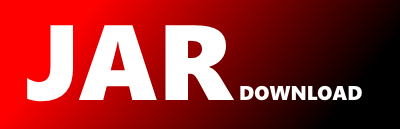
com.microsoft.azure.management.cosmosdb.implementation.MongoDBResourcesInner Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-mgmt-cosmosdb Show documentation
Show all versions of azure-mgmt-cosmosdb Show documentation
This package contains Microsoft Azure CosmosDB SDK. A new set of management libraries are now Generally Available. For documentation on how to use the new libraries, please see https://aka.ms/azsdk/java/mgmt
/**
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for
* license information.
*
* Code generated by Microsoft (R) AutoRest Code Generator.
*/
package com.microsoft.azure.management.cosmosdb.implementation;
import retrofit2.Retrofit;
import com.google.common.reflect.TypeToken;
import com.microsoft.azure.CloudException;
import com.microsoft.azure.management.cosmosdb.MongoDBCollectionCreateUpdateParameters;
import com.microsoft.azure.management.cosmosdb.MongoDBDatabaseCreateUpdateParameters;
import com.microsoft.azure.management.cosmosdb.ThroughputSettingsUpdateParameters;
import com.microsoft.rest.ServiceCallback;
import com.microsoft.rest.ServiceFuture;
import com.microsoft.rest.ServiceResponse;
import com.microsoft.rest.Validator;
import java.io.IOException;
import java.util.List;
import okhttp3.ResponseBody;
import retrofit2.http.Body;
import retrofit2.http.GET;
import retrofit2.http.Header;
import retrofit2.http.Headers;
import retrofit2.http.HTTP;
import retrofit2.http.Path;
import retrofit2.http.PUT;
import retrofit2.http.Query;
import retrofit2.Response;
import rx.functions.Func1;
import rx.Observable;
/**
* An instance of this class provides access to all the operations defined
* in MongoDBResources.
*/
public class MongoDBResourcesInner {
/** The Retrofit service to perform REST calls. */
private MongoDBResourcesService service;
/** The service client containing this operation class. */
private CosmosDBImpl client;
/**
* Initializes an instance of MongoDBResourcesInner.
*
* @param retrofit the Retrofit instance built from a Retrofit Builder.
* @param client the instance of the service client containing this operation class.
*/
public MongoDBResourcesInner(Retrofit retrofit, CosmosDBImpl client) {
this.service = retrofit.create(MongoDBResourcesService.class);
this.client = client;
}
/**
* The interface defining all the services for MongoDBResources to be
* used by Retrofit to perform actually REST calls.
*/
interface MongoDBResourcesService {
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.MongoDBResources listMongoDBDatabases" })
@GET("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/mongodbDatabases")
Observable> listMongoDBDatabases(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.MongoDBResources getMongoDBDatabase" })
@GET("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/mongodbDatabases/{databaseName}")
Observable> getMongoDBDatabase(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Path("databaseName") String databaseName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.MongoDBResources createUpdateMongoDBDatabase" })
@PUT("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/mongodbDatabases/{databaseName}")
Observable> createUpdateMongoDBDatabase(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Path("databaseName") String databaseName, @Query("api-version") String apiVersion, @Body MongoDBDatabaseCreateUpdateParameters createUpdateMongoDBDatabaseParameters, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.MongoDBResources beginCreateUpdateMongoDBDatabase" })
@PUT("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/mongodbDatabases/{databaseName}")
Observable> beginCreateUpdateMongoDBDatabase(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Path("databaseName") String databaseName, @Query("api-version") String apiVersion, @Body MongoDBDatabaseCreateUpdateParameters createUpdateMongoDBDatabaseParameters, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.MongoDBResources deleteMongoDBDatabase" })
@HTTP(path = "subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/mongodbDatabases/{databaseName}", method = "DELETE", hasBody = true)
Observable> deleteMongoDBDatabase(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Path("databaseName") String databaseName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.MongoDBResources beginDeleteMongoDBDatabase" })
@HTTP(path = "subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/mongodbDatabases/{databaseName}", method = "DELETE", hasBody = true)
Observable> beginDeleteMongoDBDatabase(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Path("databaseName") String databaseName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.MongoDBResources getMongoDBDatabaseThroughput" })
@GET("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/mongodbDatabases/{databaseName}/throughputSettings/default")
Observable> getMongoDBDatabaseThroughput(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Path("databaseName") String databaseName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.MongoDBResources updateMongoDBDatabaseThroughput" })
@PUT("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/mongodbDatabases/{databaseName}/throughputSettings/default")
Observable> updateMongoDBDatabaseThroughput(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Path("databaseName") String databaseName, @Query("api-version") String apiVersion, @Body ThroughputSettingsUpdateParameters updateThroughputParameters, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.MongoDBResources beginUpdateMongoDBDatabaseThroughput" })
@PUT("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/mongodbDatabases/{databaseName}/throughputSettings/default")
Observable> beginUpdateMongoDBDatabaseThroughput(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Path("databaseName") String databaseName, @Query("api-version") String apiVersion, @Body ThroughputSettingsUpdateParameters updateThroughputParameters, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.MongoDBResources listMongoDBCollections" })
@GET("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/mongodbDatabases/{databaseName}/collections")
Observable> listMongoDBCollections(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Path("databaseName") String databaseName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.MongoDBResources getMongoDBCollection" })
@GET("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/mongodbDatabases/{databaseName}/collections/{collectionName}")
Observable> getMongoDBCollection(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Path("databaseName") String databaseName, @Path("collectionName") String collectionName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.MongoDBResources createUpdateMongoDBCollection" })
@PUT("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/mongodbDatabases/{databaseName}/collections/{collectionName}")
Observable> createUpdateMongoDBCollection(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Path("databaseName") String databaseName, @Path("collectionName") String collectionName, @Query("api-version") String apiVersion, @Body MongoDBCollectionCreateUpdateParameters createUpdateMongoDBCollectionParameters, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.MongoDBResources beginCreateUpdateMongoDBCollection" })
@PUT("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/mongodbDatabases/{databaseName}/collections/{collectionName}")
Observable> beginCreateUpdateMongoDBCollection(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Path("databaseName") String databaseName, @Path("collectionName") String collectionName, @Query("api-version") String apiVersion, @Body MongoDBCollectionCreateUpdateParameters createUpdateMongoDBCollectionParameters, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.MongoDBResources deleteMongoDBCollection" })
@HTTP(path = "subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/mongodbDatabases/{databaseName}/collections/{collectionName}", method = "DELETE", hasBody = true)
Observable> deleteMongoDBCollection(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Path("databaseName") String databaseName, @Path("collectionName") String collectionName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.MongoDBResources beginDeleteMongoDBCollection" })
@HTTP(path = "subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/mongodbDatabases/{databaseName}/collections/{collectionName}", method = "DELETE", hasBody = true)
Observable> beginDeleteMongoDBCollection(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Path("databaseName") String databaseName, @Path("collectionName") String collectionName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.MongoDBResources getMongoDBCollectionThroughput" })
@GET("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/mongodbDatabases/{databaseName}/collections/{collectionName}/throughputSettings/default")
Observable> getMongoDBCollectionThroughput(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Path("databaseName") String databaseName, @Path("collectionName") String collectionName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.MongoDBResources updateMongoDBCollectionThroughput" })
@PUT("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/mongodbDatabases/{databaseName}/collections/{collectionName}/throughputSettings/default")
Observable> updateMongoDBCollectionThroughput(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Path("databaseName") String databaseName, @Path("collectionName") String collectionName, @Query("api-version") String apiVersion, @Body ThroughputSettingsUpdateParameters updateThroughputParameters, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.MongoDBResources beginUpdateMongoDBCollectionThroughput" })
@PUT("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/mongodbDatabases/{databaseName}/collections/{collectionName}/throughputSettings/default")
Observable> beginUpdateMongoDBCollectionThroughput(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Path("databaseName") String databaseName, @Path("collectionName") String collectionName, @Query("api-version") String apiVersion, @Body ThroughputSettingsUpdateParameters updateThroughputParameters, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
}
/**
* Lists the MongoDB databases under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the List<MongoDBDatabaseGetResultsInner> object if successful.
*/
public List listMongoDBDatabases(String resourceGroupName, String accountName) {
return listMongoDBDatabasesWithServiceResponseAsync(resourceGroupName, accountName).toBlocking().single().body();
}
/**
* Lists the MongoDB databases under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture> listMongoDBDatabasesAsync(String resourceGroupName, String accountName, final ServiceCallback> serviceCallback) {
return ServiceFuture.fromResponse(listMongoDBDatabasesWithServiceResponseAsync(resourceGroupName, accountName), serviceCallback);
}
/**
* Lists the MongoDB databases under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the List<MongoDBDatabaseGetResultsInner> object
*/
public Observable> listMongoDBDatabasesAsync(String resourceGroupName, String accountName) {
return listMongoDBDatabasesWithServiceResponseAsync(resourceGroupName, accountName).map(new Func1>, List>() {
@Override
public List call(ServiceResponse> response) {
return response.body();
}
});
}
/**
* Lists the MongoDB databases under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the List<MongoDBDatabaseGetResultsInner> object
*/
public Observable>> listMongoDBDatabasesWithServiceResponseAsync(String resourceGroupName, String accountName) {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (accountName == null) {
throw new IllegalArgumentException("Parameter accountName is required and cannot be null.");
}
final String apiVersion = "2019-08-01";
return service.listMongoDBDatabases(this.client.subscriptionId(), resourceGroupName, accountName, apiVersion, this.client.acceptLanguage(), this.client.userAgent())
.flatMap(new Func1, Observable>>>() {
@Override
public Observable>> call(Response response) {
try {
ServiceResponse> result = listMongoDBDatabasesDelegate(response);
List items = null;
if (result.body() != null) {
items = result.body().items();
}
ServiceResponse> clientResponse = new ServiceResponse>(items, result.response());
return Observable.just(clientResponse);
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse> listMongoDBDatabasesDelegate(Response response) throws CloudException, IOException, IllegalArgumentException {
return this.client.restClient().responseBuilderFactory()., CloudException>newInstance(this.client.serializerAdapter())
.register(200, new TypeToken>() { }.getType())
.registerError(CloudException.class)
.build(response);
}
/**
* Gets the MongoDB databases under an existing Azure Cosmos DB database account with the provided name.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the MongoDBDatabaseGetResultsInner object if successful.
*/
public MongoDBDatabaseGetResultsInner getMongoDBDatabase(String resourceGroupName, String accountName, String databaseName) {
return getMongoDBDatabaseWithServiceResponseAsync(resourceGroupName, accountName, databaseName).toBlocking().single().body();
}
/**
* Gets the MongoDB databases under an existing Azure Cosmos DB database account with the provided name.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture getMongoDBDatabaseAsync(String resourceGroupName, String accountName, String databaseName, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(getMongoDBDatabaseWithServiceResponseAsync(resourceGroupName, accountName, databaseName), serviceCallback);
}
/**
* Gets the MongoDB databases under an existing Azure Cosmos DB database account with the provided name.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the MongoDBDatabaseGetResultsInner object
*/
public Observable getMongoDBDatabaseAsync(String resourceGroupName, String accountName, String databaseName) {
return getMongoDBDatabaseWithServiceResponseAsync(resourceGroupName, accountName, databaseName).map(new Func1, MongoDBDatabaseGetResultsInner>() {
@Override
public MongoDBDatabaseGetResultsInner call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Gets the MongoDB databases under an existing Azure Cosmos DB database account with the provided name.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the MongoDBDatabaseGetResultsInner object
*/
public Observable> getMongoDBDatabaseWithServiceResponseAsync(String resourceGroupName, String accountName, String databaseName) {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (accountName == null) {
throw new IllegalArgumentException("Parameter accountName is required and cannot be null.");
}
if (databaseName == null) {
throw new IllegalArgumentException("Parameter databaseName is required and cannot be null.");
}
final String apiVersion = "2019-08-01";
return service.getMongoDBDatabase(this.client.subscriptionId(), resourceGroupName, accountName, databaseName, apiVersion, this.client.acceptLanguage(), this.client.userAgent())
.flatMap(new Func1, Observable>>() {
@Override
public Observable> call(Response response) {
try {
ServiceResponse clientResponse = getMongoDBDatabaseDelegate(response);
return Observable.just(clientResponse);
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse getMongoDBDatabaseDelegate(Response response) throws CloudException, IOException, IllegalArgumentException {
return this.client.restClient().responseBuilderFactory().newInstance(this.client.serializerAdapter())
.register(200, new TypeToken() { }.getType())
.registerError(CloudException.class)
.build(response);
}
/**
* Create or updates Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param createUpdateMongoDBDatabaseParameters The parameters to provide for the current MongoDB database.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the MongoDBDatabaseGetResultsInner object if successful.
*/
public MongoDBDatabaseGetResultsInner createUpdateMongoDBDatabase(String resourceGroupName, String accountName, String databaseName, MongoDBDatabaseCreateUpdateParameters createUpdateMongoDBDatabaseParameters) {
return createUpdateMongoDBDatabaseWithServiceResponseAsync(resourceGroupName, accountName, databaseName, createUpdateMongoDBDatabaseParameters).toBlocking().last().body();
}
/**
* Create or updates Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param createUpdateMongoDBDatabaseParameters The parameters to provide for the current MongoDB database.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture createUpdateMongoDBDatabaseAsync(String resourceGroupName, String accountName, String databaseName, MongoDBDatabaseCreateUpdateParameters createUpdateMongoDBDatabaseParameters, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(createUpdateMongoDBDatabaseWithServiceResponseAsync(resourceGroupName, accountName, databaseName, createUpdateMongoDBDatabaseParameters), serviceCallback);
}
/**
* Create or updates Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param createUpdateMongoDBDatabaseParameters The parameters to provide for the current MongoDB database.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable for the request
*/
public Observable createUpdateMongoDBDatabaseAsync(String resourceGroupName, String accountName, String databaseName, MongoDBDatabaseCreateUpdateParameters createUpdateMongoDBDatabaseParameters) {
return createUpdateMongoDBDatabaseWithServiceResponseAsync(resourceGroupName, accountName, databaseName, createUpdateMongoDBDatabaseParameters).map(new Func1, MongoDBDatabaseGetResultsInner>() {
@Override
public MongoDBDatabaseGetResultsInner call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Create or updates Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param createUpdateMongoDBDatabaseParameters The parameters to provide for the current MongoDB database.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable for the request
*/
public Observable> createUpdateMongoDBDatabaseWithServiceResponseAsync(String resourceGroupName, String accountName, String databaseName, MongoDBDatabaseCreateUpdateParameters createUpdateMongoDBDatabaseParameters) {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (accountName == null) {
throw new IllegalArgumentException("Parameter accountName is required and cannot be null.");
}
if (databaseName == null) {
throw new IllegalArgumentException("Parameter databaseName is required and cannot be null.");
}
if (createUpdateMongoDBDatabaseParameters == null) {
throw new IllegalArgumentException("Parameter createUpdateMongoDBDatabaseParameters is required and cannot be null.");
}
Validator.validate(createUpdateMongoDBDatabaseParameters);
final String apiVersion = "2019-08-01";
Observable> observable = service.createUpdateMongoDBDatabase(this.client.subscriptionId(), resourceGroupName, accountName, databaseName, apiVersion, createUpdateMongoDBDatabaseParameters, this.client.acceptLanguage(), this.client.userAgent());
return client.getAzureClient().getPutOrPatchResultAsync(observable, new TypeToken() { }.getType());
}
/**
* Create or updates Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param createUpdateMongoDBDatabaseParameters The parameters to provide for the current MongoDB database.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the MongoDBDatabaseGetResultsInner object if successful.
*/
public MongoDBDatabaseGetResultsInner beginCreateUpdateMongoDBDatabase(String resourceGroupName, String accountName, String databaseName, MongoDBDatabaseCreateUpdateParameters createUpdateMongoDBDatabaseParameters) {
return beginCreateUpdateMongoDBDatabaseWithServiceResponseAsync(resourceGroupName, accountName, databaseName, createUpdateMongoDBDatabaseParameters).toBlocking().single().body();
}
/**
* Create or updates Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param createUpdateMongoDBDatabaseParameters The parameters to provide for the current MongoDB database.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture beginCreateUpdateMongoDBDatabaseAsync(String resourceGroupName, String accountName, String databaseName, MongoDBDatabaseCreateUpdateParameters createUpdateMongoDBDatabaseParameters, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(beginCreateUpdateMongoDBDatabaseWithServiceResponseAsync(resourceGroupName, accountName, databaseName, createUpdateMongoDBDatabaseParameters), serviceCallback);
}
/**
* Create or updates Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param createUpdateMongoDBDatabaseParameters The parameters to provide for the current MongoDB database.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the MongoDBDatabaseGetResultsInner object
*/
public Observable beginCreateUpdateMongoDBDatabaseAsync(String resourceGroupName, String accountName, String databaseName, MongoDBDatabaseCreateUpdateParameters createUpdateMongoDBDatabaseParameters) {
return beginCreateUpdateMongoDBDatabaseWithServiceResponseAsync(resourceGroupName, accountName, databaseName, createUpdateMongoDBDatabaseParameters).map(new Func1, MongoDBDatabaseGetResultsInner>() {
@Override
public MongoDBDatabaseGetResultsInner call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Create or updates Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param createUpdateMongoDBDatabaseParameters The parameters to provide for the current MongoDB database.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the MongoDBDatabaseGetResultsInner object
*/
public Observable> beginCreateUpdateMongoDBDatabaseWithServiceResponseAsync(String resourceGroupName, String accountName, String databaseName, MongoDBDatabaseCreateUpdateParameters createUpdateMongoDBDatabaseParameters) {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (accountName == null) {
throw new IllegalArgumentException("Parameter accountName is required and cannot be null.");
}
if (databaseName == null) {
throw new IllegalArgumentException("Parameter databaseName is required and cannot be null.");
}
if (createUpdateMongoDBDatabaseParameters == null) {
throw new IllegalArgumentException("Parameter createUpdateMongoDBDatabaseParameters is required and cannot be null.");
}
Validator.validate(createUpdateMongoDBDatabaseParameters);
final String apiVersion = "2019-08-01";
return service.beginCreateUpdateMongoDBDatabase(this.client.subscriptionId(), resourceGroupName, accountName, databaseName, apiVersion, createUpdateMongoDBDatabaseParameters, this.client.acceptLanguage(), this.client.userAgent())
.flatMap(new Func1, Observable>>() {
@Override
public Observable> call(Response response) {
try {
ServiceResponse clientResponse = beginCreateUpdateMongoDBDatabaseDelegate(response);
return Observable.just(clientResponse);
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse beginCreateUpdateMongoDBDatabaseDelegate(Response response) throws CloudException, IOException, IllegalArgumentException {
return this.client.restClient().responseBuilderFactory().newInstance(this.client.serializerAdapter())
.register(200, new TypeToken() { }.getType())
.register(202, new TypeToken() { }.getType())
.registerError(CloudException.class)
.build(response);
}
/**
* Deletes an existing Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
*/
public void deleteMongoDBDatabase(String resourceGroupName, String accountName, String databaseName) {
deleteMongoDBDatabaseWithServiceResponseAsync(resourceGroupName, accountName, databaseName).toBlocking().last().body();
}
/**
* Deletes an existing Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture deleteMongoDBDatabaseAsync(String resourceGroupName, String accountName, String databaseName, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(deleteMongoDBDatabaseWithServiceResponseAsync(resourceGroupName, accountName, databaseName), serviceCallback);
}
/**
* Deletes an existing Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable for the request
*/
public Observable deleteMongoDBDatabaseAsync(String resourceGroupName, String accountName, String databaseName) {
return deleteMongoDBDatabaseWithServiceResponseAsync(resourceGroupName, accountName, databaseName).map(new Func1, Void>() {
@Override
public Void call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Deletes an existing Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable for the request
*/
public Observable> deleteMongoDBDatabaseWithServiceResponseAsync(String resourceGroupName, String accountName, String databaseName) {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (accountName == null) {
throw new IllegalArgumentException("Parameter accountName is required and cannot be null.");
}
if (databaseName == null) {
throw new IllegalArgumentException("Parameter databaseName is required and cannot be null.");
}
final String apiVersion = "2019-08-01";
Observable> observable = service.deleteMongoDBDatabase(this.client.subscriptionId(), resourceGroupName, accountName, databaseName, apiVersion, this.client.acceptLanguage(), this.client.userAgent());
return client.getAzureClient().getPostOrDeleteResultAsync(observable, new TypeToken() { }.getType());
}
/**
* Deletes an existing Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
*/
public void beginDeleteMongoDBDatabase(String resourceGroupName, String accountName, String databaseName) {
beginDeleteMongoDBDatabaseWithServiceResponseAsync(resourceGroupName, accountName, databaseName).toBlocking().single().body();
}
/**
* Deletes an existing Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture beginDeleteMongoDBDatabaseAsync(String resourceGroupName, String accountName, String databaseName, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(beginDeleteMongoDBDatabaseWithServiceResponseAsync(resourceGroupName, accountName, databaseName), serviceCallback);
}
/**
* Deletes an existing Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceResponse} object if successful.
*/
public Observable beginDeleteMongoDBDatabaseAsync(String resourceGroupName, String accountName, String databaseName) {
return beginDeleteMongoDBDatabaseWithServiceResponseAsync(resourceGroupName, accountName, databaseName).map(new Func1, Void>() {
@Override
public Void call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Deletes an existing Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceResponse} object if successful.
*/
public Observable> beginDeleteMongoDBDatabaseWithServiceResponseAsync(String resourceGroupName, String accountName, String databaseName) {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (accountName == null) {
throw new IllegalArgumentException("Parameter accountName is required and cannot be null.");
}
if (databaseName == null) {
throw new IllegalArgumentException("Parameter databaseName is required and cannot be null.");
}
final String apiVersion = "2019-08-01";
return service.beginDeleteMongoDBDatabase(this.client.subscriptionId(), resourceGroupName, accountName, databaseName, apiVersion, this.client.acceptLanguage(), this.client.userAgent())
.flatMap(new Func1, Observable>>() {
@Override
public Observable> call(Response response) {
try {
ServiceResponse clientResponse = beginDeleteMongoDBDatabaseDelegate(response);
return Observable.just(clientResponse);
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse beginDeleteMongoDBDatabaseDelegate(Response response) throws CloudException, IOException, IllegalArgumentException {
return this.client.restClient().responseBuilderFactory().newInstance(this.client.serializerAdapter())
.register(202, new TypeToken() { }.getType())
.register(204, new TypeToken() { }.getType())
.registerError(CloudException.class)
.build(response);
}
/**
* Gets the RUs per second of the MongoDB database under an existing Azure Cosmos DB database account with the provided name.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the ThroughputSettingsGetResultsInner object if successful.
*/
public ThroughputSettingsGetResultsInner getMongoDBDatabaseThroughput(String resourceGroupName, String accountName, String databaseName) {
return getMongoDBDatabaseThroughputWithServiceResponseAsync(resourceGroupName, accountName, databaseName).toBlocking().single().body();
}
/**
* Gets the RUs per second of the MongoDB database under an existing Azure Cosmos DB database account with the provided name.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture getMongoDBDatabaseThroughputAsync(String resourceGroupName, String accountName, String databaseName, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(getMongoDBDatabaseThroughputWithServiceResponseAsync(resourceGroupName, accountName, databaseName), serviceCallback);
}
/**
* Gets the RUs per second of the MongoDB database under an existing Azure Cosmos DB database account with the provided name.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the ThroughputSettingsGetResultsInner object
*/
public Observable getMongoDBDatabaseThroughputAsync(String resourceGroupName, String accountName, String databaseName) {
return getMongoDBDatabaseThroughputWithServiceResponseAsync(resourceGroupName, accountName, databaseName).map(new Func1, ThroughputSettingsGetResultsInner>() {
@Override
public ThroughputSettingsGetResultsInner call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Gets the RUs per second of the MongoDB database under an existing Azure Cosmos DB database account with the provided name.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the ThroughputSettingsGetResultsInner object
*/
public Observable> getMongoDBDatabaseThroughputWithServiceResponseAsync(String resourceGroupName, String accountName, String databaseName) {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (accountName == null) {
throw new IllegalArgumentException("Parameter accountName is required and cannot be null.");
}
if (databaseName == null) {
throw new IllegalArgumentException("Parameter databaseName is required and cannot be null.");
}
final String apiVersion = "2019-08-01";
return service.getMongoDBDatabaseThroughput(this.client.subscriptionId(), resourceGroupName, accountName, databaseName, apiVersion, this.client.acceptLanguage(), this.client.userAgent())
.flatMap(new Func1, Observable>>() {
@Override
public Observable> call(Response response) {
try {
ServiceResponse clientResponse = getMongoDBDatabaseThroughputDelegate(response);
return Observable.just(clientResponse);
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse getMongoDBDatabaseThroughputDelegate(Response response) throws CloudException, IOException, IllegalArgumentException {
return this.client.restClient().responseBuilderFactory().newInstance(this.client.serializerAdapter())
.register(200, new TypeToken() { }.getType())
.registerError(CloudException.class)
.build(response);
}
/**
* Update RUs per second of the an Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param updateThroughputParameters The RUs per second of the parameters to provide for the current MongoDB database.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the ThroughputSettingsGetResultsInner object if successful.
*/
public ThroughputSettingsGetResultsInner updateMongoDBDatabaseThroughput(String resourceGroupName, String accountName, String databaseName, ThroughputSettingsUpdateParameters updateThroughputParameters) {
return updateMongoDBDatabaseThroughputWithServiceResponseAsync(resourceGroupName, accountName, databaseName, updateThroughputParameters).toBlocking().last().body();
}
/**
* Update RUs per second of the an Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param updateThroughputParameters The RUs per second of the parameters to provide for the current MongoDB database.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture updateMongoDBDatabaseThroughputAsync(String resourceGroupName, String accountName, String databaseName, ThroughputSettingsUpdateParameters updateThroughputParameters, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(updateMongoDBDatabaseThroughputWithServiceResponseAsync(resourceGroupName, accountName, databaseName, updateThroughputParameters), serviceCallback);
}
/**
* Update RUs per second of the an Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param updateThroughputParameters The RUs per second of the parameters to provide for the current MongoDB database.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable for the request
*/
public Observable updateMongoDBDatabaseThroughputAsync(String resourceGroupName, String accountName, String databaseName, ThroughputSettingsUpdateParameters updateThroughputParameters) {
return updateMongoDBDatabaseThroughputWithServiceResponseAsync(resourceGroupName, accountName, databaseName, updateThroughputParameters).map(new Func1, ThroughputSettingsGetResultsInner>() {
@Override
public ThroughputSettingsGetResultsInner call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Update RUs per second of the an Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param updateThroughputParameters The RUs per second of the parameters to provide for the current MongoDB database.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable for the request
*/
public Observable> updateMongoDBDatabaseThroughputWithServiceResponseAsync(String resourceGroupName, String accountName, String databaseName, ThroughputSettingsUpdateParameters updateThroughputParameters) {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (accountName == null) {
throw new IllegalArgumentException("Parameter accountName is required and cannot be null.");
}
if (databaseName == null) {
throw new IllegalArgumentException("Parameter databaseName is required and cannot be null.");
}
if (updateThroughputParameters == null) {
throw new IllegalArgumentException("Parameter updateThroughputParameters is required and cannot be null.");
}
Validator.validate(updateThroughputParameters);
final String apiVersion = "2019-08-01";
Observable> observable = service.updateMongoDBDatabaseThroughput(this.client.subscriptionId(), resourceGroupName, accountName, databaseName, apiVersion, updateThroughputParameters, this.client.acceptLanguage(), this.client.userAgent());
return client.getAzureClient().getPutOrPatchResultAsync(observable, new TypeToken() { }.getType());
}
/**
* Update RUs per second of the an Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param updateThroughputParameters The RUs per second of the parameters to provide for the current MongoDB database.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the ThroughputSettingsGetResultsInner object if successful.
*/
public ThroughputSettingsGetResultsInner beginUpdateMongoDBDatabaseThroughput(String resourceGroupName, String accountName, String databaseName, ThroughputSettingsUpdateParameters updateThroughputParameters) {
return beginUpdateMongoDBDatabaseThroughputWithServiceResponseAsync(resourceGroupName, accountName, databaseName, updateThroughputParameters).toBlocking().single().body();
}
/**
* Update RUs per second of the an Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param updateThroughputParameters The RUs per second of the parameters to provide for the current MongoDB database.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture beginUpdateMongoDBDatabaseThroughputAsync(String resourceGroupName, String accountName, String databaseName, ThroughputSettingsUpdateParameters updateThroughputParameters, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(beginUpdateMongoDBDatabaseThroughputWithServiceResponseAsync(resourceGroupName, accountName, databaseName, updateThroughputParameters), serviceCallback);
}
/**
* Update RUs per second of the an Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param updateThroughputParameters The RUs per second of the parameters to provide for the current MongoDB database.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the ThroughputSettingsGetResultsInner object
*/
public Observable beginUpdateMongoDBDatabaseThroughputAsync(String resourceGroupName, String accountName, String databaseName, ThroughputSettingsUpdateParameters updateThroughputParameters) {
return beginUpdateMongoDBDatabaseThroughputWithServiceResponseAsync(resourceGroupName, accountName, databaseName, updateThroughputParameters).map(new Func1, ThroughputSettingsGetResultsInner>() {
@Override
public ThroughputSettingsGetResultsInner call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Update RUs per second of the an Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param updateThroughputParameters The RUs per second of the parameters to provide for the current MongoDB database.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the ThroughputSettingsGetResultsInner object
*/
public Observable> beginUpdateMongoDBDatabaseThroughputWithServiceResponseAsync(String resourceGroupName, String accountName, String databaseName, ThroughputSettingsUpdateParameters updateThroughputParameters) {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (accountName == null) {
throw new IllegalArgumentException("Parameter accountName is required and cannot be null.");
}
if (databaseName == null) {
throw new IllegalArgumentException("Parameter databaseName is required and cannot be null.");
}
if (updateThroughputParameters == null) {
throw new IllegalArgumentException("Parameter updateThroughputParameters is required and cannot be null.");
}
Validator.validate(updateThroughputParameters);
final String apiVersion = "2019-08-01";
return service.beginUpdateMongoDBDatabaseThroughput(this.client.subscriptionId(), resourceGroupName, accountName, databaseName, apiVersion, updateThroughputParameters, this.client.acceptLanguage(), this.client.userAgent())
.flatMap(new Func1, Observable>>() {
@Override
public Observable> call(Response response) {
try {
ServiceResponse clientResponse = beginUpdateMongoDBDatabaseThroughputDelegate(response);
return Observable.just(clientResponse);
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse beginUpdateMongoDBDatabaseThroughputDelegate(Response response) throws CloudException, IOException, IllegalArgumentException {
return this.client.restClient().responseBuilderFactory().newInstance(this.client.serializerAdapter())
.register(200, new TypeToken() { }.getType())
.register(202, new TypeToken() { }.getType())
.registerError(CloudException.class)
.build(response);
}
/**
* Lists the MongoDB collection under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the List<MongoDBCollectionGetResultsInner> object if successful.
*/
public List listMongoDBCollections(String resourceGroupName, String accountName, String databaseName) {
return listMongoDBCollectionsWithServiceResponseAsync(resourceGroupName, accountName, databaseName).toBlocking().single().body();
}
/**
* Lists the MongoDB collection under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture> listMongoDBCollectionsAsync(String resourceGroupName, String accountName, String databaseName, final ServiceCallback> serviceCallback) {
return ServiceFuture.fromResponse(listMongoDBCollectionsWithServiceResponseAsync(resourceGroupName, accountName, databaseName), serviceCallback);
}
/**
* Lists the MongoDB collection under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the List<MongoDBCollectionGetResultsInner> object
*/
public Observable> listMongoDBCollectionsAsync(String resourceGroupName, String accountName, String databaseName) {
return listMongoDBCollectionsWithServiceResponseAsync(resourceGroupName, accountName, databaseName).map(new Func1>, List>() {
@Override
public List call(ServiceResponse> response) {
return response.body();
}
});
}
/**
* Lists the MongoDB collection under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the List<MongoDBCollectionGetResultsInner> object
*/
public Observable>> listMongoDBCollectionsWithServiceResponseAsync(String resourceGroupName, String accountName, String databaseName) {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (accountName == null) {
throw new IllegalArgumentException("Parameter accountName is required and cannot be null.");
}
if (databaseName == null) {
throw new IllegalArgumentException("Parameter databaseName is required and cannot be null.");
}
final String apiVersion = "2019-08-01";
return service.listMongoDBCollections(this.client.subscriptionId(), resourceGroupName, accountName, databaseName, apiVersion, this.client.acceptLanguage(), this.client.userAgent())
.flatMap(new Func1, Observable>>>() {
@Override
public Observable>> call(Response response) {
try {
ServiceResponse> result = listMongoDBCollectionsDelegate(response);
List items = null;
if (result.body() != null) {
items = result.body().items();
}
ServiceResponse> clientResponse = new ServiceResponse>(items, result.response());
return Observable.just(clientResponse);
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse> listMongoDBCollectionsDelegate(Response response) throws CloudException, IOException, IllegalArgumentException {
return this.client.restClient().responseBuilderFactory()., CloudException>newInstance(this.client.serializerAdapter())
.register(200, new TypeToken>() { }.getType())
.registerError(CloudException.class)
.build(response);
}
/**
* Gets the MongoDB collection under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the MongoDBCollectionGetResultsInner object if successful.
*/
public MongoDBCollectionGetResultsInner getMongoDBCollection(String resourceGroupName, String accountName, String databaseName, String collectionName) {
return getMongoDBCollectionWithServiceResponseAsync(resourceGroupName, accountName, databaseName, collectionName).toBlocking().single().body();
}
/**
* Gets the MongoDB collection under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture getMongoDBCollectionAsync(String resourceGroupName, String accountName, String databaseName, String collectionName, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(getMongoDBCollectionWithServiceResponseAsync(resourceGroupName, accountName, databaseName, collectionName), serviceCallback);
}
/**
* Gets the MongoDB collection under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the MongoDBCollectionGetResultsInner object
*/
public Observable getMongoDBCollectionAsync(String resourceGroupName, String accountName, String databaseName, String collectionName) {
return getMongoDBCollectionWithServiceResponseAsync(resourceGroupName, accountName, databaseName, collectionName).map(new Func1, MongoDBCollectionGetResultsInner>() {
@Override
public MongoDBCollectionGetResultsInner call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Gets the MongoDB collection under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the MongoDBCollectionGetResultsInner object
*/
public Observable> getMongoDBCollectionWithServiceResponseAsync(String resourceGroupName, String accountName, String databaseName, String collectionName) {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (accountName == null) {
throw new IllegalArgumentException("Parameter accountName is required and cannot be null.");
}
if (databaseName == null) {
throw new IllegalArgumentException("Parameter databaseName is required and cannot be null.");
}
if (collectionName == null) {
throw new IllegalArgumentException("Parameter collectionName is required and cannot be null.");
}
final String apiVersion = "2019-08-01";
return service.getMongoDBCollection(this.client.subscriptionId(), resourceGroupName, accountName, databaseName, collectionName, apiVersion, this.client.acceptLanguage(), this.client.userAgent())
.flatMap(new Func1, Observable>>() {
@Override
public Observable> call(Response response) {
try {
ServiceResponse clientResponse = getMongoDBCollectionDelegate(response);
return Observable.just(clientResponse);
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse getMongoDBCollectionDelegate(Response response) throws CloudException, IOException, IllegalArgumentException {
return this.client.restClient().responseBuilderFactory().newInstance(this.client.serializerAdapter())
.register(200, new TypeToken() { }.getType())
.registerError(CloudException.class)
.build(response);
}
/**
* Create or update an Azure Cosmos DB MongoDB Collection.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param createUpdateMongoDBCollectionParameters The parameters to provide for the current MongoDB Collection.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the MongoDBCollectionGetResultsInner object if successful.
*/
public MongoDBCollectionGetResultsInner createUpdateMongoDBCollection(String resourceGroupName, String accountName, String databaseName, String collectionName, MongoDBCollectionCreateUpdateParameters createUpdateMongoDBCollectionParameters) {
return createUpdateMongoDBCollectionWithServiceResponseAsync(resourceGroupName, accountName, databaseName, collectionName, createUpdateMongoDBCollectionParameters).toBlocking().last().body();
}
/**
* Create or update an Azure Cosmos DB MongoDB Collection.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param createUpdateMongoDBCollectionParameters The parameters to provide for the current MongoDB Collection.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture createUpdateMongoDBCollectionAsync(String resourceGroupName, String accountName, String databaseName, String collectionName, MongoDBCollectionCreateUpdateParameters createUpdateMongoDBCollectionParameters, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(createUpdateMongoDBCollectionWithServiceResponseAsync(resourceGroupName, accountName, databaseName, collectionName, createUpdateMongoDBCollectionParameters), serviceCallback);
}
/**
* Create or update an Azure Cosmos DB MongoDB Collection.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param createUpdateMongoDBCollectionParameters The parameters to provide for the current MongoDB Collection.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable for the request
*/
public Observable createUpdateMongoDBCollectionAsync(String resourceGroupName, String accountName, String databaseName, String collectionName, MongoDBCollectionCreateUpdateParameters createUpdateMongoDBCollectionParameters) {
return createUpdateMongoDBCollectionWithServiceResponseAsync(resourceGroupName, accountName, databaseName, collectionName, createUpdateMongoDBCollectionParameters).map(new Func1, MongoDBCollectionGetResultsInner>() {
@Override
public MongoDBCollectionGetResultsInner call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Create or update an Azure Cosmos DB MongoDB Collection.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param createUpdateMongoDBCollectionParameters The parameters to provide for the current MongoDB Collection.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable for the request
*/
public Observable> createUpdateMongoDBCollectionWithServiceResponseAsync(String resourceGroupName, String accountName, String databaseName, String collectionName, MongoDBCollectionCreateUpdateParameters createUpdateMongoDBCollectionParameters) {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (accountName == null) {
throw new IllegalArgumentException("Parameter accountName is required and cannot be null.");
}
if (databaseName == null) {
throw new IllegalArgumentException("Parameter databaseName is required and cannot be null.");
}
if (collectionName == null) {
throw new IllegalArgumentException("Parameter collectionName is required and cannot be null.");
}
if (createUpdateMongoDBCollectionParameters == null) {
throw new IllegalArgumentException("Parameter createUpdateMongoDBCollectionParameters is required and cannot be null.");
}
Validator.validate(createUpdateMongoDBCollectionParameters);
final String apiVersion = "2019-08-01";
Observable> observable = service.createUpdateMongoDBCollection(this.client.subscriptionId(), resourceGroupName, accountName, databaseName, collectionName, apiVersion, createUpdateMongoDBCollectionParameters, this.client.acceptLanguage(), this.client.userAgent());
return client.getAzureClient().getPutOrPatchResultAsync(observable, new TypeToken() { }.getType());
}
/**
* Create or update an Azure Cosmos DB MongoDB Collection.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param createUpdateMongoDBCollectionParameters The parameters to provide for the current MongoDB Collection.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the MongoDBCollectionGetResultsInner object if successful.
*/
public MongoDBCollectionGetResultsInner beginCreateUpdateMongoDBCollection(String resourceGroupName, String accountName, String databaseName, String collectionName, MongoDBCollectionCreateUpdateParameters createUpdateMongoDBCollectionParameters) {
return beginCreateUpdateMongoDBCollectionWithServiceResponseAsync(resourceGroupName, accountName, databaseName, collectionName, createUpdateMongoDBCollectionParameters).toBlocking().single().body();
}
/**
* Create or update an Azure Cosmos DB MongoDB Collection.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param createUpdateMongoDBCollectionParameters The parameters to provide for the current MongoDB Collection.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture beginCreateUpdateMongoDBCollectionAsync(String resourceGroupName, String accountName, String databaseName, String collectionName, MongoDBCollectionCreateUpdateParameters createUpdateMongoDBCollectionParameters, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(beginCreateUpdateMongoDBCollectionWithServiceResponseAsync(resourceGroupName, accountName, databaseName, collectionName, createUpdateMongoDBCollectionParameters), serviceCallback);
}
/**
* Create or update an Azure Cosmos DB MongoDB Collection.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param createUpdateMongoDBCollectionParameters The parameters to provide for the current MongoDB Collection.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the MongoDBCollectionGetResultsInner object
*/
public Observable beginCreateUpdateMongoDBCollectionAsync(String resourceGroupName, String accountName, String databaseName, String collectionName, MongoDBCollectionCreateUpdateParameters createUpdateMongoDBCollectionParameters) {
return beginCreateUpdateMongoDBCollectionWithServiceResponseAsync(resourceGroupName, accountName, databaseName, collectionName, createUpdateMongoDBCollectionParameters).map(new Func1, MongoDBCollectionGetResultsInner>() {
@Override
public MongoDBCollectionGetResultsInner call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Create or update an Azure Cosmos DB MongoDB Collection.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param createUpdateMongoDBCollectionParameters The parameters to provide for the current MongoDB Collection.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the MongoDBCollectionGetResultsInner object
*/
public Observable> beginCreateUpdateMongoDBCollectionWithServiceResponseAsync(String resourceGroupName, String accountName, String databaseName, String collectionName, MongoDBCollectionCreateUpdateParameters createUpdateMongoDBCollectionParameters) {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (accountName == null) {
throw new IllegalArgumentException("Parameter accountName is required and cannot be null.");
}
if (databaseName == null) {
throw new IllegalArgumentException("Parameter databaseName is required and cannot be null.");
}
if (collectionName == null) {
throw new IllegalArgumentException("Parameter collectionName is required and cannot be null.");
}
if (createUpdateMongoDBCollectionParameters == null) {
throw new IllegalArgumentException("Parameter createUpdateMongoDBCollectionParameters is required and cannot be null.");
}
Validator.validate(createUpdateMongoDBCollectionParameters);
final String apiVersion = "2019-08-01";
return service.beginCreateUpdateMongoDBCollection(this.client.subscriptionId(), resourceGroupName, accountName, databaseName, collectionName, apiVersion, createUpdateMongoDBCollectionParameters, this.client.acceptLanguage(), this.client.userAgent())
.flatMap(new Func1, Observable>>() {
@Override
public Observable> call(Response response) {
try {
ServiceResponse clientResponse = beginCreateUpdateMongoDBCollectionDelegate(response);
return Observable.just(clientResponse);
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse beginCreateUpdateMongoDBCollectionDelegate(Response response) throws CloudException, IOException, IllegalArgumentException {
return this.client.restClient().responseBuilderFactory().newInstance(this.client.serializerAdapter())
.register(200, new TypeToken() { }.getType())
.register(202, new TypeToken() { }.getType())
.registerError(CloudException.class)
.build(response);
}
/**
* Deletes an existing Azure Cosmos DB MongoDB Collection.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
*/
public void deleteMongoDBCollection(String resourceGroupName, String accountName, String databaseName, String collectionName) {
deleteMongoDBCollectionWithServiceResponseAsync(resourceGroupName, accountName, databaseName, collectionName).toBlocking().last().body();
}
/**
* Deletes an existing Azure Cosmos DB MongoDB Collection.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture deleteMongoDBCollectionAsync(String resourceGroupName, String accountName, String databaseName, String collectionName, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(deleteMongoDBCollectionWithServiceResponseAsync(resourceGroupName, accountName, databaseName, collectionName), serviceCallback);
}
/**
* Deletes an existing Azure Cosmos DB MongoDB Collection.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable for the request
*/
public Observable deleteMongoDBCollectionAsync(String resourceGroupName, String accountName, String databaseName, String collectionName) {
return deleteMongoDBCollectionWithServiceResponseAsync(resourceGroupName, accountName, databaseName, collectionName).map(new Func1, Void>() {
@Override
public Void call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Deletes an existing Azure Cosmos DB MongoDB Collection.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable for the request
*/
public Observable> deleteMongoDBCollectionWithServiceResponseAsync(String resourceGroupName, String accountName, String databaseName, String collectionName) {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (accountName == null) {
throw new IllegalArgumentException("Parameter accountName is required and cannot be null.");
}
if (databaseName == null) {
throw new IllegalArgumentException("Parameter databaseName is required and cannot be null.");
}
if (collectionName == null) {
throw new IllegalArgumentException("Parameter collectionName is required and cannot be null.");
}
final String apiVersion = "2019-08-01";
Observable> observable = service.deleteMongoDBCollection(this.client.subscriptionId(), resourceGroupName, accountName, databaseName, collectionName, apiVersion, this.client.acceptLanguage(), this.client.userAgent());
return client.getAzureClient().getPostOrDeleteResultAsync(observable, new TypeToken() { }.getType());
}
/**
* Deletes an existing Azure Cosmos DB MongoDB Collection.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
*/
public void beginDeleteMongoDBCollection(String resourceGroupName, String accountName, String databaseName, String collectionName) {
beginDeleteMongoDBCollectionWithServiceResponseAsync(resourceGroupName, accountName, databaseName, collectionName).toBlocking().single().body();
}
/**
* Deletes an existing Azure Cosmos DB MongoDB Collection.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture beginDeleteMongoDBCollectionAsync(String resourceGroupName, String accountName, String databaseName, String collectionName, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(beginDeleteMongoDBCollectionWithServiceResponseAsync(resourceGroupName, accountName, databaseName, collectionName), serviceCallback);
}
/**
* Deletes an existing Azure Cosmos DB MongoDB Collection.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceResponse} object if successful.
*/
public Observable beginDeleteMongoDBCollectionAsync(String resourceGroupName, String accountName, String databaseName, String collectionName) {
return beginDeleteMongoDBCollectionWithServiceResponseAsync(resourceGroupName, accountName, databaseName, collectionName).map(new Func1, Void>() {
@Override
public Void call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Deletes an existing Azure Cosmos DB MongoDB Collection.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceResponse} object if successful.
*/
public Observable> beginDeleteMongoDBCollectionWithServiceResponseAsync(String resourceGroupName, String accountName, String databaseName, String collectionName) {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (accountName == null) {
throw new IllegalArgumentException("Parameter accountName is required and cannot be null.");
}
if (databaseName == null) {
throw new IllegalArgumentException("Parameter databaseName is required and cannot be null.");
}
if (collectionName == null) {
throw new IllegalArgumentException("Parameter collectionName is required and cannot be null.");
}
final String apiVersion = "2019-08-01";
return service.beginDeleteMongoDBCollection(this.client.subscriptionId(), resourceGroupName, accountName, databaseName, collectionName, apiVersion, this.client.acceptLanguage(), this.client.userAgent())
.flatMap(new Func1, Observable>>() {
@Override
public Observable> call(Response response) {
try {
ServiceResponse clientResponse = beginDeleteMongoDBCollectionDelegate(response);
return Observable.just(clientResponse);
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse beginDeleteMongoDBCollectionDelegate(Response response) throws CloudException, IOException, IllegalArgumentException {
return this.client.restClient().responseBuilderFactory().newInstance(this.client.serializerAdapter())
.register(202, new TypeToken() { }.getType())
.register(204, new TypeToken() { }.getType())
.registerError(CloudException.class)
.build(response);
}
/**
* Gets the RUs per second of the MongoDB collection under an existing Azure Cosmos DB database account with the provided name.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the ThroughputSettingsGetResultsInner object if successful.
*/
public ThroughputSettingsGetResultsInner getMongoDBCollectionThroughput(String resourceGroupName, String accountName, String databaseName, String collectionName) {
return getMongoDBCollectionThroughputWithServiceResponseAsync(resourceGroupName, accountName, databaseName, collectionName).toBlocking().single().body();
}
/**
* Gets the RUs per second of the MongoDB collection under an existing Azure Cosmos DB database account with the provided name.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture getMongoDBCollectionThroughputAsync(String resourceGroupName, String accountName, String databaseName, String collectionName, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(getMongoDBCollectionThroughputWithServiceResponseAsync(resourceGroupName, accountName, databaseName, collectionName), serviceCallback);
}
/**
* Gets the RUs per second of the MongoDB collection under an existing Azure Cosmos DB database account with the provided name.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the ThroughputSettingsGetResultsInner object
*/
public Observable getMongoDBCollectionThroughputAsync(String resourceGroupName, String accountName, String databaseName, String collectionName) {
return getMongoDBCollectionThroughputWithServiceResponseAsync(resourceGroupName, accountName, databaseName, collectionName).map(new Func1, ThroughputSettingsGetResultsInner>() {
@Override
public ThroughputSettingsGetResultsInner call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Gets the RUs per second of the MongoDB collection under an existing Azure Cosmos DB database account with the provided name.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the ThroughputSettingsGetResultsInner object
*/
public Observable> getMongoDBCollectionThroughputWithServiceResponseAsync(String resourceGroupName, String accountName, String databaseName, String collectionName) {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (accountName == null) {
throw new IllegalArgumentException("Parameter accountName is required and cannot be null.");
}
if (databaseName == null) {
throw new IllegalArgumentException("Parameter databaseName is required and cannot be null.");
}
if (collectionName == null) {
throw new IllegalArgumentException("Parameter collectionName is required and cannot be null.");
}
final String apiVersion = "2019-08-01";
return service.getMongoDBCollectionThroughput(this.client.subscriptionId(), resourceGroupName, accountName, databaseName, collectionName, apiVersion, this.client.acceptLanguage(), this.client.userAgent())
.flatMap(new Func1, Observable>>() {
@Override
public Observable> call(Response response) {
try {
ServiceResponse clientResponse = getMongoDBCollectionThroughputDelegate(response);
return Observable.just(clientResponse);
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse getMongoDBCollectionThroughputDelegate(Response response) throws CloudException, IOException, IllegalArgumentException {
return this.client.restClient().responseBuilderFactory().newInstance(this.client.serializerAdapter())
.register(200, new TypeToken() { }.getType())
.registerError(CloudException.class)
.build(response);
}
/**
* Update the RUs per second of an Azure Cosmos DB MongoDB collection.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param updateThroughputParameters The RUs per second of the parameters to provide for the current MongoDB collection.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the ThroughputSettingsGetResultsInner object if successful.
*/
public ThroughputSettingsGetResultsInner updateMongoDBCollectionThroughput(String resourceGroupName, String accountName, String databaseName, String collectionName, ThroughputSettingsUpdateParameters updateThroughputParameters) {
return updateMongoDBCollectionThroughputWithServiceResponseAsync(resourceGroupName, accountName, databaseName, collectionName, updateThroughputParameters).toBlocking().last().body();
}
/**
* Update the RUs per second of an Azure Cosmos DB MongoDB collection.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param updateThroughputParameters The RUs per second of the parameters to provide for the current MongoDB collection.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture updateMongoDBCollectionThroughputAsync(String resourceGroupName, String accountName, String databaseName, String collectionName, ThroughputSettingsUpdateParameters updateThroughputParameters, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(updateMongoDBCollectionThroughputWithServiceResponseAsync(resourceGroupName, accountName, databaseName, collectionName, updateThroughputParameters), serviceCallback);
}
/**
* Update the RUs per second of an Azure Cosmos DB MongoDB collection.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param updateThroughputParameters The RUs per second of the parameters to provide for the current MongoDB collection.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable for the request
*/
public Observable updateMongoDBCollectionThroughputAsync(String resourceGroupName, String accountName, String databaseName, String collectionName, ThroughputSettingsUpdateParameters updateThroughputParameters) {
return updateMongoDBCollectionThroughputWithServiceResponseAsync(resourceGroupName, accountName, databaseName, collectionName, updateThroughputParameters).map(new Func1, ThroughputSettingsGetResultsInner>() {
@Override
public ThroughputSettingsGetResultsInner call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Update the RUs per second of an Azure Cosmos DB MongoDB collection.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param updateThroughputParameters The RUs per second of the parameters to provide for the current MongoDB collection.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable for the request
*/
public Observable> updateMongoDBCollectionThroughputWithServiceResponseAsync(String resourceGroupName, String accountName, String databaseName, String collectionName, ThroughputSettingsUpdateParameters updateThroughputParameters) {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (accountName == null) {
throw new IllegalArgumentException("Parameter accountName is required and cannot be null.");
}
if (databaseName == null) {
throw new IllegalArgumentException("Parameter databaseName is required and cannot be null.");
}
if (collectionName == null) {
throw new IllegalArgumentException("Parameter collectionName is required and cannot be null.");
}
if (updateThroughputParameters == null) {
throw new IllegalArgumentException("Parameter updateThroughputParameters is required and cannot be null.");
}
Validator.validate(updateThroughputParameters);
final String apiVersion = "2019-08-01";
Observable> observable = service.updateMongoDBCollectionThroughput(this.client.subscriptionId(), resourceGroupName, accountName, databaseName, collectionName, apiVersion, updateThroughputParameters, this.client.acceptLanguage(), this.client.userAgent());
return client.getAzureClient().getPutOrPatchResultAsync(observable, new TypeToken() { }.getType());
}
/**
* Update the RUs per second of an Azure Cosmos DB MongoDB collection.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param updateThroughputParameters The RUs per second of the parameters to provide for the current MongoDB collection.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the ThroughputSettingsGetResultsInner object if successful.
*/
public ThroughputSettingsGetResultsInner beginUpdateMongoDBCollectionThroughput(String resourceGroupName, String accountName, String databaseName, String collectionName, ThroughputSettingsUpdateParameters updateThroughputParameters) {
return beginUpdateMongoDBCollectionThroughputWithServiceResponseAsync(resourceGroupName, accountName, databaseName, collectionName, updateThroughputParameters).toBlocking().single().body();
}
/**
* Update the RUs per second of an Azure Cosmos DB MongoDB collection.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param updateThroughputParameters The RUs per second of the parameters to provide for the current MongoDB collection.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture beginUpdateMongoDBCollectionThroughputAsync(String resourceGroupName, String accountName, String databaseName, String collectionName, ThroughputSettingsUpdateParameters updateThroughputParameters, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(beginUpdateMongoDBCollectionThroughputWithServiceResponseAsync(resourceGroupName, accountName, databaseName, collectionName, updateThroughputParameters), serviceCallback);
}
/**
* Update the RUs per second of an Azure Cosmos DB MongoDB collection.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param updateThroughputParameters The RUs per second of the parameters to provide for the current MongoDB collection.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the ThroughputSettingsGetResultsInner object
*/
public Observable