1, Void>() {
@Override
public Void call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Deletes an existing Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable for the request
*/
public Observable> deleteWithServiceResponseAsync(String resourceGroupName, String accountName) {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (accountName == null) {
throw new IllegalArgumentException("Parameter accountName is required and cannot be null.");
}
final String apiVersion = "2019-08-01";
Observable> observable = service.delete(this.client.subscriptionId(), resourceGroupName, accountName, apiVersion, this.client.acceptLanguage(), this.client.userAgent());
return client.getAzureClient().getPostOrDeleteResultAsync(observable, new TypeToken() { }.getType());
}
/**
* Deletes an existing Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
*/
public void beginDelete(String resourceGroupName, String accountName) {
beginDeleteWithServiceResponseAsync(resourceGroupName, accountName).toBlocking().single().body();
}
/**
* Deletes an existing Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture beginDeleteAsync(String resourceGroupName, String accountName, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(beginDeleteWithServiceResponseAsync(resourceGroupName, accountName), serviceCallback);
}
/**
* Deletes an existing Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceResponse} object if successful.
*/
public Observable beginDeleteAsync(String resourceGroupName, String accountName) {
return beginDeleteWithServiceResponseAsync(resourceGroupName, accountName).map(new Func1, Void>() {
@Override
public Void call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Deletes an existing Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceResponse} object if successful.
*/
public Observable> beginDeleteWithServiceResponseAsync(String resourceGroupName, String accountName) {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (accountName == null) {
throw new IllegalArgumentException("Parameter accountName is required and cannot be null.");
}
final String apiVersion = "2019-08-01";
return service.beginDelete(this.client.subscriptionId(), resourceGroupName, accountName, apiVersion, this.client.acceptLanguage(), this.client.userAgent())
.flatMap(new Func1, Observable>>() {
@Override
public Observable> call(Response response) {
try {
ServiceResponse clientResponse = beginDeleteDelegate(response);
return Observable.just(clientResponse);
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse beginDeleteDelegate(Response response) throws CloudException, IOException, IllegalArgumentException {
return this.client.restClient().responseBuilderFactory().newInstance(this.client.serializerAdapter())
.register(202, new TypeToken() { }.getType())
.register(204, new TypeToken() { }.getType())
.registerError(CloudException.class)
.build(response);
}
/**
* Changes the failover priority for the Azure Cosmos DB database account. A failover priority of 0 indicates a write region. The maximum value for a failover priority = (total number of regions - 1). Failover priority values must be unique for each of the regions in which the database account exists.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param failoverPolicies List of failover policies.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
*/
public void failoverPriorityChange(String resourceGroupName, String accountName, List failoverPolicies) {
failoverPriorityChangeWithServiceResponseAsync(resourceGroupName, accountName, failoverPolicies).toBlocking().last().body();
}
/**
* Changes the failover priority for the Azure Cosmos DB database account. A failover priority of 0 indicates a write region. The maximum value for a failover priority = (total number of regions - 1). Failover priority values must be unique for each of the regions in which the database account exists.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param failoverPolicies List of failover policies.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture failoverPriorityChangeAsync(String resourceGroupName, String accountName, List failoverPolicies, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(failoverPriorityChangeWithServiceResponseAsync(resourceGroupName, accountName, failoverPolicies), serviceCallback);
}
/**
* Changes the failover priority for the Azure Cosmos DB database account. A failover priority of 0 indicates a write region. The maximum value for a failover priority = (total number of regions - 1). Failover priority values must be unique for each of the regions in which the database account exists.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param failoverPolicies List of failover policies.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable for the request
*/
public Observable failoverPriorityChangeAsync(String resourceGroupName, String accountName, List failoverPolicies) {
return failoverPriorityChangeWithServiceResponseAsync(resourceGroupName, accountName, failoverPolicies).map(new Func1, Void>() {
@Override
public Void call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Changes the failover priority for the Azure Cosmos DB database account. A failover priority of 0 indicates a write region. The maximum value for a failover priority = (total number of regions - 1). Failover priority values must be unique for each of the regions in which the database account exists.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param failoverPolicies List of failover policies.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable for the request
*/
public Observable> failoverPriorityChangeWithServiceResponseAsync(String resourceGroupName, String accountName, List failoverPolicies) {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (accountName == null) {
throw new IllegalArgumentException("Parameter accountName is required and cannot be null.");
}
if (failoverPolicies == null) {
throw new IllegalArgumentException("Parameter failoverPolicies is required and cannot be null.");
}
Validator.validate(failoverPolicies);
final String apiVersion = "2019-08-01";
FailoverPolicies failoverParameters = new FailoverPolicies();
failoverParameters.withFailoverPolicies(failoverPolicies);
Observable> observable = service.failoverPriorityChange(this.client.subscriptionId(), resourceGroupName, accountName, apiVersion, this.client.acceptLanguage(), failoverParameters, this.client.userAgent());
return client.getAzureClient().getPostOrDeleteResultAsync(observable, new TypeToken() { }.getType());
}
/**
* Changes the failover priority for the Azure Cosmos DB database account. A failover priority of 0 indicates a write region. The maximum value for a failover priority = (total number of regions - 1). Failover priority values must be unique for each of the regions in which the database account exists.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param failoverPolicies List of failover policies.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
*/
public void beginFailoverPriorityChange(String resourceGroupName, String accountName, List failoverPolicies) {
beginFailoverPriorityChangeWithServiceResponseAsync(resourceGroupName, accountName, failoverPolicies).toBlocking().single().body();
}
/**
* Changes the failover priority for the Azure Cosmos DB database account. A failover priority of 0 indicates a write region. The maximum value for a failover priority = (total number of regions - 1). Failover priority values must be unique for each of the regions in which the database account exists.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param failoverPolicies List of failover policies.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture beginFailoverPriorityChangeAsync(String resourceGroupName, String accountName, List failoverPolicies, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(beginFailoverPriorityChangeWithServiceResponseAsync(resourceGroupName, accountName, failoverPolicies), serviceCallback);
}
/**
* Changes the failover priority for the Azure Cosmos DB database account. A failover priority of 0 indicates a write region. The maximum value for a failover priority = (total number of regions - 1). Failover priority values must be unique for each of the regions in which the database account exists.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param failoverPolicies List of failover policies.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceResponse} object if successful.
*/
public Observable beginFailoverPriorityChangeAsync(String resourceGroupName, String accountName, List failoverPolicies) {
return beginFailoverPriorityChangeWithServiceResponseAsync(resourceGroupName, accountName, failoverPolicies).map(new Func1, Void>() {
@Override
public Void call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Changes the failover priority for the Azure Cosmos DB database account. A failover priority of 0 indicates a write region. The maximum value for a failover priority = (total number of regions - 1). Failover priority values must be unique for each of the regions in which the database account exists.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param failoverPolicies List of failover policies.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceResponse} object if successful.
*/
public Observable> beginFailoverPriorityChangeWithServiceResponseAsync(String resourceGroupName, String accountName, List failoverPolicies) {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (accountName == null) {
throw new IllegalArgumentException("Parameter accountName is required and cannot be null.");
}
if (failoverPolicies == null) {
throw new IllegalArgumentException("Parameter failoverPolicies is required and cannot be null.");
}
Validator.validate(failoverPolicies);
final String apiVersion = "2019-08-01";
FailoverPolicies failoverParameters = new FailoverPolicies();
failoverParameters.withFailoverPolicies(failoverPolicies);
return service.beginFailoverPriorityChange(this.client.subscriptionId(), resourceGroupName, accountName, apiVersion, this.client.acceptLanguage(), failoverParameters, this.client.userAgent())
.flatMap(new Func1, Observable>>() {
@Override
public Observable> call(Response response) {
try {
ServiceResponse clientResponse = beginFailoverPriorityChangeDelegate(response);
return Observable.just(clientResponse);
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse beginFailoverPriorityChangeDelegate(Response response) throws CloudException, IOException, IllegalArgumentException {
return this.client.restClient().responseBuilderFactory().newInstance(this.client.serializerAdapter())
.register(202, new TypeToken() { }.getType())
.register(204, new TypeToken() { }.getType())
.registerError(CloudException.class)
.build(response);
}
/**
* Lists all the Azure Cosmos DB database accounts available under the subscription.
*
* @return the PagedList object if successful.
*/
public PagedList list() {
PageImpl page = new PageImpl<>();
page.setItems(listWithServiceResponseAsync().toBlocking().single().body());
page.setNextPageLink(null);
return new PagedList(page) {
@Override
public Page nextPage(String nextPageLink) {
return null;
}
};
}
/**
* Lists all the Azure Cosmos DB database accounts available under the subscription.
*
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @return the {@link ServiceFuture} object
*/
public ServiceFuture> listAsync(final ServiceCallback> serviceCallback) {
return ServiceFuture.fromResponse(listWithServiceResponseAsync(), serviceCallback);
}
/**
* Lists all the Azure Cosmos DB database accounts available under the subscription.
*
* @return the observable to the List<DatabaseAccountGetResultsInner> object
*/
public Observable> listAsync() {
return listWithServiceResponseAsync().map(new Func1>, Page>() {
@Override
public Page call(ServiceResponse> response) {
PageImpl page = new PageImpl<>();
page.setItems(response.body());
return page;
}
});
}
/**
* Lists all the Azure Cosmos DB database accounts available under the subscription.
*
* @return the observable to the List<DatabaseAccountGetResultsInner> object
*/
public Observable>> listWithServiceResponseAsync() {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
final String apiVersion = "2019-08-01";
return service.list(this.client.subscriptionId(), apiVersion, this.client.acceptLanguage(), this.client.userAgent())
.flatMap(new Func1, Observable>>>() {
@Override
public Observable>> call(Response response) {
try {
ServiceResponse> result = listDelegate(response);
List items = null;
if (result.body() != null) {
items = result.body().items();
}
ServiceResponse> clientResponse = new ServiceResponse>(items, result.response());
return Observable.just(clientResponse);
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse> listDelegate(Response response) throws CloudException, IOException, IllegalArgumentException {
return this.client.restClient().responseBuilderFactory()., CloudException>newInstance(this.client.serializerAdapter())
.register(200, new TypeToken>() { }.getType())
.registerError(CloudException.class)
.build(response);
}
/**
* Lists all the Azure Cosmos DB database accounts available under the given resource group.
*
* @param resourceGroupName Name of an Azure resource group.
* @return the PagedList object if successful.
*/
public PagedList listByResourceGroup(String resourceGroupName) {
PageImpl page = new PageImpl<>();
page.setItems(listByResourceGroupWithServiceResponseAsync(resourceGroupName).toBlocking().single().body());
page.setNextPageLink(null);
return new PagedList(page) {
@Override
public Page nextPage(String nextPageLink) {
return null;
}
};
}
/**
* Lists all the Azure Cosmos DB database accounts available under the given resource group.
*
* @param resourceGroupName Name of an Azure resource group.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @return the {@link ServiceFuture} object
*/
public ServiceFuture> listByResourceGroupAsync(String resourceGroupName, final ServiceCallback> serviceCallback) {
return ServiceFuture.fromResponse(listByResourceGroupWithServiceResponseAsync(resourceGroupName), serviceCallback);
}
/**
* Lists all the Azure Cosmos DB database accounts available under the given resource group.
*
* @param resourceGroupName Name of an Azure resource group.
* @return the observable to the List<DatabaseAccountGetResultsInner> object
*/
public Observable> listByResourceGroupAsync(String resourceGroupName) {
return listByResourceGroupWithServiceResponseAsync(resourceGroupName).map(new Func1>, Page>() {
@Override
public Page call(ServiceResponse> response) {
PageImpl page = new PageImpl<>();
page.setItems(response.body());
return page;
}
});
}
/**
* Lists all the Azure Cosmos DB database accounts available under the given resource group.
*
* @param resourceGroupName Name of an Azure resource group.
* @return the observable to the List<DatabaseAccountGetResultsInner> object
*/
public Observable>> listByResourceGroupWithServiceResponseAsync(String resourceGroupName) {
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
final String apiVersion = "2019-08-01";
return service.listByResourceGroup(resourceGroupName, this.client.subscriptionId(), apiVersion, this.client.acceptLanguage(), this.client.userAgent())
.flatMap(new Func1, Observable>>>() {
@Override
public Observable>> call(Response response) {
try {
ServiceResponse> result = listByResourceGroupDelegate(response);
List items = null;
if (result.body() != null) {
items = result.body().items();
}
ServiceResponse> clientResponse = new ServiceResponse>(items, result.response());
return Observable.just(clientResponse);
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse> listByResourceGroupDelegate(Response response) throws CloudException, IOException, IllegalArgumentException {
return this.client.restClient().responseBuilderFactory()., CloudException>newInstance(this.client.serializerAdapter())
.register(200, new TypeToken>() { }.getType())
.registerError(CloudException.class)
.build(response);
}
/**
* Lists the access keys for the specified Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the DatabaseAccountListKeysResultInner object if successful.
*/
public DatabaseAccountListKeysResultInner listKeys(String resourceGroupName, String accountName) {
return listKeysWithServiceResponseAsync(resourceGroupName, accountName).toBlocking().single().body();
}
/**
* Lists the access keys for the specified Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture listKeysAsync(String resourceGroupName, String accountName, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(listKeysWithServiceResponseAsync(resourceGroupName, accountName), serviceCallback);
}
/**
* Lists the access keys for the specified Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the DatabaseAccountListKeysResultInner object
*/
public Observable listKeysAsync(String resourceGroupName, String accountName) {
return listKeysWithServiceResponseAsync(resourceGroupName, accountName).map(new Func1, DatabaseAccountListKeysResultInner>() {
@Override
public DatabaseAccountListKeysResultInner call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Lists the access keys for the specified Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the DatabaseAccountListKeysResultInner object
*/
public Observable> listKeysWithServiceResponseAsync(String resourceGroupName, String accountName) {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (accountName == null) {
throw new IllegalArgumentException("Parameter accountName is required and cannot be null.");
}
final String apiVersion = "2019-08-01";
return service.listKeys(this.client.subscriptionId(), resourceGroupName, accountName, apiVersion, this.client.acceptLanguage(), this.client.userAgent())
.flatMap(new Func1, Observable>>() {
@Override
public Observable> call(Response response) {
try {
ServiceResponse clientResponse = listKeysDelegate(response);
return Observable.just(clientResponse);
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse listKeysDelegate(Response response) throws CloudException, IOException, IllegalArgumentException {
return this.client.restClient().responseBuilderFactory().newInstance(this.client.serializerAdapter())
.register(200, new TypeToken() { }.getType())
.registerError(CloudException.class)
.build(response);
}
/**
* Lists the connection strings for the specified Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the DatabaseAccountListConnectionStringsResultInner object if successful.
*/
public DatabaseAccountListConnectionStringsResultInner listConnectionStrings(String resourceGroupName, String accountName) {
return listConnectionStringsWithServiceResponseAsync(resourceGroupName, accountName).toBlocking().single().body();
}
/**
* Lists the connection strings for the specified Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture listConnectionStringsAsync(String resourceGroupName, String accountName, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(listConnectionStringsWithServiceResponseAsync(resourceGroupName, accountName), serviceCallback);
}
/**
* Lists the connection strings for the specified Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the DatabaseAccountListConnectionStringsResultInner object
*/
public Observable listConnectionStringsAsync(String resourceGroupName, String accountName) {
return listConnectionStringsWithServiceResponseAsync(resourceGroupName, accountName).map(new Func1, DatabaseAccountListConnectionStringsResultInner>() {
@Override
public DatabaseAccountListConnectionStringsResultInner call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Lists the connection strings for the specified Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the DatabaseAccountListConnectionStringsResultInner object
*/
public Observable> listConnectionStringsWithServiceResponseAsync(String resourceGroupName, String accountName) {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (accountName == null) {
throw new IllegalArgumentException("Parameter accountName is required and cannot be null.");
}
final String apiVersion = "2019-08-01";
return service.listConnectionStrings(this.client.subscriptionId(), resourceGroupName, accountName, apiVersion, this.client.acceptLanguage(), this.client.userAgent())
.flatMap(new Func1, Observable>>() {
@Override
public Observable> call(Response response) {
try {
ServiceResponse clientResponse = listConnectionStringsDelegate(response);
return Observable.just(clientResponse);
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse listConnectionStringsDelegate(Response response) throws CloudException, IOException, IllegalArgumentException {
return this.client.restClient().responseBuilderFactory().newInstance(this.client.serializerAdapter())
.register(200, new TypeToken() { }.getType())
.registerError(CloudException.class)
.build(response);
}
/**
* Offline the specified region for the specified Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param region Cosmos DB region, with spaces between words and each word capitalized.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws ErrorResponseException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
*/
public void offlineRegion(String resourceGroupName, String accountName, String region) {
offlineRegionWithServiceResponseAsync(resourceGroupName, accountName, region).toBlocking().last().body();
}
/**
* Offline the specified region for the specified Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param region Cosmos DB region, with spaces between words and each word capitalized.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture offlineRegionAsync(String resourceGroupName, String accountName, String region, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(offlineRegionWithServiceResponseAsync(resourceGroupName, accountName, region), serviceCallback);
}
/**
* Offline the specified region for the specified Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param region Cosmos DB region, with spaces between words and each word capitalized.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable for the request
*/
public Observable offlineRegionAsync(String resourceGroupName, String accountName, String region) {
return offlineRegionWithServiceResponseAsync(resourceGroupName, accountName, region).map(new Func1, Void>() {
@Override
public Void call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Offline the specified region for the specified Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param region Cosmos DB region, with spaces between words and each word capitalized.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable for the request
*/
public Observable> offlineRegionWithServiceResponseAsync(String resourceGroupName, String accountName, String region) {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (accountName == null) {
throw new IllegalArgumentException("Parameter accountName is required and cannot be null.");
}
if (region == null) {
throw new IllegalArgumentException("Parameter region is required and cannot be null.");
}
final String apiVersion = "2019-08-01";
RegionForOnlineOffline regionParameterForOffline = new RegionForOnlineOffline();
regionParameterForOffline.withRegion(region);
Observable> observable = service.offlineRegion(this.client.subscriptionId(), resourceGroupName, accountName, apiVersion, this.client.acceptLanguage(), regionParameterForOffline, this.client.userAgent());
return client.getAzureClient().getPostOrDeleteResultAsync(observable, new TypeToken() { }.getType());
}
/**
* Offline the specified region for the specified Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param region Cosmos DB region, with spaces between words and each word capitalized.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws ErrorResponseException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
*/
public void beginOfflineRegion(String resourceGroupName, String accountName, String region) {
beginOfflineRegionWithServiceResponseAsync(resourceGroupName, accountName, region).toBlocking().single().body();
}
/**
* Offline the specified region for the specified Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param region Cosmos DB region, with spaces between words and each word capitalized.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture beginOfflineRegionAsync(String resourceGroupName, String accountName, String region, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(beginOfflineRegionWithServiceResponseAsync(resourceGroupName, accountName, region), serviceCallback);
}
/**
* Offline the specified region for the specified Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param region Cosmos DB region, with spaces between words and each word capitalized.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceResponse} object if successful.
*/
public Observable beginOfflineRegionAsync(String resourceGroupName, String accountName, String region) {
return beginOfflineRegionWithServiceResponseAsync(resourceGroupName, accountName, region).map(new Func1, Void>() {
@Override
public Void call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Offline the specified region for the specified Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param region Cosmos DB region, with spaces between words and each word capitalized.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceResponse} object if successful.
*/
public Observable> beginOfflineRegionWithServiceResponseAsync(String resourceGroupName, String accountName, String region) {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (accountName == null) {
throw new IllegalArgumentException("Parameter accountName is required and cannot be null.");
}
if (region == null) {
throw new IllegalArgumentException("Parameter region is required and cannot be null.");
}
final String apiVersion = "2019-08-01";
RegionForOnlineOffline regionParameterForOffline = new RegionForOnlineOffline();
regionParameterForOffline.withRegion(region);
return service.beginOfflineRegion(this.client.subscriptionId(), resourceGroupName, accountName, apiVersion, this.client.acceptLanguage(), regionParameterForOffline, this.client.userAgent())
.flatMap(new Func1, Observable>>() {
@Override
public Observable> call(Response response) {
try {
ServiceResponse clientResponse = beginOfflineRegionDelegate(response);
return Observable.just(clientResponse);
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse beginOfflineRegionDelegate(Response response) throws ErrorResponseException, IOException, IllegalArgumentException {
return this.client.restClient().responseBuilderFactory().newInstance(this.client.serializerAdapter())
.register(200, new TypeToken() { }.getType())
.register(202, new TypeToken