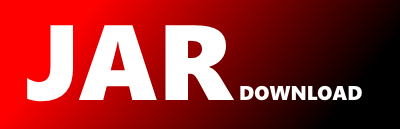
com.microsoft.azure.management.sql.models.ElasticPoolActivityProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-mgmt-sql Show documentation
Show all versions of azure-mgmt-sql Show documentation
This package contains Microsoft Azure SDK for SQL Management module.
/**
*
* Copyright (c) Microsoft and contributors. All rights reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
*
* See the License for the specific language governing permissions and
* limitations under the License.
*
*/
// Warning: This code was generated by a tool.
//
// Changes to this file may cause incorrect behavior and will be lost if the
// code is regenerated.
package com.microsoft.azure.management.sql.models;
import java.util.Calendar;
/**
* Represents the properties of an Azure SQL Database Elastic Pool.
*/
public class ElasticPoolActivityProperties {
private String elasticPoolName;
/**
* Optional. Gets the name of the Elastic Pool.
* @return The ElasticPoolName value.
*/
public String getElasticPoolName() {
return this.elasticPoolName;
}
/**
* Optional. Gets the name of the Elastic Pool.
* @param elasticPoolNameValue The ElasticPoolName value.
*/
public void setElasticPoolName(final String elasticPoolNameValue) {
this.elasticPoolName = elasticPoolNameValue;
}
private Calendar endTime;
/**
* Optional. Gets the time the operation finished.
* @return The EndTime value.
*/
public Calendar getEndTime() {
return this.endTime;
}
/**
* Optional. Gets the time the operation finished.
* @param endTimeValue The EndTime value.
*/
public void setEndTime(final Calendar endTimeValue) {
this.endTime = endTimeValue;
}
private Integer errorCode;
/**
* Optional. Gets the error code if available.
* @return The ErrorCode value.
*/
public Integer getErrorCode() {
return this.errorCode;
}
/**
* Optional. Gets the error code if available.
* @param errorCodeValue The ErrorCode value.
*/
public void setErrorCode(final Integer errorCodeValue) {
this.errorCode = errorCodeValue;
}
private String errorMessage;
/**
* Optional. Gets the error message if available.
* @return The ErrorMessage value.
*/
public String getErrorMessage() {
return this.errorMessage;
}
/**
* Optional. Gets the error message if available.
* @param errorMessageValue The ErrorMessage value.
*/
public void setErrorMessage(final String errorMessageValue) {
this.errorMessage = errorMessageValue;
}
private Integer errorSeverity;
/**
* Optional. Gets the error severity if available.
* @return The ErrorSeverity value.
*/
public Integer getErrorSeverity() {
return this.errorSeverity;
}
/**
* Optional. Gets the error severity if available.
* @param errorSeverityValue The ErrorSeverity value.
*/
public void setErrorSeverity(final Integer errorSeverityValue) {
this.errorSeverity = errorSeverityValue;
}
private String operation;
/**
* Optional. Gets the operation name.
* @return The Operation value.
*/
public String getOperation() {
return this.operation;
}
/**
* Optional. Gets the operation name.
* @param operationValue The Operation value.
*/
public void setOperation(final String operationValue) {
this.operation = operationValue;
}
private String operationId;
/**
* Optional. Gets the unique operation ID.
* @return The OperationId value.
*/
public String getOperationId() {
return this.operationId;
}
/**
* Optional. Gets the unique operation ID.
* @param operationIdValue The OperationId value.
*/
public void setOperationId(final String operationIdValue) {
this.operationId = operationIdValue;
}
private Integer percentComplete;
/**
* Optional. Gets the percentage complete if available.
* @return The PercentComplete value.
*/
public Integer getPercentComplete() {
return this.percentComplete;
}
/**
* Optional. Gets the percentage complete if available.
* @param percentCompleteValue The PercentComplete value.
*/
public void setPercentComplete(final Integer percentCompleteValue) {
this.percentComplete = percentCompleteValue;
}
private Integer requestedDatabaseDtuMax;
/**
* Optional. Gets the requested max DTU per database if available.
* @return The RequestedDatabaseDtuMax value.
*/
public Integer getRequestedDatabaseDtuMax() {
return this.requestedDatabaseDtuMax;
}
/**
* Optional. Gets the requested max DTU per database if available.
* @param requestedDatabaseDtuMaxValue The RequestedDatabaseDtuMax value.
*/
public void setRequestedDatabaseDtuMax(final Integer requestedDatabaseDtuMaxValue) {
this.requestedDatabaseDtuMax = requestedDatabaseDtuMaxValue;
}
private Integer requestedDatabaseDtuMin;
/**
* Optional. Gets the requested min DTU per database if available.
* @return The RequestedDatabaseDtuMin value.
*/
public Integer getRequestedDatabaseDtuMin() {
return this.requestedDatabaseDtuMin;
}
/**
* Optional. Gets the requested min DTU per database if available.
* @param requestedDatabaseDtuMinValue The RequestedDatabaseDtuMin value.
*/
public void setRequestedDatabaseDtuMin(final Integer requestedDatabaseDtuMinValue) {
this.requestedDatabaseDtuMin = requestedDatabaseDtuMinValue;
}
private Integer requestedDtu;
/**
* Optional. Gets the requested DTU for the pool if available.
* @return The RequestedDtu value.
*/
public Integer getRequestedDtu() {
return this.requestedDtu;
}
/**
* Optional. Gets the requested DTU for the pool if available.
* @param requestedDtuValue The RequestedDtu value.
*/
public void setRequestedDtu(final Integer requestedDtuValue) {
this.requestedDtu = requestedDtuValue;
}
private String requestedElasticPoolName;
/**
* Optional. Gets the requested name for the Elastic Pool if available.
* @return The RequestedElasticPoolName value.
*/
public String getRequestedElasticPoolName() {
return this.requestedElasticPoolName;
}
/**
* Optional. Gets the requested name for the Elastic Pool if available.
* @param requestedElasticPoolNameValue The RequestedElasticPoolName value.
*/
public void setRequestedElasticPoolName(final String requestedElasticPoolNameValue) {
this.requestedElasticPoolName = requestedElasticPoolNameValue;
}
private Long requestedStorageLimitInGB;
/**
* Optional. Gets the requested storage limit for the pool in GB if
* available.
* @return The RequestedStorageLimitInGB value.
*/
public Long getRequestedStorageLimitInGB() {
return this.requestedStorageLimitInGB;
}
/**
* Optional. Gets the requested storage limit for the pool in GB if
* available.
* @param requestedStorageLimitInGBValue The RequestedStorageLimitInGB value.
*/
public void setRequestedStorageLimitInGB(final Long requestedStorageLimitInGBValue) {
this.requestedStorageLimitInGB = requestedStorageLimitInGBValue;
}
private String serverName;
/**
* Optional. Gets the name of the Azure Sql Database Server the Elastic Pool
* is in.
* @return The ServerName value.
*/
public String getServerName() {
return this.serverName;
}
/**
* Optional. Gets the name of the Azure Sql Database Server the Elastic Pool
* is in.
* @param serverNameValue The ServerName value.
*/
public void setServerName(final String serverNameValue) {
this.serverName = serverNameValue;
}
private Calendar startTime;
/**
* Optional. Gets the time the operation started.
* @return The StartTime value.
*/
public Calendar getStartTime() {
return this.startTime;
}
/**
* Optional. Gets the time the operation started.
* @param startTimeValue The StartTime value.
*/
public void setStartTime(final Calendar startTimeValue) {
this.startTime = startTimeValue;
}
private String state;
/**
* Optional. Gets the current state of the operation.
* @return The State value.
*/
public String getState() {
return this.state;
}
/**
* Optional. Gets the current state of the operation.
* @param stateValue The State value.
*/
public void setState(final String stateValue) {
this.state = stateValue;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy