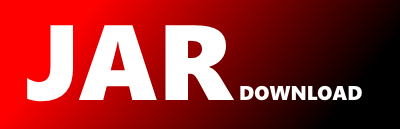
com.microsoft.bingads.v10.bulk.entities.BulkLocationTarget Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of microsoft.bingads Show documentation
Show all versions of microsoft.bingads Show documentation
The Bing Ads Java SDK is a library improving developer experience when working with the Bing Ads services by providing high-level access to features such as Bulk API, OAuth Authorization and SOAP API.
package com.microsoft.bingads.v10.bulk.entities;
import java.util.List;
import com.microsoft.bingads.v10.campaignmanagement.CityTargetBid;
import com.microsoft.bingads.v10.campaignmanagement.CountryTargetBid;
import com.microsoft.bingads.v10.campaignmanagement.MetroAreaTargetBid;
import com.microsoft.bingads.v10.campaignmanagement.PostalCodeTargetBid;
import com.microsoft.bingads.v10.campaignmanagement.StateTargetBid;
/**
* A base class for all bulk location target classes.
*
* @param see {@link BulkLocationTargetBid}
*/
abstract class BulkLocationTarget extends BulkLocationTargetWithStringLocation {
public BulkLocationTarget(Class classOfTBid) {
super(classOfTBid);
}
@Override
boolean shouldConvertPostalCodeTargetBid(PostalCodeTargetBid bid) {
return !bid.isIsExcluded();
}
@Override
void setPostalCodeBidAdditionialProperties(PostalCodeTargetBid postalCodeBid, TBid t) {
postalCodeBid.setBidAdjustment(t.getBidAdjustment());
}
@Override
void setBulkPostalCodeBidAdditionalProperties(TBid bulkBid, PostalCodeTargetBid postalCodeTargetBid) {
bulkBid.setBidAdjustment(postalCodeTargetBid.getBidAdjustment());
}
@Override
boolean shouldConvertCityTargetBid(CityTargetBid bid) {
return !bid.isIsExcluded();
}
@Override
void setCityBidAdditionialProperties(CityTargetBid cityBid, TBid t) {
cityBid.setBidAdjustment(t.getBidAdjustment());
}
@Override
void setBulkCityBidAdditionalProperties(TBid bulkBid, CityTargetBid cityTargetBid) {
bulkBid.setBidAdjustment(cityTargetBid.getBidAdjustment());
}
@Override
boolean shouldConvertMetroAreaTargetBid(MetroAreaTargetBid bid) {
return !bid.isIsExcluded();
}
@Override
void setMetroAreaBidAdditionialProperties(MetroAreaTargetBid metroAreaBid, TBid t) {
metroAreaBid.setBidAdjustment(t.getBidAdjustment());
}
@Override
void setBulkMetroAreaBidAdditionalProperties(TBid bulkBid, MetroAreaTargetBid metroAreaTargetBid) {
bulkBid.setBidAdjustment(metroAreaTargetBid.getBidAdjustment());
}
@Override
boolean shouldConvertStateTargetBid(StateTargetBid bid) {
return !bid.isIsExcluded();
}
@Override
void setStateBidAdditionialProperties(StateTargetBid cityBid, TBid t) {
cityBid.setBidAdjustment(t.getBidAdjustment());
}
@Override
void setBulkStateBidAdditionalProperties(TBid bulkBid, StateTargetBid stateTargetBid) {
bulkBid.setBidAdjustment(stateTargetBid.getBidAdjustment());
}
@Override
boolean shouldConvertCountryTargetBid(CountryTargetBid bid) {
return !bid.isIsExcluded();
}
@Override
void setCountryBidAdditionialProperties(CountryTargetBid cityBid, TBid t) {
cityBid.setBidAdjustment(t.getBidAdjustment());
}
@Override
void setBulkCountryBidAdditionalProperties(TBid bulkBid, CountryTargetBid countryTargetBid) {
bulkBid.setBidAdjustment(countryTargetBid.getBidAdjustment());
}
/**
* Reserved for internal use.
*/
@Override
List convertApiToBulkBids() {
List bulkBids = super.convertApiToBulkBids();
for (TBid bid : bulkBids) {
bid.setIntentOption(getLocation().getIntentOption());
}
return bulkBids;
}
/**
* Reserved for internal use.
*/
@Override
void reconstructSubTargets() {
super.reconstructSubTargets();
if (!getBids().isEmpty()) {
getLocation().setIntentOption(getBids().get(0).getIntentOption());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy