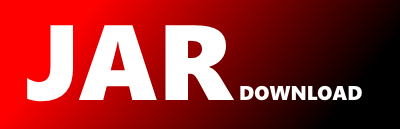
com.microsoft.azure.management.sql.models.BaseAuditingPolicyProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-mgmt-sql Show documentation
Show all versions of azure-mgmt-sql Show documentation
This package contains Microsoft Azure SDK for SQL Management module.
/**
*
* Copyright (c) Microsoft and contributors. All rights reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
*
* See the License for the specific language governing permissions and
* limitations under the License.
*
*/
// Warning: This code was generated by a tool.
//
// Changes to this file may cause incorrect behavior and will be lost if the
// code is regenerated.
package com.microsoft.azure.management.sql.models;
/**
* Represents the properties of an Azure SQL auditing policy.
*/
public class BaseAuditingPolicyProperties {
private String auditingState;
/**
* Optional. Gets the auditing state of the Azure SQL Database server
* auditing policy.
* @return The AuditingState value.
*/
public String getAuditingState() {
return this.auditingState;
}
/**
* Optional. Gets the auditing state of the Azure SQL Database server
* auditing policy.
* @param auditingStateValue The AuditingState value.
*/
public void setAuditingState(final String auditingStateValue) {
this.auditingState = auditingStateValue;
}
private String auditLogsTableName;
/**
* Optional. Gets the name of the table audit logs are written to in the
* Azure SQL Database auditing policy.
* @return The AuditLogsTableName value.
*/
public String getAuditLogsTableName() {
return this.auditLogsTableName;
}
/**
* Optional. Gets the name of the table audit logs are written to in the
* Azure SQL Database auditing policy.
* @param auditLogsTableNameValue The AuditLogsTableName value.
*/
public void setAuditLogsTableName(final String auditLogsTableNameValue) {
this.auditLogsTableName = auditLogsTableNameValue;
}
private String eventTypesToAudit;
/**
* Optional. Gets the events types of the Azure SQL Database server auditing
* policy.
* @return The EventTypesToAudit value.
*/
public String getEventTypesToAudit() {
return this.eventTypesToAudit;
}
/**
* Optional. Gets the events types of the Azure SQL Database server auditing
* policy.
* @param eventTypesToAuditValue The EventTypesToAudit value.
*/
public void setEventTypesToAudit(final String eventTypesToAuditValue) {
this.eventTypesToAudit = eventTypesToAuditValue;
}
private String fullAuditLogsTableName;
/**
* Optional. Gets the full name of the table audit logs are written to in
* the Azure SQL Database auditing policy.
* @return The FullAuditLogsTableName value.
*/
public String getFullAuditLogsTableName() {
return this.fullAuditLogsTableName;
}
/**
* Optional. Gets the full name of the table audit logs are written to in
* the Azure SQL Database auditing policy.
* @param fullAuditLogsTableNameValue The FullAuditLogsTableName value.
*/
public void setFullAuditLogsTableName(final String fullAuditLogsTableNameValue) {
this.fullAuditLogsTableName = fullAuditLogsTableNameValue;
}
private String retentionDays;
/**
* Optional. Gets the retention in days of the Azure SQL Database auditing
* policy.
* @return The RetentionDays value.
*/
public String getRetentionDays() {
return this.retentionDays;
}
/**
* Optional. Gets the retention in days of the Azure SQL Database auditing
* policy.
* @param retentionDaysValue The RetentionDays value.
*/
public void setRetentionDays(final String retentionDaysValue) {
this.retentionDays = retentionDaysValue;
}
private String storageAccountKey;
/**
* Optional. Gets the primary storage account key of the Azure SQL Database
* server auditing policy.
* @return The StorageAccountKey value.
*/
public String getStorageAccountKey() {
return this.storageAccountKey;
}
/**
* Optional. Gets the primary storage account key of the Azure SQL Database
* server auditing policy.
* @param storageAccountKeyValue The StorageAccountKey value.
*/
public void setStorageAccountKey(final String storageAccountKeyValue) {
this.storageAccountKey = storageAccountKeyValue;
}
private String storageAccountName;
/**
* Optional. Gets the storage account name of the Azure SQL Database server
* auditing policy.
* @return The StorageAccountName value.
*/
public String getStorageAccountName() {
return this.storageAccountName;
}
/**
* Optional. Gets the storage account name of the Azure SQL Database server
* auditing policy.
* @param storageAccountNameValue The StorageAccountName value.
*/
public void setStorageAccountName(final String storageAccountNameValue) {
this.storageAccountName = storageAccountNameValue;
}
private String storageAccountResourceGroupName;
/**
* Optional. Gets the resource group of the storage account of the Azure SQL
* Database server auditing policy.
* @return The StorageAccountResourceGroupName value.
*/
public String getStorageAccountResourceGroupName() {
return this.storageAccountResourceGroupName;
}
/**
* Optional. Gets the resource group of the storage account of the Azure SQL
* Database server auditing policy.
* @param storageAccountResourceGroupNameValue The
* StorageAccountResourceGroupName value.
*/
public void setStorageAccountResourceGroupName(final String storageAccountResourceGroupNameValue) {
this.storageAccountResourceGroupName = storageAccountResourceGroupNameValue;
}
private String storageAccountSecondaryKey;
/**
* Optional. Gets secondary key of the storage account of the Azure SQL
* Database server auditing policy.
* @return The StorageAccountSecondaryKey value.
*/
public String getStorageAccountSecondaryKey() {
return this.storageAccountSecondaryKey;
}
/**
* Optional. Gets secondary key of the storage account of the Azure SQL
* Database server auditing policy.
* @param storageAccountSecondaryKeyValue The StorageAccountSecondaryKey
* value.
*/
public void setStorageAccountSecondaryKey(final String storageAccountSecondaryKeyValue) {
this.storageAccountSecondaryKey = storageAccountSecondaryKeyValue;
}
private String storageAccountSubscriptionId;
/**
* Optional. Gets the subscription Id of the storage account of the Azure
* SQL Database server auditing policy.
* @return The StorageAccountSubscriptionId value.
*/
public String getStorageAccountSubscriptionId() {
return this.storageAccountSubscriptionId;
}
/**
* Optional. Gets the subscription Id of the storage account of the Azure
* SQL Database server auditing policy.
* @param storageAccountSubscriptionIdValue The StorageAccountSubscriptionId
* value.
*/
public void setStorageAccountSubscriptionId(final String storageAccountSubscriptionIdValue) {
this.storageAccountSubscriptionId = storageAccountSubscriptionIdValue;
}
private String storageTableEndpoint;
/**
* Optional. Gets the storage table endpoint of the Azure SQL Database
* Server auditing policy.
* @return The StorageTableEndpoint value.
*/
public String getStorageTableEndpoint() {
return this.storageTableEndpoint;
}
/**
* Optional. Gets the storage table endpoint of the Azure SQL Database
* Server auditing policy.
* @param storageTableEndpointValue The StorageTableEndpoint value.
*/
public void setStorageTableEndpoint(final String storageTableEndpointValue) {
this.storageTableEndpoint = storageTableEndpointValue;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy