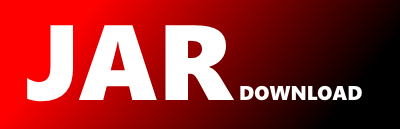
com.microsoft.azure.management.sql.RecommendedIndexOperationsImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-mgmt-sql Show documentation
Show all versions of azure-mgmt-sql Show documentation
This package contains Microsoft Azure SDK for SQL Management module.
/**
*
* Copyright (c) Microsoft and contributors. All rights reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
*
* See the License for the specific language governing permissions and
* limitations under the License.
*
*/
// Warning: This code was generated by a tool.
//
// Changes to this file may cause incorrect behavior and will be lost if the
// code is regenerated.
package com.microsoft.azure.management.sql;
import com.microsoft.azure.management.sql.models.ErrorResponse;
import com.microsoft.azure.management.sql.models.OperationImpact;
import com.microsoft.azure.management.sql.models.RecommendedIndex;
import com.microsoft.azure.management.sql.models.RecommendedIndexGetResponse;
import com.microsoft.azure.management.sql.models.RecommendedIndexProperties;
import com.microsoft.azure.management.sql.models.RecommendedIndexUpdateParameters;
import com.microsoft.azure.management.sql.models.RecommendedIndexUpdateResponse;
import com.microsoft.windowsazure.core.OperationStatus;
import com.microsoft.windowsazure.core.ServiceOperations;
import com.microsoft.windowsazure.core.utils.CollectionStringBuilder;
import com.microsoft.windowsazure.exception.ServiceException;
import com.microsoft.windowsazure.tracing.CloudTracing;
import org.apache.commons.io.IOUtils;
import org.apache.http.HttpResponse;
import org.apache.http.HttpStatus;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.client.methods.HttpPut;
import org.apache.http.entity.StringEntity;
import org.codehaus.jackson.JsonNode;
import org.codehaus.jackson.map.ObjectMapper;
import org.codehaus.jackson.node.ArrayNode;
import org.codehaus.jackson.node.NullNode;
import org.codehaus.jackson.node.ObjectNode;
import javax.xml.bind.DatatypeConverter;
import java.io.IOException;
import java.io.InputStream;
import java.io.StringWriter;
import java.net.URLEncoder;
import java.util.ArrayList;
import java.util.Calendar;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
import java.util.concurrent.Callable;
import java.util.concurrent.Future;
/**
* Represents all the operations for managing recommended indexes on Azure SQL
* Databases. Contains operations to retrieve recommended index and update
* state.
*/
public class RecommendedIndexOperationsImpl implements ServiceOperations, RecommendedIndexOperations {
/**
* Initializes a new instance of the RecommendedIndexOperationsImpl class.
*
* @param client Reference to the service client.
*/
RecommendedIndexOperationsImpl(SqlManagementClientImpl client) {
this.client = client;
}
private SqlManagementClientImpl client;
/**
* Gets a reference to the
* microsoft.azure.management.sql.SqlManagementClientImpl.
* @return The Client value.
*/
public SqlManagementClientImpl getClient() {
return this.client;
}
/**
* Returns details on recommended index.
*
* @param resourceGroupName Required. The name of the Resource Group.
* @param serverName Required. The name of the Azure SQL server.
* @param databaseName Required. The name of the Azure SQL database.
* @param schemaName Required. The name of the Azure SQL database schema.
* @param tableName Required. The name of the Azure SQL database table.
* @param indexName Required. The name of the Azure SQL database recommended
* index.
* @return Represents the response to a get recommended index request.
*/
@Override
public Future getAsync(final String resourceGroupName, final String serverName, final String databaseName, final String schemaName, final String tableName, final String indexName) {
return this.getClient().getExecutorService().submit(new Callable() {
@Override
public RecommendedIndexGetResponse call() throws Exception {
return get(resourceGroupName, serverName, databaseName, schemaName, tableName, indexName);
}
});
}
/**
* Returns details on recommended index.
*
* @param resourceGroupName Required. The name of the Resource Group.
* @param serverName Required. The name of the Azure SQL server.
* @param databaseName Required. The name of the Azure SQL database.
* @param schemaName Required. The name of the Azure SQL database schema.
* @param tableName Required. The name of the Azure SQL database table.
* @param indexName Required. The name of the Azure SQL database recommended
* index.
* @throws IOException Signals that an I/O exception of some sort has
* occurred. This class is the general class of exceptions produced by
* failed or interrupted I/O operations.
* @throws ServiceException Thrown if an unexpected response is found.
* @return Represents the response to a get recommended index request.
*/
@Override
public RecommendedIndexGetResponse get(String resourceGroupName, String serverName, String databaseName, String schemaName, String tableName, String indexName) throws IOException, ServiceException {
// Validate
if (resourceGroupName == null) {
throw new NullPointerException("resourceGroupName");
}
if (serverName == null) {
throw new NullPointerException("serverName");
}
if (databaseName == null) {
throw new NullPointerException("databaseName");
}
if (schemaName == null) {
throw new NullPointerException("schemaName");
}
if (tableName == null) {
throw new NullPointerException("tableName");
}
if (indexName == null) {
throw new NullPointerException("indexName");
}
// Tracing
boolean shouldTrace = CloudTracing.getIsEnabled();
String invocationId = null;
if (shouldTrace) {
invocationId = Long.toString(CloudTracing.getNextInvocationId());
HashMap tracingParameters = new HashMap();
tracingParameters.put("resourceGroupName", resourceGroupName);
tracingParameters.put("serverName", serverName);
tracingParameters.put("databaseName", databaseName);
tracingParameters.put("schemaName", schemaName);
tracingParameters.put("tableName", tableName);
tracingParameters.put("indexName", indexName);
CloudTracing.enter(invocationId, this, "getAsync", tracingParameters);
}
// Construct URL
String url = "";
url = url + "/subscriptions/";
if (this.getClient().getCredentials().getSubscriptionId() != null) {
url = url + URLEncoder.encode(this.getClient().getCredentials().getSubscriptionId(), "UTF-8");
}
url = url + "/resourceGroups/";
url = url + URLEncoder.encode(resourceGroupName, "UTF-8");
url = url + "/providers/";
url = url + "Microsoft.Sql";
url = url + "/servers/";
url = url + URLEncoder.encode(serverName, "UTF-8");
url = url + "/databases/";
url = url + URLEncoder.encode(databaseName, "UTF-8");
url = url + "/schemas/";
url = url + URLEncoder.encode(schemaName, "UTF-8");
url = url + "/tables/";
url = url + URLEncoder.encode(tableName, "UTF-8");
url = url + "/recommendedIndexes/";
url = url + URLEncoder.encode(indexName, "UTF-8");
ArrayList queryParameters = new ArrayList();
queryParameters.add("api-version=" + "2014-04-01");
if (queryParameters.size() > 0) {
url = url + "?" + CollectionStringBuilder.join(queryParameters, "&");
}
String baseUrl = this.getClient().getBaseUri().toString();
// Trim '/' character from the end of baseUrl and beginning of url.
if (baseUrl.charAt(baseUrl.length() - 1) == '/') {
baseUrl = baseUrl.substring(0, (baseUrl.length() - 1) + 0);
}
if (url.charAt(0) == '/') {
url = url.substring(1);
}
url = baseUrl + "/" + url;
url = url.replace(" ", "%20");
// Create HTTP transport objects
HttpGet httpRequest = new HttpGet(url);
// Set Headers
// Send Request
HttpResponse httpResponse = null;
try {
if (shouldTrace) {
CloudTracing.sendRequest(invocationId, httpRequest);
}
httpResponse = this.getClient().getHttpClient().execute(httpRequest);
if (shouldTrace) {
CloudTracing.receiveResponse(invocationId, httpResponse);
}
int statusCode = httpResponse.getStatusLine().getStatusCode();
if (statusCode != HttpStatus.SC_OK) {
ServiceException ex = ServiceException.createFromJson(httpRequest, null, httpResponse, httpResponse.getEntity());
if (shouldTrace) {
CloudTracing.error(invocationId, ex);
}
throw ex;
}
// Create Result
RecommendedIndexGetResponse result = null;
// Deserialize Response
if (statusCode == HttpStatus.SC_OK) {
InputStream responseContent = httpResponse.getEntity().getContent();
result = new RecommendedIndexGetResponse();
ObjectMapper objectMapper = new ObjectMapper();
JsonNode responseDoc = null;
String responseDocContent = IOUtils.toString(responseContent);
if (responseDocContent == null == false && responseDocContent.length() > 0) {
responseDoc = objectMapper.readTree(responseDocContent);
}
if (responseDoc != null && responseDoc instanceof NullNode == false) {
RecommendedIndex recommendedIndexInstance = new RecommendedIndex();
result.setRecommendedIndex(recommendedIndexInstance);
JsonNode propertiesValue = responseDoc.get("properties");
if (propertiesValue != null && propertiesValue instanceof NullNode == false) {
RecommendedIndexProperties propertiesInstance = new RecommendedIndexProperties();
recommendedIndexInstance.setProperties(propertiesInstance);
JsonNode actionValue = propertiesValue.get("action");
if (actionValue != null && actionValue instanceof NullNode == false) {
String actionInstance;
actionInstance = actionValue.getTextValue();
propertiesInstance.setAction(actionInstance);
}
JsonNode stateValue = propertiesValue.get("state");
if (stateValue != null && stateValue instanceof NullNode == false) {
String stateInstance;
stateInstance = stateValue.getTextValue();
propertiesInstance.setState(stateInstance);
}
JsonNode createdValue = propertiesValue.get("created");
if (createdValue != null && createdValue instanceof NullNode == false) {
Calendar createdInstance;
createdInstance = DatatypeConverter.parseDateTime(createdValue.getTextValue());
propertiesInstance.setCreated(createdInstance);
}
JsonNode lastModifiedValue = propertiesValue.get("lastModified");
if (lastModifiedValue != null && lastModifiedValue instanceof NullNode == false) {
Calendar lastModifiedInstance;
lastModifiedInstance = DatatypeConverter.parseDateTime(lastModifiedValue.getTextValue());
propertiesInstance.setLastModified(lastModifiedInstance);
}
JsonNode indexTypeValue = propertiesValue.get("indexType");
if (indexTypeValue != null && indexTypeValue instanceof NullNode == false) {
String indexTypeInstance;
indexTypeInstance = indexTypeValue.getTextValue();
propertiesInstance.setIndexType(indexTypeInstance);
}
JsonNode schemaValue = propertiesValue.get("schema");
if (schemaValue != null && schemaValue instanceof NullNode == false) {
String schemaInstance;
schemaInstance = schemaValue.getTextValue();
propertiesInstance.setSchema(schemaInstance);
}
JsonNode tableValue = propertiesValue.get("table");
if (tableValue != null && tableValue instanceof NullNode == false) {
String tableInstance;
tableInstance = tableValue.getTextValue();
propertiesInstance.setTable(tableInstance);
}
JsonNode columnsArray = propertiesValue.get("columns");
if (columnsArray != null && columnsArray instanceof NullNode == false) {
for (JsonNode columnsValue : ((ArrayNode) columnsArray)) {
propertiesInstance.getColumns().add(columnsValue.getTextValue());
}
}
JsonNode includedColumnsArray = propertiesValue.get("includedColumns");
if (includedColumnsArray != null && includedColumnsArray instanceof NullNode == false) {
for (JsonNode includedColumnsValue : ((ArrayNode) includedColumnsArray)) {
propertiesInstance.getIncludedColumns().add(includedColumnsValue.getTextValue());
}
}
JsonNode indexScriptValue = propertiesValue.get("indexScript");
if (indexScriptValue != null && indexScriptValue instanceof NullNode == false) {
String indexScriptInstance;
indexScriptInstance = indexScriptValue.getTextValue();
propertiesInstance.setIndexScript(indexScriptInstance);
}
JsonNode estimatedImpactArray = propertiesValue.get("estimatedImpact");
if (estimatedImpactArray != null && estimatedImpactArray instanceof NullNode == false) {
for (JsonNode estimatedImpactValue : ((ArrayNode) estimatedImpactArray)) {
OperationImpact operationImpactInstance = new OperationImpact();
propertiesInstance.getEstimatedImpact().add(operationImpactInstance);
JsonNode nameValue = estimatedImpactValue.get("name");
if (nameValue != null && nameValue instanceof NullNode == false) {
String nameInstance;
nameInstance = nameValue.getTextValue();
operationImpactInstance.setName(nameInstance);
}
JsonNode unitValue = estimatedImpactValue.get("unit");
if (unitValue != null && unitValue instanceof NullNode == false) {
String unitInstance;
unitInstance = unitValue.getTextValue();
operationImpactInstance.setUnit(unitInstance);
}
JsonNode changeValueAbsoluteValue = estimatedImpactValue.get("changeValueAbsolute");
if (changeValueAbsoluteValue != null && changeValueAbsoluteValue instanceof NullNode == false) {
double changeValueAbsoluteInstance;
changeValueAbsoluteInstance = changeValueAbsoluteValue.getDoubleValue();
operationImpactInstance.setChangeValueAbsolute(changeValueAbsoluteInstance);
}
JsonNode changeValueRelativeValue = estimatedImpactValue.get("changeValueRelative");
if (changeValueRelativeValue != null && changeValueRelativeValue instanceof NullNode == false) {
double changeValueRelativeInstance;
changeValueRelativeInstance = changeValueRelativeValue.getDoubleValue();
operationImpactInstance.setChangeValueRelative(changeValueRelativeInstance);
}
}
}
JsonNode reportedImpactArray = propertiesValue.get("reportedImpact");
if (reportedImpactArray != null && reportedImpactArray instanceof NullNode == false) {
for (JsonNode reportedImpactValue : ((ArrayNode) reportedImpactArray)) {
OperationImpact operationImpactInstance2 = new OperationImpact();
propertiesInstance.getReportedImpact().add(operationImpactInstance2);
JsonNode nameValue2 = reportedImpactValue.get("name");
if (nameValue2 != null && nameValue2 instanceof NullNode == false) {
String nameInstance2;
nameInstance2 = nameValue2.getTextValue();
operationImpactInstance2.setName(nameInstance2);
}
JsonNode unitValue2 = reportedImpactValue.get("unit");
if (unitValue2 != null && unitValue2 instanceof NullNode == false) {
String unitInstance2;
unitInstance2 = unitValue2.getTextValue();
operationImpactInstance2.setUnit(unitInstance2);
}
JsonNode changeValueAbsoluteValue2 = reportedImpactValue.get("changeValueAbsolute");
if (changeValueAbsoluteValue2 != null && changeValueAbsoluteValue2 instanceof NullNode == false) {
double changeValueAbsoluteInstance2;
changeValueAbsoluteInstance2 = changeValueAbsoluteValue2.getDoubleValue();
operationImpactInstance2.setChangeValueAbsolute(changeValueAbsoluteInstance2);
}
JsonNode changeValueRelativeValue2 = reportedImpactValue.get("changeValueRelative");
if (changeValueRelativeValue2 != null && changeValueRelativeValue2 instanceof NullNode == false) {
double changeValueRelativeInstance2;
changeValueRelativeInstance2 = changeValueRelativeValue2.getDoubleValue();
operationImpactInstance2.setChangeValueRelative(changeValueRelativeInstance2);
}
}
}
}
JsonNode idValue = responseDoc.get("id");
if (idValue != null && idValue instanceof NullNode == false) {
String idInstance;
idInstance = idValue.getTextValue();
recommendedIndexInstance.setId(idInstance);
}
JsonNode nameValue3 = responseDoc.get("name");
if (nameValue3 != null && nameValue3 instanceof NullNode == false) {
String nameInstance3;
nameInstance3 = nameValue3.getTextValue();
recommendedIndexInstance.setName(nameInstance3);
}
JsonNode typeValue = responseDoc.get("type");
if (typeValue != null && typeValue instanceof NullNode == false) {
String typeInstance;
typeInstance = typeValue.getTextValue();
recommendedIndexInstance.setType(typeInstance);
}
JsonNode locationValue = responseDoc.get("location");
if (locationValue != null && locationValue instanceof NullNode == false) {
String locationInstance;
locationInstance = locationValue.getTextValue();
recommendedIndexInstance.setLocation(locationInstance);
}
JsonNode tagsSequenceElement = ((JsonNode) responseDoc.get("tags"));
if (tagsSequenceElement != null && tagsSequenceElement instanceof NullNode == false) {
Iterator> itr = tagsSequenceElement.getFields();
while (itr.hasNext()) {
Map.Entry property = itr.next();
String tagsKey = property.getKey();
String tagsValue = property.getValue().getTextValue();
recommendedIndexInstance.getTags().put(tagsKey, tagsValue);
}
}
}
}
result.setStatusCode(statusCode);
if (httpResponse.getHeaders("x-ms-request-id").length > 0) {
result.setRequestId(httpResponse.getFirstHeader("x-ms-request-id").getValue());
}
if (shouldTrace) {
CloudTracing.exit(invocationId, result);
}
return result;
} finally {
if (httpResponse != null && httpResponse.getEntity() != null) {
httpResponse.getEntity().getContent().close();
}
}
}
/**
* We execute or cancel index operations by updating index state. Allowed
* state transitions are :Active -> Pending - Start index
* creation processPending -> Active - Cancel index
* creationActive/Pending -> Ignored - Ignore index
* recommendation so it will no longer show in active
* recommendationsIgnored -> Active - Restore index
* recommendationSuccess -> Pending Revert - Revert index that
* has been createdPending Revert -> Revert Canceled - Cancel index
* revert operation
*
* @param resourceGroupName Required. The name of the Resource Group to
* which the Azure SQL Database Server belongs.
* @param serverName Required. The name of the Azure SQL Database Server on
* which the database is hosted.
* @param databaseName Required. The name of the Azure SQL Database.
* @param schemaName Required. The name of the Azure SQL Database schema.
* @param tableName Required. The name of the Azure SQL Database table.
* @param indexName Required. The name of the Azure SQL Database recommended
* index.
* @param parameters Required. The required parameters for updating index
* state.
* @return Represents the response to a get recommended index request.
*/
@Override
public Future updateAsync(final String resourceGroupName, final String serverName, final String databaseName, final String schemaName, final String tableName, final String indexName, final RecommendedIndexUpdateParameters parameters) {
return this.getClient().getExecutorService().submit(new Callable() {
@Override
public RecommendedIndexUpdateResponse call() throws Exception {
return update(resourceGroupName, serverName, databaseName, schemaName, tableName, indexName, parameters);
}
});
}
/**
* We execute or cancel index operations by updating index state. Allowed
* state transitions are :Active -> Pending - Start index
* creation processPending -> Active - Cancel index
* creationActive/Pending -> Ignored - Ignore index
* recommendation so it will no longer show in active
* recommendationsIgnored -> Active - Restore index
* recommendationSuccess -> Pending Revert - Revert index that
* has been createdPending Revert -> Revert Canceled - Cancel index
* revert operation
*
* @param resourceGroupName Required. The name of the Resource Group to
* which the Azure SQL Database Server belongs.
* @param serverName Required. The name of the Azure SQL Database Server on
* which the database is hosted.
* @param databaseName Required. The name of the Azure SQL Database.
* @param schemaName Required. The name of the Azure SQL Database schema.
* @param tableName Required. The name of the Azure SQL Database table.
* @param indexName Required. The name of the Azure SQL Database recommended
* index.
* @param parameters Required. The required parameters for updating index
* state.
* @throws IOException Signals that an I/O exception of some sort has
* occurred. This class is the general class of exceptions produced by
* failed or interrupted I/O operations.
* @throws ServiceException Thrown if an unexpected response is found.
* @return Represents the response to a get recommended index request.
*/
@Override
public RecommendedIndexUpdateResponse update(String resourceGroupName, String serverName, String databaseName, String schemaName, String tableName, String indexName, RecommendedIndexUpdateParameters parameters) throws IOException, ServiceException {
// Validate
if (resourceGroupName == null) {
throw new NullPointerException("resourceGroupName");
}
if (serverName == null) {
throw new NullPointerException("serverName");
}
if (databaseName == null) {
throw new NullPointerException("databaseName");
}
if (schemaName == null) {
throw new NullPointerException("schemaName");
}
if (tableName == null) {
throw new NullPointerException("tableName");
}
if (indexName == null) {
throw new NullPointerException("indexName");
}
if (parameters == null) {
throw new NullPointerException("parameters");
}
if (parameters.getProperties() == null) {
throw new NullPointerException("parameters.Properties");
}
// Tracing
boolean shouldTrace = CloudTracing.getIsEnabled();
String invocationId = null;
if (shouldTrace) {
invocationId = Long.toString(CloudTracing.getNextInvocationId());
HashMap tracingParameters = new HashMap();
tracingParameters.put("resourceGroupName", resourceGroupName);
tracingParameters.put("serverName", serverName);
tracingParameters.put("databaseName", databaseName);
tracingParameters.put("schemaName", schemaName);
tracingParameters.put("tableName", tableName);
tracingParameters.put("indexName", indexName);
tracingParameters.put("parameters", parameters);
CloudTracing.enter(invocationId, this, "updateAsync", tracingParameters);
}
// Construct URL
String url = "";
url = url + "/subscriptions/";
if (this.getClient().getCredentials().getSubscriptionId() != null) {
url = url + URLEncoder.encode(this.getClient().getCredentials().getSubscriptionId(), "UTF-8");
}
url = url + "/resourceGroups/";
url = url + URLEncoder.encode(resourceGroupName, "UTF-8");
url = url + "/providers/";
url = url + "Microsoft.Sql";
url = url + "/servers/";
url = url + URLEncoder.encode(serverName, "UTF-8");
url = url + "/databases/";
url = url + URLEncoder.encode(databaseName, "UTF-8");
url = url + "/schemas/";
url = url + URLEncoder.encode(schemaName, "UTF-8");
url = url + "/tables/";
url = url + URLEncoder.encode(tableName, "UTF-8");
url = url + "/recommendedIndexes/";
url = url + URLEncoder.encode(indexName, "UTF-8");
ArrayList queryParameters = new ArrayList();
queryParameters.add("api-version=" + "2014-04-01");
if (queryParameters.size() > 0) {
url = url + "?" + CollectionStringBuilder.join(queryParameters, "&");
}
String baseUrl = this.getClient().getBaseUri().toString();
// Trim '/' character from the end of baseUrl and beginning of url.
if (baseUrl.charAt(baseUrl.length() - 1) == '/') {
baseUrl = baseUrl.substring(0, (baseUrl.length() - 1) + 0);
}
if (url.charAt(0) == '/') {
url = url.substring(1);
}
url = baseUrl + "/" + url;
url = url.replace(" ", "%20");
// Create HTTP transport objects
HttpPut httpRequest = new HttpPut(url);
// Set Headers
httpRequest.setHeader("Content-Type", "application/json; charset=utf-8");
// Serialize Request
String requestContent = null;
JsonNode requestDoc = null;
ObjectMapper objectMapper = new ObjectMapper();
ObjectNode recommendedIndexUpdateParametersValue = objectMapper.createObjectNode();
requestDoc = recommendedIndexUpdateParametersValue;
ObjectNode propertiesValue = objectMapper.createObjectNode();
((ObjectNode) recommendedIndexUpdateParametersValue).put("properties", propertiesValue);
if (parameters.getProperties().getState() != null) {
((ObjectNode) propertiesValue).put("state", parameters.getProperties().getState());
}
StringWriter stringWriter = new StringWriter();
objectMapper.writeValue(stringWriter, requestDoc);
requestContent = stringWriter.toString();
StringEntity entity = new StringEntity(requestContent);
httpRequest.setEntity(entity);
httpRequest.setHeader("Content-Type", "application/json; charset=utf-8");
// Send Request
HttpResponse httpResponse = null;
try {
if (shouldTrace) {
CloudTracing.sendRequest(invocationId, httpRequest);
}
httpResponse = this.getClient().getHttpClient().execute(httpRequest);
if (shouldTrace) {
CloudTracing.receiveResponse(invocationId, httpResponse);
}
int statusCode = httpResponse.getStatusLine().getStatusCode();
if (statusCode != HttpStatus.SC_OK) {
ServiceException ex = ServiceException.createFromJson(httpRequest, requestContent, httpResponse, httpResponse.getEntity());
if (shouldTrace) {
CloudTracing.error(invocationId, ex);
}
throw ex;
}
// Create Result
RecommendedIndexUpdateResponse result = null;
// Deserialize Response
if (statusCode == HttpStatus.SC_OK) {
InputStream responseContent = httpResponse.getEntity().getContent();
result = new RecommendedIndexUpdateResponse();
JsonNode responseDoc = null;
String responseDocContent = IOUtils.toString(responseContent);
if (responseDocContent == null == false && responseDocContent.length() > 0) {
responseDoc = objectMapper.readTree(responseDocContent);
}
if (responseDoc != null && responseDoc instanceof NullNode == false) {
ErrorResponse errorInstance = new ErrorResponse();
result.setError(errorInstance);
JsonNode codeValue = responseDoc.get("code");
if (codeValue != null && codeValue instanceof NullNode == false) {
String codeInstance;
codeInstance = codeValue.getTextValue();
errorInstance.setCode(codeInstance);
}
JsonNode messageValue = responseDoc.get("message");
if (messageValue != null && messageValue instanceof NullNode == false) {
String messageInstance;
messageInstance = messageValue.getTextValue();
errorInstance.setMessage(messageInstance);
}
JsonNode targetValue = responseDoc.get("target");
if (targetValue != null && targetValue instanceof NullNode == false) {
String targetInstance;
targetInstance = targetValue.getTextValue();
errorInstance.setTarget(targetInstance);
}
RecommendedIndex recommendedIndexInstance = new RecommendedIndex();
result.setRecommendedIndex(recommendedIndexInstance);
JsonNode propertiesValue2 = responseDoc.get("properties");
if (propertiesValue2 != null && propertiesValue2 instanceof NullNode == false) {
RecommendedIndexProperties propertiesInstance = new RecommendedIndexProperties();
recommendedIndexInstance.setProperties(propertiesInstance);
JsonNode actionValue = propertiesValue2.get("action");
if (actionValue != null && actionValue instanceof NullNode == false) {
String actionInstance;
actionInstance = actionValue.getTextValue();
propertiesInstance.setAction(actionInstance);
}
JsonNode stateValue = propertiesValue2.get("state");
if (stateValue != null && stateValue instanceof NullNode == false) {
String stateInstance;
stateInstance = stateValue.getTextValue();
propertiesInstance.setState(stateInstance);
}
JsonNode createdValue = propertiesValue2.get("created");
if (createdValue != null && createdValue instanceof NullNode == false) {
Calendar createdInstance;
createdInstance = DatatypeConverter.parseDateTime(createdValue.getTextValue());
propertiesInstance.setCreated(createdInstance);
}
JsonNode lastModifiedValue = propertiesValue2.get("lastModified");
if (lastModifiedValue != null && lastModifiedValue instanceof NullNode == false) {
Calendar lastModifiedInstance;
lastModifiedInstance = DatatypeConverter.parseDateTime(lastModifiedValue.getTextValue());
propertiesInstance.setLastModified(lastModifiedInstance);
}
JsonNode indexTypeValue = propertiesValue2.get("indexType");
if (indexTypeValue != null && indexTypeValue instanceof NullNode == false) {
String indexTypeInstance;
indexTypeInstance = indexTypeValue.getTextValue();
propertiesInstance.setIndexType(indexTypeInstance);
}
JsonNode schemaValue = propertiesValue2.get("schema");
if (schemaValue != null && schemaValue instanceof NullNode == false) {
String schemaInstance;
schemaInstance = schemaValue.getTextValue();
propertiesInstance.setSchema(schemaInstance);
}
JsonNode tableValue = propertiesValue2.get("table");
if (tableValue != null && tableValue instanceof NullNode == false) {
String tableInstance;
tableInstance = tableValue.getTextValue();
propertiesInstance.setTable(tableInstance);
}
JsonNode columnsArray = propertiesValue2.get("columns");
if (columnsArray != null && columnsArray instanceof NullNode == false) {
for (JsonNode columnsValue : ((ArrayNode) columnsArray)) {
propertiesInstance.getColumns().add(columnsValue.getTextValue());
}
}
JsonNode includedColumnsArray = propertiesValue2.get("includedColumns");
if (includedColumnsArray != null && includedColumnsArray instanceof NullNode == false) {
for (JsonNode includedColumnsValue : ((ArrayNode) includedColumnsArray)) {
propertiesInstance.getIncludedColumns().add(includedColumnsValue.getTextValue());
}
}
JsonNode indexScriptValue = propertiesValue2.get("indexScript");
if (indexScriptValue != null && indexScriptValue instanceof NullNode == false) {
String indexScriptInstance;
indexScriptInstance = indexScriptValue.getTextValue();
propertiesInstance.setIndexScript(indexScriptInstance);
}
JsonNode estimatedImpactArray = propertiesValue2.get("estimatedImpact");
if (estimatedImpactArray != null && estimatedImpactArray instanceof NullNode == false) {
for (JsonNode estimatedImpactValue : ((ArrayNode) estimatedImpactArray)) {
OperationImpact operationImpactInstance = new OperationImpact();
propertiesInstance.getEstimatedImpact().add(operationImpactInstance);
JsonNode nameValue = estimatedImpactValue.get("name");
if (nameValue != null && nameValue instanceof NullNode == false) {
String nameInstance;
nameInstance = nameValue.getTextValue();
operationImpactInstance.setName(nameInstance);
}
JsonNode unitValue = estimatedImpactValue.get("unit");
if (unitValue != null && unitValue instanceof NullNode == false) {
String unitInstance;
unitInstance = unitValue.getTextValue();
operationImpactInstance.setUnit(unitInstance);
}
JsonNode changeValueAbsoluteValue = estimatedImpactValue.get("changeValueAbsolute");
if (changeValueAbsoluteValue != null && changeValueAbsoluteValue instanceof NullNode == false) {
double changeValueAbsoluteInstance;
changeValueAbsoluteInstance = changeValueAbsoluteValue.getDoubleValue();
operationImpactInstance.setChangeValueAbsolute(changeValueAbsoluteInstance);
}
JsonNode changeValueRelativeValue = estimatedImpactValue.get("changeValueRelative");
if (changeValueRelativeValue != null && changeValueRelativeValue instanceof NullNode == false) {
double changeValueRelativeInstance;
changeValueRelativeInstance = changeValueRelativeValue.getDoubleValue();
operationImpactInstance.setChangeValueRelative(changeValueRelativeInstance);
}
}
}
JsonNode reportedImpactArray = propertiesValue2.get("reportedImpact");
if (reportedImpactArray != null && reportedImpactArray instanceof NullNode == false) {
for (JsonNode reportedImpactValue : ((ArrayNode) reportedImpactArray)) {
OperationImpact operationImpactInstance2 = new OperationImpact();
propertiesInstance.getReportedImpact().add(operationImpactInstance2);
JsonNode nameValue2 = reportedImpactValue.get("name");
if (nameValue2 != null && nameValue2 instanceof NullNode == false) {
String nameInstance2;
nameInstance2 = nameValue2.getTextValue();
operationImpactInstance2.setName(nameInstance2);
}
JsonNode unitValue2 = reportedImpactValue.get("unit");
if (unitValue2 != null && unitValue2 instanceof NullNode == false) {
String unitInstance2;
unitInstance2 = unitValue2.getTextValue();
operationImpactInstance2.setUnit(unitInstance2);
}
JsonNode changeValueAbsoluteValue2 = reportedImpactValue.get("changeValueAbsolute");
if (changeValueAbsoluteValue2 != null && changeValueAbsoluteValue2 instanceof NullNode == false) {
double changeValueAbsoluteInstance2;
changeValueAbsoluteInstance2 = changeValueAbsoluteValue2.getDoubleValue();
operationImpactInstance2.setChangeValueAbsolute(changeValueAbsoluteInstance2);
}
JsonNode changeValueRelativeValue2 = reportedImpactValue.get("changeValueRelative");
if (changeValueRelativeValue2 != null && changeValueRelativeValue2 instanceof NullNode == false) {
double changeValueRelativeInstance2;
changeValueRelativeInstance2 = changeValueRelativeValue2.getDoubleValue();
operationImpactInstance2.setChangeValueRelative(changeValueRelativeInstance2);
}
}
}
}
JsonNode idValue = responseDoc.get("id");
if (idValue != null && idValue instanceof NullNode == false) {
String idInstance;
idInstance = idValue.getTextValue();
recommendedIndexInstance.setId(idInstance);
}
JsonNode nameValue3 = responseDoc.get("name");
if (nameValue3 != null && nameValue3 instanceof NullNode == false) {
String nameInstance3;
nameInstance3 = nameValue3.getTextValue();
recommendedIndexInstance.setName(nameInstance3);
}
JsonNode typeValue = responseDoc.get("type");
if (typeValue != null && typeValue instanceof NullNode == false) {
String typeInstance;
typeInstance = typeValue.getTextValue();
recommendedIndexInstance.setType(typeInstance);
}
JsonNode locationValue = responseDoc.get("location");
if (locationValue != null && locationValue instanceof NullNode == false) {
String locationInstance;
locationInstance = locationValue.getTextValue();
recommendedIndexInstance.setLocation(locationInstance);
}
JsonNode tagsSequenceElement = ((JsonNode) responseDoc.get("tags"));
if (tagsSequenceElement != null && tagsSequenceElement instanceof NullNode == false) {
Iterator> itr = tagsSequenceElement.getFields();
while (itr.hasNext()) {
Map.Entry property = itr.next();
String tagsKey = property.getKey();
String tagsValue = property.getValue().getTextValue();
recommendedIndexInstance.getTags().put(tagsKey, tagsValue);
}
}
}
}
result.setStatusCode(statusCode);
if (httpResponse.getHeaders("Location").length > 0) {
result.setOperationStatusLink(httpResponse.getFirstHeader("Location").getValue());
}
if (httpResponse.getHeaders("Retry-After").length > 0) {
result.setRetryAfter(DatatypeConverter.parseInt(httpResponse.getFirstHeader("Retry-After").getValue()));
}
if (httpResponse.getHeaders("x-ms-request-id").length > 0) {
result.setRequestId(httpResponse.getFirstHeader("x-ms-request-id").getValue());
}
if (statusCode == HttpStatus.SC_OK) {
result.setStatus(OperationStatus.Succeeded);
}
if (shouldTrace) {
CloudTracing.exit(invocationId, result);
}
return result;
} finally {
if (httpResponse != null && httpResponse.getEntity() != null) {
httpResponse.getEntity().getContent().close();
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy