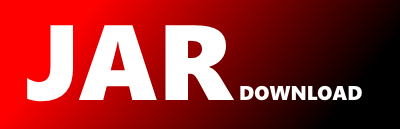
com.microsoft.azure.management.servicebus.implementation.ServiceBusChildResourcesImpl Maven / Gradle / Ivy
/**
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for
* license information.
*/
package com.microsoft.azure.management.servicebus.implementation;
import com.microsoft.azure.Page;
import com.microsoft.azure.PagedList;
import com.microsoft.azure.management.resources.fluentcore.arm.collection.SupportsGettingByNameAsync;
import com.microsoft.azure.management.resources.fluentcore.arm.collection.implementation.IndependentChildResourcesImpl;
import com.microsoft.azure.management.resources.fluentcore.arm.implementation.ManagerBase;
import com.microsoft.azure.management.resources.fluentcore.arm.models.HasResourceGroup;
import com.microsoft.azure.management.resources.fluentcore.arm.models.IndependentChildResource;
import com.microsoft.azure.management.resources.fluentcore.arm.models.Resource;
import com.microsoft.azure.management.resources.fluentcore.collection.SupportsDeletingByName;
import com.microsoft.azure.management.resources.fluentcore.collection.SupportsListing;
import com.microsoft.rest.ServiceResponse;
import rx.Observable;
import rx.functions.Func1;
import java.util.ArrayList;
import java.util.List;
/**
* Base class for Service Bus child entities.
* Note: When we refactor 'IndependentChildResourcesImpl', move features of this type
* to 'IndependentChildResourcesImpl' and remove this type.
*
* @param the model interface type
* @param the model interface implementation
* @param the inner model
* @param the inner collection
* @param the manager
* @param the parent model interface type
*/
abstract class ServiceBusChildResourcesImpl<
T extends IndependentChildResource,
ImplT extends T,
InnerT,
InnerCollectionT,
ManagerT extends ManagerBase,
ParentT extends Resource & HasResourceGroup>
extends IndependentChildResourcesImpl
implements SupportsGettingByNameAsync, SupportsListing, SupportsDeletingByName {
protected ServiceBusChildResourcesImpl(InnerCollectionT innerCollection, ManagerT manager) {
super(innerCollection, manager);
}
@Override
public Observable getByNameAsync(String name) {
return getInnerByNameAsync(name)
.map(new Func1() {
@Override
public T call(InnerT inner) {
return wrapModel(inner);
}
});
}
@Override
public T getByName(String name) {
return getByNameAsync(name).toBlocking().last();
}
@Override
public Observable listAsync() {
return this.listInnerAsync()
.flatMap(new Func1>, Observable>() {
@Override
public Observable call(ServiceResponse> r) {
return Observable.from(r.body().items()).map(new Func1() {
@Override
public T call(InnerT inner) {
return wrapModel(inner);
}
});
}
});
}
@Override
public PagedList list() {
return this.wrapList(this.listInner());
}
@Override
public void deleteByName(String name) {
deleteByNameAsync(name).await();
}
public Observable deleteByNameAsync(List names) {
List> items = new ArrayList<>();
for (final String name : names) {
items.add(this.deleteByNameAsync(name).toObservable().map(new Func1() {
@Override
public String call(String s) {
return name;
}
}));
}
return Observable.mergeDelayError(items);
}
protected abstract Observable getInnerByNameAsync(String name);
protected abstract Observable>> listInnerAsync();
protected abstract PagedList listInner();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy