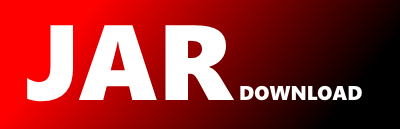
com.microsoft.bingads.v10.bulk.entities.BulkSiteLink Maven / Gradle / Ivy
Show all versions of microsoft.bingads Show documentation
package com.microsoft.bingads.v10.bulk.entities;
import com.microsoft.bingads.v10.bulk.BulkServiceManager;
import com.microsoft.bingads.v10.campaignmanagement.AdExtensionStatus;
import com.microsoft.bingads.v10.campaignmanagement.ArrayOfstring;
import com.microsoft.bingads.v10.campaignmanagement.Schedule;
import com.microsoft.bingads.v10.campaignmanagement.SiteLink;
import com.microsoft.bingads.v10.campaignmanagement.SiteLinksAdExtension;
import com.microsoft.bingads.v10.bulk.BulkFileReader;
import com.microsoft.bingads.v10.bulk.BulkFileWriter;
import com.microsoft.bingads.v10.bulk.BulkOperation;
import com.microsoft.bingads.v10.internal.bulk.StringExtensions;
import com.microsoft.bingads.v10.internal.bulk.StringTable;
import com.microsoft.bingads.v10.internal.bulk.BulkMapping;
import com.microsoft.bingads.v10.internal.bulk.MappingHelpers;
import com.microsoft.bingads.v10.internal.bulk.RowValues;
import com.microsoft.bingads.v10.internal.bulk.SimpleBulkMapping;
import com.microsoft.bingads.v10.internal.bulk.entities.MultiRecordBulkEntity;
import com.microsoft.bingads.v10.internal.bulk.entities.SingleRecordBulkEntity;
import com.microsoft.bingads.v10.internal.bulk.entities.SiteLinkAdExtensionIdentifier;
import com.microsoft.bingads.internal.functionalinterfaces.BiConsumer;
import com.microsoft.bingads.internal.functionalinterfaces.Function;
import java.text.ParseException;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
/**
* Represents a sitelink.
*
* This class exposes the {@link #setSiteLink} and {@link #getSiteLink} methods that can be used to
* read and write fields of the Sitelink Ad Extension record in a bulk file.
*
*
* For more information, see Sitelink Ad Extension at
* http://go.microsoft.com/fwlink/?LinkID=620236
*
*
*
* The Sitelink Ad Extension record includes the distinct properties of the {@link BulkSiteLink} class,
* combined with the commmon properties of the {@link BulkSiteLinkAdExtension} class,
* for example {@link BulkSiteLinkAdExtension#getAccountId} and
* {@link BulkSiteLinkAdExtension#getSiteLinksAdExtension}.
*
*
*
* One {@link BulkSiteLinkAdExtension} has one or more {@link BulkSiteLink}.
* Each {@link BulkSiteLink} instance corresponds to one Sitelink Ad Extension record in the bulk file.
* If you upload a {@link BulkSiteLinkAdExtension},
* then you are effectively replacing any existing site links for the sitelink ad extension.
*
*
* @see BulkServiceManager
* @see BulkOperation
* @see BulkFileReader
* @see BulkFileWriter
*/
public class BulkSiteLink extends SingleRecordBulkEntity {
private SiteLinkAdExtensionIdentifier identifier;
SiteLinkAdExtensionIdentifier getIdentifier() {
return identifier;
}
void setIdentifier(SiteLinkAdExtensionIdentifier identifier) {
this.identifier = identifier;
}
/**
* Gets the identifier of the ad extension.
*
*
* Corresponds to the 'Id' field in the bulk file.
*
*/
public Long getAdExtensionId() {
return this.identifier.getAdExtensionId();
}
/**
* Sets the identifier of the ad extension.
*
*
* Corresponds to the 'Id' field in the bulk file.
*
*/
public void setAdExtensionId(Long adExtensionId) {
this.identifier.setAdExtensionId(adExtensionId);
}
/**
* Gets the ad extension's parent account identifier.
*
*
* Corresponds to the 'Parent Id' field in the bulk file.
*
*/
public Long getAccountId() {
return this.identifier.getAccountId();
}
/**
* Sets the ad extension's parent account identifier.
*
*
* Corresponds to the 'Parent Id' field in the bulk file.
*
*/
public void setAccountId(Long accountId) {
this.identifier.setAccountId(accountId);
}
/**
* Gets the status of the ad extension.
*
*
* Corresponds to the 'Status' field in the bulk file.
*
*/
public AdExtensionStatus getStatus() {
return this.identifier.getStatus();
}
/**
* Sets the status of the ad extension.
*
*
* Corresponds to the 'Status' field in the bulk file.
