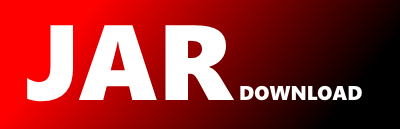
com.microsoft.azure.management.sql.RecommendedIndexOperations Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-mgmt-sql Show documentation
Show all versions of azure-mgmt-sql Show documentation
This package contains Microsoft Azure SDK for SQL Management module.
/**
*
* Copyright (c) Microsoft and contributors. All rights reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
*
* See the License for the specific language governing permissions and
* limitations under the License.
*
*/
// Warning: This code was generated by a tool.
//
// Changes to this file may cause incorrect behavior and will be lost if the
// code is regenerated.
package com.microsoft.azure.management.sql;
import com.microsoft.azure.management.sql.models.RecommendedIndexGetResponse;
import com.microsoft.azure.management.sql.models.RecommendedIndexUpdateParameters;
import com.microsoft.azure.management.sql.models.RecommendedIndexUpdateResponse;
import com.microsoft.windowsazure.exception.ServiceException;
import java.io.IOException;
import java.util.concurrent.Future;
/**
* Represents all the operations for managing recommended indexes on Azure SQL
* Databases. Contains operations to retrieve recommended index and update
* state.
*/
public interface RecommendedIndexOperations {
/**
* Returns details on recommended index.
*
* @param resourceGroupName Required. The name of the Resource Group.
* @param serverName Required. The name of the Azure SQL server.
* @param databaseName Required. The name of the Azure SQL database.
* @param schemaName Required. The name of the Azure SQL database schema.
* @param tableName Required. The name of the Azure SQL database table.
* @param indexName Required. The name of the Azure SQL database recommended
* index.
* @throws IOException Signals that an I/O exception of some sort has
* occurred. This class is the general class of exceptions produced by
* failed or interrupted I/O operations.
* @throws ServiceException Thrown if an unexpected response is found.
* @return Represents the response to a get recommended index request.
*/
RecommendedIndexGetResponse get(String resourceGroupName, String serverName, String databaseName, String schemaName, String tableName, String indexName) throws IOException, ServiceException;
/**
* Returns details on recommended index.
*
* @param resourceGroupName Required. The name of the Resource Group.
* @param serverName Required. The name of the Azure SQL server.
* @param databaseName Required. The name of the Azure SQL database.
* @param schemaName Required. The name of the Azure SQL database schema.
* @param tableName Required. The name of the Azure SQL database table.
* @param indexName Required. The name of the Azure SQL database recommended
* index.
* @return Represents the response to a get recommended index request.
*/
Future getAsync(String resourceGroupName, String serverName, String databaseName, String schemaName, String tableName, String indexName);
/**
* We execute or cancel index operations by updating index state. Allowed
* state transitions are :Active -> Pending - Start index
* creation processPending -> Active - Cancel index
* creationActive/Pending -> Ignored - Ignore index
* recommendation so it will no longer show in active
* recommendationsIgnored -> Active - Restore index
* recommendationSuccess -> Pending Revert - Revert index that
* has been createdPending Revert -> Revert Canceled - Cancel index
* revert operation
*
* @param resourceGroupName Required. The name of the Resource Group to
* which the Azure SQL Database Server belongs.
* @param serverName Required. The name of the Azure SQL Database Server on
* which the database is hosted.
* @param databaseName Required. The name of the Azure SQL Database.
* @param schemaName Required. The name of the Azure SQL Database schema.
* @param tableName Required. The name of the Azure SQL Database table.
* @param indexName Required. The name of the Azure SQL Database recommended
* index.
* @param parameters Required. The required parameters for updating index
* state.
* @throws IOException Signals that an I/O exception of some sort has
* occurred. This class is the general class of exceptions produced by
* failed or interrupted I/O operations.
* @throws ServiceException Thrown if an unexpected response is found.
* @return Represents the response to a get recommended index request.
*/
RecommendedIndexUpdateResponse update(String resourceGroupName, String serverName, String databaseName, String schemaName, String tableName, String indexName, RecommendedIndexUpdateParameters parameters) throws IOException, ServiceException;
/**
* We execute or cancel index operations by updating index state. Allowed
* state transitions are :Active -> Pending - Start index
* creation processPending -> Active - Cancel index
* creationActive/Pending -> Ignored - Ignore index
* recommendation so it will no longer show in active
* recommendationsIgnored -> Active - Restore index
* recommendationSuccess -> Pending Revert - Revert index that
* has been createdPending Revert -> Revert Canceled - Cancel index
* revert operation
*
* @param resourceGroupName Required. The name of the Resource Group to
* which the Azure SQL Database Server belongs.
* @param serverName Required. The name of the Azure SQL Database Server on
* which the database is hosted.
* @param databaseName Required. The name of the Azure SQL Database.
* @param schemaName Required. The name of the Azure SQL Database schema.
* @param tableName Required. The name of the Azure SQL Database table.
* @param indexName Required. The name of the Azure SQL Database recommended
* index.
* @param parameters Required. The required parameters for updating index
* state.
* @return Represents the response to a get recommended index request.
*/
Future updateAsync(String resourceGroupName, String serverName, String databaseName, String schemaName, String tableName, String indexName, RecommendedIndexUpdateParameters parameters);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy