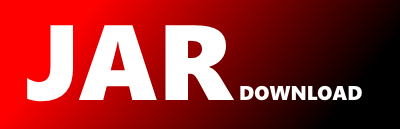
com.microsoft.azure.management.resources.DeploymentOperationProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-mgmt-resources Show documentation
Show all versions of azure-mgmt-resources Show documentation
This package contains Microsoft Azure Resource Management SDK.
/**
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for
* license information.
*
* Code generated by Microsoft (R) AutoRest Code Generator.
*/
package com.microsoft.azure.management.resources;
import org.joda.time.DateTime;
import com.fasterxml.jackson.annotation.JsonProperty;
/**
* Deployment operation properties.
*/
public class DeploymentOperationProperties {
/**
* The name of the current provisioning operation. Possible values include:
* 'NotSpecified', 'Create', 'Delete', 'Waiting',
* 'AzureAsyncOperationWaiting', 'ResourceCacheWaiting', 'Action', 'Read',
* 'EvaluateDeploymentOutput', 'DeploymentCleanup'.
*/
@JsonProperty(value = "provisioningOperation", access = JsonProperty.Access.WRITE_ONLY)
private ProvisioningOperation provisioningOperation;
/**
* The state of the provisioning.
*/
@JsonProperty(value = "provisioningState", access = JsonProperty.Access.WRITE_ONLY)
private String provisioningState;
/**
* The date and time of the operation.
*/
@JsonProperty(value = "timestamp", access = JsonProperty.Access.WRITE_ONLY)
private DateTime timestamp;
/**
* The duration of the operation.
*/
@JsonProperty(value = "duration", access = JsonProperty.Access.WRITE_ONLY)
private String duration;
/**
* Deployment operation service request id.
*/
@JsonProperty(value = "serviceRequestId", access = JsonProperty.Access.WRITE_ONLY)
private String serviceRequestId;
/**
* Operation status code from the resource provider. This property may not
* be set if a response has not yet been received.
*/
@JsonProperty(value = "statusCode", access = JsonProperty.Access.WRITE_ONLY)
private String statusCode;
/**
* Operation status message from the resource provider. This property is
* optional. It will only be provided if an error was received from the
* resource provider.
*/
@JsonProperty(value = "statusMessage", access = JsonProperty.Access.WRITE_ONLY)
private StatusMessage statusMessage;
/**
* The target resource.
*/
@JsonProperty(value = "targetResource", access = JsonProperty.Access.WRITE_ONLY)
private TargetResource targetResource;
/**
* The HTTP request message.
*/
@JsonProperty(value = "request", access = JsonProperty.Access.WRITE_ONLY)
private HttpMessage request;
/**
* The HTTP response message.
*/
@JsonProperty(value = "response", access = JsonProperty.Access.WRITE_ONLY)
private HttpMessage response;
/**
* Get the name of the current provisioning operation. Possible values include: 'NotSpecified', 'Create', 'Delete', 'Waiting', 'AzureAsyncOperationWaiting', 'ResourceCacheWaiting', 'Action', 'Read', 'EvaluateDeploymentOutput', 'DeploymentCleanup'.
*
* @return the provisioningOperation value
*/
public ProvisioningOperation provisioningOperation() {
return this.provisioningOperation;
}
/**
* Get the state of the provisioning.
*
* @return the provisioningState value
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* Get the date and time of the operation.
*
* @return the timestamp value
*/
public DateTime timestamp() {
return this.timestamp;
}
/**
* Get the duration of the operation.
*
* @return the duration value
*/
public String duration() {
return this.duration;
}
/**
* Get deployment operation service request id.
*
* @return the serviceRequestId value
*/
public String serviceRequestId() {
return this.serviceRequestId;
}
/**
* Get operation status code from the resource provider. This property may not be set if a response has not yet been received.
*
* @return the statusCode value
*/
public String statusCode() {
return this.statusCode;
}
/**
* Get operation status message from the resource provider. This property is optional. It will only be provided if an error was received from the resource provider.
*
* @return the statusMessage value
*/
public StatusMessage statusMessage() {
return this.statusMessage;
}
/**
* Get the target resource.
*
* @return the targetResource value
*/
public TargetResource targetResource() {
return this.targetResource;
}
/**
* Get the HTTP request message.
*
* @return the request value
*/
public HttpMessage request() {
return this.request;
}
/**
* Get the HTTP response message.
*
* @return the response value
*/
public HttpMessage response() {
return this.response;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy