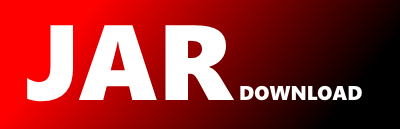
com.microsoft.azure.management.network.ApplicationGatewayProbe Maven / Gradle / Ivy
Show all versions of azure-mgmt-network Show documentation
/**
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for
* license information.
*/
package com.microsoft.azure.management.network;
import java.util.Set;
import com.microsoft.azure.management.apigeneration.Beta;
import com.microsoft.azure.management.apigeneration.Beta.SinceVersion;
import com.microsoft.azure.management.apigeneration.Fluent;
import com.microsoft.azure.management.apigeneration.Method;
import com.microsoft.azure.management.network.implementation.ApplicationGatewayProbeInner;
import com.microsoft.azure.management.network.model.HasProtocol;
import com.microsoft.azure.management.resources.fluentcore.arm.models.ChildResource;
import com.microsoft.azure.management.resources.fluentcore.model.Attachable;
import com.microsoft.azure.management.resources.fluentcore.model.Settable;
import com.microsoft.azure.management.resources.fluentcore.model.HasInner;
/**
* A client-side representation of an application gateway probe.
*/
@Fluent()
public interface ApplicationGatewayProbe extends
HasInner,
ChildResource,
HasProtocol {
/**
* @return the number of seconds between probe retries
*/
int timeBetweenProbesInSeconds();
/**
* @return HTTP response code ranges in the format ###-### returned by the backend which the probe considers healthy.
*/
@Beta(SinceVersion.V1_4_0)
Set healthyHttpResponseStatusCodeRanges();
/**
* @return the body contents of an HTTP response to a probe to check for to determine backend health, or null if none specified
*/
@Beta(SinceVersion.V1_4_0)
String healthyHttpResponseBodyContents();
/**
* @return the relative path to be called by the probe
*/
String path();
/**
* @return the number of seconds waiting for a response after which the probe times out and it is marked as failed
* Acceptable values are from 1 to 86400 seconds.
*/
int timeoutInSeconds();
/**
* @return the number of failed retry probes before the backend server is marked as being down
*
Acceptable values are from 1 second to 20.
*/
int retriesBeforeUnhealthy();
/**
* @return host name to send the probe to
*/
String host();
/**
* Grouping of application gateway probe definition stages.
*/
interface DefinitionStages {
/**
* The first stage of an application gateway probe definition.
* @param the stage of the parent application gateway definition to return to after attaching this definition
*/
interface Blank extends WithHost {
}
/**
* Stage of an application gateway probe definition allowing to specify the host to send the probe to.
* @param the stage of the parent application gateway definition to return to after attaching this definition
*/
interface WithHost {
/**
* Specifies the host name to send the probe to.
* @param host a host name
* @return the next stage of the definition
*/
WithPath withHost(String host);
}
/**
* Stage of an application gateway probe definition allowing to specify the relative path to send the probe to.
* @param the stage of the parent application gateway definition to return to after attaching this definition
*/
interface WithPath {
/**
* Specifies the relative path for the probe to call.
* A probe is sent to <protocol>://<host>:<port><path>.
* @param path a relative path
* @return the next stage of the definition
*/
WithProtocol withPath(String path);
}
/**
* Stage of an application gateway probe update allowing to specify the protocol of the probe.
* @param the stage of the parent application gateway definition to return to after attaching this definition
*/
interface WithProtocol extends HasProtocol.DefinitionStages.WithProtocol, ApplicationGatewayProtocol> {
/**
* Specifies HTTP as the probe protocol.
* @return the next stage of the definition
*/
WithTimeout withHttp();
/**
* Specifies HTTPS as the probe protocol.
* @return the next stage of the definition
*/
WithTimeout withHttps();
}
/**
* Stage of an application gateway probe definition allowing to specify the amount of time to after which the probe is considered failed.
* @param the stage of the parent application gateway definition to return to after attaching this definition
*/
interface WithTimeout {
/**
* Specifies the amount of time in seconds waiting for a response before the probe is considered failed.
* @param seconds a number of seconds, between 1 and 86400
* @return the next stage of the definition
*/
WithAttach withTimeoutInSeconds(int seconds);
}
/**
* Stage of an application gateway probe definition allowing to specify the time interval between consecutive probes.
* @param the stage of the parent application gateway definition to return to after attaching this definition
*/
interface WithInterval {
/**
* Specifies the time interval in seconds between consecutive probes.
* @param seconds a number of seconds between 1 and 86400
* @return the next stage of the definition
*/
WithAttach withTimeBetweenProbesInSeconds(int seconds);
}
/**
* Stage of an application gateway probe definition allowing to specify the number of retries before the server is considered unhealthy.
* @param the stage of the parent application gateway definition to return to after attaching this definition
*/
interface WithRetries {
/**
* Specifies the number of retries before the server is considered unhealthy.
* @param retryCount a number between 1 and 20
* @return the next stage of the definition
*/
WithAttach withRetriesBeforeUnhealthy(int retryCount);
}
/**
* The stage of an application gateway probe definition allowing to specify healthy HTTP response status code ranges.
* @param the stage of the parent application gateway definition to return to after attaching this definition
*/
interface WithHealthyHttpResponseStatusCodeRanges {
/**
* Specifies the ranges of the backend's HTTP response status codes that are to be considered healthy.
* @param ranges number ranges expressed in the format "###-###", for example "200-399", which is the default
* @return the next stage of the definition
*/
@Beta(SinceVersion.V1_4_0)
WithAttach withHealthyHttpResponseStatusCodeRanges(Set ranges);
/**
* Adds the specified range of the backend's HTTP response status codes that are to be considered healthy.
* @param range a number range expressed in the format "###-###", for example "200-399", which is the default
* @return the next stage of the definition
*/
@Beta(SinceVersion.V1_4_0)
WithAttach withHealthyHttpResponseStatusCodeRange(String range);
/**
* Adds the specified range of the backend's HTTP response status codes that are to be considered healthy.
* @param from the lowest number in the range
* @param to the highest number in the range
* @return the next stage of the definition
*/
@Beta(SinceVersion.V1_4_0)
WithAttach withHealthyHttpResponseStatusCodeRange(int from, int to);
}
/**
* The stage of an application gateway probe definition allowing to specify the body contents of a healthy HTTP response to a probe.
* @param the stage of the parent application gateway definition to return to after attaching this definition
*/
interface WithHealthyHttpResponseBodyContents {
/**
* Specifies the content, if any, to look for in the body of an HTTP response to a probe to determine the health status of the backend.
* @param text contents to look for
* @return the next stage of the definition
*/
@Beta(SinceVersion.V1_4_0)
WithAttach withHealthyHttpResponseBodyContents(String text);
}
/**
* The final stage of an application gateway probe definition.
*
* At this stage, any remaining optional settings can be specified, or the probe definition
* can be attached to the parent application gateway definition.
* @param the stage of the parent application gateway definition to return to after attaching this definition
*/
interface WithAttach extends
Attachable.InDefinitionAlt,
WithInterval,
WithRetries,
WithHealthyHttpResponseStatusCodeRanges,
WithHealthyHttpResponseBodyContents {
}
}
/**
* The entirety of an application gateway probe definition.
* @param the stage of the parent application gateway definition to return to after attaching this definition
*/
interface Definition extends
DefinitionStages.Blank,
DefinitionStages.WithAttach,
DefinitionStages.WithProtocol,
DefinitionStages.WithPath,
DefinitionStages.WithHost,
DefinitionStages.WithTimeout {
}
/**
* Grouping of application gateway probe update stages.
*/
interface UpdateStages {
/**
* Stage of an application gateway probe update allowing to specify the host to send the probe to.
*/
interface WithHost {
/**
* Specifies the host name to send the probe to.
* @param host a host name
* @return the next stage of the update
*/
Update withHost(String host);
}
/**
* Stage of an application gateway probe update allowing to specify the protocol of the probe.
*/
interface WithProtocol extends HasProtocol.UpdateStages.WithProtocol {
/**
* Specifies HTTP as the probe protocol.
* @return the next stage of the update
*/
Update withHttp();
/**
* Specifies HTTPS as the probe protocol.
* @return the next stage of the update
*/
Update withHttps();
}
/**
* Stage of an application gateway probe update allowing to specify the path to send the probe to.
*/
interface WithPath {
/**
* Specifies the relative path for the probe to call.
* A probe is sent to <protocol>://<host>:<port><path>.
* @param path a relative path
* @return the next stage of the update
*/
Update withPath(String path);
}
/**
* Stage of an application gateway probe update allowing to specify the amount of time to after which the probe is considered failed.
*/
interface WithTimeout {
/**
* Specifies the amount of time in seconds waiting for a response before the probe is considered failed.
* @param seconds a number of seconds between 1 and 86400
* @return the next stage of the update
*/
Update withTimeoutInSeconds(int seconds);
}
/**
* Stage of an application gateway probe update allowing to specify the time interval between consecutive probes.
*/
interface WithInterval {
/**
* Specifies the time interval in seconds between consecutive probes.
* @param seconds a number of seconds between 1 and 86400
* @return the next stage of the update
*/
Update withTimeBetweenProbesInSeconds(int seconds);
}
/**
* Stage of an application gateway probe update allowing to specify the number of retries before the server is considered unhealthy.
*/
interface WithRetries {
/**
* Specifies the number of retries before the server is considered unhealthy.
* @param retryCount a number between 1 and 20
* @return the next stage of the update
*/
Update withRetriesBeforeUnhealthy(int retryCount);
}
/**
* The stage of an application gateway probe update allowing to specify healthy HTTP response status code ranges.
*/
interface WithHealthyHttpResponseStatusCodeRanges {
/**
* Specifies the ranges of the backend's HTTP response status codes that are to be considered healthy.
* @param ranges number ranges expressed in the format "###-###", for example "200-399", which is the default
* @return the next stage of the update
*/
@Beta(SinceVersion.V1_4_0)
Update withHealthyHttpResponseStatusCodeRanges(Set ranges);
/**
* Adds the specified range of the backend's HTTP response status codes that are to be considered healthy.
* @param range a number range expressed in the format "###-###", for example "200-399", which is the default
* @return the next stage of the update
*/
@Beta(SinceVersion.V1_4_0)
Update withHealthyHttpResponseStatusCodeRange(String range);
/**
* Adds the specified range of the backend's HTTP response status codes that are to be considered healthy.
* @param from the lowest number in the range
* @param to the highest number in the range
* @return the next stage of the update
*/
@Beta(SinceVersion.V1_4_0)
Update withHealthyHttpResponseStatusCodeRange(int from, int to);
/**
* Removes all healthy HTTP status response code ranges.
* @return the next stage of the update
*/
@Method
@Beta(SinceVersion.V1_4_0)
Update withoutHealthyHttpResponseStatusCodeRanges();
}
/**
* The stage of an application gateway probe update allowing to specify the body contents of a healthy HTTP response to a probe.
*/
interface WithHealthyHttpResponseBodyContents {
/**
* Specifies the content, if any, to look for in the body of an HTTP response to a probe to determine the health status of the backend.
* @param text contents to look for
* @return the next stage of the definition
*/
@Beta(SinceVersion.V1_4_0)
Update withHealthyHttpResponseBodyContents(String text);
}
}
/**
* The entirety of an application gateway probe update as part of an application gateway update.
*/
interface Update extends
Settable,
UpdateStages.WithProtocol,
UpdateStages.WithPath,
UpdateStages.WithHost,
UpdateStages.WithTimeout,
UpdateStages.WithInterval,
UpdateStages.WithRetries,
UpdateStages.WithHealthyHttpResponseStatusCodeRanges,
UpdateStages.WithHealthyHttpResponseBodyContents {
}
/**
* Grouping of application gateway probe definition stages applicable as part of an application gateway update.
*/
interface UpdateDefinitionStages {
/**
* The first stage of an application gateway probe definition.
* @param the stage of the parent application gateway definition to return to after attaching this definition
*/
interface Blank extends WithHost {
}
/**
* Stage of an application gateway probe definition allowing to specify the host to send the probe to.
* @param the stage of the parent application gateway update to return to after attaching this definition
*/
interface WithHost {
/**
* Specifies the host name to send the probe to.
* @param host a host name
* @return the next stage of the definition
*/
WithPath withHost(String host);
}
/**
* Stage of an application gateway probe definition allowing to specify the protocol of the probe.
* @param the stage of the parent application gateway update to return to after attaching this definition
*/
interface WithProtocol extends HasProtocol.UpdateDefinitionStages.WithProtocol, ApplicationGatewayProtocol> {
/**
* Specifies HTTP as the probe protocol.
* @return the next stage of the definition
*/
WithTimeout withHttp();
/**
* Specifies HTTPS as the probe protocol.
* @return the next stage of the definition
*/
WithTimeout withHttps();
}
/**
* Stage of an application gateway probe definition allowing to specify the amount of time to after which the probe is considered failed.
* @param the stage of the parent application gateway update to return to after attaching this definition
*/
interface WithTimeout {
/**
* Specifies the amount of time in seconds waiting for a response before the probe is considered failed.
* @param seconds a number of seconds between 1 and 86400
* @return the next stage of the definition
*/
WithAttach withTimeoutInSeconds(int seconds);
}
/**
* Stage of an application gateway probe definition allowing to specify the path to send the probe to.
* @param the stage of the parent application gateway update to return to after attaching this definition
*/
interface WithPath {
/**
* Specifies the relative path for the probe to call.
* A probe is sent to <protocol>://<host>:<port><path>.
* @param path a relative path
* @return the next stage of the definition
*/
WithProtocol withPath(String path);
}
/**
* Stage of an application gateway probe definition allowing to specify the time interval between consecutive probes.
* @param the stage of the parent application gateway update to return to after attaching this definition
*/
interface WithInterval {
/**
* Specifies the time interval in seconds between consecutive probes.
* @param seconds a number of seconds between 1 and 86400
* @return the next stage of the definition
*/
WithAttach withTimeBetweenProbesInSeconds(int seconds);
}
/**
* Stage of an application gateway probe definition allowing to specify the number of retries before the server is considered unhealthy.
* @param the stage of the parent application gateway update to return to after attaching this definition
*/
interface WithRetries {
/**
* Specifies the number of retries before the server is considered unhealthy.
* @param retryCount a number between 1 and 20
* @return the next stage of the definition
*/
WithAttach withRetriesBeforeUnhealthy(int retryCount);
}
/**
* The stage of an application gateway probe definition allowing to specify healthy HTTP response status code ranges.
* @param the stage of the parent application gateway update to return to after attaching this definition
*/
interface WithHealthyHttpResponseStatusCodeRanges {
/**
* Specifies the ranges of the backend's HTTP response status codes that are to be considered healthy.
* @param ranges number ranges expressed in the format "###-###", for example "200-399", which is the default
* @return the next stage of the definition
*/
@Beta(SinceVersion.V1_4_0)
WithAttach withHealthyHttpResponseStatusCodeRanges(Set ranges);
/**
* Adds the specified range of the backend's HTTP response status codes that are to be considered healthy.
* @param range a number range expressed in the format "###-###", for example "200-399", which is the default
* @return the next stage of the definition
*/
@Beta(SinceVersion.V1_4_0)
WithAttach withHealthyHttpResponseStatusCodeRange(String range);
/**
* Adds the specified range of the backend's HTTP response status codes that are to be considered healthy.
* @param from the lowest number in the range
* @param to the highest number in the range
* @return the next stage of the definition
*/
@Beta(SinceVersion.V1_4_0)
WithAttach withHealthyHttpResponseStatusCodeRange(int from, int to);
}
/**
* The stage of an application gateway probe definition allowing to specify the body contents of a healthy HTTP response to a probe.
* @param the stage of the parent application gateway update to return to after attaching this definition
*/
interface WithHealthyHttpResponseBodyContents {
/**
* Specifies the content, if any, to look for in the body of an HTTP response to a probe to determine the health status of the backend.
* @param text contents to look for
* @return the next stage of the definition
*/
@Beta(SinceVersion.V1_4_0)
WithAttach withHealthyHttpResponseBodyContents(String text);
}
/** The final stage of an application gateway probe definition.
*
* At this stage, any remaining optional settings can be specified, or the probe definition
* can be attached to the parent application gateway definition.
* @param the stage of the parent application gateway definition to return to after attaching this definition
*/
interface WithAttach extends
Attachable.InUpdateAlt,
WithInterval,
WithRetries,
WithHealthyHttpResponseStatusCodeRanges,
WithHealthyHttpResponseBodyContents {
}
}
/** The entirety of an application gateway probe definition as part of an application gateway update.
* @param the stage of the parent application gateway definition to return to after attaching this definition
*/
interface UpdateDefinition extends
UpdateDefinitionStages.Blank,
UpdateDefinitionStages.WithAttach,
UpdateDefinitionStages.WithProtocol,
UpdateDefinitionStages.WithPath,
UpdateDefinitionStages.WithHost,
UpdateDefinitionStages.WithTimeout,
UpdateDefinitionStages.WithInterval {
}
}