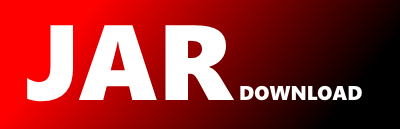
com.microsoft.aad.msal4j.ClientCredentialParameters Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of msal4j Show documentation
Show all versions of msal4j Show documentation
Microsoft Authentication Library for Java gives you the ability to obtain tokens from Azure AD v2 (work and school
accounts, MSA) and Azure AD B2C, gaining access to Microsoft Cloud API and any other API secured by Microsoft
identities
The newest version!
// Generated by delombok at Sat Dec 21 00:20:57 UTC 2024
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
package com.microsoft.aad.msal4j;
import lombok.*;
import java.util.Map;
import java.util.Set;
import static com.microsoft.aad.msal4j.ParameterValidationUtils.validateNotNull;
/**
* Object containing parameters for client credential flow. Can be used as parameter to
* {@link ConfidentialClientApplication#acquireToken(ClientCredentialParameters)}
*/
public class ClientCredentialParameters implements IAcquireTokenParameters {
/**
* Scopes for which the application is requesting access to.
*/
@NonNull
private Set scopes;
/**
* Indicates whether the request should skip looking into the token cache. Be default it is
* set to false.
*/
private Boolean skipCache;
/**
* Claims to be requested through the OIDC claims request parameter, allowing requests for standard and custom claims
*/
private ClaimsRequest claims;
/**
* Adds additional headers to the token request
*/
private Map extraHttpHeaders;
/**
* Adds additional query parameters to the token request
*/
private Map extraQueryParameters;
/**
* Overrides the tenant value in the authority URL for this request
*/
private String tenant;
/**
* Overrides the client credentials for this request
*/
private IClientCredential clientCredential;
private static ClientCredentialParametersBuilder builder() {
return new ClientCredentialParametersBuilder();
}
/**
* Builder for {@link ClientCredentialParameters}
*
* @param scopes scopes application is requesting access to
* @return builder that can be used to construct ClientCredentialParameters
*/
public static ClientCredentialParametersBuilder builder(Set scopes) {
validateNotNull("scopes", scopes);
return builder().scopes(scopes);
}
@java.lang.SuppressWarnings("all")
private static Boolean $default$skipCache() {
return false;
}
@java.lang.SuppressWarnings("all")
public static class ClientCredentialParametersBuilder {
@java.lang.SuppressWarnings("all")
private Set scopes;
@java.lang.SuppressWarnings("all")
private boolean skipCache$set;
@java.lang.SuppressWarnings("all")
private Boolean skipCache;
@java.lang.SuppressWarnings("all")
private ClaimsRequest claims;
@java.lang.SuppressWarnings("all")
private Map extraHttpHeaders;
@java.lang.SuppressWarnings("all")
private Map extraQueryParameters;
@java.lang.SuppressWarnings("all")
private String tenant;
@java.lang.SuppressWarnings("all")
private IClientCredential clientCredential;
@java.lang.SuppressWarnings("all")
ClientCredentialParametersBuilder() {
}
@java.lang.SuppressWarnings("all")
public ClientCredentialParametersBuilder scopes(final Set scopes) {
this.scopes = scopes;
return this;
}
@java.lang.SuppressWarnings("all")
public ClientCredentialParametersBuilder skipCache(final Boolean skipCache) {
this.skipCache = skipCache;
skipCache$set = true;
return this;
}
@java.lang.SuppressWarnings("all")
public ClientCredentialParametersBuilder claims(final ClaimsRequest claims) {
this.claims = claims;
return this;
}
@java.lang.SuppressWarnings("all")
public ClientCredentialParametersBuilder extraHttpHeaders(final Map extraHttpHeaders) {
this.extraHttpHeaders = extraHttpHeaders;
return this;
}
@java.lang.SuppressWarnings("all")
public ClientCredentialParametersBuilder extraQueryParameters(final Map extraQueryParameters) {
this.extraQueryParameters = extraQueryParameters;
return this;
}
@java.lang.SuppressWarnings("all")
public ClientCredentialParametersBuilder tenant(final String tenant) {
this.tenant = tenant;
return this;
}
@java.lang.SuppressWarnings("all")
public ClientCredentialParametersBuilder clientCredential(final IClientCredential clientCredential) {
this.clientCredential = clientCredential;
return this;
}
@java.lang.SuppressWarnings("all")
public ClientCredentialParameters build() {
Boolean skipCache = this.skipCache;
if (!skipCache$set) skipCache = ClientCredentialParameters.$default$skipCache();
return new ClientCredentialParameters(scopes, skipCache, claims, extraHttpHeaders, extraQueryParameters, tenant, clientCredential);
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public java.lang.String toString() {
return "ClientCredentialParameters.ClientCredentialParametersBuilder(scopes=" + this.scopes + ", skipCache=" + this.skipCache + ", claims=" + this.claims + ", extraHttpHeaders=" + this.extraHttpHeaders + ", extraQueryParameters=" + this.extraQueryParameters + ", tenant=" + this.tenant + ", clientCredential=" + this.clientCredential + ")";
}
}
/**
* Scopes for which the application is requesting access to.
*/
@NonNull
@java.lang.SuppressWarnings("all")
public Set scopes() {
return this.scopes;
}
/**
* Indicates whether the request should skip looking into the token cache. Be default it is
* set to false.
*/
@java.lang.SuppressWarnings("all")
public Boolean skipCache() {
return this.skipCache;
}
/**
* Claims to be requested through the OIDC claims request parameter, allowing requests for standard and custom claims
*/
@java.lang.SuppressWarnings("all")
public ClaimsRequest claims() {
return this.claims;
}
/**
* Adds additional headers to the token request
*/
@java.lang.SuppressWarnings("all")
public Map extraHttpHeaders() {
return this.extraHttpHeaders;
}
/**
* Adds additional query parameters to the token request
*/
@java.lang.SuppressWarnings("all")
public Map extraQueryParameters() {
return this.extraQueryParameters;
}
/**
* Overrides the tenant value in the authority URL for this request
*/
@java.lang.SuppressWarnings("all")
public String tenant() {
return this.tenant;
}
/**
* Overrides the client credentials for this request
*/
@java.lang.SuppressWarnings("all")
public IClientCredential clientCredential() {
return this.clientCredential;
}
@java.lang.SuppressWarnings("all")
private ClientCredentialParameters(@NonNull final Set scopes, final Boolean skipCache, final ClaimsRequest claims, final Map extraHttpHeaders, final Map extraQueryParameters, final String tenant, final IClientCredential clientCredential) {
if (scopes == null) {
throw new java.lang.NullPointerException("scopes is marked @NonNull but is null");
}
this.scopes = scopes;
this.skipCache = skipCache;
this.claims = claims;
this.extraHttpHeaders = extraHttpHeaders;
this.extraQueryParameters = extraQueryParameters;
this.tenant = tenant;
this.clientCredential = clientCredential;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy