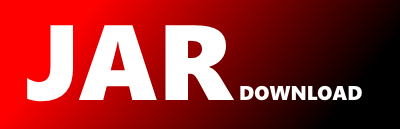
com.microsoft.azure.management.network.ApplicationGatewayBackend Maven / Gradle / Ivy
Show all versions of azure-mgmt-network Show documentation
/**
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for
* license information.
*/
package com.microsoft.azure.management.network;
import java.util.Collection;
import com.microsoft.azure.management.apigeneration.Fluent;
import com.microsoft.azure.management.network.model.HasBackendNics;
import com.microsoft.azure.management.resources.fluentcore.arm.models.ChildResource;
import com.microsoft.azure.management.resources.fluentcore.model.Attachable;
import com.microsoft.azure.management.resources.fluentcore.model.Settable;
import com.microsoft.azure.management.resources.fluentcore.model.HasInner;
/**
* A client-side representation of an application gateway backend.
*/
@Fluent()
public interface ApplicationGatewayBackend extends
HasInner,
ChildResource,
HasBackendNics {
/**
* @return addresses on the backend of the application gateway
*/
Collection addresses();
/**
* Checks whether the specified IP address is referenced by this backend address pool.
* @param ipAddress an IP address
* @return true if the specified IP address is referenced by this backend, else false
*/
boolean containsIPAddress(String ipAddress);
/**
* Checks whether the specified FQDN is referenced by this backend address pool.
* @param fqdn a fully qualified domain name (FQDN)
* @return true if the specified FQDN is referenced by this backend, else false
*/
boolean containsFqdn(String fqdn);
/**
* Grouping of application gateway backend definition stages.
*/
interface DefinitionStages {
/**
* The first stage of an application gateway backend definition.
* @param the stage of the parent application gateway definition to return to after attaching this definition
*/
interface Blank extends WithAttach {
}
/**
* The stage of an application gateway backend definition allowing to add an address to the backend.
* @param the stage of the parent application gateway definition to return to after attaching this definition
*/
interface WithAddress {
/**
* Adds the specified existing IP address to the backend.
*
* This call can be made in a sequence to add multiple IP addresses.
* @param ipAddress an IP address
* @return the next stage of the definition
*/
WithAttach withIPAddress(String ipAddress);
/**
* Adds the specified existing fully qualified domain name (FQDN) to the backend.
*
* This call can be made in a sequence to add multiple FQDNs.
* @param fqdn a fully qualified domain name (FQDN)
* @return the next stage of the definition
*/
WithAttach withFqdn(String fqdn);
}
/** The final stage of an application gateway backend definition.
*
* At this stage, any remaining optional settings can be specified, or the definition
* can be attached to the parent application gateway definition.
* @param the stage of the parent application gateway definition to return to after attaching this definition
*/
interface WithAttach extends
Attachable.InDefinition,
WithAddress {
}
}
/** The entirety of an application gateway backend definition.
* @param the stage of the parent application gateway definition to return to after attaching this definition
*/
interface Definition extends
DefinitionStages.Blank,
DefinitionStages.WithAttach {
}
/**
* Grouping of application gateway backend update stages.
*/
interface UpdateStages {
/**
* The stage of an application gateway backend update allowing to add an address to the backend.
*/
interface WithAddress {
/**
* Adds the specified existing IP address to the backend.
* @param ipAddress an IP address
* @return the next stage of the update
*/
Update withIPAddress(String ipAddress);
/**
* Adds the specified existing fully qualified domain name (FQDN) to the backend.
* @param fqdn a fully qualified domain name (FQDN)
* @return the next stage of the update
*/
Update withFqdn(String fqdn);
/**
* Ensures the specified IP address is not associated with this backend.
* @param ipAddress an IP address
* @return the next stage of the update
*/
Update withoutIPAddress(String ipAddress);
/**
* Ensure the specified address is not associated with this backend.
* @param address an existing address currently associated with the backend
* @return the next stage of the update
*/
Update withoutAddress(ApplicationGatewayBackendAddress address);
/**
* Ensures the specified fully qualified domain name (FQDN) is not associated with this backend.
* @param fqdn a fully qualified domain name
* @return the next stage of the update
*/
Update withoutFqdn(String fqdn);
}
}
/**
* The entirety of an application gateway backend update as part of an application gateway update.
*/
interface Update extends
Settable,
UpdateStages.WithAddress {
}
/**
* Grouping of application gateway backend definition stages applicable as part of an application gateway update.
*/
interface UpdateDefinitionStages {
/**
* The first stage of an application gateway backend definition.
* @param the stage of the parent application gateway definition to return to after attaching this definition
*/
interface Blank extends WithAttach {
}
/**
* The stage of an application gateway backed definition allowing to add an address to the backend.
* @param the stage of the parent application gateway definition to return to after attaching this definition
*/
interface WithAddress {
/**
* Adds the specified existing IP address to the backend.
* @param ipAddress an IP address
* @return the next stage of the definition
*/
WithAttach withIPAddress(String ipAddress);
/**
* Adds the specified existing fully qualified domain name (FQDN) to the backend.
* @param fqdn a fully qualified domain name (FQDN)
* @return the next stage of the definition
*/
WithAttach withFqdn(String fqdn);
}
/** The final stage of an application gateway backend definition.
*
* At this stage, any remaining optional settings can be specified, or the definition
* can be attached to the parent application gateway definition.
* @param the stage of the parent application gateway definition to return to after attaching this definition
*/
interface WithAttach extends
Attachable.InUpdate,
WithAddress {
}
}
/** The entirety of an application gateway backend definition as part of an application gateway update.
* @param the stage of the parent application gateway definition to return to after attaching this definition
*/
interface UpdateDefinition extends
UpdateDefinitionStages.Blank,
UpdateDefinitionStages.WithAttach {
}
}