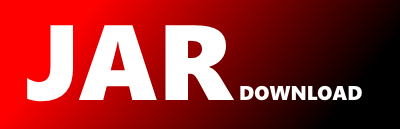
com.microsoft.azure.management.sql.implementation.DatabasesInner Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-mgmt-sql Show documentation
Show all versions of azure-mgmt-sql Show documentation
This package contains Microsoft Azure SDK for SQL Management module.
/**
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for
* license information.
*
* Code generated by Microsoft (R) AutoRest Code Generator.
*/
package com.microsoft.azure.management.sql.implementation;
import retrofit2.Retrofit;
import com.google.common.reflect.TypeToken;
import com.microsoft.azure.CloudException;
import com.microsoft.azure.management.sql.DatabaseUpdate;
import com.microsoft.azure.management.sql.ExportRequest;
import com.microsoft.azure.management.sql.ImportExtensionRequest;
import com.microsoft.azure.management.sql.ImportRequest;
import com.microsoft.azure.management.sql.ResourceMoveDefinition;
import com.microsoft.rest.ServiceCallback;
import com.microsoft.rest.ServiceFuture;
import com.microsoft.rest.ServiceResponse;
import com.microsoft.rest.Validator;
import java.io.IOException;
import java.util.List;
import okhttp3.ResponseBody;
import retrofit2.http.Body;
import retrofit2.http.GET;
import retrofit2.http.Header;
import retrofit2.http.Headers;
import retrofit2.http.HTTP;
import retrofit2.http.PATCH;
import retrofit2.http.Path;
import retrofit2.http.POST;
import retrofit2.http.PUT;
import retrofit2.http.Query;
import retrofit2.Response;
import rx.functions.Func1;
import rx.Observable;
/**
* An instance of this class provides access to all the operations defined
* in Databases.
*/
public class DatabasesInner {
/** The Retrofit service to perform REST calls. */
private DatabasesService service;
/** The service client containing this operation class. */
private SqlManagementClientImpl client;
/**
* Initializes an instance of DatabasesInner.
*
* @param retrofit the Retrofit instance built from a Retrofit Builder.
* @param client the instance of the service client containing this operation class.
*/
public DatabasesInner(Retrofit retrofit, SqlManagementClientImpl client) {
this.service = retrofit.create(DatabasesService.class);
this.client = client;
}
/**
* The interface defining all the services for Databases to be
* used by Retrofit to perform actually REST calls.
*/
interface DatabasesService {
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.sql.Databases pause" })
@POST("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Sql/servers/{serverName}/databases/{databaseName}/pause")
Observable> pause(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("serverName") String serverName, @Path("databaseName") String databaseName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.sql.Databases beginPause" })
@POST("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Sql/servers/{serverName}/databases/{databaseName}/pause")
Observable> beginPause(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("serverName") String serverName, @Path("databaseName") String databaseName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.sql.Databases resume" })
@POST("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Sql/servers/{serverName}/databases/{databaseName}/resume")
Observable> resume(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("serverName") String serverName, @Path("databaseName") String databaseName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.sql.Databases beginResume" })
@POST("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Sql/servers/{serverName}/databases/{databaseName}/resume")
Observable> beginResume(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("serverName") String serverName, @Path("databaseName") String databaseName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.sql.Databases createOrUpdate" })
@PUT("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Sql/servers/{serverName}/databases/{databaseName}")
Observable> createOrUpdate(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("serverName") String serverName, @Path("databaseName") String databaseName, @Query("api-version") String apiVersion, @Body DatabaseInner parameters, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.sql.Databases beginCreateOrUpdate" })
@PUT("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Sql/servers/{serverName}/databases/{databaseName}")
Observable> beginCreateOrUpdate(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("serverName") String serverName, @Path("databaseName") String databaseName, @Query("api-version") String apiVersion, @Body DatabaseInner parameters, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.sql.Databases update" })
@PATCH("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Sql/servers/{serverName}/databases/{databaseName}")
Observable> update(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("serverName") String serverName, @Path("databaseName") String databaseName, @Query("api-version") String apiVersion, @Body DatabaseUpdate parameters, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.sql.Databases beginUpdate" })
@PATCH("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Sql/servers/{serverName}/databases/{databaseName}")
Observable> beginUpdate(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("serverName") String serverName, @Path("databaseName") String databaseName, @Query("api-version") String apiVersion, @Body DatabaseUpdate parameters, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.sql.Databases delete" })
@HTTP(path = "subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Sql/servers/{serverName}/databases/{databaseName}", method = "DELETE", hasBody = true)
Observable> delete(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("serverName") String serverName, @Path("databaseName") String databaseName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.sql.Databases get" })
@GET("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Sql/servers/{serverName}/databases/{databaseName}")
Observable> get(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("serverName") String serverName, @Path("databaseName") String databaseName, @Query("api-version") String apiVersion, @Query("$expand") String expand, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.sql.Databases listByServer" })
@GET("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Sql/servers/{serverName}/databases")
Observable> listByServer(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("serverName") String serverName, @Query("api-version") String apiVersion, @Query("$expand") String expand, @Query("$filter") String filter, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.sql.Databases getByElasticPool" })
@GET("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Sql/servers/{serverName}/elasticPools/{elasticPoolName}/databases/{databaseName}")
Observable> getByElasticPool(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("serverName") String serverName, @Path("elasticPoolName") String elasticPoolName, @Path("databaseName") String databaseName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.sql.Databases listByElasticPool" })
@GET("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Sql/servers/{serverName}/elasticPools/{elasticPoolName}/databases")
Observable> listByElasticPool(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("serverName") String serverName, @Path("elasticPoolName") String elasticPoolName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.sql.Databases getByRecommendedElasticPool" })
@GET("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Sql/servers/{serverName}/recommendedElasticPools/{recommendedElasticPoolName}/databases/{databaseName}")
Observable> getByRecommendedElasticPool(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("serverName") String serverName, @Path("recommendedElasticPoolName") String recommendedElasticPoolName, @Path("databaseName") String databaseName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.sql.Databases listByRecommendedElasticPool" })
@GET("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Sql/servers/{serverName}/recommendedElasticPools/{recommendedElasticPoolName}/databases")
Observable> listByRecommendedElasticPool(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("serverName") String serverName, @Path("recommendedElasticPoolName") String recommendedElasticPoolName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.sql.Databases importMethod" })
@POST("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Sql/servers/{serverName}/import")
Observable> importMethod(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("serverName") String serverName, @Query("api-version") String apiVersion, @Body ImportRequest parameters, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.sql.Databases beginImportMethod" })
@POST("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Sql/servers/{serverName}/import")
Observable> beginImportMethod(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("serverName") String serverName, @Query("api-version") String apiVersion, @Body ImportRequest parameters, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.sql.Databases createImportOperation" })
@PUT("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Sql/servers/{serverName}/databases/{databaseName}/extensions/{extensionName}")
Observable> createImportOperation(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("serverName") String serverName, @Path("databaseName") String databaseName, @Path("extensionName") String extensionName, @Query("api-version") String apiVersion, @Body ImportExtensionRequest parameters, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.sql.Databases beginCreateImportOperation" })
@PUT("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Sql/servers/{serverName}/databases/{databaseName}/extensions/{extensionName}")
Observable> beginCreateImportOperation(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("serverName") String serverName, @Path("databaseName") String databaseName, @Path("extensionName") String extensionName, @Query("api-version") String apiVersion, @Body ImportExtensionRequest parameters, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.sql.Databases export" })
@POST("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Sql/servers/{serverName}/databases/{databaseName}/export")
Observable> export(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("serverName") String serverName, @Path("databaseName") String databaseName, @Query("api-version") String apiVersion, @Body ExportRequest parameters, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.sql.Databases beginExport" })
@POST("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Sql/servers/{serverName}/databases/{databaseName}/export")
Observable> beginExport(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("serverName") String serverName, @Path("databaseName") String databaseName, @Query("api-version") String apiVersion, @Body ExportRequest parameters, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.sql.Databases listMetrics" })
@GET("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Sql/servers/{serverName}/databases/{databaseName}/metrics")
Observable> listMetrics(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("serverName") String serverName, @Path("databaseName") String databaseName, @Query("api-version") String apiVersion, @Query("$filter") String filter, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.sql.Databases listMetricDefinitions" })
@GET("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Sql/servers/{serverName}/databases/{databaseName}/metricDefinitions")
Observable> listMetricDefinitions(@Path("subscriptionId") String subscriptionId, @Path("resourceGroupName") String resourceGroupName, @Path("serverName") String serverName, @Path("databaseName") String databaseName, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.sql.Databases rename" })
@POST("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Sql/servers/{serverName}/databases/{databaseName}/move")
Observable> rename(@Path("resourceGroupName") String resourceGroupName, @Path("serverName") String serverName, @Path("databaseName") String databaseName, @Path("subscriptionId") String subscriptionId, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Body ResourceMoveDefinition parameters, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.sql.Databases failover" })
@POST("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Sql/servers/{serverName}/databases/{databaseName}/failover")
Observable> failover(@Path("resourceGroupName") String resourceGroupName, @Path("serverName") String serverName, @Path("databaseName") String databaseName, @Path("subscriptionId") String subscriptionId, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
@Headers({ "Content-Type: application/json; charset=utf-8", "x-ms-logging-context: com.microsoft.azure.management.sql.Databases beginFailover" })
@POST("subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Sql/servers/{serverName}/databases/{databaseName}/failover")
Observable> beginFailover(@Path("resourceGroupName") String resourceGroupName, @Path("serverName") String serverName, @Path("databaseName") String databaseName, @Path("subscriptionId") String subscriptionId, @Query("api-version") String apiVersion, @Header("accept-language") String acceptLanguage, @Header("User-Agent") String userAgent);
}
/**
* Pauses a data warehouse.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param databaseName The name of the data warehouse to pause.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
*/
public void pause(String resourceGroupName, String serverName, String databaseName) {
pauseWithServiceResponseAsync(resourceGroupName, serverName, databaseName).toBlocking().last().body();
}
/**
* Pauses a data warehouse.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param databaseName The name of the data warehouse to pause.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture pauseAsync(String resourceGroupName, String serverName, String databaseName, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(pauseWithServiceResponseAsync(resourceGroupName, serverName, databaseName), serviceCallback);
}
/**
* Pauses a data warehouse.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param databaseName The name of the data warehouse to pause.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable for the request
*/
public Observable pauseAsync(String resourceGroupName, String serverName, String databaseName) {
return pauseWithServiceResponseAsync(resourceGroupName, serverName, databaseName).map(new Func1, Void>() {
@Override
public Void call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Pauses a data warehouse.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param databaseName The name of the data warehouse to pause.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable for the request
*/
public Observable> pauseWithServiceResponseAsync(String resourceGroupName, String serverName, String databaseName) {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (serverName == null) {
throw new IllegalArgumentException("Parameter serverName is required and cannot be null.");
}
if (databaseName == null) {
throw new IllegalArgumentException("Parameter databaseName is required and cannot be null.");
}
final String apiVersion = "2014-04-01";
Observable> observable = service.pause(this.client.subscriptionId(), resourceGroupName, serverName, databaseName, apiVersion, this.client.acceptLanguage(), this.client.userAgent());
return client.getAzureClient().getPostOrDeleteResultAsync(observable, new TypeToken() { }.getType());
}
/**
* Pauses a data warehouse.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param databaseName The name of the data warehouse to pause.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
*/
public void beginPause(String resourceGroupName, String serverName, String databaseName) {
beginPauseWithServiceResponseAsync(resourceGroupName, serverName, databaseName).toBlocking().single().body();
}
/**
* Pauses a data warehouse.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param databaseName The name of the data warehouse to pause.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture beginPauseAsync(String resourceGroupName, String serverName, String databaseName, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(beginPauseWithServiceResponseAsync(resourceGroupName, serverName, databaseName), serviceCallback);
}
/**
* Pauses a data warehouse.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param databaseName The name of the data warehouse to pause.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceResponse} object if successful.
*/
public Observable beginPauseAsync(String resourceGroupName, String serverName, String databaseName) {
return beginPauseWithServiceResponseAsync(resourceGroupName, serverName, databaseName).map(new Func1, Void>() {
@Override
public Void call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Pauses a data warehouse.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param databaseName The name of the data warehouse to pause.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceResponse} object if successful.
*/
public Observable> beginPauseWithServiceResponseAsync(String resourceGroupName, String serverName, String databaseName) {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (serverName == null) {
throw new IllegalArgumentException("Parameter serverName is required and cannot be null.");
}
if (databaseName == null) {
throw new IllegalArgumentException("Parameter databaseName is required and cannot be null.");
}
final String apiVersion = "2014-04-01";
return service.beginPause(this.client.subscriptionId(), resourceGroupName, serverName, databaseName, apiVersion, this.client.acceptLanguage(), this.client.userAgent())
.flatMap(new Func1, Observable>>() {
@Override
public Observable> call(Response response) {
try {
ServiceResponse clientResponse = beginPauseDelegate(response);
return Observable.just(clientResponse);
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse beginPauseDelegate(Response response) throws CloudException, IOException, IllegalArgumentException {
return this.client.restClient().responseBuilderFactory().newInstance(this.client.serializerAdapter())
.register(200, new TypeToken() { }.getType())
.register(202, new TypeToken() { }.getType())
.registerError(CloudException.class)
.build(response);
}
/**
* Resumes a data warehouse.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param databaseName The name of the data warehouse to resume.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
*/
public void resume(String resourceGroupName, String serverName, String databaseName) {
resumeWithServiceResponseAsync(resourceGroupName, serverName, databaseName).toBlocking().last().body();
}
/**
* Resumes a data warehouse.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param databaseName The name of the data warehouse to resume.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture resumeAsync(String resourceGroupName, String serverName, String databaseName, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(resumeWithServiceResponseAsync(resourceGroupName, serverName, databaseName), serviceCallback);
}
/**
* Resumes a data warehouse.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param databaseName The name of the data warehouse to resume.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable for the request
*/
public Observable resumeAsync(String resourceGroupName, String serverName, String databaseName) {
return resumeWithServiceResponseAsync(resourceGroupName, serverName, databaseName).map(new Func1, Void>() {
@Override
public Void call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Resumes a data warehouse.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param databaseName The name of the data warehouse to resume.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable for the request
*/
public Observable> resumeWithServiceResponseAsync(String resourceGroupName, String serverName, String databaseName) {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (serverName == null) {
throw new IllegalArgumentException("Parameter serverName is required and cannot be null.");
}
if (databaseName == null) {
throw new IllegalArgumentException("Parameter databaseName is required and cannot be null.");
}
final String apiVersion = "2014-04-01";
Observable> observable = service.resume(this.client.subscriptionId(), resourceGroupName, serverName, databaseName, apiVersion, this.client.acceptLanguage(), this.client.userAgent());
return client.getAzureClient().getPostOrDeleteResultAsync(observable, new TypeToken() { }.getType());
}
/**
* Resumes a data warehouse.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param databaseName The name of the data warehouse to resume.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
*/
public void beginResume(String resourceGroupName, String serverName, String databaseName) {
beginResumeWithServiceResponseAsync(resourceGroupName, serverName, databaseName).toBlocking().single().body();
}
/**
* Resumes a data warehouse.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param databaseName The name of the data warehouse to resume.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture beginResumeAsync(String resourceGroupName, String serverName, String databaseName, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(beginResumeWithServiceResponseAsync(resourceGroupName, serverName, databaseName), serviceCallback);
}
/**
* Resumes a data warehouse.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param databaseName The name of the data warehouse to resume.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceResponse} object if successful.
*/
public Observable beginResumeAsync(String resourceGroupName, String serverName, String databaseName) {
return beginResumeWithServiceResponseAsync(resourceGroupName, serverName, databaseName).map(new Func1, Void>() {
@Override
public Void call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Resumes a data warehouse.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param databaseName The name of the data warehouse to resume.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceResponse} object if successful.
*/
public Observable> beginResumeWithServiceResponseAsync(String resourceGroupName, String serverName, String databaseName) {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (serverName == null) {
throw new IllegalArgumentException("Parameter serverName is required and cannot be null.");
}
if (databaseName == null) {
throw new IllegalArgumentException("Parameter databaseName is required and cannot be null.");
}
final String apiVersion = "2014-04-01";
return service.beginResume(this.client.subscriptionId(), resourceGroupName, serverName, databaseName, apiVersion, this.client.acceptLanguage(), this.client.userAgent())
.flatMap(new Func1, Observable>>() {
@Override
public Observable> call(Response response) {
try {
ServiceResponse clientResponse = beginResumeDelegate(response);
return Observable.just(clientResponse);
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse beginResumeDelegate(Response response) throws CloudException, IOException, IllegalArgumentException {
return this.client.restClient().responseBuilderFactory().newInstance(this.client.serializerAdapter())
.register(200, new TypeToken() { }.getType())
.register(202, new TypeToken() { }.getType())
.registerError(CloudException.class)
.build(response);
}
/**
* Creates a new database or updates an existing database.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param databaseName The name of the database to be operated on (updated or created).
* @param parameters The required parameters for creating or updating a database.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the DatabaseInner object if successful.
*/
public DatabaseInner createOrUpdate(String resourceGroupName, String serverName, String databaseName, DatabaseInner parameters) {
return createOrUpdateWithServiceResponseAsync(resourceGroupName, serverName, databaseName, parameters).toBlocking().last().body();
}
/**
* Creates a new database or updates an existing database.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param databaseName The name of the database to be operated on (updated or created).
* @param parameters The required parameters for creating or updating a database.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture createOrUpdateAsync(String resourceGroupName, String serverName, String databaseName, DatabaseInner parameters, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(createOrUpdateWithServiceResponseAsync(resourceGroupName, serverName, databaseName, parameters), serviceCallback);
}
/**
* Creates a new database or updates an existing database.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param databaseName The name of the database to be operated on (updated or created).
* @param parameters The required parameters for creating or updating a database.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable for the request
*/
public Observable createOrUpdateAsync(String resourceGroupName, String serverName, String databaseName, DatabaseInner parameters) {
return createOrUpdateWithServiceResponseAsync(resourceGroupName, serverName, databaseName, parameters).map(new Func1, DatabaseInner>() {
@Override
public DatabaseInner call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Creates a new database or updates an existing database.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param databaseName The name of the database to be operated on (updated or created).
* @param parameters The required parameters for creating or updating a database.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable for the request
*/
public Observable> createOrUpdateWithServiceResponseAsync(String resourceGroupName, String serverName, String databaseName, DatabaseInner parameters) {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (serverName == null) {
throw new IllegalArgumentException("Parameter serverName is required and cannot be null.");
}
if (databaseName == null) {
throw new IllegalArgumentException("Parameter databaseName is required and cannot be null.");
}
if (parameters == null) {
throw new IllegalArgumentException("Parameter parameters is required and cannot be null.");
}
Validator.validate(parameters);
final String apiVersion = "2014-04-01";
Observable> observable = service.createOrUpdate(this.client.subscriptionId(), resourceGroupName, serverName, databaseName, apiVersion, parameters, this.client.acceptLanguage(), this.client.userAgent());
return client.getAzureClient().getPutOrPatchResultAsync(observable, new TypeToken() { }.getType());
}
/**
* Creates a new database or updates an existing database.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param databaseName The name of the database to be operated on (updated or created).
* @param parameters The required parameters for creating or updating a database.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the DatabaseInner object if successful.
*/
public DatabaseInner beginCreateOrUpdate(String resourceGroupName, String serverName, String databaseName, DatabaseInner parameters) {
return beginCreateOrUpdateWithServiceResponseAsync(resourceGroupName, serverName, databaseName, parameters).toBlocking().single().body();
}
/**
* Creates a new database or updates an existing database.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param databaseName The name of the database to be operated on (updated or created).
* @param parameters The required parameters for creating or updating a database.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture beginCreateOrUpdateAsync(String resourceGroupName, String serverName, String databaseName, DatabaseInner parameters, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(beginCreateOrUpdateWithServiceResponseAsync(resourceGroupName, serverName, databaseName, parameters), serviceCallback);
}
/**
* Creates a new database or updates an existing database.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param databaseName The name of the database to be operated on (updated or created).
* @param parameters The required parameters for creating or updating a database.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the DatabaseInner object
*/
public Observable beginCreateOrUpdateAsync(String resourceGroupName, String serverName, String databaseName, DatabaseInner parameters) {
return beginCreateOrUpdateWithServiceResponseAsync(resourceGroupName, serverName, databaseName, parameters).map(new Func1, DatabaseInner>() {
@Override
public DatabaseInner call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Creates a new database or updates an existing database.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param databaseName The name of the database to be operated on (updated or created).
* @param parameters The required parameters for creating or updating a database.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the DatabaseInner object
*/
public Observable> beginCreateOrUpdateWithServiceResponseAsync(String resourceGroupName, String serverName, String databaseName, DatabaseInner parameters) {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (serverName == null) {
throw new IllegalArgumentException("Parameter serverName is required and cannot be null.");
}
if (databaseName == null) {
throw new IllegalArgumentException("Parameter databaseName is required and cannot be null.");
}
if (parameters == null) {
throw new IllegalArgumentException("Parameter parameters is required and cannot be null.");
}
Validator.validate(parameters);
final String apiVersion = "2014-04-01";
return service.beginCreateOrUpdate(this.client.subscriptionId(), resourceGroupName, serverName, databaseName, apiVersion, parameters, this.client.acceptLanguage(), this.client.userAgent())
.flatMap(new Func1, Observable>>() {
@Override
public Observable> call(Response response) {
try {
ServiceResponse clientResponse = beginCreateOrUpdateDelegate(response);
return Observable.just(clientResponse);
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse beginCreateOrUpdateDelegate(Response response) throws CloudException, IOException, IllegalArgumentException {
return this.client.restClient().responseBuilderFactory().newInstance(this.client.serializerAdapter())
.register(200, new TypeToken() { }.getType())
.register(201, new TypeToken() { }.getType())
.register(202, new TypeToken() { }.getType())
.registerError(CloudException.class)
.build(response);
}
/**
* Updates an existing database.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param databaseName The name of the database to be updated.
* @param parameters The required parameters for updating a database.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the DatabaseInner object if successful.
*/
public DatabaseInner update(String resourceGroupName, String serverName, String databaseName, DatabaseUpdate parameters) {
return updateWithServiceResponseAsync(resourceGroupName, serverName, databaseName, parameters).toBlocking().last().body();
}
/**
* Updates an existing database.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param databaseName The name of the database to be updated.
* @param parameters The required parameters for updating a database.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture updateAsync(String resourceGroupName, String serverName, String databaseName, DatabaseUpdate parameters, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(updateWithServiceResponseAsync(resourceGroupName, serverName, databaseName, parameters), serviceCallback);
}
/**
* Updates an existing database.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param databaseName The name of the database to be updated.
* @param parameters The required parameters for updating a database.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable for the request
*/
public Observable updateAsync(String resourceGroupName, String serverName, String databaseName, DatabaseUpdate parameters) {
return updateWithServiceResponseAsync(resourceGroupName, serverName, databaseName, parameters).map(new Func1, DatabaseInner>() {
@Override
public DatabaseInner call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Updates an existing database.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param databaseName The name of the database to be updated.
* @param parameters The required parameters for updating a database.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable for the request
*/
public Observable> updateWithServiceResponseAsync(String resourceGroupName, String serverName, String databaseName, DatabaseUpdate parameters) {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (serverName == null) {
throw new IllegalArgumentException("Parameter serverName is required and cannot be null.");
}
if (databaseName == null) {
throw new IllegalArgumentException("Parameter databaseName is required and cannot be null.");
}
if (parameters == null) {
throw new IllegalArgumentException("Parameter parameters is required and cannot be null.");
}
Validator.validate(parameters);
final String apiVersion = "2014-04-01";
Observable> observable = service.update(this.client.subscriptionId(), resourceGroupName, serverName, databaseName, apiVersion, parameters, this.client.acceptLanguage(), this.client.userAgent());
return client.getAzureClient().getPutOrPatchResultAsync(observable, new TypeToken() { }.getType());
}
/**
* Updates an existing database.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param databaseName The name of the database to be updated.
* @param parameters The required parameters for updating a database.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the DatabaseInner object if successful.
*/
public DatabaseInner beginUpdate(String resourceGroupName, String serverName, String databaseName, DatabaseUpdate parameters) {
return beginUpdateWithServiceResponseAsync(resourceGroupName, serverName, databaseName, parameters).toBlocking().single().body();
}
/**
* Updates an existing database.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param databaseName The name of the database to be updated.
* @param parameters The required parameters for updating a database.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture beginUpdateAsync(String resourceGroupName, String serverName, String databaseName, DatabaseUpdate parameters, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(beginUpdateWithServiceResponseAsync(resourceGroupName, serverName, databaseName, parameters), serviceCallback);
}
/**
* Updates an existing database.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param databaseName The name of the database to be updated.
* @param parameters The required parameters for updating a database.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the DatabaseInner object
*/
public Observable beginUpdateAsync(String resourceGroupName, String serverName, String databaseName, DatabaseUpdate parameters) {
return beginUpdateWithServiceResponseAsync(resourceGroupName, serverName, databaseName, parameters).map(new Func1, DatabaseInner>() {
@Override
public DatabaseInner call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Updates an existing database.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param databaseName The name of the database to be updated.
* @param parameters The required parameters for updating a database.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the DatabaseInner object
*/
public Observable> beginUpdateWithServiceResponseAsync(String resourceGroupName, String serverName, String databaseName, DatabaseUpdate parameters) {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (serverName == null) {
throw new IllegalArgumentException("Parameter serverName is required and cannot be null.");
}
if (databaseName == null) {
throw new IllegalArgumentException("Parameter databaseName is required and cannot be null.");
}
if (parameters == null) {
throw new IllegalArgumentException("Parameter parameters is required and cannot be null.");
}
Validator.validate(parameters);
final String apiVersion = "2014-04-01";
return service.beginUpdate(this.client.subscriptionId(), resourceGroupName, serverName, databaseName, apiVersion, parameters, this.client.acceptLanguage(), this.client.userAgent())
.flatMap(new Func1, Observable>>() {
@Override
public Observable> call(Response response) {
try {
ServiceResponse clientResponse = beginUpdateDelegate(response);
return Observable.just(clientResponse);
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse beginUpdateDelegate(Response response) throws CloudException, IOException, IllegalArgumentException {
return this.client.restClient().responseBuilderFactory().newInstance(this.client.serializerAdapter())
.register(200, new TypeToken() { }.getType())
.register(202, new TypeToken() { }.getType())
.registerError(CloudException.class)
.build(response);
}
/**
* Deletes a database.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param databaseName The name of the database to be deleted.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
*/
public void delete(String resourceGroupName, String serverName, String databaseName) {
deleteWithServiceResponseAsync(resourceGroupName, serverName, databaseName).toBlocking().single().body();
}
/**
* Deletes a database.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param databaseName The name of the database to be deleted.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture deleteAsync(String resourceGroupName, String serverName, String databaseName, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(deleteWithServiceResponseAsync(resourceGroupName, serverName, databaseName), serviceCallback);
}
/**
* Deletes a database.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param databaseName The name of the database to be deleted.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceResponse} object if successful.
*/
public Observable deleteAsync(String resourceGroupName, String serverName, String databaseName) {
return deleteWithServiceResponseAsync(resourceGroupName, serverName, databaseName).map(new Func1, Void>() {
@Override
public Void call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Deletes a database.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param databaseName The name of the database to be deleted.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceResponse} object if successful.
*/
public Observable> deleteWithServiceResponseAsync(String resourceGroupName, String serverName, String databaseName) {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (serverName == null) {
throw new IllegalArgumentException("Parameter serverName is required and cannot be null.");
}
if (databaseName == null) {
throw new IllegalArgumentException("Parameter databaseName is required and cannot be null.");
}
final String apiVersion = "2014-04-01";
return service.delete(this.client.subscriptionId(), resourceGroupName, serverName, databaseName, apiVersion, this.client.acceptLanguage(), this.client.userAgent())
.flatMap(new Func1, Observable>>() {
@Override
public Observable> call(Response response) {
try {
ServiceResponse clientResponse = deleteDelegate(response);
return Observable.just(clientResponse);
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse deleteDelegate(Response response) throws CloudException, IOException, IllegalArgumentException {
return this.client.restClient().responseBuilderFactory().newInstance(this.client.serializerAdapter())
.register(200, new TypeToken() { }.getType())
.register(204, new TypeToken() { }.getType())
.registerError(CloudException.class)
.build(response);
}
/**
* Gets a database.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param databaseName The name of the database to be retrieved.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the DatabaseInner object if successful.
*/
public DatabaseInner get(String resourceGroupName, String serverName, String databaseName) {
return getWithServiceResponseAsync(resourceGroupName, serverName, databaseName).toBlocking().single().body();
}
/**
* Gets a database.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param databaseName The name of the database to be retrieved.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture getAsync(String resourceGroupName, String serverName, String databaseName, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(getWithServiceResponseAsync(resourceGroupName, serverName, databaseName), serviceCallback);
}
/**
* Gets a database.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param databaseName The name of the database to be retrieved.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the DatabaseInner object
*/
public Observable getAsync(String resourceGroupName, String serverName, String databaseName) {
return getWithServiceResponseAsync(resourceGroupName, serverName, databaseName).map(new Func1, DatabaseInner>() {
@Override
public DatabaseInner call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Gets a database.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param databaseName The name of the database to be retrieved.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the DatabaseInner object
*/
public Observable> getWithServiceResponseAsync(String resourceGroupName, String serverName, String databaseName) {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (serverName == null) {
throw new IllegalArgumentException("Parameter serverName is required and cannot be null.");
}
if (databaseName == null) {
throw new IllegalArgumentException("Parameter databaseName is required and cannot be null.");
}
final String apiVersion = "2014-04-01";
final String expand = null;
return service.get(this.client.subscriptionId(), resourceGroupName, serverName, databaseName, apiVersion, expand, this.client.acceptLanguage(), this.client.userAgent())
.flatMap(new Func1, Observable>>() {
@Override
public Observable> call(Response response) {
try {
ServiceResponse clientResponse = getDelegate(response);
return Observable.just(clientResponse);
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
/**
* Gets a database.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param databaseName The name of the database to be retrieved.
* @param expand A comma separated list of child objects to expand in the response. Possible properties: serviceTierAdvisors, transparentDataEncryption.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the DatabaseInner object if successful.
*/
public DatabaseInner get(String resourceGroupName, String serverName, String databaseName, String expand) {
return getWithServiceResponseAsync(resourceGroupName, serverName, databaseName, expand).toBlocking().single().body();
}
/**
* Gets a database.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param databaseName The name of the database to be retrieved.
* @param expand A comma separated list of child objects to expand in the response. Possible properties: serviceTierAdvisors, transparentDataEncryption.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture getAsync(String resourceGroupName, String serverName, String databaseName, String expand, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(getWithServiceResponseAsync(resourceGroupName, serverName, databaseName, expand), serviceCallback);
}
/**
* Gets a database.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param databaseName The name of the database to be retrieved.
* @param expand A comma separated list of child objects to expand in the response. Possible properties: serviceTierAdvisors, transparentDataEncryption.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the DatabaseInner object
*/
public Observable getAsync(String resourceGroupName, String serverName, String databaseName, String expand) {
return getWithServiceResponseAsync(resourceGroupName, serverName, databaseName, expand).map(new Func1, DatabaseInner>() {
@Override
public DatabaseInner call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Gets a database.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param databaseName The name of the database to be retrieved.
* @param expand A comma separated list of child objects to expand in the response. Possible properties: serviceTierAdvisors, transparentDataEncryption.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the DatabaseInner object
*/
public Observable> getWithServiceResponseAsync(String resourceGroupName, String serverName, String databaseName, String expand) {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (serverName == null) {
throw new IllegalArgumentException("Parameter serverName is required and cannot be null.");
}
if (databaseName == null) {
throw new IllegalArgumentException("Parameter databaseName is required and cannot be null.");
}
final String apiVersion = "2014-04-01";
return service.get(this.client.subscriptionId(), resourceGroupName, serverName, databaseName, apiVersion, expand, this.client.acceptLanguage(), this.client.userAgent())
.flatMap(new Func1, Observable>>() {
@Override
public Observable> call(Response response) {
try {
ServiceResponse clientResponse = getDelegate(response);
return Observable.just(clientResponse);
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse getDelegate(Response response) throws CloudException, IOException, IllegalArgumentException {
return this.client.restClient().responseBuilderFactory().newInstance(this.client.serializerAdapter())
.register(200, new TypeToken() { }.getType())
.registerError(CloudException.class)
.build(response);
}
/**
* Returns a list of databases in a server.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the List<DatabaseInner> object if successful.
*/
public List listByServer(String resourceGroupName, String serverName) {
return listByServerWithServiceResponseAsync(resourceGroupName, serverName).toBlocking().single().body();
}
/**
* Returns a list of databases in a server.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture> listByServerAsync(String resourceGroupName, String serverName, final ServiceCallback> serviceCallback) {
return ServiceFuture.fromResponse(listByServerWithServiceResponseAsync(resourceGroupName, serverName), serviceCallback);
}
/**
* Returns a list of databases in a server.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the List<DatabaseInner> object
*/
public Observable> listByServerAsync(String resourceGroupName, String serverName) {
return listByServerWithServiceResponseAsync(resourceGroupName, serverName).map(new Func1>, List>() {
@Override
public List call(ServiceResponse> response) {
return response.body();
}
});
}
/**
* Returns a list of databases in a server.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the List<DatabaseInner> object
*/
public Observable>> listByServerWithServiceResponseAsync(String resourceGroupName, String serverName) {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (serverName == null) {
throw new IllegalArgumentException("Parameter serverName is required and cannot be null.");
}
final String apiVersion = "2014-04-01";
final String expand = null;
final String filter = null;
return service.listByServer(this.client.subscriptionId(), resourceGroupName, serverName, apiVersion, expand, filter, this.client.acceptLanguage(), this.client.userAgent())
.flatMap(new Func1, Observable>>>() {
@Override
public Observable>> call(Response response) {
try {
ServiceResponse> result = listByServerDelegate(response);
List items = null;
if (result.body() != null) {
items = result.body().items();
}
ServiceResponse> clientResponse = new ServiceResponse>(items, result.response());
return Observable.just(clientResponse);
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
/**
* Returns a list of databases in a server.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param expand A comma separated list of child objects to expand in the response. Possible properties: serviceTierAdvisors, transparentDataEncryption.
* @param filter An OData filter expression that describes a subset of databases to return.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the List<DatabaseInner> object if successful.
*/
public List listByServer(String resourceGroupName, String serverName, String expand, String filter) {
return listByServerWithServiceResponseAsync(resourceGroupName, serverName, expand, filter).toBlocking().single().body();
}
/**
* Returns a list of databases in a server.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param expand A comma separated list of child objects to expand in the response. Possible properties: serviceTierAdvisors, transparentDataEncryption.
* @param filter An OData filter expression that describes a subset of databases to return.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture> listByServerAsync(String resourceGroupName, String serverName, String expand, String filter, final ServiceCallback> serviceCallback) {
return ServiceFuture.fromResponse(listByServerWithServiceResponseAsync(resourceGroupName, serverName, expand, filter), serviceCallback);
}
/**
* Returns a list of databases in a server.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param expand A comma separated list of child objects to expand in the response. Possible properties: serviceTierAdvisors, transparentDataEncryption.
* @param filter An OData filter expression that describes a subset of databases to return.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the List<DatabaseInner> object
*/
public Observable> listByServerAsync(String resourceGroupName, String serverName, String expand, String filter) {
return listByServerWithServiceResponseAsync(resourceGroupName, serverName, expand, filter).map(new Func1>, List>() {
@Override
public List call(ServiceResponse> response) {
return response.body();
}
});
}
/**
* Returns a list of databases in a server.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param expand A comma separated list of child objects to expand in the response. Possible properties: serviceTierAdvisors, transparentDataEncryption.
* @param filter An OData filter expression that describes a subset of databases to return.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the List<DatabaseInner> object
*/
public Observable>> listByServerWithServiceResponseAsync(String resourceGroupName, String serverName, String expand, String filter) {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (serverName == null) {
throw new IllegalArgumentException("Parameter serverName is required and cannot be null.");
}
final String apiVersion = "2014-04-01";
return service.listByServer(this.client.subscriptionId(), resourceGroupName, serverName, apiVersion, expand, filter, this.client.acceptLanguage(), this.client.userAgent())
.flatMap(new Func1, Observable>>>() {
@Override
public Observable>> call(Response response) {
try {
ServiceResponse> result = listByServerDelegate(response);
List items = null;
if (result.body() != null) {
items = result.body().items();
}
ServiceResponse> clientResponse = new ServiceResponse>(items, result.response());
return Observable.just(clientResponse);
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse> listByServerDelegate(Response response) throws CloudException, IOException, IllegalArgumentException {
return this.client.restClient().responseBuilderFactory()., CloudException>newInstance(this.client.serializerAdapter())
.register(200, new TypeToken>() { }.getType())
.registerError(CloudException.class)
.build(response);
}
/**
* Gets a database inside of an elastic pool.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param elasticPoolName The name of the elastic pool to be retrieved.
* @param databaseName The name of the database to be retrieved.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the DatabaseInner object if successful.
*/
public DatabaseInner getByElasticPool(String resourceGroupName, String serverName, String elasticPoolName, String databaseName) {
return getByElasticPoolWithServiceResponseAsync(resourceGroupName, serverName, elasticPoolName, databaseName).toBlocking().single().body();
}
/**
* Gets a database inside of an elastic pool.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param elasticPoolName The name of the elastic pool to be retrieved.
* @param databaseName The name of the database to be retrieved.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture getByElasticPoolAsync(String resourceGroupName, String serverName, String elasticPoolName, String databaseName, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(getByElasticPoolWithServiceResponseAsync(resourceGroupName, serverName, elasticPoolName, databaseName), serviceCallback);
}
/**
* Gets a database inside of an elastic pool.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param elasticPoolName The name of the elastic pool to be retrieved.
* @param databaseName The name of the database to be retrieved.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the DatabaseInner object
*/
public Observable getByElasticPoolAsync(String resourceGroupName, String serverName, String elasticPoolName, String databaseName) {
return getByElasticPoolWithServiceResponseAsync(resourceGroupName, serverName, elasticPoolName, databaseName).map(new Func1, DatabaseInner>() {
@Override
public DatabaseInner call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Gets a database inside of an elastic pool.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param elasticPoolName The name of the elastic pool to be retrieved.
* @param databaseName The name of the database to be retrieved.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the DatabaseInner object
*/
public Observable> getByElasticPoolWithServiceResponseAsync(String resourceGroupName, String serverName, String elasticPoolName, String databaseName) {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (serverName == null) {
throw new IllegalArgumentException("Parameter serverName is required and cannot be null.");
}
if (elasticPoolName == null) {
throw new IllegalArgumentException("Parameter elasticPoolName is required and cannot be null.");
}
if (databaseName == null) {
throw new IllegalArgumentException("Parameter databaseName is required and cannot be null.");
}
final String apiVersion = "2014-04-01";
return service.getByElasticPool(this.client.subscriptionId(), resourceGroupName, serverName, elasticPoolName, databaseName, apiVersion, this.client.acceptLanguage(), this.client.userAgent())
.flatMap(new Func1, Observable>>() {
@Override
public Observable> call(Response response) {
try {
ServiceResponse clientResponse = getByElasticPoolDelegate(response);
return Observable.just(clientResponse);
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse getByElasticPoolDelegate(Response response) throws CloudException, IOException, IllegalArgumentException {
return this.client.restClient().responseBuilderFactory().newInstance(this.client.serializerAdapter())
.register(200, new TypeToken() { }.getType())
.registerError(CloudException.class)
.build(response);
}
/**
* Returns a list of databases in an elastic pool.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param elasticPoolName The name of the elastic pool to be retrieved.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the List<DatabaseInner> object if successful.
*/
public List listByElasticPool(String resourceGroupName, String serverName, String elasticPoolName) {
return listByElasticPoolWithServiceResponseAsync(resourceGroupName, serverName, elasticPoolName).toBlocking().single().body();
}
/**
* Returns a list of databases in an elastic pool.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param elasticPoolName The name of the elastic pool to be retrieved.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture> listByElasticPoolAsync(String resourceGroupName, String serverName, String elasticPoolName, final ServiceCallback> serviceCallback) {
return ServiceFuture.fromResponse(listByElasticPoolWithServiceResponseAsync(resourceGroupName, serverName, elasticPoolName), serviceCallback);
}
/**
* Returns a list of databases in an elastic pool.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param elasticPoolName The name of the elastic pool to be retrieved.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the List<DatabaseInner> object
*/
public Observable> listByElasticPoolAsync(String resourceGroupName, String serverName, String elasticPoolName) {
return listByElasticPoolWithServiceResponseAsync(resourceGroupName, serverName, elasticPoolName).map(new Func1>, List>() {
@Override
public List call(ServiceResponse> response) {
return response.body();
}
});
}
/**
* Returns a list of databases in an elastic pool.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param elasticPoolName The name of the elastic pool to be retrieved.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the List<DatabaseInner> object
*/
public Observable>> listByElasticPoolWithServiceResponseAsync(String resourceGroupName, String serverName, String elasticPoolName) {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (serverName == null) {
throw new IllegalArgumentException("Parameter serverName is required and cannot be null.");
}
if (elasticPoolName == null) {
throw new IllegalArgumentException("Parameter elasticPoolName is required and cannot be null.");
}
final String apiVersion = "2014-04-01";
return service.listByElasticPool(this.client.subscriptionId(), resourceGroupName, serverName, elasticPoolName, apiVersion, this.client.acceptLanguage(), this.client.userAgent())
.flatMap(new Func1, Observable>>>() {
@Override
public Observable>> call(Response response) {
try {
ServiceResponse> result = listByElasticPoolDelegate(response);
List items = null;
if (result.body() != null) {
items = result.body().items();
}
ServiceResponse> clientResponse = new ServiceResponse>(items, result.response());
return Observable.just(clientResponse);
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse> listByElasticPoolDelegate(Response response) throws CloudException, IOException, IllegalArgumentException {
return this.client.restClient().responseBuilderFactory()., CloudException>newInstance(this.client.serializerAdapter())
.register(200, new TypeToken>() { }.getType())
.registerError(CloudException.class)
.build(response);
}
/**
* Gets a database inside of a recommended elastic pool.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param recommendedElasticPoolName The name of the elastic pool to be retrieved.
* @param databaseName The name of the database to be retrieved.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @throws CloudException thrown if the request is rejected by server
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent
* @return the DatabaseInner object if successful.
*/
public DatabaseInner getByRecommendedElasticPool(String resourceGroupName, String serverName, String recommendedElasticPoolName, String databaseName) {
return getByRecommendedElasticPoolWithServiceResponseAsync(resourceGroupName, serverName, recommendedElasticPoolName, databaseName).toBlocking().single().body();
}
/**
* Gets a database inside of a recommended elastic pool.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param recommendedElasticPoolName The name of the elastic pool to be retrieved.
* @param databaseName The name of the database to be retrieved.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the {@link ServiceFuture} object
*/
public ServiceFuture getByRecommendedElasticPoolAsync(String resourceGroupName, String serverName, String recommendedElasticPoolName, String databaseName, final ServiceCallback serviceCallback) {
return ServiceFuture.fromResponse(getByRecommendedElasticPoolWithServiceResponseAsync(resourceGroupName, serverName, recommendedElasticPoolName, databaseName), serviceCallback);
}
/**
* Gets a database inside of a recommended elastic pool.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param recommendedElasticPoolName The name of the elastic pool to be retrieved.
* @param databaseName The name of the database to be retrieved.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the DatabaseInner object
*/
public Observable getByRecommendedElasticPoolAsync(String resourceGroupName, String serverName, String recommendedElasticPoolName, String databaseName) {
return getByRecommendedElasticPoolWithServiceResponseAsync(resourceGroupName, serverName, recommendedElasticPoolName, databaseName).map(new Func1, DatabaseInner>() {
@Override
public DatabaseInner call(ServiceResponse response) {
return response.body();
}
});
}
/**
* Gets a database inside of a recommended elastic pool.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param recommendedElasticPoolName The name of the elastic pool to be retrieved.
* @param databaseName The name of the database to be retrieved.
* @throws IllegalArgumentException thrown if parameters fail the validation
* @return the observable to the DatabaseInner object
*/
public Observable> getByRecommendedElasticPoolWithServiceResponseAsync(String resourceGroupName, String serverName, String recommendedElasticPoolName, String databaseName) {
if (this.client.subscriptionId() == null) {
throw new IllegalArgumentException("Parameter this.client.subscriptionId() is required and cannot be null.");
}
if (resourceGroupName == null) {
throw new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null.");
}
if (serverName == null) {
throw new IllegalArgumentException("Parameter serverName is required and cannot be null.");
}
if (recommendedElasticPoolName == null) {
throw new IllegalArgumentException("Parameter recommendedElasticPoolName is required and cannot be null.");
}
if (databaseName == null) {
throw new IllegalArgumentException("Parameter databaseName is required and cannot be null.");
}
final String apiVersion = "2014-04-01";
return service.getByRecommendedElasticPool(this.client.subscriptionId(), resourceGroupName, serverName, recommendedElasticPoolName, databaseName, apiVersion, this.client.acceptLanguage(), this.client.userAgent())
.flatMap(new Func1, Observable>>() {
@Override
public Observable> call(Response response) {
try {
ServiceResponse clientResponse = getByRecommendedElasticPoolDelegate(response);
return Observable.just(clientResponse);
} catch (Throwable t) {
return Observable.error(t);
}
}
});
}
private ServiceResponse