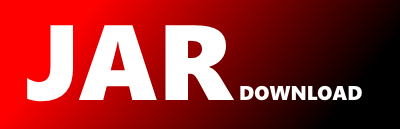
com.microsoft.azure.management.sql.ServerAdministratorOperationsImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-mgmt-sql Show documentation
Show all versions of azure-mgmt-sql Show documentation
This package contains Microsoft Azure SDK for SQL Management module.
/**
*
* Copyright (c) Microsoft and contributors. All rights reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
*
* See the License for the specific language governing permissions and
* limitations under the License.
*
*/
// Warning: This code was generated by a tool.
//
// Changes to this file may cause incorrect behavior and will be lost if the
// code is regenerated.
package com.microsoft.azure.management.sql;
import com.microsoft.azure.management.sql.models.ErrorResponse;
import com.microsoft.azure.management.sql.models.ServerAdministrator;
import com.microsoft.azure.management.sql.models.ServerAdministratorCreateOrUpdateParameters;
import com.microsoft.azure.management.sql.models.ServerAdministratorCreateOrUpdateResponse;
import com.microsoft.azure.management.sql.models.ServerAdministratorDeleteResponse;
import com.microsoft.azure.management.sql.models.ServerAdministratorGetResponse;
import com.microsoft.azure.management.sql.models.ServerAdministratorListResponse;
import com.microsoft.azure.management.sql.models.ServerAdministratorProperties;
import com.microsoft.windowsazure.core.OperationStatus;
import com.microsoft.windowsazure.core.ServiceOperations;
import com.microsoft.windowsazure.core.pipeline.apache.CustomHttpDelete;
import com.microsoft.windowsazure.core.utils.CollectionStringBuilder;
import com.microsoft.windowsazure.exception.ServiceException;
import com.microsoft.windowsazure.tracing.ClientRequestTrackingHandler;
import com.microsoft.windowsazure.tracing.CloudTracing;
import org.apache.commons.io.IOUtils;
import org.apache.http.HttpResponse;
import org.apache.http.HttpStatus;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.client.methods.HttpPut;
import org.apache.http.entity.StringEntity;
import org.codehaus.jackson.JsonNode;
import org.codehaus.jackson.map.ObjectMapper;
import org.codehaus.jackson.node.ArrayNode;
import org.codehaus.jackson.node.NullNode;
import org.codehaus.jackson.node.ObjectNode;
import javax.xml.bind.DatatypeConverter;
import java.io.IOException;
import java.io.InputStream;
import java.io.StringWriter;
import java.net.URLEncoder;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
import java.util.concurrent.Callable;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.Future;
/**
* Represents all the operations for operating on Azure SQL Server Active
* Directory Administrators. Contains operations to: Create, Retrieve, Update,
* and Delete Azure SQL Server Active Directory Administrators.
*/
public class ServerAdministratorOperationsImpl implements ServiceOperations, ServerAdministratorOperations {
/**
* Initializes a new instance of the ServerAdministratorOperationsImpl class.
*
* @param client Reference to the service client.
*/
ServerAdministratorOperationsImpl(SqlManagementClientImpl client) {
this.client = client;
}
private SqlManagementClientImpl client;
/**
* Gets a reference to the
* microsoft.azure.management.sql.SqlManagementClientImpl.
* @return The Client value.
*/
public SqlManagementClientImpl getClient() {
return this.client;
}
/**
* Begins creating a new Azure SQL Server Active Directory Administrator or
* updating an existing Azure SQL Server Active Directory Administrator. To
* determine the status of the operation call
* GetServerAdministratorOperationStatus.
*
* @param resourceGroupName Required. The name of the Resource Group to
* which the server belongs.
* @param serverName Required. The name of the Azure SQL Server to which the
* Azure SQL Server Active Directory administrator belongs
* @param administratorName Required. The name of the Azure SQL Server
* Active Directory Administrator.
* @param parameters Required. The required parameters for createing or
* updating an Active Directory Administrator.
* @return Response for long running Azure SQL Server Active Directory
* Administrator operations.
*/
@Override
public Future beginCreateOrUpdateAsync(final String resourceGroupName, final String serverName, final String administratorName, final ServerAdministratorCreateOrUpdateParameters parameters) {
return this.getClient().getExecutorService().submit(new Callable() {
@Override
public ServerAdministratorCreateOrUpdateResponse call() throws Exception {
return beginCreateOrUpdate(resourceGroupName, serverName, administratorName, parameters);
}
});
}
/**
* Begins creating a new Azure SQL Server Active Directory Administrator or
* updating an existing Azure SQL Server Active Directory Administrator. To
* determine the status of the operation call
* GetServerAdministratorOperationStatus.
*
* @param resourceGroupName Required. The name of the Resource Group to
* which the server belongs.
* @param serverName Required. The name of the Azure SQL Server to which the
* Azure SQL Server Active Directory administrator belongs
* @param administratorName Required. The name of the Azure SQL Server
* Active Directory Administrator.
* @param parameters Required. The required parameters for createing or
* updating an Active Directory Administrator.
* @throws IOException Signals that an I/O exception of some sort has
* occurred. This class is the general class of exceptions produced by
* failed or interrupted I/O operations.
* @throws ServiceException Thrown if an unexpected response is found.
* @return Response for long running Azure SQL Server Active Directory
* Administrator operations.
*/
@Override
public ServerAdministratorCreateOrUpdateResponse beginCreateOrUpdate(String resourceGroupName, String serverName, String administratorName, ServerAdministratorCreateOrUpdateParameters parameters) throws IOException, ServiceException {
// Validate
if (resourceGroupName == null) {
throw new NullPointerException("resourceGroupName");
}
if (serverName == null) {
throw new NullPointerException("serverName");
}
if (administratorName == null) {
throw new NullPointerException("administratorName");
}
if (parameters == null) {
throw new NullPointerException("parameters");
}
if (parameters.getProperties() == null) {
throw new NullPointerException("parameters.Properties");
}
if (parameters.getProperties().getAdministratorType() == null) {
throw new NullPointerException("parameters.Properties.AdministratorType");
}
if (parameters.getProperties().getLogin() == null) {
throw new NullPointerException("parameters.Properties.Login");
}
if (parameters.getProperties().getSid() == null) {
throw new NullPointerException("parameters.Properties.Sid");
}
if (parameters.getProperties().getTenantId() == null) {
throw new NullPointerException("parameters.Properties.TenantId");
}
// Tracing
boolean shouldTrace = CloudTracing.getIsEnabled();
String invocationId = null;
if (shouldTrace) {
invocationId = Long.toString(CloudTracing.getNextInvocationId());
HashMap tracingParameters = new HashMap();
tracingParameters.put("resourceGroupName", resourceGroupName);
tracingParameters.put("serverName", serverName);
tracingParameters.put("administratorName", administratorName);
tracingParameters.put("parameters", parameters);
CloudTracing.enter(invocationId, this, "beginCreateOrUpdateAsync", tracingParameters);
}
// Construct URL
String url = "";
url = url + "/subscriptions/";
if (this.getClient().getCredentials().getSubscriptionId() != null) {
url = url + URLEncoder.encode(this.getClient().getCredentials().getSubscriptionId(), "UTF-8");
}
url = url + "/resourceGroups/";
url = url + URLEncoder.encode(resourceGroupName, "UTF-8");
url = url + "/providers/";
url = url + "Microsoft.Sql";
url = url + "/servers/";
url = url + URLEncoder.encode(serverName, "UTF-8");
url = url + "/administrators/";
url = url + URLEncoder.encode(administratorName, "UTF-8");
ArrayList queryParameters = new ArrayList();
queryParameters.add("api-version=" + "2014-04-01");
if (queryParameters.size() > 0) {
url = url + "?" + CollectionStringBuilder.join(queryParameters, "&");
}
String baseUrl = this.getClient().getBaseUri().toString();
// Trim '/' character from the end of baseUrl and beginning of url.
if (baseUrl.charAt(baseUrl.length() - 1) == '/') {
baseUrl = baseUrl.substring(0, (baseUrl.length() - 1) + 0);
}
if (url.charAt(0) == '/') {
url = url.substring(1);
}
url = baseUrl + "/" + url;
url = url.replace(" ", "%20");
// Create HTTP transport objects
HttpPut httpRequest = new HttpPut(url);
// Set Headers
httpRequest.setHeader("Content-Type", "application/json; charset=utf-8");
// Serialize Request
String requestContent = null;
JsonNode requestDoc = null;
ObjectMapper objectMapper = new ObjectMapper();
ObjectNode serverAdministratorCreateOrUpdateParametersValue = objectMapper.createObjectNode();
requestDoc = serverAdministratorCreateOrUpdateParametersValue;
ObjectNode propertiesValue = objectMapper.createObjectNode();
((ObjectNode) serverAdministratorCreateOrUpdateParametersValue).put("properties", propertiesValue);
((ObjectNode) propertiesValue).put("login", parameters.getProperties().getLogin());
((ObjectNode) propertiesValue).put("sid", parameters.getProperties().getSid());
((ObjectNode) propertiesValue).put("administratorType", parameters.getProperties().getAdministratorType());
((ObjectNode) propertiesValue).put("tenantId", parameters.getProperties().getTenantId());
StringWriter stringWriter = new StringWriter();
objectMapper.writeValue(stringWriter, requestDoc);
requestContent = stringWriter.toString();
StringEntity entity = new StringEntity(requestContent);
httpRequest.setEntity(entity);
httpRequest.setHeader("Content-Type", "application/json; charset=utf-8");
// Send Request
HttpResponse httpResponse = null;
try {
if (shouldTrace) {
CloudTracing.sendRequest(invocationId, httpRequest);
}
httpResponse = this.getClient().getHttpClient().execute(httpRequest);
if (shouldTrace) {
CloudTracing.receiveResponse(invocationId, httpResponse);
}
int statusCode = httpResponse.getStatusLine().getStatusCode();
if (statusCode != HttpStatus.SC_OK && statusCode != HttpStatus.SC_CREATED && statusCode != HttpStatus.SC_ACCEPTED) {
ServiceException ex = ServiceException.createFromJson(httpRequest, requestContent, httpResponse, httpResponse.getEntity());
if (shouldTrace) {
CloudTracing.error(invocationId, ex);
}
throw ex;
}
// Create Result
ServerAdministratorCreateOrUpdateResponse result = null;
// Deserialize Response
if (statusCode == HttpStatus.SC_OK || statusCode == HttpStatus.SC_CREATED || statusCode == HttpStatus.SC_ACCEPTED) {
InputStream responseContent = httpResponse.getEntity().getContent();
result = new ServerAdministratorCreateOrUpdateResponse();
JsonNode responseDoc = null;
String responseDocContent = IOUtils.toString(responseContent);
if (responseDocContent == null == false && responseDocContent.length() > 0) {
responseDoc = objectMapper.readTree(responseDocContent);
}
if (responseDoc != null && responseDoc instanceof NullNode == false) {
ErrorResponse errorInstance = new ErrorResponse();
result.setError(errorInstance);
JsonNode codeValue = responseDoc.get("code");
if (codeValue != null && codeValue instanceof NullNode == false) {
String codeInstance;
codeInstance = codeValue.getTextValue();
errorInstance.setCode(codeInstance);
}
JsonNode messageValue = responseDoc.get("message");
if (messageValue != null && messageValue instanceof NullNode == false) {
String messageInstance;
messageInstance = messageValue.getTextValue();
errorInstance.setMessage(messageInstance);
}
JsonNode targetValue = responseDoc.get("target");
if (targetValue != null && targetValue instanceof NullNode == false) {
String targetInstance;
targetInstance = targetValue.getTextValue();
errorInstance.setTarget(targetInstance);
}
ServerAdministrator serverAdministratorInstance = new ServerAdministrator();
result.setServerAdministrator(serverAdministratorInstance);
JsonNode propertiesValue2 = responseDoc.get("properties");
if (propertiesValue2 != null && propertiesValue2 instanceof NullNode == false) {
ServerAdministratorProperties propertiesInstance = new ServerAdministratorProperties();
serverAdministratorInstance.setProperties(propertiesInstance);
JsonNode administratorTypeValue = propertiesValue2.get("administratorType");
if (administratorTypeValue != null && administratorTypeValue instanceof NullNode == false) {
String administratorTypeInstance;
administratorTypeInstance = administratorTypeValue.getTextValue();
propertiesInstance.setAdministratorType(administratorTypeInstance);
}
JsonNode loginValue = propertiesValue2.get("login");
if (loginValue != null && loginValue instanceof NullNode == false) {
String loginInstance;
loginInstance = loginValue.getTextValue();
propertiesInstance.setLogin(loginInstance);
}
JsonNode sidValue = propertiesValue2.get("sid");
if (sidValue != null && sidValue instanceof NullNode == false) {
String sidInstance;
sidInstance = sidValue.getTextValue();
propertiesInstance.setSid(sidInstance);
}
JsonNode tenantIdValue = propertiesValue2.get("tenantId");
if (tenantIdValue != null && tenantIdValue instanceof NullNode == false) {
String tenantIdInstance;
tenantIdInstance = tenantIdValue.getTextValue();
propertiesInstance.setTenantId(tenantIdInstance);
}
}
JsonNode idValue = responseDoc.get("id");
if (idValue != null && idValue instanceof NullNode == false) {
String idInstance;
idInstance = idValue.getTextValue();
serverAdministratorInstance.setId(idInstance);
}
JsonNode nameValue = responseDoc.get("name");
if (nameValue != null && nameValue instanceof NullNode == false) {
String nameInstance;
nameInstance = nameValue.getTextValue();
serverAdministratorInstance.setName(nameInstance);
}
JsonNode typeValue = responseDoc.get("type");
if (typeValue != null && typeValue instanceof NullNode == false) {
String typeInstance;
typeInstance = typeValue.getTextValue();
serverAdministratorInstance.setType(typeInstance);
}
JsonNode locationValue = responseDoc.get("location");
if (locationValue != null && locationValue instanceof NullNode == false) {
String locationInstance;
locationInstance = locationValue.getTextValue();
serverAdministratorInstance.setLocation(locationInstance);
}
JsonNode tagsSequenceElement = ((JsonNode) responseDoc.get("tags"));
if (tagsSequenceElement != null && tagsSequenceElement instanceof NullNode == false) {
Iterator> itr = tagsSequenceElement.getFields();
while (itr.hasNext()) {
Map.Entry property = itr.next();
String tagsKey = property.getKey();
String tagsValue = property.getValue().getTextValue();
serverAdministratorInstance.getTags().put(tagsKey, tagsValue);
}
}
}
}
result.setStatusCode(statusCode);
if (httpResponse.getHeaders("Location").length > 0) {
result.setOperationStatusLink(httpResponse.getFirstHeader("Location").getValue());
}
if (httpResponse.getHeaders("Retry-After").length > 0) {
result.setRetryAfter(DatatypeConverter.parseInt(httpResponse.getFirstHeader("Retry-After").getValue()));
}
if (httpResponse.getHeaders("x-ms-request-id").length > 0) {
result.setRequestId(httpResponse.getFirstHeader("x-ms-request-id").getValue());
}
if (statusCode == HttpStatus.SC_CREATED) {
result.setStatus(OperationStatus.Succeeded);
}
if (statusCode == HttpStatus.SC_OK) {
result.setStatus(OperationStatus.Succeeded);
}
if (shouldTrace) {
CloudTracing.exit(invocationId, result);
}
return result;
} finally {
if (httpResponse != null && httpResponse.getEntity() != null) {
httpResponse.getEntity().getContent().close();
}
}
}
/**
* Begins deleting an existing Azure SQL Server Active Directory
* Administrator.To determine the status of the operation call
* GetServerAdministratorDeleteOperationStatus.
*
* @param resourceGroupName Required. The name of the Resource Group to
* which the server belongs.
* @param serverName Required. The name of the Azure SQL Server to which the
* Azure SQL Server Active Directory administrator belongs
* @param administratorName Required. The name of the Azure SQL Server
* Active Directory Administrator.
* @return Response for long running Azure SQL Server Active Directory
* administrator delete operations.
*/
@Override
public Future beginDeleteAsync(final String resourceGroupName, final String serverName, final String administratorName) {
return this.getClient().getExecutorService().submit(new Callable() {
@Override
public ServerAdministratorDeleteResponse call() throws Exception {
return beginDelete(resourceGroupName, serverName, administratorName);
}
});
}
/**
* Begins deleting an existing Azure SQL Server Active Directory
* Administrator.To determine the status of the operation call
* GetServerAdministratorDeleteOperationStatus.
*
* @param resourceGroupName Required. The name of the Resource Group to
* which the server belongs.
* @param serverName Required. The name of the Azure SQL Server to which the
* Azure SQL Server Active Directory administrator belongs
* @param administratorName Required. The name of the Azure SQL Server
* Active Directory Administrator.
* @throws IOException Signals that an I/O exception of some sort has
* occurred. This class is the general class of exceptions produced by
* failed or interrupted I/O operations.
* @throws ServiceException Thrown if an unexpected response is found.
* @return Response for long running Azure SQL Server Active Directory
* administrator delete operations.
*/
@Override
public ServerAdministratorDeleteResponse beginDelete(String resourceGroupName, String serverName, String administratorName) throws IOException, ServiceException {
// Validate
if (resourceGroupName == null) {
throw new NullPointerException("resourceGroupName");
}
if (serverName == null) {
throw new NullPointerException("serverName");
}
if (administratorName == null) {
throw new NullPointerException("administratorName");
}
// Tracing
boolean shouldTrace = CloudTracing.getIsEnabled();
String invocationId = null;
if (shouldTrace) {
invocationId = Long.toString(CloudTracing.getNextInvocationId());
HashMap tracingParameters = new HashMap();
tracingParameters.put("resourceGroupName", resourceGroupName);
tracingParameters.put("serverName", serverName);
tracingParameters.put("administratorName", administratorName);
CloudTracing.enter(invocationId, this, "beginDeleteAsync", tracingParameters);
}
// Construct URL
String url = "";
url = url + "/subscriptions/";
if (this.getClient().getCredentials().getSubscriptionId() != null) {
url = url + URLEncoder.encode(this.getClient().getCredentials().getSubscriptionId(), "UTF-8");
}
url = url + "/resourceGroups/";
url = url + URLEncoder.encode(resourceGroupName, "UTF-8");
url = url + "/providers/";
url = url + "Microsoft.Sql";
url = url + "/servers/";
url = url + URLEncoder.encode(serverName, "UTF-8");
url = url + "/administrators/";
url = url + URLEncoder.encode(administratorName, "UTF-8");
ArrayList queryParameters = new ArrayList();
queryParameters.add("api-version=" + "2014-04-01");
if (queryParameters.size() > 0) {
url = url + "?" + CollectionStringBuilder.join(queryParameters, "&");
}
String baseUrl = this.getClient().getBaseUri().toString();
// Trim '/' character from the end of baseUrl and beginning of url.
if (baseUrl.charAt(baseUrl.length() - 1) == '/') {
baseUrl = baseUrl.substring(0, (baseUrl.length() - 1) + 0);
}
if (url.charAt(0) == '/') {
url = url.substring(1);
}
url = baseUrl + "/" + url;
url = url.replace(" ", "%20");
// Create HTTP transport objects
CustomHttpDelete httpRequest = new CustomHttpDelete(url);
// Set Headers
httpRequest.setHeader("Content-Type", "application/json; charset=utf-8");
// Send Request
HttpResponse httpResponse = null;
try {
if (shouldTrace) {
CloudTracing.sendRequest(invocationId, httpRequest);
}
httpResponse = this.getClient().getHttpClient().execute(httpRequest);
if (shouldTrace) {
CloudTracing.receiveResponse(invocationId, httpResponse);
}
int statusCode = httpResponse.getStatusLine().getStatusCode();
if (statusCode != HttpStatus.SC_OK && statusCode != HttpStatus.SC_ACCEPTED && statusCode != HttpStatus.SC_NO_CONTENT) {
ServiceException ex = ServiceException.createFromJson(httpRequest, null, httpResponse, httpResponse.getEntity());
if (shouldTrace) {
CloudTracing.error(invocationId, ex);
}
throw ex;
}
// Create Result
ServerAdministratorDeleteResponse result = null;
// Deserialize Response
if (statusCode == HttpStatus.SC_OK || statusCode == HttpStatus.SC_ACCEPTED || statusCode == HttpStatus.SC_NO_CONTENT) {
InputStream responseContent = httpResponse.getEntity().getContent();
result = new ServerAdministratorDeleteResponse();
ObjectMapper objectMapper = new ObjectMapper();
JsonNode responseDoc = null;
String responseDocContent = IOUtils.toString(responseContent);
if (responseDocContent == null == false && responseDocContent.length() > 0) {
responseDoc = objectMapper.readTree(responseDocContent);
}
if (responseDoc != null && responseDoc instanceof NullNode == false) {
ErrorResponse errorInstance = new ErrorResponse();
result.setError(errorInstance);
JsonNode codeValue = responseDoc.get("code");
if (codeValue != null && codeValue instanceof NullNode == false) {
String codeInstance;
codeInstance = codeValue.getTextValue();
errorInstance.setCode(codeInstance);
}
JsonNode messageValue = responseDoc.get("message");
if (messageValue != null && messageValue instanceof NullNode == false) {
String messageInstance;
messageInstance = messageValue.getTextValue();
errorInstance.setMessage(messageInstance);
}
JsonNode targetValue = responseDoc.get("target");
if (targetValue != null && targetValue instanceof NullNode == false) {
String targetInstance;
targetInstance = targetValue.getTextValue();
errorInstance.setTarget(targetInstance);
}
}
}
result.setStatusCode(statusCode);
if (httpResponse.getHeaders("Location").length > 0) {
result.setOperationStatusLink(httpResponse.getFirstHeader("Location").getValue());
}
if (httpResponse.getHeaders("Retry-After").length > 0) {
result.setRetryAfter(DatatypeConverter.parseInt(httpResponse.getFirstHeader("Retry-After").getValue()));
}
if (httpResponse.getHeaders("x-ms-request-id").length > 0) {
result.setRequestId(httpResponse.getFirstHeader("x-ms-request-id").getValue());
}
if (statusCode == HttpStatus.SC_OK) {
result.setStatus(OperationStatus.Succeeded);
}
if (statusCode == HttpStatus.SC_NO_CONTENT) {
result.setStatus(OperationStatus.Succeeded);
}
if (shouldTrace) {
CloudTracing.exit(invocationId, result);
}
return result;
} finally {
if (httpResponse != null && httpResponse.getEntity() != null) {
httpResponse.getEntity().getContent().close();
}
}
}
/**
* Creates a new Azure SQL Server Active Directory Administrator or updates
* an existing Azure SQL Server Active Directory Administrator.
*
* @param resourceGroupName Required. The name of the Resource Group to
* which the server belongs.
* @param serverName Required. The name of the Azure SQL Server on which the
* Azure SQL Server Active Directory Administrator is hosted.
* @param administratorName Required. The name of the Azure SQL Server
* Active Directory Administrator to be operated on (Updated or created).
* @param parameters Required. The required parameters for creating or
* updating a Server Administrator.
* @return Response for long running Azure SQL Server Active Directory
* Administrator operations.
*/
@Override
public Future createOrUpdateAsync(final String resourceGroupName, final String serverName, final String administratorName, final ServerAdministratorCreateOrUpdateParameters parameters) {
return this.getClient().getExecutorService().submit(new Callable() {
@Override
public ServerAdministratorCreateOrUpdateResponse call() throws Exception {
return createOrUpdate(resourceGroupName, serverName, administratorName, parameters);
}
});
}
/**
* Creates a new Azure SQL Server Active Directory Administrator or updates
* an existing Azure SQL Server Active Directory Administrator.
*
* @param resourceGroupName Required. The name of the Resource Group to
* which the server belongs.
* @param serverName Required. The name of the Azure SQL Server on which the
* Azure SQL Server Active Directory Administrator is hosted.
* @param administratorName Required. The name of the Azure SQL Server
* Active Directory Administrator to be operated on (Updated or created).
* @param parameters Required. The required parameters for creating or
* updating a Server Administrator.
* @throws InterruptedException Thrown when a thread is waiting, sleeping,
* or otherwise occupied, and the thread is interrupted, either before or
* during the activity. Occasionally a method may wish to test whether the
* current thread has been interrupted, and if so, to immediately throw
* this exception. The following code can be used to achieve this effect:
* @throws ExecutionException Thrown when attempting to retrieve the result
* of a task that aborted by throwing an exception. This exception can be
* inspected using the Throwable.getCause() method.
* @throws IOException Thrown if there was an error setting up tracing for
* the request.
* @throws ServiceException Thrown if an unexpected response is found.
* @return Response for long running Azure SQL Server Active Directory
* Administrator operations.
*/
@Override
public ServerAdministratorCreateOrUpdateResponse createOrUpdate(String resourceGroupName, String serverName, String administratorName, ServerAdministratorCreateOrUpdateParameters parameters) throws InterruptedException, ExecutionException, IOException, ServiceException {
SqlManagementClient client2 = this.getClient();
boolean shouldTrace = CloudTracing.getIsEnabled();
String invocationId = null;
if (shouldTrace) {
invocationId = Long.toString(CloudTracing.getNextInvocationId());
HashMap tracingParameters = new HashMap();
tracingParameters.put("resourceGroupName", resourceGroupName);
tracingParameters.put("serverName", serverName);
tracingParameters.put("administratorName", administratorName);
tracingParameters.put("parameters", parameters);
CloudTracing.enter(invocationId, this, "createOrUpdateAsync", tracingParameters);
}
try {
if (shouldTrace) {
client2 = this.getClient().withRequestFilterLast(new ClientRequestTrackingHandler(invocationId)).withResponseFilterLast(new ClientRequestTrackingHandler(invocationId));
}
ServerAdministratorCreateOrUpdateResponse response = client2.getServerAdministratorsOperations().beginCreateOrUpdateAsync(resourceGroupName, serverName, administratorName, parameters).get();
if (response.getStatus() == OperationStatus.Succeeded) {
return response;
}
ServerAdministratorCreateOrUpdateResponse result = client2.getServerAdministratorsOperations().getServerAdministratorOperationStatusAsync(response.getOperationStatusLink()).get();
int delayInSeconds = response.getRetryAfter();
if (delayInSeconds == 0) {
delayInSeconds = 30;
}
if (client2.getLongRunningOperationInitialTimeout() >= 0) {
delayInSeconds = client2.getLongRunningOperationInitialTimeout();
}
while (result.getStatus() != null && result.getStatus().equals(OperationStatus.InProgress)) {
Thread.sleep(delayInSeconds * 1000);
result = client2.getServerAdministratorsOperations().getServerAdministratorOperationStatusAsync(response.getOperationStatusLink()).get();
delayInSeconds = result.getRetryAfter();
if (delayInSeconds == 0) {
delayInSeconds = 15;
}
if (client2.getLongRunningOperationRetryTimeout() >= 0) {
delayInSeconds = client2.getLongRunningOperationRetryTimeout();
}
}
if (shouldTrace) {
CloudTracing.exit(invocationId, result);
}
return result;
} finally {
if (client2 != null && shouldTrace) {
client2.close();
}
}
}
/**
* Deletes an existing Azure SQL Server Active Directory Administrator.
*
* @param resourceGroupName Required. The name of the Resource Group to
* which the server belongs.
* @param serverName Required. The name of the Azure SQL Server to which the
* Azure SQL Server Active Directory administrator belongs
* @param administratorName Required. The name of the Azure SQL Server
* Active Directory Administrator to be operated on (Updated or created).
* @return Response for long running Azure SQL Server Active Directory
* administrator delete operations.
*/
@Override
public Future deleteAsync(final String resourceGroupName, final String serverName, final String administratorName) {
return this.getClient().getExecutorService().submit(new Callable() {
@Override
public ServerAdministratorDeleteResponse call() throws Exception {
return delete(resourceGroupName, serverName, administratorName);
}
});
}
/**
* Deletes an existing Azure SQL Server Active Directory Administrator.
*
* @param resourceGroupName Required. The name of the Resource Group to
* which the server belongs.
* @param serverName Required. The name of the Azure SQL Server to which the
* Azure SQL Server Active Directory administrator belongs
* @param administratorName Required. The name of the Azure SQL Server
* Active Directory Administrator to be operated on (Updated or created).
* @throws IOException Signals that an I/O exception of some sort has
* occurred. This class is the general class of exceptions produced by
* failed or interrupted I/O operations.
* @throws ServiceException Thrown if an unexpected response is found.
* @throws InterruptedException Thrown when a thread is waiting, sleeping,
* or otherwise occupied, and the thread is interrupted, either before or
* during the activity. Occasionally a method may wish to test whether the
* current thread has been interrupted, and if so, to immediately throw
* this exception. The following code can be used to achieve this effect:
* @throws ExecutionException Thrown when attempting to retrieve the result
* of a task that aborted by throwing an exception. This exception can be
* inspected using the Throwable.getCause() method.
* @return Response for long running Azure SQL Server Active Directory
* administrator delete operations.
*/
@Override
public ServerAdministratorDeleteResponse delete(String resourceGroupName, String serverName, String administratorName) throws IOException, ServiceException, InterruptedException, ExecutionException {
SqlManagementClient client2 = this.getClient();
boolean shouldTrace = CloudTracing.getIsEnabled();
String invocationId = null;
if (shouldTrace) {
invocationId = Long.toString(CloudTracing.getNextInvocationId());
HashMap tracingParameters = new HashMap();
tracingParameters.put("resourceGroupName", resourceGroupName);
tracingParameters.put("serverName", serverName);
tracingParameters.put("administratorName", administratorName);
CloudTracing.enter(invocationId, this, "deleteAsync", tracingParameters);
}
try {
if (shouldTrace) {
client2 = this.getClient().withRequestFilterLast(new ClientRequestTrackingHandler(invocationId)).withResponseFilterLast(new ClientRequestTrackingHandler(invocationId));
}
ServerAdministratorDeleteResponse response = client2.getServerAdministratorsOperations().beginDeleteAsync(resourceGroupName, serverName, administratorName).get();
if (response.getStatus() == OperationStatus.Succeeded) {
return response;
}
ServerAdministratorDeleteResponse result = client2.getServerAdministratorsOperations().getServerAdministratorDeleteOperationStatusAsync(response.getOperationStatusLink()).get();
int delayInSeconds = response.getRetryAfter();
if (delayInSeconds == 0) {
delayInSeconds = 30;
}
if (client2.getLongRunningOperationInitialTimeout() >= 0) {
delayInSeconds = client2.getLongRunningOperationInitialTimeout();
}
while (result.getStatus() != null && result.getStatus().equals(OperationStatus.InProgress)) {
Thread.sleep(delayInSeconds * 1000);
result = client2.getServerAdministratorsOperations().getServerAdministratorDeleteOperationStatusAsync(response.getOperationStatusLink()).get();
delayInSeconds = result.getRetryAfter();
if (delayInSeconds == 0) {
delayInSeconds = 15;
}
if (client2.getLongRunningOperationRetryTimeout() >= 0) {
delayInSeconds = client2.getLongRunningOperationRetryTimeout();
}
}
if (shouldTrace) {
CloudTracing.exit(invocationId, result);
}
return result;
} finally {
if (client2 != null && shouldTrace) {
client2.close();
}
}
}
/**
* Returns an Azure SQL Server Administrator.
*
* @param resourceGroupName Required. The name of the Resource Group to
* which the server belongs.
* @param serverName Required. The name of the Azure SQL Server to which the
* Azure SQL Server Active Directory administrator belongs.
* @param administratorName Required. The name of the Azure SQL Server
* Active Directory Administrator.
* @return Represents the response to a Get Azure SQL Server Active
* Directory Administrators request.
*/
@Override
public Future getAsync(final String resourceGroupName, final String serverName, final String administratorName) {
return this.getClient().getExecutorService().submit(new Callable() {
@Override
public ServerAdministratorGetResponse call() throws Exception {
return get(resourceGroupName, serverName, administratorName);
}
});
}
/**
* Returns an Azure SQL Server Administrator.
*
* @param resourceGroupName Required. The name of the Resource Group to
* which the server belongs.
* @param serverName Required. The name of the Azure SQL Server to which the
* Azure SQL Server Active Directory administrator belongs.
* @param administratorName Required. The name of the Azure SQL Server
* Active Directory Administrator.
* @throws IOException Signals that an I/O exception of some sort has
* occurred. This class is the general class of exceptions produced by
* failed or interrupted I/O operations.
* @throws ServiceException Thrown if an unexpected response is found.
* @return Represents the response to a Get Azure SQL Server Active
* Directory Administrators request.
*/
@Override
public ServerAdministratorGetResponse get(String resourceGroupName, String serverName, String administratorName) throws IOException, ServiceException {
// Validate
if (resourceGroupName == null) {
throw new NullPointerException("resourceGroupName");
}
if (serverName == null) {
throw new NullPointerException("serverName");
}
if (administratorName == null) {
throw new NullPointerException("administratorName");
}
// Tracing
boolean shouldTrace = CloudTracing.getIsEnabled();
String invocationId = null;
if (shouldTrace) {
invocationId = Long.toString(CloudTracing.getNextInvocationId());
HashMap tracingParameters = new HashMap();
tracingParameters.put("resourceGroupName", resourceGroupName);
tracingParameters.put("serverName", serverName);
tracingParameters.put("administratorName", administratorName);
CloudTracing.enter(invocationId, this, "getAsync", tracingParameters);
}
// Construct URL
String url = "";
url = url + "/subscriptions/";
if (this.getClient().getCredentials().getSubscriptionId() != null) {
url = url + URLEncoder.encode(this.getClient().getCredentials().getSubscriptionId(), "UTF-8");
}
url = url + "/resourceGroups/";
url = url + URLEncoder.encode(resourceGroupName, "UTF-8");
url = url + "/providers/";
url = url + "Microsoft.Sql";
url = url + "/servers/";
url = url + URLEncoder.encode(serverName, "UTF-8");
url = url + "/administrators/";
url = url + URLEncoder.encode(administratorName, "UTF-8");
ArrayList queryParameters = new ArrayList();
queryParameters.add("api-version=" + "2014-04-01");
if (queryParameters.size() > 0) {
url = url + "?" + CollectionStringBuilder.join(queryParameters, "&");
}
String baseUrl = this.getClient().getBaseUri().toString();
// Trim '/' character from the end of baseUrl and beginning of url.
if (baseUrl.charAt(baseUrl.length() - 1) == '/') {
baseUrl = baseUrl.substring(0, (baseUrl.length() - 1) + 0);
}
if (url.charAt(0) == '/') {
url = url.substring(1);
}
url = baseUrl + "/" + url;
url = url.replace(" ", "%20");
// Create HTTP transport objects
HttpGet httpRequest = new HttpGet(url);
// Set Headers
// Send Request
HttpResponse httpResponse = null;
try {
if (shouldTrace) {
CloudTracing.sendRequest(invocationId, httpRequest);
}
httpResponse = this.getClient().getHttpClient().execute(httpRequest);
if (shouldTrace) {
CloudTracing.receiveResponse(invocationId, httpResponse);
}
int statusCode = httpResponse.getStatusLine().getStatusCode();
if (statusCode != HttpStatus.SC_OK) {
ServiceException ex = ServiceException.createFromJson(httpRequest, null, httpResponse, httpResponse.getEntity());
if (shouldTrace) {
CloudTracing.error(invocationId, ex);
}
throw ex;
}
// Create Result
ServerAdministratorGetResponse result = null;
// Deserialize Response
if (statusCode == HttpStatus.SC_OK) {
InputStream responseContent = httpResponse.getEntity().getContent();
result = new ServerAdministratorGetResponse();
ObjectMapper objectMapper = new ObjectMapper();
JsonNode responseDoc = null;
String responseDocContent = IOUtils.toString(responseContent);
if (responseDocContent == null == false && responseDocContent.length() > 0) {
responseDoc = objectMapper.readTree(responseDocContent);
}
if (responseDoc != null && responseDoc instanceof NullNode == false) {
ServerAdministrator administratorInstance = new ServerAdministrator();
result.setAdministrator(administratorInstance);
JsonNode propertiesValue = responseDoc.get("properties");
if (propertiesValue != null && propertiesValue instanceof NullNode == false) {
ServerAdministratorProperties propertiesInstance = new ServerAdministratorProperties();
administratorInstance.setProperties(propertiesInstance);
JsonNode administratorTypeValue = propertiesValue.get("administratorType");
if (administratorTypeValue != null && administratorTypeValue instanceof NullNode == false) {
String administratorTypeInstance;
administratorTypeInstance = administratorTypeValue.getTextValue();
propertiesInstance.setAdministratorType(administratorTypeInstance);
}
JsonNode loginValue = propertiesValue.get("login");
if (loginValue != null && loginValue instanceof NullNode == false) {
String loginInstance;
loginInstance = loginValue.getTextValue();
propertiesInstance.setLogin(loginInstance);
}
JsonNode sidValue = propertiesValue.get("sid");
if (sidValue != null && sidValue instanceof NullNode == false) {
String sidInstance;
sidInstance = sidValue.getTextValue();
propertiesInstance.setSid(sidInstance);
}
JsonNode tenantIdValue = propertiesValue.get("tenantId");
if (tenantIdValue != null && tenantIdValue instanceof NullNode == false) {
String tenantIdInstance;
tenantIdInstance = tenantIdValue.getTextValue();
propertiesInstance.setTenantId(tenantIdInstance);
}
}
JsonNode idValue = responseDoc.get("id");
if (idValue != null && idValue instanceof NullNode == false) {
String idInstance;
idInstance = idValue.getTextValue();
administratorInstance.setId(idInstance);
}
JsonNode nameValue = responseDoc.get("name");
if (nameValue != null && nameValue instanceof NullNode == false) {
String nameInstance;
nameInstance = nameValue.getTextValue();
administratorInstance.setName(nameInstance);
}
JsonNode typeValue = responseDoc.get("type");
if (typeValue != null && typeValue instanceof NullNode == false) {
String typeInstance;
typeInstance = typeValue.getTextValue();
administratorInstance.setType(typeInstance);
}
JsonNode locationValue = responseDoc.get("location");
if (locationValue != null && locationValue instanceof NullNode == false) {
String locationInstance;
locationInstance = locationValue.getTextValue();
administratorInstance.setLocation(locationInstance);
}
JsonNode tagsSequenceElement = ((JsonNode) responseDoc.get("tags"));
if (tagsSequenceElement != null && tagsSequenceElement instanceof NullNode == false) {
Iterator> itr = tagsSequenceElement.getFields();
while (itr.hasNext()) {
Map.Entry property = itr.next();
String tagsKey = property.getKey();
String tagsValue = property.getValue().getTextValue();
administratorInstance.getTags().put(tagsKey, tagsValue);
}
}
}
}
result.setStatusCode(statusCode);
if (httpResponse.getHeaders("x-ms-request-id").length > 0) {
result.setRequestId(httpResponse.getFirstHeader("x-ms-request-id").getValue());
}
if (shouldTrace) {
CloudTracing.exit(invocationId, result);
}
return result;
} finally {
if (httpResponse != null && httpResponse.getEntity() != null) {
httpResponse.getEntity().getContent().close();
}
}
}
/**
* Gets the status of an Azure SQL Server Active Directory Administrator
* delete operation.
*
* @param operationStatusLink Required. Location value returned by the Begin
* operation
* @return Response for long running Azure SQL Server Active Directory
* administrator delete operations.
*/
@Override
public Future getServerAdministratorDeleteOperationStatusAsync(final String operationStatusLink) {
return this.getClient().getExecutorService().submit(new Callable() {
@Override
public ServerAdministratorDeleteResponse call() throws Exception {
return getServerAdministratorDeleteOperationStatus(operationStatusLink);
}
});
}
/**
* Gets the status of an Azure SQL Server Active Directory Administrator
* delete operation.
*
* @param operationStatusLink Required. Location value returned by the Begin
* operation
* @throws IOException Signals that an I/O exception of some sort has
* occurred. This class is the general class of exceptions produced by
* failed or interrupted I/O operations.
* @throws ServiceException Thrown if an unexpected response is found.
* @return Response for long running Azure SQL Server Active Directory
* administrator delete operations.
*/
@Override
public ServerAdministratorDeleteResponse getServerAdministratorDeleteOperationStatus(String operationStatusLink) throws IOException, ServiceException {
// Validate
if (operationStatusLink == null) {
throw new NullPointerException("operationStatusLink");
}
// Tracing
boolean shouldTrace = CloudTracing.getIsEnabled();
String invocationId = null;
if (shouldTrace) {
invocationId = Long.toString(CloudTracing.getNextInvocationId());
HashMap tracingParameters = new HashMap();
tracingParameters.put("operationStatusLink", operationStatusLink);
CloudTracing.enter(invocationId, this, "getServerAdministratorDeleteOperationStatusAsync", tracingParameters);
}
// Construct URL
String url = "";
url = url + operationStatusLink;
url = url.replace(" ", "%20");
// Create HTTP transport objects
HttpGet httpRequest = new HttpGet(url);
// Set Headers
// Send Request
HttpResponse httpResponse = null;
try {
if (shouldTrace) {
CloudTracing.sendRequest(invocationId, httpRequest);
}
httpResponse = this.getClient().getHttpClient().execute(httpRequest);
if (shouldTrace) {
CloudTracing.receiveResponse(invocationId, httpResponse);
}
int statusCode = httpResponse.getStatusLine().getStatusCode();
if (statusCode != HttpStatus.SC_OK && statusCode != HttpStatus.SC_ACCEPTED && statusCode != HttpStatus.SC_NO_CONTENT) {
ServiceException ex = ServiceException.createFromJson(httpRequest, null, httpResponse, httpResponse.getEntity());
if (shouldTrace) {
CloudTracing.error(invocationId, ex);
}
throw ex;
}
// Create Result
ServerAdministratorDeleteResponse result = null;
// Deserialize Response
if (statusCode == HttpStatus.SC_OK || statusCode == HttpStatus.SC_ACCEPTED || statusCode == HttpStatus.SC_NO_CONTENT) {
InputStream responseContent = httpResponse.getEntity().getContent();
result = new ServerAdministratorDeleteResponse();
ObjectMapper objectMapper = new ObjectMapper();
JsonNode responseDoc = null;
String responseDocContent = IOUtils.toString(responseContent);
if (responseDocContent == null == false && responseDocContent.length() > 0) {
responseDoc = objectMapper.readTree(responseDocContent);
}
if (responseDoc != null && responseDoc instanceof NullNode == false) {
ErrorResponse errorInstance = new ErrorResponse();
result.setError(errorInstance);
JsonNode codeValue = responseDoc.get("code");
if (codeValue != null && codeValue instanceof NullNode == false) {
String codeInstance;
codeInstance = codeValue.getTextValue();
errorInstance.setCode(codeInstance);
}
JsonNode messageValue = responseDoc.get("message");
if (messageValue != null && messageValue instanceof NullNode == false) {
String messageInstance;
messageInstance = messageValue.getTextValue();
errorInstance.setMessage(messageInstance);
}
JsonNode targetValue = responseDoc.get("target");
if (targetValue != null && targetValue instanceof NullNode == false) {
String targetInstance;
targetInstance = targetValue.getTextValue();
errorInstance.setTarget(targetInstance);
}
}
}
result.setStatusCode(statusCode);
if (httpResponse.getHeaders("x-ms-request-id").length > 0) {
result.setRequestId(httpResponse.getFirstHeader("x-ms-request-id").getValue());
}
if (statusCode == HttpStatus.SC_OK) {
result.setStatus(OperationStatus.Succeeded);
}
if (statusCode == HttpStatus.SC_NO_CONTENT) {
result.setStatus(OperationStatus.Succeeded);
}
if (shouldTrace) {
CloudTracing.exit(invocationId, result);
}
return result;
} finally {
if (httpResponse != null && httpResponse.getEntity() != null) {
httpResponse.getEntity().getContent().close();
}
}
}
/**
* Gets the status of an Azure SQL Server Active Directory Administrator
* create or update operation.
*
* @param operationStatusLink Required. Location value returned by the Begin
* operation
* @return Response for long running Azure SQL Server Active Directory
* Administrator operations.
*/
@Override
public Future getServerAdministratorOperationStatusAsync(final String operationStatusLink) {
return this.getClient().getExecutorService().submit(new Callable() {
@Override
public ServerAdministratorCreateOrUpdateResponse call() throws Exception {
return getServerAdministratorOperationStatus(operationStatusLink);
}
});
}
/**
* Gets the status of an Azure SQL Server Active Directory Administrator
* create or update operation.
*
* @param operationStatusLink Required. Location value returned by the Begin
* operation
* @throws IOException Signals that an I/O exception of some sort has
* occurred. This class is the general class of exceptions produced by
* failed or interrupted I/O operations.
* @throws ServiceException Thrown if an unexpected response is found.
* @return Response for long running Azure SQL Server Active Directory
* Administrator operations.
*/
@Override
public ServerAdministratorCreateOrUpdateResponse getServerAdministratorOperationStatus(String operationStatusLink) throws IOException, ServiceException {
// Validate
if (operationStatusLink == null) {
throw new NullPointerException("operationStatusLink");
}
// Tracing
boolean shouldTrace = CloudTracing.getIsEnabled();
String invocationId = null;
if (shouldTrace) {
invocationId = Long.toString(CloudTracing.getNextInvocationId());
HashMap tracingParameters = new HashMap();
tracingParameters.put("operationStatusLink", operationStatusLink);
CloudTracing.enter(invocationId, this, "getServerAdministratorOperationStatusAsync", tracingParameters);
}
// Construct URL
String url = "";
url = url + operationStatusLink;
url = url.replace(" ", "%20");
// Create HTTP transport objects
HttpGet httpRequest = new HttpGet(url);
// Set Headers
// Send Request
HttpResponse httpResponse = null;
try {
if (shouldTrace) {
CloudTracing.sendRequest(invocationId, httpRequest);
}
httpResponse = this.getClient().getHttpClient().execute(httpRequest);
if (shouldTrace) {
CloudTracing.receiveResponse(invocationId, httpResponse);
}
int statusCode = httpResponse.getStatusLine().getStatusCode();
if (statusCode != HttpStatus.SC_OK && statusCode != HttpStatus.SC_CREATED && statusCode != HttpStatus.SC_ACCEPTED) {
ServiceException ex = ServiceException.createFromJson(httpRequest, null, httpResponse, httpResponse.getEntity());
if (shouldTrace) {
CloudTracing.error(invocationId, ex);
}
throw ex;
}
// Create Result
ServerAdministratorCreateOrUpdateResponse result = null;
// Deserialize Response
if (statusCode == HttpStatus.SC_OK || statusCode == HttpStatus.SC_CREATED || statusCode == HttpStatus.SC_ACCEPTED) {
InputStream responseContent = httpResponse.getEntity().getContent();
result = new ServerAdministratorCreateOrUpdateResponse();
ObjectMapper objectMapper = new ObjectMapper();
JsonNode responseDoc = null;
String responseDocContent = IOUtils.toString(responseContent);
if (responseDocContent == null == false && responseDocContent.length() > 0) {
responseDoc = objectMapper.readTree(responseDocContent);
}
if (responseDoc != null && responseDoc instanceof NullNode == false) {
ErrorResponse errorInstance = new ErrorResponse();
result.setError(errorInstance);
JsonNode codeValue = responseDoc.get("code");
if (codeValue != null && codeValue instanceof NullNode == false) {
String codeInstance;
codeInstance = codeValue.getTextValue();
errorInstance.setCode(codeInstance);
}
JsonNode messageValue = responseDoc.get("message");
if (messageValue != null && messageValue instanceof NullNode == false) {
String messageInstance;
messageInstance = messageValue.getTextValue();
errorInstance.setMessage(messageInstance);
}
JsonNode targetValue = responseDoc.get("target");
if (targetValue != null && targetValue instanceof NullNode == false) {
String targetInstance;
targetInstance = targetValue.getTextValue();
errorInstance.setTarget(targetInstance);
}
ServerAdministrator serverAdministratorInstance = new ServerAdministrator();
result.setServerAdministrator(serverAdministratorInstance);
JsonNode propertiesValue = responseDoc.get("properties");
if (propertiesValue != null && propertiesValue instanceof NullNode == false) {
ServerAdministratorProperties propertiesInstance = new ServerAdministratorProperties();
serverAdministratorInstance.setProperties(propertiesInstance);
JsonNode administratorTypeValue = propertiesValue.get("administratorType");
if (administratorTypeValue != null && administratorTypeValue instanceof NullNode == false) {
String administratorTypeInstance;
administratorTypeInstance = administratorTypeValue.getTextValue();
propertiesInstance.setAdministratorType(administratorTypeInstance);
}
JsonNode loginValue = propertiesValue.get("login");
if (loginValue != null && loginValue instanceof NullNode == false) {
String loginInstance;
loginInstance = loginValue.getTextValue();
propertiesInstance.setLogin(loginInstance);
}
JsonNode sidValue = propertiesValue.get("sid");
if (sidValue != null && sidValue instanceof NullNode == false) {
String sidInstance;
sidInstance = sidValue.getTextValue();
propertiesInstance.setSid(sidInstance);
}
JsonNode tenantIdValue = propertiesValue.get("tenantId");
if (tenantIdValue != null && tenantIdValue instanceof NullNode == false) {
String tenantIdInstance;
tenantIdInstance = tenantIdValue.getTextValue();
propertiesInstance.setTenantId(tenantIdInstance);
}
}
JsonNode idValue = responseDoc.get("id");
if (idValue != null && idValue instanceof NullNode == false) {
String idInstance;
idInstance = idValue.getTextValue();
serverAdministratorInstance.setId(idInstance);
}
JsonNode nameValue = responseDoc.get("name");
if (nameValue != null && nameValue instanceof NullNode == false) {
String nameInstance;
nameInstance = nameValue.getTextValue();
serverAdministratorInstance.setName(nameInstance);
}
JsonNode typeValue = responseDoc.get("type");
if (typeValue != null && typeValue instanceof NullNode == false) {
String typeInstance;
typeInstance = typeValue.getTextValue();
serverAdministratorInstance.setType(typeInstance);
}
JsonNode locationValue = responseDoc.get("location");
if (locationValue != null && locationValue instanceof NullNode == false) {
String locationInstance;
locationInstance = locationValue.getTextValue();
serverAdministratorInstance.setLocation(locationInstance);
}
JsonNode tagsSequenceElement = ((JsonNode) responseDoc.get("tags"));
if (tagsSequenceElement != null && tagsSequenceElement instanceof NullNode == false) {
Iterator> itr = tagsSequenceElement.getFields();
while (itr.hasNext()) {
Map.Entry property = itr.next();
String tagsKey = property.getKey();
String tagsValue = property.getValue().getTextValue();
serverAdministratorInstance.getTags().put(tagsKey, tagsValue);
}
}
}
}
result.setStatusCode(statusCode);
if (httpResponse.getHeaders("x-ms-request-id").length > 0) {
result.setRequestId(httpResponse.getFirstHeader("x-ms-request-id").getValue());
}
if (statusCode == HttpStatus.SC_CREATED) {
result.setStatus(OperationStatus.Succeeded);
}
if (statusCode == HttpStatus.SC_OK) {
result.setStatus(OperationStatus.Succeeded);
}
if (shouldTrace) {
CloudTracing.exit(invocationId, result);
}
return result;
} finally {
if (httpResponse != null && httpResponse.getEntity() != null) {
httpResponse.getEntity().getContent().close();
}
}
}
/**
* Returns a list of Azure SQL Server Administrators.
*
* @param resourceGroupName Required. The name of the Resource Group to
* which the server belongs.
* @param serverName Required. The name of the Azure SQL Server to which the
* Azure SQL Server Active Directory administrators belongs.
* @return Represents the response to a List Azure SQL Active Directory
* Administrators request.
*/
@Override
public Future listAsync(final String resourceGroupName, final String serverName) {
return this.getClient().getExecutorService().submit(new Callable() {
@Override
public ServerAdministratorListResponse call() throws Exception {
return list(resourceGroupName, serverName);
}
});
}
/**
* Returns a list of Azure SQL Server Administrators.
*
* @param resourceGroupName Required. The name of the Resource Group to
* which the server belongs.
* @param serverName Required. The name of the Azure SQL Server to which the
* Azure SQL Server Active Directory administrators belongs.
* @throws IOException Signals that an I/O exception of some sort has
* occurred. This class is the general class of exceptions produced by
* failed or interrupted I/O operations.
* @throws ServiceException Thrown if an unexpected response is found.
* @return Represents the response to a List Azure SQL Active Directory
* Administrators request.
*/
@Override
public ServerAdministratorListResponse list(String resourceGroupName, String serverName) throws IOException, ServiceException {
// Validate
if (resourceGroupName == null) {
throw new NullPointerException("resourceGroupName");
}
if (serverName == null) {
throw new NullPointerException("serverName");
}
// Tracing
boolean shouldTrace = CloudTracing.getIsEnabled();
String invocationId = null;
if (shouldTrace) {
invocationId = Long.toString(CloudTracing.getNextInvocationId());
HashMap tracingParameters = new HashMap();
tracingParameters.put("resourceGroupName", resourceGroupName);
tracingParameters.put("serverName", serverName);
CloudTracing.enter(invocationId, this, "listAsync", tracingParameters);
}
// Construct URL
String url = "";
url = url + "/subscriptions/";
if (this.getClient().getCredentials().getSubscriptionId() != null) {
url = url + URLEncoder.encode(this.getClient().getCredentials().getSubscriptionId(), "UTF-8");
}
url = url + "/resourceGroups/";
url = url + URLEncoder.encode(resourceGroupName, "UTF-8");
url = url + "/providers/";
url = url + "Microsoft.Sql";
url = url + "/servers/";
url = url + URLEncoder.encode(serverName, "UTF-8");
url = url + "/administrators";
ArrayList queryParameters = new ArrayList();
queryParameters.add("api-version=" + "2014-04-01");
if (queryParameters.size() > 0) {
url = url + "?" + CollectionStringBuilder.join(queryParameters, "&");
}
String baseUrl = this.getClient().getBaseUri().toString();
// Trim '/' character from the end of baseUrl and beginning of url.
if (baseUrl.charAt(baseUrl.length() - 1) == '/') {
baseUrl = baseUrl.substring(0, (baseUrl.length() - 1) + 0);
}
if (url.charAt(0) == '/') {
url = url.substring(1);
}
url = baseUrl + "/" + url;
url = url.replace(" ", "%20");
// Create HTTP transport objects
HttpGet httpRequest = new HttpGet(url);
// Set Headers
// Send Request
HttpResponse httpResponse = null;
try {
if (shouldTrace) {
CloudTracing.sendRequest(invocationId, httpRequest);
}
httpResponse = this.getClient().getHttpClient().execute(httpRequest);
if (shouldTrace) {
CloudTracing.receiveResponse(invocationId, httpResponse);
}
int statusCode = httpResponse.getStatusLine().getStatusCode();
if (statusCode != HttpStatus.SC_OK) {
ServiceException ex = ServiceException.createFromJson(httpRequest, null, httpResponse, httpResponse.getEntity());
if (shouldTrace) {
CloudTracing.error(invocationId, ex);
}
throw ex;
}
// Create Result
ServerAdministratorListResponse result = null;
// Deserialize Response
if (statusCode == HttpStatus.SC_OK) {
InputStream responseContent = httpResponse.getEntity().getContent();
result = new ServerAdministratorListResponse();
ObjectMapper objectMapper = new ObjectMapper();
JsonNode responseDoc = null;
String responseDocContent = IOUtils.toString(responseContent);
if (responseDocContent == null == false && responseDocContent.length() > 0) {
responseDoc = objectMapper.readTree(responseDocContent);
}
if (responseDoc != null && responseDoc instanceof NullNode == false) {
JsonNode valueArray = responseDoc.get("value");
if (valueArray != null && valueArray instanceof NullNode == false) {
for (JsonNode valueValue : ((ArrayNode) valueArray)) {
ServerAdministrator serverAdministratorInstance = new ServerAdministrator();
result.getAdministrators().add(serverAdministratorInstance);
JsonNode propertiesValue = valueValue.get("properties");
if (propertiesValue != null && propertiesValue instanceof NullNode == false) {
ServerAdministratorProperties propertiesInstance = new ServerAdministratorProperties();
serverAdministratorInstance.setProperties(propertiesInstance);
JsonNode administratorTypeValue = propertiesValue.get("administratorType");
if (administratorTypeValue != null && administratorTypeValue instanceof NullNode == false) {
String administratorTypeInstance;
administratorTypeInstance = administratorTypeValue.getTextValue();
propertiesInstance.setAdministratorType(administratorTypeInstance);
}
JsonNode loginValue = propertiesValue.get("login");
if (loginValue != null && loginValue instanceof NullNode == false) {
String loginInstance;
loginInstance = loginValue.getTextValue();
propertiesInstance.setLogin(loginInstance);
}
JsonNode sidValue = propertiesValue.get("sid");
if (sidValue != null && sidValue instanceof NullNode == false) {
String sidInstance;
sidInstance = sidValue.getTextValue();
propertiesInstance.setSid(sidInstance);
}
JsonNode tenantIdValue = propertiesValue.get("tenantId");
if (tenantIdValue != null && tenantIdValue instanceof NullNode == false) {
String tenantIdInstance;
tenantIdInstance = tenantIdValue.getTextValue();
propertiesInstance.setTenantId(tenantIdInstance);
}
}
JsonNode idValue = valueValue.get("id");
if (idValue != null && idValue instanceof NullNode == false) {
String idInstance;
idInstance = idValue.getTextValue();
serverAdministratorInstance.setId(idInstance);
}
JsonNode nameValue = valueValue.get("name");
if (nameValue != null && nameValue instanceof NullNode == false) {
String nameInstance;
nameInstance = nameValue.getTextValue();
serverAdministratorInstance.setName(nameInstance);
}
JsonNode typeValue = valueValue.get("type");
if (typeValue != null && typeValue instanceof NullNode == false) {
String typeInstance;
typeInstance = typeValue.getTextValue();
serverAdministratorInstance.setType(typeInstance);
}
JsonNode locationValue = valueValue.get("location");
if (locationValue != null && locationValue instanceof NullNode == false) {
String locationInstance;
locationInstance = locationValue.getTextValue();
serverAdministratorInstance.setLocation(locationInstance);
}
JsonNode tagsSequenceElement = ((JsonNode) valueValue.get("tags"));
if (tagsSequenceElement != null && tagsSequenceElement instanceof NullNode == false) {
Iterator> itr = tagsSequenceElement.getFields();
while (itr.hasNext()) {
Map.Entry property = itr.next();
String tagsKey = property.getKey();
String tagsValue = property.getValue().getTextValue();
serverAdministratorInstance.getTags().put(tagsKey, tagsValue);
}
}
}
}
}
}
result.setStatusCode(statusCode);
if (httpResponse.getHeaders("x-ms-request-id").length > 0) {
result.setRequestId(httpResponse.getFirstHeader("x-ms-request-id").getValue());
}
if (shouldTrace) {
CloudTracing.exit(invocationId, result);
}
return result;
} finally {
if (httpResponse != null && httpResponse.getEntity() != null) {
httpResponse.getEntity().getContent().close();
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy