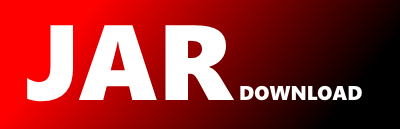
com.microsoft.azure.management.dns.RecordSetOperationsImpl Maven / Gradle / Ivy
/**
*
* Copyright (c) Microsoft and contributors. All rights reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
*
* See the License for the specific language governing permissions and
* limitations under the License.
*
*/
// Warning: This code was generated by a tool.
//
// Changes to this file may cause incorrect behavior and will be lost if the
// code is regenerated.
package com.microsoft.azure.management.dns;
import com.microsoft.azure.management.dns.models.ARecord;
import com.microsoft.azure.management.dns.models.AaaaRecord;
import com.microsoft.azure.management.dns.models.CnameRecord;
import com.microsoft.azure.management.dns.models.MxRecord;
import com.microsoft.azure.management.dns.models.NsRecord;
import com.microsoft.azure.management.dns.models.PtrRecord;
import com.microsoft.azure.management.dns.models.RecordSet;
import com.microsoft.azure.management.dns.models.RecordSetCreateOrUpdateParameters;
import com.microsoft.azure.management.dns.models.RecordSetCreateOrUpdateResponse;
import com.microsoft.azure.management.dns.models.RecordSetDeleteParameters;
import com.microsoft.azure.management.dns.models.RecordSetGetResponse;
import com.microsoft.azure.management.dns.models.RecordSetListParameters;
import com.microsoft.azure.management.dns.models.RecordSetListResponse;
import com.microsoft.azure.management.dns.models.RecordSetProperties;
import com.microsoft.azure.management.dns.models.RecordType;
import com.microsoft.azure.management.dns.models.SoaRecord;
import com.microsoft.azure.management.dns.models.SrvRecord;
import com.microsoft.azure.management.dns.models.TxtRecord;
import com.microsoft.windowsazure.core.OperationResponse;
import com.microsoft.windowsazure.core.ServiceOperations;
import com.microsoft.windowsazure.core.pipeline.apache.CustomHttpDelete;
import com.microsoft.windowsazure.core.utils.CollectionStringBuilder;
import com.microsoft.windowsazure.exception.ServiceException;
import com.microsoft.windowsazure.tracing.CloudTracing;
import org.apache.commons.io.IOUtils;
import org.apache.http.HttpResponse;
import org.apache.http.HttpStatus;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.client.methods.HttpPut;
import org.apache.http.entity.StringEntity;
import org.codehaus.jackson.JsonNode;
import org.codehaus.jackson.map.ObjectMapper;
import org.codehaus.jackson.node.ArrayNode;
import org.codehaus.jackson.node.NullNode;
import org.codehaus.jackson.node.ObjectNode;
import java.io.IOException;
import java.io.InputStream;
import java.io.StringWriter;
import java.net.URLEncoder;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
import java.util.concurrent.Callable;
import java.util.concurrent.Future;
/**
* Operations for managing the RecordSets in a DNS zone.
*/
public class RecordSetOperationsImpl implements ServiceOperations, RecordSetOperations {
/**
* Initializes a new instance of the RecordSetOperationsImpl class.
*
* @param client Reference to the service client.
*/
RecordSetOperationsImpl(DnsManagementClientImpl client) {
this.client = client;
}
private DnsManagementClientImpl client;
/**
* Gets a reference to the
* microsoft.azure.management.dns.DnsManagementClientImpl.
* @return The Client value.
*/
public DnsManagementClientImpl getClient() {
return this.client;
}
/**
* Creates a RecordSet within a DNS zone.
*
* @param resourceGroupName Required. The name of the resource group.
* @param zoneName Required. The name of the zone without a terminating dot.
* @param relativeRecordSetName Required. The name of the RecordSet,
* relative to the name of the zone.
* @param recordType Required. The type of DNS record.
* @param parameters Required. Parameters supplied to the CreateOrUpdate
* operation.
* @return The response to a RecordSet CreateOrUpdate operation.
*/
@Override
public Future createOrUpdateAsync(final String resourceGroupName, final String zoneName, final String relativeRecordSetName, final RecordType recordType, final RecordSetCreateOrUpdateParameters parameters) {
return this.getClient().getExecutorService().submit(new Callable() {
@Override
public RecordSetCreateOrUpdateResponse call() throws Exception {
return createOrUpdate(resourceGroupName, zoneName, relativeRecordSetName, recordType, parameters);
}
});
}
/**
* Creates a RecordSet within a DNS zone.
*
* @param resourceGroupName Required. The name of the resource group.
* @param zoneName Required. The name of the zone without a terminating dot.
* @param relativeRecordSetName Required. The name of the RecordSet,
* relative to the name of the zone.
* @param recordType Required. The type of DNS record.
* @param parameters Required. Parameters supplied to the CreateOrUpdate
* operation.
* @throws IOException Signals that an I/O exception of some sort has
* occurred. This class is the general class of exceptions produced by
* failed or interrupted I/O operations.
* @throws ServiceException Thrown if an unexpected response is found.
* @return The response to a RecordSet CreateOrUpdate operation.
*/
@Override
public RecordSetCreateOrUpdateResponse createOrUpdate(String resourceGroupName, String zoneName, String relativeRecordSetName, RecordType recordType, RecordSetCreateOrUpdateParameters parameters) throws IOException, ServiceException {
// Validate
if (resourceGroupName == null) {
throw new NullPointerException("resourceGroupName");
}
if (zoneName == null) {
throw new NullPointerException("zoneName");
}
if (relativeRecordSetName == null) {
throw new NullPointerException("relativeRecordSetName");
}
if (recordType == null) {
throw new NullPointerException("recordType");
}
if (parameters == null) {
throw new NullPointerException("parameters");
}
if (parameters.getRecordSet() == null) {
throw new NullPointerException("parameters.RecordSet");
}
if (parameters.getRecordSet().getLocation() == null) {
throw new NullPointerException("parameters.RecordSet.Location");
}
if (parameters.getRecordSet().getProperties() != null) {
if (parameters.getRecordSet().getProperties().getAaaaRecords() != null) {
for (AaaaRecord aaaaRecordsParameterItem : parameters.getRecordSet().getProperties().getAaaaRecords()) {
if (aaaaRecordsParameterItem.getIpv6Address() == null) {
throw new NullPointerException("parameters.RecordSet.Properties.AaaaRecords.Ipv6Address");
}
}
}
if (parameters.getRecordSet().getProperties().getARecords() != null) {
for (ARecord aRecordsParameterItem : parameters.getRecordSet().getProperties().getARecords()) {
if (aRecordsParameterItem.getIpv4Address() == null) {
throw new NullPointerException("parameters.RecordSet.Properties.ARecords.Ipv4Address");
}
}
}
if (parameters.getRecordSet().getProperties().getCnameRecord() != null) {
if (parameters.getRecordSet().getProperties().getCnameRecord().getCname() == null) {
throw new NullPointerException("parameters.RecordSet.Properties.CnameRecord.Cname");
}
}
if (parameters.getRecordSet().getProperties().getMxRecords() != null) {
for (MxRecord mxRecordsParameterItem : parameters.getRecordSet().getProperties().getMxRecords()) {
if (mxRecordsParameterItem.getExchange() == null) {
throw new NullPointerException("parameters.RecordSet.Properties.MxRecords.Exchange");
}
}
}
if (parameters.getRecordSet().getProperties().getNsRecords() != null) {
for (NsRecord nsRecordsParameterItem : parameters.getRecordSet().getProperties().getNsRecords()) {
if (nsRecordsParameterItem.getNsdname() == null) {
throw new NullPointerException("parameters.RecordSet.Properties.NsRecords.Nsdname");
}
}
}
if (parameters.getRecordSet().getProperties().getPtrRecords() != null) {
for (PtrRecord ptrRecordsParameterItem : parameters.getRecordSet().getProperties().getPtrRecords()) {
if (ptrRecordsParameterItem.getPtrdname() == null) {
throw new NullPointerException("parameters.RecordSet.Properties.PtrRecords.Ptrdname");
}
}
}
if (parameters.getRecordSet().getProperties().getSoaRecord() != null) {
if (parameters.getRecordSet().getProperties().getSoaRecord().getEmail() == null) {
throw new NullPointerException("parameters.RecordSet.Properties.SoaRecord.Email");
}
if (parameters.getRecordSet().getProperties().getSoaRecord().getHost() == null) {
throw new NullPointerException("parameters.RecordSet.Properties.SoaRecord.Host");
}
}
if (parameters.getRecordSet().getProperties().getSrvRecords() != null) {
for (SrvRecord srvRecordsParameterItem : parameters.getRecordSet().getProperties().getSrvRecords()) {
if (srvRecordsParameterItem.getTarget() == null) {
throw new NullPointerException("parameters.RecordSet.Properties.SrvRecords.Target");
}
}
}
if (parameters.getRecordSet().getProperties().getTxtRecords() != null) {
for (TxtRecord txtRecordsParameterItem : parameters.getRecordSet().getProperties().getTxtRecords()) {
if (txtRecordsParameterItem.getValue() == null) {
throw new NullPointerException("parameters.RecordSet.Properties.TxtRecords.Value");
}
}
}
}
// Tracing
boolean shouldTrace = CloudTracing.getIsEnabled();
String invocationId = null;
if (shouldTrace) {
invocationId = Long.toString(CloudTracing.getNextInvocationId());
HashMap tracingParameters = new HashMap();
tracingParameters.put("resourceGroupName", resourceGroupName);
tracingParameters.put("zoneName", zoneName);
tracingParameters.put("relativeRecordSetName", relativeRecordSetName);
tracingParameters.put("recordType", recordType);
tracingParameters.put("parameters", parameters);
CloudTracing.enter(invocationId, this, "createOrUpdateAsync", tracingParameters);
}
// Construct URL
String url = "";
url = url + "/subscriptions/";
if (this.getClient().getCredentials().getSubscriptionId() != null) {
url = url + URLEncoder.encode(this.getClient().getCredentials().getSubscriptionId(), "UTF-8");
}
url = url + "/resourceGroups/";
url = url + URLEncoder.encode(resourceGroupName, "UTF-8");
url = url + "/providers/";
url = url + "Microsoft.Network";
url = url + "/dnszones/";
url = url + URLEncoder.encode(zoneName, "UTF-8");
url = url + "/";
url = url + URLEncoder.encode(recordType.toString(), "UTF-8");
url = url + "/";
url = url + relativeRecordSetName;
ArrayList queryParameters = new ArrayList();
queryParameters.add("api-version=" + "2015-05-04-preview");
if (queryParameters.size() > 0) {
url = url + "?" + CollectionStringBuilder.join(queryParameters, "&");
}
String baseUrl = this.getClient().getBaseUri().toString();
// Trim '/' character from the end of baseUrl and beginning of url.
if (baseUrl.charAt(baseUrl.length() - 1) == '/') {
baseUrl = baseUrl.substring(0, (baseUrl.length() - 1) + 0);
}
if (url.charAt(0) == '/') {
url = url.substring(1);
}
url = baseUrl + "/" + url;
url = url.replace(" ", "%20");
// Create HTTP transport objects
HttpPut httpRequest = new HttpPut(url);
// Set Headers
httpRequest.setHeader("Content-Type", "application/json; charset=utf-8");
httpRequest.setHeader("If-Match", parameters.getRecordSet().getETag());
httpRequest.setHeader("If-None-Match", parameters.getIfNoneMatch());
// Serialize Request
String requestContent = null;
JsonNode requestDoc = null;
ObjectMapper objectMapper = new ObjectMapper();
ObjectNode recordSetCreateOrUpdateParametersValue = objectMapper.createObjectNode();
requestDoc = recordSetCreateOrUpdateParametersValue;
if (parameters.getRecordSet().getETag() != null) {
((ObjectNode) recordSetCreateOrUpdateParametersValue).put("etag", parameters.getRecordSet().getETag());
}
if (parameters.getRecordSet().getProperties() != null) {
ObjectNode propertiesValue = objectMapper.createObjectNode();
((ObjectNode) recordSetCreateOrUpdateParametersValue).put("properties", propertiesValue);
((ObjectNode) propertiesValue).put("TTL", parameters.getRecordSet().getProperties().getTtl());
if (parameters.getRecordSet().getProperties().getARecords() != null) {
ArrayNode aRecordsArray = objectMapper.createArrayNode();
for (ARecord aRecordsItem : parameters.getRecordSet().getProperties().getARecords()) {
ObjectNode aRecordValue = objectMapper.createObjectNode();
aRecordsArray.add(aRecordValue);
((ObjectNode) aRecordValue).put("ipv4Address", aRecordsItem.getIpv4Address());
}
((ObjectNode) propertiesValue).put("ARecords", aRecordsArray);
}
if (parameters.getRecordSet().getProperties().getAaaaRecords() != null) {
ArrayNode aAAARecordsArray = objectMapper.createArrayNode();
for (AaaaRecord aAAARecordsItem : parameters.getRecordSet().getProperties().getAaaaRecords()) {
ObjectNode aaaaRecordValue = objectMapper.createObjectNode();
aAAARecordsArray.add(aaaaRecordValue);
((ObjectNode) aaaaRecordValue).put("ipv6Address", aAAARecordsItem.getIpv6Address());
}
((ObjectNode) propertiesValue).put("AAAARecords", aAAARecordsArray);
}
if (parameters.getRecordSet().getProperties().getMxRecords() != null) {
ArrayNode mXRecordsArray = objectMapper.createArrayNode();
for (MxRecord mXRecordsItem : parameters.getRecordSet().getProperties().getMxRecords()) {
ObjectNode mxRecordValue = objectMapper.createObjectNode();
mXRecordsArray.add(mxRecordValue);
((ObjectNode) mxRecordValue).put("preference", mXRecordsItem.getPreference());
((ObjectNode) mxRecordValue).put("exchange", mXRecordsItem.getExchange());
}
((ObjectNode) propertiesValue).put("MXRecords", mXRecordsArray);
}
if (parameters.getRecordSet().getProperties().getNsRecords() != null) {
ArrayNode nSRecordsArray = objectMapper.createArrayNode();
for (NsRecord nSRecordsItem : parameters.getRecordSet().getProperties().getNsRecords()) {
ObjectNode nsRecordValue = objectMapper.createObjectNode();
nSRecordsArray.add(nsRecordValue);
((ObjectNode) nsRecordValue).put("nsdname", nSRecordsItem.getNsdname());
}
((ObjectNode) propertiesValue).put("NSRecords", nSRecordsArray);
}
if (parameters.getRecordSet().getProperties().getPtrRecords() != null) {
ArrayNode pTRRecordsArray = objectMapper.createArrayNode();
for (PtrRecord pTRRecordsItem : parameters.getRecordSet().getProperties().getPtrRecords()) {
ObjectNode ptrRecordValue = objectMapper.createObjectNode();
pTRRecordsArray.add(ptrRecordValue);
((ObjectNode) ptrRecordValue).put("ptrdname", pTRRecordsItem.getPtrdname());
}
((ObjectNode) propertiesValue).put("PTRRecords", pTRRecordsArray);
}
if (parameters.getRecordSet().getProperties().getSrvRecords() != null) {
ArrayNode sRVRecordsArray = objectMapper.createArrayNode();
for (SrvRecord sRVRecordsItem : parameters.getRecordSet().getProperties().getSrvRecords()) {
ObjectNode srvRecordValue = objectMapper.createObjectNode();
sRVRecordsArray.add(srvRecordValue);
((ObjectNode) srvRecordValue).put("priority", sRVRecordsItem.getPriority());
((ObjectNode) srvRecordValue).put("weight", sRVRecordsItem.getWeight());
((ObjectNode) srvRecordValue).put("port", sRVRecordsItem.getPort());
((ObjectNode) srvRecordValue).put("target", sRVRecordsItem.getTarget());
}
((ObjectNode) propertiesValue).put("SRVRecords", sRVRecordsArray);
}
if (parameters.getRecordSet().getProperties().getTxtRecords() != null) {
ArrayNode tXTRecordsArray = objectMapper.createArrayNode();
for (TxtRecord tXTRecordsItem : parameters.getRecordSet().getProperties().getTxtRecords()) {
ObjectNode txtRecordValue = objectMapper.createObjectNode();
tXTRecordsArray.add(txtRecordValue);
((ObjectNode) txtRecordValue).put("value", tXTRecordsItem.getValue());
}
((ObjectNode) propertiesValue).put("TXTRecords", tXTRecordsArray);
}
if (parameters.getRecordSet().getProperties().getCnameRecord() != null) {
ObjectNode cNAMERecordValue = objectMapper.createObjectNode();
((ObjectNode) propertiesValue).put("CNAMERecord", cNAMERecordValue);
((ObjectNode) cNAMERecordValue).put("cname", parameters.getRecordSet().getProperties().getCnameRecord().getCname());
}
if (parameters.getRecordSet().getProperties().getSoaRecord() != null) {
ObjectNode sOARecordValue = objectMapper.createObjectNode();
((ObjectNode) propertiesValue).put("SOARecord", sOARecordValue);
((ObjectNode) sOARecordValue).put("host", parameters.getRecordSet().getProperties().getSoaRecord().getHost());
((ObjectNode) sOARecordValue).put("email", parameters.getRecordSet().getProperties().getSoaRecord().getEmail());
((ObjectNode) sOARecordValue).put("serialNumber", parameters.getRecordSet().getProperties().getSoaRecord().getSerialNumber());
((ObjectNode) sOARecordValue).put("refreshTime", parameters.getRecordSet().getProperties().getSoaRecord().getRefreshTime());
((ObjectNode) sOARecordValue).put("retryTime", parameters.getRecordSet().getProperties().getSoaRecord().getRetryTime());
((ObjectNode) sOARecordValue).put("expireTime", parameters.getRecordSet().getProperties().getSoaRecord().getExpireTime());
((ObjectNode) sOARecordValue).put("minimumTTL", parameters.getRecordSet().getProperties().getSoaRecord().getMinimumTtl());
}
}
if (parameters.getRecordSet().getId() != null) {
((ObjectNode) recordSetCreateOrUpdateParametersValue).put("id", parameters.getRecordSet().getId());
}
if (parameters.getRecordSet().getName() != null) {
((ObjectNode) recordSetCreateOrUpdateParametersValue).put("name", parameters.getRecordSet().getName());
}
if (parameters.getRecordSet().getType() != null) {
((ObjectNode) recordSetCreateOrUpdateParametersValue).put("type", parameters.getRecordSet().getType());
}
((ObjectNode) recordSetCreateOrUpdateParametersValue).put("location", parameters.getRecordSet().getLocation());
if (parameters.getRecordSet().getTags() != null) {
ObjectNode tagsDictionary = objectMapper.createObjectNode();
for (Map.Entry entry : parameters.getRecordSet().getTags().entrySet()) {
String tagsKey = entry.getKey();
String tagsValue = entry.getValue();
((ObjectNode) tagsDictionary).put(tagsKey, tagsValue);
}
((ObjectNode) recordSetCreateOrUpdateParametersValue).put("tags", tagsDictionary);
}
StringWriter stringWriter = new StringWriter();
objectMapper.writeValue(stringWriter, requestDoc);
requestContent = stringWriter.toString();
StringEntity entity = new StringEntity(requestContent);
httpRequest.setEntity(entity);
httpRequest.setHeader("Content-Type", "application/json; charset=utf-8");
// Send Request
HttpResponse httpResponse = null;
try {
if (shouldTrace) {
CloudTracing.sendRequest(invocationId, httpRequest);
}
httpResponse = this.getClient().getHttpClient().execute(httpRequest);
if (shouldTrace) {
CloudTracing.receiveResponse(invocationId, httpResponse);
}
int statusCode = httpResponse.getStatusLine().getStatusCode();
if (statusCode >= HttpStatus.SC_BAD_REQUEST) {
ServiceException ex = ServiceException.createFromJson(httpRequest, requestContent, httpResponse, httpResponse.getEntity());
if (shouldTrace) {
CloudTracing.error(invocationId, ex);
}
throw ex;
}
// Create Result
RecordSetCreateOrUpdateResponse result = null;
// Deserialize Response
InputStream responseContent = httpResponse.getEntity().getContent();
result = new RecordSetCreateOrUpdateResponse();
JsonNode responseDoc = null;
String responseDocContent = IOUtils.toString(responseContent);
if (responseDocContent == null == false && responseDocContent.length() > 0) {
responseDoc = objectMapper.readTree(responseDocContent);
}
if (responseDoc != null && responseDoc instanceof NullNode == false) {
RecordSet recordSetInstance = new RecordSet();
result.setRecordSet(recordSetInstance);
JsonNode etagValue = responseDoc.get("etag");
if (etagValue != null && etagValue instanceof NullNode == false) {
String etagInstance;
etagInstance = etagValue.getTextValue();
recordSetInstance.setETag(etagInstance);
}
JsonNode propertiesValue2 = responseDoc.get("properties");
if (propertiesValue2 != null && propertiesValue2 instanceof NullNode == false) {
RecordSetProperties propertiesInstance = new RecordSetProperties();
recordSetInstance.setProperties(propertiesInstance);
JsonNode tTLValue = propertiesValue2.get("TTL");
if (tTLValue != null && tTLValue instanceof NullNode == false) {
long tTLInstance;
tTLInstance = tTLValue.getLongValue();
propertiesInstance.setTtl(tTLInstance);
}
JsonNode aRecordsArray2 = propertiesValue2.get("ARecords");
if (aRecordsArray2 != null && aRecordsArray2 instanceof NullNode == false) {
propertiesInstance.setARecords(new ArrayList());
for (JsonNode aRecordsValue : ((ArrayNode) aRecordsArray2)) {
ARecord aRecordInstance = new ARecord();
propertiesInstance.getARecords().add(aRecordInstance);
JsonNode ipv4AddressValue = aRecordsValue.get("ipv4Address");
if (ipv4AddressValue != null && ipv4AddressValue instanceof NullNode == false) {
String ipv4AddressInstance;
ipv4AddressInstance = ipv4AddressValue.getTextValue();
aRecordInstance.setIpv4Address(ipv4AddressInstance);
}
}
}
JsonNode aAAARecordsArray2 = propertiesValue2.get("AAAARecords");
if (aAAARecordsArray2 != null && aAAARecordsArray2 instanceof NullNode == false) {
propertiesInstance.setAaaaRecords(new ArrayList());
for (JsonNode aAAARecordsValue : ((ArrayNode) aAAARecordsArray2)) {
AaaaRecord aaaaRecordInstance = new AaaaRecord();
propertiesInstance.getAaaaRecords().add(aaaaRecordInstance);
JsonNode ipv6AddressValue = aAAARecordsValue.get("ipv6Address");
if (ipv6AddressValue != null && ipv6AddressValue instanceof NullNode == false) {
String ipv6AddressInstance;
ipv6AddressInstance = ipv6AddressValue.getTextValue();
aaaaRecordInstance.setIpv6Address(ipv6AddressInstance);
}
}
}
JsonNode mXRecordsArray2 = propertiesValue2.get("MXRecords");
if (mXRecordsArray2 != null && mXRecordsArray2 instanceof NullNode == false) {
propertiesInstance.setMxRecords(new ArrayList());
for (JsonNode mXRecordsValue : ((ArrayNode) mXRecordsArray2)) {
MxRecord mxRecordInstance = new MxRecord();
propertiesInstance.getMxRecords().add(mxRecordInstance);
JsonNode preferenceValue = mXRecordsValue.get("preference");
if (preferenceValue != null && preferenceValue instanceof NullNode == false) {
int preferenceInstance;
preferenceInstance = preferenceValue.getIntValue();
mxRecordInstance.setPreference(preferenceInstance);
}
JsonNode exchangeValue = mXRecordsValue.get("exchange");
if (exchangeValue != null && exchangeValue instanceof NullNode == false) {
String exchangeInstance;
exchangeInstance = exchangeValue.getTextValue();
mxRecordInstance.setExchange(exchangeInstance);
}
}
}
JsonNode nSRecordsArray2 = propertiesValue2.get("NSRecords");
if (nSRecordsArray2 != null && nSRecordsArray2 instanceof NullNode == false) {
propertiesInstance.setNsRecords(new ArrayList());
for (JsonNode nSRecordsValue : ((ArrayNode) nSRecordsArray2)) {
NsRecord nsRecordInstance = new NsRecord();
propertiesInstance.getNsRecords().add(nsRecordInstance);
JsonNode nsdnameValue = nSRecordsValue.get("nsdname");
if (nsdnameValue != null && nsdnameValue instanceof NullNode == false) {
String nsdnameInstance;
nsdnameInstance = nsdnameValue.getTextValue();
nsRecordInstance.setNsdname(nsdnameInstance);
}
}
}
JsonNode pTRRecordsArray2 = propertiesValue2.get("PTRRecords");
if (pTRRecordsArray2 != null && pTRRecordsArray2 instanceof NullNode == false) {
propertiesInstance.setPtrRecords(new ArrayList());
for (JsonNode pTRRecordsValue : ((ArrayNode) pTRRecordsArray2)) {
PtrRecord ptrRecordInstance = new PtrRecord();
propertiesInstance.getPtrRecords().add(ptrRecordInstance);
JsonNode ptrdnameValue = pTRRecordsValue.get("ptrdname");
if (ptrdnameValue != null && ptrdnameValue instanceof NullNode == false) {
String ptrdnameInstance;
ptrdnameInstance = ptrdnameValue.getTextValue();
ptrRecordInstance.setPtrdname(ptrdnameInstance);
}
}
}
JsonNode sRVRecordsArray2 = propertiesValue2.get("SRVRecords");
if (sRVRecordsArray2 != null && sRVRecordsArray2 instanceof NullNode == false) {
propertiesInstance.setSrvRecords(new ArrayList());
for (JsonNode sRVRecordsValue : ((ArrayNode) sRVRecordsArray2)) {
SrvRecord srvRecordInstance = new SrvRecord();
propertiesInstance.getSrvRecords().add(srvRecordInstance);
JsonNode priorityValue = sRVRecordsValue.get("priority");
if (priorityValue != null && priorityValue instanceof NullNode == false) {
int priorityInstance;
priorityInstance = priorityValue.getIntValue();
srvRecordInstance.setPriority(priorityInstance);
}
JsonNode weightValue = sRVRecordsValue.get("weight");
if (weightValue != null && weightValue instanceof NullNode == false) {
int weightInstance;
weightInstance = weightValue.getIntValue();
srvRecordInstance.setWeight(weightInstance);
}
JsonNode portValue = sRVRecordsValue.get("port");
if (portValue != null && portValue instanceof NullNode == false) {
int portInstance;
portInstance = portValue.getIntValue();
srvRecordInstance.setPort(portInstance);
}
JsonNode targetValue = sRVRecordsValue.get("target");
if (targetValue != null && targetValue instanceof NullNode == false) {
String targetInstance;
targetInstance = targetValue.getTextValue();
srvRecordInstance.setTarget(targetInstance);
}
}
}
JsonNode tXTRecordsArray2 = propertiesValue2.get("TXTRecords");
if (tXTRecordsArray2 != null && tXTRecordsArray2 instanceof NullNode == false) {
propertiesInstance.setTxtRecords(new ArrayList());
for (JsonNode tXTRecordsValue : ((ArrayNode) tXTRecordsArray2)) {
TxtRecord txtRecordInstance = new TxtRecord();
propertiesInstance.getTxtRecords().add(txtRecordInstance);
JsonNode valueValue = tXTRecordsValue.get("value");
if (valueValue != null && valueValue instanceof NullNode == false) {
String valueInstance;
valueInstance = valueValue.getTextValue();
txtRecordInstance.setValue(valueInstance);
}
}
}
JsonNode cNAMERecordValue2 = propertiesValue2.get("CNAMERecord");
if (cNAMERecordValue2 != null && cNAMERecordValue2 instanceof NullNode == false) {
CnameRecord cNAMERecordInstance = new CnameRecord();
propertiesInstance.setCnameRecord(cNAMERecordInstance);
JsonNode cnameValue = cNAMERecordValue2.get("cname");
if (cnameValue != null && cnameValue instanceof NullNode == false) {
String cnameInstance;
cnameInstance = cnameValue.getTextValue();
cNAMERecordInstance.setCname(cnameInstance);
}
}
JsonNode sOARecordValue2 = propertiesValue2.get("SOARecord");
if (sOARecordValue2 != null && sOARecordValue2 instanceof NullNode == false) {
SoaRecord sOARecordInstance = new SoaRecord();
propertiesInstance.setSoaRecord(sOARecordInstance);
JsonNode hostValue = sOARecordValue2.get("host");
if (hostValue != null && hostValue instanceof NullNode == false) {
String hostInstance;
hostInstance = hostValue.getTextValue();
sOARecordInstance.setHost(hostInstance);
}
JsonNode emailValue = sOARecordValue2.get("email");
if (emailValue != null && emailValue instanceof NullNode == false) {
String emailInstance;
emailInstance = emailValue.getTextValue();
sOARecordInstance.setEmail(emailInstance);
}
JsonNode serialNumberValue = sOARecordValue2.get("serialNumber");
if (serialNumberValue != null && serialNumberValue instanceof NullNode == false) {
long serialNumberInstance;
serialNumberInstance = serialNumberValue.getLongValue();
sOARecordInstance.setSerialNumber(serialNumberInstance);
}
JsonNode refreshTimeValue = sOARecordValue2.get("refreshTime");
if (refreshTimeValue != null && refreshTimeValue instanceof NullNode == false) {
long refreshTimeInstance;
refreshTimeInstance = refreshTimeValue.getLongValue();
sOARecordInstance.setRefreshTime(refreshTimeInstance);
}
JsonNode retryTimeValue = sOARecordValue2.get("retryTime");
if (retryTimeValue != null && retryTimeValue instanceof NullNode == false) {
long retryTimeInstance;
retryTimeInstance = retryTimeValue.getLongValue();
sOARecordInstance.setRetryTime(retryTimeInstance);
}
JsonNode expireTimeValue = sOARecordValue2.get("expireTime");
if (expireTimeValue != null && expireTimeValue instanceof NullNode == false) {
long expireTimeInstance;
expireTimeInstance = expireTimeValue.getLongValue();
sOARecordInstance.setExpireTime(expireTimeInstance);
}
JsonNode minimumTTLValue = sOARecordValue2.get("minimumTTL");
if (minimumTTLValue != null && minimumTTLValue instanceof NullNode == false) {
long minimumTTLInstance;
minimumTTLInstance = minimumTTLValue.getLongValue();
sOARecordInstance.setMinimumTtl(minimumTTLInstance);
}
}
}
JsonNode idValue = responseDoc.get("id");
if (idValue != null && idValue instanceof NullNode == false) {
String idInstance;
idInstance = idValue.getTextValue();
recordSetInstance.setId(idInstance);
}
JsonNode nameValue = responseDoc.get("name");
if (nameValue != null && nameValue instanceof NullNode == false) {
String nameInstance;
nameInstance = nameValue.getTextValue();
recordSetInstance.setName(nameInstance);
}
JsonNode typeValue = responseDoc.get("type");
if (typeValue != null && typeValue instanceof NullNode == false) {
String typeInstance;
typeInstance = typeValue.getTextValue();
recordSetInstance.setType(typeInstance);
}
JsonNode locationValue = responseDoc.get("location");
if (locationValue != null && locationValue instanceof NullNode == false) {
String locationInstance;
locationInstance = locationValue.getTextValue();
recordSetInstance.setLocation(locationInstance);
}
JsonNode tagsSequenceElement = ((JsonNode) responseDoc.get("tags"));
if (tagsSequenceElement != null && tagsSequenceElement instanceof NullNode == false) {
Iterator> itr = tagsSequenceElement.getFields();
while (itr.hasNext()) {
Map.Entry property = itr.next();
String tagsKey2 = property.getKey();
String tagsValue2 = property.getValue().getTextValue();
recordSetInstance.getTags().put(tagsKey2, tagsValue2);
}
}
}
result.setStatusCode(statusCode);
if (httpResponse.getHeaders("x-ms-request-id").length > 0) {
result.setRequestId(httpResponse.getFirstHeader("x-ms-request-id").getValue());
}
if (shouldTrace) {
CloudTracing.exit(invocationId, result);
}
return result;
} finally {
if (httpResponse != null && httpResponse.getEntity() != null) {
httpResponse.getEntity().getContent().close();
}
}
}
/**
* Removes a RecordSet from a DNS zone.
*
* @param resourceGroupName Required. The name of the resource group.
* @param zoneName Required. The name of the zone without a terminating dot.
* @param relativeRecordSetName Required. The name of the RecordSet,
* relative to the name of the zone.
* @param recordType Required. The type of DNS record.
* @param parameters Required. The parameters supplied to delete a record
* set.
* @return A standard service response including an HTTP status code and
* request ID.
*/
@Override
public Future deleteAsync(final String resourceGroupName, final String zoneName, final String relativeRecordSetName, final RecordType recordType, final RecordSetDeleteParameters parameters) {
return this.getClient().getExecutorService().submit(new Callable() {
@Override
public OperationResponse call() throws Exception {
return delete(resourceGroupName, zoneName, relativeRecordSetName, recordType, parameters);
}
});
}
/**
* Removes a RecordSet from a DNS zone.
*
* @param resourceGroupName Required. The name of the resource group.
* @param zoneName Required. The name of the zone without a terminating dot.
* @param relativeRecordSetName Required. The name of the RecordSet,
* relative to the name of the zone.
* @param recordType Required. The type of DNS record.
* @param parameters Required. The parameters supplied to delete a record
* set.
* @throws IOException Signals that an I/O exception of some sort has
* occurred. This class is the general class of exceptions produced by
* failed or interrupted I/O operations.
* @throws ServiceException Thrown if an unexpected response is found.
* @return A standard service response including an HTTP status code and
* request ID.
*/
@Override
public OperationResponse delete(String resourceGroupName, String zoneName, String relativeRecordSetName, RecordType recordType, RecordSetDeleteParameters parameters) throws IOException, ServiceException {
// Validate
if (resourceGroupName == null) {
throw new NullPointerException("resourceGroupName");
}
if (zoneName == null) {
throw new NullPointerException("zoneName");
}
if (relativeRecordSetName == null) {
throw new NullPointerException("relativeRecordSetName");
}
if (recordType == null) {
throw new NullPointerException("recordType");
}
if (parameters == null) {
throw new NullPointerException("parameters");
}
// Tracing
boolean shouldTrace = CloudTracing.getIsEnabled();
String invocationId = null;
if (shouldTrace) {
invocationId = Long.toString(CloudTracing.getNextInvocationId());
HashMap tracingParameters = new HashMap();
tracingParameters.put("resourceGroupName", resourceGroupName);
tracingParameters.put("zoneName", zoneName);
tracingParameters.put("relativeRecordSetName", relativeRecordSetName);
tracingParameters.put("recordType", recordType);
tracingParameters.put("parameters", parameters);
CloudTracing.enter(invocationId, this, "deleteAsync", tracingParameters);
}
// Construct URL
String url = "";
url = url + "/subscriptions/";
if (this.getClient().getCredentials().getSubscriptionId() != null) {
url = url + URLEncoder.encode(this.getClient().getCredentials().getSubscriptionId(), "UTF-8");
}
url = url + "/resourceGroups/";
url = url + URLEncoder.encode(resourceGroupName, "UTF-8");
url = url + "/providers/";
url = url + "Microsoft.Network";
url = url + "/dnszones/";
url = url + URLEncoder.encode(zoneName, "UTF-8");
url = url + "/";
url = url + URLEncoder.encode(recordType.toString(), "UTF-8");
url = url + "/";
url = url + relativeRecordSetName;
ArrayList queryParameters = new ArrayList();
queryParameters.add("api-version=" + "2015-05-04-preview");
if (queryParameters.size() > 0) {
url = url + "?" + CollectionStringBuilder.join(queryParameters, "&");
}
String baseUrl = this.getClient().getBaseUri().toString();
// Trim '/' character from the end of baseUrl and beginning of url.
if (baseUrl.charAt(baseUrl.length() - 1) == '/') {
baseUrl = baseUrl.substring(0, (baseUrl.length() - 1) + 0);
}
if (url.charAt(0) == '/') {
url = url.substring(1);
}
url = baseUrl + "/" + url;
url = url.replace(" ", "%20");
// Create HTTP transport objects
CustomHttpDelete httpRequest = new CustomHttpDelete(url);
// Set Headers
httpRequest.setHeader("Content-Type", "application/json; charset=utf-8");
httpRequest.setHeader("If-Match", parameters.getIfMatch());
// Send Request
HttpResponse httpResponse = null;
try {
if (shouldTrace) {
CloudTracing.sendRequest(invocationId, httpRequest);
}
httpResponse = this.getClient().getHttpClient().execute(httpRequest);
if (shouldTrace) {
CloudTracing.receiveResponse(invocationId, httpResponse);
}
int statusCode = httpResponse.getStatusLine().getStatusCode();
if (statusCode >= HttpStatus.SC_BAD_REQUEST) {
ServiceException ex = ServiceException.createFromXml(httpRequest, null, httpResponse, httpResponse.getEntity());
if (shouldTrace) {
CloudTracing.error(invocationId, ex);
}
throw ex;
}
// Create Result
OperationResponse result = null;
// Deserialize Response
result = new OperationResponse();
result.setStatusCode(statusCode);
if (httpResponse.getHeaders("x-ms-request-id").length > 0) {
result.setRequestId(httpResponse.getFirstHeader("x-ms-request-id").getValue());
}
if (shouldTrace) {
CloudTracing.exit(invocationId, result);
}
return result;
} finally {
if (httpResponse != null && httpResponse.getEntity() != null) {
httpResponse.getEntity().getContent().close();
}
}
}
/**
* Gets a RecordSet.
*
* @param resourceGroupName Required. The name of the resource group.
* @param zoneName Required. The name of the zone without a terminating dot.
* @param relativeRecordSetName Required. The name of the RecordSet,
* relative to the name of the zone.
* @param recordType Required. The type of DNS record.
* @return The response to a RecordSet Get operation.
*/
@Override
public Future getAsync(final String resourceGroupName, final String zoneName, final String relativeRecordSetName, final RecordType recordType) {
return this.getClient().getExecutorService().submit(new Callable() {
@Override
public RecordSetGetResponse call() throws Exception {
return get(resourceGroupName, zoneName, relativeRecordSetName, recordType);
}
});
}
/**
* Gets a RecordSet.
*
* @param resourceGroupName Required. The name of the resource group.
* @param zoneName Required. The name of the zone without a terminating dot.
* @param relativeRecordSetName Required. The name of the RecordSet,
* relative to the name of the zone.
* @param recordType Required. The type of DNS record.
* @throws IOException Signals that an I/O exception of some sort has
* occurred. This class is the general class of exceptions produced by
* failed or interrupted I/O operations.
* @throws ServiceException Thrown if an unexpected response is found.
* @return The response to a RecordSet Get operation.
*/
@Override
public RecordSetGetResponse get(String resourceGroupName, String zoneName, String relativeRecordSetName, RecordType recordType) throws IOException, ServiceException {
// Validate
if (resourceGroupName == null) {
throw new NullPointerException("resourceGroupName");
}
if (zoneName == null) {
throw new NullPointerException("zoneName");
}
if (relativeRecordSetName == null) {
throw new NullPointerException("relativeRecordSetName");
}
if (recordType == null) {
throw new NullPointerException("recordType");
}
// Tracing
boolean shouldTrace = CloudTracing.getIsEnabled();
String invocationId = null;
if (shouldTrace) {
invocationId = Long.toString(CloudTracing.getNextInvocationId());
HashMap tracingParameters = new HashMap();
tracingParameters.put("resourceGroupName", resourceGroupName);
tracingParameters.put("zoneName", zoneName);
tracingParameters.put("relativeRecordSetName", relativeRecordSetName);
tracingParameters.put("recordType", recordType);
CloudTracing.enter(invocationId, this, "getAsync", tracingParameters);
}
// Construct URL
String url = "";
url = url + "/subscriptions/";
if (this.getClient().getCredentials().getSubscriptionId() != null) {
url = url + URLEncoder.encode(this.getClient().getCredentials().getSubscriptionId(), "UTF-8");
}
url = url + "/resourceGroups/";
url = url + URLEncoder.encode(resourceGroupName, "UTF-8");
url = url + "/providers/";
url = url + "Microsoft.Network";
url = url + "/dnszones/";
url = url + URLEncoder.encode(zoneName, "UTF-8");
url = url + "/";
url = url + URLEncoder.encode(recordType.toString(), "UTF-8");
url = url + "/";
url = url + relativeRecordSetName;
ArrayList queryParameters = new ArrayList();
queryParameters.add("api-version=" + "2015-05-04-preview");
if (queryParameters.size() > 0) {
url = url + "?" + CollectionStringBuilder.join(queryParameters, "&");
}
String baseUrl = this.getClient().getBaseUri().toString();
// Trim '/' character from the end of baseUrl and beginning of url.
if (baseUrl.charAt(baseUrl.length() - 1) == '/') {
baseUrl = baseUrl.substring(0, (baseUrl.length() - 1) + 0);
}
if (url.charAt(0) == '/') {
url = url.substring(1);
}
url = baseUrl + "/" + url;
url = url.replace(" ", "%20");
// Create HTTP transport objects
HttpGet httpRequest = new HttpGet(url);
// Set Headers
httpRequest.setHeader("Content-Type", "application/json; charset=utf-8");
// Send Request
HttpResponse httpResponse = null;
try {
if (shouldTrace) {
CloudTracing.sendRequest(invocationId, httpRequest);
}
httpResponse = this.getClient().getHttpClient().execute(httpRequest);
if (shouldTrace) {
CloudTracing.receiveResponse(invocationId, httpResponse);
}
int statusCode = httpResponse.getStatusLine().getStatusCode();
if (statusCode >= HttpStatus.SC_BAD_REQUEST) {
ServiceException ex = ServiceException.createFromJson(httpRequest, null, httpResponse, httpResponse.getEntity());
if (shouldTrace) {
CloudTracing.error(invocationId, ex);
}
throw ex;
}
// Create Result
RecordSetGetResponse result = null;
// Deserialize Response
InputStream responseContent = httpResponse.getEntity().getContent();
result = new RecordSetGetResponse();
ObjectMapper objectMapper = new ObjectMapper();
JsonNode responseDoc = null;
String responseDocContent = IOUtils.toString(responseContent);
if (responseDocContent == null == false && responseDocContent.length() > 0) {
responseDoc = objectMapper.readTree(responseDocContent);
}
if (responseDoc != null && responseDoc instanceof NullNode == false) {
RecordSet recordSetInstance = new RecordSet();
result.setRecordSet(recordSetInstance);
JsonNode etagValue = responseDoc.get("etag");
if (etagValue != null && etagValue instanceof NullNode == false) {
String etagInstance;
etagInstance = etagValue.getTextValue();
recordSetInstance.setETag(etagInstance);
}
JsonNode propertiesValue = responseDoc.get("properties");
if (propertiesValue != null && propertiesValue instanceof NullNode == false) {
RecordSetProperties propertiesInstance = new RecordSetProperties();
recordSetInstance.setProperties(propertiesInstance);
JsonNode tTLValue = propertiesValue.get("TTL");
if (tTLValue != null && tTLValue instanceof NullNode == false) {
long tTLInstance;
tTLInstance = tTLValue.getLongValue();
propertiesInstance.setTtl(tTLInstance);
}
JsonNode aRecordsArray = propertiesValue.get("ARecords");
if (aRecordsArray != null && aRecordsArray instanceof NullNode == false) {
propertiesInstance.setARecords(new ArrayList());
for (JsonNode aRecordsValue : ((ArrayNode) aRecordsArray)) {
ARecord aRecordInstance = new ARecord();
propertiesInstance.getARecords().add(aRecordInstance);
JsonNode ipv4AddressValue = aRecordsValue.get("ipv4Address");
if (ipv4AddressValue != null && ipv4AddressValue instanceof NullNode == false) {
String ipv4AddressInstance;
ipv4AddressInstance = ipv4AddressValue.getTextValue();
aRecordInstance.setIpv4Address(ipv4AddressInstance);
}
}
}
JsonNode aAAARecordsArray = propertiesValue.get("AAAARecords");
if (aAAARecordsArray != null && aAAARecordsArray instanceof NullNode == false) {
propertiesInstance.setAaaaRecords(new ArrayList());
for (JsonNode aAAARecordsValue : ((ArrayNode) aAAARecordsArray)) {
AaaaRecord aaaaRecordInstance = new AaaaRecord();
propertiesInstance.getAaaaRecords().add(aaaaRecordInstance);
JsonNode ipv6AddressValue = aAAARecordsValue.get("ipv6Address");
if (ipv6AddressValue != null && ipv6AddressValue instanceof NullNode == false) {
String ipv6AddressInstance;
ipv6AddressInstance = ipv6AddressValue.getTextValue();
aaaaRecordInstance.setIpv6Address(ipv6AddressInstance);
}
}
}
JsonNode mXRecordsArray = propertiesValue.get("MXRecords");
if (mXRecordsArray != null && mXRecordsArray instanceof NullNode == false) {
propertiesInstance.setMxRecords(new ArrayList());
for (JsonNode mXRecordsValue : ((ArrayNode) mXRecordsArray)) {
MxRecord mxRecordInstance = new MxRecord();
propertiesInstance.getMxRecords().add(mxRecordInstance);
JsonNode preferenceValue = mXRecordsValue.get("preference");
if (preferenceValue != null && preferenceValue instanceof NullNode == false) {
int preferenceInstance;
preferenceInstance = preferenceValue.getIntValue();
mxRecordInstance.setPreference(preferenceInstance);
}
JsonNode exchangeValue = mXRecordsValue.get("exchange");
if (exchangeValue != null && exchangeValue instanceof NullNode == false) {
String exchangeInstance;
exchangeInstance = exchangeValue.getTextValue();
mxRecordInstance.setExchange(exchangeInstance);
}
}
}
JsonNode nSRecordsArray = propertiesValue.get("NSRecords");
if (nSRecordsArray != null && nSRecordsArray instanceof NullNode == false) {
propertiesInstance.setNsRecords(new ArrayList());
for (JsonNode nSRecordsValue : ((ArrayNode) nSRecordsArray)) {
NsRecord nsRecordInstance = new NsRecord();
propertiesInstance.getNsRecords().add(nsRecordInstance);
JsonNode nsdnameValue = nSRecordsValue.get("nsdname");
if (nsdnameValue != null && nsdnameValue instanceof NullNode == false) {
String nsdnameInstance;
nsdnameInstance = nsdnameValue.getTextValue();
nsRecordInstance.setNsdname(nsdnameInstance);
}
}
}
JsonNode pTRRecordsArray = propertiesValue.get("PTRRecords");
if (pTRRecordsArray != null && pTRRecordsArray instanceof NullNode == false) {
propertiesInstance.setPtrRecords(new ArrayList());
for (JsonNode pTRRecordsValue : ((ArrayNode) pTRRecordsArray)) {
PtrRecord ptrRecordInstance = new PtrRecord();
propertiesInstance.getPtrRecords().add(ptrRecordInstance);
JsonNode ptrdnameValue = pTRRecordsValue.get("ptrdname");
if (ptrdnameValue != null && ptrdnameValue instanceof NullNode == false) {
String ptrdnameInstance;
ptrdnameInstance = ptrdnameValue.getTextValue();
ptrRecordInstance.setPtrdname(ptrdnameInstance);
}
}
}
JsonNode sRVRecordsArray = propertiesValue.get("SRVRecords");
if (sRVRecordsArray != null && sRVRecordsArray instanceof NullNode == false) {
propertiesInstance.setSrvRecords(new ArrayList());
for (JsonNode sRVRecordsValue : ((ArrayNode) sRVRecordsArray)) {
SrvRecord srvRecordInstance = new SrvRecord();
propertiesInstance.getSrvRecords().add(srvRecordInstance);
JsonNode priorityValue = sRVRecordsValue.get("priority");
if (priorityValue != null && priorityValue instanceof NullNode == false) {
int priorityInstance;
priorityInstance = priorityValue.getIntValue();
srvRecordInstance.setPriority(priorityInstance);
}
JsonNode weightValue = sRVRecordsValue.get("weight");
if (weightValue != null && weightValue instanceof NullNode == false) {
int weightInstance;
weightInstance = weightValue.getIntValue();
srvRecordInstance.setWeight(weightInstance);
}
JsonNode portValue = sRVRecordsValue.get("port");
if (portValue != null && portValue instanceof NullNode == false) {
int portInstance;
portInstance = portValue.getIntValue();
srvRecordInstance.setPort(portInstance);
}
JsonNode targetValue = sRVRecordsValue.get("target");
if (targetValue != null && targetValue instanceof NullNode == false) {
String targetInstance;
targetInstance = targetValue.getTextValue();
srvRecordInstance.setTarget(targetInstance);
}
}
}
JsonNode tXTRecordsArray = propertiesValue.get("TXTRecords");
if (tXTRecordsArray != null && tXTRecordsArray instanceof NullNode == false) {
propertiesInstance.setTxtRecords(new ArrayList());
for (JsonNode tXTRecordsValue : ((ArrayNode) tXTRecordsArray)) {
TxtRecord txtRecordInstance = new TxtRecord();
propertiesInstance.getTxtRecords().add(txtRecordInstance);
JsonNode valueValue = tXTRecordsValue.get("value");
if (valueValue != null && valueValue instanceof NullNode == false) {
String valueInstance;
valueInstance = valueValue.getTextValue();
txtRecordInstance.setValue(valueInstance);
}
}
}
JsonNode cNAMERecordValue = propertiesValue.get("CNAMERecord");
if (cNAMERecordValue != null && cNAMERecordValue instanceof NullNode == false) {
CnameRecord cNAMERecordInstance = new CnameRecord();
propertiesInstance.setCnameRecord(cNAMERecordInstance);
JsonNode cnameValue = cNAMERecordValue.get("cname");
if (cnameValue != null && cnameValue instanceof NullNode == false) {
String cnameInstance;
cnameInstance = cnameValue.getTextValue();
cNAMERecordInstance.setCname(cnameInstance);
}
}
JsonNode sOARecordValue = propertiesValue.get("SOARecord");
if (sOARecordValue != null && sOARecordValue instanceof NullNode == false) {
SoaRecord sOARecordInstance = new SoaRecord();
propertiesInstance.setSoaRecord(sOARecordInstance);
JsonNode hostValue = sOARecordValue.get("host");
if (hostValue != null && hostValue instanceof NullNode == false) {
String hostInstance;
hostInstance = hostValue.getTextValue();
sOARecordInstance.setHost(hostInstance);
}
JsonNode emailValue = sOARecordValue.get("email");
if (emailValue != null && emailValue instanceof NullNode == false) {
String emailInstance;
emailInstance = emailValue.getTextValue();
sOARecordInstance.setEmail(emailInstance);
}
JsonNode serialNumberValue = sOARecordValue.get("serialNumber");
if (serialNumberValue != null && serialNumberValue instanceof NullNode == false) {
long serialNumberInstance;
serialNumberInstance = serialNumberValue.getLongValue();
sOARecordInstance.setSerialNumber(serialNumberInstance);
}
JsonNode refreshTimeValue = sOARecordValue.get("refreshTime");
if (refreshTimeValue != null && refreshTimeValue instanceof NullNode == false) {
long refreshTimeInstance;
refreshTimeInstance = refreshTimeValue.getLongValue();
sOARecordInstance.setRefreshTime(refreshTimeInstance);
}
JsonNode retryTimeValue = sOARecordValue.get("retryTime");
if (retryTimeValue != null && retryTimeValue instanceof NullNode == false) {
long retryTimeInstance;
retryTimeInstance = retryTimeValue.getLongValue();
sOARecordInstance.setRetryTime(retryTimeInstance);
}
JsonNode expireTimeValue = sOARecordValue.get("expireTime");
if (expireTimeValue != null && expireTimeValue instanceof NullNode == false) {
long expireTimeInstance;
expireTimeInstance = expireTimeValue.getLongValue();
sOARecordInstance.setExpireTime(expireTimeInstance);
}
JsonNode minimumTTLValue = sOARecordValue.get("minimumTTL");
if (minimumTTLValue != null && minimumTTLValue instanceof NullNode == false) {
long minimumTTLInstance;
minimumTTLInstance = minimumTTLValue.getLongValue();
sOARecordInstance.setMinimumTtl(minimumTTLInstance);
}
}
}
JsonNode idValue = responseDoc.get("id");
if (idValue != null && idValue instanceof NullNode == false) {
String idInstance;
idInstance = idValue.getTextValue();
recordSetInstance.setId(idInstance);
}
JsonNode nameValue = responseDoc.get("name");
if (nameValue != null && nameValue instanceof NullNode == false) {
String nameInstance;
nameInstance = nameValue.getTextValue();
recordSetInstance.setName(nameInstance);
}
JsonNode typeValue = responseDoc.get("type");
if (typeValue != null && typeValue instanceof NullNode == false) {
String typeInstance;
typeInstance = typeValue.getTextValue();
recordSetInstance.setType(typeInstance);
}
JsonNode locationValue = responseDoc.get("location");
if (locationValue != null && locationValue instanceof NullNode == false) {
String locationInstance;
locationInstance = locationValue.getTextValue();
recordSetInstance.setLocation(locationInstance);
}
JsonNode tagsSequenceElement = ((JsonNode) responseDoc.get("tags"));
if (tagsSequenceElement != null && tagsSequenceElement instanceof NullNode == false) {
Iterator> itr = tagsSequenceElement.getFields();
while (itr.hasNext()) {
Map.Entry property = itr.next();
String tagsKey = property.getKey();
String tagsValue = property.getValue().getTextValue();
recordSetInstance.getTags().put(tagsKey, tagsValue);
}
}
}
result.setStatusCode(statusCode);
if (httpResponse.getHeaders("x-ms-request-id").length > 0) {
result.setRequestId(httpResponse.getFirstHeader("x-ms-request-id").getValue());
}
if (shouldTrace) {
CloudTracing.exit(invocationId, result);
}
return result;
} finally {
if (httpResponse != null && httpResponse.getEntity() != null) {
httpResponse.getEntity().getContent().close();
}
}
}
/**
* Lists the RecordSets of a specified type in a DNS zone.
*
* @param resourceGroupName Required. The name of the resource group that
* contains the zone.
* @param zoneName Required. The name of the zone from which to enumerate
* RecordsSets.
* @param recordType Required. The type of record sets to enumerate.
* @param parameters Optional. Query parameters. If null is passed returns
* the default number of zones.
* @return The response to a RecordSet List operation.
*/
@Override
public Future listAsync(final String resourceGroupName, final String zoneName, final RecordType recordType, final RecordSetListParameters parameters) {
return this.getClient().getExecutorService().submit(new Callable() {
@Override
public RecordSetListResponse call() throws Exception {
return list(resourceGroupName, zoneName, recordType, parameters);
}
});
}
/**
* Lists the RecordSets of a specified type in a DNS zone.
*
* @param resourceGroupName Required. The name of the resource group that
* contains the zone.
* @param zoneName Required. The name of the zone from which to enumerate
* RecordsSets.
* @param recordType Required. The type of record sets to enumerate.
* @param parameters Optional. Query parameters. If null is passed returns
* the default number of zones.
* @throws IOException Signals that an I/O exception of some sort has
* occurred. This class is the general class of exceptions produced by
* failed or interrupted I/O operations.
* @throws ServiceException Thrown if an unexpected response is found.
* @return The response to a RecordSet List operation.
*/
@Override
public RecordSetListResponse list(String resourceGroupName, String zoneName, RecordType recordType, RecordSetListParameters parameters) throws IOException, ServiceException {
// Validate
if (resourceGroupName == null) {
throw new NullPointerException("resourceGroupName");
}
if (zoneName == null) {
throw new NullPointerException("zoneName");
}
// Tracing
boolean shouldTrace = CloudTracing.getIsEnabled();
String invocationId = null;
if (shouldTrace) {
invocationId = Long.toString(CloudTracing.getNextInvocationId());
HashMap tracingParameters = new HashMap();
tracingParameters.put("resourceGroupName", resourceGroupName);
tracingParameters.put("zoneName", zoneName);
tracingParameters.put("recordType", recordType);
tracingParameters.put("parameters", parameters);
CloudTracing.enter(invocationId, this, "listAsync", tracingParameters);
}
// Construct URL
String url = "";
url = url + "/subscriptions/";
if (this.getClient().getCredentials().getSubscriptionId() != null) {
url = url + URLEncoder.encode(this.getClient().getCredentials().getSubscriptionId(), "UTF-8");
}
url = url + "/resourceGroups/";
url = url + URLEncoder.encode(resourceGroupName, "UTF-8");
url = url + "/providers/";
url = url + "Microsoft.Network";
url = url + "/dnszones/";
url = url + URLEncoder.encode(zoneName, "UTF-8");
url = url + "/";
if (recordType != null) {
url = url + URLEncoder.encode(recordType.toString(), "UTF-8");
}
ArrayList queryParameters = new ArrayList();
if (parameters != null && parameters.getTop() != null) {
queryParameters.add("$top=" + URLEncoder.encode(parameters.getTop(), "UTF-8"));
}
ArrayList odataFilter = new ArrayList();
if (parameters != null && parameters.getFilter() != null) {
odataFilter.add(URLEncoder.encode(parameters.getFilter(), "UTF-8"));
}
if (odataFilter.size() > 0) {
queryParameters.add("$filter=" + CollectionStringBuilder.join(odataFilter, null));
}
queryParameters.add("api-version=" + "2015-05-04-preview");
if (queryParameters.size() > 0) {
url = url + "?" + CollectionStringBuilder.join(queryParameters, "&");
}
String baseUrl = this.getClient().getBaseUri().toString();
// Trim '/' character from the end of baseUrl and beginning of url.
if (baseUrl.charAt(baseUrl.length() - 1) == '/') {
baseUrl = baseUrl.substring(0, (baseUrl.length() - 1) + 0);
}
if (url.charAt(0) == '/') {
url = url.substring(1);
}
url = baseUrl + "/" + url;
url = url.replace(" ", "%20");
// Create HTTP transport objects
HttpGet httpRequest = new HttpGet(url);
// Set Headers
httpRequest.setHeader("Content-Type", "application/json; charset=utf-8");
// Send Request
HttpResponse httpResponse = null;
try {
if (shouldTrace) {
CloudTracing.sendRequest(invocationId, httpRequest);
}
httpResponse = this.getClient().getHttpClient().execute(httpRequest);
if (shouldTrace) {
CloudTracing.receiveResponse(invocationId, httpResponse);
}
int statusCode = httpResponse.getStatusLine().getStatusCode();
if (statusCode >= HttpStatus.SC_BAD_REQUEST) {
ServiceException ex = ServiceException.createFromJson(httpRequest, null, httpResponse, httpResponse.getEntity());
if (shouldTrace) {
CloudTracing.error(invocationId, ex);
}
throw ex;
}
// Create Result
RecordSetListResponse result = null;
// Deserialize Response
InputStream responseContent = httpResponse.getEntity().getContent();
result = new RecordSetListResponse();
ObjectMapper objectMapper = new ObjectMapper();
JsonNode responseDoc = null;
String responseDocContent = IOUtils.toString(responseContent);
if (responseDocContent == null == false && responseDocContent.length() > 0) {
responseDoc = objectMapper.readTree(responseDocContent);
}
if (responseDoc != null && responseDoc instanceof NullNode == false) {
JsonNode valueArray = responseDoc.get("value");
if (valueArray != null && valueArray instanceof NullNode == false) {
for (JsonNode valueValue : ((ArrayNode) valueArray)) {
RecordSet recordSetInstance = new RecordSet();
result.getRecordSets().add(recordSetInstance);
JsonNode etagValue = valueValue.get("etag");
if (etagValue != null && etagValue instanceof NullNode == false) {
String etagInstance;
etagInstance = etagValue.getTextValue();
recordSetInstance.setETag(etagInstance);
}
JsonNode propertiesValue = valueValue.get("properties");
if (propertiesValue != null && propertiesValue instanceof NullNode == false) {
RecordSetProperties propertiesInstance = new RecordSetProperties();
recordSetInstance.setProperties(propertiesInstance);
JsonNode tTLValue = propertiesValue.get("TTL");
if (tTLValue != null && tTLValue instanceof NullNode == false) {
long tTLInstance;
tTLInstance = tTLValue.getLongValue();
propertiesInstance.setTtl(tTLInstance);
}
JsonNode aRecordsArray = propertiesValue.get("ARecords");
if (aRecordsArray != null && aRecordsArray instanceof NullNode == false) {
propertiesInstance.setARecords(new ArrayList());
for (JsonNode aRecordsValue : ((ArrayNode) aRecordsArray)) {
ARecord aRecordInstance = new ARecord();
propertiesInstance.getARecords().add(aRecordInstance);
JsonNode ipv4AddressValue = aRecordsValue.get("ipv4Address");
if (ipv4AddressValue != null && ipv4AddressValue instanceof NullNode == false) {
String ipv4AddressInstance;
ipv4AddressInstance = ipv4AddressValue.getTextValue();
aRecordInstance.setIpv4Address(ipv4AddressInstance);
}
}
}
JsonNode aAAARecordsArray = propertiesValue.get("AAAARecords");
if (aAAARecordsArray != null && aAAARecordsArray instanceof NullNode == false) {
propertiesInstance.setAaaaRecords(new ArrayList());
for (JsonNode aAAARecordsValue : ((ArrayNode) aAAARecordsArray)) {
AaaaRecord aaaaRecordInstance = new AaaaRecord();
propertiesInstance.getAaaaRecords().add(aaaaRecordInstance);
JsonNode ipv6AddressValue = aAAARecordsValue.get("ipv6Address");
if (ipv6AddressValue != null && ipv6AddressValue instanceof NullNode == false) {
String ipv6AddressInstance;
ipv6AddressInstance = ipv6AddressValue.getTextValue();
aaaaRecordInstance.setIpv6Address(ipv6AddressInstance);
}
}
}
JsonNode mXRecordsArray = propertiesValue.get("MXRecords");
if (mXRecordsArray != null && mXRecordsArray instanceof NullNode == false) {
propertiesInstance.setMxRecords(new ArrayList());
for (JsonNode mXRecordsValue : ((ArrayNode) mXRecordsArray)) {
MxRecord mxRecordInstance = new MxRecord();
propertiesInstance.getMxRecords().add(mxRecordInstance);
JsonNode preferenceValue = mXRecordsValue.get("preference");
if (preferenceValue != null && preferenceValue instanceof NullNode == false) {
int preferenceInstance;
preferenceInstance = preferenceValue.getIntValue();
mxRecordInstance.setPreference(preferenceInstance);
}
JsonNode exchangeValue = mXRecordsValue.get("exchange");
if (exchangeValue != null && exchangeValue instanceof NullNode == false) {
String exchangeInstance;
exchangeInstance = exchangeValue.getTextValue();
mxRecordInstance.setExchange(exchangeInstance);
}
}
}
JsonNode nSRecordsArray = propertiesValue.get("NSRecords");
if (nSRecordsArray != null && nSRecordsArray instanceof NullNode == false) {
propertiesInstance.setNsRecords(new ArrayList());
for (JsonNode nSRecordsValue : ((ArrayNode) nSRecordsArray)) {
NsRecord nsRecordInstance = new NsRecord();
propertiesInstance.getNsRecords().add(nsRecordInstance);
JsonNode nsdnameValue = nSRecordsValue.get("nsdname");
if (nsdnameValue != null && nsdnameValue instanceof NullNode == false) {
String nsdnameInstance;
nsdnameInstance = nsdnameValue.getTextValue();
nsRecordInstance.setNsdname(nsdnameInstance);
}
}
}
JsonNode pTRRecordsArray = propertiesValue.get("PTRRecords");
if (pTRRecordsArray != null && pTRRecordsArray instanceof NullNode == false) {
propertiesInstance.setPtrRecords(new ArrayList());
for (JsonNode pTRRecordsValue : ((ArrayNode) pTRRecordsArray)) {
PtrRecord ptrRecordInstance = new PtrRecord();
propertiesInstance.getPtrRecords().add(ptrRecordInstance);
JsonNode ptrdnameValue = pTRRecordsValue.get("ptrdname");
if (ptrdnameValue != null && ptrdnameValue instanceof NullNode == false) {
String ptrdnameInstance;
ptrdnameInstance = ptrdnameValue.getTextValue();
ptrRecordInstance.setPtrdname(ptrdnameInstance);
}
}
}
JsonNode sRVRecordsArray = propertiesValue.get("SRVRecords");
if (sRVRecordsArray != null && sRVRecordsArray instanceof NullNode == false) {
propertiesInstance.setSrvRecords(new ArrayList());
for (JsonNode sRVRecordsValue : ((ArrayNode) sRVRecordsArray)) {
SrvRecord srvRecordInstance = new SrvRecord();
propertiesInstance.getSrvRecords().add(srvRecordInstance);
JsonNode priorityValue = sRVRecordsValue.get("priority");
if (priorityValue != null && priorityValue instanceof NullNode == false) {
int priorityInstance;
priorityInstance = priorityValue.getIntValue();
srvRecordInstance.setPriority(priorityInstance);
}
JsonNode weightValue = sRVRecordsValue.get("weight");
if (weightValue != null && weightValue instanceof NullNode == false) {
int weightInstance;
weightInstance = weightValue.getIntValue();
srvRecordInstance.setWeight(weightInstance);
}
JsonNode portValue = sRVRecordsValue.get("port");
if (portValue != null && portValue instanceof NullNode == false) {
int portInstance;
portInstance = portValue.getIntValue();
srvRecordInstance.setPort(portInstance);
}
JsonNode targetValue = sRVRecordsValue.get("target");
if (targetValue != null && targetValue instanceof NullNode == false) {
String targetInstance;
targetInstance = targetValue.getTextValue();
srvRecordInstance.setTarget(targetInstance);
}
}
}
JsonNode tXTRecordsArray = propertiesValue.get("TXTRecords");
if (tXTRecordsArray != null && tXTRecordsArray instanceof NullNode == false) {
propertiesInstance.setTxtRecords(new ArrayList());
for (JsonNode tXTRecordsValue : ((ArrayNode) tXTRecordsArray)) {
TxtRecord txtRecordInstance = new TxtRecord();
propertiesInstance.getTxtRecords().add(txtRecordInstance);
JsonNode valueValue2 = tXTRecordsValue.get("value");
if (valueValue2 != null && valueValue2 instanceof NullNode == false) {
String valueInstance;
valueInstance = valueValue2.getTextValue();
txtRecordInstance.setValue(valueInstance);
}
}
}
JsonNode cNAMERecordValue = propertiesValue.get("CNAMERecord");
if (cNAMERecordValue != null && cNAMERecordValue instanceof NullNode == false) {
CnameRecord cNAMERecordInstance = new CnameRecord();
propertiesInstance.setCnameRecord(cNAMERecordInstance);
JsonNode cnameValue = cNAMERecordValue.get("cname");
if (cnameValue != null && cnameValue instanceof NullNode == false) {
String cnameInstance;
cnameInstance = cnameValue.getTextValue();
cNAMERecordInstance.setCname(cnameInstance);
}
}
JsonNode sOARecordValue = propertiesValue.get("SOARecord");
if (sOARecordValue != null && sOARecordValue instanceof NullNode == false) {
SoaRecord sOARecordInstance = new SoaRecord();
propertiesInstance.setSoaRecord(sOARecordInstance);
JsonNode hostValue = sOARecordValue.get("host");
if (hostValue != null && hostValue instanceof NullNode == false) {
String hostInstance;
hostInstance = hostValue.getTextValue();
sOARecordInstance.setHost(hostInstance);
}
JsonNode emailValue = sOARecordValue.get("email");
if (emailValue != null && emailValue instanceof NullNode == false) {
String emailInstance;
emailInstance = emailValue.getTextValue();
sOARecordInstance.setEmail(emailInstance);
}
JsonNode serialNumberValue = sOARecordValue.get("serialNumber");
if (serialNumberValue != null && serialNumberValue instanceof NullNode == false) {
long serialNumberInstance;
serialNumberInstance = serialNumberValue.getLongValue();
sOARecordInstance.setSerialNumber(serialNumberInstance);
}
JsonNode refreshTimeValue = sOARecordValue.get("refreshTime");
if (refreshTimeValue != null && refreshTimeValue instanceof NullNode == false) {
long refreshTimeInstance;
refreshTimeInstance = refreshTimeValue.getLongValue();
sOARecordInstance.setRefreshTime(refreshTimeInstance);
}
JsonNode retryTimeValue = sOARecordValue.get("retryTime");
if (retryTimeValue != null && retryTimeValue instanceof NullNode == false) {
long retryTimeInstance;
retryTimeInstance = retryTimeValue.getLongValue();
sOARecordInstance.setRetryTime(retryTimeInstance);
}
JsonNode expireTimeValue = sOARecordValue.get("expireTime");
if (expireTimeValue != null && expireTimeValue instanceof NullNode == false) {
long expireTimeInstance;
expireTimeInstance = expireTimeValue.getLongValue();
sOARecordInstance.setExpireTime(expireTimeInstance);
}
JsonNode minimumTTLValue = sOARecordValue.get("minimumTTL");
if (minimumTTLValue != null && minimumTTLValue instanceof NullNode == false) {
long minimumTTLInstance;
minimumTTLInstance = minimumTTLValue.getLongValue();
sOARecordInstance.setMinimumTtl(minimumTTLInstance);
}
}
}
JsonNode idValue = valueValue.get("id");
if (idValue != null && idValue instanceof NullNode == false) {
String idInstance;
idInstance = idValue.getTextValue();
recordSetInstance.setId(idInstance);
}
JsonNode nameValue = valueValue.get("name");
if (nameValue != null && nameValue instanceof NullNode == false) {
String nameInstance;
nameInstance = nameValue.getTextValue();
recordSetInstance.setName(nameInstance);
}
JsonNode typeValue = valueValue.get("type");
if (typeValue != null && typeValue instanceof NullNode == false) {
String typeInstance;
typeInstance = typeValue.getTextValue();
recordSetInstance.setType(typeInstance);
}
JsonNode locationValue = valueValue.get("location");
if (locationValue != null && locationValue instanceof NullNode == false) {
String locationInstance;
locationInstance = locationValue.getTextValue();
recordSetInstance.setLocation(locationInstance);
}
JsonNode tagsSequenceElement = ((JsonNode) valueValue.get("tags"));
if (tagsSequenceElement != null && tagsSequenceElement instanceof NullNode == false) {
Iterator> itr = tagsSequenceElement.getFields();
while (itr.hasNext()) {
Map.Entry property = itr.next();
String tagsKey = property.getKey();
String tagsValue = property.getValue().getTextValue();
recordSetInstance.getTags().put(tagsKey, tagsValue);
}
}
}
}
JsonNode nextLinkValue = responseDoc.get("nextLink");
if (nextLinkValue != null && nextLinkValue instanceof NullNode == false) {
String nextLinkInstance;
nextLinkInstance = nextLinkValue.getTextValue();
result.setNextLink(nextLinkInstance);
}
}
result.setStatusCode(statusCode);
if (httpResponse.getHeaders("x-ms-request-id").length > 0) {
result.setRequestId(httpResponse.getFirstHeader("x-ms-request-id").getValue());
}
if (shouldTrace) {
CloudTracing.exit(invocationId, result);
}
return result;
} finally {
if (httpResponse != null && httpResponse.getEntity() != null) {
httpResponse.getEntity().getContent().close();
}
}
}
/**
* Lists all RecordSets in a DNS zone.
*
* @param resourceGroupName Required. The name of the resource group that
* contains the zone.
* @param zoneName Required. The name of the zone from which to enumerate
* RecordSets.
* @param parameters Optional. Query parameters. If null is passed returns
* the default number of zones.
* @return The response to a RecordSet List operation.
*/
@Override
public Future listAllAsync(final String resourceGroupName, final String zoneName, final RecordSetListParameters parameters) {
return this.getClient().getExecutorService().submit(new Callable() {
@Override
public RecordSetListResponse call() throws Exception {
return listAll(resourceGroupName, zoneName, parameters);
}
});
}
/**
* Lists all RecordSets in a DNS zone.
*
* @param resourceGroupName Required. The name of the resource group that
* contains the zone.
* @param zoneName Required. The name of the zone from which to enumerate
* RecordSets.
* @param parameters Optional. Query parameters. If null is passed returns
* the default number of zones.
* @throws IOException Signals that an I/O exception of some sort has
* occurred. This class is the general class of exceptions produced by
* failed or interrupted I/O operations.
* @throws ServiceException Thrown if an unexpected response is found.
* @return The response to a RecordSet List operation.
*/
@Override
public RecordSetListResponse listAll(String resourceGroupName, String zoneName, RecordSetListParameters parameters) throws IOException, ServiceException {
// Validate
if (resourceGroupName == null) {
throw new NullPointerException("resourceGroupName");
}
if (zoneName == null) {
throw new NullPointerException("zoneName");
}
// Tracing
boolean shouldTrace = CloudTracing.getIsEnabled();
String invocationId = null;
if (shouldTrace) {
invocationId = Long.toString(CloudTracing.getNextInvocationId());
HashMap tracingParameters = new HashMap();
tracingParameters.put("resourceGroupName", resourceGroupName);
tracingParameters.put("zoneName", zoneName);
tracingParameters.put("parameters", parameters);
CloudTracing.enter(invocationId, this, "listAllAsync", tracingParameters);
}
// Construct URL
String url = "";
url = url + "/subscriptions/";
if (this.getClient().getCredentials().getSubscriptionId() != null) {
url = url + URLEncoder.encode(this.getClient().getCredentials().getSubscriptionId(), "UTF-8");
}
url = url + "/resourceGroups/";
url = url + URLEncoder.encode(resourceGroupName, "UTF-8");
url = url + "/providers/";
url = url + "Microsoft.Network";
url = url + "/dnszones/";
url = url + URLEncoder.encode(zoneName, "UTF-8");
url = url + "/recordsets";
ArrayList queryParameters = new ArrayList();
if (parameters != null && parameters.getTop() != null) {
queryParameters.add("$top=" + URLEncoder.encode(parameters.getTop(), "UTF-8"));
}
ArrayList odataFilter = new ArrayList();
if (parameters != null && parameters.getFilter() != null) {
odataFilter.add(URLEncoder.encode(parameters.getFilter(), "UTF-8"));
}
if (odataFilter.size() > 0) {
queryParameters.add("$filter=" + CollectionStringBuilder.join(odataFilter, null));
}
queryParameters.add("api-version=" + "2015-05-04-preview");
if (queryParameters.size() > 0) {
url = url + "?" + CollectionStringBuilder.join(queryParameters, "&");
}
String baseUrl = this.getClient().getBaseUri().toString();
// Trim '/' character from the end of baseUrl and beginning of url.
if (baseUrl.charAt(baseUrl.length() - 1) == '/') {
baseUrl = baseUrl.substring(0, (baseUrl.length() - 1) + 0);
}
if (url.charAt(0) == '/') {
url = url.substring(1);
}
url = baseUrl + "/" + url;
url = url.replace(" ", "%20");
// Create HTTP transport objects
HttpGet httpRequest = new HttpGet(url);
// Set Headers
httpRequest.setHeader("Content-Type", "application/json; charset=utf-8");
// Send Request
HttpResponse httpResponse = null;
try {
if (shouldTrace) {
CloudTracing.sendRequest(invocationId, httpRequest);
}
httpResponse = this.getClient().getHttpClient().execute(httpRequest);
if (shouldTrace) {
CloudTracing.receiveResponse(invocationId, httpResponse);
}
int statusCode = httpResponse.getStatusLine().getStatusCode();
if (statusCode >= HttpStatus.SC_BAD_REQUEST) {
ServiceException ex = ServiceException.createFromJson(httpRequest, null, httpResponse, httpResponse.getEntity());
if (shouldTrace) {
CloudTracing.error(invocationId, ex);
}
throw ex;
}
// Create Result
RecordSetListResponse result = null;
// Deserialize Response
InputStream responseContent = httpResponse.getEntity().getContent();
result = new RecordSetListResponse();
ObjectMapper objectMapper = new ObjectMapper();
JsonNode responseDoc = null;
String responseDocContent = IOUtils.toString(responseContent);
if (responseDocContent == null == false && responseDocContent.length() > 0) {
responseDoc = objectMapper.readTree(responseDocContent);
}
if (responseDoc != null && responseDoc instanceof NullNode == false) {
JsonNode valueArray = responseDoc.get("value");
if (valueArray != null && valueArray instanceof NullNode == false) {
for (JsonNode valueValue : ((ArrayNode) valueArray)) {
RecordSet recordSetInstance = new RecordSet();
result.getRecordSets().add(recordSetInstance);
JsonNode etagValue = valueValue.get("etag");
if (etagValue != null && etagValue instanceof NullNode == false) {
String etagInstance;
etagInstance = etagValue.getTextValue();
recordSetInstance.setETag(etagInstance);
}
JsonNode propertiesValue = valueValue.get("properties");
if (propertiesValue != null && propertiesValue instanceof NullNode == false) {
RecordSetProperties propertiesInstance = new RecordSetProperties();
recordSetInstance.setProperties(propertiesInstance);
JsonNode tTLValue = propertiesValue.get("TTL");
if (tTLValue != null && tTLValue instanceof NullNode == false) {
long tTLInstance;
tTLInstance = tTLValue.getLongValue();
propertiesInstance.setTtl(tTLInstance);
}
JsonNode aRecordsArray = propertiesValue.get("ARecords");
if (aRecordsArray != null && aRecordsArray instanceof NullNode == false) {
propertiesInstance.setARecords(new ArrayList());
for (JsonNode aRecordsValue : ((ArrayNode) aRecordsArray)) {
ARecord aRecordInstance = new ARecord();
propertiesInstance.getARecords().add(aRecordInstance);
JsonNode ipv4AddressValue = aRecordsValue.get("ipv4Address");
if (ipv4AddressValue != null && ipv4AddressValue instanceof NullNode == false) {
String ipv4AddressInstance;
ipv4AddressInstance = ipv4AddressValue.getTextValue();
aRecordInstance.setIpv4Address(ipv4AddressInstance);
}
}
}
JsonNode aAAARecordsArray = propertiesValue.get("AAAARecords");
if (aAAARecordsArray != null && aAAARecordsArray instanceof NullNode == false) {
propertiesInstance.setAaaaRecords(new ArrayList());
for (JsonNode aAAARecordsValue : ((ArrayNode) aAAARecordsArray)) {
AaaaRecord aaaaRecordInstance = new AaaaRecord();
propertiesInstance.getAaaaRecords().add(aaaaRecordInstance);
JsonNode ipv6AddressValue = aAAARecordsValue.get("ipv6Address");
if (ipv6AddressValue != null && ipv6AddressValue instanceof NullNode == false) {
String ipv6AddressInstance;
ipv6AddressInstance = ipv6AddressValue.getTextValue();
aaaaRecordInstance.setIpv6Address(ipv6AddressInstance);
}
}
}
JsonNode mXRecordsArray = propertiesValue.get("MXRecords");
if (mXRecordsArray != null && mXRecordsArray instanceof NullNode == false) {
propertiesInstance.setMxRecords(new ArrayList());
for (JsonNode mXRecordsValue : ((ArrayNode) mXRecordsArray)) {
MxRecord mxRecordInstance = new MxRecord();
propertiesInstance.getMxRecords().add(mxRecordInstance);
JsonNode preferenceValue = mXRecordsValue.get("preference");
if (preferenceValue != null && preferenceValue instanceof NullNode == false) {
int preferenceInstance;
preferenceInstance = preferenceValue.getIntValue();
mxRecordInstance.setPreference(preferenceInstance);
}
JsonNode exchangeValue = mXRecordsValue.get("exchange");
if (exchangeValue != null && exchangeValue instanceof NullNode == false) {
String exchangeInstance;
exchangeInstance = exchangeValue.getTextValue();
mxRecordInstance.setExchange(exchangeInstance);
}
}
}
JsonNode nSRecordsArray = propertiesValue.get("NSRecords");
if (nSRecordsArray != null && nSRecordsArray instanceof NullNode == false) {
propertiesInstance.setNsRecords(new ArrayList());
for (JsonNode nSRecordsValue : ((ArrayNode) nSRecordsArray)) {
NsRecord nsRecordInstance = new NsRecord();
propertiesInstance.getNsRecords().add(nsRecordInstance);
JsonNode nsdnameValue = nSRecordsValue.get("nsdname");
if (nsdnameValue != null && nsdnameValue instanceof NullNode == false) {
String nsdnameInstance;
nsdnameInstance = nsdnameValue.getTextValue();
nsRecordInstance.setNsdname(nsdnameInstance);
}
}
}
JsonNode pTRRecordsArray = propertiesValue.get("PTRRecords");
if (pTRRecordsArray != null && pTRRecordsArray instanceof NullNode == false) {
propertiesInstance.setPtrRecords(new ArrayList());
for (JsonNode pTRRecordsValue : ((ArrayNode) pTRRecordsArray)) {
PtrRecord ptrRecordInstance = new PtrRecord();
propertiesInstance.getPtrRecords().add(ptrRecordInstance);
JsonNode ptrdnameValue = pTRRecordsValue.get("ptrdname");
if (ptrdnameValue != null && ptrdnameValue instanceof NullNode == false) {
String ptrdnameInstance;
ptrdnameInstance = ptrdnameValue.getTextValue();
ptrRecordInstance.setPtrdname(ptrdnameInstance);
}
}
}
JsonNode sRVRecordsArray = propertiesValue.get("SRVRecords");
if (sRVRecordsArray != null && sRVRecordsArray instanceof NullNode == false) {
propertiesInstance.setSrvRecords(new ArrayList());
for (JsonNode sRVRecordsValue : ((ArrayNode) sRVRecordsArray)) {
SrvRecord srvRecordInstance = new SrvRecord();
propertiesInstance.getSrvRecords().add(srvRecordInstance);
JsonNode priorityValue = sRVRecordsValue.get("priority");
if (priorityValue != null && priorityValue instanceof NullNode == false) {
int priorityInstance;
priorityInstance = priorityValue.getIntValue();
srvRecordInstance.setPriority(priorityInstance);
}
JsonNode weightValue = sRVRecordsValue.get("weight");
if (weightValue != null && weightValue instanceof NullNode == false) {
int weightInstance;
weightInstance = weightValue.getIntValue();
srvRecordInstance.setWeight(weightInstance);
}
JsonNode portValue = sRVRecordsValue.get("port");
if (portValue != null && portValue instanceof NullNode == false) {
int portInstance;
portInstance = portValue.getIntValue();
srvRecordInstance.setPort(portInstance);
}
JsonNode targetValue = sRVRecordsValue.get("target");
if (targetValue != null && targetValue instanceof NullNode == false) {
String targetInstance;
targetInstance = targetValue.getTextValue();
srvRecordInstance.setTarget(targetInstance);
}
}
}
JsonNode tXTRecordsArray = propertiesValue.get("TXTRecords");
if (tXTRecordsArray != null && tXTRecordsArray instanceof NullNode == false) {
propertiesInstance.setTxtRecords(new ArrayList());
for (JsonNode tXTRecordsValue : ((ArrayNode) tXTRecordsArray)) {
TxtRecord txtRecordInstance = new TxtRecord();
propertiesInstance.getTxtRecords().add(txtRecordInstance);
JsonNode valueValue2 = tXTRecordsValue.get("value");
if (valueValue2 != null && valueValue2 instanceof NullNode == false) {
String valueInstance;
valueInstance = valueValue2.getTextValue();
txtRecordInstance.setValue(valueInstance);
}
}
}
JsonNode cNAMERecordValue = propertiesValue.get("CNAMERecord");
if (cNAMERecordValue != null && cNAMERecordValue instanceof NullNode == false) {
CnameRecord cNAMERecordInstance = new CnameRecord();
propertiesInstance.setCnameRecord(cNAMERecordInstance);
JsonNode cnameValue = cNAMERecordValue.get("cname");
if (cnameValue != null && cnameValue instanceof NullNode == false) {
String cnameInstance;
cnameInstance = cnameValue.getTextValue();
cNAMERecordInstance.setCname(cnameInstance);
}
}
JsonNode sOARecordValue = propertiesValue.get("SOARecord");
if (sOARecordValue != null && sOARecordValue instanceof NullNode == false) {
SoaRecord sOARecordInstance = new SoaRecord();
propertiesInstance.setSoaRecord(sOARecordInstance);
JsonNode hostValue = sOARecordValue.get("host");
if (hostValue != null && hostValue instanceof NullNode == false) {
String hostInstance;
hostInstance = hostValue.getTextValue();
sOARecordInstance.setHost(hostInstance);
}
JsonNode emailValue = sOARecordValue.get("email");
if (emailValue != null && emailValue instanceof NullNode == false) {
String emailInstance;
emailInstance = emailValue.getTextValue();
sOARecordInstance.setEmail(emailInstance);
}
JsonNode serialNumberValue = sOARecordValue.get("serialNumber");
if (serialNumberValue != null && serialNumberValue instanceof NullNode == false) {
long serialNumberInstance;
serialNumberInstance = serialNumberValue.getLongValue();
sOARecordInstance.setSerialNumber(serialNumberInstance);
}
JsonNode refreshTimeValue = sOARecordValue.get("refreshTime");
if (refreshTimeValue != null && refreshTimeValue instanceof NullNode == false) {
long refreshTimeInstance;
refreshTimeInstance = refreshTimeValue.getLongValue();
sOARecordInstance.setRefreshTime(refreshTimeInstance);
}
JsonNode retryTimeValue = sOARecordValue.get("retryTime");
if (retryTimeValue != null && retryTimeValue instanceof NullNode == false) {
long retryTimeInstance;
retryTimeInstance = retryTimeValue.getLongValue();
sOARecordInstance.setRetryTime(retryTimeInstance);
}
JsonNode expireTimeValue = sOARecordValue.get("expireTime");
if (expireTimeValue != null && expireTimeValue instanceof NullNode == false) {
long expireTimeInstance;
expireTimeInstance = expireTimeValue.getLongValue();
sOARecordInstance.setExpireTime(expireTimeInstance);
}
JsonNode minimumTTLValue = sOARecordValue.get("minimumTTL");
if (minimumTTLValue != null && minimumTTLValue instanceof NullNode == false) {
long minimumTTLInstance;
minimumTTLInstance = minimumTTLValue.getLongValue();
sOARecordInstance.setMinimumTtl(minimumTTLInstance);
}
}
}
JsonNode idValue = valueValue.get("id");
if (idValue != null && idValue instanceof NullNode == false) {
String idInstance;
idInstance = idValue.getTextValue();
recordSetInstance.setId(idInstance);
}
JsonNode nameValue = valueValue.get("name");
if (nameValue != null && nameValue instanceof NullNode == false) {
String nameInstance;
nameInstance = nameValue.getTextValue();
recordSetInstance.setName(nameInstance);
}
JsonNode typeValue = valueValue.get("type");
if (typeValue != null && typeValue instanceof NullNode == false) {
String typeInstance;
typeInstance = typeValue.getTextValue();
recordSetInstance.setType(typeInstance);
}
JsonNode locationValue = valueValue.get("location");
if (locationValue != null && locationValue instanceof NullNode == false) {
String locationInstance;
locationInstance = locationValue.getTextValue();
recordSetInstance.setLocation(locationInstance);
}
JsonNode tagsSequenceElement = ((JsonNode) valueValue.get("tags"));
if (tagsSequenceElement != null && tagsSequenceElement instanceof NullNode == false) {
Iterator> itr = tagsSequenceElement.getFields();
while (itr.hasNext()) {
Map.Entry property = itr.next();
String tagsKey = property.getKey();
String tagsValue = property.getValue().getTextValue();
recordSetInstance.getTags().put(tagsKey, tagsValue);
}
}
}
}
JsonNode nextLinkValue = responseDoc.get("nextLink");
if (nextLinkValue != null && nextLinkValue instanceof NullNode == false) {
String nextLinkInstance;
nextLinkInstance = nextLinkValue.getTextValue();
result.setNextLink(nextLinkInstance);
}
}
result.setStatusCode(statusCode);
if (httpResponse.getHeaders("x-ms-request-id").length > 0) {
result.setRequestId(httpResponse.getFirstHeader("x-ms-request-id").getValue());
}
if (shouldTrace) {
CloudTracing.exit(invocationId, result);
}
return result;
} finally {
if (httpResponse != null && httpResponse.getEntity() != null) {
httpResponse.getEntity().getContent().close();
}
}
}
/**
* Lists RecordSets in a DNS zone. Depending on the previous call, it will
* list all types or by type.
*
* @param nextLink Required. NextLink from the previous successful call to
* List operation.
* @return The response to a RecordSet List operation.
*/
@Override
public Future listNextAsync(final String nextLink) {
return this.getClient().getExecutorService().submit(new Callable() {
@Override
public RecordSetListResponse call() throws Exception {
return listNext(nextLink);
}
});
}
/**
* Lists RecordSets in a DNS zone. Depending on the previous call, it will
* list all types or by type.
*
* @param nextLink Required. NextLink from the previous successful call to
* List operation.
* @throws IOException Signals that an I/O exception of some sort has
* occurred. This class is the general class of exceptions produced by
* failed or interrupted I/O operations.
* @throws ServiceException Thrown if an unexpected response is found.
* @return The response to a RecordSet List operation.
*/
@Override
public RecordSetListResponse listNext(String nextLink) throws IOException, ServiceException {
// Validate
if (nextLink == null) {
throw new NullPointerException("nextLink");
}
// Tracing
boolean shouldTrace = CloudTracing.getIsEnabled();
String invocationId = null;
if (shouldTrace) {
invocationId = Long.toString(CloudTracing.getNextInvocationId());
HashMap tracingParameters = new HashMap();
tracingParameters.put("nextLink", nextLink);
CloudTracing.enter(invocationId, this, "listNextAsync", tracingParameters);
}
// Construct URL
String url = "";
url = url + nextLink;
url = url.replace(" ", "%20");
// Create HTTP transport objects
HttpGet httpRequest = new HttpGet(url);
// Set Headers
httpRequest.setHeader("Content-Type", "application/json; charset=utf-8");
// Send Request
HttpResponse httpResponse = null;
try {
if (shouldTrace) {
CloudTracing.sendRequest(invocationId, httpRequest);
}
httpResponse = this.getClient().getHttpClient().execute(httpRequest);
if (shouldTrace) {
CloudTracing.receiveResponse(invocationId, httpResponse);
}
int statusCode = httpResponse.getStatusLine().getStatusCode();
if (statusCode >= HttpStatus.SC_BAD_REQUEST) {
ServiceException ex = ServiceException.createFromJson(httpRequest, null, httpResponse, httpResponse.getEntity());
if (shouldTrace) {
CloudTracing.error(invocationId, ex);
}
throw ex;
}
// Create Result
RecordSetListResponse result = null;
// Deserialize Response
InputStream responseContent = httpResponse.getEntity().getContent();
result = new RecordSetListResponse();
ObjectMapper objectMapper = new ObjectMapper();
JsonNode responseDoc = null;
String responseDocContent = IOUtils.toString(responseContent);
if (responseDocContent == null == false && responseDocContent.length() > 0) {
responseDoc = objectMapper.readTree(responseDocContent);
}
if (responseDoc != null && responseDoc instanceof NullNode == false) {
JsonNode valueArray = responseDoc.get("value");
if (valueArray != null && valueArray instanceof NullNode == false) {
for (JsonNode valueValue : ((ArrayNode) valueArray)) {
RecordSet recordSetInstance = new RecordSet();
result.getRecordSets().add(recordSetInstance);
JsonNode etagValue = valueValue.get("etag");
if (etagValue != null && etagValue instanceof NullNode == false) {
String etagInstance;
etagInstance = etagValue.getTextValue();
recordSetInstance.setETag(etagInstance);
}
JsonNode propertiesValue = valueValue.get("properties");
if (propertiesValue != null && propertiesValue instanceof NullNode == false) {
RecordSetProperties propertiesInstance = new RecordSetProperties();
recordSetInstance.setProperties(propertiesInstance);
JsonNode tTLValue = propertiesValue.get("TTL");
if (tTLValue != null && tTLValue instanceof NullNode == false) {
long tTLInstance;
tTLInstance = tTLValue.getLongValue();
propertiesInstance.setTtl(tTLInstance);
}
JsonNode aRecordsArray = propertiesValue.get("ARecords");
if (aRecordsArray != null && aRecordsArray instanceof NullNode == false) {
propertiesInstance.setARecords(new ArrayList());
for (JsonNode aRecordsValue : ((ArrayNode) aRecordsArray)) {
ARecord aRecordInstance = new ARecord();
propertiesInstance.getARecords().add(aRecordInstance);
JsonNode ipv4AddressValue = aRecordsValue.get("ipv4Address");
if (ipv4AddressValue != null && ipv4AddressValue instanceof NullNode == false) {
String ipv4AddressInstance;
ipv4AddressInstance = ipv4AddressValue.getTextValue();
aRecordInstance.setIpv4Address(ipv4AddressInstance);
}
}
}
JsonNode aAAARecordsArray = propertiesValue.get("AAAARecords");
if (aAAARecordsArray != null && aAAARecordsArray instanceof NullNode == false) {
propertiesInstance.setAaaaRecords(new ArrayList());
for (JsonNode aAAARecordsValue : ((ArrayNode) aAAARecordsArray)) {
AaaaRecord aaaaRecordInstance = new AaaaRecord();
propertiesInstance.getAaaaRecords().add(aaaaRecordInstance);
JsonNode ipv6AddressValue = aAAARecordsValue.get("ipv6Address");
if (ipv6AddressValue != null && ipv6AddressValue instanceof NullNode == false) {
String ipv6AddressInstance;
ipv6AddressInstance = ipv6AddressValue.getTextValue();
aaaaRecordInstance.setIpv6Address(ipv6AddressInstance);
}
}
}
JsonNode mXRecordsArray = propertiesValue.get("MXRecords");
if (mXRecordsArray != null && mXRecordsArray instanceof NullNode == false) {
propertiesInstance.setMxRecords(new ArrayList());
for (JsonNode mXRecordsValue : ((ArrayNode) mXRecordsArray)) {
MxRecord mxRecordInstance = new MxRecord();
propertiesInstance.getMxRecords().add(mxRecordInstance);
JsonNode preferenceValue = mXRecordsValue.get("preference");
if (preferenceValue != null && preferenceValue instanceof NullNode == false) {
int preferenceInstance;
preferenceInstance = preferenceValue.getIntValue();
mxRecordInstance.setPreference(preferenceInstance);
}
JsonNode exchangeValue = mXRecordsValue.get("exchange");
if (exchangeValue != null && exchangeValue instanceof NullNode == false) {
String exchangeInstance;
exchangeInstance = exchangeValue.getTextValue();
mxRecordInstance.setExchange(exchangeInstance);
}
}
}
JsonNode nSRecordsArray = propertiesValue.get("NSRecords");
if (nSRecordsArray != null && nSRecordsArray instanceof NullNode == false) {
propertiesInstance.setNsRecords(new ArrayList());
for (JsonNode nSRecordsValue : ((ArrayNode) nSRecordsArray)) {
NsRecord nsRecordInstance = new NsRecord();
propertiesInstance.getNsRecords().add(nsRecordInstance);
JsonNode nsdnameValue = nSRecordsValue.get("nsdname");
if (nsdnameValue != null && nsdnameValue instanceof NullNode == false) {
String nsdnameInstance;
nsdnameInstance = nsdnameValue.getTextValue();
nsRecordInstance.setNsdname(nsdnameInstance);
}
}
}
JsonNode pTRRecordsArray = propertiesValue.get("PTRRecords");
if (pTRRecordsArray != null && pTRRecordsArray instanceof NullNode == false) {
propertiesInstance.setPtrRecords(new ArrayList());
for (JsonNode pTRRecordsValue : ((ArrayNode) pTRRecordsArray)) {
PtrRecord ptrRecordInstance = new PtrRecord();
propertiesInstance.getPtrRecords().add(ptrRecordInstance);
JsonNode ptrdnameValue = pTRRecordsValue.get("ptrdname");
if (ptrdnameValue != null && ptrdnameValue instanceof NullNode == false) {
String ptrdnameInstance;
ptrdnameInstance = ptrdnameValue.getTextValue();
ptrRecordInstance.setPtrdname(ptrdnameInstance);
}
}
}
JsonNode sRVRecordsArray = propertiesValue.get("SRVRecords");
if (sRVRecordsArray != null && sRVRecordsArray instanceof NullNode == false) {
propertiesInstance.setSrvRecords(new ArrayList());
for (JsonNode sRVRecordsValue : ((ArrayNode) sRVRecordsArray)) {
SrvRecord srvRecordInstance = new SrvRecord();
propertiesInstance.getSrvRecords().add(srvRecordInstance);
JsonNode priorityValue = sRVRecordsValue.get("priority");
if (priorityValue != null && priorityValue instanceof NullNode == false) {
int priorityInstance;
priorityInstance = priorityValue.getIntValue();
srvRecordInstance.setPriority(priorityInstance);
}
JsonNode weightValue = sRVRecordsValue.get("weight");
if (weightValue != null && weightValue instanceof NullNode == false) {
int weightInstance;
weightInstance = weightValue.getIntValue();
srvRecordInstance.setWeight(weightInstance);
}
JsonNode portValue = sRVRecordsValue.get("port");
if (portValue != null && portValue instanceof NullNode == false) {
int portInstance;
portInstance = portValue.getIntValue();
srvRecordInstance.setPort(portInstance);
}
JsonNode targetValue = sRVRecordsValue.get("target");
if (targetValue != null && targetValue instanceof NullNode == false) {
String targetInstance;
targetInstance = targetValue.getTextValue();
srvRecordInstance.setTarget(targetInstance);
}
}
}
JsonNode tXTRecordsArray = propertiesValue.get("TXTRecords");
if (tXTRecordsArray != null && tXTRecordsArray instanceof NullNode == false) {
propertiesInstance.setTxtRecords(new ArrayList());
for (JsonNode tXTRecordsValue : ((ArrayNode) tXTRecordsArray)) {
TxtRecord txtRecordInstance = new TxtRecord();
propertiesInstance.getTxtRecords().add(txtRecordInstance);
JsonNode valueValue2 = tXTRecordsValue.get("value");
if (valueValue2 != null && valueValue2 instanceof NullNode == false) {
String valueInstance;
valueInstance = valueValue2.getTextValue();
txtRecordInstance.setValue(valueInstance);
}
}
}
JsonNode cNAMERecordValue = propertiesValue.get("CNAMERecord");
if (cNAMERecordValue != null && cNAMERecordValue instanceof NullNode == false) {
CnameRecord cNAMERecordInstance = new CnameRecord();
propertiesInstance.setCnameRecord(cNAMERecordInstance);
JsonNode cnameValue = cNAMERecordValue.get("cname");
if (cnameValue != null && cnameValue instanceof NullNode == false) {
String cnameInstance;
cnameInstance = cnameValue.getTextValue();
cNAMERecordInstance.setCname(cnameInstance);
}
}
JsonNode sOARecordValue = propertiesValue.get("SOARecord");
if (sOARecordValue != null && sOARecordValue instanceof NullNode == false) {
SoaRecord sOARecordInstance = new SoaRecord();
propertiesInstance.setSoaRecord(sOARecordInstance);
JsonNode hostValue = sOARecordValue.get("host");
if (hostValue != null && hostValue instanceof NullNode == false) {
String hostInstance;
hostInstance = hostValue.getTextValue();
sOARecordInstance.setHost(hostInstance);
}
JsonNode emailValue = sOARecordValue.get("email");
if (emailValue != null && emailValue instanceof NullNode == false) {
String emailInstance;
emailInstance = emailValue.getTextValue();
sOARecordInstance.setEmail(emailInstance);
}
JsonNode serialNumberValue = sOARecordValue.get("serialNumber");
if (serialNumberValue != null && serialNumberValue instanceof NullNode == false) {
long serialNumberInstance;
serialNumberInstance = serialNumberValue.getLongValue();
sOARecordInstance.setSerialNumber(serialNumberInstance);
}
JsonNode refreshTimeValue = sOARecordValue.get("refreshTime");
if (refreshTimeValue != null && refreshTimeValue instanceof NullNode == false) {
long refreshTimeInstance;
refreshTimeInstance = refreshTimeValue.getLongValue();
sOARecordInstance.setRefreshTime(refreshTimeInstance);
}
JsonNode retryTimeValue = sOARecordValue.get("retryTime");
if (retryTimeValue != null && retryTimeValue instanceof NullNode == false) {
long retryTimeInstance;
retryTimeInstance = retryTimeValue.getLongValue();
sOARecordInstance.setRetryTime(retryTimeInstance);
}
JsonNode expireTimeValue = sOARecordValue.get("expireTime");
if (expireTimeValue != null && expireTimeValue instanceof NullNode == false) {
long expireTimeInstance;
expireTimeInstance = expireTimeValue.getLongValue();
sOARecordInstance.setExpireTime(expireTimeInstance);
}
JsonNode minimumTTLValue = sOARecordValue.get("minimumTTL");
if (minimumTTLValue != null && minimumTTLValue instanceof NullNode == false) {
long minimumTTLInstance;
minimumTTLInstance = minimumTTLValue.getLongValue();
sOARecordInstance.setMinimumTtl(minimumTTLInstance);
}
}
}
JsonNode idValue = valueValue.get("id");
if (idValue != null && idValue instanceof NullNode == false) {
String idInstance;
idInstance = idValue.getTextValue();
recordSetInstance.setId(idInstance);
}
JsonNode nameValue = valueValue.get("name");
if (nameValue != null && nameValue instanceof NullNode == false) {
String nameInstance;
nameInstance = nameValue.getTextValue();
recordSetInstance.setName(nameInstance);
}
JsonNode typeValue = valueValue.get("type");
if (typeValue != null && typeValue instanceof NullNode == false) {
String typeInstance;
typeInstance = typeValue.getTextValue();
recordSetInstance.setType(typeInstance);
}
JsonNode locationValue = valueValue.get("location");
if (locationValue != null && locationValue instanceof NullNode == false) {
String locationInstance;
locationInstance = locationValue.getTextValue();
recordSetInstance.setLocation(locationInstance);
}
JsonNode tagsSequenceElement = ((JsonNode) valueValue.get("tags"));
if (tagsSequenceElement != null && tagsSequenceElement instanceof NullNode == false) {
Iterator> itr = tagsSequenceElement.getFields();
while (itr.hasNext()) {
Map.Entry property = itr.next();
String tagsKey = property.getKey();
String tagsValue = property.getValue().getTextValue();
recordSetInstance.getTags().put(tagsKey, tagsValue);
}
}
}
}
JsonNode nextLinkValue = responseDoc.get("nextLink");
if (nextLinkValue != null && nextLinkValue instanceof NullNode == false) {
String nextLinkInstance;
nextLinkInstance = nextLinkValue.getTextValue();
result.setNextLink(nextLinkInstance);
}
}
result.setStatusCode(statusCode);
if (httpResponse.getHeaders("x-ms-request-id").length > 0) {
result.setRequestId(httpResponse.getFirstHeader("x-ms-request-id").getValue());
}
if (shouldTrace) {
CloudTracing.exit(invocationId, result);
}
return result;
} finally {
if (httpResponse != null && httpResponse.getEntity() != null) {
httpResponse.getEntity().getContent().close();
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy