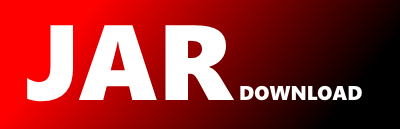
com.microsoft.bingads.v10.bulk.entities.BulkAdGroupTarget Maven / Gradle / Ivy
Show all versions of microsoft.bingads Show documentation
package com.microsoft.bingads.v10.bulk.entities;
import com.microsoft.bingads.v10.bulk.BulkServiceManager;
import com.microsoft.bingads.v10.campaignmanagement.Target;
import com.microsoft.bingads.v10.bulk.BulkFileReader;
import com.microsoft.bingads.v10.bulk.BulkFileWriter;
import com.microsoft.bingads.v10.bulk.BulkOperation;
/**
* Represents a target that is associated with an ad group.
*
* The target contains one or more sub targets, including
* age, gender, day and time, device OS, and location.
* Each target can be read or written in a bulk file.
*
*
* When requesting downloaded entities of type {@link BulkAdGroupTarget}, the results will include
* Ad Group Age Target, Ad Group DayTime Target, Ad Group DeviceOS Target, Ad Group Gender Target, Ad Group Location Target,
* Ad Group Negative Location Target, and Ad Group Radius Target records.
* For more information, see Bulk File Schema at
* http://go.microsoft.com/fwlink/?LinkID=620269.
*
*
*
* For upload you must set the {@link Target} object,
* which will effectively replace any existing bids for the corresponding target.
*
*
* @see BulkServiceManager
* @see BulkOperation
* @see BulkFileReader
* @see BulkFileWriter
*/
public class BulkAdGroupTarget extends BulkTarget{
/**
* Initializes a new instanced of the BulkAdGroupTarget class.
*/
public BulkAdGroupTarget() {
super(new BulkAdGroupAgeTarget(), BulkAdGroupAgeTargetBid.class,
new BulkAdGroupDayTimeTarget(), BulkAdGroupDayTimeTargetBid.class,
new BulkAdGroupDeviceOsTarget(), BulkAdGroupDeviceOsTargetBid.class,
new BulkAdGroupGenderTarget(), BulkAdGroupGenderTargetBid.class,
new BulkAdGroupLocationTarget(), BulkAdGroupLocationTargetBid.class,
new BulkAdGroupNegativeLocationTarget(), BulkAdGroupNegativeLocationTargetBid.class,
new BulkAdGroupRadiusTarget(), BulkAdGroupRadiusTargetBid.class
);
}
/**
* Initializes a new instanced of the BulkAdGroupTarget class with the specified bulk target bid.
*/
BulkAdGroupTarget(BulkTargetBid bid) {
super(bid,
new BulkAdGroupAgeTarget(), BulkAdGroupAgeTargetBid.class,
new BulkAdGroupDayTimeTarget(), BulkAdGroupDayTimeTargetBid.class,
new BulkAdGroupDeviceOsTarget(), BulkAdGroupDeviceOsTargetBid.class,
new BulkAdGroupGenderTarget(), BulkAdGroupGenderTargetBid.class,
new BulkAdGroupLocationTarget(), BulkAdGroupLocationTargetBid.class,
new BulkAdGroupNegativeLocationTarget(), BulkAdGroupNegativeLocationTargetBid.class,
new BulkAdGroupRadiusTarget(), BulkAdGroupRadiusTargetBid.class,
BulkAdGroupTargetIdentifier.class
);
}
/**
* Reserved for internal use.
*/
BulkAdGroupTarget(BulkAdGroupTargetIdentifier identifier) {
super(identifier,
new BulkAdGroupAgeTarget(), BulkAdGroupAgeTargetBid.class,
new BulkAdGroupDayTimeTarget(), BulkAdGroupDayTimeTargetBid.class,
new BulkAdGroupDeviceOsTarget(), BulkAdGroupDeviceOsTargetBid.class,
new BulkAdGroupGenderTarget(), BulkAdGroupGenderTargetBid.class,
new BulkAdGroupLocationTarget(), BulkAdGroupLocationTargetBid.class,
new BulkAdGroupNegativeLocationTarget(), BulkAdGroupNegativeLocationTargetBid.class,
new BulkAdGroupRadiusTarget(), BulkAdGroupRadiusTargetBid.class,
BulkAdGroupTargetIdentifier.class
);
}
/**
* Gets the identifier of the ad group that the target is associated.
*
*
* Corresponds to the 'Parent Id' field in the bulk file.
*
*/
public Long getAdGroupId() {
return getEntityId();
}
/**
* Sets the identifier of the ad group that the target is associated.
*
*
* Corresponds to the 'Parent Id' field in the bulk file.
*
*/
public void setAdGroupId(Long campaignId) {
setEntityId(campaignId);
}
/**
* Gets the name of the ad group that the target is associated.
*
*
* Corresponds to the 'Ad Group' field in the bulk file.
*
*/
public String getAdGroupName() {
return getEntityName();
}
/**
* Sets the name of the ad group that the target is associated.
*
*
* Corresponds to the 'Ad Group' field in the bulk file.
*
*/
public void setAdGroupName(String adGroupName) {
setEntityName(adGroupName);
}
/**
* Gets the name of the campaign that target is associated.
*
*
* Corresponds to the 'Campaign' field in the bulk file.
*
*/
public String getCampaignName() {
return getParentEntityName();
}
/**
* Sets the name of thecampaign that target is associated.
*
*
* Corresponds to the 'Campaign' field in the bulk file.
*
*/
public void setCampaignName(String campaignName) {
setParentEntityName(campaignName);
}
/**
* Reserved for internal use.
*/
@Override
BulkAdGroupTargetIdentifier createIdentifier(Class bidType) {
return new BulkAdGroupTargetIdentifier(bidType);
}
}