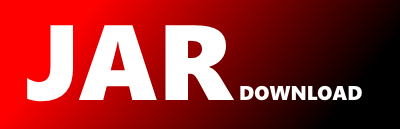
com.microsoft.azure.management.sql.ServerOperationsImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-mgmt-sql Show documentation
Show all versions of azure-mgmt-sql Show documentation
This package contains Microsoft Azure SDK for SQL Management module.
/**
*
* Copyright (c) Microsoft and contributors. All rights reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
*
* See the License for the specific language governing permissions and
* limitations under the License.
*
*/
// Warning: This code was generated by a tool.
//
// Changes to this file may cause incorrect behavior and will be lost if the
// code is regenerated.
package com.microsoft.azure.management.sql;
import com.microsoft.azure.management.sql.models.Server;
import com.microsoft.azure.management.sql.models.ServerCreateOrUpdateParameters;
import com.microsoft.azure.management.sql.models.ServerGetResponse;
import com.microsoft.azure.management.sql.models.ServerListResponse;
import com.microsoft.azure.management.sql.models.ServerMetric;
import com.microsoft.azure.management.sql.models.ServerMetricListResponse;
import com.microsoft.azure.management.sql.models.ServerProperties;
import com.microsoft.windowsazure.core.OperationResponse;
import com.microsoft.windowsazure.core.ServiceOperations;
import com.microsoft.windowsazure.core.pipeline.apache.CustomHttpDelete;
import com.microsoft.windowsazure.core.utils.CollectionStringBuilder;
import com.microsoft.windowsazure.exception.ServiceException;
import com.microsoft.windowsazure.tracing.CloudTracing;
import org.apache.commons.io.IOUtils;
import org.apache.http.HttpResponse;
import org.apache.http.HttpStatus;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.client.methods.HttpPut;
import org.apache.http.entity.StringEntity;
import org.codehaus.jackson.JsonNode;
import org.codehaus.jackson.map.ObjectMapper;
import org.codehaus.jackson.node.ArrayNode;
import org.codehaus.jackson.node.NullNode;
import org.codehaus.jackson.node.ObjectNode;
import javax.xml.bind.DatatypeConverter;
import java.io.IOException;
import java.io.InputStream;
import java.io.StringWriter;
import java.net.URLEncoder;
import java.util.ArrayList;
import java.util.Calendar;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
import java.util.concurrent.Callable;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.Future;
/**
* Represents all the operations for operating on Azure SQL Database Servers.
* Contains operations to: Create, Retrieve, Update, and Delete servers.
*/
public class ServerOperationsImpl implements ServiceOperations, ServerOperations {
/**
* Initializes a new instance of the ServerOperationsImpl class.
*
* @param client Reference to the service client.
*/
ServerOperationsImpl(SqlManagementClientImpl client) {
this.client = client;
}
private SqlManagementClientImpl client;
/**
* Gets a reference to the
* microsoft.azure.management.sql.SqlManagementClientImpl.
* @return The Client value.
*/
public SqlManagementClientImpl getClient() {
return this.client;
}
/**
* Creates a new Azure SQL Database server.
*
* @param resourceGroupName Required. The name of the Resource Group to
* which the server belongs.
* @param serverName Required. The name of the Azure SQL Database Server on
* which the database is hosted.
* @param parameters Required. The required parameters for createing or
* updating a database.
* @return Represents the response to a Get Azure Sql Database Server
* request.
*/
@Override
public Future createOrUpdateAsync(final String resourceGroupName, final String serverName, final ServerCreateOrUpdateParameters parameters) {
return this.getClient().getExecutorService().submit(new Callable() {
@Override
public ServerGetResponse call() throws Exception {
return createOrUpdate(resourceGroupName, serverName, parameters);
}
});
}
/**
* Creates a new Azure SQL Database server.
*
* @param resourceGroupName Required. The name of the Resource Group to
* which the server belongs.
* @param serverName Required. The name of the Azure SQL Database Server on
* which the database is hosted.
* @param parameters Required. The required parameters for createing or
* updating a database.
* @throws InterruptedException Thrown when a thread is waiting, sleeping,
* or otherwise occupied, and the thread is interrupted, either before or
* during the activity. Occasionally a method may wish to test whether the
* current thread has been interrupted, and if so, to immediately throw
* this exception. The following code can be used to achieve this effect:
* @throws ExecutionException Thrown when attempting to retrieve the result
* of a task that aborted by throwing an exception. This exception can be
* inspected using the Throwable.getCause() method.
* @throws IOException Thrown if there was an error setting up tracing for
* the request.
* @throws ServiceException Thrown if an unexpected response is found.
* @return Represents the response to a Get Azure Sql Database Server
* request.
*/
@Override
public ServerGetResponse createOrUpdate(String resourceGroupName, String serverName, ServerCreateOrUpdateParameters parameters) throws InterruptedException, ExecutionException, IOException, ServiceException {
// Validate
if (resourceGroupName == null) {
throw new NullPointerException("resourceGroupName");
}
if (serverName == null) {
throw new NullPointerException("serverName");
}
if (parameters == null) {
throw new NullPointerException("parameters");
}
if (parameters.getLocation() == null) {
throw new NullPointerException("parameters.Location");
}
if (parameters.getProperties() == null) {
throw new NullPointerException("parameters.Properties");
}
// Tracing
boolean shouldTrace = CloudTracing.getIsEnabled();
String invocationId = null;
if (shouldTrace) {
invocationId = Long.toString(CloudTracing.getNextInvocationId());
HashMap tracingParameters = new HashMap();
tracingParameters.put("resourceGroupName", resourceGroupName);
tracingParameters.put("serverName", serverName);
tracingParameters.put("parameters", parameters);
CloudTracing.enter(invocationId, this, "createOrUpdateAsync", tracingParameters);
}
// Construct URL
String url = "";
url = url + "/subscriptions/";
if (this.getClient().getCredentials().getSubscriptionId() != null) {
url = url + URLEncoder.encode(this.getClient().getCredentials().getSubscriptionId(), "UTF-8");
}
url = url + "/resourceGroups/";
url = url + URLEncoder.encode(resourceGroupName, "UTF-8");
url = url + "/providers/";
url = url + "Microsoft.Sql";
url = url + "/servers/";
url = url + URLEncoder.encode(serverName, "UTF-8");
ArrayList queryParameters = new ArrayList();
queryParameters.add("api-version=" + "2014-04-01");
if (queryParameters.size() > 0) {
url = url + "?" + CollectionStringBuilder.join(queryParameters, "&");
}
String baseUrl = this.getClient().getBaseUri().toString();
// Trim '/' character from the end of baseUrl and beginning of url.
if (baseUrl.charAt(baseUrl.length() - 1) == '/') {
baseUrl = baseUrl.substring(0, (baseUrl.length() - 1) + 0);
}
if (url.charAt(0) == '/') {
url = url.substring(1);
}
url = baseUrl + "/" + url;
url = url.replace(" ", "%20");
// Create HTTP transport objects
HttpPut httpRequest = new HttpPut(url);
// Set Headers
httpRequest.setHeader("Content-Type", "application/json; charset=utf-8");
// Serialize Request
String requestContent = null;
JsonNode requestDoc = null;
ObjectMapper objectMapper = new ObjectMapper();
ObjectNode serverCreateOrUpdateParametersValue = objectMapper.createObjectNode();
requestDoc = serverCreateOrUpdateParametersValue;
ObjectNode propertiesValue = objectMapper.createObjectNode();
((ObjectNode) serverCreateOrUpdateParametersValue).put("properties", propertiesValue);
if (parameters.getProperties().getVersion() != null) {
((ObjectNode) propertiesValue).put("version", parameters.getProperties().getVersion());
}
if (parameters.getProperties().getAdministratorLogin() != null) {
((ObjectNode) propertiesValue).put("administratorLogin", parameters.getProperties().getAdministratorLogin());
}
if (parameters.getProperties().getAdministratorLoginPassword() != null) {
((ObjectNode) propertiesValue).put("administratorLoginPassword", parameters.getProperties().getAdministratorLoginPassword());
}
((ObjectNode) serverCreateOrUpdateParametersValue).put("location", parameters.getLocation());
if (parameters.getTags() != null) {
ObjectNode tagsDictionary = objectMapper.createObjectNode();
for (Map.Entry entry : parameters.getTags().entrySet()) {
String tagsKey = entry.getKey();
String tagsValue = entry.getValue();
((ObjectNode) tagsDictionary).put(tagsKey, tagsValue);
}
((ObjectNode) serverCreateOrUpdateParametersValue).put("tags", tagsDictionary);
}
StringWriter stringWriter = new StringWriter();
objectMapper.writeValue(stringWriter, requestDoc);
requestContent = stringWriter.toString();
StringEntity entity = new StringEntity(requestContent);
httpRequest.setEntity(entity);
httpRequest.setHeader("Content-Type", "application/json; charset=utf-8");
// Send Request
HttpResponse httpResponse = null;
try {
if (shouldTrace) {
CloudTracing.sendRequest(invocationId, httpRequest);
}
httpResponse = this.getClient().getHttpClient().execute(httpRequest);
if (shouldTrace) {
CloudTracing.receiveResponse(invocationId, httpResponse);
}
int statusCode = httpResponse.getStatusLine().getStatusCode();
if (statusCode != HttpStatus.SC_OK && statusCode != HttpStatus.SC_CREATED) {
ServiceException ex = ServiceException.createFromJson(httpRequest, requestContent, httpResponse, httpResponse.getEntity());
if (shouldTrace) {
CloudTracing.error(invocationId, ex);
}
throw ex;
}
// Create Result
ServerGetResponse result = null;
// Deserialize Response
if (statusCode == HttpStatus.SC_OK || statusCode == HttpStatus.SC_CREATED) {
InputStream responseContent = httpResponse.getEntity().getContent();
result = new ServerGetResponse();
JsonNode responseDoc = null;
String responseDocContent = IOUtils.toString(responseContent);
if (responseDocContent == null == false && responseDocContent.length() > 0) {
responseDoc = objectMapper.readTree(responseDocContent);
}
if (responseDoc != null && responseDoc instanceof NullNode == false) {
Server serverInstance = new Server();
result.setServer(serverInstance);
JsonNode propertiesValue2 = responseDoc.get("properties");
if (propertiesValue2 != null && propertiesValue2 instanceof NullNode == false) {
ServerProperties propertiesInstance = new ServerProperties();
serverInstance.setProperties(propertiesInstance);
JsonNode fullyQualifiedDomainNameValue = propertiesValue2.get("fullyQualifiedDomainName");
if (fullyQualifiedDomainNameValue != null && fullyQualifiedDomainNameValue instanceof NullNode == false) {
String fullyQualifiedDomainNameInstance;
fullyQualifiedDomainNameInstance = fullyQualifiedDomainNameValue.getTextValue();
propertiesInstance.setFullyQualifiedDomainName(fullyQualifiedDomainNameInstance);
}
JsonNode versionValue = propertiesValue2.get("version");
if (versionValue != null && versionValue instanceof NullNode == false) {
String versionInstance;
versionInstance = versionValue.getTextValue();
propertiesInstance.setVersion(versionInstance);
}
JsonNode administratorLoginValue = propertiesValue2.get("administratorLogin");
if (administratorLoginValue != null && administratorLoginValue instanceof NullNode == false) {
String administratorLoginInstance;
administratorLoginInstance = administratorLoginValue.getTextValue();
propertiesInstance.setAdministratorLogin(administratorLoginInstance);
}
JsonNode administratorLoginPasswordValue = propertiesValue2.get("administratorLoginPassword");
if (administratorLoginPasswordValue != null && administratorLoginPasswordValue instanceof NullNode == false) {
String administratorLoginPasswordInstance;
administratorLoginPasswordInstance = administratorLoginPasswordValue.getTextValue();
propertiesInstance.setAdministratorLoginPassword(administratorLoginPasswordInstance);
}
}
JsonNode idValue = responseDoc.get("id");
if (idValue != null && idValue instanceof NullNode == false) {
String idInstance;
idInstance = idValue.getTextValue();
serverInstance.setId(idInstance);
}
JsonNode nameValue = responseDoc.get("name");
if (nameValue != null && nameValue instanceof NullNode == false) {
String nameInstance;
nameInstance = nameValue.getTextValue();
serverInstance.setName(nameInstance);
}
JsonNode typeValue = responseDoc.get("type");
if (typeValue != null && typeValue instanceof NullNode == false) {
String typeInstance;
typeInstance = typeValue.getTextValue();
serverInstance.setType(typeInstance);
}
JsonNode locationValue = responseDoc.get("location");
if (locationValue != null && locationValue instanceof NullNode == false) {
String locationInstance;
locationInstance = locationValue.getTextValue();
serverInstance.setLocation(locationInstance);
}
JsonNode tagsSequenceElement = ((JsonNode) responseDoc.get("tags"));
if (tagsSequenceElement != null && tagsSequenceElement instanceof NullNode == false) {
Iterator> itr = tagsSequenceElement.getFields();
while (itr.hasNext()) {
Map.Entry property = itr.next();
String tagsKey2 = property.getKey();
String tagsValue2 = property.getValue().getTextValue();
serverInstance.getTags().put(tagsKey2, tagsValue2);
}
}
}
}
result.setStatusCode(statusCode);
if (httpResponse.getHeaders("x-ms-request-id").length > 0) {
result.setRequestId(httpResponse.getFirstHeader("x-ms-request-id").getValue());
}
if (shouldTrace) {
CloudTracing.exit(invocationId, result);
}
return result;
} finally {
if (httpResponse != null && httpResponse.getEntity() != null) {
httpResponse.getEntity().getContent().close();
}
}
}
/**
* Returns information about an Azure SQL Database Server.
*
* @param resourceGroupName Required. The name of the Resource Group to
* which the server belongs.
* @param serverName Required. The name of the server to delete.
* @return A standard service response including an HTTP status code and
* request ID.
*/
@Override
public Future deleteAsync(final String resourceGroupName, final String serverName) {
return this.getClient().getExecutorService().submit(new Callable() {
@Override
public OperationResponse call() throws Exception {
return delete(resourceGroupName, serverName);
}
});
}
/**
* Returns information about an Azure SQL Database Server.
*
* @param resourceGroupName Required. The name of the Resource Group to
* which the server belongs.
* @param serverName Required. The name of the server to delete.
* @throws IOException Signals that an I/O exception of some sort has
* occurred. This class is the general class of exceptions produced by
* failed or interrupted I/O operations.
* @throws ServiceException Thrown if an unexpected response is found.
* @throws InterruptedException Thrown when a thread is waiting, sleeping,
* or otherwise occupied, and the thread is interrupted, either before or
* during the activity. Occasionally a method may wish to test whether the
* current thread has been interrupted, and if so, to immediately throw
* this exception. The following code can be used to achieve this effect:
* @throws ExecutionException Thrown when attempting to retrieve the result
* of a task that aborted by throwing an exception. This exception can be
* inspected using the Throwable.getCause() method.
* @return A standard service response including an HTTP status code and
* request ID.
*/
@Override
public OperationResponse delete(String resourceGroupName, String serverName) throws IOException, ServiceException, InterruptedException, ExecutionException {
// Validate
if (resourceGroupName == null) {
throw new NullPointerException("resourceGroupName");
}
if (serverName == null) {
throw new NullPointerException("serverName");
}
// Tracing
boolean shouldTrace = CloudTracing.getIsEnabled();
String invocationId = null;
if (shouldTrace) {
invocationId = Long.toString(CloudTracing.getNextInvocationId());
HashMap tracingParameters = new HashMap();
tracingParameters.put("resourceGroupName", resourceGroupName);
tracingParameters.put("serverName", serverName);
CloudTracing.enter(invocationId, this, "deleteAsync", tracingParameters);
}
// Construct URL
String url = "";
url = url + "/subscriptions/";
if (this.getClient().getCredentials().getSubscriptionId() != null) {
url = url + URLEncoder.encode(this.getClient().getCredentials().getSubscriptionId(), "UTF-8");
}
url = url + "/resourceGroups/";
url = url + URLEncoder.encode(resourceGroupName, "UTF-8");
url = url + "/providers/";
url = url + "Microsoft.Sql";
url = url + "/servers/";
url = url + URLEncoder.encode(serverName, "UTF-8");
ArrayList queryParameters = new ArrayList();
queryParameters.add("api-version=" + "2014-04-01");
if (queryParameters.size() > 0) {
url = url + "?" + CollectionStringBuilder.join(queryParameters, "&");
}
String baseUrl = this.getClient().getBaseUri().toString();
// Trim '/' character from the end of baseUrl and beginning of url.
if (baseUrl.charAt(baseUrl.length() - 1) == '/') {
baseUrl = baseUrl.substring(0, (baseUrl.length() - 1) + 0);
}
if (url.charAt(0) == '/') {
url = url.substring(1);
}
url = baseUrl + "/" + url;
url = url.replace(" ", "%20");
// Create HTTP transport objects
CustomHttpDelete httpRequest = new CustomHttpDelete(url);
// Set Headers
// Send Request
HttpResponse httpResponse = null;
try {
if (shouldTrace) {
CloudTracing.sendRequest(invocationId, httpRequest);
}
httpResponse = this.getClient().getHttpClient().execute(httpRequest);
if (shouldTrace) {
CloudTracing.receiveResponse(invocationId, httpResponse);
}
int statusCode = httpResponse.getStatusLine().getStatusCode();
if (statusCode != HttpStatus.SC_OK && statusCode != HttpStatus.SC_NO_CONTENT) {
ServiceException ex = ServiceException.createFromXml(httpRequest, null, httpResponse, httpResponse.getEntity());
if (shouldTrace) {
CloudTracing.error(invocationId, ex);
}
throw ex;
}
// Create Result
OperationResponse result = null;
// Deserialize Response
result = new OperationResponse();
result.setStatusCode(statusCode);
if (httpResponse.getHeaders("x-ms-request-id").length > 0) {
result.setRequestId(httpResponse.getFirstHeader("x-ms-request-id").getValue());
}
if (shouldTrace) {
CloudTracing.exit(invocationId, result);
}
return result;
} finally {
if (httpResponse != null && httpResponse.getEntity() != null) {
httpResponse.getEntity().getContent().close();
}
}
}
/**
* Returns information about an Azure SQL Database Server.
*
* @param resourceGroupName Required. The name of the Resource Group to
* which the server belongs.
* @param serverName Required. The name of the server to retrieve.
* @return Represents the response to a Get Azure Sql Database Server
* request.
*/
@Override
public Future getAsync(final String resourceGroupName, final String serverName) {
return this.getClient().getExecutorService().submit(new Callable() {
@Override
public ServerGetResponse call() throws Exception {
return get(resourceGroupName, serverName);
}
});
}
/**
* Returns information about an Azure SQL Database Server.
*
* @param resourceGroupName Required. The name of the Resource Group to
* which the server belongs.
* @param serverName Required. The name of the server to retrieve.
* @throws IOException Signals that an I/O exception of some sort has
* occurred. This class is the general class of exceptions produced by
* failed or interrupted I/O operations.
* @throws ServiceException Thrown if an unexpected response is found.
* @return Represents the response to a Get Azure Sql Database Server
* request.
*/
@Override
public ServerGetResponse get(String resourceGroupName, String serverName) throws IOException, ServiceException {
// Validate
if (resourceGroupName == null) {
throw new NullPointerException("resourceGroupName");
}
if (serverName == null) {
throw new NullPointerException("serverName");
}
// Tracing
boolean shouldTrace = CloudTracing.getIsEnabled();
String invocationId = null;
if (shouldTrace) {
invocationId = Long.toString(CloudTracing.getNextInvocationId());
HashMap tracingParameters = new HashMap();
tracingParameters.put("resourceGroupName", resourceGroupName);
tracingParameters.put("serverName", serverName);
CloudTracing.enter(invocationId, this, "getAsync", tracingParameters);
}
// Construct URL
String url = "";
url = url + "/subscriptions/";
if (this.getClient().getCredentials().getSubscriptionId() != null) {
url = url + URLEncoder.encode(this.getClient().getCredentials().getSubscriptionId(), "UTF-8");
}
url = url + "/resourceGroups/";
url = url + URLEncoder.encode(resourceGroupName, "UTF-8");
url = url + "/providers/";
url = url + "Microsoft.Sql";
url = url + "/servers/";
url = url + URLEncoder.encode(serverName, "UTF-8");
ArrayList queryParameters = new ArrayList();
queryParameters.add("api-version=" + "2014-04-01");
if (queryParameters.size() > 0) {
url = url + "?" + CollectionStringBuilder.join(queryParameters, "&");
}
String baseUrl = this.getClient().getBaseUri().toString();
// Trim '/' character from the end of baseUrl and beginning of url.
if (baseUrl.charAt(baseUrl.length() - 1) == '/') {
baseUrl = baseUrl.substring(0, (baseUrl.length() - 1) + 0);
}
if (url.charAt(0) == '/') {
url = url.substring(1);
}
url = baseUrl + "/" + url;
url = url.replace(" ", "%20");
// Create HTTP transport objects
HttpGet httpRequest = new HttpGet(url);
// Set Headers
// Send Request
HttpResponse httpResponse = null;
try {
if (shouldTrace) {
CloudTracing.sendRequest(invocationId, httpRequest);
}
httpResponse = this.getClient().getHttpClient().execute(httpRequest);
if (shouldTrace) {
CloudTracing.receiveResponse(invocationId, httpResponse);
}
int statusCode = httpResponse.getStatusLine().getStatusCode();
if (statusCode != HttpStatus.SC_OK) {
ServiceException ex = ServiceException.createFromJson(httpRequest, null, httpResponse, httpResponse.getEntity());
if (shouldTrace) {
CloudTracing.error(invocationId, ex);
}
throw ex;
}
// Create Result
ServerGetResponse result = null;
// Deserialize Response
if (statusCode == HttpStatus.SC_OK) {
InputStream responseContent = httpResponse.getEntity().getContent();
result = new ServerGetResponse();
ObjectMapper objectMapper = new ObjectMapper();
JsonNode responseDoc = null;
String responseDocContent = IOUtils.toString(responseContent);
if (responseDocContent == null == false && responseDocContent.length() > 0) {
responseDoc = objectMapper.readTree(responseDocContent);
}
if (responseDoc != null && responseDoc instanceof NullNode == false) {
Server serverInstance = new Server();
result.setServer(serverInstance);
JsonNode propertiesValue = responseDoc.get("properties");
if (propertiesValue != null && propertiesValue instanceof NullNode == false) {
ServerProperties propertiesInstance = new ServerProperties();
serverInstance.setProperties(propertiesInstance);
JsonNode fullyQualifiedDomainNameValue = propertiesValue.get("fullyQualifiedDomainName");
if (fullyQualifiedDomainNameValue != null && fullyQualifiedDomainNameValue instanceof NullNode == false) {
String fullyQualifiedDomainNameInstance;
fullyQualifiedDomainNameInstance = fullyQualifiedDomainNameValue.getTextValue();
propertiesInstance.setFullyQualifiedDomainName(fullyQualifiedDomainNameInstance);
}
JsonNode versionValue = propertiesValue.get("version");
if (versionValue != null && versionValue instanceof NullNode == false) {
String versionInstance;
versionInstance = versionValue.getTextValue();
propertiesInstance.setVersion(versionInstance);
}
JsonNode administratorLoginValue = propertiesValue.get("administratorLogin");
if (administratorLoginValue != null && administratorLoginValue instanceof NullNode == false) {
String administratorLoginInstance;
administratorLoginInstance = administratorLoginValue.getTextValue();
propertiesInstance.setAdministratorLogin(administratorLoginInstance);
}
JsonNode administratorLoginPasswordValue = propertiesValue.get("administratorLoginPassword");
if (administratorLoginPasswordValue != null && administratorLoginPasswordValue instanceof NullNode == false) {
String administratorLoginPasswordInstance;
administratorLoginPasswordInstance = administratorLoginPasswordValue.getTextValue();
propertiesInstance.setAdministratorLoginPassword(administratorLoginPasswordInstance);
}
}
JsonNode idValue = responseDoc.get("id");
if (idValue != null && idValue instanceof NullNode == false) {
String idInstance;
idInstance = idValue.getTextValue();
serverInstance.setId(idInstance);
}
JsonNode nameValue = responseDoc.get("name");
if (nameValue != null && nameValue instanceof NullNode == false) {
String nameInstance;
nameInstance = nameValue.getTextValue();
serverInstance.setName(nameInstance);
}
JsonNode typeValue = responseDoc.get("type");
if (typeValue != null && typeValue instanceof NullNode == false) {
String typeInstance;
typeInstance = typeValue.getTextValue();
serverInstance.setType(typeInstance);
}
JsonNode locationValue = responseDoc.get("location");
if (locationValue != null && locationValue instanceof NullNode == false) {
String locationInstance;
locationInstance = locationValue.getTextValue();
serverInstance.setLocation(locationInstance);
}
JsonNode tagsSequenceElement = ((JsonNode) responseDoc.get("tags"));
if (tagsSequenceElement != null && tagsSequenceElement instanceof NullNode == false) {
Iterator> itr = tagsSequenceElement.getFields();
while (itr.hasNext()) {
Map.Entry property = itr.next();
String tagsKey = property.getKey();
String tagsValue = property.getValue().getTextValue();
serverInstance.getTags().put(tagsKey, tagsValue);
}
}
}
}
result.setStatusCode(statusCode);
if (httpResponse.getHeaders("x-ms-request-id").length > 0) {
result.setRequestId(httpResponse.getFirstHeader("x-ms-request-id").getValue());
}
if (shouldTrace) {
CloudTracing.exit(invocationId, result);
}
return result;
} finally {
if (httpResponse != null && httpResponse.getEntity() != null) {
httpResponse.getEntity().getContent().close();
}
}
}
/**
* Returns information about an Azure SQL Database Server.
*
* @param resourceGroupName Required. The name of the Resource Group to
* which the server belongs.
* @return Represents the response to a Get Azure Sql Database Server
* request.
*/
@Override
public Future listAsync(final String resourceGroupName) {
return this.getClient().getExecutorService().submit(new Callable() {
@Override
public ServerListResponse call() throws Exception {
return list(resourceGroupName);
}
});
}
/**
* Returns information about an Azure SQL Database Server.
*
* @param resourceGroupName Required. The name of the Resource Group to
* which the server belongs.
* @throws IOException Signals that an I/O exception of some sort has
* occurred. This class is the general class of exceptions produced by
* failed or interrupted I/O operations.
* @throws ServiceException Thrown if an unexpected response is found.
* @return Represents the response to a Get Azure Sql Database Server
* request.
*/
@Override
public ServerListResponse list(String resourceGroupName) throws IOException, ServiceException {
// Validate
if (resourceGroupName == null) {
throw new NullPointerException("resourceGroupName");
}
// Tracing
boolean shouldTrace = CloudTracing.getIsEnabled();
String invocationId = null;
if (shouldTrace) {
invocationId = Long.toString(CloudTracing.getNextInvocationId());
HashMap tracingParameters = new HashMap();
tracingParameters.put("resourceGroupName", resourceGroupName);
CloudTracing.enter(invocationId, this, "listAsync", tracingParameters);
}
// Construct URL
String url = "";
url = url + "/subscriptions/";
if (this.getClient().getCredentials().getSubscriptionId() != null) {
url = url + URLEncoder.encode(this.getClient().getCredentials().getSubscriptionId(), "UTF-8");
}
url = url + "/resourceGroups/";
url = url + URLEncoder.encode(resourceGroupName, "UTF-8");
url = url + "/providers/";
url = url + "Microsoft.Sql";
url = url + "/servers";
ArrayList queryParameters = new ArrayList();
queryParameters.add("api-version=" + "2014-04-01");
if (queryParameters.size() > 0) {
url = url + "?" + CollectionStringBuilder.join(queryParameters, "&");
}
String baseUrl = this.getClient().getBaseUri().toString();
// Trim '/' character from the end of baseUrl and beginning of url.
if (baseUrl.charAt(baseUrl.length() - 1) == '/') {
baseUrl = baseUrl.substring(0, (baseUrl.length() - 1) + 0);
}
if (url.charAt(0) == '/') {
url = url.substring(1);
}
url = baseUrl + "/" + url;
url = url.replace(" ", "%20");
// Create HTTP transport objects
HttpGet httpRequest = new HttpGet(url);
// Set Headers
// Send Request
HttpResponse httpResponse = null;
try {
if (shouldTrace) {
CloudTracing.sendRequest(invocationId, httpRequest);
}
httpResponse = this.getClient().getHttpClient().execute(httpRequest);
if (shouldTrace) {
CloudTracing.receiveResponse(invocationId, httpResponse);
}
int statusCode = httpResponse.getStatusLine().getStatusCode();
if (statusCode != HttpStatus.SC_OK) {
ServiceException ex = ServiceException.createFromJson(httpRequest, null, httpResponse, httpResponse.getEntity());
if (shouldTrace) {
CloudTracing.error(invocationId, ex);
}
throw ex;
}
// Create Result
ServerListResponse result = null;
// Deserialize Response
if (statusCode == HttpStatus.SC_OK) {
InputStream responseContent = httpResponse.getEntity().getContent();
result = new ServerListResponse();
ObjectMapper objectMapper = new ObjectMapper();
JsonNode responseDoc = null;
String responseDocContent = IOUtils.toString(responseContent);
if (responseDocContent == null == false && responseDocContent.length() > 0) {
responseDoc = objectMapper.readTree(responseDocContent);
}
if (responseDoc != null && responseDoc instanceof NullNode == false) {
JsonNode valueArray = responseDoc.get("value");
if (valueArray != null && valueArray instanceof NullNode == false) {
for (JsonNode valueValue : ((ArrayNode) valueArray)) {
Server serverInstance = new Server();
result.getServers().add(serverInstance);
JsonNode propertiesValue = valueValue.get("properties");
if (propertiesValue != null && propertiesValue instanceof NullNode == false) {
ServerProperties propertiesInstance = new ServerProperties();
serverInstance.setProperties(propertiesInstance);
JsonNode fullyQualifiedDomainNameValue = propertiesValue.get("fullyQualifiedDomainName");
if (fullyQualifiedDomainNameValue != null && fullyQualifiedDomainNameValue instanceof NullNode == false) {
String fullyQualifiedDomainNameInstance;
fullyQualifiedDomainNameInstance = fullyQualifiedDomainNameValue.getTextValue();
propertiesInstance.setFullyQualifiedDomainName(fullyQualifiedDomainNameInstance);
}
JsonNode versionValue = propertiesValue.get("version");
if (versionValue != null && versionValue instanceof NullNode == false) {
String versionInstance;
versionInstance = versionValue.getTextValue();
propertiesInstance.setVersion(versionInstance);
}
JsonNode administratorLoginValue = propertiesValue.get("administratorLogin");
if (administratorLoginValue != null && administratorLoginValue instanceof NullNode == false) {
String administratorLoginInstance;
administratorLoginInstance = administratorLoginValue.getTextValue();
propertiesInstance.setAdministratorLogin(administratorLoginInstance);
}
JsonNode administratorLoginPasswordValue = propertiesValue.get("administratorLoginPassword");
if (administratorLoginPasswordValue != null && administratorLoginPasswordValue instanceof NullNode == false) {
String administratorLoginPasswordInstance;
administratorLoginPasswordInstance = administratorLoginPasswordValue.getTextValue();
propertiesInstance.setAdministratorLoginPassword(administratorLoginPasswordInstance);
}
}
JsonNode idValue = valueValue.get("id");
if (idValue != null && idValue instanceof NullNode == false) {
String idInstance;
idInstance = idValue.getTextValue();
serverInstance.setId(idInstance);
}
JsonNode nameValue = valueValue.get("name");
if (nameValue != null && nameValue instanceof NullNode == false) {
String nameInstance;
nameInstance = nameValue.getTextValue();
serverInstance.setName(nameInstance);
}
JsonNode typeValue = valueValue.get("type");
if (typeValue != null && typeValue instanceof NullNode == false) {
String typeInstance;
typeInstance = typeValue.getTextValue();
serverInstance.setType(typeInstance);
}
JsonNode locationValue = valueValue.get("location");
if (locationValue != null && locationValue instanceof NullNode == false) {
String locationInstance;
locationInstance = locationValue.getTextValue();
serverInstance.setLocation(locationInstance);
}
JsonNode tagsSequenceElement = ((JsonNode) valueValue.get("tags"));
if (tagsSequenceElement != null && tagsSequenceElement instanceof NullNode == false) {
Iterator> itr = tagsSequenceElement.getFields();
while (itr.hasNext()) {
Map.Entry property = itr.next();
String tagsKey = property.getKey();
String tagsValue = property.getValue().getTextValue();
serverInstance.getTags().put(tagsKey, tagsValue);
}
}
}
}
}
}
result.setStatusCode(statusCode);
if (httpResponse.getHeaders("x-ms-request-id").length > 0) {
result.setRequestId(httpResponse.getFirstHeader("x-ms-request-id").getValue());
}
if (shouldTrace) {
CloudTracing.exit(invocationId, result);
}
return result;
} finally {
if (httpResponse != null && httpResponse.getEntity() != null) {
httpResponse.getEntity().getContent().close();
}
}
}
/**
* Returns information about Azure SQL Database Server usage.
*
* @param resourceGroupName Required. The name of the Resource Group to
* which the server belongs.
* @param serverName Required. The name of the Azure SQL Database Server in
* which the Azure SQL Databases are hosted.
* @return Represents the response to a List Azure Sql Database Server
* metrics request.
*/
@Override
public Future listUsagesAsync(final String resourceGroupName, final String serverName) {
return this.getClient().getExecutorService().submit(new Callable() {
@Override
public ServerMetricListResponse call() throws Exception {
return listUsages(resourceGroupName, serverName);
}
});
}
/**
* Returns information about Azure SQL Database Server usage.
*
* @param resourceGroupName Required. The name of the Resource Group to
* which the server belongs.
* @param serverName Required. The name of the Azure SQL Database Server in
* which the Azure SQL Databases are hosted.
* @throws IOException Signals that an I/O exception of some sort has
* occurred. This class is the general class of exceptions produced by
* failed or interrupted I/O operations.
* @throws ServiceException Thrown if an unexpected response is found.
* @return Represents the response to a List Azure Sql Database Server
* metrics request.
*/
@Override
public ServerMetricListResponse listUsages(String resourceGroupName, String serverName) throws IOException, ServiceException {
// Validate
if (resourceGroupName == null) {
throw new NullPointerException("resourceGroupName");
}
if (serverName == null) {
throw new NullPointerException("serverName");
}
// Tracing
boolean shouldTrace = CloudTracing.getIsEnabled();
String invocationId = null;
if (shouldTrace) {
invocationId = Long.toString(CloudTracing.getNextInvocationId());
HashMap tracingParameters = new HashMap();
tracingParameters.put("resourceGroupName", resourceGroupName);
tracingParameters.put("serverName", serverName);
CloudTracing.enter(invocationId, this, "listUsagesAsync", tracingParameters);
}
// Construct URL
String url = "";
url = url + "/subscriptions/";
if (this.getClient().getCredentials().getSubscriptionId() != null) {
url = url + URLEncoder.encode(this.getClient().getCredentials().getSubscriptionId(), "UTF-8");
}
url = url + "/resourceGroups/";
url = url + URLEncoder.encode(resourceGroupName, "UTF-8");
url = url + "/providers/";
url = url + "Microsoft.Sql";
url = url + "/servers/";
url = url + URLEncoder.encode(serverName, "UTF-8");
url = url + "/usages";
ArrayList queryParameters = new ArrayList();
queryParameters.add("api-version=" + "2014-04-01");
if (queryParameters.size() > 0) {
url = url + "?" + CollectionStringBuilder.join(queryParameters, "&");
}
String baseUrl = this.getClient().getBaseUri().toString();
// Trim '/' character from the end of baseUrl and beginning of url.
if (baseUrl.charAt(baseUrl.length() - 1) == '/') {
baseUrl = baseUrl.substring(0, (baseUrl.length() - 1) + 0);
}
if (url.charAt(0) == '/') {
url = url.substring(1);
}
url = baseUrl + "/" + url;
url = url.replace(" ", "%20");
// Create HTTP transport objects
HttpGet httpRequest = new HttpGet(url);
// Set Headers
// Send Request
HttpResponse httpResponse = null;
try {
if (shouldTrace) {
CloudTracing.sendRequest(invocationId, httpRequest);
}
httpResponse = this.getClient().getHttpClient().execute(httpRequest);
if (shouldTrace) {
CloudTracing.receiveResponse(invocationId, httpResponse);
}
int statusCode = httpResponse.getStatusLine().getStatusCode();
if (statusCode != HttpStatus.SC_OK) {
ServiceException ex = ServiceException.createFromJson(httpRequest, null, httpResponse, httpResponse.getEntity());
if (shouldTrace) {
CloudTracing.error(invocationId, ex);
}
throw ex;
}
// Create Result
ServerMetricListResponse result = null;
// Deserialize Response
if (statusCode == HttpStatus.SC_OK) {
InputStream responseContent = httpResponse.getEntity().getContent();
result = new ServerMetricListResponse();
ObjectMapper objectMapper = new ObjectMapper();
JsonNode responseDoc = null;
String responseDocContent = IOUtils.toString(responseContent);
if (responseDocContent == null == false && responseDocContent.length() > 0) {
responseDoc = objectMapper.readTree(responseDocContent);
}
if (responseDoc != null && responseDoc instanceof NullNode == false) {
JsonNode valueArray = responseDoc.get("value");
if (valueArray != null && valueArray instanceof NullNode == false) {
for (JsonNode valueValue : ((ArrayNode) valueArray)) {
ServerMetric serverMetricInstance = new ServerMetric();
result.getMetrics().add(serverMetricInstance);
JsonNode resourceNameValue = valueValue.get("resourceName");
if (resourceNameValue != null && resourceNameValue instanceof NullNode == false) {
String resourceNameInstance;
resourceNameInstance = resourceNameValue.getTextValue();
serverMetricInstance.setResourceName(resourceNameInstance);
}
JsonNode displayNameValue = valueValue.get("displayName");
if (displayNameValue != null && displayNameValue instanceof NullNode == false) {
String displayNameInstance;
displayNameInstance = displayNameValue.getTextValue();
serverMetricInstance.setDisplayName(displayNameInstance);
}
JsonNode currentValueValue = valueValue.get("currentValue");
if (currentValueValue != null && currentValueValue instanceof NullNode == false) {
double currentValueInstance;
currentValueInstance = currentValueValue.getDoubleValue();
serverMetricInstance.setCurrentValue(currentValueInstance);
}
JsonNode limitValue = valueValue.get("limit");
if (limitValue != null && limitValue instanceof NullNode == false) {
double limitInstance;
limitInstance = limitValue.getDoubleValue();
serverMetricInstance.setLimit(limitInstance);
}
JsonNode unitValue = valueValue.get("unit");
if (unitValue != null && unitValue instanceof NullNode == false) {
String unitInstance;
unitInstance = unitValue.getTextValue();
serverMetricInstance.setUnit(unitInstance);
}
JsonNode nextResetTimeValue = valueValue.get("nextResetTime");
if (nextResetTimeValue != null && nextResetTimeValue instanceof NullNode == false) {
Calendar nextResetTimeInstance;
nextResetTimeInstance = DatatypeConverter.parseDateTime(nextResetTimeValue.getTextValue());
serverMetricInstance.setNextResetTime(nextResetTimeInstance);
}
}
}
}
}
result.setStatusCode(statusCode);
if (httpResponse.getHeaders("x-ms-request-id").length > 0) {
result.setRequestId(httpResponse.getFirstHeader("x-ms-request-id").getValue());
}
if (shouldTrace) {
CloudTracing.exit(invocationId, result);
}
return result;
} finally {
if (httpResponse != null && httpResponse.getEntity() != null) {
httpResponse.getEntity().getContent().close();
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy