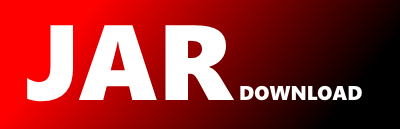
com.microsoft.azure.servicebus.MessageConverter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-servicebus Show documentation
Show all versions of azure-servicebus Show documentation
Java library for Azure Service Bus
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
package com.microsoft.azure.servicebus;
import java.time.Duration;
import java.time.Instant;
import java.util.Date;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.UUID;
import org.apache.qpid.proton.Proton;
import org.apache.qpid.proton.amqp.Binary;
import org.apache.qpid.proton.amqp.Symbol;
import org.apache.qpid.proton.amqp.messaging.AmqpSequence;
import org.apache.qpid.proton.amqp.messaging.AmqpValue;
import org.apache.qpid.proton.amqp.messaging.ApplicationProperties;
import org.apache.qpid.proton.amqp.messaging.Data;
import org.apache.qpid.proton.amqp.messaging.MessageAnnotations;
import org.apache.qpid.proton.amqp.messaging.Properties;
import org.apache.qpid.proton.amqp.messaging.Section;
import com.microsoft.azure.servicebus.primitives.ClientConstants;
import com.microsoft.azure.servicebus.primitives.MessageWithDeliveryTag;
import com.microsoft.azure.servicebus.primitives.MessageWithLockToken;
import com.microsoft.azure.servicebus.primitives.StringUtil;
import com.microsoft.azure.servicebus.primitives.Util;
class MessageConverter {
public static org.apache.qpid.proton.message.Message convertBrokeredMessageToAmqpMessage(Message brokeredMessage) {
org.apache.qpid.proton.message.Message amqpMessage = Proton.message();
MessageBody body = brokeredMessage.getMessageBody();
if (body != null) {
if (body.getBodyType() == MessageBodyType.VALUE) {
amqpMessage.setBody(new AmqpValue(body.getValueData()));
} else if (body.getBodyType() == MessageBodyType.SEQUENCE) {
amqpMessage.setBody(new AmqpSequence(Utils.getSequenceFromMessageBody(body)));
} else {
amqpMessage.setBody(new Data(new Binary(Utils.getDataFromMessageBody(body))));
}
}
if (brokeredMessage.getProperties() != null) {
amqpMessage.setApplicationProperties(new ApplicationProperties(brokeredMessage.getProperties()));
}
if (brokeredMessage.getTimeToLive() != null) {
long ttlMillis = brokeredMessage.getTimeToLive().toMillis();
if (ttlMillis > ClientConstants.UNSIGNED_INT_MAX_VALUE) {
ttlMillis = ClientConstants.UNSIGNED_INT_MAX_VALUE;
}
amqpMessage.setTtl(ttlMillis);
Instant creationTime = Instant.now();
Instant absoluteExpiryTime = creationTime.plus(brokeredMessage.getTimeToLive());
amqpMessage.setCreationTime(creationTime.toEpochMilli());
amqpMessage.setExpiryTime(absoluteExpiryTime.toEpochMilli());
}
amqpMessage.setMessageId(brokeredMessage.getMessageId());
amqpMessage.setContentType(brokeredMessage.getContentType());
amqpMessage.setCorrelationId(brokeredMessage.getCorrelationId());
amqpMessage.setSubject(brokeredMessage.getLabel());
amqpMessage.getProperties().setTo(brokeredMessage.getTo());
amqpMessage.setReplyTo(brokeredMessage.getReplyTo());
amqpMessage.setReplyToGroupId(brokeredMessage.getReplyToSessionId());
amqpMessage.setGroupId(brokeredMessage.getSessionId());
Map messageAnnotationsMap = new HashMap<>();
if (brokeredMessage.getScheduledEnqueueTimeUtc() != null) {
messageAnnotationsMap.put(Symbol.valueOf(ClientConstants.SCHEDULEDENQUEUETIMENAME), Date.from(brokeredMessage.getScheduledEnqueueTimeUtc()));
}
if (!StringUtil.isNullOrEmpty(brokeredMessage.getPartitionKey())) {
messageAnnotationsMap.put(Symbol.valueOf(ClientConstants.PARTITIONKEYNAME), brokeredMessage.getPartitionKey());
}
if (!StringUtil.isNullOrEmpty(brokeredMessage.getViaPartitionKey())) {
messageAnnotationsMap.put(Symbol.valueOf(ClientConstants.VIAPARTITIONKEYNAME), brokeredMessage.getViaPartitionKey());
}
amqpMessage.setMessageAnnotations(new MessageAnnotations(messageAnnotationsMap));
return amqpMessage;
}
public static Message convertAmqpMessageToBrokeredMessage(org.apache.qpid.proton.message.Message amqpMessage) {
return convertAmqpMessageToBrokeredMessage(amqpMessage, null);
}
public static Message convertAmqpMessageToBrokeredMessage(MessageWithDeliveryTag amqpMessageWithDeliveryTag) {
org.apache.qpid.proton.message.Message amqpMessage = amqpMessageWithDeliveryTag.getMessage();
byte[] deliveryTag = amqpMessageWithDeliveryTag.getDeliveryTag();
return convertAmqpMessageToBrokeredMessage(amqpMessage, deliveryTag);
}
public static Message convertAmqpMessageToBrokeredMessage(MessageWithLockToken amqpMessageWithLockToken) {
Message convertedMessage = convertAmqpMessageToBrokeredMessage(amqpMessageWithLockToken.getMessage(), null);
convertedMessage.setLockToken(amqpMessageWithLockToken.getLockToken());
return convertedMessage;
}
public static Message convertAmqpMessageToBrokeredMessage(org.apache.qpid.proton.message.Message amqpMessage, byte[] deliveryTag) {
Message brokeredMessage;
Section body = amqpMessage.getBody();
if (body != null) {
if (body instanceof Data) {
Binary messageData = ((Data) body).getValue();
brokeredMessage = new Message(Utils.fromBinay(messageData.getArray()));
} else if (body instanceof AmqpValue) {
Object messageData = ((AmqpValue) body).getValue();
brokeredMessage = new Message(MessageBody.fromValueData(messageData));
} else if (body instanceof AmqpSequence) {
List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy