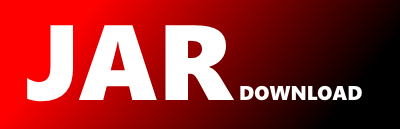
com.microsoft.azure.management.sql.DatabaseOperations Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-mgmt-sql Show documentation
Show all versions of azure-mgmt-sql Show documentation
This package contains Microsoft Azure SDK for SQL Management module.
/**
*
* Copyright (c) Microsoft and contributors. All rights reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
*
* See the License for the specific language governing permissions and
* limitations under the License.
*
*/
// Warning: This code was generated by a tool.
//
// Changes to this file may cause incorrect behavior and will be lost if the
// code is regenerated.
package com.microsoft.azure.management.sql;
import com.microsoft.azure.management.sql.models.DatabaseCreateOrUpdateParameters;
import com.microsoft.azure.management.sql.models.DatabaseCreateOrUpdateResponse;
import com.microsoft.azure.management.sql.models.DatabaseGetResponse;
import com.microsoft.azure.management.sql.models.DatabaseListResponse;
import com.microsoft.azure.management.sql.models.DatabaseMetricListResponse;
import com.microsoft.windowsazure.core.OperationResponse;
import com.microsoft.windowsazure.exception.ServiceException;
import java.io.IOException;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.Future;
/**
* Represents all the operations for operating on Azure SQL Databases. Contains
* operations to: Create, Retrieve, Update, and Delete databases, and also
* includes the ability to get the event logs for a database.
*/
public interface DatabaseOperations {
/**
* Begins creating a new Azure SQL Database or updating an existing Azure
* SQL Database. To determine the status of the operation call
* GetDatabaseOperationStatus.
*
* @param resourceGroupName Required. The name of the Resource Group to
* which the Azure SQL Database Server belongs.
* @param serverName Required. The name of the Azure SQL Database Server on
* which the database is hosted.
* @param databaseName Required. The name of the Azure SQL Database to be
* operated on (Updated or created).
* @param parameters Required. The required parameters for creating or
* updating a database.
* @throws IOException Signals that an I/O exception of some sort has
* occurred. This class is the general class of exceptions produced by
* failed or interrupted I/O operations.
* @throws ServiceException Thrown if an unexpected response is found.
* @return Response for long running Azure Sql Database operations.
*/
DatabaseCreateOrUpdateResponse beginCreateOrUpdate(String resourceGroupName, String serverName, String databaseName, DatabaseCreateOrUpdateParameters parameters) throws IOException, ServiceException;
/**
* Begins creating a new Azure SQL Database or updating an existing Azure
* SQL Database. To determine the status of the operation call
* GetDatabaseOperationStatus.
*
* @param resourceGroupName Required. The name of the Resource Group to
* which the Azure SQL Database Server belongs.
* @param serverName Required. The name of the Azure SQL Database Server on
* which the database is hosted.
* @param databaseName Required. The name of the Azure SQL Database to be
* operated on (Updated or created).
* @param parameters Required. The required parameters for creating or
* updating a database.
* @return Response for long running Azure Sql Database operations.
*/
Future beginCreateOrUpdateAsync(String resourceGroupName, String serverName, String databaseName, DatabaseCreateOrUpdateParameters parameters);
/**
* Creates a new Azure SQL Database or updates an existing Azure SQL
* Database.
*
* @param resourceGroupName Required. The name of the Resource Group to
* which the server belongs.
* @param serverName Required. The name of the Azure SQL Database Server on
* which the database is hosted.
* @param databaseName Required. The name of the Azure SQL Database to be
* operated on (Updated or created).
* @param parameters Required. The required parameters for creating or
* updating a database.
* @throws InterruptedException Thrown when a thread is waiting, sleeping,
* or otherwise occupied, and the thread is interrupted, either before or
* during the activity. Occasionally a method may wish to test whether the
* current thread has been interrupted, and if so, to immediately throw
* this exception. The following code can be used to achieve this effect:
* @throws ExecutionException Thrown when attempting to retrieve the result
* of a task that aborted by throwing an exception. This exception can be
* inspected using the Throwable.getCause() method.
* @throws IOException Thrown if there was an error setting up tracing for
* the request.
* @throws ServiceException Thrown if an unexpected response is found.
* @return Response for long running Azure Sql Database operations.
*/
DatabaseCreateOrUpdateResponse createOrUpdate(String resourceGroupName, String serverName, String databaseName, DatabaseCreateOrUpdateParameters parameters) throws InterruptedException, ExecutionException, IOException, ServiceException;
/**
* Creates a new Azure SQL Database or updates an existing Azure SQL
* Database.
*
* @param resourceGroupName Required. The name of the Resource Group to
* which the server belongs.
* @param serverName Required. The name of the Azure SQL Database Server on
* which the database is hosted.
* @param databaseName Required. The name of the Azure SQL Database to be
* operated on (Updated or created).
* @param parameters Required. The required parameters for creating or
* updating a database.
* @return Response for long running Azure Sql Database operations.
*/
Future createOrUpdateAsync(String resourceGroupName, String serverName, String databaseName, DatabaseCreateOrUpdateParameters parameters);
/**
* Deletes the Azure SQL Database with the given name.
*
* @param resourceGroupName Required. The name of the Resource Group to
* which the Azure SQL Database Server belongs.
* @param serverName Required. The name of the Azure SQL Database Server on
* which the Azure SQL Database Database is hosted.
* @param databaseName Required. The name of the Azure SQL Database to be
* deleted.
* @throws IOException Signals that an I/O exception of some sort has
* occurred. This class is the general class of exceptions produced by
* failed or interrupted I/O operations.
* @throws ServiceException Thrown if an unexpected response is found.
* @throws InterruptedException Thrown when a thread is waiting, sleeping,
* or otherwise occupied, and the thread is interrupted, either before or
* during the activity. Occasionally a method may wish to test whether the
* current thread has been interrupted, and if so, to immediately throw
* this exception. The following code can be used to achieve this effect:
* @throws ExecutionException Thrown when attempting to retrieve the result
* of a task that aborted by throwing an exception. This exception can be
* inspected using the Throwable.getCause() method.
* @return A standard service response including an HTTP status code and
* request ID.
*/
OperationResponse delete(String resourceGroupName, String serverName, String databaseName) throws IOException, ServiceException, InterruptedException, ExecutionException;
/**
* Deletes the Azure SQL Database with the given name.
*
* @param resourceGroupName Required. The name of the Resource Group to
* which the Azure SQL Database Server belongs.
* @param serverName Required. The name of the Azure SQL Database Server on
* which the Azure SQL Database Database is hosted.
* @param databaseName Required. The name of the Azure SQL Database to be
* deleted.
* @return A standard service response including an HTTP status code and
* request ID.
*/
Future deleteAsync(String resourceGroupName, String serverName, String databaseName);
/**
* Returns information about an Azure SQL Database.
*
* @param resourceGroupName Required. The name of the Resource Group to
* which the server belongs.
* @param serverName Required. The name of the Azure SQL Database Server on
* which the database is hosted.
* @param databaseName Required. The name of the Azure SQL Database to be
* retrieved.
* @throws IOException Signals that an I/O exception of some sort has
* occurred. This class is the general class of exceptions produced by
* failed or interrupted I/O operations.
* @throws ServiceException Thrown if an unexpected response is found.
* @return Represents the response to a Get Azure Sql Database request.
*/
DatabaseGetResponse get(String resourceGroupName, String serverName, String databaseName) throws IOException, ServiceException;
/**
* Returns information about an Azure SQL Database.
*
* @param resourceGroupName Required. The name of the Resource Group to
* which the server belongs.
* @param serverName Required. The name of the Azure SQL Database Server on
* which the database is hosted.
* @param databaseName Required. The name of the Azure SQL Database to be
* retrieved.
* @return Represents the response to a Get Azure Sql Database request.
*/
Future getAsync(String resourceGroupName, String serverName, String databaseName);
/**
* Returns information about an Azure SQL Database.
*
* @param resourceGroupName Required. The name of the Resource Group to
* which the server belongs.
* @param serverName Required. The name of the Azure SQL Database Server on
* which the database is hosted.
* @param databaseId Required. The Id of the Azure SQL Database to be
* retrieved.
* @throws IOException Signals that an I/O exception of some sort has
* occurred. This class is the general class of exceptions produced by
* failed or interrupted I/O operations.
* @throws ServiceException Thrown if an unexpected response is found.
* @return Represents the response to a List Azure Sql Database request.
*/
DatabaseListResponse getById(String resourceGroupName, String serverName, String databaseId) throws IOException, ServiceException;
/**
* Returns information about an Azure SQL Database.
*
* @param resourceGroupName Required. The name of the Resource Group to
* which the server belongs.
* @param serverName Required. The name of the Azure SQL Database Server on
* which the database is hosted.
* @param databaseId Required. The Id of the Azure SQL Database to be
* retrieved.
* @return Represents the response to a List Azure Sql Database request.
*/
Future getByIdAsync(String resourceGroupName, String serverName, String databaseId);
/**
* Gets the status of an Azure Sql Database create or update operation.
*
* @param operationStatusLink Required. Location value returned by the Begin
* operation
* @throws IOException Signals that an I/O exception of some sort has
* occurred. This class is the general class of exceptions produced by
* failed or interrupted I/O operations.
* @throws ServiceException Thrown if an unexpected response is found.
* @return Response for long running Azure Sql Database operations.
*/
DatabaseCreateOrUpdateResponse getDatabaseOperationStatus(String operationStatusLink) throws IOException, ServiceException;
/**
* Gets the status of an Azure Sql Database create or update operation.
*
* @param operationStatusLink Required. Location value returned by the Begin
* operation
* @return Response for long running Azure Sql Database operations.
*/
Future getDatabaseOperationStatusAsync(String operationStatusLink);
/**
* Returns information about an Azure SQL Database.
*
* @param resourceGroupName Required. The name of the Resource Group to
* which the server belongs.
* @param serverName Required. The name of the Azure SQL Database Server on
* which the database is hosted.
* @param databaseName Required. The name of the Azure SQL Database to be
* retrieved.
* @param expand Required. The comma separated list of child objects that we
* want to expand on in response.
* @throws IOException Signals that an I/O exception of some sort has
* occurred. This class is the general class of exceptions produced by
* failed or interrupted I/O operations.
* @throws ServiceException Thrown if an unexpected response is found.
* @return Represents the response to a Get Azure Sql Database request.
*/
DatabaseGetResponse getExpanded(String resourceGroupName, String serverName, String databaseName, String expand) throws IOException, ServiceException;
/**
* Returns information about an Azure SQL Database.
*
* @param resourceGroupName Required. The name of the Resource Group to
* which the server belongs.
* @param serverName Required. The name of the Azure SQL Database Server on
* which the database is hosted.
* @param databaseName Required. The name of the Azure SQL Database to be
* retrieved.
* @param expand Required. The comma separated list of child objects that we
* want to expand on in response.
* @return Represents the response to a Get Azure Sql Database request.
*/
Future getExpandedAsync(String resourceGroupName, String serverName, String databaseName, String expand);
/**
* Returns information about Azure SQL Databases.
*
* @param resourceGroupName Required. The name of the Resource Group to
* which the server belongs.
* @param serverName Required. The name of the Azure SQL Database Server in
* which the Azure SQL Databases are hosted.
* @throws IOException Signals that an I/O exception of some sort has
* occurred. This class is the general class of exceptions produced by
* failed or interrupted I/O operations.
* @throws ServiceException Thrown if an unexpected response is found.
* @return Represents the response to a List Azure Sql Database request.
*/
DatabaseListResponse list(String resourceGroupName, String serverName) throws IOException, ServiceException;
/**
* Returns information about Azure SQL Databases.
*
* @param resourceGroupName Required. The name of the Resource Group to
* which the server belongs.
* @param serverName Required. The name of the Azure SQL Database Server in
* which the Azure SQL Databases are hosted.
* @return Represents the response to a List Azure Sql Database request.
*/
Future listAsync(String resourceGroupName, String serverName);
/**
* Returns information about Azure SQL Databases.
*
* @param resourceGroupName Required. The name of the Resource Group to
* which the server belongs.
* @param serverName Required. The name of the Azure SQL Database Server in
* which the Azure SQL Databases are hosted.
* @param expand Required. The comma separated list of child objects that we
* want to expand on in response.
* @throws IOException Signals that an I/O exception of some sort has
* occurred. This class is the general class of exceptions produced by
* failed or interrupted I/O operations.
* @throws ServiceException Thrown if an unexpected response is found.
* @return Represents the response to a List Azure Sql Database request.
*/
DatabaseListResponse listExpanded(String resourceGroupName, String serverName, String expand) throws IOException, ServiceException;
/**
* Returns information about Azure SQL Databases.
*
* @param resourceGroupName Required. The name of the Resource Group to
* which the server belongs.
* @param serverName Required. The name of the Azure SQL Database Server in
* which the Azure SQL Databases are hosted.
* @param expand Required. The comma separated list of child objects that we
* want to expand on in response.
* @return Represents the response to a List Azure Sql Database request.
*/
Future listExpandedAsync(String resourceGroupName, String serverName, String expand);
/**
* Returns information about Azure SQL Database usages.
*
* @param resourceGroupName Required. The name of the Resource Group to
* which the server belongs.
* @param serverName Required. The name of the Azure SQL Database Server in
* which the Azure SQL Databases are hosted.
* @param databaseName Required. The name of the Azure SQL Database.
* @throws IOException Signals that an I/O exception of some sort has
* occurred. This class is the general class of exceptions produced by
* failed or interrupted I/O operations.
* @throws ServiceException Thrown if an unexpected response is found.
* @return Represents the response to a List Azure Sql Database metrics
* request.
*/
DatabaseMetricListResponse listUsages(String resourceGroupName, String serverName, String databaseName) throws IOException, ServiceException;
/**
* Returns information about Azure SQL Database usages.
*
* @param resourceGroupName Required. The name of the Resource Group to
* which the server belongs.
* @param serverName Required. The name of the Azure SQL Database Server in
* which the Azure SQL Databases are hosted.
* @param databaseName Required. The name of the Azure SQL Database.
* @return Represents the response to a List Azure Sql Database metrics
* request.
*/
Future listUsagesAsync(String resourceGroupName, String serverName, String databaseName);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy