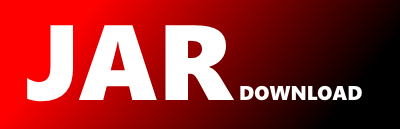
com.microsoft.azure.management.sql.SqlElasticPool Maven / Gradle / Ivy
Show all versions of azure-mgmt-sql Show documentation
/**
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for
* license information.
*/
package com.microsoft.azure.management.sql;
import com.microsoft.azure.management.apigeneration.Beta;
import com.microsoft.azure.management.apigeneration.Fluent;
import com.microsoft.azure.management.apigeneration.Method;
import com.microsoft.azure.management.resources.fluentcore.arm.Region;
import com.microsoft.azure.management.resources.fluentcore.arm.models.ExternalChildResource;
import com.microsoft.azure.management.resources.fluentcore.arm.models.HasResourceGroup;
import com.microsoft.azure.management.resources.fluentcore.arm.models.Resource;
import com.microsoft.azure.management.resources.fluentcore.model.Appliable;
import com.microsoft.azure.management.resources.fluentcore.model.Attachable;
import com.microsoft.azure.management.resources.fluentcore.model.HasInner;
import com.microsoft.azure.management.resources.fluentcore.model.Refreshable;
import com.microsoft.azure.management.resources.fluentcore.model.Updatable;
import com.microsoft.azure.management.sql.implementation.ElasticPoolInner;
import org.joda.time.DateTime;
import rx.Completable;
import rx.Observable;
import java.util.List;
/**
* An immutable client-side representation of an Azure SQL Elastic Pool.
*/
@Fluent
@Beta(Beta.SinceVersion.V1_7_0)
public interface SqlElasticPool
extends
ExternalChildResource,
HasInner,
HasResourceGroup,
Refreshable,
Updatable {
/**
* @return name of the SQL Server to which this elastic pool belongs
*/
String sqlServerName();
/**
* @return the creation date of the Azure SQL Elastic Pool
*/
DateTime creationDate();
/**
* @return the state of the Azure SQL Elastic Pool
*/
ElasticPoolState state();
/**
* @return the edition of Azure SQL Elastic Pool
*/
ElasticPoolEdition edition();
/**
* @return The total shared DTU for the SQL Azure Database Elastic Pool
*/
int dtu();
/**
* @return the maximum DTU any one SQL Azure database can consume.
*/
int databaseDtuMax();
/**
* @return the minimum DTU all SQL Azure Databases are guaranteed
*/
int databaseDtuMin();
/**
* @return the storage limit for the SQL Azure Database Elastic Pool in MB
*/
@Deprecated
int storageMB();
/**
* @return the storage capacity limit for the SQL Azure Database Elastic Pool in MB
*/
@Beta(Beta.SinceVersion.V1_7_0)
int storageCapacityInMB();
/**
* @return the parent SQL server ID
*/
@Beta(Beta.SinceVersion.V1_7_0)
String parentId();
/**
* @return the name of the region the resource is in
*/
@Beta(Beta.SinceVersion.V1_7_0)
String regionName();
/**
* @return the region the resource is in
*/
@Beta(Beta.SinceVersion.V1_7_0)
Region region();
// Actions
/**
* @return the information about elastic pool activities
*/
@Method
List listActivities();
/**
* @return a representation of the deferred computation of the information about elastic pool activities
*/
@Beta(Beta.SinceVersion.V1_7_0)
@Method
Observable listActivitiesAsync();
/**
* @return the information about elastic pool database activities
*/
@Method
List listDatabaseActivities();
/**
* @return the information about elastic pool database activities
*/
@Beta(Beta.SinceVersion.V1_7_0)
@Method
Observable listDatabaseActivitiesAsync();
/**
* Lists the database metrics for this SQL Elastic Pool.
*
* @param filter an OData filter expression that describes a subset of metrics to return
* @return the elastic pool's database metrics
*/
@Method
@Beta(Beta.SinceVersion.V1_7_0)
List listDatabaseMetrics(String filter);
/**
* Asynchronously lists the database metrics for this SQL Elastic Pool.
*
* @param filter an OData filter expression that describes a subset of metrics to return
* @return a representation of the deferred computation of this call
*/
@Method
@Beta(Beta.SinceVersion.V1_7_0)
Observable listDatabaseMetricsAsync(String filter);
/**
* Lists the database metric definitions for this SQL Elastic Pool.
*
* @return the elastic pool's metric definitions
*/
@Method
@Beta(Beta.SinceVersion.V1_7_0)
List listDatabaseMetricDefinitions();
/**
* Asynchronously lists the database metric definitions for this SQL Elastic Pool.
*
* @return a representation of the deferred computation of this call
*/
@Method
@Beta(Beta.SinceVersion.V1_7_0)
Observable listDatabaseMetricDefinitionsAsync();
/**
* Lists the SQL databases in this SQL Elastic Pool.
*
* @return the information about databases in elastic pool
*/
@Method
List listDatabases();
/**
* Asynchronously lists the SQL databases in this SQL Elastic Pool.
*
* @return a representation of the deferred computation of this call
*/
@Method
@Beta(Beta.SinceVersion.V1_7_0)
Observable listDatabasesAsync();
/**
* Gets the specific database in the elastic pool.
*
* @param databaseName name of the database to look into
* @return the information about specific database in elastic pool
*/
SqlDatabase getDatabase(String databaseName);
/**
* Adds a new SQL Database to the Elastic Pool.
*
* @param databaseName name of the database
* @return the database
*/
@Beta(Beta.SinceVersion.V1_7_0)
SqlDatabase addNewDatabase(String databaseName);
/**
* Adds an existing SQL Database to the Elastic Pool.
*
* @param databaseName name of the database
* @return the database
*/
@Beta(Beta.SinceVersion.V1_7_0)
SqlDatabase addExistingDatabase(String databaseName);
/**
* Adds an existing SQL Database to the Elastic Pool.
*
* @param database the database to be added
* @return the database
*/
@Beta(Beta.SinceVersion.V1_7_0)
SqlDatabase addExistingDatabase(SqlDatabase database);
/**
* Removes an existing SQL Database from the Elastic Pool.
*
* @param databaseName name of the database
* @return the database
*/
@Beta(Beta.SinceVersion.V1_7_0)
SqlDatabase removeDatabase(String databaseName);
/**
* Deletes this SQL Elastic Pool from the parent SQL server.
*/
@Method
void delete();
/**
* Deletes this SQL Elastic Pool asynchronously from the parent SQL server.
*
* @return a representation of the deferred computation of this call
*/
@Method
Completable deleteAsync();
/**************************************************************
* Fluent interfaces to provision a SQL Elastic Pool
**************************************************************/
/**
* Container interface for all the definitions that need to be implemented.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface SqlElasticPoolDefinition extends
DefinitionStages.Blank,
DefinitionStages.WithEdition,
DefinitionStages.WithBasicEdition,
DefinitionStages.WithStandardEdition,
DefinitionStages.WithPremiumEdition,
DefinitionStages.WithAttach {
}
/**
* Grouping of all the storage account definition stages.
*/
interface DefinitionStages {
/**
* The first stage of the SQL Server definition.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface Blank extends SqlElasticPool.DefinitionStages.WithEdition {
}
/**
* The SQL Elastic Pool definition to set the edition for database.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithEdition {
/**
* Sets the edition for the SQL Elastic Pool.
*
* @param edition edition to be set for elastic pool.
* @return The next stage of the definition.
*/
@Deprecated
@Beta(Beta.SinceVersion.V1_7_0)
SqlElasticPool.DefinitionStages.WithAttach withEdition(ElasticPoolEdition edition);
/**
* Sets the basic edition for the SQL Elastic Pool.
*
* @return The next stage of the definition.
*/
@Beta(Beta.SinceVersion.V1_7_0)
@Method
SqlElasticPool.DefinitionStages.WithBasicEdition withBasicPool();
/**
* Sets the standard edition for the SQL Elastic Pool.
*
* @return The next stage of the definition.
*/
@Beta(Beta.SinceVersion.V1_7_0)
@Method
SqlElasticPool.DefinitionStages.WithStandardEdition withStandardPool();
/**
* Sets the premium edition for the SQL Elastic Pool.
*
* @return The next stage of the definition.
*/
@Beta(Beta.SinceVersion.V1_7_0)
@Method
SqlElasticPool.DefinitionStages.WithPremiumEdition withPremiumPool();
}
/**
* The SQL Elastic Pool definition to set the eDTU and storage capacity limits for a basic pool.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithBasicEdition extends SqlElasticPool.DefinitionStages.WithAttach {
/**
* Sets the total shared eDTU for the SQL Azure Database Elastic Pool.
*
* @param eDTU total shared eDTU for the SQL Azure Database Elastic Pool
* @return The next stage of the definition.
*/
@Beta(Beta.SinceVersion.V1_7_0)
SqlElasticPool.DefinitionStages.WithBasicEdition withReservedDtu(SqlElasticPoolBasicEDTUs eDTU);
/**
* Sets the maximum number of eDTU a database in the pool can consume.
*
* @param eDTU maximum eDTU a database in the pool can consume
* @return The next stage of the definition.
*/
@Beta(Beta.SinceVersion.V1_7_0)
SqlElasticPool.DefinitionStages.WithBasicEdition withDatabaseDtuMax(SqlElasticPoolBasicMaxEDTUs eDTU);
/**
* Sets the minimum number of eDTU for each database in the pool are regardless of its activity.
*
* @param eDTU minimum eDTU for all SQL Azure databases
* @return The next stage of the definition.
*/
@Beta(Beta.SinceVersion.V1_7_0)
SqlElasticPool.DefinitionStages.WithBasicEdition withDatabaseDtuMin(SqlElasticPoolBasicMinEDTUs eDTU);
}
/**
* The SQL Elastic Pool definition to set the eDTU and storage capacity limits for a standard pool.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithStandardEdition extends SqlElasticPool.DefinitionStages.WithAttach {
/**
* Sets the total shared eDTU for the SQL Azure Database Elastic Pool.
*
* @param eDTU total shared eDTU for the SQL Azure Database Elastic Pool
* @return The next stage of the definition.
*/
@Beta(Beta.SinceVersion.V1_7_0)
SqlElasticPool.DefinitionStages.WithStandardEdition withReservedDtu(SqlElasticPoolStandardEDTUs eDTU);
/**
* Sets the maximum number of eDTU a database in the pool can consume.
*
* @param eDTU maximum eDTU a database in the pool can consume
* @return The next stage of the definition.
*/
@Beta(Beta.SinceVersion.V1_7_0)
SqlElasticPool.DefinitionStages.WithStandardEdition withDatabaseDtuMax(SqlElasticPoolStandardMaxEDTUs eDTU);
/**
* Sets the minimum number of eDTU for each database in the pool are regardless of its activity.
*
* @param eDTU minimum eDTU for all SQL Azure databases
* @return The next stage of the definition.
*/
@Beta(Beta.SinceVersion.V1_7_0)
SqlElasticPool.DefinitionStages.WithStandardEdition withDatabaseDtuMin(SqlElasticPoolStandardMinEDTUs eDTU);
/**
* Sets the storage capacity for the SQL Azure Database Elastic Pool.
*
* @param storageCapacity storage capacity for the SQL Azure Database Elastic Pool
* @return The next stage of the definition.
*/
@Beta(Beta.SinceVersion.V1_7_0)
SqlElasticPool.DefinitionStages.WithStandardEdition withStorageCapacity(SqlElasticPoolStandardStorage storageCapacity);
}
/**
* The SQL Elastic Pool definition to set the eDTU and storage capacity limits for a premium pool.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithPremiumEdition extends SqlElasticPool.DefinitionStages.WithAttach {
/**
* Sets the total shared eDTU for the SQL Azure Database Elastic Pool.
*
* @param eDTU total shared eDTU for the SQL Azure Database Elastic Pool
* @return The next stage of the definition.
*/
@Beta(Beta.SinceVersion.V1_7_0)
SqlElasticPool.DefinitionStages.WithPremiumEdition withReservedDtu(SqlElasticPoolPremiumEDTUs eDTU);
/**
* Sets the maximum number of eDTU a database in the pool can consume.
*
* @param eDTU maximum eDTU a database in the pool can consume
* @return The next stage of the definition.
*/
@Beta(Beta.SinceVersion.V1_7_0)
SqlElasticPool.DefinitionStages.WithPremiumEdition withDatabaseDtuMax(SqlElasticPoolPremiumMaxEDTUs eDTU);
/**
* Sets the minimum number of eDTU for each database in the pool are regardless of its activity.
*
* @param eDTU minimum eDTU for all SQL Azure databases
* @return The next stage of the definition.
*/
@Beta(Beta.SinceVersion.V1_7_0)
SqlElasticPool.DefinitionStages.WithPremiumEdition withDatabaseDtuMin(SqlElasticPoolPremiumMinEDTUs eDTU);
/**
* Sets the storage capacity for the SQL Azure Database Elastic Pool.
*
* @param storageCapacity storage capacity for the SQL Azure Database Elastic Pool
* @return The next stage of the definition.
*/
@Beta(Beta.SinceVersion.V1_7_0)
SqlElasticPool.DefinitionStages.WithPremiumEdition withStorageCapacity(SqlElasticPoolPremiumSorage storageCapacity);
}
/**
* The SQL Elastic Pool definition to set the minimum DTU for database.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithDatabaseDtuMin {
/**
* Sets the minimum DTU all SQL Azure Databases are guaranteed.
*
* @param databaseDtuMin minimum DTU for all SQL Azure databases
* @return The next stage of the definition.
*/
@Deprecated
SqlElasticPool.DefinitionStages.WithAttach withDatabaseDtuMin(int databaseDtuMin);
}
/**
* The SQL Elastic Pool definition to set the maximum DTU for one database.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithDatabaseDtuMax {
/**
* Sets the maximum DTU any one SQL Azure Database can consume.
*
* @param databaseDtuMax maximum DTU any one SQL Azure Database can consume
* @return The next stage of the definition.
*/
@Deprecated
SqlElasticPool.DefinitionStages.WithAttach withDatabaseDtuMax(int databaseDtuMax);
}
/**
* The SQL Elastic Pool definition to set the number of shared DTU for elastic pool.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithDtu {
/**
* Sets the total shared DTU for the SQL Azure Database Elastic Pool.
*
* @param dtu total shared DTU for the SQL Azure Database Elastic Pool
* @return The next stage of the definition.
*/
@Deprecated
SqlElasticPool.DefinitionStages.WithAttach withDtu(int dtu);
}
/**
* The SQL Elastic Pool definition to set the storage limit for the SQL Azure Database Elastic Pool in MB.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithStorageCapacity {
/**
* Sets the storage limit for the SQL Azure Database Elastic Pool in MB.
*
* @param storageMB storage limit for the SQL Azure Database Elastic Pool in MB
* @return The next stage of the definition.
*/
@Deprecated
SqlElasticPool.DefinitionStages.WithAttach withStorageCapacity(int storageMB);
}
/** The final stage of the SQL Elastic Pool definition.
*
* At this stage, any remaining optional settings can be specified, or the SQL Elastic Pool definition
* can be attached to the parent SQL Server definition.
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithAttach extends
WithDatabaseDtuMin,
WithDatabaseDtuMax,
WithDtu,
WithStorageCapacity,
Attachable.InDefinition {
}
}
/**
* The template for a SQL Elastic Pool update operation, containing all the settings that can be modified.
*/
interface Update extends
UpdateStages.WithReservedDTUAndStorageCapacity,
UpdateStages.WithDatabaseDtuMax,
UpdateStages.WithDatabaseDtuMin,
UpdateStages.WithDtu,
UpdateStages.WithStorageCapacity,
UpdateStages.WithDatabase,
Resource.UpdateWithTags,
Appliable {
}
/**
* Grouping of all the SQL Elastic Pool update stages.
*/
interface UpdateStages {
/**
* The SQL Elastic Pool definition to set the minimum DTU for database.
*/
interface WithDatabaseDtuMin {
/**
* Sets the minimum DTU all SQL Azure Databases are guaranteed.
*
* @param databaseDtuMin minimum DTU for all SQL Azure databases
* @return The next stage of definition.
*/
@Deprecated
Update withDatabaseDtuMin(int databaseDtuMin);
}
/**
* The SQL Elastic Pool definition to set the maximum DTU for one database.
*/
interface WithDatabaseDtuMax {
/**
* Sets the maximum DTU any one SQL Azure Database can consume.
*
* @param databaseDtuMax maximum DTU any one SQL Azure Database can consume
* @return The next stage of definition.
*/
@Deprecated
Update withDatabaseDtuMax(int databaseDtuMax);
}
/**
* The SQL Elastic Pool definition to set the number of shared DTU for elastic pool.
*/
interface WithDtu {
/**
* Sets the total shared DTU for the SQL Azure Database Elastic Pool.
*
* @param dtu total shared DTU for the SQL Azure Database Elastic Pool
* @return The next stage of definition.
*/
@Deprecated
Update withDtu(int dtu);
}
/**
* The SQL Elastic Pool definition to set the storage limit for the SQL Azure Database Elastic Pool in MB.
*/
interface WithStorageCapacity {
/**
* Sets the storage limit for the SQL Azure Database Elastic Pool in MB.
*
* @param storageMB storage limit for the SQL Azure Database Elastic Pool in MB
* @return The next stage of definition.
*/
@Deprecated
Update withStorageCapacity(int storageMB);
}
/**
* The SQL Elastic Pool update definition to set the eDTU and storage capacity limits.
*/
interface WithReservedDTUAndStorageCapacity {
/**
* Sets the total shared eDTU for the SQL Azure Database Elastic Pool.
*
* @param eDTU total shared eDTU for the SQL Azure Database Elastic Pool
* @return The next stage of the update definition.
*/
@Beta(Beta.SinceVersion.V1_7_0)
Update withReservedDtu(SqlElasticPoolBasicEDTUs eDTU);
/**
* Sets the maximum number of eDTU a database in the pool can consume.
*
* @param eDTU maximum eDTU a database in the pool can consume
* @return The next stage of the update definition.
*/
@Beta(Beta.SinceVersion.V1_7_0)
Update withDatabaseDtuMax(SqlElasticPoolBasicMaxEDTUs eDTU);
/**
* Sets the minimum number of eDTU for each database in the pool are regardless of its activity.
*
* @param eDTU minimum eDTU for all SQL Azure databases
* @return The next stage of the update definition.
*/
@Beta(Beta.SinceVersion.V1_7_0)
Update withDatabaseDtuMin(SqlElasticPoolBasicMinEDTUs eDTU);
/**
* Sets the total shared eDTU for the SQL Azure Database Elastic Pool.
*
* @param eDTU total shared eDTU for the SQL Azure Database Elastic Pool
* @return The next stage of the update definition.
*/
@Beta(Beta.SinceVersion.V1_7_0)
Update withReservedDtu(SqlElasticPoolStandardEDTUs eDTU);
/**
* Sets the maximum number of eDTU a database in the pool can consume.
*
* @param eDTU maximum eDTU a database in the pool can consume
* @return The next stage of the update definition.
*/
@Beta(Beta.SinceVersion.V1_7_0)
Update withDatabaseDtuMax(SqlElasticPoolStandardMaxEDTUs eDTU);
/**
* Sets the minimum number of eDTU for each database in the pool are regardless of its activity.
*
* @param eDTU minimum eDTU for all SQL Azure databases
* @return The next stage of the update definition.
*/
@Beta(Beta.SinceVersion.V1_7_0)
Update withDatabaseDtuMin(SqlElasticPoolStandardMinEDTUs eDTU);
/**
* Sets the storage capacity for the SQL Azure Database Elastic Pool.
*
* @param storageCapacity storage capacity for the SQL Azure Database Elastic Pool
* @return The next stage of the update definition.
*/
@Beta(Beta.SinceVersion.V1_7_0)
Update withStorageCapacity(SqlElasticPoolStandardStorage storageCapacity);
/**
* Sets the total shared eDTU for the SQL Azure Database Elastic Pool.
*
* @param eDTU total shared eDTU for the SQL Azure Database Elastic Pool
* @return The next stage of the update definition.
*/
@Beta(Beta.SinceVersion.V1_7_0)
Update withReservedDtu(SqlElasticPoolPremiumEDTUs eDTU);
/**
* Sets the maximum number of eDTU a database in the pool can consume.
*
* @param eDTU maximum eDTU a database in the pool can consume
* @return The next stage of the update definition.
*/
@Beta(Beta.SinceVersion.V1_7_0)
Update withDatabaseDtuMax(SqlElasticPoolPremiumMaxEDTUs eDTU);
/**
* Sets the minimum number of eDTU for each database in the pool are regardless of its activity.
*
* @param eDTU minimum eDTU for all SQL Azure databases
* @return The next stage of the update definition.
*/
@Beta(Beta.SinceVersion.V1_7_0)
Update withDatabaseDtuMin(SqlElasticPoolPremiumMinEDTUs eDTU);
/**
* Sets the storage capacity for the SQL Azure Database Elastic Pool.
*
* @param storageCapacity storage capacity for the SQL Azure Database Elastic Pool
* @return The next stage of the update definition.
*/
@Beta(Beta.SinceVersion.V1_7_0)
Update withStorageCapacity(SqlElasticPoolPremiumSorage storageCapacity);
}
/**
* The SQL Elastic Pool definition to add the Database in the elastic pool.
*/
interface WithDatabase {
/**
* Creates a new database in the SQL elastic pool.
*
* @param databaseName name of the new database to be added in the elastic pool
* @return The next stage of the definition.
*/
Update withNewDatabase(String databaseName);
/**
* Adds an existing database in the SQL elastic pool.
*
* @param databaseName name of the existing database to be added in the elastic pool
* @return The next stage of the definition.
*/
Update withExistingDatabase(String databaseName);
/**
* Adds the database in the SQL elastic pool.
*
* @param database database instance to be added in SQL elastic pool
* @return The next stage of the definition.
*/
Update withExistingDatabase(SqlDatabase database);
}
}
}