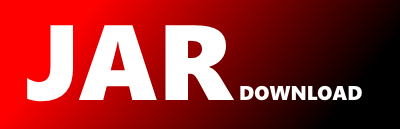
com.microsoft.azure.management.network.Subnet Maven / Gradle / Ivy
Show all versions of azure-mgmt-network Show documentation
/**
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for
* license information.
*/
package com.microsoft.azure.management.network;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.microsoft.azure.management.apigeneration.Beta;
import com.microsoft.azure.management.apigeneration.Fluent;
import com.microsoft.azure.management.apigeneration.Method;
import com.microsoft.azure.management.apigeneration.Beta.SinceVersion;
import com.microsoft.azure.management.network.implementation.SubnetInner;
import com.microsoft.azure.management.resources.fluentcore.arm.Region;
import com.microsoft.azure.management.resources.fluentcore.arm.models.ChildResource;
import com.microsoft.azure.management.resources.fluentcore.model.Attachable;
import com.microsoft.azure.management.resources.fluentcore.model.Settable;
import com.microsoft.azure.management.resources.fluentcore.model.HasInner;
/**
* A client-side representation of a subnet of a virtual network.
*/
@Fluent()
public interface Subnet extends
HasInner,
ChildResource {
/**
* @return network interface IP configurations that are associated with this subnet
*
* Note that this call may result in multiple calls to Azure to fetch all the referenced interfaces each time it is invoked.
*
* @deprecated Use {@link Subnet#listNetworkInterfaceIPConfigurations()} instead.
*/
@Method
@Deprecated
Set getNetworkInterfaceIPConfigurations();
/**
* @return network interface IP configurations that are associated with this subnet
*
* Note that this call may result in multiple calls to Azure to fetch all the referenced interfaces each time it is invoked.
*/
@Method
Collection listNetworkInterfaceIPConfigurations();
/**
* @return available private IP addresses within this network
*/
@Beta(SinceVersion.V1_3_0)
Set listAvailablePrivateIPAddresses();
/**
* @return number of network interface IP configurations associated with this subnet
*/
int networkInterfaceIPConfigurationCount();
/**
* @return the address space prefix, in CIDR notation, assigned to this subnet
*/
String addressPrefix();
/**
* @return the network security group associated with this subnet, if any
*
* Note that this method will result in a call to Azure each time it is invoked.
*/
NetworkSecurityGroup getNetworkSecurityGroup();
/**
* @return the resource ID of the network security group associated with this subnet, if any
*/
String networkSecurityGroupId();
/**
* @return the route table associated with this subnet, if any
*
* Note that this method will result in a call to Azure each time it is invoked.
*/
RouteTable getRouteTable();
/**
* @return the resource ID of the route table associated with this subnet, if any
*/
String routeTableId();
/**
* @return the services that has access to the subnet.
*/
@Beta(SinceVersion.V1_6_0)
Map> servicesWithAccess();
/**
* Grouping of subnet definition stages.
*/
interface DefinitionStages {
/**
* The first stage of the subnet definition.
* @param the stage of the parent definition to return to after attaching this definition
*/
interface Blank extends WithAddressPrefix {
}
/**
* The stage of the subnet definition allowing to specify the address space for the subnet.
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithAddressPrefix {
/**
* Specifies the IP address space of the subnet, within the address space of the network.
* @param cidr the IP address space prefix using the CIDR notation
* @return the next stage of the definition
*/
WithAttach withAddressPrefix(String cidr);
}
/**
* The stage of the subnet definition allowing to specify the network security group to assign to the subnet.
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithNetworkSecurityGroup {
/**
* Assigns an existing network security group to this subnet.
* @param resourceId the resource ID of the network security group
* @return the next stage of the definition
*/
WithAttach withExistingNetworkSecurityGroup(String resourceId);
/**
* Assigns an existing network security group to this subnet.
* @param nsg the network security group to assign
* @return the next stage of the definition
*/
WithAttach withExistingNetworkSecurityGroup(NetworkSecurityGroup nsg);
}
/**
* The stage of a subnet definition allowing to specify a route table to associate with the subnet.
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithRouteTable {
/**
* Specifies an existing route table to associate with the subnet.
* @param routeTable an existing route table to associate
* @return the next stage of the definition
*/
WithAttach withExistingRouteTable(RouteTable routeTable);
/**
* Specifies an existing route table to associate with the subnet.
* @param resourceId the resource ID of an existing route table
* @return the next stage of the definition
*/
WithAttach withExistingRouteTable(String resourceId);
}
/**
* The stage of a subnet definition allowing to enable access from a service endpoint to the subnet.
* @param the stage of the parent definition to return to after attaching this definition
*/
@Beta(SinceVersion.V1_6_0)
interface WithServiceEndpoint {
/**
* Specifies a service endpoint to enable access from.
* @param service the service type
* @return the next stage of the definition
*/
@Beta(SinceVersion.V1_6_0)
WithAttach withAccessFromService(ServiceEndpointType service);
}
/** The final stage of the subnet definition.
*
* At this stage, any remaining optional settings can be specified, or the subnet definition
* can be attached to the parent virtual network definition.
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithAttach extends
Attachable.InDefinition,
WithNetworkSecurityGroup,
WithRouteTable,
WithServiceEndpoint {
}
}
/** The entirety of a Subnet definition.
* @param the stage of the parent definition to return to after attaching this definition
*/
interface Definition extends
DefinitionStages.Blank,
DefinitionStages.WithAddressPrefix,
DefinitionStages.WithAttach {
}
/**
* Grouping of subnet update stages.
*/
interface UpdateStages {
/**
* The stage of the subnet update allowing to change the address space for the subnet.
*/
interface WithAddressPrefix {
/**
* Specifies the IP address space of the subnet, within the address space of the network.
* @param cidr the IP address space prefix using the CIDR notation
* @return the next stage
*/
Update withAddressPrefix(String cidr);
}
/**
* The stage of the subnet update allowing to change the network security group to assign to the subnet.
*/
interface WithNetworkSecurityGroup {
/**
* Assigns an existing network security group to this subnet.
* @param resourceId the resource ID of the network security group
* @return the next stage of the update
*/
Update withExistingNetworkSecurityGroup(String resourceId);
/**
* Assigns an existing network security group to this subnet.
* @param nsg the network security group to assign
* @return the next stage of the update
*/
Update withExistingNetworkSecurityGroup(NetworkSecurityGroup nsg);
/**
* Removes the association of this subnet with any network security group.
* @return the next stage of the update
*/
Update withoutNetworkSecurityGroup();
}
/**
* The stage of a subnet update allowing to specify a route table to associate with the subnet, or remove an existing association.
*/
interface WithRouteTable {
/**
* Specifies an existing route table to associate with the subnet.
* @param routeTable an existing route table to associate
* @return the next stage of the update
*/
Update withExistingRouteTable(RouteTable routeTable);
/**
* Specifies an existing route table to associate with the subnet.
* @param resourceId the resource ID of an existing route table
* @return the next stage of the update
*/
Update withExistingRouteTable(String resourceId);
/**
* Removes the association with a route table, if any.
* @return the next stage of the update
*/
Update withoutRouteTable();
}
/**
* The stage of a subnet definition allowing to enable or disable access from a service endpoint
* to the subnet.
*/
@Beta(SinceVersion.V1_6_0)
interface WithServiceEndpoint {
/**
* Specifies a service endpoint to enable access from.
* @param service the service type
* @return the next stage of the definition
*/
@Beta(SinceVersion.V1_6_0)
Update withAccessFromService(ServiceEndpointType service);
/**
* Specifies that existing access from a service endpoint should be removed.
* @param service the service type
* @return the next stage of the definition
*/
@Beta(SinceVersion.V1_6_0)
Update withoutAccessFromService(ServiceEndpointType service);
}
}
/**
* The entirety of a subnet update as part of a network update.
*/
interface Update extends
UpdateStages.WithAddressPrefix,
UpdateStages.WithNetworkSecurityGroup,
UpdateStages.WithRouteTable,
UpdateStages.WithServiceEndpoint,
Settable {
}
/**
* Grouping of subnet definition stages applicable as part of a virtual network update.
*/
interface UpdateDefinitionStages {
/**
* The first stage of the subnet definition.
* @param the stage of the parent definition to return to after attaching this definition
*/
interface Blank extends WithAddressPrefix {
}
/**
* The stage of the subnet definition allowing to specify the address space for the subnet.
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithAddressPrefix {
/**
* Specifies the IP address space of the subnet, within the address space of the network.
* @param cidr the IP address space prefix using the CIDR notation
* @return the next stage of the definition
*/
WithAttach withAddressPrefix(String cidr);
}
/**
* The stage of the subnet definition allowing to specify the network security group to assign to the subnet.
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithNetworkSecurityGroup {
/**
* Assigns an existing network security group to this subnet.
* @param resourceId the resource ID of the network security group
* @return the next stage of the definition
*/
WithAttach withExistingNetworkSecurityGroup(String resourceId);
/**
* Assigns an existing network security group to this subnet.
* @param nsg the network security group to assign
* @return the next stage of the definition
*/
WithAttach withExistingNetworkSecurityGroup(NetworkSecurityGroup nsg);
}
/**
* The stage of a subnet definition allowing to specify a route table to associate with the subnet.
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithRouteTable {
/**
* Specifies an existing route table to associate with the subnet.
* @param routeTable an existing route table to associate
* @return the next stage of the definition
*/
WithAttach withExistingRouteTable(RouteTable routeTable);
/**
* Specifies an existing route table to associate with the subnet.
* @param resourceId the resource ID of an existing route table
* @return the next stage of the definition
*/
WithAttach withExistingRouteTable(String resourceId);
}
/**
* The stage of a subnet definition allowing to enable access from a service endpoint to the subnet.
* @param the stage of the parent definition to return to after attaching this definition
*/
@Beta(SinceVersion.V1_6_0)
interface WithServiceEndpoint {
/**
* Specifies a service endpoint to enable access from.
* @param service the service type
* @return the next stage of the definition
*/
@Beta(SinceVersion.V1_6_0)
WithAttach withAccessFromService(ServiceEndpointType service);
}
/** The final stage of the subnet definition.
*
* At this stage, any remaining optional settings can be specified, or the subnet definition
* can be attached to the parent virtual network definition.
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithAttach extends
Attachable.InUpdate,
WithNetworkSecurityGroup,
WithRouteTable,
WithServiceEndpoint {
}
}
/** The entirety of a subnet definition as part of a virtual network update.
* @param the stage of the parent definition to return to after attaching this definition
*/
interface UpdateDefinition extends
UpdateDefinitionStages.Blank,
UpdateDefinitionStages.WithAddressPrefix,
UpdateDefinitionStages.WithNetworkSecurityGroup,
UpdateDefinitionStages.WithAttach {
}
}