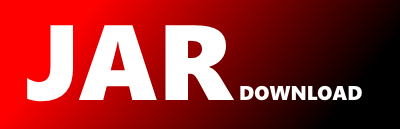
com.microsoft.azure.management.cosmosdb.implementation.SqlResourcesInner Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-mgmt-cosmosdb Show documentation
Show all versions of azure-mgmt-cosmosdb Show documentation
This package contains Microsoft Azure CosmosDB SDK. A new set of management libraries are now Generally Available. For documentation on how to use the new libraries, please see https://aka.ms/azsdk/java/mgmt
/**
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for
* license information.
*
* Code generated by Microsoft (R) AutoRest Code Generator.
*/
package com.microsoft.azure.management.cosmosdb.implementation;
import retrofit2.Retrofit;
import com.google.common.reflect.TypeToken;
import com.microsoft.azure.CloudException;
import com.microsoft.azure.management.cosmosdb.SqlContainerCreateUpdateParameters;
import com.microsoft.azure.management.cosmosdb.SqlDatabaseCreateUpdateParameters;
import com.microsoft.azure.management.cosmosdb.SqlStoredProcedureCreateUpdateParameters;
import com.microsoft.azure.management.cosmosdb.SqlTriggerCreateUpdateParameters;
import com.microsoft.azure.management.cosmosdb.SqlUserDefinedFunctionCreateUpdateParameters;
import com.microsoft.azure.management.cosmosdb.ThroughputSettingsUpdateParameters;
import com.microsoft.rest.ServiceCallback;
import com.microsoft.rest.ServiceFuture;
import com.microsoft.rest.ServiceResponse;
import com.microsoft.rest.Validator;
import java.io.IOException;
import java.util.List;
import okhttp3.ResponseBody;
import retrofit2.http.Body;
import retrofit2.http.GET;
import retrofit2.http.Header;
import retrofit2.http.Headers;
import retrofit2.http.HTTP;
import retrofit2.http.Path;
import retrofit2.http.PUT;
import retrofit2.http.Query;
import retrofit2.Response;
import rx.functions.Func1;
import rx.Observable;
/**
* An instance of this class provides access to all the operations defined
* in SqlResources.
*/
public class SqlResourcesInner {
/** The Retrofit service to perform REST calls. */
private SqlResourcesService service;
/** The service client containing this operation class. */
private CosmosDBImpl client;
/**
* Initializes an instance of SqlResourcesInner.
*
* @param retrofit the Retrofit instance built from a Retrofit Builder.
* @param client the instance of the service client containing this operation class.
*/
public SqlResourcesInner(Retrofit retrofit, CosmosDBImpl client) {
this.service = retrofit.create(SqlResourcesService.class);
this.client = client;
}
/**
* The interface defining all the services for SqlResources to be
* used by Retrofit to perform actually REST calls.
*/
interface SqlResourcesService {
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.SqlResources listSqlDatabases" })
@GET("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/sqlDatabases")
Observable> listSqlDatabases(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.SqlResources getSqlDatabase" })
@GET("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/sqlDatabases/{databaseName}")
Observable> getSqlDatabase(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Path("databaseName") String databaseName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.SqlResources createUpdateSqlDatabase" })
@PUT("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/sqlDatabases/{databaseName}")
Observable> createUpdateSqlDatabase(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Path("databaseName") String databaseName, @Query("api-version") String apiVersion, @Body SqlDatabaseCreateUpdateParameters createUpdateSqlDatabaseParameters, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.SqlResources beginCreateUpdateSqlDatabase" })
@PUT("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/sqlDatabases/{databaseName}")
Observable> beginCreateUpdateSqlDatabase(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Path("databaseName") String databaseName, @Query("api-version") String apiVersion, @Body SqlDatabaseCreateUpdateParameters createUpdateSqlDatabaseParameters, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.SqlResources deleteSqlDatabase" })
@HTTP(path = "subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/sqlDatabases/{databaseName}", method = "DELETE", hasBody = true)
Observable> deleteSqlDatabase(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Path("databaseName") String databaseName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.SqlResources beginDeleteSqlDatabase" })
@HTTP(path = "subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/sqlDatabases/{databaseName}", method = "DELETE", hasBody = true)
Observable> beginDeleteSqlDatabase(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Path("databaseName") String databaseName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.SqlResources getSqlDatabaseThroughput" })
@GET("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/sqlDatabases/{databaseName}/throughputSettings/default")
Observable> getSqlDatabaseThroughput(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Path("databaseName") String databaseName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.SqlResources updateSqlDatabaseThroughput" })
@PUT("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/sqlDatabases/{databaseName}/throughputSettings/default")
Observable> updateSqlDatabaseThroughput(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Path("databaseName") String databaseName, @Query("api-version") String apiVersion, @Body ThroughputSettingsUpdateParameters updateThroughputParameters, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.SqlResources beginUpdateSqlDatabaseThroughput" })
@PUT("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/sqlDatabases/{databaseName}/throughputSettings/default")
Observable> beginUpdateSqlDatabaseThroughput(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Path("databaseName") String databaseName, @Query("api-version") String apiVersion, @Body ThroughputSettingsUpdateParameters updateThroughputParameters, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.SqlResources listSqlContainers" })
@GET("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/sqlDatabases/{databaseName}/containers")
Observable> listSqlContainers(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Path("databaseName") String databaseName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.SqlResources getSqlContainer" })
@GET("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/sqlDatabases/{databaseName}/containers/{containerName}")
Observable> getSqlContainer(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Path("databaseName") String databaseName, @Path("containerName") String containerName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.SqlResources createUpdateSqlContainer" })
@PUT("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/sqlDatabases/{databaseName}/containers/{containerName}")
Observable> createUpdateSqlContainer(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Path("databaseName") String databaseName, @Path("containerName") String containerName, @Query("api-version") String apiVersion, @Body SqlContainerCreateUpdateParameters createUpdateSqlContainerParameters, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.SqlResources beginCreateUpdateSqlContainer" })
@PUT("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/sqlDatabases/{databaseName}/containers/{containerName}")
Observable> beginCreateUpdateSqlContainer(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Path("databaseName") String databaseName, @Path("containerName") String containerName, @Query("api-version") String apiVersion, @Body SqlContainerCreateUpdateParameters createUpdateSqlContainerParameters, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.SqlResources deleteSqlContainer" })
@HTTP(path = "subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/sqlDatabases/{databaseName}/containers/{containerName}", method = "DELETE", hasBody = true)
Observable> deleteSqlContainer(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Path("databaseName") String databaseName, @Path("containerName") String containerName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.SqlResources beginDeleteSqlContainer" })
@HTTP(path = "subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/sqlDatabases/{databaseName}/containers/{containerName}", method = "DELETE", hasBody = true)
Observable> beginDeleteSqlContainer(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Path("databaseName") String databaseName, @Path("containerName") String containerName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.SqlResources getSqlContainerThroughput" })
@GET("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/sqlDatabases/{databaseName}/containers/{containerName}/throughputSettings/default")
Observable> getSqlContainerThroughput(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Path("databaseName") String databaseName, @Path("containerName") String containerName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.SqlResources updateSqlContainerThroughput" })
@PUT("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/sqlDatabases/{databaseName}/containers/{containerName}/throughputSettings/default")
Observable> updateSqlContainerThroughput(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Path("databaseName") String databaseName, @Path("containerName") String containerName, @Query("api-version") String apiVersion, @Body ThroughputSettingsUpdateParameters updateThroughputParameters, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.SqlResources beginUpdateSqlContainerThroughput" })
@PUT("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/sqlDatabases/{databaseName}/containers/{containerName}/throughputSettings/default")
Observable> beginUpdateSqlContainerThroughput(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Path("databaseName") String databaseName, @Path("containerName") String containerName, @Query("api-version") String apiVersion, @Body ThroughputSettingsUpdateParameters updateThroughputParameters, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.SqlResources listSqlStoredProcedures" })
@GET("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/sqlDatabases/{databaseName}/containers/{containerName}/storedProcedures/")
Observable> listSqlStoredProcedures(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Path("databaseName") String databaseName, @Path("containerName") String containerName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.SqlResources getSqlStoredProcedure" })
@GET("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/sqlDatabases/{databaseName}/containers/{containerName}/storedProcedures/{storedProcedureName}")
Observable> getSqlStoredProcedure(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Path("databaseName") String databaseName, @Path("containerName") String containerName, @Path("storedProcedureName") String storedProcedureName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.SqlResources createUpdateSqlStoredProcedure" })
@PUT("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/sqlDatabases/{databaseName}/containers/{containerName}/storedProcedures/{storedProcedureName}")
Observable> createUpdateSqlStoredProcedure(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Path("databaseName") String databaseName, @Path("containerName") String containerName, @Path("storedProcedureName") String storedProcedureName, @Query("api-version") String apiVersion, @Body SqlStoredProcedureCreateUpdateParameters createUpdateSqlStoredProcedureParameters, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.SqlResources beginCreateUpdateSqlStoredProcedure" })
@PUT("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/sqlDatabases/{databaseName}/containers/{containerName}/storedProcedures/{storedProcedureName}")
Observable> beginCreateUpdateSqlStoredProcedure(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Path("databaseName") String databaseName, @Path("containerName") String containerName, @Path("storedProcedureName") String storedProcedureName, @Query("api-version") String apiVersion, @Body SqlStoredProcedureCreateUpdateParameters createUpdateSqlStoredProcedureParameters, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.SqlResources deleteSqlStoredProcedure" })
@HTTP(path = "subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/sqlDatabases/{databaseName}/containers/{containerName}/storedProcedures/{storedProcedureName}", method = "DELETE", hasBody = true)
Observable> deleteSqlStoredProcedure(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Path("databaseName") String databaseName, @Path("containerName") String containerName, @Path("storedProcedureName") String storedProcedureName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.SqlResources beginDeleteSqlStoredProcedure" })
@HTTP(path = "subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/sqlDatabases/{databaseName}/containers/{containerName}/storedProcedures/{storedProcedureName}", method = "DELETE", hasBody = true)
Observable> beginDeleteSqlStoredProcedure(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Path("databaseName") String databaseName, @Path("containerName") String containerName, @Path("storedProcedureName") String storedProcedureName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.SqlResources listSqlUserDefinedFunctions" })
@GET("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/sqlDatabases/{databaseName}/containers/{containerName}/userDefinedFunctions/")
Observable> listSqlUserDefinedFunctions(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Path("databaseName") String databaseName, @Path("containerName") String containerName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.SqlResources getSqlUserDefinedFunction" })
@GET("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/sqlDatabases/{databaseName}/containers/{containerName}/userDefinedFunctions/{userDefinedFunctionName}")
Observable> getSqlUserDefinedFunction(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Path("databaseName") String databaseName, @Path("containerName") String containerName, @Path("userDefinedFunctionName") String userDefinedFunctionName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.SqlResources createUpdateSqlUserDefinedFunction" })
@PUT("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/sqlDatabases/{databaseName}/containers/{containerName}/userDefinedFunctions/{userDefinedFunctionName}")
Observable> createUpdateSqlUserDefinedFunction(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Path("databaseName") String databaseName, @Path("containerName") String containerName, @Path("userDefinedFunctionName") String userDefinedFunctionName, @Query("api-version") String apiVersion, @Body SqlUserDefinedFunctionCreateUpdateParameters createUpdateSqlUserDefinedFunctionParameters, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.SqlResources beginCreateUpdateSqlUserDefinedFunction" })
@PUT("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/sqlDatabases/{databaseName}/containers/{containerName}/userDefinedFunctions/{userDefinedFunctionName}")
Observable> beginCreateUpdateSqlUserDefinedFunction(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Path("databaseName") String databaseName, @Path("containerName") String containerName, @Path("userDefinedFunctionName") String userDefinedFunctionName, @Query("api-version") String apiVersion, @Body SqlUserDefinedFunctionCreateUpdateParameters createUpdateSqlUserDefinedFunctionParameters, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.SqlResources deleteSqlUserDefinedFunction" })
@HTTP(path = "subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/sqlDatabases/{databaseName}/containers/{containerName}/userDefinedFunctions/{userDefinedFunctionName}", method = "DELETE", hasBody = true)
Observable> deleteSqlUserDefinedFunction(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Path("databaseName") String databaseName, @Path("containerName") String containerName, @Path("userDefinedFunctionName") String userDefinedFunctionName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.SqlResources beginDeleteSqlUserDefinedFunction" })
@HTTP(path = "subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/sqlDatabases/{databaseName}/containers/{containerName}/userDefinedFunctions/{userDefinedFunctionName}", method = "DELETE", hasBody = true)
Observable> beginDeleteSqlUserDefinedFunction(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Path("databaseName") String databaseName, @Path("containerName") String containerName, @Path("userDefinedFunctionName") String userDefinedFunctionName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.SqlResources listSqlTriggers" })
@GET("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/sqlDatabases/{databaseName}/containers/{containerName}/triggers/")
Observable> listSqlTriggers(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Path("databaseName") String databaseName, @Path("containerName") String containerName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.SqlResources getSqlTrigger" })
@GET("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/sqlDatabases/{databaseName}/containers/{containerName}/triggers/{triggerName}")
Observable> getSqlTrigger(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Path("databaseName") String databaseName, @Path("containerName") String containerName, @Path("triggerName") String triggerName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.SqlResources createUpdateSqlTrigger" })
@PUT("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/sqlDatabases/{databaseName}/containers/{containerName}/triggers/{triggerName}")
Observable> createUpdateSqlTrigger(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Path("databaseName") String databaseName, @Path("containerName") String containerName, @Path("triggerName") String triggerName, @Query("api-version") String apiVersion, @Body SqlTriggerCreateUpdateParameters createUpdateSqlTriggerParameters, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.SqlResources beginCreateUpdateSqlTrigger" })
@PUT("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/sqlDatabases/{databaseName}/containers/{containerName}/triggers/{triggerName}")
Observable> beginCreateUpdateSqlTrigger(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Path("databaseName") String databaseName, @Path("containerName") String containerName, @Path("triggerName") String triggerName, @Query("api-version") String apiVersion, @Body SqlTriggerCreateUpdateParameters createUpdateSqlTriggerParameters, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.SqlResources deleteSqlTrigger" })
@HTTP(path = "subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/sqlDatabases/{databaseName}/containers/{containerName}/triggers/{triggerName}", method = "DELETE", hasBody = true)
Observable> deleteSqlTrigger(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Path("databaseName") String databaseName, @Path("containerName") String containerName, @Path("triggerName") String triggerName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.cosmosdb.SqlResources beginDeleteSqlTrigger" })
@HTTP(path = "subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/sqlDatabases/{databaseName}/containers/{containerName}/triggers/{triggerName}", method = "DELETE", hasBody = true)
Observable> beginDeleteSqlTrigger(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("accountName") String accountName, @Path("databaseName") String databaseName, @Path("containerName") String containerName, @Path("triggerName") String triggerName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
}
/**
* Lists the SQL databases under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the List<SqlDatabaseGetResultsInner> object if successful.
*/
public List listSqlDatabases(String resourceGroupName, String accountName) {
return listSqlDatabasesWithServiceResponseAsync(resourceGroupName, accountName).toBlocking().single().body();
}
/**
* Lists the SQL databases under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture> listSqlDatabasesAsync(String resourceGroupName, String accountName, final ServiceCallback> serviceCallback) {
return ServiceFuture.fromResponse(listSqlDatabasesWithServiceResponseAsync(resourceGroupName, accountName), serviceCallback);
}
/**
* Lists the SQL databases under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the List<SqlDatabaseGetResultsInner> object
*/
public Observable> listSqlDatabasesAsync(String resourceGroupName, String accountName) {
return listSqlDatabasesWithServiceResponseAsync(resourceGroupName, accountName).map(new Func1>, List>() {
@Override
public List call(ServiceResponse> response) {
return response.body();
}
});
}
/**
* Lists the SQL databases under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the List<SqlDatabaseGetResultsInner> object
*/
public Observable>> listSqlDatabasesWithServiceResponseAsync(String resourceGroupName, String accountName) {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (accountName == null) {
throw new IllegalArgumentException("Parameter accountName is required and cannot be null.");
}
final String apiVersion = "2019-08-01";
return service.listSqlDatabases(this.client.subscriptionId(), resourceGroupName, accountName, apiVersion, this.client.acceptLanguage(), this.client.userAgent())
.flatMap(new Func1, Observable>>>() {
@Override
public Observable>> call(Response response) {
try {
ServiceResponse> result = listSqlDatabasesDelegate(response);
List items = null;
if (result.body() != null) {
items = result.body().items();
}
ServiceResponse> clientResponse = new ServiceResponse>(items, result.response());
return Observable.just(clientResponse);
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse> listSqlDatabasesDelegate(Response response) throws CloudException, IOException, IllegalArgumentException {
return this.client.restClient().responseBuilderFactory()., CloudException>newInstance(this.client.serializerAdapter())
.register(200, new TypeToken>() { }.getType())
.registerError(CloudException.class)
.build(response);
}
/**
* Gets the SQL database under an existing Azure Cosmos DB database account with the provided name.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the SqlDatabaseGetResultsInner object if successful.
*/
public SqlDatabaseGetResultsInner getSqlDatabase(String resourceGroupName, String accountName, String databaseName) {
return getSqlDatabaseWithServiceResponseAsync(resourceGroupName, accountName, databaseName).toBlocking().single().body();
}
/**
* Gets the SQL database under an existing Azure Cosmos DB database account with the provided name.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture getSqlDatabaseAsync(String resourceGroupName, String accountName, String databaseName, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(getSqlDatabaseWithServiceResponseAsync(resourceGroupName, accountName, databaseName), serviceCallback);
}
/**
* Gets the SQL database under an existing Azure Cosmos DB database account with the provided name.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the SqlDatabaseGetResultsInner object
*/
public Observable getSqlDatabaseAsync(String resourceGroupName, String accountName, String databaseName) {
return getSqlDatabaseWithServiceResponseAsync(resourceGroupName, accountName, databaseName).map(new Func1, SqlDatabaseGetResultsInner>() {
@Override
public SqlDatabaseGetResultsInner call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Gets the SQL database under an existing Azure Cosmos DB database account with the provided name.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the SqlDatabaseGetResultsInner object
*/
public Observable> getSqlDatabaseWithServiceResponseAsync(String resourceGroupName, String accountName, String databaseName) {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (accountName == null) {
throw new IllegalArgumentException("Parameter accountName is required and cannot be null.");
}
if (databaseName == null) {
throw new IllegalArgumentException("Parameter databaseName is required and cannot be null.");
}
final String apiVersion = "2019-08-01";
return service.getSqlDatabase(this.client.subscriptionId(), resourceGroupName, accountName, databaseName, apiVersion, this.client.acceptLanguage(), this.client.userAgent())
.flatMap(new Func1, Observable>>() {
@Override
public Observable> call(Response response) {
try {
ServiceResponse clientResponse = getSqlDatabaseDelegate(response);
return Observable.just(clientResponse);
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse getSqlDatabaseDelegate(Response response) throws CloudException, IOException, IllegalArgumentException {
return this.client.restClient().responseBuilderFactory().newInstance(this.client.serializerAdapter())
.register(200, new TypeToken() { }.getType())
.registerError(CloudException.class)
.build(response);
}
/**
* Create or update an Azure Cosmos DB SQL database.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param createUpdateSqlDatabaseParameters The parameters to provide for the current SQL database.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the SqlDatabaseGetResultsInner object if successful.
*/
public SqlDatabaseGetResultsInner createUpdateSqlDatabase(String resourceGroupName, String accountName, String databaseName, SqlDatabaseCreateUpdateParameters createUpdateSqlDatabaseParameters) {
return createUpdateSqlDatabaseWithServiceResponseAsync(resourceGroupName, accountName, databaseName, createUpdateSqlDatabaseParameters).toBlocking().last().body();
}
/**
* Create or update an Azure Cosmos DB SQL database.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param createUpdateSqlDatabaseParameters The parameters to provide for the current SQL database.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture createUpdateSqlDatabaseAsync(String resourceGroupName, String accountName, String databaseName, SqlDatabaseCreateUpdateParameters createUpdateSqlDatabaseParameters, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(createUpdateSqlDatabaseWithServiceResponseAsync(resourceGroupName, accountName, databaseName, createUpdateSqlDatabaseParameters), serviceCallback);
}
/**
* Create or update an Azure Cosmos DB SQL database.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param createUpdateSqlDatabaseParameters The parameters to provide for the current SQL database.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable for the request
*/
public Observable createUpdateSqlDatabaseAsync(String resourceGroupName, String accountName, String databaseName, SqlDatabaseCreateUpdateParameters createUpdateSqlDatabaseParameters) {
return createUpdateSqlDatabaseWithServiceResponseAsync(resourceGroupName, accountName, databaseName, createUpdateSqlDatabaseParameters).map(new Func1, SqlDatabaseGetResultsInner>() {
@Override
public SqlDatabaseGetResultsInner call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Create or update an Azure Cosmos DB SQL database.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param createUpdateSqlDatabaseParameters The parameters to provide for the current SQL database.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable for the request
*/
public Observable> createUpdateSqlDatabaseWithServiceResponseAsync(String resourceGroupName, String accountName, String databaseName, SqlDatabaseCreateUpdateParameters createUpdateSqlDatabaseParameters) {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (accountName == null) {
throw new IllegalArgumentException("Parameter accountName is required and cannot be null.");
}
if (databaseName == null) {
throw new IllegalArgumentException("Parameter databaseName is required and cannot be null.");
}
if (createUpdateSqlDatabaseParameters == null) {
throw new IllegalArgumentException("Parameter createUpdateSqlDatabaseParameters is required and cannot be null.");
}
Validator.validate(createUpdateSqlDatabaseParameters);
final String apiVersion = "2019-08-01";
Observable> observable = service.createUpdateSqlDatabase(this.client.subscriptionId(), resourceGroupName, accountName, databaseName, apiVersion, createUpdateSqlDatabaseParameters, this.client.acceptLanguage(), this.client.userAgent());
return client.getAzureClient().getPutOrPatchResultAsync(observable, new TypeToken() { }.getType());
}
/**
* Create or update an Azure Cosmos DB SQL database.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param createUpdateSqlDatabaseParameters The parameters to provide for the current SQL database.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the SqlDatabaseGetResultsInner object if successful.
*/
public SqlDatabaseGetResultsInner beginCreateUpdateSqlDatabase(String resourceGroupName, String accountName, String databaseName, SqlDatabaseCreateUpdateParameters createUpdateSqlDatabaseParameters) {
return beginCreateUpdateSqlDatabaseWithServiceResponseAsync(resourceGroupName, accountName, databaseName, createUpdateSqlDatabaseParameters).toBlocking().single().body();
}
/**
* Create or update an Azure Cosmos DB SQL database.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param createUpdateSqlDatabaseParameters The parameters to provide for the current SQL database.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture beginCreateUpdateSqlDatabaseAsync(String resourceGroupName, String accountName, String databaseName, SqlDatabaseCreateUpdateParameters createUpdateSqlDatabaseParameters, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(beginCreateUpdateSqlDatabaseWithServiceResponseAsync(resourceGroupName, accountName, databaseName, createUpdateSqlDatabaseParameters), serviceCallback);
}
/**
* Create or update an Azure Cosmos DB SQL database.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param createUpdateSqlDatabaseParameters The parameters to provide for the current SQL database.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the SqlDatabaseGetResultsInner object
*/
public Observable beginCreateUpdateSqlDatabaseAsync(String resourceGroupName, String accountName, String databaseName, SqlDatabaseCreateUpdateParameters createUpdateSqlDatabaseParameters) {
return beginCreateUpdateSqlDatabaseWithServiceResponseAsync(resourceGroupName, accountName, databaseName, createUpdateSqlDatabaseParameters).map(new Func1, SqlDatabaseGetResultsInner>() {
@Override
public SqlDatabaseGetResultsInner call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Create or update an Azure Cosmos DB SQL database.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param createUpdateSqlDatabaseParameters The parameters to provide for the current SQL database.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the SqlDatabaseGetResultsInner object
*/
public Observable> beginCreateUpdateSqlDatabaseWithServiceResponseAsync(String resourceGroupName, String accountName, String databaseName, SqlDatabaseCreateUpdateParameters createUpdateSqlDatabaseParameters) {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (accountName == null) {
throw new IllegalArgumentException("Parameter accountName is required and cannot be null.");
}
if (databaseName == null) {
throw new IllegalArgumentException("Parameter databaseName is required and cannot be null.");
}
if (createUpdateSqlDatabaseParameters == null) {
throw new IllegalArgumentException("Parameter createUpdateSqlDatabaseParameters is required and cannot be null.");
}
Validator.validate(createUpdateSqlDatabaseParameters);
final String apiVersion = "2019-08-01";
return service.beginCreateUpdateSqlDatabase(this.client.subscriptionId(), resourceGroupName, accountName, databaseName, apiVersion, createUpdateSqlDatabaseParameters, this.client.acceptLanguage(), this.client.userAgent())
.flatMap(new Func1, Observable>>() {
@Override
public Observable> call(Response response) {
try {
ServiceResponse clientResponse = beginCreateUpdateSqlDatabaseDelegate(response);
return Observable.just(clientResponse);
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse beginCreateUpdateSqlDatabaseDelegate(Response response) throws CloudException, IOException, IllegalArgumentException {
return this.client.restClient().responseBuilderFactory().newInstance(this.client.serializerAdapter())
.register(200, new TypeToken() { }.getType())
.register(202, new TypeToken() { }.getType())
.registerError(CloudException.class)
.build(response);
}
/**
* Deletes an existing Azure Cosmos DB SQL database.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
*/
public void deleteSqlDatabase(String resourceGroupName, String accountName, String databaseName) {
deleteSqlDatabaseWithServiceResponseAsync(resourceGroupName, accountName, databaseName).toBlocking().last().body();
}
/**
* Deletes an existing Azure Cosmos DB SQL database.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture deleteSqlDatabaseAsync(String resourceGroupName, String accountName, String databaseName, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(deleteSqlDatabaseWithServiceResponseAsync(resourceGroupName, accountName, databaseName), serviceCallback);
}
/**
* Deletes an existing Azure Cosmos DB SQL database.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable for the request
*/
public Observable deleteSqlDatabaseAsync(String resourceGroupName, String accountName, String databaseName) {
return deleteSqlDatabaseWithServiceResponseAsync(resourceGroupName, accountName, databaseName).map(new Func1, Void>() {
@Override
public Void call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Deletes an existing Azure Cosmos DB SQL database.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable for the request
*/
public Observable> deleteSqlDatabaseWithServiceResponseAsync(String resourceGroupName, String accountName, String databaseName) {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (accountName == null) {
throw new IllegalArgumentException("Parameter accountName is required and cannot be null.");
}
if (databaseName == null) {
throw new IllegalArgumentException("Parameter databaseName is required and cannot be null.");
}
final String apiVersion = "2019-08-01";
Observable> observable = service.deleteSqlDatabase(this.client.subscriptionId(), resourceGroupName, accountName, databaseName, apiVersion, this.client.acceptLanguage(), this.client.userAgent());
return client.getAzureClient().getPostOrDeleteResultAsync(observable, new TypeToken() { }.getType());
}
/**
* Deletes an existing Azure Cosmos DB SQL database.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
*/
public void beginDeleteSqlDatabase(String resourceGroupName, String accountName, String databaseName) {
beginDeleteSqlDatabaseWithServiceResponseAsync(resourceGroupName, accountName, databaseName).toBlocking().single().body();
}
/**
* Deletes an existing Azure Cosmos DB SQL database.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture beginDeleteSqlDatabaseAsync(String resourceGroupName, String accountName, String databaseName, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(beginDeleteSqlDatabaseWithServiceResponseAsync(resourceGroupName, accountName, databaseName), serviceCallback);
}
/**
* Deletes an existing Azure Cosmos DB SQL database.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceResponse} object if successful.
*/
public Observable beginDeleteSqlDatabaseAsync(String resourceGroupName, String accountName, String databaseName) {
return beginDeleteSqlDatabaseWithServiceResponseAsync(resourceGroupName, accountName, databaseName).map(new Func1, Void>() {
@Override
public Void call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Deletes an existing Azure Cosmos DB SQL database.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceResponse} object if successful.
*/
public Observable> beginDeleteSqlDatabaseWithServiceResponseAsync(String resourceGroupName, String accountName, String databaseName) {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (accountName == null) {
throw new IllegalArgumentException("Parameter accountName is required and cannot be null.");
}
if (databaseName == null) {
throw new IllegalArgumentException("Parameter databaseName is required and cannot be null.");
}
final String apiVersion = "2019-08-01";
return service.beginDeleteSqlDatabase(this.client.subscriptionId(), resourceGroupName, accountName, databaseName, apiVersion, this.client.acceptLanguage(), this.client.userAgent())
.flatMap(new Func1, Observable>>() {
@Override
public Observable> call(Response response) {
try {
ServiceResponse clientResponse = beginDeleteSqlDatabaseDelegate(response);
return Observable.just(clientResponse);
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse beginDeleteSqlDatabaseDelegate(Response response) throws CloudException, IOException, IllegalArgumentException {
return this.client.restClient().responseBuilderFactory().newInstance(this.client.serializerAdapter())
.register(202, new TypeToken() { }.getType())
.register(204, new TypeToken() { }.getType())
.registerError(CloudException.class)
.build(response);
}
/**
* Gets the RUs per second of the SQL database under an existing Azure Cosmos DB database account with the provided name.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the ThroughputSettingsGetResultsInner object if successful.
*/
public ThroughputSettingsGetResultsInner getSqlDatabaseThroughput(String resourceGroupName, String accountName, String databaseName) {
return getSqlDatabaseThroughputWithServiceResponseAsync(resourceGroupName, accountName, databaseName).toBlocking().single().body();
}
/**
* Gets the RUs per second of the SQL database under an existing Azure Cosmos DB database account with the provided name.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture getSqlDatabaseThroughputAsync(String resourceGroupName, String accountName, String databaseName, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(getSqlDatabaseThroughputWithServiceResponseAsync(resourceGroupName, accountName, databaseName), serviceCallback);
}
/**
* Gets the RUs per second of the SQL database under an existing Azure Cosmos DB database account with the provided name.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the ThroughputSettingsGetResultsInner object
*/
public Observable getSqlDatabaseThroughputAsync(String resourceGroupName, String accountName, String databaseName) {
return getSqlDatabaseThroughputWithServiceResponseAsync(resourceGroupName, accountName, databaseName).map(new Func1, ThroughputSettingsGetResultsInner>() {
@Override
public ThroughputSettingsGetResultsInner call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Gets the RUs per second of the SQL database under an existing Azure Cosmos DB database account with the provided name.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the ThroughputSettingsGetResultsInner object
*/
public Observable> getSqlDatabaseThroughputWithServiceResponseAsync(String resourceGroupName, String accountName, String databaseName) {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (accountName == null) {
throw new IllegalArgumentException("Parameter accountName is required and cannot be null.");
}
if (databaseName == null) {
throw new IllegalArgumentException("Parameter databaseName is required and cannot be null.");
}
final String apiVersion = "2019-08-01";
return service.getSqlDatabaseThroughput(this.client.subscriptionId(), resourceGroupName, accountName, databaseName, apiVersion, this.client.acceptLanguage(), this.client.userAgent())
.flatMap(new Func1, Observable>>() {
@Override
public Observable> call(Response response) {
try {
ServiceResponse clientResponse = getSqlDatabaseThroughputDelegate(response);
return Observable.just(clientResponse);
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse getSqlDatabaseThroughputDelegate(Response response) throws CloudException, IOException, IllegalArgumentException {
return this.client.restClient().responseBuilderFactory().newInstance(this.client.serializerAdapter())
.register(200, new TypeToken() { }.getType())
.registerError(CloudException.class)
.build(response);
}
/**
* Update RUs per second of an Azure Cosmos DB SQL database.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param updateThroughputParameters The parameters to provide for the RUs per second of the current SQL database.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the ThroughputSettingsGetResultsInner object if successful.
*/
public ThroughputSettingsGetResultsInner updateSqlDatabaseThroughput(String resourceGroupName, String accountName, String databaseName, ThroughputSettingsUpdateParameters updateThroughputParameters) {
return updateSqlDatabaseThroughputWithServiceResponseAsync(resourceGroupName, accountName, databaseName, updateThroughputParameters).toBlocking().last().body();
}
/**
* Update RUs per second of an Azure Cosmos DB SQL database.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param updateThroughputParameters The parameters to provide for the RUs per second of the current SQL database.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture updateSqlDatabaseThroughputAsync(String resourceGroupName, String accountName, String databaseName, ThroughputSettingsUpdateParameters updateThroughputParameters, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(updateSqlDatabaseThroughputWithServiceResponseAsync(resourceGroupName, accountName, databaseName, updateThroughputParameters), serviceCallback);
}
/**
* Update RUs per second of an Azure Cosmos DB SQL database.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param updateThroughputParameters The parameters to provide for the RUs per second of the current SQL database.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable for the request
*/
public Observable updateSqlDatabaseThroughputAsync(String resourceGroupName, String accountName, String databaseName, ThroughputSettingsUpdateParameters updateThroughputParameters) {
return updateSqlDatabaseThroughputWithServiceResponseAsync(resourceGroupName, accountName, databaseName, updateThroughputParameters).map(new Func1, ThroughputSettingsGetResultsInner>() {
@Override
public ThroughputSettingsGetResultsInner call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Update RUs per second of an Azure Cosmos DB SQL database.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param updateThroughputParameters The parameters to provide for the RUs per second of the current SQL database.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable for the request
*/
public Observable> updateSqlDatabaseThroughputWithServiceResponseAsync(String resourceGroupName, String accountName, String databaseName, ThroughputSettingsUpdateParameters updateThroughputParameters) {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (accountName == null) {
throw new IllegalArgumentException("Parameter accountName is required and cannot be null.");
}
if (databaseName == null) {
throw new IllegalArgumentException("Parameter databaseName is required and cannot be null.");
}
if (updateThroughputParameters == null) {
throw new IllegalArgumentException("Parameter updateThroughputParameters is required and cannot be null.");
}
Validator.validate(updateThroughputParameters);
final String apiVersion = "2019-08-01";
Observable> observable = service.updateSqlDatabaseThroughput(this.client.subscriptionId(), resourceGroupName, accountName, databaseName, apiVersion, updateThroughputParameters, this.client.acceptLanguage(), this.client.userAgent());
return client.getAzureClient().getPutOrPatchResultAsync(observable, new TypeToken() { }.getType());
}
/**
* Update RUs per second of an Azure Cosmos DB SQL database.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param updateThroughputParameters The parameters to provide for the RUs per second of the current SQL database.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the ThroughputSettingsGetResultsInner object if successful.
*/
public ThroughputSettingsGetResultsInner beginUpdateSqlDatabaseThroughput(String resourceGroupName, String accountName, String databaseName, ThroughputSettingsUpdateParameters updateThroughputParameters) {
return beginUpdateSqlDatabaseThroughputWithServiceResponseAsync(resourceGroupName, accountName, databaseName, updateThroughputParameters).toBlocking().single().body();
}
/**
* Update RUs per second of an Azure Cosmos DB SQL database.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param updateThroughputParameters The parameters to provide for the RUs per second of the current SQL database.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture beginUpdateSqlDatabaseThroughputAsync(String resourceGroupName, String accountName, String databaseName, ThroughputSettingsUpdateParameters updateThroughputParameters, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(beginUpdateSqlDatabaseThroughputWithServiceResponseAsync(resourceGroupName, accountName, databaseName, updateThroughputParameters), serviceCallback);
}
/**
* Update RUs per second of an Azure Cosmos DB SQL database.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param updateThroughputParameters The parameters to provide for the RUs per second of the current SQL database.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the ThroughputSettingsGetResultsInner object
*/
public Observable beginUpdateSqlDatabaseThroughputAsync(String resourceGroupName, String accountName, String databaseName, ThroughputSettingsUpdateParameters updateThroughputParameters) {
return beginUpdateSqlDatabaseThroughputWithServiceResponseAsync(resourceGroupName, accountName, databaseName, updateThroughputParameters).map(new Func1, ThroughputSettingsGetResultsInner>() {
@Override
public ThroughputSettingsGetResultsInner call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Update RUs per second of an Azure Cosmos DB SQL database.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param updateThroughputParameters The parameters to provide for the RUs per second of the current SQL database.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the ThroughputSettingsGetResultsInner object
*/
public Observable> beginUpdateSqlDatabaseThroughputWithServiceResponseAsync(String resourceGroupName, String accountName, String databaseName, ThroughputSettingsUpdateParameters updateThroughputParameters) {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (accountName == null) {
throw new IllegalArgumentException("Parameter accountName is required and cannot be null.");
}
if (databaseName == null) {
throw new IllegalArgumentException("Parameter databaseName is required and cannot be null.");
}
if (updateThroughputParameters == null) {
throw new IllegalArgumentException("Parameter updateThroughputParameters is required and cannot be null.");
}
Validator.validate(updateThroughputParameters);
final String apiVersion = "2019-08-01";
return service.beginUpdateSqlDatabaseThroughput(this.client.subscriptionId(), resourceGroupName, accountName, databaseName, apiVersion, updateThroughputParameters, this.client.acceptLanguage(), this.client.userAgent())
.flatMap(new Func1, Observable>>() {
@Override
public Observable> call(Response response) {
try {
ServiceResponse clientResponse = beginUpdateSqlDatabaseThroughputDelegate(response);
return Observable.just(clientResponse);
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse beginUpdateSqlDatabaseThroughputDelegate(Response response) throws CloudException, IOException, IllegalArgumentException {
return this.client.restClient().responseBuilderFactory().newInstance(this.client.serializerAdapter())
.register(200, new TypeToken() { }.getType())
.register(202, new TypeToken() { }.getType())
.registerError(CloudException.class)
.build(response);
}
/**
* Lists the SQL container under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the List<SqlContainerGetResultsInner> object if successful.
*/
public List listSqlContainers(String resourceGroupName, String accountName, String databaseName) {
return listSqlContainersWithServiceResponseAsync(resourceGroupName, accountName, databaseName).toBlocking().single().body();
}
/**
* Lists the SQL container under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture> listSqlContainersAsync(String resourceGroupName, String accountName, String databaseName, final ServiceCallback> serviceCallback) {
return ServiceFuture.fromResponse(listSqlContainersWithServiceResponseAsync(resourceGroupName, accountName, databaseName), serviceCallback);
}
/**
* Lists the SQL container under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the List<SqlContainerGetResultsInner> object
*/
public Observable> listSqlContainersAsync(String resourceGroupName, String accountName, String databaseName) {
return listSqlContainersWithServiceResponseAsync(resourceGroupName, accountName, databaseName).map(new Func1>, List>() {
@Override
public List call(ServiceResponse> response) {
return response.body();
}
});
}
/**
* Lists the SQL container under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the List<SqlContainerGetResultsInner> object
*/
public Observable>> listSqlContainersWithServiceResponseAsync(String resourceGroupName, String accountName, String databaseName) {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (accountName == null) {
throw new IllegalArgumentException("Parameter accountName is required and cannot be null.");
}
if (databaseName == null) {
throw new IllegalArgumentException("Parameter databaseName is required and cannot be null.");
}
final String apiVersion = "2019-08-01";
return service.listSqlContainers(this.client.subscriptionId(), resourceGroupName, accountName, databaseName, apiVersion, this.client.acceptLanguage(), this.client.userAgent())
.flatMap(new Func1, Observable>>>() {
@Override
public Observable>> call(Response response) {
try {
ServiceResponse> result = listSqlContainersDelegate(response);
List items = null;
if (result.body() != null) {
items = result.body().items();
}
ServiceResponse> clientResponse = new ServiceResponse>(items, result.response());
return Observable.just(clientResponse);
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse> listSqlContainersDelegate(Response response) throws CloudException, IOException, IllegalArgumentException {
return this.client.restClient().responseBuilderFactory()., CloudException>newInstance(this.client.serializerAdapter())
.register(200, new TypeToken>() { }.getType())
.registerError(CloudException.class)
.build(response);
}
/**
* Gets the SQL container under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the SqlContainerGetResultsInner object if successful.
*/
public SqlContainerGetResultsInner getSqlContainer(String resourceGroupName, String accountName, String databaseName, String containerName) {
return getSqlContainerWithServiceResponseAsync(resourceGroupName, accountName, databaseName, containerName).toBlocking().single().body();
}
/**
* Gets the SQL container under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture getSqlContainerAsync(String resourceGroupName, String accountName, String databaseName, String containerName, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(getSqlContainerWithServiceResponseAsync(resourceGroupName, accountName, databaseName, containerName), serviceCallback);
}
/**
* Gets the SQL container under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the SqlContainerGetResultsInner object
*/
public Observable getSqlContainerAsync(String resourceGroupName, String accountName, String databaseName, String containerName) {
return getSqlContainerWithServiceResponseAsync(resourceGroupName, accountName, databaseName, containerName).map(new Func1, SqlContainerGetResultsInner>() {
@Override
public SqlContainerGetResultsInner call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Gets the SQL container under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the SqlContainerGetResultsInner object
*/
public Observable> getSqlContainerWithServiceResponseAsync(String resourceGroupName, String accountName, String databaseName, String containerName) {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (accountName == null) {
throw new IllegalArgumentException("Parameter accountName is required and cannot be null.");
}
if (databaseName == null) {
throw new IllegalArgumentException("Parameter databaseName is required and cannot be null.");
}
if (containerName == null) {
throw new IllegalArgumentException("Parameter containerName is required and cannot be null.");
}
final String apiVersion = "2019-08-01";
return service.getSqlContainer(this.client.subscriptionId(), resourceGroupName, accountName, databaseName, containerName, apiVersion, this.client.acceptLanguage(), this.client.userAgent())
.flatMap(new Func1, Observable>>() {
@Override
public Observable> call(Response response) {
try {
ServiceResponse clientResponse = getSqlContainerDelegate(response);
return Observable.just(clientResponse);
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse getSqlContainerDelegate(Response response) throws CloudException, IOException, IllegalArgumentException {
return this.client.restClient().responseBuilderFactory().newInstance(this.client.serializerAdapter())
.register(200, new TypeToken() { }.getType())
.registerError(CloudException.class)
.build(response);
}
/**
* Create or update an Azure Cosmos DB SQL container.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param createUpdateSqlContainerParameters The parameters to provide for the current SQL container.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the SqlContainerGetResultsInner object if successful.
*/
public SqlContainerGetResultsInner createUpdateSqlContainer(String resourceGroupName, String accountName, String databaseName, String containerName, SqlContainerCreateUpdateParameters createUpdateSqlContainerParameters) {
return createUpdateSqlContainerWithServiceResponseAsync(resourceGroupName, accountName, databaseName, containerName, createUpdateSqlContainerParameters).toBlocking().last().body();
}
/**
* Create or update an Azure Cosmos DB SQL container.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param createUpdateSqlContainerParameters The parameters to provide for the current SQL container.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture createUpdateSqlContainerAsync(String resourceGroupName, String accountName, String databaseName, String containerName, SqlContainerCreateUpdateParameters createUpdateSqlContainerParameters, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(createUpdateSqlContainerWithServiceResponseAsync(resourceGroupName, accountName, databaseName, containerName, createUpdateSqlContainerParameters), serviceCallback);
}
/**
* Create or update an Azure Cosmos DB SQL container.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param createUpdateSqlContainerParameters The parameters to provide for the current SQL container.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable for the request
*/
public Observable createUpdateSqlContainerAsync(String resourceGroupName, String accountName, String databaseName, String containerName, SqlContainerCreateUpdateParameters createUpdateSqlContainerParameters) {
return createUpdateSqlContainerWithServiceResponseAsync(resourceGroupName, accountName, databaseName, containerName, createUpdateSqlContainerParameters).map(new Func1, SqlContainerGetResultsInner>() {
@Override
public SqlContainerGetResultsInner call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Create or update an Azure Cosmos DB SQL container.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param createUpdateSqlContainerParameters The parameters to provide for the current SQL container.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable for the request
*/
public Observable> createUpdateSqlContainerWithServiceResponseAsync(String resourceGroupName, String accountName, String databaseName, String containerName, SqlContainerCreateUpdateParameters createUpdateSqlContainerParameters) {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (accountName == null) {
throw new IllegalArgumentException("Parameter accountName is required and cannot be null.");
}
if (databaseName == null) {
throw new IllegalArgumentException("Parameter databaseName is required and cannot be null.");
}
if (containerName == null) {
throw new IllegalArgumentException("Parameter containerName is required and cannot be null.");
}
if (createUpdateSqlContainerParameters == null) {
throw new IllegalArgumentException("Parameter createUpdateSqlContainerParameters is required and cannot be null.");
}
Validator.validate(createUpdateSqlContainerParameters);
final String apiVersion = "2019-08-01";
Observable> observable = service.createUpdateSqlContainer(this.client.subscriptionId(), resourceGroupName, accountName, databaseName, containerName, apiVersion, createUpdateSqlContainerParameters, this.client.acceptLanguage(), this.client.userAgent());
return client.getAzureClient().getPutOrPatchResultAsync(observable, new TypeToken() { }.getType());
}
/**
* Create or update an Azure Cosmos DB SQL container.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param createUpdateSqlContainerParameters The parameters to provide for the current SQL container.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the SqlContainerGetResultsInner object if successful.
*/
public SqlContainerGetResultsInner beginCreateUpdateSqlContainer(String resourceGroupName, String accountName, String databaseName, String containerName, SqlContainerCreateUpdateParameters createUpdateSqlContainerParameters) {
return beginCreateUpdateSqlContainerWithServiceResponseAsync(resourceGroupName, accountName, databaseName, containerName, createUpdateSqlContainerParameters).toBlocking().single().body();
}
/**
* Create or update an Azure Cosmos DB SQL container.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param createUpdateSqlContainerParameters The parameters to provide for the current SQL container.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture beginCreateUpdateSqlContainerAsync(String resourceGroupName, String accountName, String databaseName, String containerName, SqlContainerCreateUpdateParameters createUpdateSqlContainerParameters, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(beginCreateUpdateSqlContainerWithServiceResponseAsync(resourceGroupName, accountName, databaseName, containerName, createUpdateSqlContainerParameters), serviceCallback);
}
/**
* Create or update an Azure Cosmos DB SQL container.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param createUpdateSqlContainerParameters The parameters to provide for the current SQL container.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the SqlContainerGetResultsInner object
*/
public Observable beginCreateUpdateSqlContainerAsync(String resourceGroupName, String accountName, String databaseName, String containerName, SqlContainerCreateUpdateParameters createUpdateSqlContainerParameters) {
return beginCreateUpdateSqlContainerWithServiceResponseAsync(resourceGroupName, accountName, databaseName, containerName, createUpdateSqlContainerParameters).map(new Func1, SqlContainerGetResultsInner>() {
@Override
public SqlContainerGetResultsInner call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Create or update an Azure Cosmos DB SQL container.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param createUpdateSqlContainerParameters The parameters to provide for the current SQL container.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the SqlContainerGetResultsInner object
*/
public Observable> beginCreateUpdateSqlContainerWithServiceResponseAsync(String resourceGroupName, String accountName, String databaseName, String containerName, SqlContainerCreateUpdateParameters createUpdateSqlContainerParameters) {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (accountName == null) {
throw new IllegalArgumentException("Parameter accountName is required and cannot be null.");
}
if (databaseName == null) {
throw new IllegalArgumentException("Parameter databaseName is required and cannot be null.");
}
if (containerName == null) {
throw new IllegalArgumentException("Parameter containerName is required and cannot be null.");
}
if (createUpdateSqlContainerParameters == null) {
throw new IllegalArgumentException("Parameter createUpdateSqlContainerParameters is required and cannot be null.");
}
Validator.validate(createUpdateSqlContainerParameters);
final String apiVersion = "2019-08-01";
return service.beginCreateUpdateSqlContainer(this.client.subscriptionId(), resourceGroupName, accountName, databaseName, containerName, apiVersion, createUpdateSqlContainerParameters, this.client.acceptLanguage(), this.client.userAgent())
.flatMap(new Func1, Observable>>() {
@Override
public Observable> call(Response response) {
try {
ServiceResponse clientResponse = beginCreateUpdateSqlContainerDelegate(response);
return Observable.just(clientResponse);
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse beginCreateUpdateSqlContainerDelegate(Response response) throws CloudException, IOException, IllegalArgumentException {
return this.client.restClient().responseBuilderFactory().newInstance(this.client.serializerAdapter())
.register(200, new TypeToken() { }.getType())
.register(202, new TypeToken() { }.getType())
.registerError(CloudException.class)
.build(response);
}
/**
* Deletes an existing Azure Cosmos DB SQL container.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
*/
public void deleteSqlContainer(String resourceGroupName, String accountName, String databaseName, String containerName) {
deleteSqlContainerWithServiceResponseAsync(resourceGroupName, accountName, databaseName, containerName).toBlocking().last().body();
}
/**
* Deletes an existing Azure Cosmos DB SQL container.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture deleteSqlContainerAsync(String resourceGroupName, String accountName, String databaseName, String containerName, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(deleteSqlContainerWithServiceResponseAsync(resourceGroupName, accountName, databaseName, containerName), serviceCallback);
}
/**
* Deletes an existing Azure Cosmos DB SQL container.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable for the request
*/
public Observable deleteSqlContainerAsync(String resourceGroupName, String accountName, String databaseName, String containerName) {
return deleteSqlContainerWithServiceResponseAsync(resourceGroupName, accountName, databaseName, containerName).map(new Func1, Void>() {
@Override
public Void call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Deletes an existing Azure Cosmos DB SQL container.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable for the request
*/
public Observable> deleteSqlContainerWithServiceResponseAsync(String resourceGroupName, String accountName, String databaseName, String containerName) {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (accountName == null) {
throw new IllegalArgumentException("Parameter accountName is required and cannot be null.");
}
if (databaseName == null) {
throw new IllegalArgumentException("Parameter databaseName is required and cannot be null.");
}
if (containerName == null) {
throw new IllegalArgumentException("Parameter containerName is required and cannot be null.");
}
final String apiVersion = "2019-08-01";
Observable> observable = service.deleteSqlContainer(this.client.subscriptionId(), resourceGroupName, accountName, databaseName, containerName, apiVersion, this.client.acceptLanguage(), this.client.userAgent());
return client.getAzureClient().getPostOrDeleteResultAsync(observable, new TypeToken() { }.getType());
}
/**
* Deletes an existing Azure Cosmos DB SQL container.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
*/
public void beginDeleteSqlContainer(String resourceGroupName, String accountName, String databaseName, String containerName) {
beginDeleteSqlContainerWithServiceResponseAsync(resourceGroupName, accountName, databaseName, containerName).toBlocking().single().body();
}
/**
* Deletes an existing Azure Cosmos DB SQL container.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture beginDeleteSqlContainerAsync(String resourceGroupName, String accountName, String databaseName, String containerName, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(beginDeleteSqlContainerWithServiceResponseAsync(resourceGroupName, accountName, databaseName, containerName), serviceCallback);
}
/**
* Deletes an existing Azure Cosmos DB SQL container.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceResponse} object if successful.
*/
public Observable beginDeleteSqlContainerAsync(String resourceGroupName, String accountName, String databaseName, String containerName) {
return beginDeleteSqlContainerWithServiceResponseAsync(resourceGroupName, accountName, databaseName, containerName).map(new Func1, Void>() {
@Override
public Void call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Deletes an existing Azure Cosmos DB SQL container.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceResponse} object if successful.
*/
public Observable> beginDeleteSqlContainerWithServiceResponseAsync(String resourceGroupName, String accountName, String databaseName, String containerName) {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (accountName == null) {
throw new IllegalArgumentException("Parameter accountName is required and cannot be null.");
}
if (databaseName == null) {
throw new IllegalArgumentException("Parameter databaseName is required and cannot be null.");
}
if (containerName == null) {
throw new IllegalArgumentException("Parameter containerName is required and cannot be null.");
}
final String apiVersion = "2019-08-01";
return service.beginDeleteSqlContainer(this.client.subscriptionId(), resourceGroupName, accountName, databaseName, containerName, apiVersion, this.client.acceptLanguage(), this.client.userAgent())
.flatMap(new Func1, Observable>>() {
@Override
public Observable> call(Response response) {
try {
ServiceResponse clientResponse = beginDeleteSqlContainerDelegate(response);
return Observable.just(clientResponse);
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse beginDeleteSqlContainerDelegate(Response response) throws CloudException, IOException, IllegalArgumentException {
return this.client.restClient().responseBuilderFactory().newInstance(this.client.serializerAdapter())
.register(202, new TypeToken() { }.getType())
.register(204, new TypeToken() { }.getType())
.registerError(CloudException.class)
.build(response);
}
/**
* Gets the RUs per second of the SQL container under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the ThroughputSettingsGetResultsInner object if successful.
*/
public ThroughputSettingsGetResultsInner getSqlContainerThroughput(String resourceGroupName, String accountName, String databaseName, String containerName) {
return getSqlContainerThroughputWithServiceResponseAsync(resourceGroupName, accountName, databaseName, containerName).toBlocking().single().body();
}
/**
* Gets the RUs per second of the SQL container under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture getSqlContainerThroughputAsync(String resourceGroupName, String accountName, String databaseName, String containerName, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(getSqlContainerThroughputWithServiceResponseAsync(resourceGroupName, accountName, databaseName, containerName), serviceCallback);
}
/**
* Gets the RUs per second of the SQL container under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the ThroughputSettingsGetResultsInner object
*/
public Observable getSqlContainerThroughputAsync(String resourceGroupName, String accountName, String databaseName, String containerName) {
return getSqlContainerThroughputWithServiceResponseAsync(resourceGroupName, accountName, databaseName, containerName).map(new Func1, ThroughputSettingsGetResultsInner>() {
@Override
public ThroughputSettingsGetResultsInner call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Gets the RUs per second of the SQL container under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName Name of an Azure resource group.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the ThroughputSettingsGetResultsInner object
*/
public Observable> getSqlContainerThroughputWithServiceResponseAsync(String resourceGroupName, String accountName, String databaseName, String containerName) {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (accountName == null) {
throw new IllegalArgumentException("Parameter accountName is required and cannot be null.");
}
if (databaseName == null) {
throw new IllegalArgumentException("Parameter databaseName is required and cannot be null.");
}
if (containerName == null) {
throw new IllegalArgumentException("Parameter containerName is required and cannot be null.");
}
final String apiVersion = "2019-08-01";
return service.getSqlContainerThroughput(this.client.subscriptionId(), resourceGroupName, accountName, databaseName, containerName, apiVersion, this.client.acceptLanguage(), this.client.userAgent())
.flatMap(new Func1, Observable