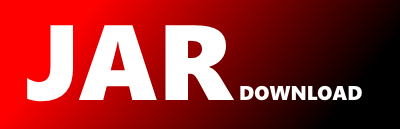
com.microsoft.azure.management.resources.DeploymentPropertiesExtended Maven / Gradle / Ivy
/**
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for
* license information.
*
* Code generated by Microsoft (R) AutoRest Code Generator.
*/
package com.microsoft.azure.management.resources;
import org.joda.time.DateTime;
import java.util.List;
import com.microsoft.azure.management.resources.implementation.ProviderInner;
import com.fasterxml.jackson.annotation.JsonProperty;
/**
* Deployment properties with additional details.
*/
public class DeploymentPropertiesExtended {
/**
* The state of the provisioning.
*/
@JsonProperty(value = "provisioningState", access = JsonProperty.Access.WRITE_ONLY)
private String provisioningState;
/**
* The correlation ID of the deployment.
*/
@JsonProperty(value = "correlationId", access = JsonProperty.Access.WRITE_ONLY)
private String correlationId;
/**
* The timestamp of the template deployment.
*/
@JsonProperty(value = "timestamp", access = JsonProperty.Access.WRITE_ONLY)
private DateTime timestamp;
/**
* Key/value pairs that represent deploymentoutput.
*/
@JsonProperty(value = "outputs")
private Object outputs;
/**
* The list of resource providers needed for the deployment.
*/
@JsonProperty(value = "providers")
private List providers;
/**
* The list of deployment dependencies.
*/
@JsonProperty(value = "dependencies")
private List dependencies;
/**
* The template content. Use only one of Template or TemplateLink.
*/
@JsonProperty(value = "template")
private Object template;
/**
* The URI referencing the template. Use only one of Template or
* TemplateLink.
*/
@JsonProperty(value = "templateLink")
private TemplateLink templateLink;
/**
* Deployment parameters. Use only one of Parameters or ParametersLink.
*/
@JsonProperty(value = "parameters")
private Object parameters;
/**
* The URI referencing the parameters. Use only one of Parameters or
* ParametersLink.
*/
@JsonProperty(value = "parametersLink")
private ParametersLink parametersLink;
/**
* The deployment mode. Possible values are Incremental and Complete.
* Possible values include: 'Incremental', 'Complete'.
*/
@JsonProperty(value = "mode")
private DeploymentMode mode;
/**
* The debug setting of the deployment.
*/
@JsonProperty(value = "debugSetting")
private DebugSetting debugSetting;
/**
* Get the provisioningState value.
*
* @return the provisioningState value
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* Get the correlationId value.
*
* @return the correlationId value
*/
public String correlationId() {
return this.correlationId;
}
/**
* Get the timestamp value.
*
* @return the timestamp value
*/
public DateTime timestamp() {
return this.timestamp;
}
/**
* Get the outputs value.
*
* @return the outputs value
*/
public Object outputs() {
return this.outputs;
}
/**
* Set the outputs value.
*
* @param outputs the outputs value to set
* @return the DeploymentPropertiesExtended object itself.
*/
public DeploymentPropertiesExtended withOutputs(Object outputs) {
this.outputs = outputs;
return this;
}
/**
* Get the providers value.
*
* @return the providers value
*/
public List providers() {
return this.providers;
}
/**
* Set the providers value.
*
* @param providers the providers value to set
* @return the DeploymentPropertiesExtended object itself.
*/
public DeploymentPropertiesExtended withProviders(List providers) {
this.providers = providers;
return this;
}
/**
* Get the dependencies value.
*
* @return the dependencies value
*/
public List dependencies() {
return this.dependencies;
}
/**
* Set the dependencies value.
*
* @param dependencies the dependencies value to set
* @return the DeploymentPropertiesExtended object itself.
*/
public DeploymentPropertiesExtended withDependencies(List dependencies) {
this.dependencies = dependencies;
return this;
}
/**
* Get the template value.
*
* @return the template value
*/
public Object template() {
return this.template;
}
/**
* Set the template value.
*
* @param template the template value to set
* @return the DeploymentPropertiesExtended object itself.
*/
public DeploymentPropertiesExtended withTemplate(Object template) {
this.template = template;
return this;
}
/**
* Get the templateLink value.
*
* @return the templateLink value
*/
public TemplateLink templateLink() {
return this.templateLink;
}
/**
* Set the templateLink value.
*
* @param templateLink the templateLink value to set
* @return the DeploymentPropertiesExtended object itself.
*/
public DeploymentPropertiesExtended withTemplateLink(TemplateLink templateLink) {
this.templateLink = templateLink;
return this;
}
/**
* Get the parameters value.
*
* @return the parameters value
*/
public Object parameters() {
return this.parameters;
}
/**
* Set the parameters value.
*
* @param parameters the parameters value to set
* @return the DeploymentPropertiesExtended object itself.
*/
public DeploymentPropertiesExtended withParameters(Object parameters) {
this.parameters = parameters;
return this;
}
/**
* Get the parametersLink value.
*
* @return the parametersLink value
*/
public ParametersLink parametersLink() {
return this.parametersLink;
}
/**
* Set the parametersLink value.
*
* @param parametersLink the parametersLink value to set
* @return the DeploymentPropertiesExtended object itself.
*/
public DeploymentPropertiesExtended withParametersLink(ParametersLink parametersLink) {
this.parametersLink = parametersLink;
return this;
}
/**
* Get the mode value.
*
* @return the mode value
*/
public DeploymentMode mode() {
return this.mode;
}
/**
* Set the mode value.
*
* @param mode the mode value to set
* @return the DeploymentPropertiesExtended object itself.
*/
public DeploymentPropertiesExtended withMode(DeploymentMode mode) {
this.mode = mode;
return this;
}
/**
* Get the debugSetting value.
*
* @return the debugSetting value
*/
public DebugSetting debugSetting() {
return this.debugSetting;
}
/**
* Set the debugSetting value.
*
* @param debugSetting the debugSetting value to set
* @return the DeploymentPropertiesExtended object itself.
*/
public DeploymentPropertiesExtended withDebugSetting(DebugSetting debugSetting) {
this.debugSetting = debugSetting;
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy