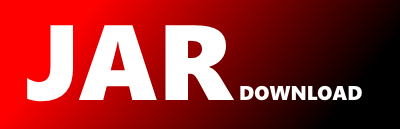
com.microsoft.azure.management.network.Ipv6PeeringConfig Maven / Gradle / Ivy
Show all versions of azure-mgmt-network Show documentation
/**
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for
* license information.
*/
package com.microsoft.azure.management.network;
import java.util.List;
import com.microsoft.azure.management.apigeneration.Beta;
import com.microsoft.azure.management.apigeneration.Fluent;
import com.microsoft.azure.management.apigeneration.Method;
import com.microsoft.azure.management.resources.fluentcore.model.Attachable;
import com.microsoft.azure.management.resources.fluentcore.model.HasInner;
import com.microsoft.azure.management.resources.fluentcore.model.Settable;
/**
* An client-side representation of a load balancer frontend.
*/
@Fluent
@Beta(Beta.SinceVersion.V1_11_0)
public interface Ipv6PeeringConfig extends
HasInner {
/**
* Grouping of public frontend definition stages.
*/
interface DefinitionStages {
/**
* The first stage of a public frontend definition.
* @param the stage of the parent definition to return to after attaching this definition
*/
interface Blank extends WithAdvertisedPublicPrefixes {
}
/**
* The stage of Cross Connection Peering IPv6 configuration definition allowing to specify primary address prefix.
*/
interface WithPrimaryPeerAddressPrefix {
/**
* @param addressPrefix primary peer address prefix
* @return the next stage of the definition
*/
WithSecondaryPeerAddressPrefix withPrimaryPeerAddressPrefix(String addressPrefix);
}
/**
* The stage of Cross Connection Peering IPv6 configuration definition allowing to specify secondary address prefix.
*/
interface WithSecondaryPeerAddressPrefix {
/**
* @param addressPrefix secondary peer address prefix
* @return the next stage of the definition
*/
WithAttach withSecondaryPeerAddressPrefix(String addressPrefix);
}
/**
* The stage of Cross Connection Peering IPv6 configuration definition allowing to specify customer ASN.
*/
interface WithCustomerASN {
/**
* Specifies customer ASN.
* @param customerASN customer ASN
* @return the next satge of the definition
*/
WithRoutingRegistryName withCustomerASN(int customerASN);
}
/**
* The stage of Cross Connection Peering IPv6 configuration definition allowing to specify routing registry name.
*/
interface WithRoutingRegistryName {
/**
* Specifies routing registry name.
* @param routingRegistryName routing registry name
* @return the next stage of the definition
*/
WithPrimaryPeerAddressPrefix withRoutingRegistryName(String routingRegistryName);
}
/**
* The stage of Cross Connection Peering IPv6 configuration definition allowing to specify secondary address prefix.
*/
interface WithAdvertisedPublicPrefixes {
/**
* Specify advertised prefixes: sets a list of all prefixes that are planned to advertise over the BGP session. Method will overwrite existing list.
* Only public IP address prefixes are accepted. A set of prefixes can be sent as a comma-separated list.
* These prefixes must be registered to you in an RIR / IRR.
* @param publicPrefixes advertised prefixes
* @return next stage of definition
*/
WithCustomerASN withAdvertisedPublicPrefixes(List publicPrefixes);
/**
* Specify advertised prefix: sets a prefix that is planned to advertise over the BGP session. Method will add a prefix to existing list.
* Only public IP address prefixes are accepted. A set of prefixes can be sent as a comma-separated list.
* These prefixes must be registered to you in an RIR / IRR.
* @param publicPrefix advertised prefix
* @return next stage of definition
*/
WithCustomerASN withAdvertisedPublicPrefix(String publicPrefix);
}
/**
* The stage of Cross Connection Peering IPv6 configuration definition allowing to specify route filter.
*/
interface WithRouteFilter {
/**
* Sets route filter id.
* @param routeFilterId route filter id
* @return the next stage of the definition
*/
WithAttach withRouteFilter(String routeFilterId);
/**
* Remove route filter from IPv6 configuration.
* @return the next stage of the definition
*/
@Method
WithAttach withoutRouteFilter();
}
/**
* The final stage of a public frontend definition.
*
* At this stage, any remaining optional settings can be specified, or the frontend definition
* can be attached to the parent load balancer definition.
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithAttach extends
Attachable.InDefinition,
WithRouteFilter {
}
}
/** The entirety of a public frontend definition.
* @param the stage of the parent definition to return to after attaching this definition
*/
interface Definition extends
DefinitionStages.Blank,
DefinitionStages.WithAttach,
DefinitionStages.WithPrimaryPeerAddressPrefix,
DefinitionStages.WithSecondaryPeerAddressPrefix,
DefinitionStages.WithCustomerASN,
DefinitionStages.WithRoutingRegistryName {
}
/**
* Grouping of public frontend update stages.
*/
interface UpdateStages {
/**
* The stage of a public frontend update allowing to specify an existing public IP address.
*/
interface WithAdvertisedPublicPrefixes {
/**
* Specify advertised prefixes: sets a list of all prefixes that are planned to advertise over the BGP session.
* Only public IP address prefixes are accepted. A set of prefixes can be sent as a comma-separated list.
* These prefixes must be registered to you in an RIR / IRR.
* @param publicPrefixes advertised prefixes
* @return next stage of update
*/
Update withAdvertisedPublicPrefixes(List publicPrefixes);
/**
* Specify advertised prefix: sets a prefix that is planned to advertise over the BGP session. Method will add a prefix to existing list.
* Only public IP address prefixes are accepted. A set of prefixes can be sent as a comma-separated list.
* These prefixes must be registered to you in an RIR / IRR.
* @param publicPrefix advertised prefix
* @return next stage of definition
*/
Update withAdvertisedPublicPrefix(String publicPrefix);
}
/**
* The stage of Cross Connection Peering IPv6 configuration update allowing to specify primary address prefix.
*/
interface WithPrimaryPeerAddressPrefix {
/**
* @param addressPrefix primary peer address prefix
* @return the next stage of the update
*/
Update withPrimaryPeerAddressPrefix(String addressPrefix);
}
/**
* The stage of Cross Connection Peering IPv6 configuration update allowing to specify secondary address prefix.
*/
interface WithSecondaryPeerAddressPrefix {
/**
* @param addressPrefix secondary peer address prefix
* @return the next stage of the update
*/
Update withSecondaryPeerAddressPrefix(String addressPrefix);
}
/**
* The stage of Cross Connection Peering IPv6 configuration update allowing to specify secondary customer ASN.
*/
interface WithCustomerASN {
/**
* Specifies customer ASN.
* @param customerASN customer ASN
* @return the next stage of the update
*/
Update withCustomerASN(int customerASN);
}
/**
* The stage of Cross Connection Peering IPv6 configuration update allowing to specify routing registry name.
*/
interface WithRoutingRegistryName {
/**
* Specifies routing registry name.
* @param routingRegistryName routing registry name
* @return the next stage of the definition
*/
Update withRoutingRegistryName(String routingRegistryName);
}
/**
* The stage of Cross Connection Peering IPv6 configuration update allowing to specify route filter.
*/
interface WithRouteFilter {
/**
* Sets route filter id.
* @param routeFilterId route filter id
* @return the next stage of the definition
*/
Update withRouteFilter(String routeFilterId);
/**
* Remove route filter from IPv6 configuration.
* @return the next stage of the definition
*/
@Method
Update withoutRouteFilter();
}
}
/**
* The entirety of a public frontend update as part of an Internet-facing load balancer update.
*/
interface Update extends
Settable,
UpdateStages.WithAdvertisedPublicPrefixes,
UpdateStages.WithPrimaryPeerAddressPrefix,
UpdateStages.WithSecondaryPeerAddressPrefix,
UpdateStages.WithCustomerASN,
UpdateStages.WithRoutingRegistryName,
UpdateStages.WithRouteFilter {
}
/**
* Grouping of public frontend definition stages applicable as part of an Internet-facing load balancer update.
*/
interface UpdateDefinitionStages {
/**
* The first stage of a public frontend definition.
* @param the stage of the parent definition to return to after attaching this definition
*/
interface Blank extends UpdateDefinitionStages.WithAdvertisedPublicPrefixes {
}
interface WithAdvertisedPublicPrefixes {
/**
* Specify advertised prefixes: sets a list of all prefixes that are planned to advertise over the BGP session.
* Only public IP address prefixes are accepted. A set of prefixes can be sent as a comma-separated list.
* These prefixes must be registered to you in an RIR / IRR.
* @param publicPrefixes advertised prefixes
* @return next stage of definition
*/
WithCustomerASN withAdvertisedPublicPrefixes(List publicPrefixes);
/**
* Specify advertised prefix: sets a prefix that is planned to advertise over the BGP session. Method will add a prefix to existing list.
* Only public IP address prefixes are accepted. A set of prefixes can be sent as a comma-separated list.
* These prefixes must be registered to you in an RIR / IRR.
* @param publicPrefix advertised prefix
* @return next stage of definition
*/
WithCustomerASN withAdvertisedPublicPrefix(String publicPrefix);
}
/**
* The stage of Cross Connection Peering IPv6 configuration definition allowing to specify primary address prefix.
*/
interface WithPrimaryPeerAddressPrefix {
/**
* @param addressPrefix primary peer address prefix
* @return the next stage of the definition
*/
WithSecondaryPeerAddressPrefix withPrimaryPeerAddressPrefix(String addressPrefix);
}
/**
* The stage of Cross Connection Peering IPv6 configuration definition allowing to specify secondary address prefix.
*/
interface WithSecondaryPeerAddressPrefix {
/**
* @param addressPrefix secondary peer address prefix
* @return the next stage of the definition
*/
WithAttach withSecondaryPeerAddressPrefix(String addressPrefix);
}
/**
* The stage of Cross Connection Peering IPv6 configuration definition allowing to specify secondary customer ASN.
*/
interface WithCustomerASN {
/**
* Specifies customer ASN.
* @param customerASN customer ASN
* @return the next satge of the definition
*/
WithRoutingRegistryName withCustomerASN(int customerASN);
}
/**
* The stage of Cross Connection Peering IPv6 configuration definition allowing to specify routing registry name.
*/
interface WithRoutingRegistryName {
/**
* Specifies routing registry name.
* @param routingRegistryName routing registry name
* @return the next stage of the definition
*/
WithPrimaryPeerAddressPrefix withRoutingRegistryName(String routingRegistryName);
}
/**
* The stage of Cross Connection Peering IPv6 configuration definition allowing to specify route filter.
*/
interface WithRouteFilter {
/**
* Sets route filter id.
* @param routeFilterId route filter id
* @return the next stage of the definition
*/
WithAttach withRouteFilter(String routeFilterId);
/**
* Remove route filter from IPv6 configuration.
* @return the next stage of the definition
*/
@Method
WithAttach withoutRouteFilter();
}
/** The final stage of peering IPv6 configuration definition.
*
* At this stage, any remaining optional settings can be specified, or the frontend definition
* can be attached to the parent peering definition definition.
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithAttach extends
Attachable.InUpdate,
WithRouteFilter {
}
}
/** The entirety of Cross Connection Peering IPv6 configuration definition as part of Cross Connection Peering update.
* @param the stage of the parent definition to return to after attaching this definition
*/
interface UpdateDefinition extends
UpdateDefinitionStages.Blank,
UpdateDefinitionStages.WithAttach,
UpdateDefinitionStages.WithAdvertisedPublicPrefixes,
UpdateDefinitionStages.WithPrimaryPeerAddressPrefix,
UpdateDefinitionStages.WithSecondaryPeerAddressPrefix,
UpdateDefinitionStages.WithCustomerASN,
UpdateDefinitionStages.WithRoutingRegistryName {
}
}