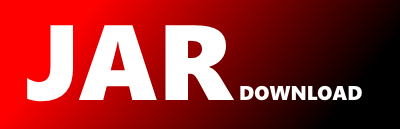
com.microsoft.azure.storage.GeneratedBlobs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-storage-blob Show documentation
Show all versions of azure-storage-blob Show documentation
The Azure Storage Java Blob library.
/**
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for
* license information.
*
* Code generated by Microsoft (R) AutoRest Code Generator.
* Changes may cause incorrect behavior and will be lost if the code is
* regenerated.
*/
package com.microsoft.azure.storage;
import com.microsoft.azure.storage.blob.models.AccessTier;
import com.microsoft.azure.storage.blob.models.BlobAbortCopyFromURLResponse;
import com.microsoft.azure.storage.blob.models.BlobAcquireLeaseResponse;
import com.microsoft.azure.storage.blob.models.BlobBreakLeaseResponse;
import com.microsoft.azure.storage.blob.models.BlobChangeLeaseResponse;
import com.microsoft.azure.storage.blob.models.BlobCreateSnapshotResponse;
import com.microsoft.azure.storage.blob.models.BlobDeleteResponse;
import com.microsoft.azure.storage.blob.models.BlobDownloadResponse;
import com.microsoft.azure.storage.blob.models.BlobGetAccountInfoResponse;
import com.microsoft.azure.storage.blob.models.BlobGetPropertiesResponse;
import com.microsoft.azure.storage.blob.models.BlobReleaseLeaseResponse;
import com.microsoft.azure.storage.blob.models.BlobRenewLeaseResponse;
import com.microsoft.azure.storage.blob.models.BlobSetHTTPHeadersResponse;
import com.microsoft.azure.storage.blob.models.BlobSetMetadataResponse;
import com.microsoft.azure.storage.blob.models.BlobSetTierResponse;
import com.microsoft.azure.storage.blob.models.BlobStartCopyFromURLResponse;
import com.microsoft.azure.storage.blob.models.BlobUndeleteResponse;
import com.microsoft.azure.storage.blob.models.DeleteSnapshotsOptionType;
import com.microsoft.azure.storage.blob.models.StorageErrorException;
import com.microsoft.rest.v2.DateTimeRfc1123;
import com.microsoft.rest.v2.RestProxy;
import com.microsoft.rest.v2.ServiceCallback;
import com.microsoft.rest.v2.ServiceFuture;
import com.microsoft.rest.v2.Validator;
import com.microsoft.rest.v2.annotations.DELETE;
import com.microsoft.rest.v2.annotations.ExpectedResponses;
import com.microsoft.rest.v2.annotations.GET;
import com.microsoft.rest.v2.annotations.HEAD;
import com.microsoft.rest.v2.annotations.HeaderParam;
import com.microsoft.rest.v2.annotations.Host;
import com.microsoft.rest.v2.annotations.HostParam;
import com.microsoft.rest.v2.annotations.PUT;
import com.microsoft.rest.v2.annotations.QueryParam;
import com.microsoft.rest.v2.annotations.UnexpectedResponseExceptionType;
import com.microsoft.rest.v2.util.Base64Util;
import io.reactivex.Completable;
import io.reactivex.Flowable;
import io.reactivex.Maybe;
import io.reactivex.Single;
import io.reactivex.annotations.NonNull;
import java.net.URL;
import java.nio.ByteBuffer;
import java.time.OffsetDateTime;
import java.util.HashMap;
import java.util.Map;
/**
* An instance of this class provides access to all the operations defined in
* GeneratedBlobs.
*/
public final class GeneratedBlobs {
/**
* The proxy service used to perform REST calls.
*/
private BlobsService service;
/**
* The service client containing this operation class.
*/
private GeneratedStorageClient client;
/**
* Initializes an instance of GeneratedBlobs.
*
* @param client the instance of the service client containing this operation class.
*/
public GeneratedBlobs(GeneratedStorageClient client) {
this.service = RestProxy.create(BlobsService.class, client);
this.client = client;
}
/**
* The interface defining all the services for GeneratedBlobs to be used by
* the proxy service to perform REST calls.
*/
@Host("{url}")
private interface BlobsService {
@GET("{containerName}/{blob}")
@ExpectedResponses({200, 206})
@UnexpectedResponseExceptionType(StorageErrorException.class)
Single download(@HostParam("url") String url, @QueryParam("snapshot") String snapshot, @QueryParam("timeout") Integer timeout, @HeaderParam("x-ms-range") String range, @HeaderParam("x-ms-lease-id") String leaseId, @HeaderParam("x-ms-range-get-content-md5") Boolean rangeGetContentMD5, @HeaderParam("If-Modified-Since") DateTimeRfc1123 ifModifiedSince, @HeaderParam("If-Unmodified-Since") DateTimeRfc1123 ifUnmodifiedSince, @HeaderParam("If-Match") String ifMatches, @HeaderParam("If-None-Match") String ifNoneMatch, @HeaderParam("x-ms-version") String version, @HeaderParam("x-ms-client-request-id") String requestId);
@HEAD("{containerName}/{blob}")
@ExpectedResponses({200})
@UnexpectedResponseExceptionType(StorageErrorException.class)
Single getProperties(@HostParam("url") String url, @QueryParam("snapshot") String snapshot, @QueryParam("timeout") Integer timeout, @HeaderParam("x-ms-lease-id") String leaseId, @HeaderParam("If-Modified-Since") DateTimeRfc1123 ifModifiedSince, @HeaderParam("If-Unmodified-Since") DateTimeRfc1123 ifUnmodifiedSince, @HeaderParam("If-Match") String ifMatches, @HeaderParam("If-None-Match") String ifNoneMatch, @HeaderParam("x-ms-version") String version, @HeaderParam("x-ms-client-request-id") String requestId);
@DELETE("{containerName}/{blob}")
@ExpectedResponses({202})
@UnexpectedResponseExceptionType(StorageErrorException.class)
Single delete(@HostParam("url") String url, @QueryParam("snapshot") String snapshot, @QueryParam("timeout") Integer timeout, @HeaderParam("x-ms-lease-id") String leaseId, @HeaderParam("x-ms-delete-snapshots") DeleteSnapshotsOptionType deleteSnapshots, @HeaderParam("If-Modified-Since") DateTimeRfc1123 ifModifiedSince, @HeaderParam("If-Unmodified-Since") DateTimeRfc1123 ifUnmodifiedSince, @HeaderParam("If-Match") String ifMatches, @HeaderParam("If-None-Match") String ifNoneMatch, @HeaderParam("x-ms-version") String version, @HeaderParam("x-ms-client-request-id") String requestId);
@PUT("{containerName}/{blob}")
@ExpectedResponses({200})
@UnexpectedResponseExceptionType(StorageErrorException.class)
Single undelete(@HostParam("url") String url, @QueryParam("timeout") Integer timeout, @HeaderParam("x-ms-version") String version, @HeaderParam("x-ms-client-request-id") String requestId, @QueryParam("comp") String comp);
@PUT("{containerName}/{blob}")
@ExpectedResponses({200})
@UnexpectedResponseExceptionType(StorageErrorException.class)
Single setHTTPHeaders(@HostParam("url") String url, @QueryParam("timeout") Integer timeout, @HeaderParam("x-ms-blob-cache-control") String blobCacheControl, @HeaderParam("x-ms-blob-content-type") String blobContentType, @HeaderParam("x-ms-blob-content-md5") String blobContentMD5, @HeaderParam("x-ms-blob-content-encoding") String blobContentEncoding, @HeaderParam("x-ms-blob-content-language") String blobContentLanguage, @HeaderParam("x-ms-lease-id") String leaseId, @HeaderParam("If-Modified-Since") DateTimeRfc1123 ifModifiedSince, @HeaderParam("If-Unmodified-Since") DateTimeRfc1123 ifUnmodifiedSince, @HeaderParam("If-Match") String ifMatches, @HeaderParam("If-None-Match") String ifNoneMatch, @HeaderParam("x-ms-blob-content-disposition") String blobContentDisposition, @HeaderParam("x-ms-version") String version, @HeaderParam("x-ms-client-request-id") String requestId, @QueryParam("comp") String comp);
@PUT("{containerName}/{blob}")
@ExpectedResponses({200})
@UnexpectedResponseExceptionType(StorageErrorException.class)
Single setMetadata(@HostParam("url") String url, @QueryParam("timeout") Integer timeout, @HeaderParam("x-ms-meta-") Map metadata, @HeaderParam("x-ms-lease-id") String leaseId, @HeaderParam("If-Modified-Since") DateTimeRfc1123 ifModifiedSince, @HeaderParam("If-Unmodified-Since") DateTimeRfc1123 ifUnmodifiedSince, @HeaderParam("If-Match") String ifMatches, @HeaderParam("If-None-Match") String ifNoneMatch, @HeaderParam("x-ms-version") String version, @HeaderParam("x-ms-client-request-id") String requestId, @QueryParam("comp") String comp);
@PUT("{containerName}/{blob}")
@ExpectedResponses({201})
@UnexpectedResponseExceptionType(StorageErrorException.class)
Single acquireLease(@HostParam("url") String url, @QueryParam("timeout") Integer timeout, @HeaderParam("x-ms-lease-duration") Integer duration, @HeaderParam("x-ms-proposed-lease-id") String proposedLeaseId, @HeaderParam("If-Modified-Since") DateTimeRfc1123 ifModifiedSince, @HeaderParam("If-Unmodified-Since") DateTimeRfc1123 ifUnmodifiedSince, @HeaderParam("If-Match") String ifMatches, @HeaderParam("If-None-Match") String ifNoneMatch, @HeaderParam("x-ms-version") String version, @HeaderParam("x-ms-client-request-id") String requestId, @QueryParam("comp") String comp, @HeaderParam("x-ms-lease-action") String action);
@PUT("{containerName}/{blob}")
@ExpectedResponses({200})
@UnexpectedResponseExceptionType(StorageErrorException.class)
Single releaseLease(@HostParam("url") String url, @QueryParam("timeout") Integer timeout, @HeaderParam("x-ms-lease-id") String leaseId, @HeaderParam("If-Modified-Since") DateTimeRfc1123 ifModifiedSince, @HeaderParam("If-Unmodified-Since") DateTimeRfc1123 ifUnmodifiedSince, @HeaderParam("If-Match") String ifMatches, @HeaderParam("If-None-Match") String ifNoneMatch, @HeaderParam("x-ms-version") String version, @HeaderParam("x-ms-client-request-id") String requestId, @QueryParam("comp") String comp, @HeaderParam("x-ms-lease-action") String action);
@PUT("{containerName}/{blob}")
@ExpectedResponses({200})
@UnexpectedResponseExceptionType(StorageErrorException.class)
Single renewLease(@HostParam("url") String url, @QueryParam("timeout") Integer timeout, @HeaderParam("x-ms-lease-id") String leaseId, @HeaderParam("If-Modified-Since") DateTimeRfc1123 ifModifiedSince, @HeaderParam("If-Unmodified-Since") DateTimeRfc1123 ifUnmodifiedSince, @HeaderParam("If-Match") String ifMatches, @HeaderParam("If-None-Match") String ifNoneMatch, @HeaderParam("x-ms-version") String version, @HeaderParam("x-ms-client-request-id") String requestId, @QueryParam("comp") String comp, @HeaderParam("x-ms-lease-action") String action);
@PUT("{containerName}/{blob}")
@ExpectedResponses({200})
@UnexpectedResponseExceptionType(StorageErrorException.class)
Single changeLease(@HostParam("url") String url, @QueryParam("timeout") Integer timeout, @HeaderParam("x-ms-lease-id") String leaseId, @HeaderParam("x-ms-proposed-lease-id") String proposedLeaseId, @HeaderParam("If-Modified-Since") DateTimeRfc1123 ifModifiedSince, @HeaderParam("If-Unmodified-Since") DateTimeRfc1123 ifUnmodifiedSince, @HeaderParam("If-Match") String ifMatches, @HeaderParam("If-None-Match") String ifNoneMatch, @HeaderParam("x-ms-version") String version, @HeaderParam("x-ms-client-request-id") String requestId, @QueryParam("comp") String comp, @HeaderParam("x-ms-lease-action") String action);
@PUT("{containerName}/{blob}")
@ExpectedResponses({202})
@UnexpectedResponseExceptionType(StorageErrorException.class)
Single breakLease(@HostParam("url") String url, @QueryParam("timeout") Integer timeout, @HeaderParam("x-ms-lease-break-period") Integer breakPeriod, @HeaderParam("If-Modified-Since") DateTimeRfc1123 ifModifiedSince, @HeaderParam("If-Unmodified-Since") DateTimeRfc1123 ifUnmodifiedSince, @HeaderParam("If-Match") String ifMatches, @HeaderParam("If-None-Match") String ifNoneMatch, @HeaderParam("x-ms-version") String version, @HeaderParam("x-ms-client-request-id") String requestId, @QueryParam("comp") String comp, @HeaderParam("x-ms-lease-action") String action);
@PUT("{containerName}/{blob}")
@ExpectedResponses({201})
@UnexpectedResponseExceptionType(StorageErrorException.class)
Single createSnapshot(@HostParam("url") String url, @QueryParam("timeout") Integer timeout, @HeaderParam("x-ms-meta-") Map metadata, @HeaderParam("If-Modified-Since") DateTimeRfc1123 ifModifiedSince, @HeaderParam("If-Unmodified-Since") DateTimeRfc1123 ifUnmodifiedSince, @HeaderParam("If-Match") String ifMatches, @HeaderParam("If-None-Match") String ifNoneMatch, @HeaderParam("x-ms-lease-id") String leaseId, @HeaderParam("x-ms-version") String version, @HeaderParam("x-ms-client-request-id") String requestId, @QueryParam("comp") String comp);
@PUT("{containerName}/{blob}")
@ExpectedResponses({202})
@UnexpectedResponseExceptionType(StorageErrorException.class)
Single startCopyFromURL(@HostParam("url") String url, @QueryParam("timeout") Integer timeout, @HeaderParam("x-ms-meta-") Map metadata, @HeaderParam("x-ms-source-if-modified-since") DateTimeRfc1123 sourceIfModifiedSince, @HeaderParam("x-ms-source-if-unmodified-since") DateTimeRfc1123 sourceIfUnmodifiedSince, @HeaderParam("x-ms-source-if-match") String sourceIfMatches, @HeaderParam("x-ms-source-if-none-match") String sourceIfNoneMatch, @HeaderParam("If-Modified-Since") DateTimeRfc1123 ifModifiedSince, @HeaderParam("If-Unmodified-Since") DateTimeRfc1123 ifUnmodifiedSince, @HeaderParam("If-Match") String ifMatches, @HeaderParam("If-None-Match") String ifNoneMatch, @HeaderParam("x-ms-copy-source") URL copySource, @HeaderParam("x-ms-lease-id") String leaseId, @HeaderParam("x-ms-version") String version, @HeaderParam("x-ms-client-request-id") String requestId);
@PUT("{containerName}/{blob}")
@ExpectedResponses({204})
@UnexpectedResponseExceptionType(StorageErrorException.class)
Single abortCopyFromURL(@HostParam("url") String url, @QueryParam("copyid") String copyId, @QueryParam("timeout") Integer timeout, @HeaderParam("x-ms-lease-id") String leaseId, @HeaderParam("x-ms-version") String version, @HeaderParam("x-ms-client-request-id") String requestId, @QueryParam("comp") String comp, @HeaderParam("x-ms-copy-action") String copyActionAbortConstant);
@PUT("{containerName}/{blob}")
@ExpectedResponses({200, 202})
@UnexpectedResponseExceptionType(StorageErrorException.class)
Single setTier(@HostParam("url") String url, @QueryParam("timeout") Integer timeout, @HeaderParam("x-ms-access-tier") AccessTier tier, @HeaderParam("x-ms-version") String version, @HeaderParam("x-ms-client-request-id") String requestId, @QueryParam("comp") String comp);
@GET("{containerName}/{blobName}")
@ExpectedResponses({200})
@UnexpectedResponseExceptionType(StorageErrorException.class)
Single getAccountInfo(@HostParam("url") String url, @HeaderParam("x-ms-version") String version, @QueryParam("restype") String restype, @QueryParam("comp") String comp);
}
/**
* The Download operation reads or downloads a blob from the system, including its metadata and properties. You can also call Download to read a snapshot.
*
* @param snapshot The snapshot parameter is an opaque DateTime value that, when present, specifies the blob snapshot to retrieve. For more information on working with blob snapshots, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/creating-a-snapshot-of-a-blob">Creating a Snapshot of a Blob.</a>.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting Timeouts for Blob Service Operations.</a>.
* @param range Return only the bytes of the blob in the specified range.
* @param leaseId If specified, the operation only succeeds if the container's lease is active and matches this ID.
* @param rangeGetContentMD5 When set to true and specified together with the Range, the service returns the MD5 hash for the range, as long as the range is less than or equal to 4 MB in size.
* @param ifModifiedSince Specify this header value to operate only on a blob if it has been modified since the specified date/time.
* @param ifUnmodifiedSince Specify this header value to operate only on a blob if it has not been modified since the specified date/time.
* @param ifMatches Specify an ETag value to operate only on blobs with a matching value.
* @param ifNoneMatch Specify an ETag value to operate only on blobs without a matching value.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws StorageErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the Flowable<ByteBuffer> object if successful.
*/
public Flowable download(String snapshot, Integer timeout, String range, String leaseId, Boolean rangeGetContentMD5, OffsetDateTime ifModifiedSince, OffsetDateTime ifUnmodifiedSince, String ifMatches, String ifNoneMatch, String requestId) {
return downloadAsync(snapshot, timeout, range, leaseId, rangeGetContentMD5, ifModifiedSince, ifUnmodifiedSince, ifMatches, ifNoneMatch, requestId).blockingGet();
}
/**
* The Download operation reads or downloads a blob from the system, including its metadata and properties. You can also call Download to read a snapshot.
*
* @param snapshot The snapshot parameter is an opaque DateTime value that, when present, specifies the blob snapshot to retrieve. For more information on working with blob snapshots, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/creating-a-snapshot-of-a-blob">Creating a Snapshot of a Blob.</a>.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting Timeouts for Blob Service Operations.</a>.
* @param range Return only the bytes of the blob in the specified range.
* @param leaseId If specified, the operation only succeeds if the container's lease is active and matches this ID.
* @param rangeGetContentMD5 When set to true and specified together with the Range, the service returns the MD5 hash for the range, as long as the range is less than or equal to 4 MB in size.
* @param ifModifiedSince Specify this header value to operate only on a blob if it has been modified since the specified date/time.
* @param ifUnmodifiedSince Specify this header value to operate only on a blob if it has not been modified since the specified date/time.
* @param ifMatches Specify an ETag value to operate only on blobs with a matching value.
* @param ifNoneMatch Specify an ETag value to operate only on blobs without a matching value.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @return a ServiceFuture which will be completed with the result of the network request.
*/
public ServiceFuture> downloadAsync(String snapshot, Integer timeout, String range, String leaseId, Boolean rangeGetContentMD5, OffsetDateTime ifModifiedSince, OffsetDateTime ifUnmodifiedSince, String ifMatches, String ifNoneMatch, String requestId, ServiceCallback> serviceCallback) {
return ServiceFuture.fromBody(downloadAsync(snapshot, timeout, range, leaseId, rangeGetContentMD5, ifModifiedSince, ifUnmodifiedSince, ifMatches, ifNoneMatch, requestId), serviceCallback);
}
/**
* The Download operation reads or downloads a blob from the system, including its metadata and properties. You can also call Download to read a snapshot.
*
* @param snapshot The snapshot parameter is an opaque DateTime value that, when present, specifies the blob snapshot to retrieve. For more information on working with blob snapshots, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/creating-a-snapshot-of-a-blob">Creating a Snapshot of a Blob.</a>.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting Timeouts for Blob Service Operations.</a>.
* @param range Return only the bytes of the blob in the specified range.
* @param leaseId If specified, the operation only succeeds if the container's lease is active and matches this ID.
* @param rangeGetContentMD5 When set to true and specified together with the Range, the service returns the MD5 hash for the range, as long as the range is less than or equal to 4 MB in size.
* @param ifModifiedSince Specify this header value to operate only on a blob if it has been modified since the specified date/time.
* @param ifUnmodifiedSince Specify this header value to operate only on a blob if it has not been modified since the specified date/time.
* @param ifMatches Specify an ETag value to operate only on blobs with a matching value.
* @param ifNoneMatch Specify an ETag value to operate only on blobs without a matching value.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @return a Single which performs the network request upon subscription.
*/
public Single downloadWithRestResponseAsync(String snapshot, Integer timeout, String range, String leaseId, Boolean rangeGetContentMD5, OffsetDateTime ifModifiedSince, OffsetDateTime ifUnmodifiedSince, String ifMatches, String ifNoneMatch, String requestId) {
if (this.client.url() == null) {
throw new IllegalArgumentException("Parameter this.client.url() is required and cannot be null.");
}
if (this.client.version() == null) {
throw new IllegalArgumentException("Parameter this.client.version() is required and cannot be null.");
}
DateTimeRfc1123 ifModifiedSinceConverted = null;
if (ifModifiedSince != null) {
ifModifiedSinceConverted = new DateTimeRfc1123(ifModifiedSince);
}
DateTimeRfc1123 ifUnmodifiedSinceConverted = null;
if (ifUnmodifiedSince != null) {
ifUnmodifiedSinceConverted = new DateTimeRfc1123(ifUnmodifiedSince);
}
return service.download(this.client.url(), snapshot, timeout, range, leaseId, rangeGetContentMD5, ifModifiedSinceConverted, ifUnmodifiedSinceConverted, ifMatches, ifNoneMatch, this.client.version(), requestId);
}
/**
* The Download operation reads or downloads a blob from the system, including its metadata and properties. You can also call Download to read a snapshot.
*
* @param snapshot The snapshot parameter is an opaque DateTime value that, when present, specifies the blob snapshot to retrieve. For more information on working with blob snapshots, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/creating-a-snapshot-of-a-blob">Creating a Snapshot of a Blob.</a>.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting Timeouts for Blob Service Operations.</a>.
* @param range Return only the bytes of the blob in the specified range.
* @param leaseId If specified, the operation only succeeds if the container's lease is active and matches this ID.
* @param rangeGetContentMD5 When set to true and specified together with the Range, the service returns the MD5 hash for the range, as long as the range is less than or equal to 4 MB in size.
* @param ifModifiedSince Specify this header value to operate only on a blob if it has been modified since the specified date/time.
* @param ifUnmodifiedSince Specify this header value to operate only on a blob if it has not been modified since the specified date/time.
* @param ifMatches Specify an ETag value to operate only on blobs with a matching value.
* @param ifNoneMatch Specify an ETag value to operate only on blobs without a matching value.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @return a Single which performs the network request upon subscription.
*/
public Maybe> downloadAsync(String snapshot, Integer timeout, String range, String leaseId, Boolean rangeGetContentMD5, OffsetDateTime ifModifiedSince, OffsetDateTime ifUnmodifiedSince, String ifMatches, String ifNoneMatch, String requestId) {
return downloadWithRestResponseAsync(snapshot, timeout, range, leaseId, rangeGetContentMD5, ifModifiedSince, ifUnmodifiedSince, ifMatches, ifNoneMatch, requestId)
.flatMapMaybe((BlobDownloadResponse res) -> res.body() == null ? Maybe.empty() : Maybe.just(res.body()));
}
/**
* The Get Properties operation returns all user-defined metadata, standard HTTP properties, and system properties for the blob. It does not return the content of the blob.
*
* @param snapshot The snapshot parameter is an opaque DateTime value that, when present, specifies the blob snapshot to retrieve. For more information on working with blob snapshots, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/creating-a-snapshot-of-a-blob">Creating a Snapshot of a Blob.</a>.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting Timeouts for Blob Service Operations.</a>.
* @param leaseId If specified, the operation only succeeds if the container's lease is active and matches this ID.
* @param ifModifiedSince Specify this header value to operate only on a blob if it has been modified since the specified date/time.
* @param ifUnmodifiedSince Specify this header value to operate only on a blob if it has not been modified since the specified date/time.
* @param ifMatches Specify an ETag value to operate only on blobs with a matching value.
* @param ifNoneMatch Specify an ETag value to operate only on blobs without a matching value.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws StorageErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
public void getProperties(String snapshot, Integer timeout, String leaseId, OffsetDateTime ifModifiedSince, OffsetDateTime ifUnmodifiedSince, String ifMatches, String ifNoneMatch, String requestId) {
getPropertiesAsync(snapshot, timeout, leaseId, ifModifiedSince, ifUnmodifiedSince, ifMatches, ifNoneMatch, requestId).blockingAwait();
}
/**
* The Get Properties operation returns all user-defined metadata, standard HTTP properties, and system properties for the blob. It does not return the content of the blob.
*
* @param snapshot The snapshot parameter is an opaque DateTime value that, when present, specifies the blob snapshot to retrieve. For more information on working with blob snapshots, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/creating-a-snapshot-of-a-blob">Creating a Snapshot of a Blob.</a>.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting Timeouts for Blob Service Operations.</a>.
* @param leaseId If specified, the operation only succeeds if the container's lease is active and matches this ID.
* @param ifModifiedSince Specify this header value to operate only on a blob if it has been modified since the specified date/time.
* @param ifUnmodifiedSince Specify this header value to operate only on a blob if it has not been modified since the specified date/time.
* @param ifMatches Specify an ETag value to operate only on blobs with a matching value.
* @param ifNoneMatch Specify an ETag value to operate only on blobs without a matching value.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @return a ServiceFuture which will be completed with the result of the network request.
*/
public ServiceFuture getPropertiesAsync(String snapshot, Integer timeout, String leaseId, OffsetDateTime ifModifiedSince, OffsetDateTime ifUnmodifiedSince, String ifMatches, String ifNoneMatch, String requestId, ServiceCallback serviceCallback) {
return ServiceFuture.fromBody(getPropertiesAsync(snapshot, timeout, leaseId, ifModifiedSince, ifUnmodifiedSince, ifMatches, ifNoneMatch, requestId), serviceCallback);
}
/**
* The Get Properties operation returns all user-defined metadata, standard HTTP properties, and system properties for the blob. It does not return the content of the blob.
*
* @param snapshot The snapshot parameter is an opaque DateTime value that, when present, specifies the blob snapshot to retrieve. For more information on working with blob snapshots, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/creating-a-snapshot-of-a-blob">Creating a Snapshot of a Blob.</a>.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting Timeouts for Blob Service Operations.</a>.
* @param leaseId If specified, the operation only succeeds if the container's lease is active and matches this ID.
* @param ifModifiedSince Specify this header value to operate only on a blob if it has been modified since the specified date/time.
* @param ifUnmodifiedSince Specify this header value to operate only on a blob if it has not been modified since the specified date/time.
* @param ifMatches Specify an ETag value to operate only on blobs with a matching value.
* @param ifNoneMatch Specify an ETag value to operate only on blobs without a matching value.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @return a Single which performs the network request upon subscription.
*/
public Single getPropertiesWithRestResponseAsync(String snapshot, Integer timeout, String leaseId, OffsetDateTime ifModifiedSince, OffsetDateTime ifUnmodifiedSince, String ifMatches, String ifNoneMatch, String requestId) {
if (this.client.url() == null) {
throw new IllegalArgumentException("Parameter this.client.url() is required and cannot be null.");
}
if (this.client.version() == null) {
throw new IllegalArgumentException("Parameter this.client.version() is required and cannot be null.");
}
DateTimeRfc1123 ifModifiedSinceConverted = null;
if (ifModifiedSince != null) {
ifModifiedSinceConverted = new DateTimeRfc1123(ifModifiedSince);
}
DateTimeRfc1123 ifUnmodifiedSinceConverted = null;
if (ifUnmodifiedSince != null) {
ifUnmodifiedSinceConverted = new DateTimeRfc1123(ifUnmodifiedSince);
}
return service.getProperties(this.client.url(), snapshot, timeout, leaseId, ifModifiedSinceConverted, ifUnmodifiedSinceConverted, ifMatches, ifNoneMatch, this.client.version(), requestId);
}
/**
* The Get Properties operation returns all user-defined metadata, standard HTTP properties, and system properties for the blob. It does not return the content of the blob.
*
* @param snapshot The snapshot parameter is an opaque DateTime value that, when present, specifies the blob snapshot to retrieve. For more information on working with blob snapshots, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/creating-a-snapshot-of-a-blob">Creating a Snapshot of a Blob.</a>.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting Timeouts for Blob Service Operations.</a>.
* @param leaseId If specified, the operation only succeeds if the container's lease is active and matches this ID.
* @param ifModifiedSince Specify this header value to operate only on a blob if it has been modified since the specified date/time.
* @param ifUnmodifiedSince Specify this header value to operate only on a blob if it has not been modified since the specified date/time.
* @param ifMatches Specify an ETag value to operate only on blobs with a matching value.
* @param ifNoneMatch Specify an ETag value to operate only on blobs without a matching value.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @return a Single which performs the network request upon subscription.
*/
public Completable getPropertiesAsync(String snapshot, Integer timeout, String leaseId, OffsetDateTime ifModifiedSince, OffsetDateTime ifUnmodifiedSince, String ifMatches, String ifNoneMatch, String requestId) {
return getPropertiesWithRestResponseAsync(snapshot, timeout, leaseId, ifModifiedSince, ifUnmodifiedSince, ifMatches, ifNoneMatch, requestId)
.toCompletable();
}
/**
* If the storage account's soft delete feature is disabled then, when a blob is deleted, it is permanently removed from the storage account. If the storage account's soft delete feature is enabled, then, when a blob is deleted, it is marked for deletion and becomes inaccessible immediately. However, the blob service retains the blob or snapshot for the number of days specified by the DeleteRetentionPolicy section of [Storage service properties] (Set-Blob-Service-Properties.md). After the specified number of days has passed, the blob's data is permanently removed from the storage account. Note that you continue to be charged for the soft-deleted blob's storage until it is permanently removed. Use the List Blobs API and specify the "include=deleted" query parameter to discover which blobs and snapshots have been soft deleted. You can then use the Undelete Blob API to restore a soft-deleted blob. All other operations on a soft-deleted blob or snapshot causes the service to return an HTTP status code of 404 (ResourceNotFound).
*
* @param snapshot The snapshot parameter is an opaque DateTime value that, when present, specifies the blob snapshot to retrieve. For more information on working with blob snapshots, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/creating-a-snapshot-of-a-blob">Creating a Snapshot of a Blob.</a>.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting Timeouts for Blob Service Operations.</a>.
* @param leaseId If specified, the operation only succeeds if the container's lease is active and matches this ID.
* @param deleteSnapshots Required if the blob has associated snapshots. Specify one of the following two options: include: Delete the base blob and all of its snapshots. only: Delete only the blob's snapshots and not the blob itself. Possible values include: 'include', 'only'.
* @param ifModifiedSince Specify this header value to operate only on a blob if it has been modified since the specified date/time.
* @param ifUnmodifiedSince Specify this header value to operate only on a blob if it has not been modified since the specified date/time.
* @param ifMatches Specify an ETag value to operate only on blobs with a matching value.
* @param ifNoneMatch Specify an ETag value to operate only on blobs without a matching value.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws StorageErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
public void delete(String snapshot, Integer timeout, String leaseId, DeleteSnapshotsOptionType deleteSnapshots, OffsetDateTime ifModifiedSince, OffsetDateTime ifUnmodifiedSince, String ifMatches, String ifNoneMatch, String requestId) {
deleteAsync(snapshot, timeout, leaseId, deleteSnapshots, ifModifiedSince, ifUnmodifiedSince, ifMatches, ifNoneMatch, requestId).blockingAwait();
}
/**
* If the storage account's soft delete feature is disabled then, when a blob is deleted, it is permanently removed from the storage account. If the storage account's soft delete feature is enabled, then, when a blob is deleted, it is marked for deletion and becomes inaccessible immediately. However, the blob service retains the blob or snapshot for the number of days specified by the DeleteRetentionPolicy section of [Storage service properties] (Set-Blob-Service-Properties.md). After the specified number of days has passed, the blob's data is permanently removed from the storage account. Note that you continue to be charged for the soft-deleted blob's storage until it is permanently removed. Use the List Blobs API and specify the "include=deleted" query parameter to discover which blobs and snapshots have been soft deleted. You can then use the Undelete Blob API to restore a soft-deleted blob. All other operations on a soft-deleted blob or snapshot causes the service to return an HTTP status code of 404 (ResourceNotFound).
*
* @param snapshot The snapshot parameter is an opaque DateTime value that, when present, specifies the blob snapshot to retrieve. For more information on working with blob snapshots, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/creating-a-snapshot-of-a-blob">Creating a Snapshot of a Blob.</a>.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting Timeouts for Blob Service Operations.</a>.
* @param leaseId If specified, the operation only succeeds if the container's lease is active and matches this ID.
* @param deleteSnapshots Required if the blob has associated snapshots. Specify one of the following two options: include: Delete the base blob and all of its snapshots. only: Delete only the blob's snapshots and not the blob itself. Possible values include: 'include', 'only'.
* @param ifModifiedSince Specify this header value to operate only on a blob if it has been modified since the specified date/time.
* @param ifUnmodifiedSince Specify this header value to operate only on a blob if it has not been modified since the specified date/time.
* @param ifMatches Specify an ETag value to operate only on blobs with a matching value.
* @param ifNoneMatch Specify an ETag value to operate only on blobs without a matching value.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @return a ServiceFuture which will be completed with the result of the network request.
*/
public ServiceFuture deleteAsync(String snapshot, Integer timeout, String leaseId, DeleteSnapshotsOptionType deleteSnapshots, OffsetDateTime ifModifiedSince, OffsetDateTime ifUnmodifiedSince, String ifMatches, String ifNoneMatch, String requestId, ServiceCallback serviceCallback) {
return ServiceFuture.fromBody(deleteAsync(snapshot, timeout, leaseId, deleteSnapshots, ifModifiedSince, ifUnmodifiedSince, ifMatches, ifNoneMatch, requestId), serviceCallback);
}
/**
* If the storage account's soft delete feature is disabled then, when a blob is deleted, it is permanently removed from the storage account. If the storage account's soft delete feature is enabled, then, when a blob is deleted, it is marked for deletion and becomes inaccessible immediately. However, the blob service retains the blob or snapshot for the number of days specified by the DeleteRetentionPolicy section of [Storage service properties] (Set-Blob-Service-Properties.md). After the specified number of days has passed, the blob's data is permanently removed from the storage account. Note that you continue to be charged for the soft-deleted blob's storage until it is permanently removed. Use the List Blobs API and specify the "include=deleted" query parameter to discover which blobs and snapshots have been soft deleted. You can then use the Undelete Blob API to restore a soft-deleted blob. All other operations on a soft-deleted blob or snapshot causes the service to return an HTTP status code of 404 (ResourceNotFound).
*
* @param snapshot The snapshot parameter is an opaque DateTime value that, when present, specifies the blob snapshot to retrieve. For more information on working with blob snapshots, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/creating-a-snapshot-of-a-blob">Creating a Snapshot of a Blob.</a>.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting Timeouts for Blob Service Operations.</a>.
* @param leaseId If specified, the operation only succeeds if the container's lease is active and matches this ID.
* @param deleteSnapshots Required if the blob has associated snapshots. Specify one of the following two options: include: Delete the base blob and all of its snapshots. only: Delete only the blob's snapshots and not the blob itself. Possible values include: 'include', 'only'.
* @param ifModifiedSince Specify this header value to operate only on a blob if it has been modified since the specified date/time.
* @param ifUnmodifiedSince Specify this header value to operate only on a blob if it has not been modified since the specified date/time.
* @param ifMatches Specify an ETag value to operate only on blobs with a matching value.
* @param ifNoneMatch Specify an ETag value to operate only on blobs without a matching value.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @return a Single which performs the network request upon subscription.
*/
public Single deleteWithRestResponseAsync(String snapshot, Integer timeout, String leaseId, DeleteSnapshotsOptionType deleteSnapshots, OffsetDateTime ifModifiedSince, OffsetDateTime ifUnmodifiedSince, String ifMatches, String ifNoneMatch, String requestId) {
if (this.client.url() == null) {
throw new IllegalArgumentException("Parameter this.client.url() is required and cannot be null.");
}
if (this.client.version() == null) {
throw new IllegalArgumentException("Parameter this.client.version() is required and cannot be null.");
}
DateTimeRfc1123 ifModifiedSinceConverted = null;
if (ifModifiedSince != null) {
ifModifiedSinceConverted = new DateTimeRfc1123(ifModifiedSince);
}
DateTimeRfc1123 ifUnmodifiedSinceConverted = null;
if (ifUnmodifiedSince != null) {
ifUnmodifiedSinceConverted = new DateTimeRfc1123(ifUnmodifiedSince);
}
return service.delete(this.client.url(), snapshot, timeout, leaseId, deleteSnapshots, ifModifiedSinceConverted, ifUnmodifiedSinceConverted, ifMatches, ifNoneMatch, this.client.version(), requestId);
}
/**
* If the storage account's soft delete feature is disabled then, when a blob is deleted, it is permanently removed from the storage account. If the storage account's soft delete feature is enabled, then, when a blob is deleted, it is marked for deletion and becomes inaccessible immediately. However, the blob service retains the blob or snapshot for the number of days specified by the DeleteRetentionPolicy section of [Storage service properties] (Set-Blob-Service-Properties.md). After the specified number of days has passed, the blob's data is permanently removed from the storage account. Note that you continue to be charged for the soft-deleted blob's storage until it is permanently removed. Use the List Blobs API and specify the "include=deleted" query parameter to discover which blobs and snapshots have been soft deleted. You can then use the Undelete Blob API to restore a soft-deleted blob. All other operations on a soft-deleted blob or snapshot causes the service to return an HTTP status code of 404 (ResourceNotFound).
*
* @param snapshot The snapshot parameter is an opaque DateTime value that, when present, specifies the blob snapshot to retrieve. For more information on working with blob snapshots, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/creating-a-snapshot-of-a-blob">Creating a Snapshot of a Blob.</a>.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting Timeouts for Blob Service Operations.</a>.
* @param leaseId If specified, the operation only succeeds if the container's lease is active and matches this ID.
* @param deleteSnapshots Required if the blob has associated snapshots. Specify one of the following two options: include: Delete the base blob and all of its snapshots. only: Delete only the blob's snapshots and not the blob itself. Possible values include: 'include', 'only'.
* @param ifModifiedSince Specify this header value to operate only on a blob if it has been modified since the specified date/time.
* @param ifUnmodifiedSince Specify this header value to operate only on a blob if it has not been modified since the specified date/time.
* @param ifMatches Specify an ETag value to operate only on blobs with a matching value.
* @param ifNoneMatch Specify an ETag value to operate only on blobs without a matching value.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @return a Single which performs the network request upon subscription.
*/
public Completable deleteAsync(String snapshot, Integer timeout, String leaseId, DeleteSnapshotsOptionType deleteSnapshots, OffsetDateTime ifModifiedSince, OffsetDateTime ifUnmodifiedSince, String ifMatches, String ifNoneMatch, String requestId) {
return deleteWithRestResponseAsync(snapshot, timeout, leaseId, deleteSnapshots, ifModifiedSince, ifUnmodifiedSince, ifMatches, ifNoneMatch, requestId)
.toCompletable();
}
/**
* Undelete a blob that was previously soft deleted.
*
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting Timeouts for Blob Service Operations.</a>.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws StorageErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
public void undelete(Integer timeout, String requestId) {
undeleteAsync(timeout, requestId).blockingAwait();
}
/**
* Undelete a blob that was previously soft deleted.
*
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting Timeouts for Blob Service Operations.</a>.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @return a ServiceFuture which will be completed with the result of the network request.
*/
public ServiceFuture undeleteAsync(Integer timeout, String requestId, ServiceCallback serviceCallback) {
return ServiceFuture.fromBody(undeleteAsync(timeout, requestId), serviceCallback);
}
/**
* Undelete a blob that was previously soft deleted.
*
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting Timeouts for Blob Service Operations.</a>.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @return a Single which performs the network request upon subscription.
*/
public Single undeleteWithRestResponseAsync(Integer timeout, String requestId) {
if (this.client.url() == null) {
throw new IllegalArgumentException("Parameter this.client.url() is required and cannot be null.");
}
if (this.client.version() == null) {
throw new IllegalArgumentException("Parameter this.client.version() is required and cannot be null.");
}
final String comp = "undelete";
return service.undelete(this.client.url(), timeout, this.client.version(), requestId, comp);
}
/**
* Undelete a blob that was previously soft deleted.
*
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting Timeouts for Blob Service Operations.</a>.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @return a Single which performs the network request upon subscription.
*/
public Completable undeleteAsync(Integer timeout, String requestId) {
return undeleteWithRestResponseAsync(timeout, requestId)
.toCompletable();
}
/**
* The Set HTTP Headers operation sets system properties on the blob.
*
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting Timeouts for Blob Service Operations.</a>.
* @param blobCacheControl Optional. Sets the blob's cache control. If specified, this property is stored with the blob and returned with a read request.
* @param blobContentType Optional. Sets the blob's content type. If specified, this property is stored with the blob and returned with a read request.
* @param blobContentMD5 Optional. An MD5 hash of the blob content. Note that this hash is not validated, as the hashes for the individual blocks were validated when each was uploaded.
* @param blobContentEncoding Optional. Sets the blob's content encoding. If specified, this property is stored with the blob and returned with a read request.
* @param blobContentLanguage Optional. Set the blob's content language. If specified, this property is stored with the blob and returned with a read request.
* @param leaseId If specified, the operation only succeeds if the container's lease is active and matches this ID.
* @param ifModifiedSince Specify this header value to operate only on a blob if it has been modified since the specified date/time.
* @param ifUnmodifiedSince Specify this header value to operate only on a blob if it has not been modified since the specified date/time.
* @param ifMatches Specify an ETag value to operate only on blobs with a matching value.
* @param ifNoneMatch Specify an ETag value to operate only on blobs without a matching value.
* @param blobContentDisposition Optional. Sets the blob's Content-Disposition header.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws StorageErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
public void setHTTPHeaders(Integer timeout, String blobCacheControl, String blobContentType, byte[] blobContentMD5, String blobContentEncoding, String blobContentLanguage, String leaseId, OffsetDateTime ifModifiedSince, OffsetDateTime ifUnmodifiedSince, String ifMatches, String ifNoneMatch, String blobContentDisposition, String requestId) {
setHTTPHeadersAsync(timeout, blobCacheControl, blobContentType, blobContentMD5, blobContentEncoding, blobContentLanguage, leaseId, ifModifiedSince, ifUnmodifiedSince, ifMatches, ifNoneMatch, blobContentDisposition, requestId).blockingAwait();
}
/**
* The Set HTTP Headers operation sets system properties on the blob.
*
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting Timeouts for Blob Service Operations.</a>.
* @param blobCacheControl Optional. Sets the blob's cache control. If specified, this property is stored with the blob and returned with a read request.
* @param blobContentType Optional. Sets the blob's content type. If specified, this property is stored with the blob and returned with a read request.
* @param blobContentMD5 Optional. An MD5 hash of the blob content. Note that this hash is not validated, as the hashes for the individual blocks were validated when each was uploaded.
* @param blobContentEncoding Optional. Sets the blob's content encoding. If specified, this property is stored with the blob and returned with a read request.
* @param blobContentLanguage Optional. Set the blob's content language. If specified, this property is stored with the blob and returned with a read request.
* @param leaseId If specified, the operation only succeeds if the container's lease is active and matches this ID.
* @param ifModifiedSince Specify this header value to operate only on a blob if it has been modified since the specified date/time.
* @param ifUnmodifiedSince Specify this header value to operate only on a blob if it has not been modified since the specified date/time.
* @param ifMatches Specify an ETag value to operate only on blobs with a matching value.
* @param ifNoneMatch Specify an ETag value to operate only on blobs without a matching value.
* @param blobContentDisposition Optional. Sets the blob's Content-Disposition header.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @return a ServiceFuture which will be completed with the result of the network request.
*/
public ServiceFuture setHTTPHeadersAsync(Integer timeout, String blobCacheControl, String blobContentType, byte[] blobContentMD5, String blobContentEncoding, String blobContentLanguage, String leaseId, OffsetDateTime ifModifiedSince, OffsetDateTime ifUnmodifiedSince, String ifMatches, String ifNoneMatch, String blobContentDisposition, String requestId, ServiceCallback serviceCallback) {
return ServiceFuture.fromBody(setHTTPHeadersAsync(timeout, blobCacheControl, blobContentType, blobContentMD5, blobContentEncoding, blobContentLanguage, leaseId, ifModifiedSince, ifUnmodifiedSince, ifMatches, ifNoneMatch, blobContentDisposition, requestId), serviceCallback);
}
/**
* The Set HTTP Headers operation sets system properties on the blob.
*
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting Timeouts for Blob Service Operations.</a>.
* @param blobCacheControl Optional. Sets the blob's cache control. If specified, this property is stored with the blob and returned with a read request.
* @param blobContentType Optional. Sets the blob's content type. If specified, this property is stored with the blob and returned with a read request.
* @param blobContentMD5 Optional. An MD5 hash of the blob content. Note that this hash is not validated, as the hashes for the individual blocks were validated when each was uploaded.
* @param blobContentEncoding Optional. Sets the blob's content encoding. If specified, this property is stored with the blob and returned with a read request.
* @param blobContentLanguage Optional. Set the blob's content language. If specified, this property is stored with the blob and returned with a read request.
* @param leaseId If specified, the operation only succeeds if the container's lease is active and matches this ID.
* @param ifModifiedSince Specify this header value to operate only on a blob if it has been modified since the specified date/time.
* @param ifUnmodifiedSince Specify this header value to operate only on a blob if it has not been modified since the specified date/time.
* @param ifMatches Specify an ETag value to operate only on blobs with a matching value.
* @param ifNoneMatch Specify an ETag value to operate only on blobs without a matching value.
* @param blobContentDisposition Optional. Sets the blob's Content-Disposition header.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @return a Single which performs the network request upon subscription.
*/
public Single setHTTPHeadersWithRestResponseAsync(Integer timeout, String blobCacheControl, String blobContentType, byte[] blobContentMD5, String blobContentEncoding, String blobContentLanguage, String leaseId, OffsetDateTime ifModifiedSince, OffsetDateTime ifUnmodifiedSince, String ifMatches, String ifNoneMatch, String blobContentDisposition, String requestId) {
if (this.client.url() == null) {
throw new IllegalArgumentException("Parameter this.client.url() is required and cannot be null.");
}
if (this.client.version() == null) {
throw new IllegalArgumentException("Parameter this.client.version() is required and cannot be null.");
}
final String comp = "properties";
String blobContentMD5Converted = Base64Util.encodeToString(blobContentMD5);
DateTimeRfc1123 ifModifiedSinceConverted = null;
if (ifModifiedSince != null) {
ifModifiedSinceConverted = new DateTimeRfc1123(ifModifiedSince);
}
DateTimeRfc1123 ifUnmodifiedSinceConverted = null;
if (ifUnmodifiedSince != null) {
ifUnmodifiedSinceConverted = new DateTimeRfc1123(ifUnmodifiedSince);
}
return service.setHTTPHeaders(this.client.url(), timeout, blobCacheControl, blobContentType, blobContentMD5Converted, blobContentEncoding, blobContentLanguage, leaseId, ifModifiedSinceConverted, ifUnmodifiedSinceConverted, ifMatches, ifNoneMatch, blobContentDisposition, this.client.version(), requestId, comp);
}
/**
* The Set HTTP Headers operation sets system properties on the blob.
*
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting Timeouts for Blob Service Operations.</a>.
* @param blobCacheControl Optional. Sets the blob's cache control. If specified, this property is stored with the blob and returned with a read request.
* @param blobContentType Optional. Sets the blob's content type. If specified, this property is stored with the blob and returned with a read request.
* @param blobContentMD5 Optional. An MD5 hash of the blob content. Note that this hash is not validated, as the hashes for the individual blocks were validated when each was uploaded.
* @param blobContentEncoding Optional. Sets the blob's content encoding. If specified, this property is stored with the blob and returned with a read request.
* @param blobContentLanguage Optional. Set the blob's content language. If specified, this property is stored with the blob and returned with a read request.
* @param leaseId If specified, the operation only succeeds if the container's lease is active and matches this ID.
* @param ifModifiedSince Specify this header value to operate only on a blob if it has been modified since the specified date/time.
* @param ifUnmodifiedSince Specify this header value to operate only on a blob if it has not been modified since the specified date/time.
* @param ifMatches Specify an ETag value to operate only on blobs with a matching value.
* @param ifNoneMatch Specify an ETag value to operate only on blobs without a matching value.
* @param blobContentDisposition Optional. Sets the blob's Content-Disposition header.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @return a Single which performs the network request upon subscription.
*/
public Completable setHTTPHeadersAsync(Integer timeout, String blobCacheControl, String blobContentType, byte[] blobContentMD5, String blobContentEncoding, String blobContentLanguage, String leaseId, OffsetDateTime ifModifiedSince, OffsetDateTime ifUnmodifiedSince, String ifMatches, String ifNoneMatch, String blobContentDisposition, String requestId) {
return setHTTPHeadersWithRestResponseAsync(timeout, blobCacheControl, blobContentType, blobContentMD5, blobContentEncoding, blobContentLanguage, leaseId, ifModifiedSince, ifUnmodifiedSince, ifMatches, ifNoneMatch, blobContentDisposition, requestId)
.toCompletable();
}
/**
* The Set Blob Metadata operation sets user-defined metadata for the specified blob as one or more name-value pairs.
*
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting Timeouts for Blob Service Operations.</a>.
* @param metadata Optional. Specifies a user-defined name-value pair associated with the blob. If no name-value pairs are specified, the operation will copy the metadata from the source blob or file to the destination blob. If one or more name-value pairs are specified, the destination blob is created with the specified metadata, and metadata is not copied from the source blob or file. Note that beginning with version 2009-09-19, metadata names must adhere to the naming rules for C# identifiers. See Naming and Referencing Containers, Blobs, and Metadata for more information.
* @param leaseId If specified, the operation only succeeds if the container's lease is active and matches this ID.
* @param ifModifiedSince Specify this header value to operate only on a blob if it has been modified since the specified date/time.
* @param ifUnmodifiedSince Specify this header value to operate only on a blob if it has not been modified since the specified date/time.
* @param ifMatches Specify an ETag value to operate only on blobs with a matching value.
* @param ifNoneMatch Specify an ETag value to operate only on blobs without a matching value.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws StorageErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
public void setMetadata(Integer timeout, Map metadata, String leaseId, OffsetDateTime ifModifiedSince, OffsetDateTime ifUnmodifiedSince, String ifMatches, String ifNoneMatch, String requestId) {
setMetadataAsync(timeout, metadata, leaseId, ifModifiedSince, ifUnmodifiedSince, ifMatches, ifNoneMatch, requestId).blockingAwait();
}
/**
* The Set Blob Metadata operation sets user-defined metadata for the specified blob as one or more name-value pairs.
*
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting Timeouts for Blob Service Operations.</a>.
* @param metadata Optional. Specifies a user-defined name-value pair associated with the blob. If no name-value pairs are specified, the operation will copy the metadata from the source blob or file to the destination blob. If one or more name-value pairs are specified, the destination blob is created with the specified metadata, and metadata is not copied from the source blob or file. Note that beginning with version 2009-09-19, metadata names must adhere to the naming rules for C# identifiers. See Naming and Referencing Containers, Blobs, and Metadata for more information.
* @param leaseId If specified, the operation only succeeds if the container's lease is active and matches this ID.
* @param ifModifiedSince Specify this header value to operate only on a blob if it has been modified since the specified date/time.
* @param ifUnmodifiedSince Specify this header value to operate only on a blob if it has not been modified since the specified date/time.
* @param ifMatches Specify an ETag value to operate only on blobs with a matching value.
* @param ifNoneMatch Specify an ETag value to operate only on blobs without a matching value.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @return a ServiceFuture which will be completed with the result of the network request.
*/
public ServiceFuture setMetadataAsync(Integer timeout, Map metadata, String leaseId, OffsetDateTime ifModifiedSince, OffsetDateTime ifUnmodifiedSince, String ifMatches, String ifNoneMatch, String requestId, ServiceCallback serviceCallback) {
return ServiceFuture.fromBody(setMetadataAsync(timeout, metadata, leaseId, ifModifiedSince, ifUnmodifiedSince, ifMatches, ifNoneMatch, requestId), serviceCallback);
}
/**
* The Set Blob Metadata operation sets user-defined metadata for the specified blob as one or more name-value pairs.
*
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting Timeouts for Blob Service Operations.</a>.
* @param metadata Optional. Specifies a user-defined name-value pair associated with the blob. If no name-value pairs are specified, the operation will copy the metadata from the source blob or file to the destination blob. If one or more name-value pairs are specified, the destination blob is created with the specified metadata, and metadata is not copied from the source blob or file. Note that beginning with version 2009-09-19, metadata names must adhere to the naming rules for C# identifiers. See Naming and Referencing Containers, Blobs, and Metadata for more information.
* @param leaseId If specified, the operation only succeeds if the container's lease is active and matches this ID.
* @param ifModifiedSince Specify this header value to operate only on a blob if it has been modified since the specified date/time.
* @param ifUnmodifiedSince Specify this header value to operate only on a blob if it has not been modified since the specified date/time.
* @param ifMatches Specify an ETag value to operate only on blobs with a matching value.
* @param ifNoneMatch Specify an ETag value to operate only on blobs without a matching value.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @return a Single which performs the network request upon subscription.
*/
public Single setMetadataWithRestResponseAsync(Integer timeout, Map metadata, String leaseId, OffsetDateTime ifModifiedSince, OffsetDateTime ifUnmodifiedSince, String ifMatches, String ifNoneMatch, String requestId) {
if (this.client.url() == null) {
throw new IllegalArgumentException("Parameter this.client.url() is required and cannot be null.");
}
if (this.client.version() == null) {
throw new IllegalArgumentException("Parameter this.client.version() is required and cannot be null.");
}
Validator.validate(metadata);
final String comp = "metadata";
DateTimeRfc1123 ifModifiedSinceConverted = null;
if (ifModifiedSince != null) {
ifModifiedSinceConverted = new DateTimeRfc1123(ifModifiedSince);
}
DateTimeRfc1123 ifUnmodifiedSinceConverted = null;
if (ifUnmodifiedSince != null) {
ifUnmodifiedSinceConverted = new DateTimeRfc1123(ifUnmodifiedSince);
}
return service.setMetadata(this.client.url(), timeout, metadata, leaseId, ifModifiedSinceConverted, ifUnmodifiedSinceConverted, ifMatches, ifNoneMatch, this.client.version(), requestId, comp);
}
/**
* The Set Blob Metadata operation sets user-defined metadata for the specified blob as one or more name-value pairs.
*
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting Timeouts for Blob Service Operations.</a>.
* @param metadata Optional. Specifies a user-defined name-value pair associated with the blob. If no name-value pairs are specified, the operation will copy the metadata from the source blob or file to the destination blob. If one or more name-value pairs are specified, the destination blob is created with the specified metadata, and metadata is not copied from the source blob or file. Note that beginning with version 2009-09-19, metadata names must adhere to the naming rules for C# identifiers. See Naming and Referencing Containers, Blobs, and Metadata for more information.
* @param leaseId If specified, the operation only succeeds if the container's lease is active and matches this ID.
* @param ifModifiedSince Specify this header value to operate only on a blob if it has been modified since the specified date/time.
* @param ifUnmodifiedSince Specify this header value to operate only on a blob if it has not been modified since the specified date/time.
* @param ifMatches Specify an ETag value to operate only on blobs with a matching value.
* @param ifNoneMatch Specify an ETag value to operate only on blobs without a matching value.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @return a Single which performs the network request upon subscription.
*/
public Completable setMetadataAsync(Integer timeout, Map metadata, String leaseId, OffsetDateTime ifModifiedSince, OffsetDateTime ifUnmodifiedSince, String ifMatches, String ifNoneMatch, String requestId) {
return setMetadataWithRestResponseAsync(timeout, metadata, leaseId, ifModifiedSince, ifUnmodifiedSince, ifMatches, ifNoneMatch, requestId)
.toCompletable();
}
/**
* [Update] The Lease Blob operation establishes and manages a lock on a blob for write and delete operations.
*
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting Timeouts for Blob Service Operations.</a>.
* @param duration Specifies the duration of the lease, in seconds, or negative one (-1) for a lease that never expires. A non-infinite lease can be between 15 and 60 seconds. A lease duration cannot be changed using renew or change.
* @param proposedLeaseId Proposed lease ID, in a GUID string format. The Blob service returns 400 (Invalid request) if the proposed lease ID is not in the correct format. See Guid Constructor (String) for a list of valid GUID string formats.
* @param ifModifiedSince Specify this header value to operate only on a blob if it has been modified since the specified date/time.
* @param ifUnmodifiedSince Specify this header value to operate only on a blob if it has not been modified since the specified date/time.
* @param ifMatches Specify an ETag value to operate only on blobs with a matching value.
* @param ifNoneMatch Specify an ETag value to operate only on blobs without a matching value.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws StorageErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
public void acquireLease(Integer timeout, Integer duration, String proposedLeaseId, OffsetDateTime ifModifiedSince, OffsetDateTime ifUnmodifiedSince, String ifMatches, String ifNoneMatch, String requestId) {
acquireLeaseAsync(timeout, duration, proposedLeaseId, ifModifiedSince, ifUnmodifiedSince, ifMatches, ifNoneMatch, requestId).blockingAwait();
}
/**
* [Update] The Lease Blob operation establishes and manages a lock on a blob for write and delete operations.
*
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting Timeouts for Blob Service Operations.</a>.
* @param duration Specifies the duration of the lease, in seconds, or negative one (-1) for a lease that never expires. A non-infinite lease can be between 15 and 60 seconds. A lease duration cannot be changed using renew or change.
* @param proposedLeaseId Proposed lease ID, in a GUID string format. The Blob service returns 400 (Invalid request) if the proposed lease ID is not in the correct format. See Guid Constructor (String) for a list of valid GUID string formats.
* @param ifModifiedSince Specify this header value to operate only on a blob if it has been modified since the specified date/time.
* @param ifUnmodifiedSince Specify this header value to operate only on a blob if it has not been modified since the specified date/time.
* @param ifMatches Specify an ETag value to operate only on blobs with a matching value.
* @param ifNoneMatch Specify an ETag value to operate only on blobs without a matching value.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @return a ServiceFuture which will be completed with the result of the network request.
*/
public ServiceFuture acquireLeaseAsync(Integer timeout, Integer duration, String proposedLeaseId, OffsetDateTime ifModifiedSince, OffsetDateTime ifUnmodifiedSince, String ifMatches, String ifNoneMatch, String requestId, ServiceCallback serviceCallback) {
return ServiceFuture.fromBody(acquireLeaseAsync(timeout, duration, proposedLeaseId, ifModifiedSince, ifUnmodifiedSince, ifMatches, ifNoneMatch, requestId), serviceCallback);
}
/**
* [Update] The Lease Blob operation establishes and manages a lock on a blob for write and delete operations.
*
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting Timeouts for Blob Service Operations.</a>.
* @param duration Specifies the duration of the lease, in seconds, or negative one (-1) for a lease that never expires. A non-infinite lease can be between 15 and 60 seconds. A lease duration cannot be changed using renew or change.
* @param proposedLeaseId Proposed lease ID, in a GUID string format. The Blob service returns 400 (Invalid request) if the proposed lease ID is not in the correct format. See Guid Constructor (String) for a list of valid GUID string formats.
* @param ifModifiedSince Specify this header value to operate only on a blob if it has been modified since the specified date/time.
* @param ifUnmodifiedSince Specify this header value to operate only on a blob if it has not been modified since the specified date/time.
* @param ifMatches Specify an ETag value to operate only on blobs with a matching value.
* @param ifNoneMatch Specify an ETag value to operate only on blobs without a matching value.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @return a Single which performs the network request upon subscription.
*/
public Single acquireLeaseWithRestResponseAsync(Integer timeout, Integer duration, String proposedLeaseId, OffsetDateTime ifModifiedSince, OffsetDateTime ifUnmodifiedSince, String ifMatches, String ifNoneMatch, String requestId) {
if (this.client.url() == null) {
throw new IllegalArgumentException("Parameter this.client.url() is required and cannot be null.");
}
if (this.client.version() == null) {
throw new IllegalArgumentException("Parameter this.client.version() is required and cannot be null.");
}
final String comp = "lease";
final String action = "acquire";
DateTimeRfc1123 ifModifiedSinceConverted = null;
if (ifModifiedSince != null) {
ifModifiedSinceConverted = new DateTimeRfc1123(ifModifiedSince);
}
DateTimeRfc1123 ifUnmodifiedSinceConverted = null;
if (ifUnmodifiedSince != null) {
ifUnmodifiedSinceConverted = new DateTimeRfc1123(ifUnmodifiedSince);
}
return service.acquireLease(this.client.url(), timeout, duration, proposedLeaseId, ifModifiedSinceConverted, ifUnmodifiedSinceConverted, ifMatches, ifNoneMatch, this.client.version(), requestId, comp, action);
}
/**
* [Update] The Lease Blob operation establishes and manages a lock on a blob for write and delete operations.
*
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting Timeouts for Blob Service Operations.</a>.
* @param duration Specifies the duration of the lease, in seconds, or negative one (-1) for a lease that never expires. A non-infinite lease can be between 15 and 60 seconds. A lease duration cannot be changed using renew or change.
* @param proposedLeaseId Proposed lease ID, in a GUID string format. The Blob service returns 400 (Invalid request) if the proposed lease ID is not in the correct format. See Guid Constructor (String) for a list of valid GUID string formats.
* @param ifModifiedSince Specify this header value to operate only on a blob if it has been modified since the specified date/time.
* @param ifUnmodifiedSince Specify this header value to operate only on a blob if it has not been modified since the specified date/time.
* @param ifMatches Specify an ETag value to operate only on blobs with a matching value.
* @param ifNoneMatch Specify an ETag value to operate only on blobs without a matching value.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @return a Single which performs the network request upon subscription.
*/
public Completable acquireLeaseAsync(Integer timeout, Integer duration, String proposedLeaseId, OffsetDateTime ifModifiedSince, OffsetDateTime ifUnmodifiedSince, String ifMatches, String ifNoneMatch, String requestId) {
return acquireLeaseWithRestResponseAsync(timeout, duration, proposedLeaseId, ifModifiedSince, ifUnmodifiedSince, ifMatches, ifNoneMatch, requestId)
.toCompletable();
}
/**
* [Update] The Lease Blob operation establishes and manages a lock on a blob for write and delete operations.
*
* @param leaseId If specified, the operation only succeeds if the container's lease is active and matches this ID.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting Timeouts for Blob Service Operations.</a>.
* @param ifModifiedSince Specify this header value to operate only on a blob if it has been modified since the specified date/time.
* @param ifUnmodifiedSince Specify this header value to operate only on a blob if it has not been modified since the specified date/time.
* @param ifMatches Specify an ETag value to operate only on blobs with a matching value.
* @param ifNoneMatch Specify an ETag value to operate only on blobs without a matching value.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws StorageErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
public void releaseLease(@NonNull String leaseId, Integer timeout, OffsetDateTime ifModifiedSince, OffsetDateTime ifUnmodifiedSince, String ifMatches, String ifNoneMatch, String requestId) {
releaseLeaseAsync(leaseId, timeout, ifModifiedSince, ifUnmodifiedSince, ifMatches, ifNoneMatch, requestId).blockingAwait();
}
/**
* [Update] The Lease Blob operation establishes and manages a lock on a blob for write and delete operations.
*
* @param leaseId If specified, the operation only succeeds if the container's lease is active and matches this ID.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting Timeouts for Blob Service Operations.</a>.
* @param ifModifiedSince Specify this header value to operate only on a blob if it has been modified since the specified date/time.
* @param ifUnmodifiedSince Specify this header value to operate only on a blob if it has not been modified since the specified date/time.
* @param ifMatches Specify an ETag value to operate only on blobs with a matching value.
* @param ifNoneMatch Specify an ETag value to operate only on blobs without a matching value.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @return a ServiceFuture which will be completed with the result of the network request.
*/
public ServiceFuture releaseLeaseAsync(@NonNull String leaseId, Integer timeout, OffsetDateTime ifModifiedSince, OffsetDateTime ifUnmodifiedSince, String ifMatches, String ifNoneMatch, String requestId, ServiceCallback serviceCallback) {
return ServiceFuture.fromBody(releaseLeaseAsync(leaseId, timeout, ifModifiedSince, ifUnmodifiedSince, ifMatches, ifNoneMatch, requestId), serviceCallback);
}
/**
* [Update] The Lease Blob operation establishes and manages a lock on a blob for write and delete operations.
*
* @param leaseId If specified, the operation only succeeds if the container's lease is active and matches this ID.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting Timeouts for Blob Service Operations.</a>.
* @param ifModifiedSince Specify this header value to operate only on a blob if it has been modified since the specified date/time.
* @param ifUnmodifiedSince Specify this header value to operate only on a blob if it has not been modified since the specified date/time.
* @param ifMatches Specify an ETag value to operate only on blobs with a matching value.
* @param ifNoneMatch Specify an ETag value to operate only on blobs without a matching value.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @return a Single which performs the network request upon subscription.
*/
public Single releaseLeaseWithRestResponseAsync(@NonNull String leaseId, Integer timeout, OffsetDateTime ifModifiedSince, OffsetDateTime ifUnmodifiedSince, String ifMatches, String ifNoneMatch, String requestId) {
if (this.client.url() == null) {
throw new IllegalArgumentException("Parameter this.client.url() is required and cannot be null.");
}
if (leaseId == null) {
throw new IllegalArgumentException("Parameter leaseId is required and cannot be null.");
}
if (this.client.version() == null) {
throw new IllegalArgumentException("Parameter this.client.version() is required and cannot be null.");
}
final String comp = "lease";
final String action = "release";
DateTimeRfc1123 ifModifiedSinceConverted = null;
if (ifModifiedSince != null) {
ifModifiedSinceConverted = new DateTimeRfc1123(ifModifiedSince);
}
DateTimeRfc1123 ifUnmodifiedSinceConverted = null;
if (ifUnmodifiedSince != null) {
ifUnmodifiedSinceConverted = new DateTimeRfc1123(ifUnmodifiedSince);
}
return service.releaseLease(this.client.url(), timeout, leaseId, ifModifiedSinceConverted, ifUnmodifiedSinceConverted, ifMatches, ifNoneMatch, this.client.version(), requestId, comp, action);
}
/**
* [Update] The Lease Blob operation establishes and manages a lock on a blob for write and delete operations.
*
* @param leaseId If specified, the operation only succeeds if the container's lease is active and matches this ID.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting Timeouts for Blob Service Operations.</a>.
* @param ifModifiedSince Specify this header value to operate only on a blob if it has been modified since the specified date/time.
* @param ifUnmodifiedSince Specify this header value to operate only on a blob if it has not been modified since the specified date/time.
* @param ifMatches Specify an ETag value to operate only on blobs with a matching value.
* @param ifNoneMatch Specify an ETag value to operate only on blobs without a matching value.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @return a Single which performs the network request upon subscription.
*/
public Completable releaseLeaseAsync(@NonNull String leaseId, Integer timeout, OffsetDateTime ifModifiedSince, OffsetDateTime ifUnmodifiedSince, String ifMatches, String ifNoneMatch, String requestId) {
return releaseLeaseWithRestResponseAsync(leaseId, timeout, ifModifiedSince, ifUnmodifiedSince, ifMatches, ifNoneMatch, requestId)
.toCompletable();
}
/**
* [Update] The Lease Blob operation establishes and manages a lock on a blob for write and delete operations.
*
* @param leaseId If specified, the operation only succeeds if the container's lease is active and matches this ID.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting Timeouts for Blob Service Operations.</a>.
* @param ifModifiedSince Specify this header value to operate only on a blob if it has been modified since the specified date/time.
* @param ifUnmodifiedSince Specify this header value to operate only on a blob if it has not been modified since the specified date/time.
* @param ifMatches Specify an ETag value to operate only on blobs with a matching value.
* @param ifNoneMatch Specify an ETag value to operate only on blobs without a matching value.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws StorageErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
public void renewLease(@NonNull String leaseId, Integer timeout, OffsetDateTime ifModifiedSince, OffsetDateTime ifUnmodifiedSince, String ifMatches, String ifNoneMatch, String requestId) {
renewLeaseAsync(leaseId, timeout, ifModifiedSince, ifUnmodifiedSince, ifMatches, ifNoneMatch, requestId).blockingAwait();
}
/**
* [Update] The Lease Blob operation establishes and manages a lock on a blob for write and delete operations.
*
* @param leaseId If specified, the operation only succeeds if the container's lease is active and matches this ID.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting Timeouts for Blob Service Operations.</a>.
* @param ifModifiedSince Specify this header value to operate only on a blob if it has been modified since the specified date/time.
* @param ifUnmodifiedSince Specify this header value to operate only on a blob if it has not been modified since the specified date/time.
* @param ifMatches Specify an ETag value to operate only on blobs with a matching value.
* @param ifNoneMatch Specify an ETag value to operate only on blobs without a matching value.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @return a ServiceFuture which will be completed with the result of the network request.
*/
public ServiceFuture renewLeaseAsync(@NonNull String leaseId, Integer timeout, OffsetDateTime ifModifiedSince, OffsetDateTime ifUnmodifiedSince, String ifMatches, String ifNoneMatch, String requestId, ServiceCallback serviceCallback) {
return ServiceFuture.fromBody(renewLeaseAsync(leaseId, timeout, ifModifiedSince, ifUnmodifiedSince, ifMatches, ifNoneMatch, requestId), serviceCallback);
}
/**
* [Update] The Lease Blob operation establishes and manages a lock on a blob for write and delete operations.
*
* @param leaseId If specified, the operation only succeeds if the container's lease is active and matches this ID.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting Timeouts for Blob Service Operations.</a>.
* @param ifModifiedSince Specify this header value to operate only on a blob if it has been modified since the specified date/time.
* @param ifUnmodifiedSince Specify this header value to operate only on a blob if it has not been modified since the specified date/time.
* @param ifMatches Specify an ETag value to operate only on blobs with a matching value.
* @param ifNoneMatch Specify an ETag value to operate only on blobs without a matching value.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @return a Single which performs the network request upon subscription.
*/
public Single renewLeaseWithRestResponseAsync(@NonNull String leaseId, Integer timeout, OffsetDateTime ifModifiedSince, OffsetDateTime ifUnmodifiedSince, String ifMatches, String ifNoneMatch, String requestId) {
if (this.client.url() == null) {
throw new IllegalArgumentException("Parameter this.client.url() is required and cannot be null.");
}
if (leaseId == null) {
throw new IllegalArgumentException("Parameter leaseId is required and cannot be null.");
}
if (this.client.version() == null) {
throw new IllegalArgumentException("Parameter this.client.version() is required and cannot be null.");
}
final String comp = "lease";
final String action = "renew";
DateTimeRfc1123 ifModifiedSinceConverted = null;
if (ifModifiedSince != null) {
ifModifiedSinceConverted = new DateTimeRfc1123(ifModifiedSince);
}
DateTimeRfc1123 ifUnmodifiedSinceConverted = null;
if (ifUnmodifiedSince != null) {
ifUnmodifiedSinceConverted = new DateTimeRfc1123(ifUnmodifiedSince);
}
return service.renewLease(this.client.url(), timeout, leaseId, ifModifiedSinceConverted, ifUnmodifiedSinceConverted, ifMatches, ifNoneMatch, this.client.version(), requestId, comp, action);
}
/**
* [Update] The Lease Blob operation establishes and manages a lock on a blob for write and delete operations.
*
* @param leaseId If specified, the operation only succeeds if the container's lease is active and matches this ID.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting Timeouts for Blob Service Operations.</a>.
* @param ifModifiedSince Specify this header value to operate only on a blob if it has been modified since the specified date/time.
* @param ifUnmodifiedSince Specify this header value to operate only on a blob if it has not been modified since the specified date/time.
* @param ifMatches Specify an ETag value to operate only on blobs with a matching value.
* @param ifNoneMatch Specify an ETag value to operate only on blobs without a matching value.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @return a Single which performs the network request upon subscription.
*/
public Completable renewLeaseAsync(@NonNull String leaseId, Integer timeout, OffsetDateTime ifModifiedSince, OffsetDateTime ifUnmodifiedSince, String ifMatches, String ifNoneMatch, String requestId) {
return renewLeaseWithRestResponseAsync(leaseId, timeout, ifModifiedSince, ifUnmodifiedSince, ifMatches, ifNoneMatch, requestId)
.toCompletable();
}
/**
* [Update] The Lease Blob operation establishes and manages a lock on a blob for write and delete operations.
*
* @param leaseId If specified, the operation only succeeds if the container's lease is active and matches this ID.
* @param proposedLeaseId Proposed lease ID, in a GUID string format. The Blob service returns 400 (Invalid request) if the proposed lease ID is not in the correct format. See Guid Constructor (String) for a list of valid GUID string formats.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting Timeouts for Blob Service Operations.</a>.
* @param ifModifiedSince Specify this header value to operate only on a blob if it has been modified since the specified date/time.
* @param ifUnmodifiedSince Specify this header value to operate only on a blob if it has not been modified since the specified date/time.
* @param ifMatches Specify an ETag value to operate only on blobs with a matching value.
* @param ifNoneMatch Specify an ETag value to operate only on blobs without a matching value.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws StorageErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
public void changeLease(@NonNull String leaseId, @NonNull String proposedLeaseId, Integer timeout, OffsetDateTime ifModifiedSince, OffsetDateTime ifUnmodifiedSince, String ifMatches, String ifNoneMatch, String requestId) {
changeLeaseAsync(leaseId, proposedLeaseId, timeout, ifModifiedSince, ifUnmodifiedSince, ifMatches, ifNoneMatch, requestId).blockingAwait();
}
/**
* [Update] The Lease Blob operation establishes and manages a lock on a blob for write and delete operations.
*
* @param leaseId If specified, the operation only succeeds if the container's lease is active and matches this ID.
* @param proposedLeaseId Proposed lease ID, in a GUID string format. The Blob service returns 400 (Invalid request) if the proposed lease ID is not in the correct format. See Guid Constructor (String) for a list of valid GUID string formats.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting Timeouts for Blob Service Operations.</a>.
* @param ifModifiedSince Specify this header value to operate only on a blob if it has been modified since the specified date/time.
* @param ifUnmodifiedSince Specify this header value to operate only on a blob if it has not been modified since the specified date/time.
* @param ifMatches Specify an ETag value to operate only on blobs with a matching value.
* @param ifNoneMatch Specify an ETag value to operate only on blobs without a matching value.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @return a ServiceFuture which will be completed with the result of the network request.
*/
public ServiceFuture changeLeaseAsync(@NonNull String leaseId, @NonNull String proposedLeaseId, Integer timeout, OffsetDateTime ifModifiedSince, OffsetDateTime ifUnmodifiedSince, String ifMatches, String ifNoneMatch, String requestId, ServiceCallback serviceCallback) {
return ServiceFuture.fromBody(changeLeaseAsync(leaseId, proposedLeaseId, timeout, ifModifiedSince, ifUnmodifiedSince, ifMatches, ifNoneMatch, requestId), serviceCallback);
}
/**
* [Update] The Lease Blob operation establishes and manages a lock on a blob for write and delete operations.
*
* @param leaseId If specified, the operation only succeeds if the container's lease is active and matches this ID.
* @param proposedLeaseId Proposed lease ID, in a GUID string format. The Blob service returns 400 (Invalid request) if the proposed lease ID is not in the correct format. See Guid Constructor (String) for a list of valid GUID string formats.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting Timeouts for Blob Service Operations.</a>.
* @param ifModifiedSince Specify this header value to operate only on a blob if it has been modified since the specified date/time.
* @param ifUnmodifiedSince Specify this header value to operate only on a blob if it has not been modified since the specified date/time.
* @param ifMatches Specify an ETag value to operate only on blobs with a matching value.
* @param ifNoneMatch Specify an ETag value to operate only on blobs without a matching value.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @return a Single which performs the network request upon subscription.
*/
public Single changeLeaseWithRestResponseAsync(@NonNull String leaseId, @NonNull String proposedLeaseId, Integer timeout, OffsetDateTime ifModifiedSince, OffsetDateTime ifUnmodifiedSince, String ifMatches, String ifNoneMatch, String requestId) {
if (this.client.url() == null) {
throw new IllegalArgumentException("Parameter this.client.url() is required and cannot be null.");
}
if (leaseId == null) {
throw new IllegalArgumentException("Parameter leaseId is required and cannot be null.");
}
if (proposedLeaseId == null) {
throw new IllegalArgumentException("Parameter proposedLeaseId is required and cannot be null.");
}
if (this.client.version() == null) {
throw new IllegalArgumentException("Parameter this.client.version() is required and cannot be null.");
}
final String comp = "lease";
final String action = "change";
DateTimeRfc1123 ifModifiedSinceConverted = null;
if (ifModifiedSince != null) {
ifModifiedSinceConverted = new DateTimeRfc1123(ifModifiedSince);
}
DateTimeRfc1123 ifUnmodifiedSinceConverted = null;
if (ifUnmodifiedSince != null) {
ifUnmodifiedSinceConverted = new DateTimeRfc1123(ifUnmodifiedSince);
}
return service.changeLease(this.client.url(), timeout, leaseId, proposedLeaseId, ifModifiedSinceConverted, ifUnmodifiedSinceConverted, ifMatches, ifNoneMatch, this.client.version(), requestId, comp, action);
}
/**
* [Update] The Lease Blob operation establishes and manages a lock on a blob for write and delete operations.
*
* @param leaseId If specified, the operation only succeeds if the container's lease is active and matches this ID.
* @param proposedLeaseId Proposed lease ID, in a GUID string format. The Blob service returns 400 (Invalid request) if the proposed lease ID is not in the correct format. See Guid Constructor (String) for a list of valid GUID string formats.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting Timeouts for Blob Service Operations.</a>.
* @param ifModifiedSince Specify this header value to operate only on a blob if it has been modified since the specified date/time.
* @param ifUnmodifiedSince Specify this header value to operate only on a blob if it has not been modified since the specified date/time.
* @param ifMatches Specify an ETag value to operate only on blobs with a matching value.
* @param ifNoneMatch Specify an ETag value to operate only on blobs without a matching value.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @return a Single which performs the network request upon subscription.
*/
public Completable changeLeaseAsync(@NonNull String leaseId, @NonNull String proposedLeaseId, Integer timeout, OffsetDateTime ifModifiedSince, OffsetDateTime ifUnmodifiedSince, String ifMatches, String ifNoneMatch, String requestId) {
return changeLeaseWithRestResponseAsync(leaseId, proposedLeaseId, timeout, ifModifiedSince, ifUnmodifiedSince, ifMatches, ifNoneMatch, requestId)
.toCompletable();
}
/**
* [Update] The Lease Blob operation establishes and manages a lock on a blob for write and delete operations.
*
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting Timeouts for Blob Service Operations.</a>.
* @param breakPeriod For a break operation, proposed duration the lease should continue before it is broken, in seconds, between 0 and 60. This break period is only used if it is shorter than the time remaining on the lease. If longer, the time remaining on the lease is used. A new lease will not be available before the break period has expired, but the lease may be held for longer than the break period. If this header does not appear with a break operation, a fixed-duration lease breaks after the remaining lease period elapses, and an infinite lease breaks immediately.
* @param ifModifiedSince Specify this header value to operate only on a blob if it has been modified since the specified date/time.
* @param ifUnmodifiedSince Specify this header value to operate only on a blob if it has not been modified since the specified date/time.
* @param ifMatches Specify an ETag value to operate only on blobs with a matching value.
* @param ifNoneMatch Specify an ETag value to operate only on blobs without a matching value.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws StorageErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
public void breakLease(Integer timeout, Integer breakPeriod, OffsetDateTime ifModifiedSince, OffsetDateTime ifUnmodifiedSince, String ifMatches, String ifNoneMatch, String requestId) {
breakLeaseAsync(timeout, breakPeriod, ifModifiedSince, ifUnmodifiedSince, ifMatches, ifNoneMatch, requestId).blockingAwait();
}
/**
* [Update] The Lease Blob operation establishes and manages a lock on a blob for write and delete operations.
*
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting Timeouts for Blob Service Operations.</a>.
* @param breakPeriod For a break operation, proposed duration the lease should continue before it is broken, in seconds, between 0 and 60. This break period is only used if it is shorter than the time remaining on the lease. If longer, the time remaining on the lease is used. A new lease will not be available before the break period has expired, but the lease may be held for longer than the break period. If this header does not appear with a break operation, a fixed-duration lease breaks after the remaining lease period elapses, and an infinite lease breaks immediately.
* @param ifModifiedSince Specify this header value to operate only on a blob if it has been modified since the specified date/time.
* @param ifUnmodifiedSince Specify this header value to operate only on a blob if it has not been modified since the specified date/time.
* @param ifMatches Specify an ETag value to operate only on blobs with a matching value.
* @param ifNoneMatch Specify an ETag value to operate only on blobs without a matching value.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @return a ServiceFuture which will be completed with the result of the network request.
*/
public ServiceFuture breakLeaseAsync(Integer timeout, Integer breakPeriod, OffsetDateTime ifModifiedSince, OffsetDateTime ifUnmodifiedSince, String ifMatches, String ifNoneMatch, String requestId, ServiceCallback serviceCallback) {
return ServiceFuture.fromBody(breakLeaseAsync(timeout, breakPeriod, ifModifiedSince, ifUnmodifiedSince, ifMatches, ifNoneMatch, requestId), serviceCallback);
}
/**
* [Update] The Lease Blob operation establishes and manages a lock on a blob for write and delete operations.
*
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting Timeouts for Blob Service Operations.</a>.
* @param breakPeriod For a break operation, proposed duration the lease should continue before it is broken, in seconds, between 0 and 60. This break period is only used if it is shorter than the time remaining on the lease. If longer, the time remaining on the lease is used. A new lease will not be available before the break period has expired, but the lease may be held for longer than the break period. If this header does not appear with a break operation, a fixed-duration lease breaks after the remaining lease period elapses, and an infinite lease breaks immediately.
* @param ifModifiedSince Specify this header value to operate only on a blob if it has been modified since the specified date/time.
* @param ifUnmodifiedSince Specify this header value to operate only on a blob if it has not been modified since the specified date/time.
* @param ifMatches Specify an ETag value to operate only on blobs with a matching value.
* @param ifNoneMatch Specify an ETag value to operate only on blobs without a matching value.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @return a Single which performs the network request upon subscription.
*/
public Single breakLeaseWithRestResponseAsync(Integer timeout, Integer breakPeriod, OffsetDateTime ifModifiedSince, OffsetDateTime ifUnmodifiedSince, String ifMatches, String ifNoneMatch, String requestId) {
if (this.client.url() == null) {
throw new IllegalArgumentException("Parameter this.client.url() is required and cannot be null.");
}
if (this.client.version() == null) {
throw new IllegalArgumentException("Parameter this.client.version() is required and cannot be null.");
}
final String comp = "lease";
final String action = "break";
DateTimeRfc1123 ifModifiedSinceConverted = null;
if (ifModifiedSince != null) {
ifModifiedSinceConverted = new DateTimeRfc1123(ifModifiedSince);
}
DateTimeRfc1123 ifUnmodifiedSinceConverted = null;
if (ifUnmodifiedSince != null) {
ifUnmodifiedSinceConverted = new DateTimeRfc1123(ifUnmodifiedSince);
}
return service.breakLease(this.client.url(), timeout, breakPeriod, ifModifiedSinceConverted, ifUnmodifiedSinceConverted, ifMatches, ifNoneMatch, this.client.version(), requestId, comp, action);
}
/**
* [Update] The Lease Blob operation establishes and manages a lock on a blob for write and delete operations.
*
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting Timeouts for Blob Service Operations.</a>.
* @param breakPeriod For a break operation, proposed duration the lease should continue before it is broken, in seconds, between 0 and 60. This break period is only used if it is shorter than the time remaining on the lease. If longer, the time remaining on the lease is used. A new lease will not be available before the break period has expired, but the lease may be held for longer than the break period. If this header does not appear with a break operation, a fixed-duration lease breaks after the remaining lease period elapses, and an infinite lease breaks immediately.
* @param ifModifiedSince Specify this header value to operate only on a blob if it has been modified since the specified date/time.
* @param ifUnmodifiedSince Specify this header value to operate only on a blob if it has not been modified since the specified date/time.
* @param ifMatches Specify an ETag value to operate only on blobs with a matching value.
* @param ifNoneMatch Specify an ETag value to operate only on blobs without a matching value.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @return a Single which performs the network request upon subscription.
*/
public Completable breakLeaseAsync(Integer timeout, Integer breakPeriod, OffsetDateTime ifModifiedSince, OffsetDateTime ifUnmodifiedSince, String ifMatches, String ifNoneMatch, String requestId) {
return breakLeaseWithRestResponseAsync(timeout, breakPeriod, ifModifiedSince, ifUnmodifiedSince, ifMatches, ifNoneMatch, requestId)
.toCompletable();
}
/**
* The Create Snapshot operation creates a read-only snapshot of a blob.
*
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting Timeouts for Blob Service Operations.</a>.
* @param metadata Optional. Specifies a user-defined name-value pair associated with the blob. If no name-value pairs are specified, the operation will copy the metadata from the source blob or file to the destination blob. If one or more name-value pairs are specified, the destination blob is created with the specified metadata, and metadata is not copied from the source blob or file. Note that beginning with version 2009-09-19, metadata names must adhere to the naming rules for C# identifiers. See Naming and Referencing Containers, Blobs, and Metadata for more information.
* @param ifModifiedSince Specify this header value to operate only on a blob if it has been modified since the specified date/time.
* @param ifUnmodifiedSince Specify this header value to operate only on a blob if it has not been modified since the specified date/time.
* @param ifMatches Specify an ETag value to operate only on blobs with a matching value.
* @param ifNoneMatch Specify an ETag value to operate only on blobs without a matching value.
* @param leaseId If specified, the operation only succeeds if the container's lease is active and matches this ID.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws StorageErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
public void createSnapshot(Integer timeout, Map metadata, OffsetDateTime ifModifiedSince, OffsetDateTime ifUnmodifiedSince, String ifMatches, String ifNoneMatch, String leaseId, String requestId) {
createSnapshotAsync(timeout, metadata, ifModifiedSince, ifUnmodifiedSince, ifMatches, ifNoneMatch, leaseId, requestId).blockingAwait();
}
/**
* The Create Snapshot operation creates a read-only snapshot of a blob.
*
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting Timeouts for Blob Service Operations.</a>.
* @param metadata Optional. Specifies a user-defined name-value pair associated with the blob. If no name-value pairs are specified, the operation will copy the metadata from the source blob or file to the destination blob. If one or more name-value pairs are specified, the destination blob is created with the specified metadata, and metadata is not copied from the source blob or file. Note that beginning with version 2009-09-19, metadata names must adhere to the naming rules for C# identifiers. See Naming and Referencing Containers, Blobs, and Metadata for more information.
* @param ifModifiedSince Specify this header value to operate only on a blob if it has been modified since the specified date/time.
* @param ifUnmodifiedSince Specify this header value to operate only on a blob if it has not been modified since the specified date/time.
* @param ifMatches Specify an ETag value to operate only on blobs with a matching value.
* @param ifNoneMatch Specify an ETag value to operate only on blobs without a matching value.
* @param leaseId If specified, the operation only succeeds if the container's lease is active and matches this ID.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @return a ServiceFuture which will be completed with the result of the network request.
*/
public ServiceFuture createSnapshotAsync(Integer timeout, Map metadata, OffsetDateTime ifModifiedSince, OffsetDateTime ifUnmodifiedSince, String ifMatches, String ifNoneMatch, String leaseId, String requestId, ServiceCallback serviceCallback) {
return ServiceFuture.fromBody(createSnapshotAsync(timeout, metadata, ifModifiedSince, ifUnmodifiedSince, ifMatches, ifNoneMatch, leaseId, requestId), serviceCallback);
}
/**
* The Create Snapshot operation creates a read-only snapshot of a blob.
*
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting Timeouts for Blob Service Operations.</a>.
* @param metadata Optional. Specifies a user-defined name-value pair associated with the blob. If no name-value pairs are specified, the operation will copy the metadata from the source blob or file to the destination blob. If one or more name-value pairs are specified, the destination blob is created with the specified metadata, and metadata is not copied from the source blob or file. Note that beginning with version 2009-09-19, metadata names must adhere to the naming rules for C# identifiers. See Naming and Referencing Containers, Blobs, and Metadata for more information.
* @param ifModifiedSince Specify this header value to operate only on a blob if it has been modified since the specified date/time.
* @param ifUnmodifiedSince Specify this header value to operate only on a blob if it has not been modified since the specified date/time.
* @param ifMatches Specify an ETag value to operate only on blobs with a matching value.
* @param ifNoneMatch Specify an ETag value to operate only on blobs without a matching value.
* @param leaseId If specified, the operation only succeeds if the container's lease is active and matches this ID.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @return a Single which performs the network request upon subscription.
*/
public Single createSnapshotWithRestResponseAsync(Integer timeout, Map metadata, OffsetDateTime ifModifiedSince, OffsetDateTime ifUnmodifiedSince, String ifMatches, String ifNoneMatch, String leaseId, String requestId) {
if (this.client.url() == null) {
throw new IllegalArgumentException("Parameter this.client.url() is required and cannot be null.");
}
if (this.client.version() == null) {
throw new IllegalArgumentException("Parameter this.client.version() is required and cannot be null.");
}
Validator.validate(metadata);
final String comp = "snapshot";
DateTimeRfc1123 ifModifiedSinceConverted = null;
if (ifModifiedSince != null) {
ifModifiedSinceConverted = new DateTimeRfc1123(ifModifiedSince);
}
DateTimeRfc1123 ifUnmodifiedSinceConverted = null;
if (ifUnmodifiedSince != null) {
ifUnmodifiedSinceConverted = new DateTimeRfc1123(ifUnmodifiedSince);
}
return service.createSnapshot(this.client.url(), timeout, metadata, ifModifiedSinceConverted, ifUnmodifiedSinceConverted, ifMatches, ifNoneMatch, leaseId, this.client.version(), requestId, comp);
}
/**
* The Create Snapshot operation creates a read-only snapshot of a blob.
*
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting Timeouts for Blob Service Operations.</a>.
* @param metadata Optional. Specifies a user-defined name-value pair associated with the blob. If no name-value pairs are specified, the operation will copy the metadata from the source blob or file to the destination blob. If one or more name-value pairs are specified, the destination blob is created with the specified metadata, and metadata is not copied from the source blob or file. Note that beginning with version 2009-09-19, metadata names must adhere to the naming rules for C# identifiers. See Naming and Referencing Containers, Blobs, and Metadata for more information.
* @param ifModifiedSince Specify this header value to operate only on a blob if it has been modified since the specified date/time.
* @param ifUnmodifiedSince Specify this header value to operate only on a blob if it has not been modified since the specified date/time.
* @param ifMatches Specify an ETag value to operate only on blobs with a matching value.
* @param ifNoneMatch Specify an ETag value to operate only on blobs without a matching value.
* @param leaseId If specified, the operation only succeeds if the container's lease is active and matches this ID.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @return a Single which performs the network request upon subscription.
*/
public Completable createSnapshotAsync(Integer timeout, Map metadata, OffsetDateTime ifModifiedSince, OffsetDateTime ifUnmodifiedSince, String ifMatches, String ifNoneMatch, String leaseId, String requestId) {
return createSnapshotWithRestResponseAsync(timeout, metadata, ifModifiedSince, ifUnmodifiedSince, ifMatches, ifNoneMatch, leaseId, requestId)
.toCompletable();
}
/**
* The Start Copy From URL operation copies a blob or an internet resource to a new blob.
*
* @param copySource Specifies the name of the source page blob snapshot. This value is a URL of up to 2 KB in length that specifies a page blob snapshot. The value should be URL-encoded as it would appear in a request URI. The source blob must either be public or must be authenticated via a shared access signature.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting Timeouts for Blob Service Operations.</a>.
* @param metadata Optional. Specifies a user-defined name-value pair associated with the blob. If no name-value pairs are specified, the operation will copy the metadata from the source blob or file to the destination blob. If one or more name-value pairs are specified, the destination blob is created with the specified metadata, and metadata is not copied from the source blob or file. Note that beginning with version 2009-09-19, metadata names must adhere to the naming rules for C# identifiers. See Naming and Referencing Containers, Blobs, and Metadata for more information.
* @param sourceIfModifiedSince Specify this header value to operate only on a blob if it has been modified since the specified date/time.
* @param sourceIfUnmodifiedSince Specify this header value to operate only on a blob if it has not been modified since the specified date/time.
* @param sourceIfMatches Specify an ETag value to operate only on blobs with a matching value.
* @param sourceIfNoneMatch Specify an ETag value to operate only on blobs without a matching value.
* @param ifModifiedSince Specify this header value to operate only on a blob if it has been modified since the specified date/time.
* @param ifUnmodifiedSince Specify this header value to operate only on a blob if it has not been modified since the specified date/time.
* @param ifMatches Specify an ETag value to operate only on blobs with a matching value.
* @param ifNoneMatch Specify an ETag value to operate only on blobs without a matching value.
* @param leaseId If specified, the operation only succeeds if the container's lease is active and matches this ID.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws StorageErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
public void startCopyFromURL(@NonNull URL copySource, Integer timeout, Map metadata, OffsetDateTime sourceIfModifiedSince, OffsetDateTime sourceIfUnmodifiedSince, String sourceIfMatches, String sourceIfNoneMatch, OffsetDateTime ifModifiedSince, OffsetDateTime ifUnmodifiedSince, String ifMatches, String ifNoneMatch, String leaseId, String requestId) {
startCopyFromURLAsync(copySource, timeout, metadata, sourceIfModifiedSince, sourceIfUnmodifiedSince, sourceIfMatches, sourceIfNoneMatch, ifModifiedSince, ifUnmodifiedSince, ifMatches, ifNoneMatch, leaseId, requestId).blockingAwait();
}
/**
* The Start Copy From URL operation copies a blob or an internet resource to a new blob.
*
* @param copySource Specifies the name of the source page blob snapshot. This value is a URL of up to 2 KB in length that specifies a page blob snapshot. The value should be URL-encoded as it would appear in a request URI. The source blob must either be public or must be authenticated via a shared access signature.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting Timeouts for Blob Service Operations.</a>.
* @param metadata Optional. Specifies a user-defined name-value pair associated with the blob. If no name-value pairs are specified, the operation will copy the metadata from the source blob or file to the destination blob. If one or more name-value pairs are specified, the destination blob is created with the specified metadata, and metadata is not copied from the source blob or file. Note that beginning with version 2009-09-19, metadata names must adhere to the naming rules for C# identifiers. See Naming and Referencing Containers, Blobs, and Metadata for more information.
* @param sourceIfModifiedSince Specify this header value to operate only on a blob if it has been modified since the specified date/time.
* @param sourceIfUnmodifiedSince Specify this header value to operate only on a blob if it has not been modified since the specified date/time.
* @param sourceIfMatches Specify an ETag value to operate only on blobs with a matching value.
* @param sourceIfNoneMatch Specify an ETag value to operate only on blobs without a matching value.
* @param ifModifiedSince Specify this header value to operate only on a blob if it has been modified since the specified date/time.
* @param ifUnmodifiedSince Specify this header value to operate only on a blob if it has not been modified since the specified date/time.
* @param ifMatches Specify an ETag value to operate only on blobs with a matching value.
* @param ifNoneMatch Specify an ETag value to operate only on blobs without a matching value.
* @param leaseId If specified, the operation only succeeds if the container's lease is active and matches this ID.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @return a ServiceFuture which will be completed with the result of the network request.
*/
public ServiceFuture startCopyFromURLAsync(@NonNull URL copySource, Integer timeout, Map metadata, OffsetDateTime sourceIfModifiedSince, OffsetDateTime sourceIfUnmodifiedSince, String sourceIfMatches, String sourceIfNoneMatch, OffsetDateTime ifModifiedSince, OffsetDateTime ifUnmodifiedSince, String ifMatches, String ifNoneMatch, String leaseId, String requestId, ServiceCallback serviceCallback) {
return ServiceFuture.fromBody(startCopyFromURLAsync(copySource, timeout, metadata, sourceIfModifiedSince, sourceIfUnmodifiedSince, sourceIfMatches, sourceIfNoneMatch, ifModifiedSince, ifUnmodifiedSince, ifMatches, ifNoneMatch, leaseId, requestId), serviceCallback);
}
/**
* The Start Copy From URL operation copies a blob or an internet resource to a new blob.
*
* @param copySource Specifies the name of the source page blob snapshot. This value is a URL of up to 2 KB in length that specifies a page blob snapshot. The value should be URL-encoded as it would appear in a request URI. The source blob must either be public or must be authenticated via a shared access signature.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting Timeouts for Blob Service Operations.</a>.
* @param metadata Optional. Specifies a user-defined name-value pair associated with the blob. If no name-value pairs are specified, the operation will copy the metadata from the source blob or file to the destination blob. If one or more name-value pairs are specified, the destination blob is created with the specified metadata, and metadata is not copied from the source blob or file. Note that beginning with version 2009-09-19, metadata names must adhere to the naming rules for C# identifiers. See Naming and Referencing Containers, Blobs, and Metadata for more information.
* @param sourceIfModifiedSince Specify this header value to operate only on a blob if it has been modified since the specified date/time.
* @param sourceIfUnmodifiedSince Specify this header value to operate only on a blob if it has not been modified since the specified date/time.
* @param sourceIfMatches Specify an ETag value to operate only on blobs with a matching value.
* @param sourceIfNoneMatch Specify an ETag value to operate only on blobs without a matching value.
* @param ifModifiedSince Specify this header value to operate only on a blob if it has been modified since the specified date/time.
* @param ifUnmodifiedSince Specify this header value to operate only on a blob if it has not been modified since the specified date/time.
* @param ifMatches Specify an ETag value to operate only on blobs with a matching value.
* @param ifNoneMatch Specify an ETag value to operate only on blobs without a matching value.
* @param leaseId If specified, the operation only succeeds if the container's lease is active and matches this ID.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @return a Single which performs the network request upon subscription.
*/
public Single startCopyFromURLWithRestResponseAsync(@NonNull URL copySource, Integer timeout, Map metadata, OffsetDateTime sourceIfModifiedSince, OffsetDateTime sourceIfUnmodifiedSince, String sourceIfMatches, String sourceIfNoneMatch, OffsetDateTime ifModifiedSince, OffsetDateTime ifUnmodifiedSince, String ifMatches, String ifNoneMatch, String leaseId, String requestId) {
if (this.client.url() == null) {
throw new IllegalArgumentException("Parameter this.client.url() is required and cannot be null.");
}
if (copySource == null) {
throw new IllegalArgumentException("Parameter copySource is required and cannot be null.");
}
if (this.client.version() == null) {
throw new IllegalArgumentException("Parameter this.client.version() is required and cannot be null.");
}
Validator.validate(metadata);
Validator.validate(copySource);
DateTimeRfc1123 sourceIfModifiedSinceConverted = null;
if (sourceIfModifiedSince != null) {
sourceIfModifiedSinceConverted = new DateTimeRfc1123(sourceIfModifiedSince);
}
DateTimeRfc1123 sourceIfUnmodifiedSinceConverted = null;
if (sourceIfUnmodifiedSince != null) {
sourceIfUnmodifiedSinceConverted = new DateTimeRfc1123(sourceIfUnmodifiedSince);
}
DateTimeRfc1123 ifModifiedSinceConverted = null;
if (ifModifiedSince != null) {
ifModifiedSinceConverted = new DateTimeRfc1123(ifModifiedSince);
}
DateTimeRfc1123 ifUnmodifiedSinceConverted = null;
if (ifUnmodifiedSince != null) {
ifUnmodifiedSinceConverted = new DateTimeRfc1123(ifUnmodifiedSince);
}
return service.startCopyFromURL(this.client.url(), timeout, metadata, sourceIfModifiedSinceConverted, sourceIfUnmodifiedSinceConverted, sourceIfMatches, sourceIfNoneMatch, ifModifiedSinceConverted, ifUnmodifiedSinceConverted, ifMatches, ifNoneMatch, copySource, leaseId, this.client.version(), requestId);
}
/**
* The Start Copy From URL operation copies a blob or an internet resource to a new blob.
*
* @param copySource Specifies the name of the source page blob snapshot. This value is a URL of up to 2 KB in length that specifies a page blob snapshot. The value should be URL-encoded as it would appear in a request URI. The source blob must either be public or must be authenticated via a shared access signature.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting Timeouts for Blob Service Operations.</a>.
* @param metadata Optional. Specifies a user-defined name-value pair associated with the blob. If no name-value pairs are specified, the operation will copy the metadata from the source blob or file to the destination blob. If one or more name-value pairs are specified, the destination blob is created with the specified metadata, and metadata is not copied from the source blob or file. Note that beginning with version 2009-09-19, metadata names must adhere to the naming rules for C# identifiers. See Naming and Referencing Containers, Blobs, and Metadata for more information.
* @param sourceIfModifiedSince Specify this header value to operate only on a blob if it has been modified since the specified date/time.
* @param sourceIfUnmodifiedSince Specify this header value to operate only on a blob if it has not been modified since the specified date/time.
* @param sourceIfMatches Specify an ETag value to operate only on blobs with a matching value.
* @param sourceIfNoneMatch Specify an ETag value to operate only on blobs without a matching value.
* @param ifModifiedSince Specify this header value to operate only on a blob if it has been modified since the specified date/time.
* @param ifUnmodifiedSince Specify this header value to operate only on a blob if it has not been modified since the specified date/time.
* @param ifMatches Specify an ETag value to operate only on blobs with a matching value.
* @param ifNoneMatch Specify an ETag value to operate only on blobs without a matching value.
* @param leaseId If specified, the operation only succeeds if the container's lease is active and matches this ID.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @return a Single which performs the network request upon subscription.
*/
public Completable startCopyFromURLAsync(@NonNull URL copySource, Integer timeout, Map metadata, OffsetDateTime sourceIfModifiedSince, OffsetDateTime sourceIfUnmodifiedSince, String sourceIfMatches, String sourceIfNoneMatch, OffsetDateTime ifModifiedSince, OffsetDateTime ifUnmodifiedSince, String ifMatches, String ifNoneMatch, String leaseId, String requestId) {
return startCopyFromURLWithRestResponseAsync(copySource, timeout, metadata, sourceIfModifiedSince, sourceIfUnmodifiedSince, sourceIfMatches, sourceIfNoneMatch, ifModifiedSince, ifUnmodifiedSince, ifMatches, ifNoneMatch, leaseId, requestId)
.toCompletable();
}
/**
* The Abort Copy From URL operation aborts a pending Copy From URL operation, and leaves a destination blob with zero length and full metadata.
*
* @param copyId The copy identifier provided in the x-ms-copy-id header of the original Copy Blob operation.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting Timeouts for Blob Service Operations.</a>.
* @param leaseId If specified, the operation only succeeds if the container's lease is active and matches this ID.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws StorageErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
public void abortCopyFromURL(@NonNull String copyId, Integer timeout, String leaseId, String requestId) {
abortCopyFromURLAsync(copyId, timeout, leaseId, requestId).blockingAwait();
}
/**
* The Abort Copy From URL operation aborts a pending Copy From URL operation, and leaves a destination blob with zero length and full metadata.
*
* @param copyId The copy identifier provided in the x-ms-copy-id header of the original Copy Blob operation.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting Timeouts for Blob Service Operations.</a>.
* @param leaseId If specified, the operation only succeeds if the container's lease is active and matches this ID.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @return a ServiceFuture which will be completed with the result of the network request.
*/
public ServiceFuture abortCopyFromURLAsync(@NonNull String copyId, Integer timeout, String leaseId, String requestId, ServiceCallback serviceCallback) {
return ServiceFuture.fromBody(abortCopyFromURLAsync(copyId, timeout, leaseId, requestId), serviceCallback);
}
/**
* The Abort Copy From URL operation aborts a pending Copy From URL operation, and leaves a destination blob with zero length and full metadata.
*
* @param copyId The copy identifier provided in the x-ms-copy-id header of the original Copy Blob operation.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting Timeouts for Blob Service Operations.</a>.
* @param leaseId If specified, the operation only succeeds if the container's lease is active and matches this ID.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @return a Single which performs the network request upon subscription.
*/
public Single abortCopyFromURLWithRestResponseAsync(@NonNull String copyId, Integer timeout, String leaseId, String requestId) {
if (this.client.url() == null) {
throw new IllegalArgumentException("Parameter this.client.url() is required and cannot be null.");
}
if (copyId == null) {
throw new IllegalArgumentException("Parameter copyId is required and cannot be null.");
}
if (this.client.version() == null) {
throw new IllegalArgumentException("Parameter this.client.version() is required and cannot be null.");
}
final String comp = "copy";
final String copyActionAbortConstant = "abort";
return service.abortCopyFromURL(this.client.url(), copyId, timeout, leaseId, this.client.version(), requestId, comp, copyActionAbortConstant);
}
/**
* The Abort Copy From URL operation aborts a pending Copy From URL operation, and leaves a destination blob with zero length and full metadata.
*
* @param copyId The copy identifier provided in the x-ms-copy-id header of the original Copy Blob operation.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting Timeouts for Blob Service Operations.</a>.
* @param leaseId If specified, the operation only succeeds if the container's lease is active and matches this ID.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @return a Single which performs the network request upon subscription.
*/
public Completable abortCopyFromURLAsync(@NonNull String copyId, Integer timeout, String leaseId, String requestId) {
return abortCopyFromURLWithRestResponseAsync(copyId, timeout, leaseId, requestId)
.toCompletable();
}
/**
* The Set Tier operation sets the tier on a blob. The operation is allowed on a page blob in a premium storage account and on a block blob in a blob storage account (locally redundant storage only). A premium page blob's tier determines the allowed size, IOPS, and bandwidth of the blob. A block blob's tier determines Hot/Cool/Archive storage type. This operation does not update the blob's ETag.
*
* @param tier Indicates the tier to be set on the blob. Possible values include: 'P4', 'P6', 'P10', 'P20', 'P30', 'P40', 'P50', 'Hot', 'Cool', 'Archive'.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting Timeouts for Blob Service Operations.</a>.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws StorageErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
public void setTier(@NonNull AccessTier tier, Integer timeout, String requestId) {
setTierAsync(tier, timeout, requestId).blockingAwait();
}
/**
* The Set Tier operation sets the tier on a blob. The operation is allowed on a page blob in a premium storage account and on a block blob in a blob storage account (locally redundant storage only). A premium page blob's tier determines the allowed size, IOPS, and bandwidth of the blob. A block blob's tier determines Hot/Cool/Archive storage type. This operation does not update the blob's ETag.
*
* @param tier Indicates the tier to be set on the blob. Possible values include: 'P4', 'P6', 'P10', 'P20', 'P30', 'P40', 'P50', 'Hot', 'Cool', 'Archive'.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting Timeouts for Blob Service Operations.</a>.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @return a ServiceFuture which will be completed with the result of the network request.
*/
public ServiceFuture setTierAsync(@NonNull AccessTier tier, Integer timeout, String requestId, ServiceCallback serviceCallback) {
return ServiceFuture.fromBody(setTierAsync(tier, timeout, requestId), serviceCallback);
}
/**
* The Set Tier operation sets the tier on a blob. The operation is allowed on a page blob in a premium storage account and on a block blob in a blob storage account (locally redundant storage only). A premium page blob's tier determines the allowed size, IOPS, and bandwidth of the blob. A block blob's tier determines Hot/Cool/Archive storage type. This operation does not update the blob's ETag.
*
* @param tier Indicates the tier to be set on the blob. Possible values include: 'P4', 'P6', 'P10', 'P20', 'P30', 'P40', 'P50', 'Hot', 'Cool', 'Archive'.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting Timeouts for Blob Service Operations.</a>.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @return a Single which performs the network request upon subscription.
*/
public Single setTierWithRestResponseAsync(@NonNull AccessTier tier, Integer timeout, String requestId) {
if (this.client.url() == null) {
throw new IllegalArgumentException("Parameter this.client.url() is required and cannot be null.");
}
if (tier == null) {
throw new IllegalArgumentException("Parameter tier is required and cannot be null.");
}
if (this.client.version() == null) {
throw new IllegalArgumentException("Parameter this.client.version() is required and cannot be null.");
}
final String comp = "tier";
return service.setTier(this.client.url(), timeout, tier, this.client.version(), requestId, comp);
}
/**
* The Set Tier operation sets the tier on a blob. The operation is allowed on a page blob in a premium storage account and on a block blob in a blob storage account (locally redundant storage only). A premium page blob's tier determines the allowed size, IOPS, and bandwidth of the blob. A block blob's tier determines Hot/Cool/Archive storage type. This operation does not update the blob's ETag.
*
* @param tier Indicates the tier to be set on the blob. Possible values include: 'P4', 'P6', 'P10', 'P20', 'P30', 'P40', 'P50', 'Hot', 'Cool', 'Archive'.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting Timeouts for Blob Service Operations.</a>.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @return a Single which performs the network request upon subscription.
*/
public Completable setTierAsync(@NonNull AccessTier tier, Integer timeout, String requestId) {
return setTierWithRestResponseAsync(tier, timeout, requestId)
.toCompletable();
}
/**
* Returns the sku name and account kind.
*
* @throws StorageErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
public void getAccountInfo() {
getAccountInfoAsync().blockingAwait();
}
/**
* Returns the sku name and account kind.
*
* @param serviceCallback the async ServiceCallback to handle successful and failed responses.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @return a ServiceFuture which will be completed with the result of the network request.
*/
public ServiceFuture getAccountInfoAsync(ServiceCallback serviceCallback) {
return ServiceFuture.fromBody(getAccountInfoAsync(), serviceCallback);
}
/**
* Returns the sku name and account kind.
*
* @return a Single which performs the network request upon subscription.
*/
public Single getAccountInfoWithRestResponseAsync() {
if (this.client.url() == null) {
throw new IllegalArgumentException("Parameter this.client.url() is required and cannot be null.");
}
if (this.client.version() == null) {
throw new IllegalArgumentException("Parameter this.client.version() is required and cannot be null.");
}
final String restype = "account";
final String comp = "properties";
return service.getAccountInfo(this.client.url(), this.client.version(), restype, comp);
}
/**
* Returns the sku name and account kind.
*
* @return a Single which performs the network request upon subscription.
*/
public Completable getAccountInfoAsync() {
return getAccountInfoWithRestResponseAsync()
.toCompletable();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy