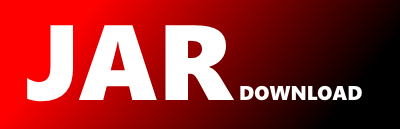
com.microsoft.azure.management.sql.SqlManagementClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-mgmt-sql Show documentation
Show all versions of azure-mgmt-sql Show documentation
This package contains Microsoft Azure SDK for SQL Management module.
/**
*
* Copyright (c) Microsoft and contributors. All rights reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
*
* See the License for the specific language governing permissions and
* limitations under the License.
*
*/
// Warning: This code was generated by a tool.
//
// Changes to this file may cause incorrect behavior and will be lost if the
// code is regenerated.
package com.microsoft.azure.management.sql;
import com.microsoft.windowsazure.core.FilterableService;
import com.microsoft.windowsazure.credentials.SubscriptionCloudCredentials;
import java.io.Closeable;
import java.net.URI;
/**
* The Windows Azure SQL Database management API provides a RESTful set of web
* services that interact with Windows Azure SQL Database services to manage
* your databases. The API enables users to create, retrieve, update, and
* delete databases and servers.
*/
public interface SqlManagementClient extends Closeable, FilterableService {
/**
* Gets the API version.
* @return The ApiVersion value.
*/
String getApiVersion();
/**
* Gets the URI used as the base for all cloud service requests.
* @return The BaseUri value.
*/
URI getBaseUri();
/**
* Gets subscription credentials which uniquely identify Microsoft Azure
* subscription. The subscription ID forms part of the URI for every
* service call.
* @return The Credentials value.
*/
SubscriptionCloudCredentials getCredentials();
/**
* Gets or sets the initial timeout for Long Running Operations.
* @return The LongRunningOperationInitialTimeout value.
*/
int getLongRunningOperationInitialTimeout();
/**
* Gets or sets the initial timeout for Long Running Operations.
* @param longRunningOperationInitialTimeoutValue The
* LongRunningOperationInitialTimeout value.
*/
void setLongRunningOperationInitialTimeout(final int longRunningOperationInitialTimeoutValue);
/**
* Gets or sets the retry timeout for Long Running Operations.
* @return The LongRunningOperationRetryTimeout value.
*/
int getLongRunningOperationRetryTimeout();
/**
* Gets or sets the retry timeout for Long Running Operations.
* @param longRunningOperationRetryTimeoutValue The
* LongRunningOperationRetryTimeout value.
*/
void setLongRunningOperationRetryTimeout(final int longRunningOperationRetryTimeoutValue);
/**
* Represents all the operations to manage Azure SQL Database and Database
* Server Audit policy. Contains operations to: Create, Retrieve and
* Update audit policy.
* @return The AuditingPolicyOperations value.
*/
AuditingPolicyOperations getAuditingPolicyOperations();
/**
* Represents all the operations for determining the set of capabilites
* available in a specified region.
* @return The CapabilitiesOperations value.
*/
CapabilitiesOperations getCapabilitiesOperations();
/**
* Represents all the operations for operating pertaining to activation on
* Azure SQL Data Warehouse databases. Contains operations to: Pause and
* Resume databases
* @return The DatabaseActivationOperations value.
*/
DatabaseActivationOperations getDatabaseActivationOperations();
/**
* Represents all the operations for operating on Azure SQL Database restore
* points. Contains operations to: List restore points.
* @return The DatabaseBackupOperations value.
*/
DatabaseBackupOperations getDatabaseBackupOperations();
/**
* Represents all the operations for operating on Azure SQL Databases.
* Contains operations to: Create, Retrieve, Update, and Delete databases,
* and also includes the ability to get the event logs for a database.
* @return The DatabasesOperations value.
*/
DatabaseOperations getDatabasesOperations();
/**
* Represents all the operations for operating on Azure SQL Database data
* masking. Contains operations to: Create, Retrieve, Update, and Delete
* data masking rules, as well as Create, Retreive and Update data masking
* policy.
* @return The DataMaskingOperations value.
*/
DataMaskingOperations getDataMaskingOperations();
/**
* Represents all the operations for operating on Azure SQL Database Elastic
* Pools. Contains operations to: Create, Retrieve, Update, and Delete.
* @return The ElasticPoolsOperations value.
*/
ElasticPoolOperations getElasticPoolsOperations();
/**
* Represents all the operations for operating on Azure SQL Database Server
* Firewall Rules. Contains operations to: Create, Retrieve, Update, and
* Delete firewall rules.
* @return The FirewallRulesOperations value.
*/
FirewallRuleOperations getFirewallRulesOperations();
/**
* Represents all the operations for operating on Azure SQL Recommended
* Elastic Pools. Contains operations to: Retrieve.
* @return The RecommendedElasticPoolsOperations value.
*/
RecommendedElasticPoolOperations getRecommendedElasticPoolsOperations();
/**
* Represents all the operations for managing recommended indexes on Azure
* SQL Databases. Contains operations to retrieve recommended index and
* update state.
* @return The RecommendedIndexesOperations value.
*/
RecommendedIndexOperations getRecommendedIndexesOperations();
/**
* Represents all the operations for operating on Azure SQL Database
* Replication Links. Contains operations to: Delete and Retrieve
* replication links.
* @return The DatabaseReplicationLinksOperations value.
*/
ReplicationLinkOperations getDatabaseReplicationLinksOperations();
/**
* Represents all the operations for managing Azure SQL Database secure
* connection. Contains operations to: Create, Retrieve and Update secure
* connection policy .
* @return The SecureConnectionOperations value.
*/
SecureConnectionPolicyOperations getSecureConnectionOperations();
/**
* Represents all the operations for operating on Azure SQL Server Active
* Directory Administrators. Contains operations to: Create, Retrieve,
* Update, and Delete Azure SQL Server Active Directory Administrators.
* @return The ServerAdministratorsOperations value.
*/
ServerAdministratorOperations getServerAdministratorsOperations();
/**
* Represents all the operations for operating on Azure SQL Database
* Servers. Contains operations to: Create, Retrieve, Update, and Delete
* servers.
* @return The ServersOperations value.
*/
ServerOperations getServersOperations();
/**
* Represents all the operations for Azure SQL Database Server Upgrade
* @return The ServerUpgradesOperations value.
*/
ServerUpgradeOperations getServerUpgradesOperations();
/**
* Represents all the operations for operating on Azure SQL Database Service
* Objectives. Contains operations to: Retrieve service objectives.
* @return The ServiceObjectivesOperations value.
*/
ServiceObjectiveOperations getServiceObjectivesOperations();
/**
* Represents all the operations for operating on service tier advisors.
* Contains operations to: Retrieve.
* @return The ServiceTierAdvisorsOperations value.
*/
ServiceTierAdvisorOperations getServiceTierAdvisorsOperations();
/**
* Represents all the operations of Azure SQL Database Transparent Data
* Encryption. Contains operations to: Retrieve, and Update Transparent
* Data Encryption.
* @return The TransparentDataEncryptionOperations value.
*/
TransparentDataEncryptionOperations getTransparentDataEncryptionOperations();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy