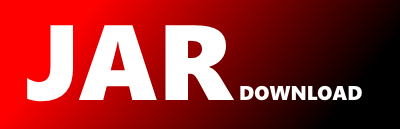
com.microsoft.azure.management.resources.implementation.ResourceManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-mgmt-resources Show documentation
Show all versions of azure-mgmt-resources Show documentation
This package contains Microsoft Azure Resource Management SDK.
/**
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for
* license information.
*/
package com.microsoft.azure.management.resources.implementation;
import com.microsoft.azure.AzureEnvironment;
import com.microsoft.azure.AzureResponseBuilder;
import com.microsoft.azure.credentials.AzureTokenCredentials;
import com.microsoft.azure.management.resources.Deployments;
import com.microsoft.azure.management.resources.Features;
import com.microsoft.azure.management.resources.GenericResources;
import com.microsoft.azure.management.resources.PolicyAssignments;
import com.microsoft.azure.management.resources.PolicyDefinitions;
import com.microsoft.azure.management.resources.Providers;
import com.microsoft.azure.management.resources.ResourceGroups;
import com.microsoft.azure.management.resources.Subscriptions;
import com.microsoft.azure.management.resources.Tenants;
import com.microsoft.azure.management.resources.fluentcore.arm.AzureConfigurable;
import com.microsoft.azure.management.resources.fluentcore.arm.implementation.AzureConfigurableImpl;
import com.microsoft.azure.management.resources.fluentcore.arm.implementation.ManagerBase;
import com.microsoft.azure.management.resources.fluentcore.model.HasInner;
import com.microsoft.azure.management.resources.fluentcore.utils.ProviderRegistrationInterceptor;
import com.microsoft.azure.management.resources.fluentcore.utils.ResourceManagerThrottlingInterceptor;
import com.microsoft.azure.serializer.AzureJacksonAdapter;
import com.microsoft.rest.RestClient;
/**
* Entry point to Azure resource management.
*/
public final class ResourceManager extends ManagerBase implements HasInner {
// The sdk clients
private final ResourceManagementClientImpl resourceManagementClient;
private final FeatureClientImpl featureClient;
private final PolicyClientImpl policyClient;
private final SubscriptionClientImpl subscriptionClient;
// The collections
private ResourceGroups resourceGroups;
private GenericResources genericResources;
private Deployments deployments;
private Features features;
private Providers providers;
private PolicyDefinitions policyDefinitions;
private PolicyAssignments policyAssignments;
private Subscriptions subscriptions;
private Tenants tenants;
/**
* Creates an instance of ResourceManager that exposes resource management API entry points.
*
* @param credentials the credentials to use
* @return the ResourceManager instance
*/
public static ResourceManager.Authenticated authenticate(AzureTokenCredentials credentials) {
return new AuthenticatedImpl(new RestClient.Builder()
.withBaseUrl(credentials.environment(), AzureEnvironment.Endpoint.RESOURCE_MANAGER)
.withCredentials(credentials)
.withSerializerAdapter(new AzureJacksonAdapter())
.withResponseBuilderFactory(new AzureResponseBuilder.Factory())
.withInterceptor(new ProviderRegistrationInterceptor(credentials))
.withInterceptor(new ResourceManagerThrottlingInterceptor())
.build());
}
/**
* Creates an instance of ResourceManager that exposes resource management API entry points.
*
* @param restClient the RestClient to be used for API calls
* @return the interface exposing resource management API entry points that work across subscriptions
*/
public static ResourceManager.Authenticated authenticate(RestClient restClient) {
return new AuthenticatedImpl(restClient);
}
/**
* Get a Configurable instance that can be used to create ResourceManager with optional configuration.
*
* @return the instance allowing configurations
*/
public static Configurable configure() {
return new ResourceManager.ConfigurableImpl();
}
/**
* The interface allowing configurations to be set.
*/
public interface Configurable extends AzureConfigurable {
/**
* Creates an instance of ResourceManager that exposes resource management API entry points.
*
* @param credentials the credentials to use
* @return the interface exposing resource management API entry points that work across subscriptions
*/
ResourceManager.Authenticated authenticate(AzureTokenCredentials credentials);
}
/**
* The implementation for Configurable interface.
*/
private static class ConfigurableImpl extends AzureConfigurableImpl implements Configurable {
public ResourceManager.Authenticated authenticate(AzureTokenCredentials credentials) {
return ResourceManager.authenticate(buildRestClient(credentials));
}
}
/**
* The interface exposing resource management API entry points that work across subscriptions.
*/
public interface Authenticated {
/**
* @return the entry point to tenant management API.
*/
Tenants tenants();
/**
* @return the entry point to subscription management API.
*/
Subscriptions subscriptions();
/**
* Specifies a subscription to expose resource management API entry points that work in a subscription.
*
* @param subscriptionId the subscription UUID
* @return the ResourceManager instance with entry points that work in a subscription
*/
ResourceManager withSubscription(String subscriptionId);
}
/**
* The implementation for Authenticated interface.
*/
private static final class AuthenticatedImpl implements Authenticated {
private RestClient restClient;
private SubscriptionClientImpl subscriptionClient;
// The subscription less collections
private Subscriptions subscriptions;
private Tenants tenants;
AuthenticatedImpl(RestClient restClient) {
this.restClient = restClient;
this.subscriptionClient = new SubscriptionClientImpl(restClient);
}
public Subscriptions subscriptions() {
if (subscriptions == null) {
subscriptions = new SubscriptionsImpl(subscriptionClient.subscriptions());
}
return subscriptions;
}
public Tenants tenants() {
if (tenants == null) {
tenants = new TenantsImpl(subscriptionClient.tenants());
}
return tenants;
}
@Override
public ResourceManager withSubscription(String subscriptionId) {
return new ResourceManager(restClient, subscriptionId);
}
}
private ResourceManager(RestClient restClient, String subscriptionId) {
super(null, subscriptionId);
super.setResourceManager(this);
this.resourceManagementClient = new ResourceManagementClientImpl(restClient);
this.resourceManagementClient.withSubscriptionId(subscriptionId);
this.featureClient = new FeatureClientImpl(restClient);
this.featureClient.withSubscriptionId(subscriptionId);
this.policyClient = new PolicyClientImpl(restClient);
this.policyClient.withSubscriptionId(subscriptionId);
this.subscriptionClient = new SubscriptionClientImpl(restClient);
}
/**
* @return the resource group management API entry point
*/
public ResourceGroups resourceGroups() {
if (resourceGroups == null) {
resourceGroups = new ResourceGroupsImpl(resourceManagementClient);
}
return resourceGroups;
}
/**
* @return the subscription management API entry point
*/
public Subscriptions subscriptions() {
if (subscriptions == null) {
subscriptions = new SubscriptionsImpl(subscriptionClient.subscriptions());
}
return subscriptions;
}
/**
* @return the tenant management API entry point
*/
public Tenants tenants() {
if (tenants == null) {
tenants = new TenantsImpl(subscriptionClient.tenants());
}
return tenants;
}
/**
* @return the generic resource management API entry point
*/
public GenericResources genericResources() {
if (genericResources == null) {
genericResources = new GenericResourcesImpl(this);
}
return genericResources;
}
/**
* @return the deployment management API entry point
*/
public Deployments deployments() {
if (deployments == null) {
deployments = new DeploymentsImpl(this);
}
return deployments;
}
/**
* @return the feature management API entry point
*/
public Features features() {
if (features == null) {
features = new FeaturesImpl(featureClient.features());
}
return features;
}
/**
* @return the resource provider management API entry point
*/
public Providers providers() {
if (providers == null) {
providers = new ProvidersImpl(resourceManagementClient.providers());
}
return providers;
}
/**
* @return the policy definition management API entry point
*/
public PolicyDefinitions policyDefinitions() {
if (policyDefinitions == null) {
policyDefinitions = new PolicyDefinitionsImpl(policyClient.policyDefinitions());
}
return policyDefinitions;
}
/**
* @return the policy assignment management API entry point
*/
public PolicyAssignments policyAssignments() {
if (policyAssignments == null) {
policyAssignments = new PolicyAssignmentsImpl(policyClient.policyAssignments());
}
return policyAssignments;
}
@Override
public ResourceManagementClientImpl inner() {
return this.resourceManagementClient;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy