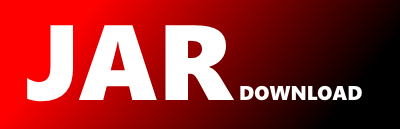
com.microsoft.bingads.v10.bulk.entities.BulkAdGroupNegativeLocationTargetBid Maven / Gradle / Ivy
Show all versions of microsoft.bingads Show documentation
package com.microsoft.bingads.v10.bulk.entities;
import com.microsoft.bingads.v10.bulk.BulkServiceManager;
import com.microsoft.bingads.v10.campaignmanagement.CityTargetBid;
import com.microsoft.bingads.v10.bulk.BulkFileReader;
import com.microsoft.bingads.v10.bulk.BulkFileWriter;
import com.microsoft.bingads.v10.bulk.BulkOperation;
/**
* Represents one sub negative location target bid within a negative location target that is associated with an ad group.
*
* This class exposes properties that can be read and written as fields of the Ad Group Negative Location Target record in a bulk file.
*
*
* For more information, see Ad Group Negative Location Target at
* http://go.microsoft.com/fwlink/?LinkID=620256.
*
*
*
* Each negative location sub type contains a list of bids.
* For example {@link BulkLocationTargetWithStringLocation#getCityTarget} contains a list of {@link CityTargetBid}.
* Each {@link CityTargetBid} instance corresponds to one Ad Group Negative Location Target record in the bulk file.
* If you upload a {@link BulkLocationTargetWithStringLocation#getCityTarget},
* then you are effectively replacing any existing city bids for the corresponding negative location target
*
*
*
* The {@link BulkLocationTargetBidWithStringLocation#getLocationType} property determines the geographical location sub type.
*
*
* @see BulkServiceManager
* @see BulkOperation
* @see BulkFileReader
* @see BulkFileWriter
*/
public class BulkAdGroupNegativeLocationTargetBid extends BulkNegativeLocationTargetBid {
/**
* Initializes a new instanced of the BulkAdGroupNegativeLocationTargetBid class.
*/
public BulkAdGroupNegativeLocationTargetBid() {
super(new BulkAdGroupTargetIdentifier(BulkAdGroupNegativeLocationTargetBid.class));
}
/**
* Gets the identifier of the ad group that the target is associated.
*
*
* Corresponds to the 'Parent Id' field in the bulk file.
*
*/
public Long getAdGroupId() {
return getEntityId();
}
/**
* Sets the identifier of the ad group that the target is associated.
*
*
* Corresponds to the 'Parent Id' field in the bulk file.
*
*/
public void setAdGroupId(Long adGroupId) {
setEntityId(adGroupId);
}
/**
* Gets the name of the ad group that the target is associated.
*
*
* Corresponds to the 'Ad Group' field in the bulk file.
*
*/
public String getAdGroupName() {
return getEntityName();
}
/**
* Sets the name of the ad group that the target is associated.
*
*
* Corresponds to the 'Ad Group' field in the bulk file.
*
*/
public void setAdGroupName(String adGroupName) {
setEntityName(adGroupName);
}
/**
* Gets the name of the campaign that target is associated.
*
*
* Corresponds to the 'Campaign' field in the bulk file.
*
*/
public String getCampaignName() {
return getParentEntityName();
}
/**
* Sets the name of the campaign that target is associated.
*
*
* Corresponds to the 'Campaign' field in the bulk file.
*
*/
public void setCampaignName(String campaignName) {
setParentEntityName(campaignName);
}
}