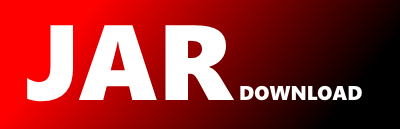
com.microsoft.azure.javamsalruntime.MsalRuntimeLibrary Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of javamsalruntime Show documentation
Show all versions of javamsalruntime Show documentation
The Java/MSALRuntime interop layer facilitates communication between MSAL Java and the MSALRuntime API, allowing developers to easily access WAM from a Java program
The newest version!
// Copyright (c) Microsoft Corporation.
// Licensed under the MIT License.
package com.microsoft.azure.javamsalruntime;
import com.sun.jna.*;
import com.sun.jna.ptr.IntByReference;
import com.sun.jna.ptr.LongByReference;
/**
* Uses Java Native Access (JNA) to call C++ functions from Java, and perform conversions between
* Java classes and native types
*
* In order for JNA to call the correct MSALRuntime API, the names and number of parameters in this
* interface's methods must match those found in MSALRuntime's header files
*
* As for data types, JNA should be able to convert any basic Java type (int, long, String, etc.),
* and it offers a number of classes to mimic native types that have no clear Java equivalent
* (LongByReference for long*, Pointer for void*, etc.)
*/
public interface MsalRuntimeLibrary extends Library {
// MSALRuntime.h
ErrorHandle MSALRUNTIME_Startup();
void MSALRUNTIME_Shutdown();
ErrorHandle MSALRUNTIME_ReadAccountByIdAsync(
WString accountId,
WString correlationId,
Callbacks.ReadAccountResultCallback callback,
Integer callbackData,
AsyncHandle asyncHandle);
ErrorHandle MSALRUNTIME_SignInAsync(
long parentWindowHandle,
long authParametersHandle,
WString correlationId,
WString accountHint,
Callbacks.AuthResultCallback callback,
Integer callbackData,
AsyncHandle asyncHandle);
ErrorHandle MSALRUNTIME_SignInSilentlyAsync(
long authParametersHandle,
WString correlationId,
Callbacks.AuthResultCallback callback,
Integer callbackData,
AsyncHandle asyncHandle);
ErrorHandle MSALRUNTIME_SignInInteractivelyAsync(
long parentWindowHandle,
long authParametersHandle,
WString correlationId,
WString accountHint,
Callbacks.AuthResultCallback callback,
Integer callbackData,
AsyncHandle asyncHandle);
ErrorHandle MSALRUNTIME_AcquireTokenSilentlyAsync(
long authParametersHandle,
WString correlationId,
long accountHandle,
Callbacks.AuthResultCallback callback,
Integer callbackData,
AsyncHandle asyncHandle);
ErrorHandle MSALRUNTIME_AcquireTokenInteractivelyAsync(
long parentWindowHandle,
long authParametersHandle,
WString correlationId,
long accountHandle,
Callbacks.AuthResultCallback callback,
Integer callbackData,
AsyncHandle asyncHandle);
ErrorHandle MSALRUNTIME_SignOutSilentlyAsync(
WString clientId,
WString correlationId,
long accountHandle,
Callbacks.SignOutResultCallback callback,
Integer callbackData,
AsyncHandle asyncHandle);
// MSALRuntimeAccount.h
ErrorHandle MSALRUNTIME_ReleaseAccount(long accountHandle);
ErrorHandle MSALRUNTIME_GetAccountId(long accountHandle, Pointer accountId, IntByReference bufferSize);
ErrorHandle MSALRUNTIME_GetClientInfo(long accountHandle, Pointer clientInfo, IntByReference bufferSize);
// MSALRuntimeAuthParameters.h
ErrorHandle MSALRUNTIME_CreateAuthParameters(WString clientId, WString authority, AuthParametersHandle authParametersHandle);
ErrorHandle MSALRUNTIME_ReleaseAuthParameters(long authParametersHandle);
ErrorHandle MSALRUNTIME_SetRequestedScopes(long authParametersHandle, WString scopes);
ErrorHandle MSALRUNTIME_SetRedirectUri(long authParametersHandle, WString redirectUri);
ErrorHandle MSALRUNTIME_SetDecodedClaims(long authParametersHandle, WString claims);
ErrorHandle MSALRUNTIME_SetAdditionalParameter(long authParametersHandle, WString key, WString value);
ErrorHandle MSALRUNTIME_SetPopParams(
long authParametersHandle,
WString httpMethod,
WString uriHost,
WString uriPath,
WString nonce);
// MSALRuntimeCancel.h
ErrorHandle MSALRUNTIME_ReleaseAsyncHandle(long asyncHandle);
ErrorHandle MSALRUNTIME_CancelAsyncOperation(AsyncHandle asyncHandle);
// MSALRuntimeError.h
ErrorHandle MSALRUNTIME_ReleaseError(long errorHandle);
ErrorHandle MSALRUNTIME_GetStatus(ErrorHandle errorHandle, IntByReference responseStatus);
ErrorHandle MSALRUNTIME_GetStatus(long errorHandle, IntByReference responseStatus);
ErrorHandle MSALRUNTIME_GetErrorCode(long errorHandle, LongByReference responseErrorCode);
ErrorHandle MSALRUNTIME_GetTag(long errorHandle, IntByReference responseErrorTag);
ErrorHandle MSALRUNTIME_GetContext(ErrorHandle errorHandle, Pointer context, IntByReference bufferSize);
// MSALRuntimeAuthResult.h
ErrorHandle MSALRUNTIME_ReleaseAuthResult(long authResultHandle);
ErrorHandle MSALRUNTIME_GetAccount(AuthResultHandle authResultHandle, AccountHandle accountHandle);
ErrorHandle MSALRUNTIME_GetRawIdToken(AuthResultHandle authResultHandle, Pointer rawIdToken, IntByReference bufferSize);
ErrorHandle MSALRUNTIME_GetAccessToken(AuthResultHandle authResultHandle, Pointer accessToken, IntByReference bufferSize);
ErrorHandle MSALRUNTIME_GetError(AuthResultHandle authResultHandle, ErrorHandle errorHandle);
ErrorHandle MSALRUNTIME_IsPopAuthorization(AuthResultHandle authResult, IntByReference isPopAuthorization);
ErrorHandle MSALRUNTIME_GetAuthorizationHeader(AuthResultHandle authResult, Pointer authHeader, IntByReference bufferSize);
// MSALRuntimeReadAccountResult.h
ErrorHandle MSALRUNTIME_ReleaseReadAccountResult(long readAccountResultHandle);
ErrorHandle MSALRUNTIME_GetReadAccount(ReadAccountResultHandle readAccountResultHandle, AccountHandle account);
ErrorHandle MSALRUNTIME_GetReadAccountError(ReadAccountResultHandle readAccountResultHandle, ErrorHandle errorHandle);
// MSALRuntimeSignoutResult.h
ErrorHandle MSALRUNTIME_ReleaseSignOutResult(long signOutResultHandle);
ErrorHandle MSALRUNTIME_GetSignOutError(SignOutResultHandle signOutResultHandle, ErrorHandle errorHandle);
// MSALRuntimeLogging.h
ErrorHandle MSALRUNTIME_RegisterLogCallback(
Callbacks.LogCallback callback,
Integer callbackdata,
LogCallbackHandle logCallbackHandle);
ErrorHandle MSALRUNTIME_ReleaseLogCallbackHandle(long logCallbackHandle);
void MSALRUNTIME_SetIsPiiEnabled(int enabled); // 1 = PII enabled, anything else = disabled
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy