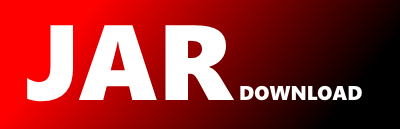
com.microsoft.azure.management.sql.models.DataMaskingRuleProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-mgmt-sql Show documentation
Show all versions of azure-mgmt-sql Show documentation
This package contains Microsoft Azure SDK for SQL Management module.
/**
*
* Copyright (c) Microsoft and contributors. All rights reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
*
* See the License for the specific language governing permissions and
* limitations under the License.
*
*/
// Warning: This code was generated by a tool.
//
// Changes to this file may cause incorrect behavior and will be lost if the
// code is regenerated.
package com.microsoft.azure.management.sql.models;
/**
* Represents the properties of an Azure SQL Database data masking rule.
*/
public class DataMaskingRuleProperties {
private String columnName;
/**
* Optional. Gets or sets the column name on which the data masking rule is
* applied.
* @return The ColumnName value.
*/
public String getColumnName() {
return this.columnName;
}
/**
* Optional. Gets or sets the column name on which the data masking rule is
* applied.
* @param columnNameValue The ColumnName value.
*/
public void setColumnName(final String columnNameValue) {
this.columnName = columnNameValue;
}
private String id;
/**
* Required. Gets or sets the rule Id.
* @return The Id value.
*/
public String getId() {
return this.id;
}
/**
* Required. Gets or sets the rule Id.
* @param idValue The Id value.
*/
public void setId(final String idValue) {
this.id = idValue;
}
private String maskingFunction;
/**
* Required. Gets or sets the masking function that is used for the data
* masking rule.
* @return The MaskingFunction value.
*/
public String getMaskingFunction() {
return this.maskingFunction;
}
/**
* Required. Gets or sets the masking function that is used for the data
* masking rule.
* @param maskingFunctionValue The MaskingFunction value.
*/
public void setMaskingFunction(final String maskingFunctionValue) {
this.maskingFunction = maskingFunctionValue;
}
private String numberFrom;
/**
* Optional. Gets or sets the numberFrom property of the masking rule.
* @return The NumberFrom value.
*/
public String getNumberFrom() {
return this.numberFrom;
}
/**
* Optional. Gets or sets the numberFrom property of the masking rule.
* @param numberFromValue The NumberFrom value.
*/
public void setNumberFrom(final String numberFromValue) {
this.numberFrom = numberFromValue;
}
private String numberTo;
/**
* Optional. Gets or sets the numberTo property of the data masking rule.
* @return The NumberTo value.
*/
public String getNumberTo() {
return this.numberTo;
}
/**
* Optional. Gets or sets the numberTo property of the data masking rule.
* @param numberToValue The NumberTo value.
*/
public void setNumberTo(final String numberToValue) {
this.numberTo = numberToValue;
}
private String prefixSize;
/**
* Optional. Gets or sets the prefixSize property that is used for the data
* masking rule.
* @return The PrefixSize value.
*/
public String getPrefixSize() {
return this.prefixSize;
}
/**
* Optional. Gets or sets the prefixSize property that is used for the data
* masking rule.
* @param prefixSizeValue The PrefixSize value.
*/
public void setPrefixSize(final String prefixSizeValue) {
this.prefixSize = prefixSizeValue;
}
private String replacementString;
/**
* Optional. Gets or sets the replacementString property that is used for
* the data masking rule.
* @return The ReplacementString value.
*/
public String getReplacementString() {
return this.replacementString;
}
/**
* Optional. Gets or sets the replacementString property that is used for
* the data masking rule.
* @param replacementStringValue The ReplacementString value.
*/
public void setReplacementString(final String replacementStringValue) {
this.replacementString = replacementStringValue;
}
private String schemaName;
/**
* Optional. Gets or sets the schema name on which the data masking rule is
* applied.
* @return The SchemaName value.
*/
public String getSchemaName() {
return this.schemaName;
}
/**
* Optional. Gets or sets the schema name on which the data masking rule is
* applied.
* @param schemaNameValue The SchemaName value.
*/
public void setSchemaName(final String schemaNameValue) {
this.schemaName = schemaNameValue;
}
private String suffixSize;
/**
* Optional. Gets or sets the suffixSize property that is used for the data
* masking rule.
* @return The SuffixSize value.
*/
public String getSuffixSize() {
return this.suffixSize;
}
/**
* Optional. Gets or sets the suffixSize property that is used for the data
* masking rule.
* @param suffixSizeValue The SuffixSize value.
*/
public void setSuffixSize(final String suffixSizeValue) {
this.suffixSize = suffixSizeValue;
}
private String tableName;
/**
* Optional. Gets or sets the table name on which the data masking rule is
* applied.
* @return The TableName value.
*/
public String getTableName() {
return this.tableName;
}
/**
* Optional. Gets or sets the table name on which the data masking rule is
* applied.
* @param tableNameValue The TableName value.
*/
public void setTableName(final String tableNameValue) {
this.tableName = tableNameValue;
}
/**
* Initializes a new instance of the DataMaskingRuleProperties class.
*
*/
public DataMaskingRuleProperties() {
}
/**
* Initializes a new instance of the DataMaskingRuleProperties class with
* required arguments.
*
* @param id Gets or sets the rule Id.
* @param maskingFunction Gets or sets the masking function that is used for
* the data masking rule.
*/
public DataMaskingRuleProperties(String id, String maskingFunction) {
if (id == null) {
throw new NullPointerException("id");
}
if (maskingFunction == null) {
throw new NullPointerException("maskingFunction");
}
this.setId(id);
this.setMaskingFunction(maskingFunction);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy