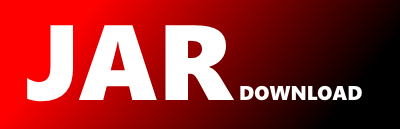
com.microsoft.azure.management.resources.implementation.SubscriptionImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-mgmt-resources Show documentation
Show all versions of azure-mgmt-resources Show documentation
This package contains Microsoft Azure Resource Management SDK.
/**
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for
* license information.
*/
package com.microsoft.azure.management.resources.implementation;
import com.microsoft.azure.Page;
import com.microsoft.azure.PagedList;
import com.microsoft.azure.management.resources.Location;
import com.microsoft.azure.management.resources.ManagedByTenant;
import com.microsoft.azure.management.resources.Subscription;
import com.microsoft.azure.management.resources.SubscriptionPolicies;
import com.microsoft.azure.management.resources.SubscriptionState;
import com.microsoft.azure.management.resources.fluentcore.arm.Region;
import com.microsoft.azure.management.resources.fluentcore.model.implementation.IndexableWrapperImpl;
import com.microsoft.azure.management.resources.fluentcore.utils.PagedListConverter;
import rx.Observable;
import java.util.List;
/**
* The implementation of {@link Subscription}.
*/
final class SubscriptionImpl extends
IndexableWrapperImpl
implements
Subscription {
private final SubscriptionsInner client;
SubscriptionImpl(SubscriptionInner innerModel, final SubscriptionsInner client) {
super(innerModel);
this.client = client;
}
@Override
public String subscriptionId() {
return this.inner().subscriptionId();
}
@Override
public String tenantId() {
return this.inner().tenantId();
}
@Override
public String displayName() {
return this.inner().displayName();
}
@Override
public SubscriptionState state() {
return this.inner().state();
}
@Override
public SubscriptionPolicies subscriptionPolicies() {
return this.inner().subscriptionPolicies();
}
@Override
public List managedByTenants() {
return this.inner().managedByTenants();
}
@Override
public PagedList listLocations() {
PagedListConverter converter = new PagedListConverter() {
@Override
public Observable typeConvertAsync(LocationInner locationInner) {
return Observable.just((Location) new LocationImpl(locationInner));
}
};
return converter.convert(toPagedList(client.listLocations(this.subscriptionId())));
}
@Override
public Location getLocationByRegion(Region region) {
if (region != null) {
PagedList locations = listLocations();
for (Location location : locations) {
if (region.equals(location.region())) {
return location;
}
}
}
return null;
}
private PagedList toPagedList(List list) {
PageImpl page = new PageImpl<>();
page.setItems(list);
page.setNextPageLink(null);
return new PagedList(page) {
@Override
public Page nextPage(String nextPageLink) {
return null;
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy