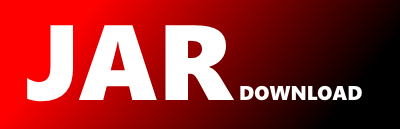
com.microsoft.bingads.v10.bulk.entities.BulkCampaignAgeTarget Maven / Gradle / Ivy
Show all versions of microsoft.bingads Show documentation
package com.microsoft.bingads.v10.bulk.entities;
import com.microsoft.bingads.v10.bulk.BulkServiceManager;
import com.microsoft.bingads.v10.bulk.BulkFileReader;
import com.microsoft.bingads.v10.bulk.BulkFileWriter;
import com.microsoft.bingads.v10.bulk.BulkOperation;
/**
* Represents an age target that is associated with a campaign.
*
* This class exposes the {@link BulkAgeTarget#setAgeTarget} and {@link BulkAgeTarget#getAgeTarget} methods
* that can be used to read and write fields of the Campaign Age Target record in a bulk file.
*
*
* One {@link BulkCampaignAgeTarget} exposes a read only list of {@link BulkCampaignAgeTargetBid}.
* Each {@link BulkCampaignAgeTargetBid} instance corresponds to one Campaign Age Target record in the bulk file.
* If you upload a {@link BulkCampaignAgeTarget},
* then you are effectively replacing any existing bids for the corresponding age target.
*
*
*
* For more information, see Campaign Age Target at
* http://go.microsoft.com/fwlink/?LinkID=620248.
*
*
* @see BulkServiceManager
* @see BulkOperation
* @see BulkFileReader
* @see BulkFileWriter
*/
public class BulkCampaignAgeTarget extends BulkAgeTarget {
/**
* Initializes a new instanced of the BulkCampaignAgeTarget class.
*/
public BulkCampaignAgeTarget() {
super(BulkCampaignAgeTargetBid.class);
}
/**
* Gets the identifier of the campaign that the target is associated.
*
*
* Corresponds to the 'Parent Id' field in the bulk file.
*
*/
public Long getCampaignId() {
return getEntityId();
}
/**
* Sets the identifier of the campaign that the target is associated.
*
*
* Corresponds to the 'Parent Id' field in the bulk file.
*
*/
public void setCampaignId(Long campaignId) {
setEntityId(campaignId);
}
/**
* Gets the name of the campaign that target is associated.
*
*
* Corresponds to the 'Campaign' field in the bulk file.
*
*/
public String getCampaignName() {
return getEntityName();
}
/**
* Sets the name of the campaign that target is associated.
*
*
* Corresponds to the 'Campaign' field in the bulk file.
*
*/
public void setCampaignName(String campaignName) {
setEntityName(campaignName);
}
/**
* Reserved for internal use.
*/
@Override
BulkCampaignAgeTargetBid createBid() {
return new BulkCampaignAgeTargetBid();
}
}