*
*/
public void setStatus(AdExtensionStatus status) {
this.identifier.setStatus(status);
}
/**
* Gets the version of the ad extension.
*
*
* Corresponds to the 'Version' field in the bulk file.
*
*/
public Integer getVersion() {
return this.identifier.getVersion();
}
/**
* Sets the version of the ad extension.
*
*
* Corresponds to the 'Version' field in the bulk file.
*
*/
public void setVersion(Integer version) {
this.identifier.setVersion(version);
}
private int order;
private SiteLink siteLink;
/**
* Gets the order of the sitelink displayed to a search user in the ad.
*
*
* Corresponds to the 'Sitelink Extension Order' field in the bulk file.
*
*/
public int getOrder() {
return order;
}
/**
* Gets the order of the sitelink displayed to a search user in the ad.
*
*
* Corresponds to the 'Sitelink Extension Order' field in the bulk file.
*
*/
public void setOrder(int order) {
this.order = order;
}
/**
* Gets the sitelink.
*/
public SiteLink getSiteLink() {
return siteLink;
}
/**
* Sets the sitelink.
*/
public void setSiteLink(SiteLink siteLink) {
this.siteLink = siteLink;
}
/**
* Initializes a new instance of the BulkSiteLink class.
*/
public BulkSiteLink() {
this.identifier = new SiteLinkAdExtensionIdentifier();
}
private static final List> MAPPINGS;
static {
List> m = new ArrayList>();
m.add(new SimpleBulkMapping(StringTable.SiteLinkExtensionOrder,
new Function() {
@Override
public Integer apply(BulkSiteLink c) {
return c.getOrder();
}
},
new BiConsumer() {
@Override
public void accept(String v, BulkSiteLink c) {
c.setOrder(StringExtensions.parseInt(v));
}
}
));
m.add(new SimpleBulkMapping(StringTable.SiteLinkDisplayText,
new Function() {
@Override
public String apply(BulkSiteLink c) {
return c.getSiteLink().getDisplayText();
}
},
new BiConsumer() {
@Override
public void accept(String v, BulkSiteLink c) {
c.getSiteLink().setDisplayText(v);
}
}
));
m.add(new SimpleBulkMapping(StringTable.SiteLinkDestinationUrl,
new Function() {
@Override
public String apply(BulkSiteLink c) {
return StringExtensions.toOptionalBulkString(c.getSiteLink().getDestinationUrl());
}
},
new BiConsumer() {
@Override
public void accept(String v, BulkSiteLink c) {
c.getSiteLink().setDestinationUrl(StringExtensions.getValueOrEmptyString(v));
}
},
true
));
m.add(new SimpleBulkMapping(StringTable.SiteLinkDescription1,
new Function() {
@Override
public String apply(BulkSiteLink c) {
return c.getSiteLink().getDescription1();
}
},
new BiConsumer() {
@Override
public void accept(String v, BulkSiteLink c) {
c.getSiteLink().setDescription1(v);
}
}
));
m.add(new SimpleBulkMapping(StringTable.SiteLinkDescription2,
new Function() {
@Override
public String apply(BulkSiteLink c) {
return c.getSiteLink().getDescription2();
}
},
new BiConsumer() {
@Override
public void accept(String v, BulkSiteLink c) {
c.getSiteLink().setDescription2(v);
}
}
));
m.add(new SimpleBulkMapping(StringTable.DevicePreference,
new Function() {
@Override
public String apply(BulkSiteLink c) {
return StringExtensions.toDevicePreferenceBulkString(c.getSiteLink().getDevicePreference());
}
},
new BiConsumer() {
@Override
public void accept(String v, BulkSiteLink c) {
c.getSiteLink().setDevicePreference(StringExtensions.parseDevicePreference(v));
}
}
));
m.add(new SimpleBulkMapping(StringTable.FinalUrl,
new Function() {
@Override
public String apply(BulkSiteLink c) {
return StringExtensions.writeUrls("; ", c.getSiteLink().getFinalUrls());
}
},
new BiConsumer() {
@Override
public void accept(String v, BulkSiteLink c) {
ArrayOfstring urls = new ArrayOfstring();
List urlArray = StringExtensions.parseUrls(v);
if(urlArray == null) {
urls = null;
} else {
urls.getStrings().addAll(urlArray);
}
c.getSiteLink().setFinalUrls(urls);
}
}
));
m.add(new SimpleBulkMapping(StringTable.FinalMobileUrl,
new Function() {
@Override
public String apply(BulkSiteLink c) {
return StringExtensions.writeUrls("; ", c.getSiteLink().getFinalMobileUrls());
}
},
new BiConsumer() {
@Override
public void accept(String v, BulkSiteLink c) {
ArrayOfstring urls = new ArrayOfstring();
List urlArray = StringExtensions.parseUrls(v);
if(urlArray == null) {
urls = null;
} else {
urls.getStrings().addAll(urlArray);
}
c.getSiteLink().setFinalMobileUrls(urls);
}
}
));
m.add(new SimpleBulkMapping(StringTable.TrackingTemplate,
new Function() {
@Override
public String apply(BulkSiteLink c) {
return StringExtensions.toOptionalBulkString(c.getSiteLink().getTrackingUrlTemplate());
}
},
new BiConsumer() {
@Override
public void accept(String v, BulkSiteLink c) {
c.getSiteLink().setTrackingUrlTemplate(StringExtensions.getValueOrEmptyString(v));
}
}
));
m.add(new SimpleBulkMapping(StringTable.CustomParameter,
new Function() {
@Override
public String apply(BulkSiteLink c) {
return StringExtensions.toCustomParaBulkString(c.getSiteLink().getUrlCustomParameters());
}
},
new BiConsumer() {
@Override
public void accept(String v, BulkSiteLink c) {
try {
c.getSiteLink().setUrlCustomParameters(StringExtensions.parseCustomParameters(v));
} catch (Exception e) {
e.printStackTrace();
}
}
}
));
m.add(new SimpleBulkMapping(StringTable.AdSchedule,
new Function() {
@Override
public String apply(BulkSiteLink t) {
if (t.getSiteLink().getScheduling() == null) {
return null;
}
return StringExtensions.toDayTimeRangesBulkString(t.getSiteLink().getScheduling().getDayTimeRanges());
}
},
new BiConsumer() {
@Override
public void accept(String v, BulkSiteLink c) {
if (c.getSiteLink().getScheduling() == null) {
c.getSiteLink().setScheduling(new Schedule());
}
c.getSiteLink().getScheduling().setDayTimeRanges(StringExtensions.parseDayTimeRanges(v));
}
}
));
m.add(new SimpleBulkMapping(StringTable.StartDate,
new Function() {
@Override
public String apply(BulkSiteLink t) {
if (t.getSiteLink().getScheduling() == null) {
return null;
}
return StringExtensions.toScheduleDateBulkString(t.getSiteLink().getScheduling().getStartDate());
}
},
new BiConsumer() {
@Override
public void accept(String v, BulkSiteLink c) {
if (c.getSiteLink().getScheduling() == null) {
c.getSiteLink().setScheduling(new Schedule());
}
try {
c.getSiteLink().getScheduling().setStartDate(StringExtensions.parseDate(v));
} catch (ParseException e) {
e.printStackTrace();
}
}
}
));
m.add(new SimpleBulkMapping(StringTable.EndDate,
new Function() {
@Override
public String apply(BulkSiteLink t) {
if (t.getSiteLink().getScheduling() == null) {
return null;
}
return StringExtensions.toScheduleDateBulkString(t.getSiteLink().getScheduling().getEndDate());
}
},
new BiConsumer() {
@Override
public void accept(String v, BulkSiteLink c) {
if (c.getSiteLink().getScheduling() == null) {
c.getSiteLink().setScheduling(new Schedule());
}
try {
c.getSiteLink().getScheduling().setEndDate(StringExtensions.parseDate(v));
} catch (ParseException e) {
e.printStackTrace();
}
}
}
));
m.add(new SimpleBulkMapping(StringTable.UseSearcherTimeZone,
new Function() {
@Override
public String apply(BulkSiteLink t) {
if (t.getSiteLink().getScheduling() == null) {
return null;
}
return StringExtensions.toUseSearcherTimeZoneBulkString(t.getSiteLink().getScheduling().getUseSearcherTimeZone());
}
},
new BiConsumer() {
@Override
public void accept(String v, BulkSiteLink c) {
if (c.getSiteLink().getScheduling() == null) {
c.getSiteLink().setScheduling(new Schedule());
}
c.getSiteLink().getScheduling().setUseSearcherTimeZone(StringExtensions.parseUseSearcherTimeZone(v));
}
},
true
));
MAPPINGS = Collections.unmodifiableList(m);
}
@Override
public void processMappingsFromRowValues(RowValues values) {
this.siteLink = new SiteLink();
this.identifier.readFromRowValues(values);
MappingHelpers.convertToEntity(values, MAPPINGS, this);
}
@Override
public void processMappingsToRowValues(RowValues values, boolean excludeReadonlyData) {
this.validatePropertyNotNull(this.siteLink, StringTable.SiteLink);
this.identifier.writeToRowValues(values, excludeReadonlyData);
MappingHelpers.convertToValues(this, values, MAPPINGS);
}
@Override
public boolean canEncloseInMultilineEntity() {
return true;
}
@Override
public MultiRecordBulkEntity encloseInMultilineEntity() {
return new BulkSiteLinkAdExtension(this);
}
}