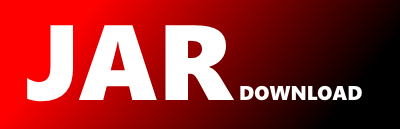
com.microsoft.azure.servicebus.primitives.SendWorkItem Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-servicebus Show documentation
Show all versions of azure-servicebus Show documentation
Java library for Azure Service Bus
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
package com.microsoft.azure.servicebus.primitives;
import com.microsoft.azure.servicebus.TransactionContext;
import java.time.Duration;
import java.util.concurrent.CompletableFuture;
class SendWorkItem extends WorkItem {
private byte[] amqpMessage;
private int messageFormat;
private int encodedMessageSize;
private boolean waitingForAck;
private String deliveryTag;
private TransactionContext transaction;
SendWorkItem(byte[] amqpMessage, int encodedMessageSize, int messageFormat, String deliveryTag, TransactionContext transaction, CompletableFuture completableFuture, Duration timeout) {
super(completableFuture, timeout);
this.initialize(amqpMessage, encodedMessageSize, messageFormat, deliveryTag, transaction);
}
SendWorkItem(byte[] amqpMessage, int encodedMessageSize, int messageFormat, String deliveryTag, TransactionContext transaction, CompletableFuture completableFuture, TimeoutTracker timeout) {
super(completableFuture, timeout);
this.initialize(amqpMessage, encodedMessageSize, messageFormat, deliveryTag, transaction);
}
private void initialize(byte[] amqpMessage, int encodedMessageSize, int messageFormat, String deliveryTag, TransactionContext transaction) {
this.amqpMessage = amqpMessage;
this.messageFormat = messageFormat;
this.encodedMessageSize = encodedMessageSize;
this.deliveryTag = deliveryTag;
this.transaction = transaction;
}
public byte[] getMessage() {
return this.amqpMessage;
}
public int getEncodedMessageSize() {
return this.encodedMessageSize;
}
public int getMessageFormat() {
return this.messageFormat;
}
public void setWaitingForAck() {
this.waitingForAck = true;
}
public boolean isWaitingForAck() {
return this.waitingForAck;
}
public String getDeliveryTag() {
return this.deliveryTag;
}
public void setDeliveryTag(String deliveryTag) {
this.deliveryTag = deliveryTag;
}
public TransactionContext getTransaction() {
return this.transaction;
}
public void setTransaction(TransactionContext txnId) {
this.transaction = txnId;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy