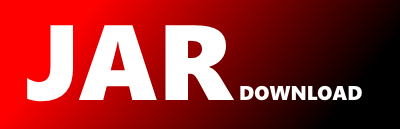
com.microsoft.azure.management.keyvault.implementation.OperationsInner Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-mgmt-keyvault Show documentation
Show all versions of azure-mgmt-keyvault Show documentation
This package contains Microsoft Azure Key Vault Management SDK. This package is in low maintenance mode and being phased out. To use the latest Azure SDK for resource management, please see https://aka.ms/azsdk/java/mgmt
/**
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for
* license information.
*
* Code generated by Microsoft (R) AutoRest Code Generator.
*/
package com.microsoft.azure.management.keyvault.implementation;
import retrofit2.Retrofit;
import com.google.common.reflect.TypeToken;
import com.microsoft.azure.AzureServiceFuture;
import com.microsoft.azure.CloudException;
import com.microsoft.azure.ListOperationCallback;
import com.microsoft.azure.Page;
import com.microsoft.azure.PagedList;
import com.microsoft.rest.ServiceFuture;
import com.microsoft.rest.ServiceResponse;
import java.io.IOException;
import java.util.List;
import okhttp3.ResponseBody;
import retrofit2.http.GET;
import retrofit2.http.Header;
import retrofit2.http.Headers;
import retrofit2.http.Query;
import retrofit2.http.Url;
import retrofit2.Response;
import rx.functions.Func1;
import rx.Observable;
/**
* An instance of this class provides access to all the operations defined
* in Operations.
*/
public class OperationsInner {
/** The Retrofit service to perform REST calls. */
private OperationsService service;
/** The service client containing this operation class. */
private KeyVaultManagementClientImpl client;
/**
* Initializes an instance of OperationsInner.
*
* @param retrofit the Retrofit instance built from a Retrofit Builder.
* @param client the instance of the service client containing this operation class.
*/
public OperationsInner(Retrofit retrofit, KeyVaultManagementClientImpl client) {
this.service = retrofit.create(OperationsService.class);
this.client = client;
}
/**
* The interface defining all the services for Operations to be
* used by Retrofit to perform actually REST calls.
*/
interface OperationsService {
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.keyvault.Operations list" })
@GET("providers/Microsoft.KeyVault/operations")
Observable> list(@Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.keyvault.Operations listNext" })
@GET
Observable> listNext(@Url String nextUrl, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
}
/**
* Lists all of the available Key Vault Rest API operations.
*
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the PagedList<OperationInner> object if successful.
*/
public PagedList list() {
ServiceResponse> response = listSinglePageAsync().toBlocking().single();
return new PagedList(response.body()) {
@Override
public Page nextPage(String nextPageLink) {
return listNextSinglePageAsync(nextPageLink).toBlocking().single().body();
}
};
}
/**
* Lists all of the available Key Vault Rest API operations.
*
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture> listAsync(final ListOperationCallback serviceCallback) {
return AzureServiceFuture.fromPageResponse(
listSinglePageAsync(),
new Func1>>>() {
@Override
public Observable>> call(String nextPageLink) {
return listNextSinglePageAsync(nextPageLink);
}
},
serviceCallback);
}
/**
* Lists all of the available Key Vault Rest API operations.
*
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the PagedList<OperationInner> object
*/
public Observable> listAsync() {
return listWithServiceResponseAsync()
.map(new Func1>, Page>() {
@Override
public Page call(ServiceResponse> response) {
return response.body();
}
});
}
/**
* Lists all of the available Key Vault Rest API operations.
*
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the PagedList<OperationInner> object
*/
public Observable>> listWithServiceResponseAsync() {
return listSinglePageAsync()
.concatMap(new Func1>, Observable>>>() {
@Override
public Observable>> call(ServiceResponse> page) {
String nextPageLink = page.body().nextPageLink();
if (nextPageLink == null) {
return Observable.just(page);
}
return Observable.just(page).concatWith(listNextWithServiceResponseAsync(nextPageLink));
}
});
}
/**
* Lists all of the available Key Vault Rest API operations.
*
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the PagedList<OperationInner> object wrapped in {@link ServiceResponse} if successful.
*/
public Observable>> listSinglePageAsync() {
if (this.client.apiVersion() == null) {
throw new IllegalArgumentException("Parameter this.client.apiVersion() is required and cannot be null.");
}
return service.list(this.client.apiVersion(), this.client.acceptLanguage(), this.client.userAgent())
.flatMap(new Func1, Observable>>>() {
@Override
public Observable>> call(Response response) {
try {
ServiceResponse> result = listDelegate(response);
return Observable.just(new ServiceResponse>(result.body(), result.response()));
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse> listDelegate(Response response) throws CloudException, IOException, IllegalArgumentException {
return this.client.restClient().responseBuilderFactory()., CloudException>newInstance(this.client.serializerAdapter())
.register(200, new TypeToken>() { }.getType())
.registerError(CloudException.class)
.build(response);
}
/**
* Lists all of the available Key Vault Rest API operations.
*
* @param nextPageLink The NextLink from the previous successful call to List operation.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the PagedList<OperationInner> object if successful.
*/
public PagedList listNext(final String nextPageLink) {
ServiceResponse> response = listNextSinglePageAsync(nextPageLink).toBlocking().single();
return new PagedList(response.body()) {
@Override
public Page nextPage(String nextPageLink) {
return listNextSinglePageAsync(nextPageLink).toBlocking().single().body();
}
};
}
/**
* Lists all of the available Key Vault Rest API operations.
*
* @param nextPageLink The NextLink from the previous successful call to List operation.
* @param serviceFuture the ServiceFuture object tracking the Retrofit calls
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture> listNextAsync(final String nextPageLink, final ServiceFuture> serviceFuture, final ListOperationCallback serviceCallback) {
return AzureServiceFuture.fromPageResponse(
listNextSinglePageAsync(nextPageLink),
new Func1>>>() {
@Override
public Observable>> call(String nextPageLink) {
return listNextSinglePageAsync(nextPageLink);
}
},
serviceCallback);
}
/**
* Lists all of the available Key Vault Rest API operations.
*
* @param nextPageLink The NextLink from the previous successful call to List operation.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the PagedList<OperationInner> object
*/
public Observable> listNextAsync(final String nextPageLink) {
return listNextWithServiceResponseAsync(nextPageLink)
.map(new Func1>, Page>() {
@Override
public Page call(ServiceResponse> response) {
return response.body();
}
});
}
/**
* Lists all of the available Key Vault Rest API operations.
*
* @param nextPageLink The NextLink from the previous successful call to List operation.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the PagedList<OperationInner> object
*/
public Observable>> listNextWithServiceResponseAsync(final String nextPageLink) {
return listNextSinglePageAsync(nextPageLink)
.concatMap(new Func1>, Observable>>>() {
@Override
public Observable>> call(ServiceResponse> page) {
String nextPageLink = page.body().nextPageLink();
if (nextPageLink == null) {
return Observable.just(page);
}
return Observable.just(page).concatWith(listNextWithServiceResponseAsync(nextPageLink));
}
});
}
/**
* Lists all of the available Key Vault Rest API operations.
*
ServiceResponse> * @param nextPageLink The NextLink from the previous successful call to List operation.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the PagedList<OperationInner> object wrapped in {@link ServiceResponse} if successful.
*/
public Observable>> listNextSinglePageAsync(final String nextPageLink) {
if (nextPageLink == null) {
throw new IllegalArgumentException("Parameter nextPageLink is required and cannot be null.");
}
String nextUrl = String.format("%s", nextPageLink);
return service.listNext(nextUrl, this.client.acceptLanguage(), this.client.userAgent())
.flatMap(new Func1, Observable>>>() {
@Override
public Observable>> call(Response response) {
try {
ServiceResponse> result = listNextDelegate(response);
return Observable.just(new ServiceResponse>(result.body(), result.response()));
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse> listNextDelegate(Response response) throws CloudException, IOException, IllegalArgumentException {
return this.client.restClient().responseBuilderFactory()., CloudException>newInstance(this.client.serializerAdapter())
.register(200, new TypeToken>() { }.getType())
.registerError(CloudException.class)
.build(response);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy