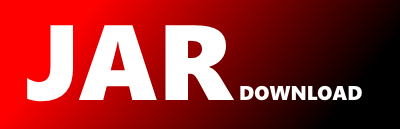
com.microsoft.azure.management.keyvault.implementation.KeyVaultManagementClientImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-mgmt-keyvault Show documentation
Show all versions of azure-mgmt-keyvault Show documentation
This package contains Microsoft Azure Key Vault Management SDK. This package is in low maintenance mode and being phased out. To use the latest Azure SDK for resource management, please see https://aka.ms/azsdk/java/mgmt
/**
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for
* license information.
*
* Code generated by Microsoft (R) AutoRest Code Generator.
*/
package com.microsoft.azure.management.keyvault.implementation;
import com.microsoft.azure.AzureClient;
import com.microsoft.azure.AzureServiceClient;
import com.microsoft.rest.credentials.ServiceClientCredentials;
import com.microsoft.rest.RestClient;
/**
* Initializes a new instance of the KeyVaultManagementClientImpl class.
*/
public class KeyVaultManagementClientImpl extends AzureServiceClient {
/** the {@link AzureClient} used for long running operations. */
private AzureClient azureClient;
/**
* Gets the {@link AzureClient} used for long running operations.
* @return the azure client;
*/
public AzureClient getAzureClient() {
return this.azureClient;
}
/** Subscription credentials which uniquely identify Microsoft Azure subscription. The subscription ID forms part of the URI for every service call. */
private String subscriptionId;
/**
* Gets Subscription credentials which uniquely identify Microsoft Azure subscription. The subscription ID forms part of the URI for every service call.
*
* @return the subscriptionId value.
*/
public String subscriptionId() {
return this.subscriptionId;
}
/**
* Sets Subscription credentials which uniquely identify Microsoft Azure subscription. The subscription ID forms part of the URI for every service call.
*
* @param subscriptionId the subscriptionId value.
* @return the service client itself
*/
public KeyVaultManagementClientImpl withSubscriptionId(String subscriptionId) {
this.subscriptionId = subscriptionId;
return this;
}
/** Client Api Version. */
private String apiVersion;
/**
* Gets Client Api Version.
*
* @return the apiVersion value.
*/
public String apiVersion() {
return this.apiVersion;
}
/** Gets or sets the preferred language for the response. */
private String acceptLanguage;
/**
* Gets Gets or sets the preferred language for the response.
*
* @return the acceptLanguage value.
*/
public String acceptLanguage() {
return this.acceptLanguage;
}
/**
* Sets Gets or sets the preferred language for the response.
*
* @param acceptLanguage the acceptLanguage value.
* @return the service client itself
*/
public KeyVaultManagementClientImpl withAcceptLanguage(String acceptLanguage) {
this.acceptLanguage = acceptLanguage;
return this;
}
/** Gets or sets the retry timeout in seconds for Long Running Operations. Default value is 30. */
private int longRunningOperationRetryTimeout;
/**
* Gets Gets or sets the retry timeout in seconds for Long Running Operations. Default value is 30.
*
* @return the longRunningOperationRetryTimeout value.
*/
public int longRunningOperationRetryTimeout() {
return this.longRunningOperationRetryTimeout;
}
/**
* Sets Gets or sets the retry timeout in seconds for Long Running Operations. Default value is 30.
*
* @param longRunningOperationRetryTimeout the longRunningOperationRetryTimeout value.
* @return the service client itself
*/
public KeyVaultManagementClientImpl withLongRunningOperationRetryTimeout(int longRunningOperationRetryTimeout) {
this.longRunningOperationRetryTimeout = longRunningOperationRetryTimeout;
return this;
}
/** When set to true a unique x-ms-client-request-id value is generated and included in each request. Default is true. */
private boolean generateClientRequestId;
/**
* Gets When set to true a unique x-ms-client-request-id value is generated and included in each request. Default is true.
*
* @return the generateClientRequestId value.
*/
public boolean generateClientRequestId() {
return this.generateClientRequestId;
}
/**
* Sets When set to true a unique x-ms-client-request-id value is generated and included in each request. Default is true.
*
* @param generateClientRequestId the generateClientRequestId value.
* @return the service client itself
*/
public KeyVaultManagementClientImpl withGenerateClientRequestId(boolean generateClientRequestId) {
this.generateClientRequestId = generateClientRequestId;
return this;
}
/**
* The VaultsInner object to access its operations.
*/
private VaultsInner vaults;
/**
* Gets the VaultsInner object to access its operations.
* @return the VaultsInner object.
*/
public VaultsInner vaults() {
return this.vaults;
}
/**
* The OperationsInner object to access its operations.
*/
private OperationsInner operations;
/**
* Gets the OperationsInner object to access its operations.
* @return the OperationsInner object.
*/
public OperationsInner operations() {
return this.operations;
}
/**
* Initializes an instance of KeyVaultManagementClient client.
*
* @param credentials the management credentials for Azure
*/
public KeyVaultManagementClientImpl(ServiceClientCredentials credentials) {
this("https://management.azure.com", credentials);
}
/**
* Initializes an instance of KeyVaultManagementClient client.
*
* @param baseUrl the base URL of the host
* @param credentials the management credentials for Azure
*/
public KeyVaultManagementClientImpl(String baseUrl, ServiceClientCredentials credentials) {
super(baseUrl, credentials);
initialize();
}
/**
* Initializes an instance of KeyVaultManagementClient client.
*
* @param restClient the REST client to connect to Azure.
*/
public KeyVaultManagementClientImpl(RestClient restClient) {
super(restClient);
initialize();
}
protected void initialize() {
this.apiVersion = "2018-02-14-preview";
this.acceptLanguage = "en-US";
this.longRunningOperationRetryTimeout = 30;
this.generateClientRequestId = true;
this.vaults = new VaultsInner(restClient().retrofit(), this);
this.operations = new OperationsInner(restClient().retrofit(), this);
this.azureClient = new AzureClient(this);
}
/**
* Gets the User-Agent header for the client.
*
* @return the user agent string.
*/
@Override
public String userAgent() {
return String.format("%s (%s, %s)", super.userAgent(), "KeyVaultManagementClient", "2018-02-14-preview");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy