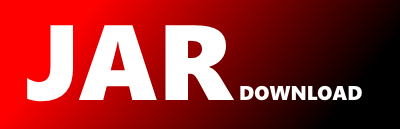
com.microsoft.azure.management.dns.DnsRecordSet Maven / Gradle / Ivy
Show all versions of azure-mgmt-dns Show documentation
/**
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for
* license information.
*/
package com.microsoft.azure.management.dns;
import com.microsoft.azure.management.apigeneration.Beta;
import com.microsoft.azure.management.apigeneration.Fluent;
import com.microsoft.azure.management.dns.implementation.RecordSetInner;
import com.microsoft.azure.management.resources.fluentcore.arm.models.ExternalChildResource;
import com.microsoft.azure.management.resources.fluentcore.model.Attachable;
import com.microsoft.azure.management.resources.fluentcore.model.Settable;
import com.microsoft.azure.management.resources.fluentcore.model.HasInner;
import java.util.List;
import java.util.Map;
/**
* An immutable client-side representation of a record set in Azure DNS Zone.
*/
@Fluent
public interface DnsRecordSet extends
ExternalChildResource,
HasInner {
/**
* @return the type of records in this record set
*/
RecordType recordType();
/**
* @return TTL of the records in this record set
*/
long timeToLive();
/**
* @return the metadata associated with this record set.
*/
Map metadata();
/**
* @return the fully qualified domain name of the record set.
*/
@Beta(Beta.SinceVersion.V1_9_0)
String fqdn();
/**
* @return the etag associated with the record set.
*/
String eTag();
/**
* The entirety of a DNS zone record set definition as a part of parent definition.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface Definition extends
DefinitionStages.ARecordSetBlank,
DefinitionStages.WithARecordIPv4Address,
DefinitionStages.WithARecordIPv4AddressOrAttachable,
DefinitionStages.AaaaRecordSetBlank,
DefinitionStages.WithAaaaRecordIPv6Address,
DefinitionStages.WithAaaaRecordIPv6AddressOrAttachable,
DefinitionStages.CaaRecordSetBlank,
DefinitionStages.WithCaaRecordEntry,
DefinitionStages.WithCaaRecordEntryOrAttachable,
DefinitionStages.CNameRecordSetBlank,
DefinitionStages.WithCNameRecordAlias,
DefinitionStages.WithCNameRecordSetAttachable,
DefinitionStages.MXRecordSetBlank,
DefinitionStages.WithMXRecordMailExchange,
DefinitionStages.WithMXRecordMailExchangeOrAttachable,
DefinitionStages.NSRecordSetBlank,
DefinitionStages.WithNSRecordNameServer,
DefinitionStages.WithNSRecordNameServerOrAttachable,
DefinitionStages.PtrRecordSetBlank,
DefinitionStages.WithPtrRecordTargetDomainName,
DefinitionStages.WithPtrRecordTargetDomainNameOrAttachable,
DefinitionStages.SrvRecordSetBlank,
DefinitionStages.WithSrvRecordEntry,
DefinitionStages.WithSrvRecordEntryOrAttachable,
DefinitionStages.TxtRecordSetBlank,
DefinitionStages.WithTxtRecordTextValue,
DefinitionStages.WithTxtRecordTextValueOrAttachable,
DefinitionStages.WithAttach {
}
/**
* Grouping of DNS zone record set definition stages as a part of parent DNS zone definition.
*/
interface DefinitionStages {
/**
* The first stage of an A record definition.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface ARecordSetBlank extends WithARecordIPv4Address {
}
/**
* The stage of the A record set definition allowing to add first A record.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithARecordIPv4Address {
/**
* Creates an A record with the provided IPv4 address in this record set.
*
* @param ipv4Address the IPv4 address
* @return the next stage of the definition
*/
WithARecordIPv4AddressOrAttachable withIPv4Address(String ipv4Address);
}
/**
* The stage of the A record set definition allowing to add additional A records or
* attach the record set to the parent.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithARecordIPv4AddressOrAttachable
extends WithARecordIPv4Address, WithAttach {
}
/**
* The first stage of a AAAA record definition.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface AaaaRecordSetBlank extends WithAaaaRecordIPv6Address {
}
/**
* The stage of the AAAA record set definition allowing to add first AAAA record.
*
* @param the return type of {@link WithAttach#attach()}
*/
interface WithAaaaRecordIPv6Address {
/**
* Creates an AAAA record with the provided IPv6 address in this record set.
*
* @param ipv6Address an IPv6 address
* @return the next stage of the definition
*/
WithAaaaRecordIPv6AddressOrAttachable withIPv6Address(String ipv6Address);
}
/**
* The stage of the AAAA record set definition allowing to add additional AAAA records or
* attach the record set to the parent.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithAaaaRecordIPv6AddressOrAttachable
extends WithAaaaRecordIPv6Address, WithAttach {
}
/**
* The first stage of a Caa record definition.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
@Beta(Beta.SinceVersion.V1_9_0)
interface CaaRecordSetBlank extends WithCaaRecordEntry {
}
/**
* The stage of the Caa record definition allowing to add first service record.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
@Beta(Beta.SinceVersion.V1_9_0)
interface WithCaaRecordEntry {
/**
* Specifies a Caa record for a service.
*
* @param flags the flags for this CAA record as an integer between 0 and 255
* @param tag the tag for this CAA record
* @param value the value for this CAA record
* @return the next stage of the definition
*/
@Beta(Beta.SinceVersion.V1_9_0)
WithCaaRecordEntryOrAttachable withRecord(int flags, String tag, String value);
}
/**
* The stage of the Caa record set definition allowing to add additional Caa records or attach the record set
* to the parent.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
@Beta(Beta.SinceVersion.V1_9_0)
interface WithCaaRecordEntryOrAttachable
extends WithCaaRecordEntry, WithAttach {
}
/**
* The first stage of a CNAME record set definition.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface CNameRecordSetBlank extends WithCNameRecordAlias {
}
/**
* The stage of a CNAME record definition allowing to add alias.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithCNameRecordAlias {
/**
* Creates a CNAME record with the provided alias.
*
* @param alias the alias
* @return the next stage of the definition
*/
WithCNameRecordSetAttachable withAlias(String alias);
}
/**
* The stage of the CNAME record set definition allowing attach the record set to the parent.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithCNameRecordSetAttachable extends WithAttach {
}
/**
* The first stage of a MX record definition.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface MXRecordSetBlank extends WithMXRecordMailExchange {
}
/**
* The stage of the MX record set definition allowing to add first MX record.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithMXRecordMailExchange {
/**
* Creates and assigns priority to a MX record with the provided mail exchange server in this record set.
*
* @param mailExchangeHostName the host name of the mail exchange server
* @param priority the priority for the mail exchange host, lower the value higher the priority
* @return the next stage of the definition
*/
WithMXRecordMailExchangeOrAttachable withMailExchange(String mailExchangeHostName, int priority);
}
/**
* The stage of the MX record set definition allowing to add additional MX records or attach the record set
* to the parent.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithMXRecordMailExchangeOrAttachable
extends WithMXRecordMailExchange, WithAttach {
}
/**
* The first stage of a NS record definition.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface NSRecordSetBlank extends WithNSRecordNameServer {
}
/**
* The stage of the NS record set definition allowing to add a NS record.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithNSRecordNameServer {
/**
* Creates a NS record with the provided name server in this record set.
*
* @param nameServerHostName the name server host name
* @return the next stage of the definition
*/
WithNSRecordNameServerOrAttachable withNameServer(String nameServerHostName);
}
/**
* The stage of the NS record set definition allowing to add additional NS records or
* attach the record set to the parent.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithNSRecordNameServerOrAttachable
extends WithNSRecordNameServer, WithAttach {
}
/**
* The first stage of a PTR record definition.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface PtrRecordSetBlank extends WithPtrRecordTargetDomainName {
}
/**
* The stage of the PTR record set definition allowing to add first CNAME record.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithPtrRecordTargetDomainName {
/**
* Creates a PTR record with the provided target domain name in this record set.
*
* @param targetDomainName the target domain name
* @return the next stage of the definition
*/
WithPtrRecordTargetDomainNameOrAttachable withTargetDomainName(String targetDomainName);
}
/**
* The stage of the PTR record set definition allowing to add additional PTR records or
* attach the record set to the parent.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithPtrRecordTargetDomainNameOrAttachable
extends WithPtrRecordTargetDomainName, WithAttach {
}
/**
* The first stage of a SRV record definition.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface SrvRecordSetBlank extends WithSrvRecordEntry {
}
/**
* The stage of the SRV record definition allowing to add first service record.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithSrvRecordEntry {
/**
* Specifies a service record for a service.
*
* @param target the canonical name of the target host running the service
* @param port the port on which the service is bounded
* @param priority the priority of the target host, lower the value higher the priority
* @param weight the relative weight (preference) of the records with the same priority, higher the value more the preference
* @return the next stage of the definition
*/
WithSrvRecordEntryOrAttachable withRecord(String target, int port, int priority, int weight);
}
/**
* The stage of the SRV record set definition allowing to add additional SRV records or attach the record set
* to the parent.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithSrvRecordEntryOrAttachable
extends WithSrvRecordEntry, WithAttach {
}
/**
* The first stage of a TXT record definition.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface TxtRecordSetBlank extends WithTxtRecordTextValue {
}
/**
* The stage of the TXT record definition allowing to add first TXT record.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithTxtRecordTextValue {
/**
* Creates a Txt record with the given text in this record set.
*
* @param text the text value
* @return the next stage of the definition
*/
WithTxtRecordTextValueOrAttachable withText(String text);
}
/**
* The stage of the TXT record set definition allowing to add additional TXT records or attach the record set
* to the parent.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithTxtRecordTextValueOrAttachable
extends WithTxtRecordTextValue, WithAttach {
}
/**
* The stage of the record set definition allowing to specify the Time To Live (TTL) for the records in this record set.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithTtl {
/**
* Specifies the Time To Live for the records in the record set.
*
* @param ttlInSeconds TTL in seconds
* @return the next stage of the definition
*/
WithAttach withTimeToLive(long ttlInSeconds);
}
/**
* The stage of the record set definition allowing to specify metadata.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithMetadata {
/**
* Adds a metadata to the resource.
*
* @param key the key for the metadata
* @param value the value for the metadata
* @return the next stage of the definition
*/
WithAttach withMetadata(String key, String value);
}
/**
* The stage of the record set definition allowing to enable ETag validation.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithETagCheck {
/**
* Specifies that If-None-Match header needs to set to * to prevent updating an existing record set.
*
* @return the next stage of the definition
*/
WithAttach withETagCheck();
}
/** The final stage of the DNS zone record set definition.
*
* At this stage, any remaining optional settings can be specified, or the DNS zone record set
* definition can be attached to the parent traffic manager profile definition using {@link DnsRecordSet.DefinitionStages.WithAttach#attach()}.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithAttach extends
Attachable.InDefinition,
DefinitionStages.WithTtl,
DefinitionStages.WithMetadata,
DefinitionStages.WithETagCheck {
}
}
/**
* The entirety of a DNS zone record set definition as a part of parent update.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface UpdateDefinition extends
UpdateDefinitionStages.ARecordSetBlank,
UpdateDefinitionStages.WithARecordIPv4Address,
UpdateDefinitionStages.WithARecordIPv4AddressOrAttachable,
UpdateDefinitionStages.AaaaRecordSetBlank,
UpdateDefinitionStages.WithAaaaRecordIPv6Address,
UpdateDefinitionStages.WithAaaaRecordIPv6AddressOrAttachable,
UpdateDefinitionStages.CaaRecordSetBlank,
UpdateDefinitionStages.WithCaaRecordEntry,
UpdateDefinitionStages.WithCaaRecordEntryOrAttachable,
UpdateDefinitionStages.CNameRecordSetBlank,
UpdateDefinitionStages.WithCNameRecordAlias,
UpdateDefinitionStages.WithCNameRecordSetAttachable,
UpdateDefinitionStages.MXRecordSetBlank,
UpdateDefinitionStages.WithMXRecordMailExchange,
UpdateDefinitionStages.WithMXRecordMailExchangeOrAttachable,
UpdateDefinitionStages.NSRecordSetBlank,
UpdateDefinitionStages.WithNSRecordNameServer,
UpdateDefinitionStages.WithNSRecordNameServerOrAttachable,
UpdateDefinitionStages.PtrRecordSetBlank,
UpdateDefinitionStages.WithPtrRecordTargetDomainName,
UpdateDefinitionStages.WithPtrRecordTargetDomainNameOrAttachable,
UpdateDefinitionStages.SrvRecordSetBlank,
UpdateDefinitionStages.WithSrvRecordEntry,
UpdateDefinitionStages.WithSrvRecordEntryOrAttachable,
UpdateDefinitionStages.TxtRecordSetBlank,
UpdateDefinitionStages.WithTxtRecordTextValue,
UpdateDefinitionStages.WithTxtRecordTextValueOrAttachable,
UpdateDefinitionStages.WithAttach {
}
/**
* Grouping of DNS zone record set definition stages as a part of parent DNS zone update.
*/
interface UpdateDefinitionStages {
/**
* The first stage of a A record definition.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface ARecordSetBlank extends WithARecordIPv4Address {
}
/**
* The stage of the A record set definition allowing to add first A record.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithARecordIPv4Address {
/**
* Creates an A record with the provided IPv4 address in this record set.
*
* @param ipv4Address the IPv4 address
* @return the next stage of the definition
*/
WithARecordIPv4AddressOrAttachable withIPv4Address(String ipv4Address);
}
/**
* The stage of the A record set definition allowing to add additional A records or
* attach the record set to the parent.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithARecordIPv4AddressOrAttachable
extends WithARecordIPv4Address, WithAttach {
}
/**
* The first stage of a AAAA record definition.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface AaaaRecordSetBlank extends WithAaaaRecordIPv6Address {
}
/**
* The stage of the AAAA record set definition allowing to add first AAAA record.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithAaaaRecordIPv6Address {
/**
* Creates an AAAA record with the provided IPv6 address in this record set.
*
* @param ipv6Address the IPv6 address
* @return the next stage of the definition
*/
WithAaaaRecordIPv6AddressOrAttachable withIPv6Address(String ipv6Address);
}
/**
* The stage of the AAAA record set definition allowing to add additional A records or
* attach the record set to the parent.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithAaaaRecordIPv6AddressOrAttachable
extends WithAaaaRecordIPv6Address, WithAttach {
}
/**
* The first stage of a Caa record definition.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
@Beta(Beta.SinceVersion.V1_9_0)
interface CaaRecordSetBlank extends WithCaaRecordEntry {
}
/**
* The stage of the Caa record definition allowing to add first service record.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
@Beta(Beta.SinceVersion.V1_9_0)
interface WithCaaRecordEntry {
/**
* Specifies a Caa record for a service.
*
* @param flags the flags for this CAA record as an integer between 0 and 255
* @param tag the tag for this CAA record
* @param value the value for this CAA record
* @return the next stage of the definition
*/
@Beta(Beta.SinceVersion.V1_9_0)
WithCaaRecordEntryOrAttachable withRecord(int flags, String tag, String value);
}
/**
* The stage of the Caa record set definition allowing to add additional Caa records or attach the record set
* to the parent.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
@Beta(Beta.SinceVersion.V1_9_0)
interface WithCaaRecordEntryOrAttachable
extends WithCaaRecordEntry, WithAttach {
}
/**
* The first stage of a CNAME record set definition.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface CNameRecordSetBlank extends WithCNameRecordAlias {
}
/**
* The stage of a CNAME record definition allowing to add alias.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithCNameRecordAlias {
/**
* Creates a CNAME record with the provided alias.
*
* @param alias the alias
* @return the next stage of the definition
*/
WithCNameRecordSetAttachable withAlias(String alias);
}
/**
* The stage of the CNAME record set definition allowing attach the record set to the parent.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithCNameRecordSetAttachable extends WithAttach {
}
/**
* The first stage of an MX record definition.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface MXRecordSetBlank extends WithMXRecordMailExchange {
}
/**
* The stage of the MX record set definition allowing to add first MX record.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithMXRecordMailExchange {
/**
* Creates and assigns priority to a MX record with the provided mail exchange server in this record set.
*
* @param mailExchangeHostName the host name of the mail exchange server
* @param priority the priority for the mail exchange host, lower the value higher the priority
* @return the next stage of the definition
*/
WithMXRecordMailExchangeOrAttachable withMailExchange(String mailExchangeHostName, int priority);
}
/**
* The stage of the MX record set definition allowing to add additional MX records or attach the record set
* to the parent.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithMXRecordMailExchangeOrAttachable
extends WithMXRecordMailExchange, WithAttach {
}
/**
* The first stage of a NS record definition.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface NSRecordSetBlank extends WithNSRecordNameServer {
}
/**
* The stage of the NS record set definition allowing to add a NS record.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithNSRecordNameServer {
/**
* Creates a NS record with the provided name server in this record set.
*
* @param nameServerHostName the name server host name
* @return the next stage of the definition
*/
WithNSRecordNameServerOrAttachable withNameServer(String nameServerHostName);
}
/**
* The stage of the NS record set definition allowing to add additional NS records or
* attach the record set to the parent.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithNSRecordNameServerOrAttachable
extends WithNSRecordNameServer, WithAttach {
}
/**
* The first stage of a PTR record definition.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface PtrRecordSetBlank extends WithPtrRecordTargetDomainName {
}
/**
* The stage of the PTR record set definition allowing to add first CNAME record.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithPtrRecordTargetDomainName {
/**
* Creates a PTR record with the provided target domain name in this record set.
*
* @param targetDomainName the target domain name
* @return the next stage of the definition
*/
WithPtrRecordTargetDomainNameOrAttachable withTargetDomainName(String targetDomainName);
}
/**
* The stage of the PTR record set definition allowing to add additional PTR records or
* attach the record set to the parent.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithPtrRecordTargetDomainNameOrAttachable
extends WithPtrRecordTargetDomainName, WithAttach {
}
/**
* The first stage of a SRV record definition.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface SrvRecordSetBlank extends WithSrvRecordEntry {
}
/**
* The stage of the SRV record definition allowing to add first service record.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithSrvRecordEntry {
/**
* Specifies a service record for a service.
*
* @param target the canonical name of the target host running the service
* @param port the port on which the service is bounded
* @param priority the priority of the target host, lower the value higher the priority
* @param weight the relative weight (preference) of the records with the same priority, higher the value more the preference
* @return the next stage of the definition
*/
WithSrvRecordEntryOrAttachable withRecord(String target, int port, int priority, int weight);
}
/**
* The stage of the SRV record set definition allowing to add additional SRV records or attach the record set
* to the parent.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithSrvRecordEntryOrAttachable
extends WithSrvRecordEntry, WithAttach {
}
/**
* The first stage of a TXT record definition.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface TxtRecordSetBlank extends WithTxtRecordTextValue {
}
/**
* The stage of the TXT record definition allowing to add first Txt record.
*
* @param