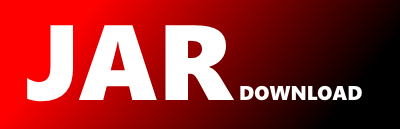
com.microsoft.azure.management.sql.models.RecommendedIndexProperties Maven / Gradle / Ivy
/**
*
* Copyright (c) Microsoft and contributors. All rights reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
*
* See the License for the specific language governing permissions and
* limitations under the License.
*
*/
// Warning: This code was generated by a tool.
//
// Changes to this file may cause incorrect behavior and will be lost if the
// code is regenerated.
package com.microsoft.azure.management.sql.models;
import com.microsoft.windowsazure.core.LazyArrayList;
import java.util.ArrayList;
import java.util.Calendar;
/**
* Represents the properties of an Azure SQL Database recommended index.
*/
public class RecommendedIndexProperties {
private String action;
/**
* Optional. Gets the proposed index action. We suggest user to create
* missing index, drop unused index or to rebuild already existing index to
* improve its performance. Possible values are 'Create', 'Drop', 'Rebuild'.
* @return The Action value.
*/
public String getAction() {
return this.action;
}
/**
* Optional. Gets the proposed index action. We suggest user to create
* missing index, drop unused index or to rebuild already existing index to
* improve its performance. Possible values are 'Create', 'Drop', 'Rebuild'.
* @param actionValue The Action value.
*/
public void setAction(final String actionValue) {
this.action = actionValue;
}
private ArrayList columns;
/**
* Optional. Columns over which to build index
* @return The Columns value.
*/
public ArrayList getColumns() {
return this.columns;
}
/**
* Optional. Columns over which to build index
* @param columnsValue The Columns value.
*/
public void setColumns(final ArrayList columnsValue) {
this.columns = columnsValue;
}
private Calendar created;
/**
* Optional. Gets the UTC datetime showing when this resource was created.
* @return The Created value.
*/
public Calendar getCreated() {
return this.created;
}
/**
* Optional. Gets the UTC datetime showing when this resource was created.
* @param createdValue The Created value.
*/
public void setCreated(final Calendar createdValue) {
this.created = createdValue;
}
private ArrayList estimatedImpact;
/**
* Optional. Gets the estimated impact of doing recommended index action.
* @return The EstimatedImpact value.
*/
public ArrayList getEstimatedImpact() {
return this.estimatedImpact;
}
/**
* Optional. Gets the estimated impact of doing recommended index action.
* @param estimatedImpactValue The EstimatedImpact value.
*/
public void setEstimatedImpact(final ArrayList estimatedImpactValue) {
this.estimatedImpact = estimatedImpactValue;
}
private ArrayList includedColumns;
/**
* Optional. Gets the list of column names to be included in the index
* @return The IncludedColumns value.
*/
public ArrayList getIncludedColumns() {
return this.includedColumns;
}
/**
* Optional. Gets the list of column names to be included in the index
* @param includedColumnsValue The IncludedColumns value.
*/
public void setIncludedColumns(final ArrayList includedColumnsValue) {
this.includedColumns = includedColumnsValue;
}
private String indexScript;
/**
* Optional. Gets the full build index script
* @return The IndexScript value.
*/
public String getIndexScript() {
return this.indexScript;
}
/**
* Optional. Gets the full build index script
* @param indexScriptValue The IndexScript value.
*/
public void setIndexScript(final String indexScriptValue) {
this.indexScript = indexScriptValue;
}
private String indexType;
/**
* Optional. Gets the type of index (CLUSTERED, NONCLUSTERED, COLUMNSTORE,
* CLUSTERED COLUMNSTORE)
* @return The IndexType value.
*/
public String getIndexType() {
return this.indexType;
}
/**
* Optional. Gets the type of index (CLUSTERED, NONCLUSTERED, COLUMNSTORE,
* CLUSTERED COLUMNSTORE)
* @param indexTypeValue The IndexType value.
*/
public void setIndexType(final String indexTypeValue) {
this.indexType = indexTypeValue;
}
private Calendar lastModified;
/**
* Optional. Gets the UTC datetime of when was this resource last changed.
* @return The LastModified value.
*/
public Calendar getLastModified() {
return this.lastModified;
}
/**
* Optional. Gets the UTC datetime of when was this resource last changed.
* @param lastModifiedValue The LastModified value.
*/
public void setLastModified(final Calendar lastModifiedValue) {
this.lastModified = lastModifiedValue;
}
private ArrayList reportedImpact;
/**
* Optional. Gets the values reported after index action is complete.
* @return The ReportedImpact value.
*/
public ArrayList getReportedImpact() {
return this.reportedImpact;
}
/**
* Optional. Gets the values reported after index action is complete.
* @param reportedImpactValue The ReportedImpact value.
*/
public void setReportedImpact(final ArrayList reportedImpactValue) {
this.reportedImpact = reportedImpactValue;
}
private String schema;
/**
* Optional. Gets the schema where table to build index over resides
* @return The Schema value.
*/
public String getSchema() {
return this.schema;
}
/**
* Optional. Gets the schema where table to build index over resides
* @param schemaValue The Schema value.
*/
public void setSchema(final String schemaValue) {
this.schema = schemaValue;
}
private String state;
/**
* Optional. Gets the state recommendation is in. Current options are:
* 'Active', 'Pending', 'Executing', 'Verifying', 'Pending Revert',
* 'Reverting', 'Reverted', 'Ignored', 'Expired', 'Blocked', 'Success'.
* @return The State value.
*/
public String getState() {
return this.state;
}
/**
* Optional. Gets the state recommendation is in. Current options are:
* 'Active', 'Pending', 'Executing', 'Verifying', 'Pending Revert',
* 'Reverting', 'Reverted', 'Ignored', 'Expired', 'Blocked', 'Success'.
* @param stateValue The State value.
*/
public void setState(final String stateValue) {
this.state = stateValue;
}
private String table;
/**
* Optional. Gets the table on which to build index.
* @return The Table value.
*/
public String getTable() {
return this.table;
}
/**
* Optional. Gets the table on which to build index.
* @param tableValue The Table value.
*/
public void setTable(final String tableValue) {
this.table = tableValue;
}
/**
* Initializes a new instance of the RecommendedIndexProperties class.
*
*/
public RecommendedIndexProperties() {
this.setColumns(new LazyArrayList());
this.setEstimatedImpact(new LazyArrayList());
this.setIncludedColumns(new LazyArrayList());
this.setReportedImpact(new LazyArrayList());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy