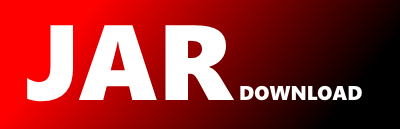
com.microsoft.bingads.internal.OperationStatusRetry Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of microsoft.bingads Show documentation
Show all versions of microsoft.bingads Show documentation
The Bing Ads Java SDK is a library improving developer experience when working with the Bing Ads services by providing high-level access to features such as Bulk API, OAuth Authorization and SOAP API.
package com.microsoft.bingads.internal;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.Executors;
import java.util.concurrent.Future;
import java.util.concurrent.ScheduledExecutorService;
import java.util.concurrent.TimeUnit;
import com.microsoft.bingads.AsyncCallback;
import com.microsoft.bingads.ServiceClient;
import com.microsoft.bingads.internal.functionalinterfaces.Consumer;
import com.microsoft.bingads.internal.functionalinterfaces.TriConsumer;
public class OperationStatusRetry {
private final int INTERVAL_OF_RETRY = 1000; // TimeUnit Milliseconds
public void executeWithRetry(
final TriConsumer, AsyncCallback> action,
final TOperationStatusProvider statusProvider, final ServiceClient serviceClient,
final Consumer statusConsumer, final Consumer exceptionConsumer,
final int maxRetryCount) {
action.accept(statusProvider, serviceClient, new AsyncCallback() {
@Override
public void onCompleted(Future result) {
try {
statusConsumer.accept(result.get());
} catch (InterruptedException exception) {
if (maxRetryCount > 0) {
retry(action, statusProvider, serviceClient, statusConsumer,exceptionConsumer, maxRetryCount - 1);
} else {
exceptionConsumer.accept(exception);
}
} catch (ExecutionException exception) {
if (maxRetryCount > 0) {
retry(action, statusProvider, serviceClient, statusConsumer,exceptionConsumer, maxRetryCount - 1);
} else {
exceptionConsumer.accept(exception);
}
}
}
});
}
private void retry(final TriConsumer, AsyncCallback> action,
final TOperationStatusProvider statusProvider, final ServiceClient serviceClient,
final Consumer statusConsumer, final Consumer exceptionConsumer,
final int maxRetryCount) {
ScheduledExecutorService executor = Executors.newSingleThreadScheduledExecutor();
executor.schedule(new Runnable() {
@Override
public void run() {
executeWithRetry(action, statusProvider, serviceClient, statusConsumer,
exceptionConsumer, maxRetryCount - 1);
}
}, INTERVAL_OF_RETRY, TimeUnit.MILLISECONDS);
}
}