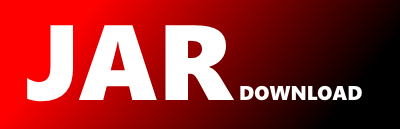
com.microsoft.azure.management.keyvault.implementation.VaultsInner Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-mgmt-keyvault Show documentation
Show all versions of azure-mgmt-keyvault Show documentation
This package contains Microsoft Azure Key Vault Management SDK. This package is in low maintenance mode and being phased out. To use the latest Azure SDK for resource management, please see https://aka.ms/azsdk/java/mgmt
/**
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for
* license information.
*
* Code generated by Microsoft (R) AutoRest Code Generator.
*/
package com.microsoft.azure.management.keyvault.implementation;
import com.microsoft.azure.management.resources.fluentcore.collection.InnerSupportsGet;
import com.microsoft.azure.management.resources.fluentcore.collection.InnerSupportsDelete;
import retrofit2.Retrofit;
import com.google.common.reflect.TypeToken;
import com.microsoft.azure.AzureServiceFuture;
import com.microsoft.azure.CloudException;
import com.microsoft.azure.ListOperationCallback;
import com.microsoft.azure.management.keyvault.AccessPolicyUpdateKind;
import com.microsoft.azure.management.keyvault.VaultAccessPolicyProperties;
import com.microsoft.azure.management.keyvault.VaultCheckNameAvailabilityParameters;
import com.microsoft.azure.management.keyvault.VaultCreateOrUpdateParameters;
import com.microsoft.azure.management.keyvault.VaultPatchParameters;
import com.microsoft.azure.Page;
import com.microsoft.azure.PagedList;
import com.microsoft.azure.Resource;
import com.microsoft.rest.ServiceCallback;
import com.microsoft.rest.ServiceFuture;
import com.microsoft.rest.ServiceResponse;
import com.microsoft.rest.Validator;
import java.io.IOException;
import java.util.List;
import okhttp3.ResponseBody;
import retrofit2.http.Body;
import retrofit2.http.GET;
import retrofit2.http.Header;
import retrofit2.http.Headers;
import retrofit2.http.HTTP;
import retrofit2.http.PATCH;
import retrofit2.http.Path;
import retrofit2.http.POST;
import retrofit2.http.PUT;
import retrofit2.http.Query;
import retrofit2.http.Url;
import retrofit2.Response;
import rx.functions.Func1;
import rx.Observable;
/**
* An instance of this class provides access to all the operations defined
* in Vaults.
*/
public class VaultsInner implements InnerSupportsGet, InnerSupportsDelete {
/** The Retrofit service to perform REST calls. */
private VaultsService service;
/** The service client containing this operation class. */
private KeyVaultManagementClientImpl client;
/**
* Initializes an instance of VaultsInner.
*
* @param retrofit the Retrofit instance built from a Retrofit Builder.
* @param client the instance of the service client containing this operation class.
*/
public VaultsInner(Retrofit retrofit, KeyVaultManagementClientImpl client) {
this.service = retrofit.create(VaultsService.class);
this.client = client;
}
/**
* The interface defining all the services for Vaults to be
* used by Retrofit to perform actually REST calls.
*/
interface VaultsService {
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.keyvault.Vaults createOrUpdate" })
@PUT("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.KeyVault/vaults/{vaultName}")
Observable> createOrUpdate(@Path("resourceGroupName") String resourceGroupName, @Path("vaultName") String vaultName, @Path("subscriptionId") String subscriptionId, @Query("api-version") String apiVersion, @Body VaultCreateOrUpdateParameters parameters, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.keyvault.Vaults beginCreateOrUpdate" })
@PUT("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.KeyVault/vaults/{vaultName}")
Observable> beginCreateOrUpdate(@Path("resourceGroupName") String resourceGroupName, @Path("vaultName") String vaultName, @Path("subscriptionId") String subscriptionId, @Query("api-version") String apiVersion, @Body VaultCreateOrUpdateParameters parameters, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.keyvault.Vaults update" })
@PATCH("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.KeyVault/vaults/{vaultName}")
Observable> update(@Path("resourceGroupName") String resourceGroupName, @Path("vaultName") String vaultName, @Path("subscriptionId") String subscriptionId, @Query("api-version") String apiVersion, @Body VaultPatchParameters parameters, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.keyvault.Vaults delete" })
@HTTP(path = "subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.KeyVault/vaults/{vaultName}", method = "DELETE", hasBody = true)
Observable> delete(@Path("resourceGroupName") String resourceGroupName, @Path("vaultName") String vaultName, @Path("subscriptionId") String subscriptionId, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.keyvault.Vaults getByResourceGroup" })
@GET("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.KeyVault/vaults/{vaultName}")
Observable> getByResourceGroup(@Path("resourceGroupName") String resourceGroupName, @Path("vaultName") String vaultName, @Path("subscriptionId") String subscriptionId, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.keyvault.Vaults updateAccessPolicy" })
@PUT("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.KeyVault/vaults/{vaultName}/accessPolicies/{operationKind}")
Observable> updateAccessPolicy(@Path("resourceGroupName") String resourceGroupName, @Path("vaultName") String vaultName, @Path("operationKind") AccessPolicyUpdateKind operationKind, @Path("subscriptionId") String subscriptionId, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Body VaultAccessPolicyParametersInner parameters, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.keyvault.Vaults listByResourceGroup" })
@GET("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.KeyVault/vaults")
Observable> listByResourceGroup(@Path("resourceGroupName") String resourceGroupName, @Path("subscriptionId") String subscriptionId, @Query("$top") Integer top, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.keyvault.Vaults listBySubscription" })
@GET("subscriptions/{subscriptionId}/providers/Microsoft.KeyVault/vaults")
Observable> listBySubscription(@Path("subscriptionId") String subscriptionId, @Query("$top") Integer top, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.keyvault.Vaults listDeleted" })
@GET("subscriptions/{subscriptionId}/providers/Microsoft.KeyVault/deletedVaults")
Observable> listDeleted(@Path("subscriptionId") String subscriptionId, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.keyvault.Vaults getDeleted" })
@GET("subscriptions/{subscriptionId}/providers/Microsoft.KeyVault/locations/{location}/deletedVaults/{vaultName}")
Observable> getDeleted(@Path("vaultName") String vaultName, @Path("location") String location, @Path("subscriptionId") String subscriptionId, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.keyvault.Vaults purgeDeleted" })
@POST("subscriptions/{subscriptionId}/providers/Microsoft.KeyVault/locations/{location}/deletedVaults/{vaultName}/purge")
Observable> purgeDeleted(@Path("vaultName") String vaultName, @Path("location") String location, @Path("subscriptionId") String subscriptionId, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.keyvault.Vaults beginPurgeDeleted" })
@POST("subscriptions/{subscriptionId}/providers/Microsoft.KeyVault/locations/{location}/deletedVaults/{vaultName}/purge")
Observable> beginPurgeDeleted(@Path("vaultName") String vaultName, @Path("location") String location, @Path("subscriptionId") String subscriptionId, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.keyvault.Vaults list" })
@GET("subscriptions/{subscriptionId}/resources")
Observable> list(@Path("subscriptionId") String subscriptionId, @Query("$filter") String filter, @Query("$top") Integer top, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.keyvault.Vaults checkNameAvailability" })
@POST("subscriptions/{subscriptionId}/providers/Microsoft.KeyVault/checkNameAvailability")
Observable> checkNameAvailability(@Path("subscriptionId") String subscriptionId, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Body VaultCheckNameAvailabilityParameters vaultName, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.keyvault.Vaults listByResourceGroupNext" })
@GET
Observable> listByResourceGroupNext(@Url String nextUrl, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.keyvault.Vaults listBySubscriptionNext" })
@GET
Observable> listBySubscriptionNext(@Url String nextUrl, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.keyvault.Vaults listDeletedNext" })
@GET
Observable> listDeletedNext(@Url String nextUrl, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.keyvault.Vaults listNext" })
@GET
Observable> listNext(@Url String nextUrl, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
}
/**
* Create or update a key vault in the specified subscription.
*
* @param resourceGroupName The name of the Resource Group to which the server belongs.
* @param vaultName Name of the vault
* @param parameters Parameters to create or update the vault
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the VaultInner object if successful.
*/
public VaultInner createOrUpdate(String resourceGroupName, String vaultName, VaultCreateOrUpdateParameters parameters) {
return createOrUpdateWithServiceResponseAsync(resourceGroupName, vaultName, parameters).toBlocking().last().body();
}
/**
* Create or update a key vault in the specified subscription.
*
* @param resourceGroupName The name of the Resource Group to which the server belongs.
* @param vaultName Name of the vault
* @param parameters Parameters to create or update the vault
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture createOrUpdateAsync(String resourceGroupName, String vaultName, VaultCreateOrUpdateParameters parameters, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(createOrUpdateWithServiceResponseAsync(resourceGroupName, vaultName, parameters), serviceCallback);
}
/**
* Create or update a key vault in the specified subscription.
*
* @param resourceGroupName The name of the Resource Group to which the server belongs.
* @param vaultName Name of the vault
* @param parameters Parameters to create or update the vault
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable for the request
*/
public Observable createOrUpdateAsync(String resourceGroupName, String vaultName, VaultCreateOrUpdateParameters parameters) {
return createOrUpdateWithServiceResponseAsync(resourceGroupName, vaultName, parameters).map(new Func1, VaultInner>() {
@Override
public VaultInner call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Create or update a key vault in the specified subscription.
*
* @param resourceGroupName The name of the Resource Group to which the server belongs.
* @param vaultName Name of the vault
* @param parameters Parameters to create or update the vault
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable for the request
*/
public Observable> createOrUpdateWithServiceResponseAsync(String resourceGroupName, String vaultName, VaultCreateOrUpdateParameters parameters) {
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (vaultName == null) {
throw new IllegalArgumentException("Parameter vaultName is required and cannot be null.");
}
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (this.client.apiVersion() == null) {
throw new IllegalArgumentException("Parameter this.client.apiVersion() is required and cannot be null.");
}
if (parameters == null) {
throw new IllegalArgumentException("Parameter parameters is required and cannot be null.");
}
Validator.validate(parameters);
Observable> observable = service.createOrUpdate(resourceGroupName, vaultName, this.client.subscriptionId(), this.client.apiVersion(), parameters, this.client.acceptLanguage(), this.client.userAgent());
return client.getAzureClient().getPutOrPatchResultAsync(observable, new TypeToken() { }.getType());
}
/**
* Create or update a key vault in the specified subscription.
*
* @param resourceGroupName The name of the Resource Group to which the server belongs.
* @param vaultName Name of the vault
* @param parameters Parameters to create or update the vault
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the VaultInner object if successful.
*/
public VaultInner beginCreateOrUpdate(String resourceGroupName, String vaultName, VaultCreateOrUpdateParameters parameters) {
return beginCreateOrUpdateWithServiceResponseAsync(resourceGroupName, vaultName, parameters).toBlocking().single().body();
}
/**
* Create or update a key vault in the specified subscription.
*
* @param resourceGroupName The name of the Resource Group to which the server belongs.
* @param vaultName Name of the vault
* @param parameters Parameters to create or update the vault
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture beginCreateOrUpdateAsync(String resourceGroupName, String vaultName, VaultCreateOrUpdateParameters parameters, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(beginCreateOrUpdateWithServiceResponseAsync(resourceGroupName, vaultName, parameters), serviceCallback);
}
/**
* Create or update a key vault in the specified subscription.
*
* @param resourceGroupName The name of the Resource Group to which the server belongs.
* @param vaultName Name of the vault
* @param parameters Parameters to create or update the vault
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the VaultInner object
*/
public Observable beginCreateOrUpdateAsync(String resourceGroupName, String vaultName, VaultCreateOrUpdateParameters parameters) {
return beginCreateOrUpdateWithServiceResponseAsync(resourceGroupName, vaultName, parameters).map(new Func1, VaultInner>() {
@Override
public VaultInner call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Create or update a key vault in the specified subscription.
*
* @param resourceGroupName The name of the Resource Group to which the server belongs.
* @param vaultName Name of the vault
* @param parameters Parameters to create or update the vault
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the VaultInner object
*/
public Observable> beginCreateOrUpdateWithServiceResponseAsync(String resourceGroupName, String vaultName, VaultCreateOrUpdateParameters parameters) {
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (vaultName == null) {
throw new IllegalArgumentException("Parameter vaultName is required and cannot be null.");
}
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (this.client.apiVersion() == null) {
throw new IllegalArgumentException("Parameter this.client.apiVersion() is required and cannot be null.");
}
if (parameters == null) {
throw new IllegalArgumentException("Parameter parameters is required and cannot be null.");
}
Validator.validate(parameters);
return service.beginCreateOrUpdate(resourceGroupName, vaultName, this.client.subscriptionId(), this.client.apiVersion(), parameters, this.client.acceptLanguage(), this.client.userAgent())
.flatMap(new Func1, Observable>>() {
@Override
public Observable> call(Response response) {
try {
ServiceResponse clientResponse = beginCreateOrUpdateDelegate(response);
return Observable.just(clientResponse);
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse beginCreateOrUpdateDelegate(Response response) throws CloudException, IOException, IllegalArgumentException {
return this.client.restClient().responseBuilderFactory().newInstance(this.client.serializerAdapter())
.register(200, new TypeToken() { }.getType())
.register(201, new TypeToken() { }.getType())
.registerError(CloudException.class)
.build(response);
}
/**
* Update a key vault in the specified subscription.
*
* @param resourceGroupName The name of the Resource Group to which the server belongs.
* @param vaultName Name of the vault
* @param parameters Parameters to patch the vault
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the VaultInner object if successful.
*/
public VaultInner update(String resourceGroupName, String vaultName, VaultPatchParameters parameters) {
return updateWithServiceResponseAsync(resourceGroupName, vaultName, parameters).toBlocking().single().body();
}
/**
* Update a key vault in the specified subscription.
*
* @param resourceGroupName The name of the Resource Group to which the server belongs.
* @param vaultName Name of the vault
* @param parameters Parameters to patch the vault
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture updateAsync(String resourceGroupName, String vaultName, VaultPatchParameters parameters, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(updateWithServiceResponseAsync(resourceGroupName, vaultName, parameters), serviceCallback);
}
/**
* Update a key vault in the specified subscription.
*
* @param resourceGroupName The name of the Resource Group to which the server belongs.
* @param vaultName Name of the vault
* @param parameters Parameters to patch the vault
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the VaultInner object
*/
public Observable updateAsync(String resourceGroupName, String vaultName, VaultPatchParameters parameters) {
return updateWithServiceResponseAsync(resourceGroupName, vaultName, parameters).map(new Func1, VaultInner>() {
@Override
public VaultInner call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Update a key vault in the specified subscription.
*
* @param resourceGroupName The name of the Resource Group to which the server belongs.
* @param vaultName Name of the vault
* @param parameters Parameters to patch the vault
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the VaultInner object
*/
public Observable> updateWithServiceResponseAsync(String resourceGroupName, String vaultName, VaultPatchParameters parameters) {
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (vaultName == null) {
throw new IllegalArgumentException("Parameter vaultName is required and cannot be null.");
}
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (this.client.apiVersion() == null) {
throw new IllegalArgumentException("Parameter this.client.apiVersion() is required and cannot be null.");
}
if (parameters == null) {
throw new IllegalArgumentException("Parameter parameters is required and cannot be null.");
}
Validator.validate(parameters);
return service.update(resourceGroupName, vaultName, this.client.subscriptionId(), this.client.apiVersion(), parameters, this.client.acceptLanguage(), this.client.userAgent())
.flatMap(new Func1, Observable>>() {
@Override
public Observable> call(Response response) {
try {
ServiceResponse clientResponse = updateDelegate(response);
return Observable.just(clientResponse);
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse updateDelegate(Response response) throws CloudException, IOException, IllegalArgumentException {
return this.client.restClient().responseBuilderFactory().newInstance(this.client.serializerAdapter())
.register(200, new TypeToken() { }.getType())
.register(201, new TypeToken() { }.getType())
.registerError(CloudException.class)
.build(response);
}
/**
* Deletes the specified Azure key vault.
*
* @param resourceGroupName The name of the Resource Group to which the vault belongs.
* @param vaultName The name of the vault to delete
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
*/
public void delete(String resourceGroupName, String vaultName) {
deleteWithServiceResponseAsync(resourceGroupName, vaultName).toBlocking().single().body();
}
/**
* Deletes the specified Azure key vault.
*
* @param resourceGroupName The name of the Resource Group to which the vault belongs.
* @param vaultName The name of the vault to delete
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture deleteAsync(String resourceGroupName, String vaultName, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(deleteWithServiceResponseAsync(resourceGroupName, vaultName), serviceCallback);
}
/**
* Deletes the specified Azure key vault.
*
* @param resourceGroupName The name of the Resource Group to which the vault belongs.
* @param vaultName The name of the vault to delete
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceResponse} object if successful.
*/
public Observable deleteAsync(String resourceGroupName, String vaultName) {
return deleteWithServiceResponseAsync(resourceGroupName, vaultName).map(new Func1, Void>() {
@Override
public Void call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Deletes the specified Azure key vault.
*
* @param resourceGroupName The name of the Resource Group to which the vault belongs.
* @param vaultName The name of the vault to delete
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceResponse} object if successful.
*/
public Observable> deleteWithServiceResponseAsync(String resourceGroupName, String vaultName) {
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (vaultName == null) {
throw new IllegalArgumentException("Parameter vaultName is required and cannot be null.");
}
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (this.client.apiVersion() == null) {
throw new IllegalArgumentException("Parameter this.client.apiVersion() is required and cannot be null.");
}
return service.delete(resourceGroupName, vaultName, this.client.subscriptionId(), this.client.apiVersion(), this.client.acceptLanguage(), this.client.userAgent())
.flatMap(new Func1, Observable>>() {
@Override
public Observable> call(Response response) {
try {
ServiceResponse clientResponse = deleteDelegate(response);
return Observable.just(clientResponse);
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse deleteDelegate(Response response) throws CloudException, IOException, IllegalArgumentException {
return this.client.restClient().responseBuilderFactory().newInstance(this.client.serializerAdapter())
.register(200, new TypeToken() { }.getType())
.registerError(CloudException.class)
.build(response);
}
/**
* Gets the specified Azure key vault.
*
* @param resourceGroupName The name of the Resource Group to which the vault belongs.
* @param vaultName The name of the vault.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the VaultInner object if successful.
*/
public VaultInner getByResourceGroup(String resourceGroupName, String vaultName) {
return getByResourceGroupWithServiceResponseAsync(resourceGroupName, vaultName).toBlocking().single().body();
}
/**
* Gets the specified Azure key vault.
*
* @param resourceGroupName The name of the Resource Group to which the vault belongs.
* @param vaultName The name of the vault.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture getByResourceGroupAsync(String resourceGroupName, String vaultName, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(getByResourceGroupWithServiceResponseAsync(resourceGroupName, vaultName), serviceCallback);
}
/**
* Gets the specified Azure key vault.
*
* @param resourceGroupName The name of the Resource Group to which the vault belongs.
* @param vaultName The name of the vault.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the VaultInner object
*/
public Observable getByResourceGroupAsync(String resourceGroupName, String vaultName) {
return getByResourceGroupWithServiceResponseAsync(resourceGroupName, vaultName).map(new Func1, VaultInner>() {
@Override
public VaultInner call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Gets the specified Azure key vault.
*
* @param resourceGroupName The name of the Resource Group to which the vault belongs.
* @param vaultName The name of the vault.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the VaultInner object
*/
public Observable> getByResourceGroupWithServiceResponseAsync(String resourceGroupName, String vaultName) {
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (vaultName == null) {
throw new IllegalArgumentException("Parameter vaultName is required and cannot be null.");
}
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (this.client.apiVersion() == null) {
throw new IllegalArgumentException("Parameter this.client.apiVersion() is required and cannot be null.");
}
return service.getByResourceGroup(resourceGroupName, vaultName, this.client.subscriptionId(), this.client.apiVersion(), this.client.acceptLanguage(), this.client.userAgent())
.flatMap(new Func1, Observable>>() {
@Override
public Observable> call(Response response) {
try {
ServiceResponse clientResponse = getByResourceGroupDelegate(response);
return Observable.just(clientResponse);
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse getByResourceGroupDelegate(Response response) throws CloudException, IOException, IllegalArgumentException {
return this.client.restClient().responseBuilderFactory().newInstance(this.client.serializerAdapter())
.register(200, new TypeToken() { }.getType())
.registerError(CloudException.class)
.build(response);
}
/**
* Update access policies in a key vault in the specified subscription.
*
* @param resourceGroupName The name of the Resource Group to which the vault belongs.
* @param vaultName Name of the vault
* @param operationKind Name of the operation. Possible values include: 'add', 'replace', 'remove'
* @param properties Properties of the access policy
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the VaultAccessPolicyParametersInner object if successful.
*/
public VaultAccessPolicyParametersInner updateAccessPolicy(String resourceGroupName, String vaultName, AccessPolicyUpdateKind operationKind, VaultAccessPolicyProperties properties) {
return updateAccessPolicyWithServiceResponseAsync(resourceGroupName, vaultName, operationKind, properties).toBlocking().single().body();
}
/**
* Update access policies in a key vault in the specified subscription.
*
* @param resourceGroupName The name of the Resource Group to which the vault belongs.
* @param vaultName Name of the vault
* @param operationKind Name of the operation. Possible values include: 'add', 'replace', 'remove'
* @param properties Properties of the access policy
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture updateAccessPolicyAsync(String resourceGroupName, String vaultName, AccessPolicyUpdateKind operationKind, VaultAccessPolicyProperties properties, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(updateAccessPolicyWithServiceResponseAsync(resourceGroupName, vaultName, operationKind, properties), serviceCallback);
}
/**
* Update access policies in a key vault in the specified subscription.
*
* @param resourceGroupName The name of the Resource Group to which the vault belongs.
* @param vaultName Name of the vault
* @param operationKind Name of the operation. Possible values include: 'add', 'replace', 'remove'
* @param properties Properties of the access policy
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the VaultAccessPolicyParametersInner object
*/
public Observable updateAccessPolicyAsync(String resourceGroupName, String vaultName, AccessPolicyUpdateKind operationKind, VaultAccessPolicyProperties properties) {
return updateAccessPolicyWithServiceResponseAsync(resourceGroupName, vaultName, operationKind, properties).map(new Func1, VaultAccessPolicyParametersInner>() {
@Override
public VaultAccessPolicyParametersInner call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Update access policies in a key vault in the specified subscription.
*
* @param resourceGroupName The name of the Resource Group to which the vault belongs.
* @param vaultName Name of the vault
* @param operationKind Name of the operation. Possible values include: 'add', 'replace', 'remove'
* @param properties Properties of the access policy
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the VaultAccessPolicyParametersInner object
*/
public Observable> updateAccessPolicyWithServiceResponseAsync(String resourceGroupName, String vaultName, AccessPolicyUpdateKind operationKind, VaultAccessPolicyProperties properties) {
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (vaultName == null) {
throw new IllegalArgumentException("Parameter vaultName is required and cannot be null.");
}
if (operationKind == null) {
throw new IllegalArgumentException("Parameter operationKind is required and cannot be null.");
}
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (this.client.apiVersion() == null) {
throw new IllegalArgumentException("Parameter this.client.apiVersion() is required and cannot be null.");
}
if (properties == null) {
throw new IllegalArgumentException("Parameter properties is required and cannot be null.");
}
Validator.validate(properties);
VaultAccessPolicyParametersInner parameters = new VaultAccessPolicyParametersInner();
parameters.withProperties(properties);
return service.updateAccessPolicy(resourceGroupName, vaultName, operationKind, this.client.subscriptionId(), this.client.apiVersion(), this.client.acceptLanguage(), parameters, this.client.userAgent())
.flatMap(new Func1, Observable>>() {
@Override
public Observable> call(Response response) {
try {
ServiceResponse clientResponse = updateAccessPolicyDelegate(response);
return Observable.just(clientResponse);
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse updateAccessPolicyDelegate(Response response) throws CloudException, IOException, IllegalArgumentException {
return this.client.restClient().responseBuilderFactory().newInstance(this.client.serializerAdapter())
.register(200, new TypeToken() { }.getType())
.register(201, new TypeToken() { }.getType())
.registerError(CloudException.class)
.build(response);
}
/**
* The List operation gets information about the vaults associated with the subscription and within the specified resource group.
*
* @param resourceGroupName The name of the Resource Group to which the vault belongs.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the PagedList<VaultInner> object if successful.
*/
public PagedList listByResourceGroup(final String resourceGroupName) {
ServiceResponse> response = listByResourceGroupSinglePageAsync(resourceGroupName).toBlocking().single();
return new PagedList(response.body()) {
@Override
public Page nextPage(String nextPageLink) {
return listByResourceGroupNextSinglePageAsync(nextPageLink).toBlocking().single().body();
}
};
}
/**
* The List operation gets information about the vaults associated with the subscription and within the specified resource group.
*
* @param resourceGroupName The name of the Resource Group to which the vault belongs.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture> listByResourceGroupAsync(final String resourceGroupName, final ListOperationCallback serviceCallback) {
return AzureServiceFuture.fromPageResponse(
listByResourceGroupSinglePageAsync(resourceGroupName),
new Func1>>>() {
@Override
public Observable>> call(String nextPageLink) {
return listByResourceGroupNextSinglePageAsync(nextPageLink);
}
},
serviceCallback);
}
/**
* The List operation gets information about the vaults associated with the subscription and within the specified resource group.
*
* @param resourceGroupName The name of the Resource Group to which the vault belongs.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the PagedList<VaultInner> object
*/
public Observable> listByResourceGroupAsync(final String resourceGroupName) {
return listByResourceGroupWithServiceResponseAsync(resourceGroupName)
.map(new Func1>, Page>() {
@Override
public Page call(ServiceResponse> response) {
return response.body();
}
});
}
/**
* The List operation gets information about the vaults associated with the subscription and within the specified resource group.
*
* @param resourceGroupName The name of the Resource Group to which the vault belongs.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the PagedList<VaultInner> object
*/
public Observable>> listByResourceGroupWithServiceResponseAsync(final String resourceGroupName) {
return listByResourceGroupSinglePageAsync(resourceGroupName)
.concatMap(new Func1>, Observable>>>() {
@Override
public Observable>> call(ServiceResponse> page) {
String nextPageLink = page.body().nextPageLink();
if (nextPageLink == null) {
return Observable.just(page);
}
return Observable.just(page).concatWith(listByResourceGroupNextWithServiceResponseAsync(nextPageLink));
}
});
}
/**
* The List operation gets information about the vaults associated with the subscription and within the specified resource group.
*
* @param resourceGroupName The name of the Resource Group to which the vault belongs.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the PagedList<VaultInner> object wrapped in {@link ServiceResponse} if successful.
*/
public Observable>> listByResourceGroupSinglePageAsync(final String resourceGroupName) {
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (this.client.apiVersion() == null) {
throw new IllegalArgumentException("Parameter this.client.apiVersion() is required and cannot be null.");
}
final Integer top = null;
return service.listByResourceGroup(resourceGroupName, this.client.subscriptionId(), top, this.client.apiVersion(), this.client.acceptLanguage(), this.client.userAgent())
.flatMap(new Func1, Observable>>>() {
@Override
public Observable>> call(Response response) {
try {
ServiceResponse> result = listByResourceGroupDelegate(response);
return Observable.just(new ServiceResponse>(result.body(), result.response()));
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
/**
* The List operation gets information about the vaults associated with the subscription and within the specified resource group.
*
* @param resourceGroupName The name of the Resource Group to which the vault belongs.
* @param top Maximum number of results to return.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the PagedList<VaultInner> object if successful.
*/
public PagedList listByResourceGroup(final String resourceGroupName, final Integer top) {
ServiceResponse> response = listByResourceGroupSinglePageAsync(resourceGroupName, top).toBlocking().single();
return new PagedList(response.body()) {
@Override
public Page nextPage(String nextPageLink) {
return listByResourceGroupNextSinglePageAsync(nextPageLink).toBlocking().single().body();
}
};
}
/**
* The List operation gets information about the vaults associated with the subscription and within the specified resource group.
*
* @param resourceGroupName The name of the Resource Group to which the vault belongs.
* @param top Maximum number of results to return.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture> listByResourceGroupAsync(final String resourceGroupName, final Integer top, final ListOperationCallback serviceCallback) {
return AzureServiceFuture.fromPageResponse(
listByResourceGroupSinglePageAsync(resourceGroupName, top),
new Func1>>>() {
@Override
public Observable>> call(String nextPageLink) {
return listByResourceGroupNextSinglePageAsync(nextPageLink);
}
},
serviceCallback);
}
/**
* The List operation gets information about the vaults associated with the subscription and within the specified resource group.
*
* @param resourceGroupName The name of the Resource Group to which the vault belongs.
* @param top Maximum number of results to return.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the PagedList<VaultInner> object
*/
public Observable> listByResourceGroupAsync(final String resourceGroupName, final Integer top) {
return listByResourceGroupWithServiceResponseAsync(resourceGroupName, top)
.map(new Func1>, Page>() {
@Override
public Page call(ServiceResponse> response) {
return response.body();
}
});
}
/**
* The List operation gets information about the vaults associated with the subscription and within the specified resource group.
*
* @param resourceGroupName The name of the Resource Group to which the vault belongs.
* @param top Maximum number of results to return.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the PagedList<VaultInner> object
*/
public Observable>> listByResourceGroupWithServiceResponseAsync(final String resourceGroupName, final Integer top) {
return listByResourceGroupSinglePageAsync(resourceGroupName, top)
.concatMap(new Func1>, Observable>>>() {
@Override
public Observable>> call(ServiceResponse> page) {
String nextPageLink = page.body().nextPageLink();
if (nextPageLink == null) {
return Observable.just(page);
}
return Observable.just(page).concatWith(listByResourceGroupNextWithServiceResponseAsync(nextPageLink));
}
});
}
/**
* The List operation gets information about the vaults associated with the subscription and within the specified resource group.
*
ServiceResponse> * @param resourceGroupName The name of the Resource Group to which the vault belongs.
ServiceResponse> * @param top Maximum number of results to return.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the PagedList<VaultInner> object wrapped in {@link ServiceResponse} if successful.
*/
public Observable>> listByResourceGroupSinglePageAsync(final String resourceGroupName, final Integer top) {
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (this.client.apiVersion() == null) {
throw new IllegalArgumentException("Parameter this.client.apiVersion() is required and cannot be null.");
}
return service.listByResourceGroup(resourceGroupName, this.client.subscriptionId(), top, this.client.apiVersion(), this.client.acceptLanguage(), this.client.userAgent())
.flatMap(new Func1, Observable>>>() {
@Override
public Observable>> call(Response response) {
try {
ServiceResponse> result = listByResourceGroupDelegate(response);
return Observable.just(new ServiceResponse>(result.body(), result.response()));
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse> listByResourceGroupDelegate(Response response) throws CloudException, IOException, IllegalArgumentException {
return this.client.restClient().responseBuilderFactory()., CloudException>newInstance(this.client.serializerAdapter())
.register(200, new TypeToken>() { }.getType())
.registerError(CloudException.class)
.build(response);
}
/**
* The List operation gets information about the vaults associated with the subscription.
*
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the PagedList<VaultInner> object if successful.
*/
public PagedList listBySubscription() {
ServiceResponse> response = listBySubscriptionSinglePageAsync().toBlocking().single();
return new PagedList(response.body()) {
@Override
public Page nextPage(String nextPageLink) {
return listBySubscriptionNextSinglePageAsync(nextPageLink).toBlocking().single().body();
}
};
}
/**
* The List operation gets information about the vaults associated with the subscription.
*
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture> listBySubscriptionAsync(final ListOperationCallback serviceCallback) {
return AzureServiceFuture.fromPageResponse(
listBySubscriptionSinglePageAsync(),
new Func1>>>() {
@Override
public Observable>> call(String nextPageLink) {
return listBySubscriptionNextSinglePageAsync(nextPageLink);
}
},
serviceCallback);
}
/**
* The List operation gets information about the vaults associated with the subscription.
*
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the PagedList<VaultInner> object
*/
public Observable> listBySubscriptionAsync() {
return listBySubscriptionWithServiceResponseAsync()
.map(new Func1>, Page>() {
@Override
public Page call(ServiceResponse> response) {
return response.body();
}
});
}
/**
* The List operation gets information about the vaults associated with the subscription.
*
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the PagedList<VaultInner> object
*/
public Observable>> listBySubscriptionWithServiceResponseAsync() {
return listBySubscriptionSinglePageAsync()
.concatMap(new Func1>, Observable>>>() {
@Override
public Observable>> call(ServiceResponse> page) {
String nextPageLink = page.body().nextPageLink();
if (nextPageLink == null) {
return Observable.just(page);
}
return Observable.just(page).concatWith(listBySubscriptionNextWithServiceResponseAsync(nextPageLink));
}
});
}
/**
* The List operation gets information about the vaults associated with the subscription.
*
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the PagedList<VaultInner> object wrapped in {@link ServiceResponse} if successful.
*/
public Observable>> listBySubscriptionSinglePageAsync() {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (this.client.apiVersion() == null) {
throw new IllegalArgumentException("Parameter this.client.apiVersion() is required and cannot be null.");
}
final Integer top = null;
return service.listBySubscription(this.client.subscriptionId(), top, this.client.apiVersion(), this.client.acceptLanguage(), this.client.userAgent())
.flatMap(new Func1, Observable>>>() {
@Override
public Observable>> call(Response response) {
try {
ServiceResponse> result = listBySubscriptionDelegate(response);
return Observable.just(new ServiceResponse>(result.body(), result.response()));
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
/**
* The List operation gets information about the vaults associated with the subscription.
*
* @param top Maximum number of results to return.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the PagedList<VaultInner> object if successful.
*/
public PagedList listBySubscription(final Integer top) {
ServiceResponse> response = listBySubscriptionSinglePageAsync(top).toBlocking().single();
return new PagedList(response.body()) {
@Override
public Page nextPage(String nextPageLink) {
return listBySubscriptionNextSinglePageAsync(nextPageLink).toBlocking().single().body();
}
};
}
/**
* The List operation gets information about the vaults associated with the subscription.
*
* @param top Maximum number of results to return.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture> listBySubscriptionAsync(final Integer top, final ListOperationCallback serviceCallback) {
return AzureServiceFuture.fromPageResponse(
listBySubscriptionSinglePageAsync(top),
new Func1>>>() {
@Override
public Observable>> call(String nextPageLink) {
return listBySubscriptionNextSinglePageAsync(nextPageLink);
}
},
serviceCallback);
}
/**
* The List operation gets information about the vaults associated with the subscription.
*
* @param top Maximum number of results to return.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the PagedList<VaultInner> object
*/
public Observable> listBySubscriptionAsync(final Integer top) {
return listBySubscriptionWithServiceResponseAsync(top)
.map(new Func1>, Page>() {
@Override
public Page call(ServiceResponse> response) {
return response.body();
}
});
}
/**
* The List operation gets information about the vaults associated with the subscription.
*
* @param top Maximum number of results to return.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the PagedList<VaultInner> object
*/
public Observable>> listBySubscriptionWithServiceResponseAsync(final Integer top) {
return listBySubscriptionSinglePageAsync(top)
.concatMap(new Func1>, Observable>>>() {
@Override
public Observable>> call(ServiceResponse> page) {
String nextPageLink = page.body().nextPageLink();
if (nextPageLink == null) {
return Observable.just(page);
}
return Observable.just(page).concatWith(listBySubscriptionNextWithServiceResponseAsync(nextPageLink));
}
});
}
/**
* The List operation gets information about the vaults associated with the subscription.
*
ServiceResponse> * @param top Maximum number of results to return.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the PagedList<VaultInner> object wrapped in {@link ServiceResponse} if successful.
*/
public Observable>> listBySubscriptionSinglePageAsync(final Integer top) {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (this.client.apiVersion() == null) {
throw new IllegalArgumentException("Parameter this.client.apiVersion() is required and cannot be null.");
}
return service.listBySubscription(this.client.subscriptionId(), top, this.client.apiVersion(), this.client.acceptLanguage(), this.client.userAgent())
.flatMap(new Func1, Observable>>>() {
@Override
public Observable>> call(Response response) {
try {
ServiceResponse> result = listBySubscriptionDelegate(response);
return Observable.just(new ServiceResponse>(result.body(), result.response()));
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse> listBySubscriptionDelegate(Response response) throws CloudException, IOException, IllegalArgumentException {
return this.client.restClient().responseBuilderFactory()., CloudException>newInstance(this.client.serializerAdapter())
.register(200, new TypeToken>() { }.getType())
.registerError(CloudException.class)
.build(response);
}
/**
* Gets information about the deleted vaults in a subscription.
*
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the PagedList<DeletedVaultInner> object if successful.
*/
public PagedList listDeleted() {
ServiceResponse> response = listDeletedSinglePageAsync().toBlocking().single();
return new PagedList(response.body()) {
@Override
public Page nextPage(String nextPageLink) {
return listDeletedNextSinglePageAsync(nextPageLink).toBlocking().single().body();
}
};
}
/**
* Gets information about the deleted vaults in a subscription.
*
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture> listDeletedAsync(final ListOperationCallback serviceCallback) {
return AzureServiceFuture.fromPageResponse(
listDeletedSinglePageAsync(),
new Func1>>>() {
@Override
public Observable>> call(String nextPageLink) {
return listDeletedNextSinglePageAsync(nextPageLink);
}
},
serviceCallback);
}
/**
* Gets information about the deleted vaults in a subscription.
*
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the PagedList<DeletedVaultInner> object
*/
public Observable> listDeletedAsync() {
return listDeletedWithServiceResponseAsync()
.map(new Func1>, Page>() {
@Override
public Page call(ServiceResponse> response) {
return response.body();
}
});
}
/**
* Gets information about the deleted vaults in a subscription.
*
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the PagedList<DeletedVaultInner> object
*/
public Observable>> listDeletedWithServiceResponseAsync() {
return listDeletedSinglePageAsync()
.concatMap(new Func1>, Observable>>>() {
@Override
public Observable>> call(ServiceResponse> page) {
String nextPageLink = page.body().nextPageLink();
if (nextPageLink == null) {
return Observable.just(page);
}
return Observable.just(page).concatWith(listDeletedNextWithServiceResponseAsync(nextPageLink));
}
});
}
/**
* Gets information about the deleted vaults in a subscription.
*
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the PagedList<DeletedVaultInner> object wrapped in {@link ServiceResponse} if successful.
*/
public Observable>> listDeletedSinglePageAsync() {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (this.client.apiVersion() == null) {
throw new IllegalArgumentException("Parameter this.client.apiVersion() is required and cannot be null.");
}
return service.listDeleted(this.client.subscriptionId(), this.client.apiVersion(), this.client.acceptLanguage(), this.client.userAgent())
.flatMap(new Func1, Observable>>>() {
@Override
public Observable>> call(Response response) {
try {
ServiceResponse> result = listDeletedDelegate(response);
return Observable.just(new ServiceResponse>(result.body(), result.response()));
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse> listDeletedDelegate(Response response) throws CloudException, IOException, IllegalArgumentException {
return this.client.restClient().responseBuilderFactory()., CloudException>newInstance(this.client.serializerAdapter())
.register(200, new TypeToken>() { }.getType())
.registerError(CloudException.class)
.build(response);
}
/**
* Gets the deleted Azure key vault.
*
* @param vaultName The name of the vault.
* @param location The location of the deleted vault.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the DeletedVaultInner object if successful.
*/
public DeletedVaultInner getDeleted(String vaultName, String location) {
return getDeletedWithServiceResponseAsync(vaultName, location).toBlocking().single().body();
}
/**
* Gets the deleted Azure key vault.
*
* @param vaultName The name of the vault.
* @param location The location of the deleted vault.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture getDeletedAsync(String vaultName, String location, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(getDeletedWithServiceResponseAsync(vaultName, location), serviceCallback);
}
/**
* Gets the deleted Azure key vault.
*
* @param vaultName The name of the vault.
* @param location The location of the deleted vault.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the DeletedVaultInner object
*/
public Observable getDeletedAsync(String vaultName, String location) {
return getDeletedWithServiceResponseAsync(vaultName, location).map(new Func1, DeletedVaultInner>() {
@Override
public DeletedVaultInner call(ServiceResponse