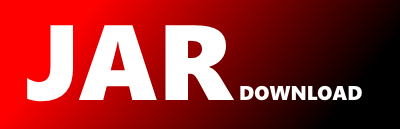
com.microsoft.azure.management.monitor.implementation.LogSearchRuleResourceInner Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-mgmt-monitor Show documentation
Show all versions of azure-mgmt-monitor Show documentation
This package contains Microsoft Azure Monitor SDK.
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy